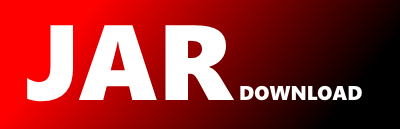
com.google.api.services.dfareporting.model.Creative Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dfareporting.model;
/**
* Contains properties of a Creative.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Campaign Manager 360 API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Creative extends com.google.api.client.json.GenericJson {
/**
* Account ID of this creative. This field, if left unset, will be auto-generated for both insert
* and update operations. Applicable to all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long accountId;
/**
* Whether the creative is active. Applicable to all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean active;
/**
* Ad parameters user for VPAID creative. This is a read-only field. Applicable to the following
* creative types: all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String adParameters;
/**
* Keywords for a Rich Media creative. Keywords let you customize the creative settings of a Rich
* Media ad running on your site without having to contact the advertiser. You can use keywords to
* dynamically change the look or functionality of a creative. Applicable to the following
* creative types: all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List adTagKeys;
/**
* Additional sizes associated with a responsive creative. When inserting or updating a creative
* either the size ID field or size width and height fields can be used. Applicable to DISPLAY
* creatives when the primary asset type is HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List additionalSizes;
/**
* Required. Advertiser ID of this creative. This is a required field. Applicable to all creative
* types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long advertiserId;
/**
* Whether script access is allowed for this creative. This is a read-only and deprecated field
* which will automatically be set to true on update. Applicable to the following creative types:
* FLASH_INPAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allowScriptAccess;
/**
* Whether the creative is archived. Applicable to all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean archived;
/**
* Type of artwork used for the creative. This is a read-only field. Applicable to the following
* creative types: all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String artworkType;
/**
* Source application where creative was authored. Presently, only DBM authored creatives will
* have this field set. Applicable to all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String authoringSource;
/**
* Authoring tool for HTML5 banner creatives. This is a read-only field. Applicable to the
* following creative types: HTML5_BANNER.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String authoringTool;
/**
* Whether images are automatically advanced for image gallery creatives. Applicable to the
* following creative types: DISPLAY_IMAGE_GALLERY.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean autoAdvanceImages;
/**
* The 6-character HTML color code, beginning with #, for the background of the window area where
* the Flash file is displayed. Default is white. Applicable to the following creative types:
* FLASH_INPAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String backgroundColor;
/**
* Click-through URL for backup image. Applicable to ENHANCED_BANNER when the primary asset type
* is not HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CreativeClickThroughUrl backupImageClickThroughUrl;
/**
* List of feature dependencies that will cause a backup image to be served if the browser that
* serves the ad does not support them. Feature dependencies are features that a browser must be
* able to support in order to render your HTML5 creative asset correctly. This field is initially
* auto-generated to contain all features detected by Campaign Manager for all the assets of this
* creative and can then be modified by the client. To reset this field, copy over all the
* creativeAssets' detected features. Applicable to the following creative types: HTML5_BANNER.
* Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List backupImageFeatures;
/**
* Reporting label used for HTML5 banner backup image. Applicable to the following creative types:
* DISPLAY when the primary asset type is not HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String backupImageReportingLabel;
/**
* Target window for backup image. Applicable to the following creative types: FLASH_INPAGE and
* HTML5_BANNER. Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TargetWindow backupImageTargetWindow;
/**
* Click tags of the creative. For DISPLAY, FLASH_INPAGE, and HTML5_BANNER creatives, this is a
* subset of detected click tags for the assets associated with this creative. After creating a
* flash asset, detected click tags will be returned in the creativeAssetMetadata. When inserting
* the creative, populate the creative clickTags field using the creativeAssetMetadata.clickTags
* field. For DISPLAY_IMAGE_GALLERY creatives, there should be exactly one entry in this list for
* each image creative asset. A click tag is matched with a corresponding creative asset by
* matching the clickTag.name field with the creativeAsset.assetIdentifier.name field. Applicable
* to the following creative types: DISPLAY_IMAGE_GALLERY, FLASH_INPAGE, HTML5_BANNER. Applicable
* to DISPLAY when the primary asset type is not HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List clickTags;
static {
// hack to force ProGuard to consider ClickTag used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(ClickTag.class);
}
/**
* Industry standard ID assigned to creative for reach and frequency. Applicable to
* INSTREAM_VIDEO_REDIRECT creatives.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String commercialId;
/**
* List of companion creatives assigned to an in-Stream video creative. Acceptable values include
* IDs of existing flash and image creatives. Applicable to the following creative types: all
* VPAID, all INSTREAM_AUDIO and all INSTREAM_VIDEO with dynamicAssetSelection set to false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.util.List companionCreatives;
/**
* Compatibilities associated with this creative. This is a read-only field. DISPLAY and
* DISPLAY_INTERSTITIAL refer to rendering either on desktop or on mobile devices or in mobile
* apps for regular or interstitial ads, respectively. APP and APP_INTERSTITIAL are for rendering
* in mobile apps. Only pre-existing creatives may have these compatibilities since new creatives
* will either be assigned DISPLAY or DISPLAY_INTERSTITIAL instead. IN_STREAM_VIDEO refers to
* rendering in in-stream video ads developed with the VAST standard. IN_STREAM_AUDIO refers to
* rendering in in-stream audio ads developed with the VAST standard. Applicable to all creative
* types. Acceptable values are: - "APP" - "APP_INTERSTITIAL" - "IN_STREAM_VIDEO" -
* "IN_STREAM_AUDIO" - "DISPLAY" - "DISPLAY_INTERSTITIAL"
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List compatibility;
/**
* Whether Flash assets associated with the creative need to be automatically converted to HTML5.
* This flag is enabled by default and users can choose to disable it if they don't want the
* system to generate and use HTML5 asset for this creative. Applicable to the following creative
* type: FLASH_INPAGE. Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean convertFlashToHtml5;
/**
* List of counter events configured for the creative. For DISPLAY_IMAGE_GALLERY creatives, these
* are read-only and auto-generated from clickTags. Applicable to the following creative types:
* DISPLAY_IMAGE_GALLERY, all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List counterCustomEvents;
/**
* Required if dynamicAssetSelection is true.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CreativeAssetSelection creativeAssetSelection;
/**
* Assets associated with a creative. Applicable to all but the following creative types:
* INTERNAL_REDIRECT, INTERSTITIAL_INTERNAL_REDIRECT, and REDIRECT
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List creativeAssets;
/**
* Creative field assignments for this creative. Applicable to all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List creativeFieldAssignments;
/**
* Custom key-values for a Rich Media creative. Key-values let you customize the creative settings
* of a Rich Media ad running on your site without having to contact the advertiser. You can use
* key-values to dynamically change the look or functionality of a creative. Applicable to the
* following creative types: all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List customKeyValues;
/**
* Set this to true to enable the use of rules to target individual assets in this creative. When
* set to true creativeAssetSelection must be set. This also controls asset-level companions. When
* this is true, companion creatives should be assigned to creative assets. Learn more. Applicable
* to INSTREAM_VIDEO creatives.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean dynamicAssetSelection;
/**
* List of exit events configured for the creative. For DISPLAY and DISPLAY_IMAGE_GALLERY
* creatives, these are read-only and auto-generated from clickTags, For DISPLAY, an event is also
* created from the backupImageReportingLabel. Applicable to the following creative types:
* DISPLAY_IMAGE_GALLERY, all RICH_MEDIA, and all VPAID. Applicable to DISPLAY when the primary
* asset type is not HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List exitCustomEvents;
/**
* OpenWindow FSCommand of this creative. This lets the SWF file communicate with either Flash
* Player or the program hosting Flash Player, such as a web browser. This is only triggered if
* allowScriptAccess field is true. Applicable to the following creative types: FLASH_INPAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FsCommand fsCommand;
/**
* HTML code for the creative. This is a required field when applicable. This field is ignored if
* htmlCodeLocked is true. Applicable to the following creative types: all CUSTOM, FLASH_INPAGE,
* and HTML5_BANNER, and all RICH_MEDIA.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String htmlCode;
/**
* Whether HTML code is generated by Campaign Manager or manually entered. Set to true to ignore
* changes to htmlCode. Applicable to the following creative types: FLASH_INPAGE and HTML5_BANNER.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean htmlCodeLocked;
/**
* ID of this creative. This is a read-only, auto-generated field. Applicable to all creative
* types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long id;
/**
* Dimension value for the ID of this creative. This is a read-only field. Applicable to all
* creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DimensionValue idDimensionValue;
/**
* Identifies what kind of resource this is. Value: the fixed string "dfareporting#creative".
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Creative last modification information. This is a read-only field. Applicable to all creative
* types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LastModifiedInfo lastModifiedInfo;
/**
* Latest Studio trafficked creative ID associated with rich media and VPAID creatives. This is a
* read-only field. Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long latestTraffickedCreativeId;
/**
* Description of the audio or video ad. Applicable to the following creative types: all
* INSTREAM_VIDEO, INSTREAM_AUDIO, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String mediaDescription;
/**
* Creative audio or video duration in seconds. This is a read-only field. Applicable to the
* following creative types: INSTREAM_VIDEO, INSTREAM_AUDIO, all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float mediaDuration;
/**
* Required. Name of the creative. This must be less than 256 characters long. Applicable to all
* creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Online behavioral advertising icon to be added to the creative. Applicable to the following
* creative types: all INSTREAM_VIDEO.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ObaIcon obaIcon;
/**
* Override CSS value for rich media creatives. Applicable to the following creative types: all
* RICH_MEDIA.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String overrideCss;
/**
* Amount of time to play the video before counting a view. Applicable to the following creative
* types: all INSTREAM_VIDEO.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private VideoOffset progressOffset;
/**
* URL of hosted image or hosted video or another ad tag. For INSTREAM_VIDEO_REDIRECT creatives
* this is the in-stream video redirect URL. The standard for a VAST (Video Ad Serving Template)
* ad response allows for a redirect link to another VAST 2.0 or 3.0 call. This is a required
* field when applicable. Applicable to the following creative types: DISPLAY_REDIRECT,
* INTERNAL_REDIRECT, INTERSTITIAL_INTERNAL_REDIRECT, and INSTREAM_VIDEO_REDIRECT
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String redirectUrl;
/**
* ID of current rendering version. This is a read-only field. Applicable to all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long renderingId;
/**
* Dimension value for the rendering ID of this creative. This is a read-only field. Applicable to
* all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DimensionValue renderingIdDimensionValue;
/**
* The minimum required Flash plugin version for this creative. For example, 11.2.202.235. This is
* a read-only field. Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String requiredFlashPluginVersion;
/**
* The internal Flash version for this creative as calculated by Studio. This is a read-only
* field. Applicable to the following creative types: FLASH_INPAGE all RICH_MEDIA, and all VPAID.
* Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer requiredFlashVersion;
/**
* Size associated with this creative. When inserting or updating a creative either the size ID
* field or size width and height fields can be used. This is a required field when applicable;
* however for IMAGE, FLASH_INPAGE creatives, and for DISPLAY creatives with a primary asset of
* type HTML_IMAGE, if left blank, this field will be automatically set using the actual size of
* the associated image assets. Applicable to the following creative types: DISPLAY,
* DISPLAY_IMAGE_GALLERY, FLASH_INPAGE, HTML5_BANNER, IMAGE, and all RICH_MEDIA.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Size size;
/**
* Amount of time to play the video before the skip button appears. Applicable to the following
* creative types: all INSTREAM_VIDEO.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private VideoOffset skipOffset;
/**
* Whether the user can choose to skip the creative. Applicable to the following creative types:
* all INSTREAM_VIDEO and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean skippable;
/**
* Whether the creative is SSL-compliant. This is a read-only field. Applicable to all creative
* types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean sslCompliant;
/**
* Whether creative should be treated as SSL compliant even if the system scan shows it's not.
* Applicable to all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean sslOverride;
/**
* Studio advertiser ID associated with rich media and VPAID creatives. This is a read-only field.
* Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long studioAdvertiserId;
/**
* Studio creative ID associated with rich media and VPAID creatives. This is a read-only field.
* Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long studioCreativeId;
/**
* Studio trafficked creative ID associated with rich media and VPAID creatives. This is a read-
* only field. Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long studioTraffickedCreativeId;
/**
* Subaccount ID of this creative. This field, if left unset, will be auto-generated for both
* insert and update operations. Applicable to all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long subaccountId;
/**
* Third-party URL used to record backup image impressions. Applicable to the following creative
* types: all RICH_MEDIA.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String thirdPartyBackupImageImpressionsUrl;
/**
* Third-party URL used to record rich media impressions. Applicable to the following creative
* types: all RICH_MEDIA.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String thirdPartyRichMediaImpressionsUrl;
/**
* Third-party URLs for tracking in-stream creative events. Applicable to the following creative
* types: all INSTREAM_VIDEO, all INSTREAM_AUDIO, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List thirdPartyUrls;
/**
* List of timer events configured for the creative. For DISPLAY_IMAGE_GALLERY creatives, these
* are read-only and auto-generated from clickTags. Applicable to the following creative types:
* DISPLAY_IMAGE_GALLERY, all RICH_MEDIA, and all VPAID. Applicable to DISPLAY when the primary
* asset is not HTML_IMAGE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List timerCustomEvents;
/**
* Combined size of all creative assets. This is a read-only field. Applicable to the following
* creative types: all RICH_MEDIA, and all VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long totalFileSize;
/**
* Required. Type of this creative. Applicable to all creative types. *Note:* FLASH_INPAGE,
* HTML5_BANNER, and IMAGE are only used for existing creatives. New creatives should use DISPLAY
* as a replacement for these types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* A Universal Ad ID as per the VAST 4.0 spec. Applicable to the following creative types:
* INSTREAM_AUDIO and INSTREAM_VIDEO and VPAID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private UniversalAdId universalAdId;
/**
* The version number helps you keep track of multiple versions of your creative in your reports.
* The version number will always be auto-generated during insert operations to start at 1. For
* tracking creatives the version cannot be incremented and will always remain at 1. For all other
* creative types the version can be incremented only by 1 during update operations. In addition,
* the version will be automatically incremented by 1 when undergoing Rich Media creative merging.
* Applicable to all creative types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer version;
/**
* Account ID of this creative. This field, if left unset, will be auto-generated for both insert
* and update operations. Applicable to all creative types.
* @return value or {@code null} for none
*/
public java.lang.Long getAccountId() {
return accountId;
}
/**
* Account ID of this creative. This field, if left unset, will be auto-generated for both insert
* and update operations. Applicable to all creative types.
* @param accountId accountId or {@code null} for none
*/
public Creative setAccountId(java.lang.Long accountId) {
this.accountId = accountId;
return this;
}
/**
* Whether the creative is active. Applicable to all creative types.
* @return value or {@code null} for none
*/
public java.lang.Boolean getActive() {
return active;
}
/**
* Whether the creative is active. Applicable to all creative types.
* @param active active or {@code null} for none
*/
public Creative setActive(java.lang.Boolean active) {
this.active = active;
return this;
}
/**
* Ad parameters user for VPAID creative. This is a read-only field. Applicable to the following
* creative types: all VPAID.
* @return value or {@code null} for none
*/
public java.lang.String getAdParameters() {
return adParameters;
}
/**
* Ad parameters user for VPAID creative. This is a read-only field. Applicable to the following
* creative types: all VPAID.
* @param adParameters adParameters or {@code null} for none
*/
public Creative setAdParameters(java.lang.String adParameters) {
this.adParameters = adParameters;
return this;
}
/**
* Keywords for a Rich Media creative. Keywords let you customize the creative settings of a Rich
* Media ad running on your site without having to contact the advertiser. You can use keywords to
* dynamically change the look or functionality of a creative. Applicable to the following
* creative types: all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.util.List getAdTagKeys() {
return adTagKeys;
}
/**
* Keywords for a Rich Media creative. Keywords let you customize the creative settings of a Rich
* Media ad running on your site without having to contact the advertiser. You can use keywords to
* dynamically change the look or functionality of a creative. Applicable to the following
* creative types: all RICH_MEDIA, and all VPAID.
* @param adTagKeys adTagKeys or {@code null} for none
*/
public Creative setAdTagKeys(java.util.List adTagKeys) {
this.adTagKeys = adTagKeys;
return this;
}
/**
* Additional sizes associated with a responsive creative. When inserting or updating a creative
* either the size ID field or size width and height fields can be used. Applicable to DISPLAY
* creatives when the primary asset type is HTML_IMAGE.
* @return value or {@code null} for none
*/
public java.util.List getAdditionalSizes() {
return additionalSizes;
}
/**
* Additional sizes associated with a responsive creative. When inserting or updating a creative
* either the size ID field or size width and height fields can be used. Applicable to DISPLAY
* creatives when the primary asset type is HTML_IMAGE.
* @param additionalSizes additionalSizes or {@code null} for none
*/
public Creative setAdditionalSizes(java.util.List additionalSizes) {
this.additionalSizes = additionalSizes;
return this;
}
/**
* Required. Advertiser ID of this creative. This is a required field. Applicable to all creative
* types.
* @return value or {@code null} for none
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/**
* Required. Advertiser ID of this creative. This is a required field. Applicable to all creative
* types.
* @param advertiserId advertiserId or {@code null} for none
*/
public Creative setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
/**
* Whether script access is allowed for this creative. This is a read-only and deprecated field
* which will automatically be set to true on update. Applicable to the following creative types:
* FLASH_INPAGE.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAllowScriptAccess() {
return allowScriptAccess;
}
/**
* Whether script access is allowed for this creative. This is a read-only and deprecated field
* which will automatically be set to true on update. Applicable to the following creative types:
* FLASH_INPAGE.
* @param allowScriptAccess allowScriptAccess or {@code null} for none
*/
public Creative setAllowScriptAccess(java.lang.Boolean allowScriptAccess) {
this.allowScriptAccess = allowScriptAccess;
return this;
}
/**
* Whether the creative is archived. Applicable to all creative types.
* @return value or {@code null} for none
*/
public java.lang.Boolean getArchived() {
return archived;
}
/**
* Whether the creative is archived. Applicable to all creative types.
* @param archived archived or {@code null} for none
*/
public Creative setArchived(java.lang.Boolean archived) {
this.archived = archived;
return this;
}
/**
* Type of artwork used for the creative. This is a read-only field. Applicable to the following
* creative types: all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.String getArtworkType() {
return artworkType;
}
/**
* Type of artwork used for the creative. This is a read-only field. Applicable to the following
* creative types: all RICH_MEDIA, and all VPAID.
* @param artworkType artworkType or {@code null} for none
*/
public Creative setArtworkType(java.lang.String artworkType) {
this.artworkType = artworkType;
return this;
}
/**
* Source application where creative was authored. Presently, only DBM authored creatives will
* have this field set. Applicable to all creative types.
* @return value or {@code null} for none
*/
public java.lang.String getAuthoringSource() {
return authoringSource;
}
/**
* Source application where creative was authored. Presently, only DBM authored creatives will
* have this field set. Applicable to all creative types.
* @param authoringSource authoringSource or {@code null} for none
*/
public Creative setAuthoringSource(java.lang.String authoringSource) {
this.authoringSource = authoringSource;
return this;
}
/**
* Authoring tool for HTML5 banner creatives. This is a read-only field. Applicable to the
* following creative types: HTML5_BANNER.
* @return value or {@code null} for none
*/
public java.lang.String getAuthoringTool() {
return authoringTool;
}
/**
* Authoring tool for HTML5 banner creatives. This is a read-only field. Applicable to the
* following creative types: HTML5_BANNER.
* @param authoringTool authoringTool or {@code null} for none
*/
public Creative setAuthoringTool(java.lang.String authoringTool) {
this.authoringTool = authoringTool;
return this;
}
/**
* Whether images are automatically advanced for image gallery creatives. Applicable to the
* following creative types: DISPLAY_IMAGE_GALLERY.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAutoAdvanceImages() {
return autoAdvanceImages;
}
/**
* Whether images are automatically advanced for image gallery creatives. Applicable to the
* following creative types: DISPLAY_IMAGE_GALLERY.
* @param autoAdvanceImages autoAdvanceImages or {@code null} for none
*/
public Creative setAutoAdvanceImages(java.lang.Boolean autoAdvanceImages) {
this.autoAdvanceImages = autoAdvanceImages;
return this;
}
/**
* The 6-character HTML color code, beginning with #, for the background of the window area where
* the Flash file is displayed. Default is white. Applicable to the following creative types:
* FLASH_INPAGE.
* @return value or {@code null} for none
*/
public java.lang.String getBackgroundColor() {
return backgroundColor;
}
/**
* The 6-character HTML color code, beginning with #, for the background of the window area where
* the Flash file is displayed. Default is white. Applicable to the following creative types:
* FLASH_INPAGE.
* @param backgroundColor backgroundColor or {@code null} for none
*/
public Creative setBackgroundColor(java.lang.String backgroundColor) {
this.backgroundColor = backgroundColor;
return this;
}
/**
* Click-through URL for backup image. Applicable to ENHANCED_BANNER when the primary asset type
* is not HTML_IMAGE.
* @return value or {@code null} for none
*/
public CreativeClickThroughUrl getBackupImageClickThroughUrl() {
return backupImageClickThroughUrl;
}
/**
* Click-through URL for backup image. Applicable to ENHANCED_BANNER when the primary asset type
* is not HTML_IMAGE.
* @param backupImageClickThroughUrl backupImageClickThroughUrl or {@code null} for none
*/
public Creative setBackupImageClickThroughUrl(CreativeClickThroughUrl backupImageClickThroughUrl) {
this.backupImageClickThroughUrl = backupImageClickThroughUrl;
return this;
}
/**
* List of feature dependencies that will cause a backup image to be served if the browser that
* serves the ad does not support them. Feature dependencies are features that a browser must be
* able to support in order to render your HTML5 creative asset correctly. This field is initially
* auto-generated to contain all features detected by Campaign Manager for all the assets of this
* creative and can then be modified by the client. To reset this field, copy over all the
* creativeAssets' detected features. Applicable to the following creative types: HTML5_BANNER.
* Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* @return value or {@code null} for none
*/
public java.util.List getBackupImageFeatures() {
return backupImageFeatures;
}
/**
* List of feature dependencies that will cause a backup image to be served if the browser that
* serves the ad does not support them. Feature dependencies are features that a browser must be
* able to support in order to render your HTML5 creative asset correctly. This field is initially
* auto-generated to contain all features detected by Campaign Manager for all the assets of this
* creative and can then be modified by the client. To reset this field, copy over all the
* creativeAssets' detected features. Applicable to the following creative types: HTML5_BANNER.
* Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* @param backupImageFeatures backupImageFeatures or {@code null} for none
*/
public Creative setBackupImageFeatures(java.util.List backupImageFeatures) {
this.backupImageFeatures = backupImageFeatures;
return this;
}
/**
* Reporting label used for HTML5 banner backup image. Applicable to the following creative types:
* DISPLAY when the primary asset type is not HTML_IMAGE.
* @return value or {@code null} for none
*/
public java.lang.String getBackupImageReportingLabel() {
return backupImageReportingLabel;
}
/**
* Reporting label used for HTML5 banner backup image. Applicable to the following creative types:
* DISPLAY when the primary asset type is not HTML_IMAGE.
* @param backupImageReportingLabel backupImageReportingLabel or {@code null} for none
*/
public Creative setBackupImageReportingLabel(java.lang.String backupImageReportingLabel) {
this.backupImageReportingLabel = backupImageReportingLabel;
return this;
}
/**
* Target window for backup image. Applicable to the following creative types: FLASH_INPAGE and
* HTML5_BANNER. Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* @return value or {@code null} for none
*/
public TargetWindow getBackupImageTargetWindow() {
return backupImageTargetWindow;
}
/**
* Target window for backup image. Applicable to the following creative types: FLASH_INPAGE and
* HTML5_BANNER. Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* @param backupImageTargetWindow backupImageTargetWindow or {@code null} for none
*/
public Creative setBackupImageTargetWindow(TargetWindow backupImageTargetWindow) {
this.backupImageTargetWindow = backupImageTargetWindow;
return this;
}
/**
* Click tags of the creative. For DISPLAY, FLASH_INPAGE, and HTML5_BANNER creatives, this is a
* subset of detected click tags for the assets associated with this creative. After creating a
* flash asset, detected click tags will be returned in the creativeAssetMetadata. When inserting
* the creative, populate the creative clickTags field using the creativeAssetMetadata.clickTags
* field. For DISPLAY_IMAGE_GALLERY creatives, there should be exactly one entry in this list for
* each image creative asset. A click tag is matched with a corresponding creative asset by
* matching the clickTag.name field with the creativeAsset.assetIdentifier.name field. Applicable
* to the following creative types: DISPLAY_IMAGE_GALLERY, FLASH_INPAGE, HTML5_BANNER. Applicable
* to DISPLAY when the primary asset type is not HTML_IMAGE.
* @return value or {@code null} for none
*/
public java.util.List getClickTags() {
return clickTags;
}
/**
* Click tags of the creative. For DISPLAY, FLASH_INPAGE, and HTML5_BANNER creatives, this is a
* subset of detected click tags for the assets associated with this creative. After creating a
* flash asset, detected click tags will be returned in the creativeAssetMetadata. When inserting
* the creative, populate the creative clickTags field using the creativeAssetMetadata.clickTags
* field. For DISPLAY_IMAGE_GALLERY creatives, there should be exactly one entry in this list for
* each image creative asset. A click tag is matched with a corresponding creative asset by
* matching the clickTag.name field with the creativeAsset.assetIdentifier.name field. Applicable
* to the following creative types: DISPLAY_IMAGE_GALLERY, FLASH_INPAGE, HTML5_BANNER. Applicable
* to DISPLAY when the primary asset type is not HTML_IMAGE.
* @param clickTags clickTags or {@code null} for none
*/
public Creative setClickTags(java.util.List clickTags) {
this.clickTags = clickTags;
return this;
}
/**
* Industry standard ID assigned to creative for reach and frequency. Applicable to
* INSTREAM_VIDEO_REDIRECT creatives.
* @return value or {@code null} for none
*/
public java.lang.String getCommercialId() {
return commercialId;
}
/**
* Industry standard ID assigned to creative for reach and frequency. Applicable to
* INSTREAM_VIDEO_REDIRECT creatives.
* @param commercialId commercialId or {@code null} for none
*/
public Creative setCommercialId(java.lang.String commercialId) {
this.commercialId = commercialId;
return this;
}
/**
* List of companion creatives assigned to an in-Stream video creative. Acceptable values include
* IDs of existing flash and image creatives. Applicable to the following creative types: all
* VPAID, all INSTREAM_AUDIO and all INSTREAM_VIDEO with dynamicAssetSelection set to false.
* @return value or {@code null} for none
*/
public java.util.List getCompanionCreatives() {
return companionCreatives;
}
/**
* List of companion creatives assigned to an in-Stream video creative. Acceptable values include
* IDs of existing flash and image creatives. Applicable to the following creative types: all
* VPAID, all INSTREAM_AUDIO and all INSTREAM_VIDEO with dynamicAssetSelection set to false.
* @param companionCreatives companionCreatives or {@code null} for none
*/
public Creative setCompanionCreatives(java.util.List companionCreatives) {
this.companionCreatives = companionCreatives;
return this;
}
/**
* Compatibilities associated with this creative. This is a read-only field. DISPLAY and
* DISPLAY_INTERSTITIAL refer to rendering either on desktop or on mobile devices or in mobile
* apps for regular or interstitial ads, respectively. APP and APP_INTERSTITIAL are for rendering
* in mobile apps. Only pre-existing creatives may have these compatibilities since new creatives
* will either be assigned DISPLAY or DISPLAY_INTERSTITIAL instead. IN_STREAM_VIDEO refers to
* rendering in in-stream video ads developed with the VAST standard. IN_STREAM_AUDIO refers to
* rendering in in-stream audio ads developed with the VAST standard. Applicable to all creative
* types. Acceptable values are: - "APP" - "APP_INTERSTITIAL" - "IN_STREAM_VIDEO" -
* "IN_STREAM_AUDIO" - "DISPLAY" - "DISPLAY_INTERSTITIAL"
* @return value or {@code null} for none
*/
public java.util.List getCompatibility() {
return compatibility;
}
/**
* Compatibilities associated with this creative. This is a read-only field. DISPLAY and
* DISPLAY_INTERSTITIAL refer to rendering either on desktop or on mobile devices or in mobile
* apps for regular or interstitial ads, respectively. APP and APP_INTERSTITIAL are for rendering
* in mobile apps. Only pre-existing creatives may have these compatibilities since new creatives
* will either be assigned DISPLAY or DISPLAY_INTERSTITIAL instead. IN_STREAM_VIDEO refers to
* rendering in in-stream video ads developed with the VAST standard. IN_STREAM_AUDIO refers to
* rendering in in-stream audio ads developed with the VAST standard. Applicable to all creative
* types. Acceptable values are: - "APP" - "APP_INTERSTITIAL" - "IN_STREAM_VIDEO" -
* "IN_STREAM_AUDIO" - "DISPLAY" - "DISPLAY_INTERSTITIAL"
* @param compatibility compatibility or {@code null} for none
*/
public Creative setCompatibility(java.util.List compatibility) {
this.compatibility = compatibility;
return this;
}
/**
* Whether Flash assets associated with the creative need to be automatically converted to HTML5.
* This flag is enabled by default and users can choose to disable it if they don't want the
* system to generate and use HTML5 asset for this creative. Applicable to the following creative
* type: FLASH_INPAGE. Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* @return value or {@code null} for none
*/
public java.lang.Boolean getConvertFlashToHtml5() {
return convertFlashToHtml5;
}
/**
* Whether Flash assets associated with the creative need to be automatically converted to HTML5.
* This flag is enabled by default and users can choose to disable it if they don't want the
* system to generate and use HTML5 asset for this creative. Applicable to the following creative
* type: FLASH_INPAGE. Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* @param convertFlashToHtml5 convertFlashToHtml5 or {@code null} for none
*/
public Creative setConvertFlashToHtml5(java.lang.Boolean convertFlashToHtml5) {
this.convertFlashToHtml5 = convertFlashToHtml5;
return this;
}
/**
* List of counter events configured for the creative. For DISPLAY_IMAGE_GALLERY creatives, these
* are read-only and auto-generated from clickTags. Applicable to the following creative types:
* DISPLAY_IMAGE_GALLERY, all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.util.List getCounterCustomEvents() {
return counterCustomEvents;
}
/**
* List of counter events configured for the creative. For DISPLAY_IMAGE_GALLERY creatives, these
* are read-only and auto-generated from clickTags. Applicable to the following creative types:
* DISPLAY_IMAGE_GALLERY, all RICH_MEDIA, and all VPAID.
* @param counterCustomEvents counterCustomEvents or {@code null} for none
*/
public Creative setCounterCustomEvents(java.util.List counterCustomEvents) {
this.counterCustomEvents = counterCustomEvents;
return this;
}
/**
* Required if dynamicAssetSelection is true.
* @return value or {@code null} for none
*/
public CreativeAssetSelection getCreativeAssetSelection() {
return creativeAssetSelection;
}
/**
* Required if dynamicAssetSelection is true.
* @param creativeAssetSelection creativeAssetSelection or {@code null} for none
*/
public Creative setCreativeAssetSelection(CreativeAssetSelection creativeAssetSelection) {
this.creativeAssetSelection = creativeAssetSelection;
return this;
}
/**
* Assets associated with a creative. Applicable to all but the following creative types:
* INTERNAL_REDIRECT, INTERSTITIAL_INTERNAL_REDIRECT, and REDIRECT
* @return value or {@code null} for none
*/
public java.util.List getCreativeAssets() {
return creativeAssets;
}
/**
* Assets associated with a creative. Applicable to all but the following creative types:
* INTERNAL_REDIRECT, INTERSTITIAL_INTERNAL_REDIRECT, and REDIRECT
* @param creativeAssets creativeAssets or {@code null} for none
*/
public Creative setCreativeAssets(java.util.List creativeAssets) {
this.creativeAssets = creativeAssets;
return this;
}
/**
* Creative field assignments for this creative. Applicable to all creative types.
* @return value or {@code null} for none
*/
public java.util.List getCreativeFieldAssignments() {
return creativeFieldAssignments;
}
/**
* Creative field assignments for this creative. Applicable to all creative types.
* @param creativeFieldAssignments creativeFieldAssignments or {@code null} for none
*/
public Creative setCreativeFieldAssignments(java.util.List creativeFieldAssignments) {
this.creativeFieldAssignments = creativeFieldAssignments;
return this;
}
/**
* Custom key-values for a Rich Media creative. Key-values let you customize the creative settings
* of a Rich Media ad running on your site without having to contact the advertiser. You can use
* key-values to dynamically change the look or functionality of a creative. Applicable to the
* following creative types: all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.util.List getCustomKeyValues() {
return customKeyValues;
}
/**
* Custom key-values for a Rich Media creative. Key-values let you customize the creative settings
* of a Rich Media ad running on your site without having to contact the advertiser. You can use
* key-values to dynamically change the look or functionality of a creative. Applicable to the
* following creative types: all RICH_MEDIA, and all VPAID.
* @param customKeyValues customKeyValues or {@code null} for none
*/
public Creative setCustomKeyValues(java.util.List customKeyValues) {
this.customKeyValues = customKeyValues;
return this;
}
/**
* Set this to true to enable the use of rules to target individual assets in this creative. When
* set to true creativeAssetSelection must be set. This also controls asset-level companions. When
* this is true, companion creatives should be assigned to creative assets. Learn more. Applicable
* to INSTREAM_VIDEO creatives.
* @return value or {@code null} for none
*/
public java.lang.Boolean getDynamicAssetSelection() {
return dynamicAssetSelection;
}
/**
* Set this to true to enable the use of rules to target individual assets in this creative. When
* set to true creativeAssetSelection must be set. This also controls asset-level companions. When
* this is true, companion creatives should be assigned to creative assets. Learn more. Applicable
* to INSTREAM_VIDEO creatives.
* @param dynamicAssetSelection dynamicAssetSelection or {@code null} for none
*/
public Creative setDynamicAssetSelection(java.lang.Boolean dynamicAssetSelection) {
this.dynamicAssetSelection = dynamicAssetSelection;
return this;
}
/**
* List of exit events configured for the creative. For DISPLAY and DISPLAY_IMAGE_GALLERY
* creatives, these are read-only and auto-generated from clickTags, For DISPLAY, an event is also
* created from the backupImageReportingLabel. Applicable to the following creative types:
* DISPLAY_IMAGE_GALLERY, all RICH_MEDIA, and all VPAID. Applicable to DISPLAY when the primary
* asset type is not HTML_IMAGE.
* @return value or {@code null} for none
*/
public java.util.List getExitCustomEvents() {
return exitCustomEvents;
}
/**
* List of exit events configured for the creative. For DISPLAY and DISPLAY_IMAGE_GALLERY
* creatives, these are read-only and auto-generated from clickTags, For DISPLAY, an event is also
* created from the backupImageReportingLabel. Applicable to the following creative types:
* DISPLAY_IMAGE_GALLERY, all RICH_MEDIA, and all VPAID. Applicable to DISPLAY when the primary
* asset type is not HTML_IMAGE.
* @param exitCustomEvents exitCustomEvents or {@code null} for none
*/
public Creative setExitCustomEvents(java.util.List exitCustomEvents) {
this.exitCustomEvents = exitCustomEvents;
return this;
}
/**
* OpenWindow FSCommand of this creative. This lets the SWF file communicate with either Flash
* Player or the program hosting Flash Player, such as a web browser. This is only triggered if
* allowScriptAccess field is true. Applicable to the following creative types: FLASH_INPAGE.
* @return value or {@code null} for none
*/
public FsCommand getFsCommand() {
return fsCommand;
}
/**
* OpenWindow FSCommand of this creative. This lets the SWF file communicate with either Flash
* Player or the program hosting Flash Player, such as a web browser. This is only triggered if
* allowScriptAccess field is true. Applicable to the following creative types: FLASH_INPAGE.
* @param fsCommand fsCommand or {@code null} for none
*/
public Creative setFsCommand(FsCommand fsCommand) {
this.fsCommand = fsCommand;
return this;
}
/**
* HTML code for the creative. This is a required field when applicable. This field is ignored if
* htmlCodeLocked is true. Applicable to the following creative types: all CUSTOM, FLASH_INPAGE,
* and HTML5_BANNER, and all RICH_MEDIA.
* @return value or {@code null} for none
*/
public java.lang.String getHtmlCode() {
return htmlCode;
}
/**
* HTML code for the creative. This is a required field when applicable. This field is ignored if
* htmlCodeLocked is true. Applicable to the following creative types: all CUSTOM, FLASH_INPAGE,
* and HTML5_BANNER, and all RICH_MEDIA.
* @param htmlCode htmlCode or {@code null} for none
*/
public Creative setHtmlCode(java.lang.String htmlCode) {
this.htmlCode = htmlCode;
return this;
}
/**
* Whether HTML code is generated by Campaign Manager or manually entered. Set to true to ignore
* changes to htmlCode. Applicable to the following creative types: FLASH_INPAGE and HTML5_BANNER.
* @return value or {@code null} for none
*/
public java.lang.Boolean getHtmlCodeLocked() {
return htmlCodeLocked;
}
/**
* Whether HTML code is generated by Campaign Manager or manually entered. Set to true to ignore
* changes to htmlCode. Applicable to the following creative types: FLASH_INPAGE and HTML5_BANNER.
* @param htmlCodeLocked htmlCodeLocked or {@code null} for none
*/
public Creative setHtmlCodeLocked(java.lang.Boolean htmlCodeLocked) {
this.htmlCodeLocked = htmlCodeLocked;
return this;
}
/**
* ID of this creative. This is a read-only, auto-generated field. Applicable to all creative
* types.
* @return value or {@code null} for none
*/
public java.lang.Long getId() {
return id;
}
/**
* ID of this creative. This is a read-only, auto-generated field. Applicable to all creative
* types.
* @param id id or {@code null} for none
*/
public Creative setId(java.lang.Long id) {
this.id = id;
return this;
}
/**
* Dimension value for the ID of this creative. This is a read-only field. Applicable to all
* creative types.
* @return value or {@code null} for none
*/
public DimensionValue getIdDimensionValue() {
return idDimensionValue;
}
/**
* Dimension value for the ID of this creative. This is a read-only field. Applicable to all
* creative types.
* @param idDimensionValue idDimensionValue or {@code null} for none
*/
public Creative setIdDimensionValue(DimensionValue idDimensionValue) {
this.idDimensionValue = idDimensionValue;
return this;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "dfareporting#creative".
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies what kind of resource this is. Value: the fixed string "dfareporting#creative".
* @param kind kind or {@code null} for none
*/
public Creative setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Creative last modification information. This is a read-only field. Applicable to all creative
* types.
* @return value or {@code null} for none
*/
public LastModifiedInfo getLastModifiedInfo() {
return lastModifiedInfo;
}
/**
* Creative last modification information. This is a read-only field. Applicable to all creative
* types.
* @param lastModifiedInfo lastModifiedInfo or {@code null} for none
*/
public Creative setLastModifiedInfo(LastModifiedInfo lastModifiedInfo) {
this.lastModifiedInfo = lastModifiedInfo;
return this;
}
/**
* Latest Studio trafficked creative ID associated with rich media and VPAID creatives. This is a
* read-only field. Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.Long getLatestTraffickedCreativeId() {
return latestTraffickedCreativeId;
}
/**
* Latest Studio trafficked creative ID associated with rich media and VPAID creatives. This is a
* read-only field. Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @param latestTraffickedCreativeId latestTraffickedCreativeId or {@code null} for none
*/
public Creative setLatestTraffickedCreativeId(java.lang.Long latestTraffickedCreativeId) {
this.latestTraffickedCreativeId = latestTraffickedCreativeId;
return this;
}
/**
* Description of the audio or video ad. Applicable to the following creative types: all
* INSTREAM_VIDEO, INSTREAM_AUDIO, and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.String getMediaDescription() {
return mediaDescription;
}
/**
* Description of the audio or video ad. Applicable to the following creative types: all
* INSTREAM_VIDEO, INSTREAM_AUDIO, and all VPAID.
* @param mediaDescription mediaDescription or {@code null} for none
*/
public Creative setMediaDescription(java.lang.String mediaDescription) {
this.mediaDescription = mediaDescription;
return this;
}
/**
* Creative audio or video duration in seconds. This is a read-only field. Applicable to the
* following creative types: INSTREAM_VIDEO, INSTREAM_AUDIO, all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.Float getMediaDuration() {
return mediaDuration;
}
/**
* Creative audio or video duration in seconds. This is a read-only field. Applicable to the
* following creative types: INSTREAM_VIDEO, INSTREAM_AUDIO, all RICH_MEDIA, and all VPAID.
* @param mediaDuration mediaDuration or {@code null} for none
*/
public Creative setMediaDuration(java.lang.Float mediaDuration) {
this.mediaDuration = mediaDuration;
return this;
}
/**
* Required. Name of the creative. This must be less than 256 characters long. Applicable to all
* creative types.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the creative. This must be less than 256 characters long. Applicable to all
* creative types.
* @param name name or {@code null} for none
*/
public Creative setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Online behavioral advertising icon to be added to the creative. Applicable to the following
* creative types: all INSTREAM_VIDEO.
* @return value or {@code null} for none
*/
public ObaIcon getObaIcon() {
return obaIcon;
}
/**
* Online behavioral advertising icon to be added to the creative. Applicable to the following
* creative types: all INSTREAM_VIDEO.
* @param obaIcon obaIcon or {@code null} for none
*/
public Creative setObaIcon(ObaIcon obaIcon) {
this.obaIcon = obaIcon;
return this;
}
/**
* Override CSS value for rich media creatives. Applicable to the following creative types: all
* RICH_MEDIA.
* @return value or {@code null} for none
*/
public java.lang.String getOverrideCss() {
return overrideCss;
}
/**
* Override CSS value for rich media creatives. Applicable to the following creative types: all
* RICH_MEDIA.
* @param overrideCss overrideCss or {@code null} for none
*/
public Creative setOverrideCss(java.lang.String overrideCss) {
this.overrideCss = overrideCss;
return this;
}
/**
* Amount of time to play the video before counting a view. Applicable to the following creative
* types: all INSTREAM_VIDEO.
* @return value or {@code null} for none
*/
public VideoOffset getProgressOffset() {
return progressOffset;
}
/**
* Amount of time to play the video before counting a view. Applicable to the following creative
* types: all INSTREAM_VIDEO.
* @param progressOffset progressOffset or {@code null} for none
*/
public Creative setProgressOffset(VideoOffset progressOffset) {
this.progressOffset = progressOffset;
return this;
}
/**
* URL of hosted image or hosted video or another ad tag. For INSTREAM_VIDEO_REDIRECT creatives
* this is the in-stream video redirect URL. The standard for a VAST (Video Ad Serving Template)
* ad response allows for a redirect link to another VAST 2.0 or 3.0 call. This is a required
* field when applicable. Applicable to the following creative types: DISPLAY_REDIRECT,
* INTERNAL_REDIRECT, INTERSTITIAL_INTERNAL_REDIRECT, and INSTREAM_VIDEO_REDIRECT
* @return value or {@code null} for none
*/
public java.lang.String getRedirectUrl() {
return redirectUrl;
}
/**
* URL of hosted image or hosted video or another ad tag. For INSTREAM_VIDEO_REDIRECT creatives
* this is the in-stream video redirect URL. The standard for a VAST (Video Ad Serving Template)
* ad response allows for a redirect link to another VAST 2.0 or 3.0 call. This is a required
* field when applicable. Applicable to the following creative types: DISPLAY_REDIRECT,
* INTERNAL_REDIRECT, INTERSTITIAL_INTERNAL_REDIRECT, and INSTREAM_VIDEO_REDIRECT
* @param redirectUrl redirectUrl or {@code null} for none
*/
public Creative setRedirectUrl(java.lang.String redirectUrl) {
this.redirectUrl = redirectUrl;
return this;
}
/**
* ID of current rendering version. This is a read-only field. Applicable to all creative types.
* @return value or {@code null} for none
*/
public java.lang.Long getRenderingId() {
return renderingId;
}
/**
* ID of current rendering version. This is a read-only field. Applicable to all creative types.
* @param renderingId renderingId or {@code null} for none
*/
public Creative setRenderingId(java.lang.Long renderingId) {
this.renderingId = renderingId;
return this;
}
/**
* Dimension value for the rendering ID of this creative. This is a read-only field. Applicable to
* all creative types.
* @return value or {@code null} for none
*/
public DimensionValue getRenderingIdDimensionValue() {
return renderingIdDimensionValue;
}
/**
* Dimension value for the rendering ID of this creative. This is a read-only field. Applicable to
* all creative types.
* @param renderingIdDimensionValue renderingIdDimensionValue or {@code null} for none
*/
public Creative setRenderingIdDimensionValue(DimensionValue renderingIdDimensionValue) {
this.renderingIdDimensionValue = renderingIdDimensionValue;
return this;
}
/**
* The minimum required Flash plugin version for this creative. For example, 11.2.202.235. This is
* a read-only field. Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.String getRequiredFlashPluginVersion() {
return requiredFlashPluginVersion;
}
/**
* The minimum required Flash plugin version for this creative. For example, 11.2.202.235. This is
* a read-only field. Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @param requiredFlashPluginVersion requiredFlashPluginVersion or {@code null} for none
*/
public Creative setRequiredFlashPluginVersion(java.lang.String requiredFlashPluginVersion) {
this.requiredFlashPluginVersion = requiredFlashPluginVersion;
return this;
}
/**
* The internal Flash version for this creative as calculated by Studio. This is a read-only
* field. Applicable to the following creative types: FLASH_INPAGE all RICH_MEDIA, and all VPAID.
* Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* @return value or {@code null} for none
*/
public java.lang.Integer getRequiredFlashVersion() {
return requiredFlashVersion;
}
/**
* The internal Flash version for this creative as calculated by Studio. This is a read-only
* field. Applicable to the following creative types: FLASH_INPAGE all RICH_MEDIA, and all VPAID.
* Applicable to DISPLAY when the primary asset type is not HTML_IMAGE.
* @param requiredFlashVersion requiredFlashVersion or {@code null} for none
*/
public Creative setRequiredFlashVersion(java.lang.Integer requiredFlashVersion) {
this.requiredFlashVersion = requiredFlashVersion;
return this;
}
/**
* Size associated with this creative. When inserting or updating a creative either the size ID
* field or size width and height fields can be used. This is a required field when applicable;
* however for IMAGE, FLASH_INPAGE creatives, and for DISPLAY creatives with a primary asset of
* type HTML_IMAGE, if left blank, this field will be automatically set using the actual size of
* the associated image assets. Applicable to the following creative types: DISPLAY,
* DISPLAY_IMAGE_GALLERY, FLASH_INPAGE, HTML5_BANNER, IMAGE, and all RICH_MEDIA.
* @return value or {@code null} for none
*/
public Size getSize() {
return size;
}
/**
* Size associated with this creative. When inserting or updating a creative either the size ID
* field or size width and height fields can be used. This is a required field when applicable;
* however for IMAGE, FLASH_INPAGE creatives, and for DISPLAY creatives with a primary asset of
* type HTML_IMAGE, if left blank, this field will be automatically set using the actual size of
* the associated image assets. Applicable to the following creative types: DISPLAY,
* DISPLAY_IMAGE_GALLERY, FLASH_INPAGE, HTML5_BANNER, IMAGE, and all RICH_MEDIA.
* @param size size or {@code null} for none
*/
public Creative setSize(Size size) {
this.size = size;
return this;
}
/**
* Amount of time to play the video before the skip button appears. Applicable to the following
* creative types: all INSTREAM_VIDEO.
* @return value or {@code null} for none
*/
public VideoOffset getSkipOffset() {
return skipOffset;
}
/**
* Amount of time to play the video before the skip button appears. Applicable to the following
* creative types: all INSTREAM_VIDEO.
* @param skipOffset skipOffset or {@code null} for none
*/
public Creative setSkipOffset(VideoOffset skipOffset) {
this.skipOffset = skipOffset;
return this;
}
/**
* Whether the user can choose to skip the creative. Applicable to the following creative types:
* all INSTREAM_VIDEO and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSkippable() {
return skippable;
}
/**
* Whether the user can choose to skip the creative. Applicable to the following creative types:
* all INSTREAM_VIDEO and all VPAID.
* @param skippable skippable or {@code null} for none
*/
public Creative setSkippable(java.lang.Boolean skippable) {
this.skippable = skippable;
return this;
}
/**
* Whether the creative is SSL-compliant. This is a read-only field. Applicable to all creative
* types.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSslCompliant() {
return sslCompliant;
}
/**
* Whether the creative is SSL-compliant. This is a read-only field. Applicable to all creative
* types.
* @param sslCompliant sslCompliant or {@code null} for none
*/
public Creative setSslCompliant(java.lang.Boolean sslCompliant) {
this.sslCompliant = sslCompliant;
return this;
}
/**
* Whether creative should be treated as SSL compliant even if the system scan shows it's not.
* Applicable to all creative types.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSslOverride() {
return sslOverride;
}
/**
* Whether creative should be treated as SSL compliant even if the system scan shows it's not.
* Applicable to all creative types.
* @param sslOverride sslOverride or {@code null} for none
*/
public Creative setSslOverride(java.lang.Boolean sslOverride) {
this.sslOverride = sslOverride;
return this;
}
/**
* Studio advertiser ID associated with rich media and VPAID creatives. This is a read-only field.
* Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.Long getStudioAdvertiserId() {
return studioAdvertiserId;
}
/**
* Studio advertiser ID associated with rich media and VPAID creatives. This is a read-only field.
* Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @param studioAdvertiserId studioAdvertiserId or {@code null} for none
*/
public Creative setStudioAdvertiserId(java.lang.Long studioAdvertiserId) {
this.studioAdvertiserId = studioAdvertiserId;
return this;
}
/**
* Studio creative ID associated with rich media and VPAID creatives. This is a read-only field.
* Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.Long getStudioCreativeId() {
return studioCreativeId;
}
/**
* Studio creative ID associated with rich media and VPAID creatives. This is a read-only field.
* Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @param studioCreativeId studioCreativeId or {@code null} for none
*/
public Creative setStudioCreativeId(java.lang.Long studioCreativeId) {
this.studioCreativeId = studioCreativeId;
return this;
}
/**
* Studio trafficked creative ID associated with rich media and VPAID creatives. This is a read-
* only field. Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.Long getStudioTraffickedCreativeId() {
return studioTraffickedCreativeId;
}
/**
* Studio trafficked creative ID associated with rich media and VPAID creatives. This is a read-
* only field. Applicable to the following creative types: all RICH_MEDIA, and all VPAID.
* @param studioTraffickedCreativeId studioTraffickedCreativeId or {@code null} for none
*/
public Creative setStudioTraffickedCreativeId(java.lang.Long studioTraffickedCreativeId) {
this.studioTraffickedCreativeId = studioTraffickedCreativeId;
return this;
}
/**
* Subaccount ID of this creative. This field, if left unset, will be auto-generated for both
* insert and update operations. Applicable to all creative types.
* @return value or {@code null} for none
*/
public java.lang.Long getSubaccountId() {
return subaccountId;
}
/**
* Subaccount ID of this creative. This field, if left unset, will be auto-generated for both
* insert and update operations. Applicable to all creative types.
* @param subaccountId subaccountId or {@code null} for none
*/
public Creative setSubaccountId(java.lang.Long subaccountId) {
this.subaccountId = subaccountId;
return this;
}
/**
* Third-party URL used to record backup image impressions. Applicable to the following creative
* types: all RICH_MEDIA.
* @return value or {@code null} for none
*/
public java.lang.String getThirdPartyBackupImageImpressionsUrl() {
return thirdPartyBackupImageImpressionsUrl;
}
/**
* Third-party URL used to record backup image impressions. Applicable to the following creative
* types: all RICH_MEDIA.
* @param thirdPartyBackupImageImpressionsUrl thirdPartyBackupImageImpressionsUrl or {@code null} for none
*/
public Creative setThirdPartyBackupImageImpressionsUrl(java.lang.String thirdPartyBackupImageImpressionsUrl) {
this.thirdPartyBackupImageImpressionsUrl = thirdPartyBackupImageImpressionsUrl;
return this;
}
/**
* Third-party URL used to record rich media impressions. Applicable to the following creative
* types: all RICH_MEDIA.
* @return value or {@code null} for none
*/
public java.lang.String getThirdPartyRichMediaImpressionsUrl() {
return thirdPartyRichMediaImpressionsUrl;
}
/**
* Third-party URL used to record rich media impressions. Applicable to the following creative
* types: all RICH_MEDIA.
* @param thirdPartyRichMediaImpressionsUrl thirdPartyRichMediaImpressionsUrl or {@code null} for none
*/
public Creative setThirdPartyRichMediaImpressionsUrl(java.lang.String thirdPartyRichMediaImpressionsUrl) {
this.thirdPartyRichMediaImpressionsUrl = thirdPartyRichMediaImpressionsUrl;
return this;
}
/**
* Third-party URLs for tracking in-stream creative events. Applicable to the following creative
* types: all INSTREAM_VIDEO, all INSTREAM_AUDIO, and all VPAID.
* @return value or {@code null} for none
*/
public java.util.List getThirdPartyUrls() {
return thirdPartyUrls;
}
/**
* Third-party URLs for tracking in-stream creative events. Applicable to the following creative
* types: all INSTREAM_VIDEO, all INSTREAM_AUDIO, and all VPAID.
* @param thirdPartyUrls thirdPartyUrls or {@code null} for none
*/
public Creative setThirdPartyUrls(java.util.List thirdPartyUrls) {
this.thirdPartyUrls = thirdPartyUrls;
return this;
}
/**
* List of timer events configured for the creative. For DISPLAY_IMAGE_GALLERY creatives, these
* are read-only and auto-generated from clickTags. Applicable to the following creative types:
* DISPLAY_IMAGE_GALLERY, all RICH_MEDIA, and all VPAID. Applicable to DISPLAY when the primary
* asset is not HTML_IMAGE.
* @return value or {@code null} for none
*/
public java.util.List getTimerCustomEvents() {
return timerCustomEvents;
}
/**
* List of timer events configured for the creative. For DISPLAY_IMAGE_GALLERY creatives, these
* are read-only and auto-generated from clickTags. Applicable to the following creative types:
* DISPLAY_IMAGE_GALLERY, all RICH_MEDIA, and all VPAID. Applicable to DISPLAY when the primary
* asset is not HTML_IMAGE.
* @param timerCustomEvents timerCustomEvents or {@code null} for none
*/
public Creative setTimerCustomEvents(java.util.List timerCustomEvents) {
this.timerCustomEvents = timerCustomEvents;
return this;
}
/**
* Combined size of all creative assets. This is a read-only field. Applicable to the following
* creative types: all RICH_MEDIA, and all VPAID.
* @return value or {@code null} for none
*/
public java.lang.Long getTotalFileSize() {
return totalFileSize;
}
/**
* Combined size of all creative assets. This is a read-only field. Applicable to the following
* creative types: all RICH_MEDIA, and all VPAID.
* @param totalFileSize totalFileSize or {@code null} for none
*/
public Creative setTotalFileSize(java.lang.Long totalFileSize) {
this.totalFileSize = totalFileSize;
return this;
}
/**
* Required. Type of this creative. Applicable to all creative types. *Note:* FLASH_INPAGE,
* HTML5_BANNER, and IMAGE are only used for existing creatives. New creatives should use DISPLAY
* as a replacement for these types.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Required. Type of this creative. Applicable to all creative types. *Note:* FLASH_INPAGE,
* HTML5_BANNER, and IMAGE are only used for existing creatives. New creatives should use DISPLAY
* as a replacement for these types.
* @param type type or {@code null} for none
*/
public Creative setType(java.lang.String type) {
this.type = type;
return this;
}
/**
* A Universal Ad ID as per the VAST 4.0 spec. Applicable to the following creative types:
* INSTREAM_AUDIO and INSTREAM_VIDEO and VPAID.
* @return value or {@code null} for none
*/
public UniversalAdId getUniversalAdId() {
return universalAdId;
}
/**
* A Universal Ad ID as per the VAST 4.0 spec. Applicable to the following creative types:
* INSTREAM_AUDIO and INSTREAM_VIDEO and VPAID.
* @param universalAdId universalAdId or {@code null} for none
*/
public Creative setUniversalAdId(UniversalAdId universalAdId) {
this.universalAdId = universalAdId;
return this;
}
/**
* The version number helps you keep track of multiple versions of your creative in your reports.
* The version number will always be auto-generated during insert operations to start at 1. For
* tracking creatives the version cannot be incremented and will always remain at 1. For all other
* creative types the version can be incremented only by 1 during update operations. In addition,
* the version will be automatically incremented by 1 when undergoing Rich Media creative merging.
* Applicable to all creative types.
* @return value or {@code null} for none
*/
public java.lang.Integer getVersion() {
return version;
}
/**
* The version number helps you keep track of multiple versions of your creative in your reports.
* The version number will always be auto-generated during insert operations to start at 1. For
* tracking creatives the version cannot be incremented and will always remain at 1. For all other
* creative types the version can be incremented only by 1 during update operations. In addition,
* the version will be automatically incremented by 1 when undergoing Rich Media creative merging.
* Applicable to all creative types.
* @param version version or {@code null} for none
*/
public Creative setVersion(java.lang.Integer version) {
this.version = version;
return this;
}
@Override
public Creative set(String fieldName, Object value) {
return (Creative) super.set(fieldName, value);
}
@Override
public Creative clone() {
return (Creative) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy