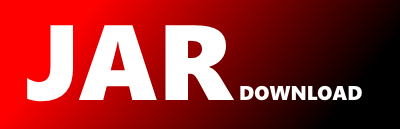
com.google.api.services.dialogflow.v3.Dialogflow Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dialogflow.v3;
/**
* Service definition for Dialogflow (v3).
*
*
* Builds conversational interfaces (for example, chatbots, and voice-powered apps and devices).
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link DialogflowRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Dialogflow extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Dialogflow API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://dialogflow.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://dialogflow.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Dialogflow(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Dialogflow(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Projects.List request = dialogflow.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Locations.List request = dialogflow.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the location.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Gets information about a location.
*
* Create a request for the method "locations.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the location.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudLocationLocation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Resource name for the location. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the location.
*/
public java.lang.String getName() {
return name;
}
/** Resource name for the location. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The resource that owns the locations collection, if applicable.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}/locations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists information about the supported locations for this service.
*
* Create a request for the method "locations.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The resource that owns the locations collection, if applicable.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudLocationListLocationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The resource that owns the locations collection, if applicable. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The resource that owns the locations collection, if applicable.
*/
public java.lang.String getName() {
return name;
}
/** The resource that owns the locations collection, if applicable. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A filter to narrow down results to a preferred subset. The filtering language accepts strings like
`"displayName=tokyo"`, and is documented in more detail in [AIP-160](https://google.aip.dev/160).
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A filter to narrow down results to a preferred subset. The filtering language accepts
* strings like `"displayName=tokyo"`, and is documented in more detail in
* [AIP-160](https://google.aip.dev/160).
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The maximum number of results to return. If not set, the service selects a default. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If not set, the service selects a default.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The maximum number of results to return. If not set, the service selects a default. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received from the `next_page_token` field in the response. Send that page token to
receive the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received from the `next_page_token` field in the response. Send that page
* token to receive the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Agents collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Agents.List request = dialogflow.agents().list(parameters ...)}
*
*
* @return the resource collection
*/
public Agents agents() {
return new Agents();
}
/**
* The "agents" collection of methods.
*/
public class Agents {
/**
* Creates an agent in the specified location. Note: You should always train flows prior to sending
* them queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "agents.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The location to create a agent for. Format: `projects//locations/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/agents";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Creates an agent in the specified location. Note: You should always train flows prior to
* sending them queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "agents.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The location to create a agent for. Format: `projects//locations/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/** Required. The location to create a agent for. Format: `projects//locations/`. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The location to create a agent for. Format: `projects//locations/`.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The location to create a agent for. Format: `projects//locations/`. */
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified agent.
*
* Create a request for the method "agents.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the agent to delete. Format: `projects//locations//agents/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Deletes the specified agent.
*
* Create a request for the method "agents.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the agent to delete. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the agent to delete. Format: `projects//locations//agents/`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the agent to delete. Format: `projects//locations//agents/`.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the agent to delete. Format: `projects//locations//agents/`. */
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Exports the specified agent to a binary file. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: An empty [Struct
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#struct) -
* `response`: ExportAgentResponse
*
* Create a request for the method "agents.export".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the agent to export. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportAgentRequest}
* @return the request
*/
public Export export(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportAgentRequest content) throws java.io.IOException {
Export result = new Export(name, content);
initialize(result);
return result;
}
public class Export extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:export";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Exports the specified agent to a binary file. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`: An empty
* [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: ExportAgentResponse
*
* Create a request for the method "agents.export".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Export#execute()} method to invoke the remote operation.
* {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the agent to export. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportAgentRequest}
* @since 1.13
*/
protected Export(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportAgentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the agent to export. Format: `projects//locations//agents/`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the agent to export. Format: `projects//locations//agents/`.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the agent to export. Format: `projects//locations//agents/`. */
public Export setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Retrieves the specified agent.
*
* Create a request for the method "agents.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the agent. Format: `projects//locations//agents/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Retrieves the specified agent.
*
* Create a request for the method "agents.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the agent. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the agent. Format: `projects//locations//agents/`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the agent. Format: `projects//locations//agents/`.
*/
public java.lang.String getName() {
return name;
}
/** Required. The name of the agent. Format: `projects//locations//agents/`. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the generative settings for the agent.
*
* Create a request for the method "agents.getGenerativeSettings".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link GetGenerativeSettings#execute()} method to invoke the remote
* operation.
*
* @param name Required. Format: `projects//locations//agents//generativeSettings`.
* @return the request
*/
public GetGenerativeSettings getGenerativeSettings(java.lang.String name) throws java.io.IOException {
GetGenerativeSettings result = new GetGenerativeSettings(name);
initialize(result);
return result;
}
public class GetGenerativeSettings extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/generativeSettings$");
/**
* Gets the generative settings for the agent.
*
* Create a request for the method "agents.getGenerativeSettings".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link GetGenerativeSettings#execute()} method to invoke the
* remote operation. {@link GetGenerativeSettings#initialize(com.google.api.client.googleapis.
* services.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Required. Format: `projects//locations//agents//generativeSettings`.
* @since 1.13
*/
protected GetGenerativeSettings(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3GenerativeSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generativeSettings$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetGenerativeSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetGenerativeSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetGenerativeSettings setAccessToken(java.lang.String accessToken) {
return (GetGenerativeSettings) super.setAccessToken(accessToken);
}
@Override
public GetGenerativeSettings setAlt(java.lang.String alt) {
return (GetGenerativeSettings) super.setAlt(alt);
}
@Override
public GetGenerativeSettings setCallback(java.lang.String callback) {
return (GetGenerativeSettings) super.setCallback(callback);
}
@Override
public GetGenerativeSettings setFields(java.lang.String fields) {
return (GetGenerativeSettings) super.setFields(fields);
}
@Override
public GetGenerativeSettings setKey(java.lang.String key) {
return (GetGenerativeSettings) super.setKey(key);
}
@Override
public GetGenerativeSettings setOauthToken(java.lang.String oauthToken) {
return (GetGenerativeSettings) super.setOauthToken(oauthToken);
}
@Override
public GetGenerativeSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetGenerativeSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetGenerativeSettings setQuotaUser(java.lang.String quotaUser) {
return (GetGenerativeSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetGenerativeSettings setUploadType(java.lang.String uploadType) {
return (GetGenerativeSettings) super.setUploadType(uploadType);
}
@Override
public GetGenerativeSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetGenerativeSettings) super.setUploadProtocol(uploadProtocol);
}
/** Required. Format: `projects//locations//agents//generativeSettings`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Format: `projects//locations//agents//generativeSettings`.
*/
public java.lang.String getName() {
return name;
}
/** Required. Format: `projects//locations//agents//generativeSettings`. */
public GetGenerativeSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generativeSettings$");
}
this.name = name;
return this;
}
/** Required. Language code of the generative settings. */
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** Required. Language code of the generative settings.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/** Required. Language code of the generative settings. */
public GetGenerativeSettings setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public GetGenerativeSettings set(String parameterName, Object value) {
return (GetGenerativeSettings) super.set(parameterName, value);
}
}
/**
* Gets the latest agent validation result. Agent validation is performed when ValidateAgent is
* called.
*
* Create a request for the method "agents.getValidationResult".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link GetValidationResult#execute()} method to invoke the remote operation.
*
* @param name Required. The agent name. Format: `projects//locations//agents//validationResult`.
* @return the request
*/
public GetValidationResult getValidationResult(java.lang.String name) throws java.io.IOException {
GetValidationResult result = new GetValidationResult(name);
initialize(result);
return result;
}
public class GetValidationResult extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/validationResult$");
/**
* Gets the latest agent validation result. Agent validation is performed when ValidateAgent is
* called.
*
* Create a request for the method "agents.getValidationResult".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link GetValidationResult#execute()} method to invoke the remote
* operation. {@link GetValidationResult#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The agent name. Format: `projects//locations//agents//validationResult`.
* @since 1.13
*/
protected GetValidationResult(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3AgentValidationResult.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/validationResult$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetValidationResult set$Xgafv(java.lang.String $Xgafv) {
return (GetValidationResult) super.set$Xgafv($Xgafv);
}
@Override
public GetValidationResult setAccessToken(java.lang.String accessToken) {
return (GetValidationResult) super.setAccessToken(accessToken);
}
@Override
public GetValidationResult setAlt(java.lang.String alt) {
return (GetValidationResult) super.setAlt(alt);
}
@Override
public GetValidationResult setCallback(java.lang.String callback) {
return (GetValidationResult) super.setCallback(callback);
}
@Override
public GetValidationResult setFields(java.lang.String fields) {
return (GetValidationResult) super.setFields(fields);
}
@Override
public GetValidationResult setKey(java.lang.String key) {
return (GetValidationResult) super.setKey(key);
}
@Override
public GetValidationResult setOauthToken(java.lang.String oauthToken) {
return (GetValidationResult) super.setOauthToken(oauthToken);
}
@Override
public GetValidationResult setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetValidationResult) super.setPrettyPrint(prettyPrint);
}
@Override
public GetValidationResult setQuotaUser(java.lang.String quotaUser) {
return (GetValidationResult) super.setQuotaUser(quotaUser);
}
@Override
public GetValidationResult setUploadType(java.lang.String uploadType) {
return (GetValidationResult) super.setUploadType(uploadType);
}
@Override
public GetValidationResult setUploadProtocol(java.lang.String uploadProtocol) {
return (GetValidationResult) super.setUploadProtocol(uploadProtocol);
}
/** Required. The agent name. Format: `projects//locations//agents//validationResult`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The agent name. Format: `projects//locations//agents//validationResult`.
*/
public java.lang.String getName() {
return name;
}
/** Required. The agent name. Format: `projects//locations//agents//validationResult`. */
public GetValidationResult setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/validationResult$");
}
this.name = name;
return this;
}
/** If not specified, the agent's default language is used. */
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** If not specified, the agent's default language is used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/** If not specified, the agent's default language is used. */
public GetValidationResult setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public GetValidationResult set(String parameterName, Object value) {
return (GetValidationResult) super.set(parameterName, value);
}
}
/**
* Returns the list of all agents in the specified location.
*
* Create a request for the method "agents.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The location to list all agents for. Format: `projects//locations/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/agents";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Returns the list of all agents in the specified location.
*
* Create a request for the method "agents.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The location to list all agents for. Format: `projects//locations/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListAgentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The location to list all agents for. Format: `projects//locations/`. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The location to list all agents for. Format: `projects//locations/`.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. The location to list all agents for. Format: `projects//locations/`. */
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified agent. Note: You should always train flows prior to sending them queries.
* See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "agents.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the agent. Required for the Agents.UpdateAgent method. Agents.CreateAgent
* populates the name automatically. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Updates the specified agent. Note: You should always train flows prior to sending them queries.
* See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "agents.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the agent. Required for the Agents.UpdateAgent method. Agents.CreateAgent
* populates the name automatically. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Agent.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the agent. Required for the Agents.UpdateAgent method.
* Agents.CreateAgent populates the name automatically. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the agent. Required for the Agents.UpdateAgent method. Agents.CreateAgent
populates the name automatically. Format: `projects//locations//agents/`.
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the agent. Required for the Agents.UpdateAgent method.
* Agents.CreateAgent populates the name automatically. Format:
* `projects//locations//agents/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.name = name;
return this;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated. If the mask is not present, all fields will be
updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Restores the specified agent from a binary file. Replaces the current agent with a new one. Note
* that all existing resources in agent (e.g. intents, entity types, flows) will be removed. This
* method is a [long-running operation](https://cloud.google.com/dialogflow/cx/docs/how/long-
* running-operation). The returned `Operation` type has the following method-specific fields: -
* `metadata`: An empty [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: An [Empty
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#empty)
* Note: You should always train flows prior to sending them queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "agents.restore".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Restore#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the agent to restore into. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RestoreAgentRequest}
* @return the request
*/
public Restore restore(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RestoreAgentRequest content) throws java.io.IOException {
Restore result = new Restore(name, content);
initialize(result);
return result;
}
public class Restore extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:restore";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Restores the specified agent from a binary file. Replaces the current agent with a new one.
* Note that all existing resources in agent (e.g. intents, entity types, flows) will be removed.
* This method is a [long-running operation](https://cloud.google.com/dialogflow/cx/docs/how/long-
* running-operation). The returned `Operation` type has the following method-specific fields: -
* `metadata`: An empty [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: An [Empty
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#empty)
* Note: You should always train flows prior to sending them queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "agents.restore".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Restore#execute()} method to invoke the remote operation.
* {@link
* Restore#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the agent to restore into. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RestoreAgentRequest}
* @since 1.13
*/
protected Restore(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RestoreAgentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Restore set$Xgafv(java.lang.String $Xgafv) {
return (Restore) super.set$Xgafv($Xgafv);
}
@Override
public Restore setAccessToken(java.lang.String accessToken) {
return (Restore) super.setAccessToken(accessToken);
}
@Override
public Restore setAlt(java.lang.String alt) {
return (Restore) super.setAlt(alt);
}
@Override
public Restore setCallback(java.lang.String callback) {
return (Restore) super.setCallback(callback);
}
@Override
public Restore setFields(java.lang.String fields) {
return (Restore) super.setFields(fields);
}
@Override
public Restore setKey(java.lang.String key) {
return (Restore) super.setKey(key);
}
@Override
public Restore setOauthToken(java.lang.String oauthToken) {
return (Restore) super.setOauthToken(oauthToken);
}
@Override
public Restore setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Restore) super.setPrettyPrint(prettyPrint);
}
@Override
public Restore setQuotaUser(java.lang.String quotaUser) {
return (Restore) super.setQuotaUser(quotaUser);
}
@Override
public Restore setUploadType(java.lang.String uploadType) {
return (Restore) super.setUploadType(uploadType);
}
@Override
public Restore setUploadProtocol(java.lang.String uploadProtocol) {
return (Restore) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the agent to restore into. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the agent to restore into. Format: `projects//locations//agents/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the agent to restore into. Format:
* `projects//locations//agents/`.
*/
public Restore setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Restore set(String parameterName, Object value) {
return (Restore) super.set(parameterName, value);
}
}
/**
* Updates the generative settings for the agent.
*
* Create a request for the method "agents.updateGenerativeSettings".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link UpdateGenerativeSettings#execute()} method to invoke the remote
* operation.
*
* @param name Format: `projects//locations//agents//generativeSettings`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3GenerativeSettings}
* @return the request
*/
public UpdateGenerativeSettings updateGenerativeSettings(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3GenerativeSettings content) throws java.io.IOException {
UpdateGenerativeSettings result = new UpdateGenerativeSettings(name, content);
initialize(result);
return result;
}
public class UpdateGenerativeSettings extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/generativeSettings$");
/**
* Updates the generative settings for the agent.
*
* Create a request for the method "agents.updateGenerativeSettings".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link UpdateGenerativeSettings#execute()} method to invoke the
* remote operation. {@link UpdateGenerativeSettings#initialize(com.google.api.client.googleap
* is.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name Format: `projects//locations//agents//generativeSettings`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3GenerativeSettings}
* @since 1.13
*/
protected UpdateGenerativeSettings(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3GenerativeSettings content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3GenerativeSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generativeSettings$");
}
}
@Override
public UpdateGenerativeSettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateGenerativeSettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateGenerativeSettings setAccessToken(java.lang.String accessToken) {
return (UpdateGenerativeSettings) super.setAccessToken(accessToken);
}
@Override
public UpdateGenerativeSettings setAlt(java.lang.String alt) {
return (UpdateGenerativeSettings) super.setAlt(alt);
}
@Override
public UpdateGenerativeSettings setCallback(java.lang.String callback) {
return (UpdateGenerativeSettings) super.setCallback(callback);
}
@Override
public UpdateGenerativeSettings setFields(java.lang.String fields) {
return (UpdateGenerativeSettings) super.setFields(fields);
}
@Override
public UpdateGenerativeSettings setKey(java.lang.String key) {
return (UpdateGenerativeSettings) super.setKey(key);
}
@Override
public UpdateGenerativeSettings setOauthToken(java.lang.String oauthToken) {
return (UpdateGenerativeSettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateGenerativeSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateGenerativeSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateGenerativeSettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateGenerativeSettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateGenerativeSettings setUploadType(java.lang.String uploadType) {
return (UpdateGenerativeSettings) super.setUploadType(uploadType);
}
@Override
public UpdateGenerativeSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateGenerativeSettings) super.setUploadProtocol(uploadProtocol);
}
/** Format: `projects//locations//agents//generativeSettings`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Format: `projects//locations//agents//generativeSettings`.
*/
public java.lang.String getName() {
return name;
}
/** Format: `projects//locations//agents//generativeSettings`. */
public UpdateGenerativeSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generativeSettings$");
}
this.name = name;
return this;
}
/**
* Optional. The mask to control which fields get updated. If the mask is not present, all
* fields will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Optional. The mask to control which fields get updated. If the mask is not present, all fields will
be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Optional. The mask to control which fields get updated. If the mask is not present, all
* fields will be updated.
*/
public UpdateGenerativeSettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateGenerativeSettings set(String parameterName, Object value) {
return (UpdateGenerativeSettings) super.set(parameterName, value);
}
}
/**
* Validates the specified agent and creates or updates validation results. The agent in draft
* version is validated. Please call this API after the training is completed to get the complete
* validation results.
*
* Create a request for the method "agents.validate".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Validate#execute()} method to invoke the remote operation.
*
* @param name Required. The agent to validate. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ValidateAgentRequest}
* @return the request
*/
public Validate validate(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ValidateAgentRequest content) throws java.io.IOException {
Validate result = new Validate(name, content);
initialize(result);
return result;
}
public class Validate extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:validate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Validates the specified agent and creates or updates validation results. The agent in draft
* version is validated. Please call this API after the training is completed to get the complete
* validation results.
*
* Create a request for the method "agents.validate".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Validate#execute()} method to invoke the remote operation.
* {@link
* Validate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The agent to validate. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ValidateAgentRequest}
* @since 1.13
*/
protected Validate(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ValidateAgentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3AgentValidationResult.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Validate set$Xgafv(java.lang.String $Xgafv) {
return (Validate) super.set$Xgafv($Xgafv);
}
@Override
public Validate setAccessToken(java.lang.String accessToken) {
return (Validate) super.setAccessToken(accessToken);
}
@Override
public Validate setAlt(java.lang.String alt) {
return (Validate) super.setAlt(alt);
}
@Override
public Validate setCallback(java.lang.String callback) {
return (Validate) super.setCallback(callback);
}
@Override
public Validate setFields(java.lang.String fields) {
return (Validate) super.setFields(fields);
}
@Override
public Validate setKey(java.lang.String key) {
return (Validate) super.setKey(key);
}
@Override
public Validate setOauthToken(java.lang.String oauthToken) {
return (Validate) super.setOauthToken(oauthToken);
}
@Override
public Validate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Validate) super.setPrettyPrint(prettyPrint);
}
@Override
public Validate setQuotaUser(java.lang.String quotaUser) {
return (Validate) super.setQuotaUser(quotaUser);
}
@Override
public Validate setUploadType(java.lang.String uploadType) {
return (Validate) super.setUploadType(uploadType);
}
@Override
public Validate setUploadProtocol(java.lang.String uploadProtocol) {
return (Validate) super.setUploadProtocol(uploadProtocol);
}
/** Required. The agent to validate. Format: `projects//locations//agents/`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The agent to validate. Format: `projects//locations//agents/`.
*/
public java.lang.String getName() {
return name;
}
/** Required. The agent to validate. Format: `projects//locations//agents/`. */
public Validate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Validate set(String parameterName, Object value) {
return (Validate) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Changelogs collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Changelogs.List request = dialogflow.changelogs().list(parameters ...)}
*
*
* @return the resource collection
*/
public Changelogs changelogs() {
return new Changelogs();
}
/**
* The "changelogs" collection of methods.
*/
public class Changelogs {
/**
* Retrieves the specified Changelog.
*
* Create a request for the method "changelogs.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the changelog to get. Format: `projects//locations//agents//changelogs/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/changelogs/[^/]+$");
/**
* Retrieves the specified Changelog.
*
* Create a request for the method "changelogs.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the changelog to get. Format: `projects//locations//agents//changelogs/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Changelog.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/changelogs/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the changelog to get. Format:
* `projects//locations//agents//changelogs/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the changelog to get. Format: `projects//locations//agents//changelogs/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the changelog to get. Format:
* `projects//locations//agents//changelogs/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/changelogs/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of Changelogs.
*
* Create a request for the method "changelogs.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent containing the changelogs. Format: `projects//locations//agents/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/changelogs";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Returns the list of Changelogs.
*
* Create a request for the method "changelogs.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent containing the changelogs. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListChangelogsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent containing the changelogs. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent containing the changelogs. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent containing the changelogs. Format:
* `projects//locations//agents/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The filter string. Supports filter by user_email, resource, type and create_time.
* Some examples: 1. By user email: user_email = "[email protected]" 2. By resource
* name: resource = "projects/123/locations/global/agents/456/flows/789" 3. By resource
* display name: display_name = "my agent" 4. By action: action = "Create" 5. By type:
* type = "flows" 6. By create time. Currently predicates on `create_time` and
* `create_time_epoch_seconds` are supported: create_time_epoch_seconds > 1551790877 AND
* create_time <= 2017-01-15T01:30:15.01Z 7. Combination of above filters: resource =
* "projects/123/locations/global/agents/456/flows/789" AND user_email =
* "[email protected]" AND create_time <= 2017-01-15T01:30:15.01Z
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** The filter string. Supports filter by user_email, resource, type and create_time. Some examples: 1.
By user email: user_email = "[email protected]" 2. By resource name: resource =
"projects/123/locations/global/agents/456/flows/789" 3. By resource display name: display_name =
"my agent" 4. By action: action = "Create" 5. By type: type = "flows" 6. By create time. Currently
predicates on `create_time` and `create_time_epoch_seconds` are supported:
create_time_epoch_seconds > 1551790877 AND create_time <= 2017-01-15T01:30:15.01Z 7. Combination of
above filters: resource = "projects/123/locations/global/agents/456/flows/789" AND user_email =
"[email protected]" AND create_time <= 2017-01-15T01:30:15.01Z
*/
public java.lang.String getFilter() {
return filter;
}
/**
* The filter string. Supports filter by user_email, resource, type and create_time.
* Some examples: 1. By user email: user_email = "[email protected]" 2. By resource
* name: resource = "projects/123/locations/global/agents/456/flows/789" 3. By resource
* display name: display_name = "my agent" 4. By action: action = "Create" 5. By type:
* type = "flows" 6. By create time. Currently predicates on `create_time` and
* `create_time_epoch_seconds` are supported: create_time_epoch_seconds > 1551790877 AND
* create_time <= 2017-01-15T01:30:15.01Z 7. Combination of above filters: resource =
* "projects/123/locations/global/agents/456/flows/789" AND user_email =
* "[email protected]" AND create_time <= 2017-01-15T01:30:15.01Z
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the EntityTypes collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.EntityTypes.List request = dialogflow.entityTypes().list(parameters ...)}
*
*
* @return the resource collection
*/
public EntityTypes entityTypes() {
return new EntityTypes();
}
/**
* The "entityTypes" collection of methods.
*/
public class EntityTypes {
/**
* Creates an entity type in the specified agent. Note: You should always train a flow prior to
* sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "entityTypes.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to create a entity type for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/entityTypes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Creates an entity type in the specified agent. Note: You should always train a flow prior to
* sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "entityTypes.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to create a entity type for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to create a entity type for. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to create a entity type for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to create a entity type for. Format:
* `projects//locations//agents/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language of the following fields in `entity_type`: * `EntityType.entities.value`
* * `EntityType.entities.synonyms` * `EntityType.excluded_phrases.value` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `entity_type`: * `EntityType.entities.value` *
`EntityType.entities.synonyms` * `EntityType.excluded_phrases.value` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `entity_type`: * `EntityType.entities.value`
* * `EntityType.entities.synonyms` * `EntityType.excluded_phrases.value` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Create setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified entity type. Note: You should always train a flow prior to sending it
* queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "entityTypes.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entity type to delete. Format:
* `projects//locations//agents//entityTypes/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/entityTypes/[^/]+$");
/**
* Deletes the specified entity type. Note: You should always train a flow prior to sending it
* queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "entityTypes.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the entity type to delete. Format:
* `projects//locations//agents//entityTypes/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/entityTypes/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the entity type to delete. Format:
* `projects//locations//agents//entityTypes/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entity type to delete. Format:
`projects//locations//agents//entityTypes/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the entity type to delete. Format:
* `projects//locations//agents//entityTypes/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/entityTypes/[^/]+$");
}
this.name = name;
return this;
}
/**
* This field has no effect for entity type not being used. For entity types that are
* used by intents or pages: * If `force` is set to false, an error will be returned
* with message indicating the referencing resources. * If `force` is set to true,
* Dialogflow will remove the entity type, as well as any references to the entity type
* (i.e. Page parameter of the entity type will be changed to '@sys.any' and intent
* parameter of the entity type will be removed).
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** This field has no effect for entity type not being used. For entity types that are used by intents
or pages: * If `force` is set to false, an error will be returned with message indicating the
referencing resources. * If `force` is set to true, Dialogflow will remove the entity type, as well
as any references to the entity type (i.e. Page parameter of the entity type will be changed to
'@sys.any' and intent parameter of the entity type will be removed).
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* This field has no effect for entity type not being used. For entity types that are
* used by intents or pages: * If `force` is set to false, an error will be returned
* with message indicating the referencing resources. * If `force` is set to true,
* Dialogflow will remove the entity type, as well as any references to the entity type
* (i.e. Page parameter of the entity type will be changed to '@sys.any' and intent
* parameter of the entity type will be removed).
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Exports the selected entity types.
*
* Create a request for the method "entityTypes.export".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the parent agent to export entity types. Format:
* `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportEntityTypesRequest}
* @return the request
*/
public Export export(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportEntityTypesRequest content) throws java.io.IOException {
Export result = new Export(parent, content);
initialize(result);
return result;
}
public class Export extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/entityTypes:export";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Exports the selected entity types.
*
* Create a request for the method "entityTypes.export".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Export#execute()} method to invoke the remote operation.
* {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the parent agent to export entity types. Format:
* `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportEntityTypesRequest}
* @since 1.13
*/
protected Export(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportEntityTypesRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the parent agent to export entity types. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the parent agent to export entity types. Format:
`projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the parent agent to export entity types. Format:
* `projects//locations//agents/`.
*/
public Export setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Retrieves the specified entity type.
*
* Create a request for the method "entityTypes.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the entity type. Format: `projects//locations//agents//entityTypes/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/entityTypes/[^/]+$");
/**
* Retrieves the specified entity type.
*
* Create a request for the method "entityTypes.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the entity type. Format: `projects//locations//agents//entityTypes/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/entityTypes/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the entity type. Format:
* `projects//locations//agents//entityTypes/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the entity type. Format: `projects//locations//agents//entityTypes/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the entity type. Format:
* `projects//locations//agents//entityTypes/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/entityTypes/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language to retrieve the entity type for. The following fields are language
* dependent: * `EntityType.entities.value` * `EntityType.entities.synonyms` *
* `EntityType.excluded_phrases.value` If not specified, the agent's default language is
* used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to retrieve the entity type for. The following fields are language dependent: *
`EntityType.entities.value` * `EntityType.entities.synonyms` * `EntityType.excluded_phrases.value`
If not specified, the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to retrieve the entity type for. The following fields are language
* dependent: * `EntityType.entities.value` * `EntityType.entities.synonyms` *
* `EntityType.excluded_phrases.value` If not specified, the agent's default language is
* used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Get setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Imports the specified entitytypes into the agent.
*
* Create a request for the method "entityTypes.import".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link DialogflowImport#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to import the entity types into. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportEntityTypesRequest}
* @return the request
*/
public DialogflowImport dialogflowImport(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportEntityTypesRequest content) throws java.io.IOException {
DialogflowImport result = new DialogflowImport(parent, content);
initialize(result);
return result;
}
public class DialogflowImport extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/entityTypes:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Imports the specified entitytypes into the agent.
*
* Create a request for the method "entityTypes.import".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link DialogflowImport#execute()} method to invoke the remote
* operation. {@link DialogflowImport#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The agent to import the entity types into. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportEntityTypesRequest}
* @since 1.13
*/
protected DialogflowImport(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportEntityTypesRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public DialogflowImport set$Xgafv(java.lang.String $Xgafv) {
return (DialogflowImport) super.set$Xgafv($Xgafv);
}
@Override
public DialogflowImport setAccessToken(java.lang.String accessToken) {
return (DialogflowImport) super.setAccessToken(accessToken);
}
@Override
public DialogflowImport setAlt(java.lang.String alt) {
return (DialogflowImport) super.setAlt(alt);
}
@Override
public DialogflowImport setCallback(java.lang.String callback) {
return (DialogflowImport) super.setCallback(callback);
}
@Override
public DialogflowImport setFields(java.lang.String fields) {
return (DialogflowImport) super.setFields(fields);
}
@Override
public DialogflowImport setKey(java.lang.String key) {
return (DialogflowImport) super.setKey(key);
}
@Override
public DialogflowImport setOauthToken(java.lang.String oauthToken) {
return (DialogflowImport) super.setOauthToken(oauthToken);
}
@Override
public DialogflowImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DialogflowImport) super.setPrettyPrint(prettyPrint);
}
@Override
public DialogflowImport setQuotaUser(java.lang.String quotaUser) {
return (DialogflowImport) super.setQuotaUser(quotaUser);
}
@Override
public DialogflowImport setUploadType(java.lang.String uploadType) {
return (DialogflowImport) super.setUploadType(uploadType);
}
@Override
public DialogflowImport setUploadProtocol(java.lang.String uploadProtocol) {
return (DialogflowImport) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to import the entity types into. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to import the entity types into. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to import the entity types into. Format:
* `projects//locations//agents/`.
*/
public DialogflowImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public DialogflowImport set(String parameterName, Object value) {
return (DialogflowImport) super.set(parameterName, value);
}
}
/**
* Returns the list of all entity types in the specified agent.
*
* Create a request for the method "entityTypes.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to list all entity types for. Format: `projects//locations//agents/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/entityTypes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Returns the list of all entity types in the specified agent.
*
* Create a request for the method "entityTypes.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to list all entity types for. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListEntityTypesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to list all entity types for. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to list all entity types for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to list all entity types for. Format:
* `projects//locations//agents/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language to list entity types for. The following fields are language dependent: *
* `EntityType.entities.value` * `EntityType.entities.synonyms` *
* `EntityType.excluded_phrases.value` If not specified, the agent's default language is
* used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to list entity types for. The following fields are language dependent: *
`EntityType.entities.value` * `EntityType.entities.synonyms` * `EntityType.excluded_phrases.value`
If not specified, the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to list entity types for. The following fields are language dependent: *
* `EntityType.entities.value` * `EntityType.entities.synonyms` *
* `EntityType.excluded_phrases.value` If not specified, the agent's default language is
* used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified entity type. Note: You should always train a flow prior to sending it
* queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "entityTypes.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the entity type. Required for EntityTypes.UpdateEntityType. Format:
* `projects//locations//agents//entityTypes/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/entityTypes/[^/]+$");
/**
* Updates the specified entity type. Note: You should always train a flow prior to sending it
* queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "entityTypes.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the entity type. Required for EntityTypes.UpdateEntityType. Format:
* `projects//locations//agents//entityTypes/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3EntityType.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/entityTypes/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the entity type. Required for EntityTypes.UpdateEntityType.
* Format: `projects//locations//agents//entityTypes/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the entity type. Required for EntityTypes.UpdateEntityType. Format:
`projects//locations//agents//entityTypes/`.
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the entity type. Required for EntityTypes.UpdateEntityType.
* Format: `projects//locations//agents//entityTypes/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/entityTypes/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language of the following fields in `entity_type`: * `EntityType.entities.value`
* * `EntityType.entities.synonyms` * `EntityType.excluded_phrases.value` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `entity_type`: * `EntityType.entities.value` *
`EntityType.entities.synonyms` * `EntityType.excluded_phrases.value` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `entity_type`: * `EntityType.entities.value`
* * `EntityType.entities.synonyms` * `EntityType.excluded_phrases.value` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Patch setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/** The mask to control which fields get updated. */
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** The mask to control which fields get updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Environments collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Environments.List request = dialogflow.environments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Environments environments() {
return new Environments();
}
/**
* The "environments" collection of methods.
*/
public class Environments {
/**
* Creates an Environment in the specified Agent. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: An empty [Struct
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#struct) -
* `response`: Environment
*
* Create a request for the method "environments.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The Agent to create an Environment for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Environment}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Environment content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/environments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Creates an Environment in the specified Agent. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`: An empty
* [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: Environment
*
* Create a request for the method "environments.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Agent to create an Environment for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Environment}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Environment content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Agent to create an Environment for. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Agent to create an Environment for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Agent to create an Environment for. Format:
* `projects//locations//agents/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified Environment.
*
* Create a request for the method "environments.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Environment to delete. Format:
* `projects//locations//agents//environments/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Deletes the specified Environment.
*
* Create a request for the method "environments.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Environment to delete. Format:
* `projects//locations//agents//environments/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Environment to delete. Format:
* `projects//locations//agents//environments/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Environment to delete. Format:
`projects//locations//agents//environments/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Environment to delete. Format:
* `projects//locations//agents//environments/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Deploys a flow to the specified Environment. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: DeployFlowMetadata -
* `response`: DeployFlowResponse
*
* Create a request for the method "environments.deployFlow".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link DeployFlow#execute()} method to invoke the remote operation.
*
* @param environment Required. The environment to deploy the flow to. Format: `projects//locations//agents//
* environments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DeployFlowRequest}
* @return the request
*/
public DeployFlow deployFlow(java.lang.String environment, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DeployFlowRequest content) throws java.io.IOException {
DeployFlow result = new DeployFlow(environment, content);
initialize(result);
return result;
}
public class DeployFlow extends DialogflowRequest {
private static final String REST_PATH = "v3/{+environment}:deployFlow";
private final java.util.regex.Pattern ENVIRONMENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Deploys a flow to the specified Environment. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`:
* DeployFlowMetadata - `response`: DeployFlowResponse
*
* Create a request for the method "environments.deployFlow".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link DeployFlow#execute()} method to invoke the remote
* operation. {@link
* DeployFlow#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param environment Required. The environment to deploy the flow to. Format: `projects//locations//agents//
* environments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DeployFlowRequest}
* @since 1.13
*/
protected DeployFlow(java.lang.String environment, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DeployFlowRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.environment = com.google.api.client.util.Preconditions.checkNotNull(environment, "Required parameter environment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ENVIRONMENT_PATTERN.matcher(environment).matches(),
"Parameter environment must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public DeployFlow set$Xgafv(java.lang.String $Xgafv) {
return (DeployFlow) super.set$Xgafv($Xgafv);
}
@Override
public DeployFlow setAccessToken(java.lang.String accessToken) {
return (DeployFlow) super.setAccessToken(accessToken);
}
@Override
public DeployFlow setAlt(java.lang.String alt) {
return (DeployFlow) super.setAlt(alt);
}
@Override
public DeployFlow setCallback(java.lang.String callback) {
return (DeployFlow) super.setCallback(callback);
}
@Override
public DeployFlow setFields(java.lang.String fields) {
return (DeployFlow) super.setFields(fields);
}
@Override
public DeployFlow setKey(java.lang.String key) {
return (DeployFlow) super.setKey(key);
}
@Override
public DeployFlow setOauthToken(java.lang.String oauthToken) {
return (DeployFlow) super.setOauthToken(oauthToken);
}
@Override
public DeployFlow setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DeployFlow) super.setPrettyPrint(prettyPrint);
}
@Override
public DeployFlow setQuotaUser(java.lang.String quotaUser) {
return (DeployFlow) super.setQuotaUser(quotaUser);
}
@Override
public DeployFlow setUploadType(java.lang.String uploadType) {
return (DeployFlow) super.setUploadType(uploadType);
}
@Override
public DeployFlow setUploadProtocol(java.lang.String uploadProtocol) {
return (DeployFlow) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The environment to deploy the flow to. Format:
* `projects//locations//agents// environments/`.
*/
@com.google.api.client.util.Key
private java.lang.String environment;
/** Required. The environment to deploy the flow to. Format: `projects//locations//agents//
environments/`.
*/
public java.lang.String getEnvironment() {
return environment;
}
/**
* Required. The environment to deploy the flow to. Format:
* `projects//locations//agents// environments/`.
*/
public DeployFlow setEnvironment(java.lang.String environment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ENVIRONMENT_PATTERN.matcher(environment).matches(),
"Parameter environment must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.environment = environment;
return this;
}
@Override
public DeployFlow set(String parameterName, Object value) {
return (DeployFlow) super.set(parameterName, value);
}
}
/**
* Retrieves the specified Environment.
*
* Create a request for the method "environments.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Environment. Format: `projects//locations//agents//environments/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Retrieves the specified Environment.
*
* Create a request for the method "environments.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Environment. Format: `projects//locations//agents//environments/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Environment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Environment. Format:
* `projects//locations//agents//environments/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Environment. Format: `projects//locations//agents//environments/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Environment. Format:
* `projects//locations//agents//environments/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all environments in the specified Agent.
*
* Create a request for the method "environments.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The Agent to list all environments for. Format: `projects//locations//agents/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/environments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Returns the list of all environments in the specified Agent.
*
* Create a request for the method "environments.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Agent to list all environments for. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListEnvironmentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Agent to list all environments for. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Agent to list all environments for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Agent to list all environments for. Format:
* `projects//locations//agents/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most
* 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 20 and at most 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most
* 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Looks up the history of the specified Environment.
*
* Create a request for the method "environments.lookupEnvironmentHistory".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link LookupEnvironmentHistory#execute()} method to invoke the remote
* operation.
*
* @param name Required. Resource name of the environment to look up the history for. Format:
* `projects//locations//agents//environments/`.
* @return the request
*/
public LookupEnvironmentHistory lookupEnvironmentHistory(java.lang.String name) throws java.io.IOException {
LookupEnvironmentHistory result = new LookupEnvironmentHistory(name);
initialize(result);
return result;
}
public class LookupEnvironmentHistory extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:lookupEnvironmentHistory";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Looks up the history of the specified Environment.
*
* Create a request for the method "environments.lookupEnvironmentHistory".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link LookupEnvironmentHistory#execute()} method to invoke the
* remote operation. {@link LookupEnvironmentHistory#initialize(com.google.api.client.googleap
* is.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param name Required. Resource name of the environment to look up the history for. Format:
* `projects//locations//agents//environments/`.
* @since 1.13
*/
protected LookupEnvironmentHistory(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3LookupEnvironmentHistoryResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public LookupEnvironmentHistory set$Xgafv(java.lang.String $Xgafv) {
return (LookupEnvironmentHistory) super.set$Xgafv($Xgafv);
}
@Override
public LookupEnvironmentHistory setAccessToken(java.lang.String accessToken) {
return (LookupEnvironmentHistory) super.setAccessToken(accessToken);
}
@Override
public LookupEnvironmentHistory setAlt(java.lang.String alt) {
return (LookupEnvironmentHistory) super.setAlt(alt);
}
@Override
public LookupEnvironmentHistory setCallback(java.lang.String callback) {
return (LookupEnvironmentHistory) super.setCallback(callback);
}
@Override
public LookupEnvironmentHistory setFields(java.lang.String fields) {
return (LookupEnvironmentHistory) super.setFields(fields);
}
@Override
public LookupEnvironmentHistory setKey(java.lang.String key) {
return (LookupEnvironmentHistory) super.setKey(key);
}
@Override
public LookupEnvironmentHistory setOauthToken(java.lang.String oauthToken) {
return (LookupEnvironmentHistory) super.setOauthToken(oauthToken);
}
@Override
public LookupEnvironmentHistory setPrettyPrint(java.lang.Boolean prettyPrint) {
return (LookupEnvironmentHistory) super.setPrettyPrint(prettyPrint);
}
@Override
public LookupEnvironmentHistory setQuotaUser(java.lang.String quotaUser) {
return (LookupEnvironmentHistory) super.setQuotaUser(quotaUser);
}
@Override
public LookupEnvironmentHistory setUploadType(java.lang.String uploadType) {
return (LookupEnvironmentHistory) super.setUploadType(uploadType);
}
@Override
public LookupEnvironmentHistory setUploadProtocol(java.lang.String uploadProtocol) {
return (LookupEnvironmentHistory) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the environment to look up the history for. Format:
* `projects//locations//agents//environments/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the environment to look up the history for. Format:
`projects//locations//agents//environments/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the environment to look up the history for. Format:
* `projects//locations//agents//environments/`.
*/
public LookupEnvironmentHistory setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.name = name;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public LookupEnvironmentHistory setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public LookupEnvironmentHistory setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public LookupEnvironmentHistory set(String parameterName, Object value) {
return (LookupEnvironmentHistory) super.set(parameterName, value);
}
}
/**
* Updates the specified Environment. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: An empty [Struct
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#struct) -
* `response`: Environment
*
* Create a request for the method "environments.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The name of the environment. Format: `projects//locations//agents//environments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Environment}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Environment content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Updates the specified Environment. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`: An empty
* [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: Environment
*
* Create a request for the method "environments.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the environment. Format: `projects//locations//agents//environments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Environment}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Environment content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the environment. Format: `projects//locations//agents//environments/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the environment. Format: `projects//locations//agents//environments/`.
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the environment. Format: `projects//locations//agents//environments/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.name = name;
return this;
}
/** Required. The mask to control which fields get updated. */
@com.google.api.client.util.Key
private String updateMask;
/** Required. The mask to control which fields get updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** Required. The mask to control which fields get updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Kicks off a continuous test under the specified Environment. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`:
* RunContinuousTestMetadata - `response`: RunContinuousTestResponse
*
* Create a request for the method "environments.runContinuousTest".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link RunContinuousTest#execute()} method to invoke the remote operation.
*
* @param environment Required. Format: `projects//locations//agents//environments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RunContinuousTestRequest}
* @return the request
*/
public RunContinuousTest runContinuousTest(java.lang.String environment, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RunContinuousTestRequest content) throws java.io.IOException {
RunContinuousTest result = new RunContinuousTest(environment, content);
initialize(result);
return result;
}
public class RunContinuousTest extends DialogflowRequest {
private static final String REST_PATH = "v3/{+environment}:runContinuousTest";
private final java.util.regex.Pattern ENVIRONMENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Kicks off a continuous test under the specified Environment. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`:
* RunContinuousTestMetadata - `response`: RunContinuousTestResponse
*
* Create a request for the method "environments.runContinuousTest".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link RunContinuousTest#execute()} method to invoke the remote
* operation. {@link RunContinuousTest#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param environment Required. Format: `projects//locations//agents//environments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RunContinuousTestRequest}
* @since 1.13
*/
protected RunContinuousTest(java.lang.String environment, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RunContinuousTestRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.environment = com.google.api.client.util.Preconditions.checkNotNull(environment, "Required parameter environment must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ENVIRONMENT_PATTERN.matcher(environment).matches(),
"Parameter environment must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public RunContinuousTest set$Xgafv(java.lang.String $Xgafv) {
return (RunContinuousTest) super.set$Xgafv($Xgafv);
}
@Override
public RunContinuousTest setAccessToken(java.lang.String accessToken) {
return (RunContinuousTest) super.setAccessToken(accessToken);
}
@Override
public RunContinuousTest setAlt(java.lang.String alt) {
return (RunContinuousTest) super.setAlt(alt);
}
@Override
public RunContinuousTest setCallback(java.lang.String callback) {
return (RunContinuousTest) super.setCallback(callback);
}
@Override
public RunContinuousTest setFields(java.lang.String fields) {
return (RunContinuousTest) super.setFields(fields);
}
@Override
public RunContinuousTest setKey(java.lang.String key) {
return (RunContinuousTest) super.setKey(key);
}
@Override
public RunContinuousTest setOauthToken(java.lang.String oauthToken) {
return (RunContinuousTest) super.setOauthToken(oauthToken);
}
@Override
public RunContinuousTest setPrettyPrint(java.lang.Boolean prettyPrint) {
return (RunContinuousTest) super.setPrettyPrint(prettyPrint);
}
@Override
public RunContinuousTest setQuotaUser(java.lang.String quotaUser) {
return (RunContinuousTest) super.setQuotaUser(quotaUser);
}
@Override
public RunContinuousTest setUploadType(java.lang.String uploadType) {
return (RunContinuousTest) super.setUploadType(uploadType);
}
@Override
public RunContinuousTest setUploadProtocol(java.lang.String uploadProtocol) {
return (RunContinuousTest) super.setUploadProtocol(uploadProtocol);
}
/** Required. Format: `projects//locations//agents//environments/`. */
@com.google.api.client.util.Key
private java.lang.String environment;
/** Required. Format: `projects//locations//agents//environments/`.
*/
public java.lang.String getEnvironment() {
return environment;
}
/** Required. Format: `projects//locations//agents//environments/`. */
public RunContinuousTest setEnvironment(java.lang.String environment) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(ENVIRONMENT_PATTERN.matcher(environment).matches(),
"Parameter environment must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.environment = environment;
return this;
}
@Override
public RunContinuousTest set(String parameterName, Object value) {
return (RunContinuousTest) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ContinuousTestResults collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.ContinuousTestResults.List request = dialogflow.continuousTestResults().list(parameters ...)}
*
*
* @return the resource collection
*/
public ContinuousTestResults continuousTestResults() {
return new ContinuousTestResults();
}
/**
* The "continuousTestResults" collection of methods.
*/
public class ContinuousTestResults {
/**
* Fetches a list of continuous test results for a given environment.
*
* Create a request for the method "continuousTestResults.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The environment to list results for. Format: `projects//locations//agents//
* environments/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/continuousTestResults";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Fetches a list of continuous test results for a given environment.
*
* Create a request for the method "continuousTestResults.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The environment to list results for. Format: `projects//locations//agents//
* environments/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListContinuousTestResultsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The environment to list results for. Format:
* `projects//locations//agents// environments/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The environment to list results for. Format: `projects//locations//agents//
environments/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The environment to list results for. Format:
* `projects//locations//agents// environments/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Deployments collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Deployments.List request = dialogflow.deployments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Deployments deployments() {
return new Deployments();
}
/**
* The "deployments" collection of methods.
*/
public class Deployments {
/**
* Retrieves the specified Deployment.
*
* Create a request for the method "deployments.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Deployment. Format:
* `projects//locations//agents//environments//deployments/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/deployments/[^/]+$");
/**
* Retrieves the specified Deployment.
*
* Create a request for the method "deployments.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Deployment. Format:
* `projects//locations//agents//environments//deployments/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Deployment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/deployments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Deployment. Format:
* `projects//locations//agents//environments//deployments/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Deployment. Format:
`projects//locations//agents//environments//deployments/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Deployment. Format:
* `projects//locations//agents//environments//deployments/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/deployments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all deployments in the specified Environment.
*
* Create a request for the method "deployments.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The Environment to list all environments for. Format:
* `projects//locations//agents//environments/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/deployments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Returns the list of all deployments in the specified Environment.
*
* Create a request for the method "deployments.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Environment to list all environments for. Format:
* `projects//locations//agents//environments/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListDeploymentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Environment to list all environments for. Format:
* `projects//locations//agents//environments/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Environment to list all environments for. Format:
`projects//locations//agents//environments/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Environment to list all environments for. Format:
* `projects//locations//agents//environments/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most
* 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 20 and at most 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most
* 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Experiments collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Experiments.List request = dialogflow.experiments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Experiments experiments() {
return new Experiments();
}
/**
* The "experiments" collection of methods.
*/
public class Experiments {
/**
* Creates an Experiment in the specified Environment.
*
* Create a request for the method "experiments.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The Agent to create an Environment for. Format:
* `projects//locations//agents//environments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/experiments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Creates an Experiment in the specified Environment.
*
* Create a request for the method "experiments.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Agent to create an Environment for. Format:
* `projects//locations//agents//environments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Agent to create an Environment for. Format:
* `projects//locations//agents//environments/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Agent to create an Environment for. Format:
`projects//locations//agents//environments/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Agent to create an Environment for. Format:
* `projects//locations//agents//environments/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified Experiment.
*
* Create a request for the method "experiments.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Environment to delete. Format:
* `projects//locations//agents//environments//experiments/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
/**
* Deletes the specified Experiment.
*
* Create a request for the method "experiments.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Environment to delete. Format:
* `projects//locations//agents//environments//experiments/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Environment to delete. Format:
* `projects//locations//agents//environments//experiments/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Environment to delete. Format:
`projects//locations//agents//environments//experiments/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Environment to delete. Format:
* `projects//locations//agents//environments//experiments/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified Experiment.
*
* Create a request for the method "experiments.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Environment. Format:
* `projects//locations//agents//environments//experiments/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
/**
* Retrieves the specified Experiment.
*
* Create a request for the method "experiments.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Environment. Format:
* `projects//locations//agents//environments//experiments/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Environment. Format:
* `projects//locations//agents//environments//experiments/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Environment. Format:
`projects//locations//agents//environments//experiments/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Environment. Format:
* `projects//locations//agents//environments//experiments/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all experiments in the specified Environment.
*
* Create a request for the method "experiments.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The Environment to list all environments for. Format:
* `projects//locations//agents//environments/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/experiments";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
/**
* Returns the list of all experiments in the specified Environment.
*
* Create a request for the method "experiments.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Environment to list all environments for. Format:
* `projects//locations//agents//environments/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListExperimentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Environment to list all environments for. Format:
* `projects//locations//agents//environments/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Environment to list all environments for. Format:
`projects//locations//agents//environments/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Environment to list all environments for. Format:
* `projects//locations//agents//environments/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most
* 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 20 and at most 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most
* 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified Experiment.
*
* Create a request for the method "experiments.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The name of the experiment. Format: projects//locations//agents//environments//experiments/..
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
/**
* Updates the specified Experiment.
*
* Create a request for the method "experiments.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the experiment. Format: projects//locations//agents//environments//experiments/..
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the experiment. Format:
* projects//locations//agents//environments//experiments/..
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the experiment. Format: projects//locations//agents//environments//experiments/..
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the experiment. Format:
* projects//locations//agents//environments//experiments/..
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
this.name = name;
return this;
}
/** Required. The mask to control which fields get updated. */
@com.google.api.client.util.Key
private String updateMask;
/** Required. The mask to control which fields get updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** Required. The mask to control which fields get updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Starts the specified Experiment. This rpc only changes the state of experiment from PENDING to
* RUNNING.
*
* Create a request for the method "experiments.start".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Start#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the experiment to start. Format:
* `projects//locations//agents//environments//experiments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3StartExperimentRequest}
* @return the request
*/
public Start start(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3StartExperimentRequest content) throws java.io.IOException {
Start result = new Start(name, content);
initialize(result);
return result;
}
public class Start extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:start";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
/**
* Starts the specified Experiment. This rpc only changes the state of experiment from PENDING to
* RUNNING.
*
* Create a request for the method "experiments.start".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Start#execute()} method to invoke the remote operation.
* {@link
* Start#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the experiment to start. Format:
* `projects//locations//agents//environments//experiments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3StartExperimentRequest}
* @since 1.13
*/
protected Start(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3StartExperimentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
}
@Override
public Start set$Xgafv(java.lang.String $Xgafv) {
return (Start) super.set$Xgafv($Xgafv);
}
@Override
public Start setAccessToken(java.lang.String accessToken) {
return (Start) super.setAccessToken(accessToken);
}
@Override
public Start setAlt(java.lang.String alt) {
return (Start) super.setAlt(alt);
}
@Override
public Start setCallback(java.lang.String callback) {
return (Start) super.setCallback(callback);
}
@Override
public Start setFields(java.lang.String fields) {
return (Start) super.setFields(fields);
}
@Override
public Start setKey(java.lang.String key) {
return (Start) super.setKey(key);
}
@Override
public Start setOauthToken(java.lang.String oauthToken) {
return (Start) super.setOauthToken(oauthToken);
}
@Override
public Start setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Start) super.setPrettyPrint(prettyPrint);
}
@Override
public Start setQuotaUser(java.lang.String quotaUser) {
return (Start) super.setQuotaUser(quotaUser);
}
@Override
public Start setUploadType(java.lang.String uploadType) {
return (Start) super.setUploadType(uploadType);
}
@Override
public Start setUploadProtocol(java.lang.String uploadProtocol) {
return (Start) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the experiment to start. Format:
* `projects//locations//agents//environments//experiments/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the experiment to start. Format:
`projects//locations//agents//environments//experiments/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the experiment to start. Format:
* `projects//locations//agents//environments//experiments/`.
*/
public Start setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Start set(String parameterName, Object value) {
return (Start) super.set(parameterName, value);
}
}
/**
* Stops the specified Experiment. This rpc only changes the state of experiment from RUNNING to
* DONE.
*
* Create a request for the method "experiments.stop".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Stop#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the experiment to stop. Format:
* `projects//locations//agents//environments//experiments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3StopExperimentRequest}
* @return the request
*/
public Stop stop(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3StopExperimentRequest content) throws java.io.IOException {
Stop result = new Stop(name, content);
initialize(result);
return result;
}
public class Stop extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:stop";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
/**
* Stops the specified Experiment. This rpc only changes the state of experiment from RUNNING to
* DONE.
*
* Create a request for the method "experiments.stop".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Stop#execute()} method to invoke the remote operation.
* {@link Stop#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the experiment to stop. Format:
* `projects//locations//agents//environments//experiments/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3StopExperimentRequest}
* @since 1.13
*/
protected Stop(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3StopExperimentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
}
@Override
public Stop set$Xgafv(java.lang.String $Xgafv) {
return (Stop) super.set$Xgafv($Xgafv);
}
@Override
public Stop setAccessToken(java.lang.String accessToken) {
return (Stop) super.setAccessToken(accessToken);
}
@Override
public Stop setAlt(java.lang.String alt) {
return (Stop) super.setAlt(alt);
}
@Override
public Stop setCallback(java.lang.String callback) {
return (Stop) super.setCallback(callback);
}
@Override
public Stop setFields(java.lang.String fields) {
return (Stop) super.setFields(fields);
}
@Override
public Stop setKey(java.lang.String key) {
return (Stop) super.setKey(key);
}
@Override
public Stop setOauthToken(java.lang.String oauthToken) {
return (Stop) super.setOauthToken(oauthToken);
}
@Override
public Stop setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Stop) super.setPrettyPrint(prettyPrint);
}
@Override
public Stop setQuotaUser(java.lang.String quotaUser) {
return (Stop) super.setQuotaUser(quotaUser);
}
@Override
public Stop setUploadType(java.lang.String uploadType) {
return (Stop) super.setUploadType(uploadType);
}
@Override
public Stop setUploadProtocol(java.lang.String uploadProtocol) {
return (Stop) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the experiment to stop. Format:
* `projects//locations//agents//environments//experiments/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the experiment to stop. Format:
`projects//locations//agents//environments//experiments/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the experiment to stop. Format:
* `projects//locations//agents//environments//experiments/`.
*/
public Stop setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/experiments/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Stop set(String parameterName, Object value) {
return (Stop) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Sessions collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Sessions.List request = dialogflow.sessions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Sessions sessions() {
return new Sessions();
}
/**
* The "sessions" collection of methods.
*/
public class Sessions {
/**
* Processes a natural language query and returns structured, actionable data as a result. This
* method is not idempotent, because it may cause session entity types to be updated, which in turn
* might affect results of future queries. Note: Always use agent versions for production traffic.
* See [Versions and environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*
* Create a request for the method "sessions.detectIntent".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link DetectIntent#execute()} method to invoke the remote operation.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest}
* @return the request
*/
public DetectIntent detectIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest content) throws java.io.IOException {
DetectIntent result = new DetectIntent(session, content);
initialize(result);
return result;
}
public class DetectIntent extends DialogflowRequest {
private static final String REST_PATH = "v3/{+session}:detectIntent";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
/**
* Processes a natural language query and returns structured, actionable data as a result. This
* method is not idempotent, because it may cause session entity types to be updated, which in
* turn might affect results of future queries. Note: Always use agent versions for production
* traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*
* Create a request for the method "sessions.detectIntent".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link DetectIntent#execute()} method to invoke the remote
* operation. {@link
* DetectIntent#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest}
* @since 1.13
*/
protected DetectIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
}
@Override
public DetectIntent set$Xgafv(java.lang.String $Xgafv) {
return (DetectIntent) super.set$Xgafv($Xgafv);
}
@Override
public DetectIntent setAccessToken(java.lang.String accessToken) {
return (DetectIntent) super.setAccessToken(accessToken);
}
@Override
public DetectIntent setAlt(java.lang.String alt) {
return (DetectIntent) super.setAlt(alt);
}
@Override
public DetectIntent setCallback(java.lang.String callback) {
return (DetectIntent) super.setCallback(callback);
}
@Override
public DetectIntent setFields(java.lang.String fields) {
return (DetectIntent) super.setFields(fields);
}
@Override
public DetectIntent setKey(java.lang.String key) {
return (DetectIntent) super.setKey(key);
}
@Override
public DetectIntent setOauthToken(java.lang.String oauthToken) {
return (DetectIntent) super.setOauthToken(oauthToken);
}
@Override
public DetectIntent setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DetectIntent) super.setPrettyPrint(prettyPrint);
}
@Override
public DetectIntent setQuotaUser(java.lang.String quotaUser) {
return (DetectIntent) super.setQuotaUser(quotaUser);
}
@Override
public DetectIntent setUploadType(java.lang.String uploadType) {
return (DetectIntent) super.setUploadType(uploadType);
}
@Override
public DetectIntent setUploadProtocol(java.lang.String uploadProtocol) {
return (DetectIntent) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to
* choose an appropriate `Session ID`. It can be a random number or some type of
* session identifiers (preferably hashed). The length of the `Session ID` must not
* exceed 36 characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always
* use agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The name of the session this query is sent to. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment. It's up to the API
caller to choose an appropriate `Session ID`. It can be a random number or some type of session
identifiers (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
more information, see the [sessions
guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use agent
versions for production traffic. See [Versions and
environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
public java.lang.String getSession() {
return session;
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to
* choose an appropriate `Session ID`. It can be a random number or some type of
* session identifiers (preferably hashed). The length of the `Session ID` must not
* exceed 36 characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always
* use agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
public DetectIntent setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public DetectIntent set(String parameterName, Object value) {
return (DetectIntent) super.set(parameterName, value);
}
}
/**
* Fulfills a matched intent returned by MatchIntent. Must be called after MatchIntent, with input
* from MatchIntentResponse. Otherwise, the behavior is undefined.
*
* Create a request for the method "sessions.fulfillIntent".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link FulfillIntent#execute()} method to invoke the remote operation.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentRequest}
* @return the request
*/
public FulfillIntent fulfillIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentRequest content) throws java.io.IOException {
FulfillIntent result = new FulfillIntent(session, content);
initialize(result);
return result;
}
public class FulfillIntent extends DialogflowRequest {
private static final String REST_PATH = "v3/{+session}:fulfillIntent";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
/**
* Fulfills a matched intent returned by MatchIntent. Must be called after MatchIntent, with input
* from MatchIntentResponse. Otherwise, the behavior is undefined.
*
* Create a request for the method "sessions.fulfillIntent".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link FulfillIntent#execute()} method to invoke the remote
* operation. {@link FulfillIntent#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentRequest}
* @since 1.13
*/
protected FulfillIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
}
@Override
public FulfillIntent set$Xgafv(java.lang.String $Xgafv) {
return (FulfillIntent) super.set$Xgafv($Xgafv);
}
@Override
public FulfillIntent setAccessToken(java.lang.String accessToken) {
return (FulfillIntent) super.setAccessToken(accessToken);
}
@Override
public FulfillIntent setAlt(java.lang.String alt) {
return (FulfillIntent) super.setAlt(alt);
}
@Override
public FulfillIntent setCallback(java.lang.String callback) {
return (FulfillIntent) super.setCallback(callback);
}
@Override
public FulfillIntent setFields(java.lang.String fields) {
return (FulfillIntent) super.setFields(fields);
}
@Override
public FulfillIntent setKey(java.lang.String key) {
return (FulfillIntent) super.setKey(key);
}
@Override
public FulfillIntent setOauthToken(java.lang.String oauthToken) {
return (FulfillIntent) super.setOauthToken(oauthToken);
}
@Override
public FulfillIntent setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FulfillIntent) super.setPrettyPrint(prettyPrint);
}
@Override
public FulfillIntent setQuotaUser(java.lang.String quotaUser) {
return (FulfillIntent) super.setQuotaUser(quotaUser);
}
@Override
public FulfillIntent setUploadType(java.lang.String uploadType) {
return (FulfillIntent) super.setUploadType(uploadType);
}
@Override
public FulfillIntent setUploadProtocol(java.lang.String uploadProtocol) {
return (FulfillIntent) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to
* choose an appropriate `Session ID`. It can be a random number or some type of
* session identifiers (preferably hashed). The length of the `Session ID` must not
* exceed 36 characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The name of the session this query is sent to. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment. It's up to the API
caller to choose an appropriate `Session ID`. It can be a random number or some type of session
identifiers (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
more information, see the [sessions
guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
public java.lang.String getSession() {
return session;
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to
* choose an appropriate `Session ID`. It can be a random number or some type of
* session identifiers (preferably hashed). The length of the `Session ID` must not
* exceed 36 characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
public FulfillIntent setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public FulfillIntent set(String parameterName, Object value) {
return (FulfillIntent) super.set(parameterName, value);
}
}
/**
* Returns preliminary intent match results, doesn't change the session status.
*
* Create a request for the method "sessions.matchIntent".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link MatchIntent#execute()} method to invoke the remote operation.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentRequest}
* @return the request
*/
public MatchIntent matchIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentRequest content) throws java.io.IOException {
MatchIntent result = new MatchIntent(session, content);
initialize(result);
return result;
}
public class MatchIntent extends DialogflowRequest {
private static final String REST_PATH = "v3/{+session}:matchIntent";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
/**
* Returns preliminary intent match results, doesn't change the session status.
*
* Create a request for the method "sessions.matchIntent".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link MatchIntent#execute()} method to invoke the remote
* operation. {@link
* MatchIntent#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentRequest}
* @since 1.13
*/
protected MatchIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
}
@Override
public MatchIntent set$Xgafv(java.lang.String $Xgafv) {
return (MatchIntent) super.set$Xgafv($Xgafv);
}
@Override
public MatchIntent setAccessToken(java.lang.String accessToken) {
return (MatchIntent) super.setAccessToken(accessToken);
}
@Override
public MatchIntent setAlt(java.lang.String alt) {
return (MatchIntent) super.setAlt(alt);
}
@Override
public MatchIntent setCallback(java.lang.String callback) {
return (MatchIntent) super.setCallback(callback);
}
@Override
public MatchIntent setFields(java.lang.String fields) {
return (MatchIntent) super.setFields(fields);
}
@Override
public MatchIntent setKey(java.lang.String key) {
return (MatchIntent) super.setKey(key);
}
@Override
public MatchIntent setOauthToken(java.lang.String oauthToken) {
return (MatchIntent) super.setOauthToken(oauthToken);
}
@Override
public MatchIntent setPrettyPrint(java.lang.Boolean prettyPrint) {
return (MatchIntent) super.setPrettyPrint(prettyPrint);
}
@Override
public MatchIntent setQuotaUser(java.lang.String quotaUser) {
return (MatchIntent) super.setQuotaUser(quotaUser);
}
@Override
public MatchIntent setUploadType(java.lang.String uploadType) {
return (MatchIntent) super.setUploadType(uploadType);
}
@Override
public MatchIntent setUploadProtocol(java.lang.String uploadProtocol) {
return (MatchIntent) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to
* choose an appropriate `Session ID`. It can be a random number or some type of
* session identifiers (preferably hashed). The length of the `Session ID` must not
* exceed 36 characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The name of the session this query is sent to. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment. It's up to the API
caller to choose an appropriate `Session ID`. It can be a random number or some type of session
identifiers (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
more information, see the [sessions
guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
public java.lang.String getSession() {
return session;
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to
* choose an appropriate `Session ID`. It can be a random number or some type of
* session identifiers (preferably hashed). The length of the `Session ID` must not
* exceed 36 characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
public MatchIntent setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public MatchIntent set(String parameterName, Object value) {
return (MatchIntent) super.set(parameterName, value);
}
}
/**
* Processes a natural language query and returns structured, actionable data as a result through
* server-side streaming. Server-side streaming allows Dialogflow to send [partial
* responses](https://cloud.google.com/dialogflow/cx/docs/concept/fulfillment#partial-response)
* earlier in a single request.
*
* Create a request for the method "sessions.serverStreamingDetectIntent".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link ServerStreamingDetectIntent#execute()} method to invoke the remote
* operation.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest}
* @return the request
*/
public ServerStreamingDetectIntent serverStreamingDetectIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest content) throws java.io.IOException {
ServerStreamingDetectIntent result = new ServerStreamingDetectIntent(session, content);
initialize(result);
return result;
}
public class ServerStreamingDetectIntent extends DialogflowRequest {
private static final String REST_PATH = "v3/{+session}:serverStreamingDetectIntent";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
/**
* Processes a natural language query and returns structured, actionable data as a result through
* server-side streaming. Server-side streaming allows Dialogflow to send [partial
* responses](https://cloud.google.com/dialogflow/cx/docs/concept/fulfillment#partial-response)
* earlier in a single request.
*
* Create a request for the method "sessions.serverStreamingDetectIntent".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link ServerStreamingDetectIntent#execute()} method to invoke
* the remote operation. {@link ServerStreamingDetectIntent#initialize(com.google.api.client.g
* oogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest}
* @since 1.13
*/
protected ServerStreamingDetectIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
}
@Override
public ServerStreamingDetectIntent set$Xgafv(java.lang.String $Xgafv) {
return (ServerStreamingDetectIntent) super.set$Xgafv($Xgafv);
}
@Override
public ServerStreamingDetectIntent setAccessToken(java.lang.String accessToken) {
return (ServerStreamingDetectIntent) super.setAccessToken(accessToken);
}
@Override
public ServerStreamingDetectIntent setAlt(java.lang.String alt) {
return (ServerStreamingDetectIntent) super.setAlt(alt);
}
@Override
public ServerStreamingDetectIntent setCallback(java.lang.String callback) {
return (ServerStreamingDetectIntent) super.setCallback(callback);
}
@Override
public ServerStreamingDetectIntent setFields(java.lang.String fields) {
return (ServerStreamingDetectIntent) super.setFields(fields);
}
@Override
public ServerStreamingDetectIntent setKey(java.lang.String key) {
return (ServerStreamingDetectIntent) super.setKey(key);
}
@Override
public ServerStreamingDetectIntent setOauthToken(java.lang.String oauthToken) {
return (ServerStreamingDetectIntent) super.setOauthToken(oauthToken);
}
@Override
public ServerStreamingDetectIntent setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ServerStreamingDetectIntent) super.setPrettyPrint(prettyPrint);
}
@Override
public ServerStreamingDetectIntent setQuotaUser(java.lang.String quotaUser) {
return (ServerStreamingDetectIntent) super.setQuotaUser(quotaUser);
}
@Override
public ServerStreamingDetectIntent setUploadType(java.lang.String uploadType) {
return (ServerStreamingDetectIntent) super.setUploadType(uploadType);
}
@Override
public ServerStreamingDetectIntent setUploadProtocol(java.lang.String uploadProtocol) {
return (ServerStreamingDetectIntent) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to
* choose an appropriate `Session ID`. It can be a random number or some type of
* session identifiers (preferably hashed). The length of the `Session ID` must not
* exceed 36 characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always
* use agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The name of the session this query is sent to. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment. It's up to the API
caller to choose an appropriate `Session ID`. It can be a random number or some type of session
identifiers (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
more information, see the [sessions
guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use agent
versions for production traffic. See [Versions and
environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
public java.lang.String getSession() {
return session;
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to
* choose an appropriate `Session ID`. It can be a random number or some type of
* session identifiers (preferably hashed). The length of the `Session ID` must not
* exceed 36 characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always
* use agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
public ServerStreamingDetectIntent setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public ServerStreamingDetectIntent set(String parameterName, Object value) {
return (ServerStreamingDetectIntent) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the EntityTypes collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.EntityTypes.List request = dialogflow.entityTypes().list(parameters ...)}
*
*
* @return the resource collection
*/
public EntityTypes entityTypes() {
return new EntityTypes();
}
/**
* The "entityTypes" collection of methods.
*/
public class EntityTypes {
/**
* Creates a session entity type.
*
* Create a request for the method "entityTypes.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The session to create a session entity type for. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/entityTypes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
/**
* Creates a session entity type.
*
* Create a request for the method "entityTypes.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The session to create a session entity type for. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The session to create a session entity type for. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is
* not specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The session to create a session entity type for. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The session to create a session entity type for. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is
* not specified, we assume default 'draft' environment.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified session entity type.
*
* Create a request for the method "entityTypes.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the session entity type to delete. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
/**
* Deletes the specified session entity type.
*
* Create a request for the method "entityTypes.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the session entity type to delete. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session entity type to delete. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the session entity type to delete. Format:
`projects//locations//agents//sessions//entityTypes/` or
`projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID` is not
specified, we assume default 'draft' environment.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the session entity type to delete. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified session entity type.
*
* Create a request for the method "entityTypes.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
/**
* Retrieves the specified session entity type.
*
* Create a request for the method "entityTypes.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the session entity type. Format:
`projects//locations//agents//sessions//entityTypes/` or
`projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID` is not
specified, we assume default 'draft' environment.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all session entity types in the specified session.
*
* Create a request for the method "entityTypes.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The session to list all session entity types from. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/entityTypes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
/**
* Returns the list of all session entity types in the specified session.
*
* Create a request for the method "entityTypes.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The session to list all session entity types from. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListSessionEntityTypesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The session to list all session entity types from. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is
* not specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The session to list all session entity types from. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The session to list all session entity types from. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is
* not specified, we assume default 'draft' environment.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at
* most 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at
* most 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified session entity type.
*
* Create a request for the method "entityTypes.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The unique identifier of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
/**
* Updates the specified session entity type.
*
* Create a request for the method "entityTypes.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The unique identifier of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The unique identifier of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The unique identifier of the session entity type. Format:
`projects//locations//agents//sessions//entityTypes/` or
`projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID` is not
specified, we assume default 'draft' environment.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The unique identifier of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/environments/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
this.name = name;
return this;
}
/** The mask to control which fields get updated. */
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** The mask to control which fields get updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Flows collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Flows.List request = dialogflow.flows().list(parameters ...)}
*
*
* @return the resource collection
*/
public Flows flows() {
return new Flows();
}
/**
* The "flows" collection of methods.
*/
public class Flows {
/**
* Creates a flow in the specified agent. Note: You should always train a flow prior to sending it
* queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "flows.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to create a flow for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/flows";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Creates a flow in the specified agent. Note: You should always train a flow prior to sending it
* queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "flows.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to create a flow for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to create a flow for. Format: `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to create a flow for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to create a flow for. Format: `projects//locations//agents/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language of the following fields in `flow`: *
* `Flow.event_handlers.trigger_fulfillment.messages` *
* `Flow.event_handlers.trigger_fulfillment.conditional_cases` *
* `Flow.transition_routes.trigger_fulfillment.messages` *
* `Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the
* agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `flow`: *
`Flow.event_handlers.trigger_fulfillment.messages` *
`Flow.event_handlers.trigger_fulfillment.conditional_cases` *
`Flow.transition_routes.trigger_fulfillment.messages` *
`Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `flow`: *
* `Flow.event_handlers.trigger_fulfillment.messages` *
* `Flow.event_handlers.trigger_fulfillment.conditional_cases` *
* `Flow.transition_routes.trigger_fulfillment.messages` *
* `Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the
* agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Create setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a specified flow.
*
* Create a request for the method "flows.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the flow to delete. Format: `projects//locations//agents//flows/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Deletes a specified flow.
*
* Create a request for the method "flows.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the flow to delete. Format: `projects//locations//agents//flows/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the flow to delete. Format:
* `projects//locations//agents//flows/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the flow to delete. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the flow to delete. Format:
* `projects//locations//agents//flows/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.name = name;
return this;
}
/**
* This field has no effect for flows with no incoming transitions. For flows with
* incoming transitions: * If `force` is set to false, an error will be returned with
* message indicating the incoming transitions. * If `force` is set to true, Dialogflow
* will remove the flow, as well as any transitions to the flow (i.e. Target flow in
* event handlers or Target flow in transition routes that point to this flow will be
* cleared).
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** This field has no effect for flows with no incoming transitions. For flows with incoming
transitions: * If `force` is set to false, an error will be returned with message indicating the
incoming transitions. * If `force` is set to true, Dialogflow will remove the flow, as well as any
transitions to the flow (i.e. Target flow in event handlers or Target flow in transition routes
that point to this flow will be cleared).
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* This field has no effect for flows with no incoming transitions. For flows with
* incoming transitions: * If `force` is set to false, an error will be returned with
* message indicating the incoming transitions. * If `force` is set to true, Dialogflow
* will remove the flow, as well as any transitions to the flow (i.e. Target flow in
* event handlers or Target flow in transition routes that point to this flow will be
* cleared).
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Exports the specified flow to a binary file. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: An empty [Struct
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#struct) -
* `response`: ExportFlowResponse Note that resources (e.g. intents, entities, webhooks) that the
* flow references will also be exported.
*
* Create a request for the method "flows.export".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the flow to export. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportFlowRequest}
* @return the request
*/
public Export export(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportFlowRequest content) throws java.io.IOException {
Export result = new Export(name, content);
initialize(result);
return result;
}
public class Export extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:export";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Exports the specified flow to a binary file. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`: An empty
* [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: ExportFlowResponse Note that
* resources (e.g. intents, entities, webhooks) that the flow references will also be exported.
*
* Create a request for the method "flows.export".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Export#execute()} method to invoke the remote operation.
* {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the flow to export. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportFlowRequest}
* @since 1.13
*/
protected Export(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportFlowRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the flow to export. Format:
* `projects//locations//agents//flows/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the flow to export. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the flow to export. Format:
* `projects//locations//agents//flows/`.
*/
public Export setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Retrieves the specified flow.
*
* Create a request for the method "flows.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the flow to get. Format: `projects//locations//agents//flows/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Retrieves the specified flow.
*
* Create a request for the method "flows.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the flow to get. Format: `projects//locations//agents//flows/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the flow to get. Format: `projects//locations//agents//flows/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the flow to get. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the flow to get. Format: `projects//locations//agents//flows/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language to retrieve the flow for. The following fields are language dependent: *
* `Flow.event_handlers.trigger_fulfillment.messages` *
* `Flow.event_handlers.trigger_fulfillment.conditional_cases` *
* `Flow.transition_routes.trigger_fulfillment.messages` *
* `Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the
* agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to retrieve the flow for. The following fields are language dependent: *
`Flow.event_handlers.trigger_fulfillment.messages` *
`Flow.event_handlers.trigger_fulfillment.conditional_cases` *
`Flow.transition_routes.trigger_fulfillment.messages` *
`Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to retrieve the flow for. The following fields are language dependent: *
* `Flow.event_handlers.trigger_fulfillment.messages` *
* `Flow.event_handlers.trigger_fulfillment.conditional_cases` *
* `Flow.transition_routes.trigger_fulfillment.messages` *
* `Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the
* agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Get setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the latest flow validation result. Flow validation is performed when ValidateFlow is called.
*
* Create a request for the method "flows.getValidationResult".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link GetValidationResult#execute()} method to invoke the remote operation.
*
* @param name Required. The flow name. Format: `projects//locations//agents//flows//validationResult`.
* @return the request
*/
public GetValidationResult getValidationResult(java.lang.String name) throws java.io.IOException {
GetValidationResult result = new GetValidationResult(name);
initialize(result);
return result;
}
public class GetValidationResult extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/validationResult$");
/**
* Gets the latest flow validation result. Flow validation is performed when ValidateFlow is
* called.
*
* Create a request for the method "flows.getValidationResult".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link GetValidationResult#execute()} method to invoke the remote
* operation. {@link GetValidationResult#initialize(com.google.api.client.googleapis.services.
* AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The flow name. Format: `projects//locations//agents//flows//validationResult`.
* @since 1.13
*/
protected GetValidationResult(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FlowValidationResult.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/validationResult$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetValidationResult set$Xgafv(java.lang.String $Xgafv) {
return (GetValidationResult) super.set$Xgafv($Xgafv);
}
@Override
public GetValidationResult setAccessToken(java.lang.String accessToken) {
return (GetValidationResult) super.setAccessToken(accessToken);
}
@Override
public GetValidationResult setAlt(java.lang.String alt) {
return (GetValidationResult) super.setAlt(alt);
}
@Override
public GetValidationResult setCallback(java.lang.String callback) {
return (GetValidationResult) super.setCallback(callback);
}
@Override
public GetValidationResult setFields(java.lang.String fields) {
return (GetValidationResult) super.setFields(fields);
}
@Override
public GetValidationResult setKey(java.lang.String key) {
return (GetValidationResult) super.setKey(key);
}
@Override
public GetValidationResult setOauthToken(java.lang.String oauthToken) {
return (GetValidationResult) super.setOauthToken(oauthToken);
}
@Override
public GetValidationResult setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetValidationResult) super.setPrettyPrint(prettyPrint);
}
@Override
public GetValidationResult setQuotaUser(java.lang.String quotaUser) {
return (GetValidationResult) super.setQuotaUser(quotaUser);
}
@Override
public GetValidationResult setUploadType(java.lang.String uploadType) {
return (GetValidationResult) super.setUploadType(uploadType);
}
@Override
public GetValidationResult setUploadProtocol(java.lang.String uploadProtocol) {
return (GetValidationResult) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The flow name. Format:
* `projects//locations//agents//flows//validationResult`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The flow name. Format: `projects//locations//agents//flows//validationResult`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The flow name. Format:
* `projects//locations//agents//flows//validationResult`.
*/
public GetValidationResult setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/validationResult$");
}
this.name = name;
return this;
}
/** If not specified, the agent's default language is used. */
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** If not specified, the agent's default language is used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/** If not specified, the agent's default language is used. */
public GetValidationResult setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public GetValidationResult set(String parameterName, Object value) {
return (GetValidationResult) super.set(parameterName, value);
}
}
/**
* Imports the specified flow to the specified agent from a binary file. This method is a [long-
* running operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`: An empty
* [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: ImportFlowResponse Note: You should
* always train a flow prior to sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "flows.import".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link DialogflowImport#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to import the flow into. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportFlowRequest}
* @return the request
*/
public DialogflowImport dialogflowImport(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportFlowRequest content) throws java.io.IOException {
DialogflowImport result = new DialogflowImport(parent, content);
initialize(result);
return result;
}
public class DialogflowImport extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/flows:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Imports the specified flow to the specified agent from a binary file. This method is a [long-
* running operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`: An empty
* [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: ImportFlowResponse Note: You
* should always train a flow prior to sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "flows.import".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link DialogflowImport#execute()} method to invoke the remote
* operation. {@link DialogflowImport#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The agent to import the flow into. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportFlowRequest}
* @since 1.13
*/
protected DialogflowImport(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportFlowRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public DialogflowImport set$Xgafv(java.lang.String $Xgafv) {
return (DialogflowImport) super.set$Xgafv($Xgafv);
}
@Override
public DialogflowImport setAccessToken(java.lang.String accessToken) {
return (DialogflowImport) super.setAccessToken(accessToken);
}
@Override
public DialogflowImport setAlt(java.lang.String alt) {
return (DialogflowImport) super.setAlt(alt);
}
@Override
public DialogflowImport setCallback(java.lang.String callback) {
return (DialogflowImport) super.setCallback(callback);
}
@Override
public DialogflowImport setFields(java.lang.String fields) {
return (DialogflowImport) super.setFields(fields);
}
@Override
public DialogflowImport setKey(java.lang.String key) {
return (DialogflowImport) super.setKey(key);
}
@Override
public DialogflowImport setOauthToken(java.lang.String oauthToken) {
return (DialogflowImport) super.setOauthToken(oauthToken);
}
@Override
public DialogflowImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DialogflowImport) super.setPrettyPrint(prettyPrint);
}
@Override
public DialogflowImport setQuotaUser(java.lang.String quotaUser) {
return (DialogflowImport) super.setQuotaUser(quotaUser);
}
@Override
public DialogflowImport setUploadType(java.lang.String uploadType) {
return (DialogflowImport) super.setUploadType(uploadType);
}
@Override
public DialogflowImport setUploadProtocol(java.lang.String uploadProtocol) {
return (DialogflowImport) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to import the flow into. Format: `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to import the flow into. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to import the flow into. Format: `projects//locations//agents/`.
*/
public DialogflowImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public DialogflowImport set(String parameterName, Object value) {
return (DialogflowImport) super.set(parameterName, value);
}
}
/**
* Returns the list of all flows in the specified agent.
*
* Create a request for the method "flows.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent containing the flows. Format: `projects//locations//agents/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/flows";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Returns the list of all flows in the specified agent.
*
* Create a request for the method "flows.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent containing the flows. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListFlowsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent containing the flows. Format: `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent containing the flows. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent containing the flows. Format: `projects//locations//agents/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language to list flows for. The following fields are language dependent: *
* `Flow.event_handlers.trigger_fulfillment.messages` *
* `Flow.event_handlers.trigger_fulfillment.conditional_cases` *
* `Flow.transition_routes.trigger_fulfillment.messages` *
* `Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the
* agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to list flows for. The following fields are language dependent: *
`Flow.event_handlers.trigger_fulfillment.messages` *
`Flow.event_handlers.trigger_fulfillment.conditional_cases` *
`Flow.transition_routes.trigger_fulfillment.messages` *
`Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to list flows for. The following fields are language dependent: *
* `Flow.event_handlers.trigger_fulfillment.messages` *
* `Flow.event_handlers.trigger_fulfillment.conditional_cases` *
* `Flow.transition_routes.trigger_fulfillment.messages` *
* `Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the
* agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified flow. Note: You should always train a flow prior to sending it queries. See
* the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "flows.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the flow. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Updates the specified flow. Note: You should always train a flow prior to sending it queries.
* See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "flows.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the flow. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Flow.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the flow. Format: `projects//locations//agents//flows/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the flow. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the flow. Format: `projects//locations//agents//flows/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language of the following fields in `flow`: *
* `Flow.event_handlers.trigger_fulfillment.messages` *
* `Flow.event_handlers.trigger_fulfillment.conditional_cases` *
* `Flow.transition_routes.trigger_fulfillment.messages` *
* `Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the
* agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `flow`: *
`Flow.event_handlers.trigger_fulfillment.messages` *
`Flow.event_handlers.trigger_fulfillment.conditional_cases` *
`Flow.transition_routes.trigger_fulfillment.messages` *
`Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `flow`: *
* `Flow.event_handlers.trigger_fulfillment.messages` *
* `Flow.event_handlers.trigger_fulfillment.conditional_cases` *
* `Flow.transition_routes.trigger_fulfillment.messages` *
* `Flow.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the
* agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Patch setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated. If the mask is not present, all fields will be
updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Trains the specified flow. Note that only the flow in 'draft' environment is trained. This method
* is a [long-running operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-
* operation). The returned `Operation` type has the following method-specific fields: - `metadata`:
* An empty [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: An [Empty
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#empty)
* Note: You should always train a flow prior to sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "flows.train".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Train#execute()} method to invoke the remote operation.
*
* @param name Required. The flow to train. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TrainFlowRequest}
* @return the request
*/
public Train train(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TrainFlowRequest content) throws java.io.IOException {
Train result = new Train(name, content);
initialize(result);
return result;
}
public class Train extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:train";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Trains the specified flow. Note that only the flow in 'draft' environment is trained. This
* method is a [long-running operation](https://cloud.google.com/dialogflow/cx/docs/how/long-
* running-operation). The returned `Operation` type has the following method-specific fields: -
* `metadata`: An empty [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: An [Empty
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#empty)
* Note: You should always train a flow prior to sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "flows.train".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Train#execute()} method to invoke the remote operation.
* {@link
* Train#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The flow to train. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TrainFlowRequest}
* @since 1.13
*/
protected Train(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TrainFlowRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public Train set$Xgafv(java.lang.String $Xgafv) {
return (Train) super.set$Xgafv($Xgafv);
}
@Override
public Train setAccessToken(java.lang.String accessToken) {
return (Train) super.setAccessToken(accessToken);
}
@Override
public Train setAlt(java.lang.String alt) {
return (Train) super.setAlt(alt);
}
@Override
public Train setCallback(java.lang.String callback) {
return (Train) super.setCallback(callback);
}
@Override
public Train setFields(java.lang.String fields) {
return (Train) super.setFields(fields);
}
@Override
public Train setKey(java.lang.String key) {
return (Train) super.setKey(key);
}
@Override
public Train setOauthToken(java.lang.String oauthToken) {
return (Train) super.setOauthToken(oauthToken);
}
@Override
public Train setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Train) super.setPrettyPrint(prettyPrint);
}
@Override
public Train setQuotaUser(java.lang.String quotaUser) {
return (Train) super.setQuotaUser(quotaUser);
}
@Override
public Train setUploadType(java.lang.String uploadType) {
return (Train) super.setUploadType(uploadType);
}
@Override
public Train setUploadProtocol(java.lang.String uploadProtocol) {
return (Train) super.setUploadProtocol(uploadProtocol);
}
/** Required. The flow to train. Format: `projects//locations//agents//flows/`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The flow to train. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getName() {
return name;
}
/** Required. The flow to train. Format: `projects//locations//agents//flows/`. */
public Train setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Train set(String parameterName, Object value) {
return (Train) super.set(parameterName, value);
}
}
/**
* Validates the specified flow and creates or updates validation results. Please call this API
* after the training is completed to get the complete validation results.
*
* Create a request for the method "flows.validate".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Validate#execute()} method to invoke the remote operation.
*
* @param name Required. The flow to validate. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ValidateFlowRequest}
* @return the request
*/
public Validate validate(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ValidateFlowRequest content) throws java.io.IOException {
Validate result = new Validate(name, content);
initialize(result);
return result;
}
public class Validate extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:validate";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Validates the specified flow and creates or updates validation results. Please call this API
* after the training is completed to get the complete validation results.
*
* Create a request for the method "flows.validate".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Validate#execute()} method to invoke the remote operation.
* {@link
* Validate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The flow to validate. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ValidateFlowRequest}
* @since 1.13
*/
protected Validate(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ValidateFlowRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FlowValidationResult.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public Validate set$Xgafv(java.lang.String $Xgafv) {
return (Validate) super.set$Xgafv($Xgafv);
}
@Override
public Validate setAccessToken(java.lang.String accessToken) {
return (Validate) super.setAccessToken(accessToken);
}
@Override
public Validate setAlt(java.lang.String alt) {
return (Validate) super.setAlt(alt);
}
@Override
public Validate setCallback(java.lang.String callback) {
return (Validate) super.setCallback(callback);
}
@Override
public Validate setFields(java.lang.String fields) {
return (Validate) super.setFields(fields);
}
@Override
public Validate setKey(java.lang.String key) {
return (Validate) super.setKey(key);
}
@Override
public Validate setOauthToken(java.lang.String oauthToken) {
return (Validate) super.setOauthToken(oauthToken);
}
@Override
public Validate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Validate) super.setPrettyPrint(prettyPrint);
}
@Override
public Validate setQuotaUser(java.lang.String quotaUser) {
return (Validate) super.setQuotaUser(quotaUser);
}
@Override
public Validate setUploadType(java.lang.String uploadType) {
return (Validate) super.setUploadType(uploadType);
}
@Override
public Validate setUploadProtocol(java.lang.String uploadProtocol) {
return (Validate) super.setUploadProtocol(uploadProtocol);
}
/** Required. The flow to validate. Format: `projects//locations//agents//flows/`. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The flow to validate. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getName() {
return name;
}
/** Required. The flow to validate. Format: `projects//locations//agents//flows/`. */
public Validate setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Validate set(String parameterName, Object value) {
return (Validate) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Pages collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Pages.List request = dialogflow.pages().list(parameters ...)}
*
*
* @return the resource collection
*/
public Pages pages() {
return new Pages();
}
/**
* The "pages" collection of methods.
*/
public class Pages {
/**
* Creates a page in the specified flow. Note: You should always train a flow prior to sending it
* queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "pages.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The flow to create a page for. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/pages";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Creates a page in the specified flow. Note: You should always train a flow prior to sending it
* queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "pages.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The flow to create a page for. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The flow to create a page for. Format:
* `projects//locations//agents//flows/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The flow to create a page for. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The flow to create a page for. Format:
* `projects//locations//agents//flows/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language of the following fields in `page`: * `Page.entry_fulfillment.messages`
* * `Page.entry_fulfillment.conditional_cases` *
* `Page.event_handlers.trigger_fulfillment.messages` *
* `Page.event_handlers.trigger_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
* `Page.transition_routes.trigger_fulfillment.messages` *
* `Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
* the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `page`: * `Page.entry_fulfillment.messages` *
`Page.entry_fulfillment.conditional_cases` * `Page.event_handlers.trigger_fulfillment.messages` *
`Page.event_handlers.trigger_fulfillment.conditional_cases` *
`Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
`Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
`Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
`Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
`Page.transition_routes.trigger_fulfillment.messages` *
`Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `page`: * `Page.entry_fulfillment.messages`
* * `Page.entry_fulfillment.conditional_cases` *
* `Page.event_handlers.trigger_fulfillment.messages` *
* `Page.event_handlers.trigger_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
* `Page.transition_routes.trigger_fulfillment.messages` *
* `Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
* the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Create setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified page. Note: You should always train a flow prior to sending it queries. See
* the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "pages.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the page to delete. Format: `projects//locations//agents//Flows//pages/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/pages/[^/]+$");
/**
* Deletes the specified page. Note: You should always train a flow prior to sending it queries.
* See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "pages.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the page to delete. Format: `projects//locations//agents//Flows//pages/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/pages/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the page to delete. Format:
* `projects//locations//agents//Flows//pages/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the page to delete. Format: `projects//locations//agents//Flows//pages/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the page to delete. Format:
* `projects//locations//agents//Flows//pages/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/pages/[^/]+$");
}
this.name = name;
return this;
}
/**
* This field has no effect for pages with no incoming transitions. For pages with
* incoming transitions: * If `force` is set to false, an error will be returned with
* message indicating the incoming transitions. * If `force` is set to true,
* Dialogflow will remove the page, as well as any transitions to the page (i.e.
* Target page in event handlers or Target page in transition routes that point to
* this page will be cleared).
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** This field has no effect for pages with no incoming transitions. For pages with incoming
transitions: * If `force` is set to false, an error will be returned with message indicating the
incoming transitions. * If `force` is set to true, Dialogflow will remove the page, as well as any
transitions to the page (i.e. Target page in event handlers or Target page in transition routes
that point to this page will be cleared).
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* This field has no effect for pages with no incoming transitions. For pages with
* incoming transitions: * If `force` is set to false, an error will be returned with
* message indicating the incoming transitions. * If `force` is set to true,
* Dialogflow will remove the page, as well as any transitions to the page (i.e.
* Target page in event handlers or Target page in transition routes that point to
* this page will be cleared).
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified page.
*
* Create a request for the method "pages.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the page. Format: `projects//locations//agents//flows//pages/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/pages/[^/]+$");
/**
* Retrieves the specified page.
*
* Create a request for the method "pages.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the page. Format: `projects//locations//agents//flows//pages/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/pages/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the page. Format:
* `projects//locations//agents//flows//pages/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the page. Format: `projects//locations//agents//flows//pages/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the page. Format:
* `projects//locations//agents//flows//pages/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/pages/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language to retrieve the page for. The following fields are language dependent:
* * `Page.entry_fulfillment.messages` * `Page.entry_fulfillment.conditional_cases` *
* `Page.event_handlers.trigger_fulfillment.messages` *
* `Page.event_handlers.trigger_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
* `Page.transition_routes.trigger_fulfillment.messages` *
* `Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
* the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to retrieve the page for. The following fields are language dependent: *
`Page.entry_fulfillment.messages` * `Page.entry_fulfillment.conditional_cases` *
`Page.event_handlers.trigger_fulfillment.messages` *
`Page.event_handlers.trigger_fulfillment.conditional_cases` *
`Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
`Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
`Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
`Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
`Page.transition_routes.trigger_fulfillment.messages` *
`Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to retrieve the page for. The following fields are language dependent:
* * `Page.entry_fulfillment.messages` * `Page.entry_fulfillment.conditional_cases` *
* `Page.event_handlers.trigger_fulfillment.messages` *
* `Page.event_handlers.trigger_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
* `Page.transition_routes.trigger_fulfillment.messages` *
* `Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
* the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Get setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all pages in the specified flow.
*
* Create a request for the method "pages.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The flow to list all pages for. Format: `projects//locations//agents//flows/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/pages";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Returns the list of all pages in the specified flow.
*
* Create a request for the method "pages.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The flow to list all pages for. Format: `projects//locations//agents//flows/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListPagesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The flow to list all pages for. Format:
* `projects//locations//agents//flows/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The flow to list all pages for. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The flow to list all pages for. Format:
* `projects//locations//agents//flows/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language to list pages for. The following fields are language dependent: *
* `Page.entry_fulfillment.messages` * `Page.entry_fulfillment.conditional_cases` *
* `Page.event_handlers.trigger_fulfillment.messages` *
* `Page.event_handlers.trigger_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
* `Page.transition_routes.trigger_fulfillment.messages` *
* `Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
* the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to list pages for. The following fields are language dependent: *
`Page.entry_fulfillment.messages` * `Page.entry_fulfillment.conditional_cases` *
`Page.event_handlers.trigger_fulfillment.messages` *
`Page.event_handlers.trigger_fulfillment.conditional_cases` *
`Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
`Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
`Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
`Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
`Page.transition_routes.trigger_fulfillment.messages` *
`Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to list pages for. The following fields are language dependent: *
* `Page.entry_fulfillment.messages` * `Page.entry_fulfillment.conditional_cases` *
* `Page.event_handlers.trigger_fulfillment.messages` *
* `Page.event_handlers.trigger_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
* `Page.transition_routes.trigger_fulfillment.messages` *
* `Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
* the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified page. Note: You should always train a flow prior to sending it queries. See
* the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "pages.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the page. Required for the Pages.UpdatePage method. Pages.CreatePage
* populates the name automatically. Format: `projects//locations//agents//flows//pages/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/pages/[^/]+$");
/**
* Updates the specified page. Note: You should always train a flow prior to sending it queries.
* See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "pages.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the page. Required for the Pages.UpdatePage method. Pages.CreatePage
* populates the name automatically. Format: `projects//locations//agents//flows//pages/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Page.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/pages/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the page. Required for the Pages.UpdatePage method.
* Pages.CreatePage populates the name automatically. Format:
* `projects//locations//agents//flows//pages/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the page. Required for the Pages.UpdatePage method. Pages.CreatePage
populates the name automatically. Format: `projects//locations//agents//flows//pages/`.
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the page. Required for the Pages.UpdatePage method.
* Pages.CreatePage populates the name automatically. Format:
* `projects//locations//agents//flows//pages/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/pages/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language of the following fields in `page`: * `Page.entry_fulfillment.messages`
* * `Page.entry_fulfillment.conditional_cases` *
* `Page.event_handlers.trigger_fulfillment.messages` *
* `Page.event_handlers.trigger_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
* `Page.transition_routes.trigger_fulfillment.messages` *
* `Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
* the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `page`: * `Page.entry_fulfillment.messages` *
`Page.entry_fulfillment.conditional_cases` * `Page.event_handlers.trigger_fulfillment.messages` *
`Page.event_handlers.trigger_fulfillment.conditional_cases` *
`Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
`Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
`Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
`Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
`Page.transition_routes.trigger_fulfillment.messages` *
`Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified, the agent's
default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `page`: * `Page.entry_fulfillment.messages`
* * `Page.entry_fulfillment.conditional_cases` *
* `Page.event_handlers.trigger_fulfillment.messages` *
* `Page.event_handlers.trigger_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.messages` *
* `Page.form.parameters.fill_behavior.initial_prompt_fulfillment.conditional_cases` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.messages` *
* `Page.form.parameters.fill_behavior.reprompt_event_handlers.conditional_cases` *
* `Page.transition_routes.trigger_fulfillment.messages` *
* `Page.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
* the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Patch setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The mask to control which fields get updated. If the mask is not present, all
* fields will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated. If the mask is not present, all fields will be
updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The mask to control which fields get updated. If the mask is not present, all
* fields will be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the TransitionRouteGroups collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.TransitionRouteGroups.List request = dialogflow.transitionRouteGroups().list(parameters ...)}
*
*
* @return the resource collection
*/
public TransitionRouteGroups transitionRouteGroups() {
return new TransitionRouteGroups();
}
/**
* The "transitionRouteGroups" collection of methods.
*/
public class TransitionRouteGroups {
/**
* Creates an TransitionRouteGroup in the specified flow. Note: You should always train a flow prior
* to sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The flow to create an TransitionRouteGroup for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/` for agent-level
* groups.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/transitionRouteGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Creates an TransitionRouteGroup in the specified flow. Note: You should always train a flow
* prior to sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The flow to create an TransitionRouteGroup for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/` for agent-level
* groups.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The flow to create an TransitionRouteGroup for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/` for agent-
* level groups.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The flow to create an TransitionRouteGroup for. Format:
`projects//locations//agents//flows/` or `projects//locations//agents/` for agent-level groups.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The flow to create an TransitionRouteGroup for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/` for agent-
* level groups.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language of the following fields in `TransitionRouteGroup`: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If
* not specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `TransitionRouteGroup`: *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `TransitionRouteGroup`: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If
* not specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Create setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified TransitionRouteGroup. Note: You should always train a flow prior to sending
* it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the TransitionRouteGroup to delete. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/transitionRouteGroups/[^/]+$");
/**
* Deletes the specified TransitionRouteGroup. Note: You should always train a flow prior to
* sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the TransitionRouteGroup to delete. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/transitionRouteGroups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the TransitionRouteGroup to delete. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the TransitionRouteGroup to delete. Format:
`projects//locations//agents//flows//transitionRouteGroups/` or
`projects//locations//agents//transitionRouteGroups/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the TransitionRouteGroup to delete. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/transitionRouteGroups/[^/]+$");
}
this.name = name;
return this;
}
/**
* This field has no effect for transition route group that no page is using. If the
* transition route group is referenced by any page: * If `force` is set to false, an
* error will be returned with message indicating pages that reference the transition
* route group. * If `force` is set to true, Dialogflow will remove the transition
* route group, as well as any reference to it.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** This field has no effect for transition route group that no page is using. If the transition route
group is referenced by any page: * If `force` is set to false, an error will be returned with
message indicating pages that reference the transition route group. * If `force` is set to true,
Dialogflow will remove the transition route group, as well as any reference to it.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* This field has no effect for transition route group that no page is using. If the
* transition route group is referenced by any page: * If `force` is set to false, an
* error will be returned with message indicating pages that reference the transition
* route group. * If `force` is set to true, Dialogflow will remove the transition
* route group, as well as any reference to it.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified TransitionRouteGroup.
*
* Create a request for the method "transitionRouteGroups.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the TransitionRouteGroup. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/transitionRouteGroups/[^/]+$");
/**
* Retrieves the specified TransitionRouteGroup.
*
* Create a request for the method "transitionRouteGroups.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the TransitionRouteGroup. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/transitionRouteGroups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the TransitionRouteGroup. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the TransitionRouteGroup. Format:
`projects//locations//agents//flows//transitionRouteGroups/` or
`projects//locations//agents//transitionRouteGroups/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the TransitionRouteGroup. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/transitionRouteGroups/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language to retrieve the transition route group for. The following fields are
* language dependent: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If
* not specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to retrieve the transition route group for. The following fields are language
dependent: * `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to retrieve the transition route group for. The following fields are
* language dependent: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If
* not specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Get setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all transition route groups in the specified flow.
*
* Create a request for the method "transitionRouteGroups.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The flow to list all transition route groups for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/transitionRouteGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Returns the list of all transition route groups in the specified flow.
*
* Create a request for the method "transitionRouteGroups.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The flow to list all transition route groups for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListTransitionRouteGroupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The flow to list all transition route groups for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The flow to list all transition route groups for. Format:
`projects//locations//agents//flows/` or `projects//locations//agents/.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The flow to list all transition route groups for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language to list transition route groups for. The following fields are language
* dependent: * `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages`
* * `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If
* not specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to list transition route groups for. The following fields are language dependent: *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to list transition route groups for. The following fields are language
* dependent: * `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages`
* * `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If
* not specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified TransitionRouteGroup. Note: You should always train a flow prior to sending
* it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the transition route group.
* TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` .
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/transitionRouteGroups/[^/]+$");
/**
* Updates the specified TransitionRouteGroup. Note: You should always train a flow prior to
* sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the transition route group.
* TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` .
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/transitionRouteGroups/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the transition route group.
* TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically.
* Format: `projects//locations//agents//flows//transitionRouteGroups/` .
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the transition route group.
TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically. Format:
`projects//locations//agents//flows//transitionRouteGroups/` .
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the transition route group.
* TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically.
* Format: `projects//locations//agents//flows//transitionRouteGroups/` .
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/transitionRouteGroups/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language of the following fields in `TransitionRouteGroup`: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If
* not specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `TransitionRouteGroup`: *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `TransitionRouteGroup`: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If
* not specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Patch setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/** The mask to control which fields get updated. */
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** The mask to control which fields get updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Versions collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Versions.List request = dialogflow.versions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Versions versions() {
return new Versions();
}
/**
* The "versions" collection of methods.
*/
public class Versions {
/**
* Compares the specified base version with target version.
*
* Create a request for the method "versions.compareVersions".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link CompareVersions#execute()} method to invoke the remote operation.
*
* @param baseVersion Required. Name of the base flow version to compare with the target version. Use version ID `0` to
* indicate the draft version of the specified flow. Format: `projects//locations//agents/
* /flows//versions/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3CompareVersionsRequest}
* @return the request
*/
public CompareVersions compareVersions(java.lang.String baseVersion, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3CompareVersionsRequest content) throws java.io.IOException {
CompareVersions result = new CompareVersions(baseVersion, content);
initialize(result);
return result;
}
public class CompareVersions extends DialogflowRequest {
private static final String REST_PATH = "v3/{+baseVersion}:compareVersions";
private final java.util.regex.Pattern BASE_VERSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
/**
* Compares the specified base version with target version.
*
* Create a request for the method "versions.compareVersions".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link CompareVersions#execute()} method to invoke the remote
* operation. {@link CompareVersions#initialize(com.google.api.client.googleapis.services.Abst
* ractGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param baseVersion Required. Name of the base flow version to compare with the target version. Use version ID `0` to
* indicate the draft version of the specified flow. Format: `projects//locations//agents/
* /flows//versions/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3CompareVersionsRequest}
* @since 1.13
*/
protected CompareVersions(java.lang.String baseVersion, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3CompareVersionsRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3CompareVersionsResponse.class);
this.baseVersion = com.google.api.client.util.Preconditions.checkNotNull(baseVersion, "Required parameter baseVersion must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(BASE_VERSION_PATTERN.matcher(baseVersion).matches(),
"Parameter baseVersion must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
}
@Override
public CompareVersions set$Xgafv(java.lang.String $Xgafv) {
return (CompareVersions) super.set$Xgafv($Xgafv);
}
@Override
public CompareVersions setAccessToken(java.lang.String accessToken) {
return (CompareVersions) super.setAccessToken(accessToken);
}
@Override
public CompareVersions setAlt(java.lang.String alt) {
return (CompareVersions) super.setAlt(alt);
}
@Override
public CompareVersions setCallback(java.lang.String callback) {
return (CompareVersions) super.setCallback(callback);
}
@Override
public CompareVersions setFields(java.lang.String fields) {
return (CompareVersions) super.setFields(fields);
}
@Override
public CompareVersions setKey(java.lang.String key) {
return (CompareVersions) super.setKey(key);
}
@Override
public CompareVersions setOauthToken(java.lang.String oauthToken) {
return (CompareVersions) super.setOauthToken(oauthToken);
}
@Override
public CompareVersions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CompareVersions) super.setPrettyPrint(prettyPrint);
}
@Override
public CompareVersions setQuotaUser(java.lang.String quotaUser) {
return (CompareVersions) super.setQuotaUser(quotaUser);
}
@Override
public CompareVersions setUploadType(java.lang.String uploadType) {
return (CompareVersions) super.setUploadType(uploadType);
}
@Override
public CompareVersions setUploadProtocol(java.lang.String uploadProtocol) {
return (CompareVersions) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the base flow version to compare with the target version. Use
* version ID `0` to indicate the draft version of the specified flow. Format:
* `projects//locations//agents/ /flows//versions/`.
*/
@com.google.api.client.util.Key
private java.lang.String baseVersion;
/** Required. Name of the base flow version to compare with the target version. Use version ID `0` to
indicate the draft version of the specified flow. Format: `projects//locations//agents/
/flows//versions/`.
*/
public java.lang.String getBaseVersion() {
return baseVersion;
}
/**
* Required. Name of the base flow version to compare with the target version. Use
* version ID `0` to indicate the draft version of the specified flow. Format:
* `projects//locations//agents/ /flows//versions/`.
*/
public CompareVersions setBaseVersion(java.lang.String baseVersion) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(BASE_VERSION_PATTERN.matcher(baseVersion).matches(),
"Parameter baseVersion must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
this.baseVersion = baseVersion;
return this;
}
@Override
public CompareVersions set(String parameterName, Object value) {
return (CompareVersions) super.set(parameterName, value);
}
}
/**
* Creates a Version in the specified Flow. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`:
* CreateVersionOperationMetadata - `response`: Version
*
* Create a request for the method "versions.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The Flow to create an Version for. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/versions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Creates a Version in the specified Flow. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`:
* CreateVersionOperationMetadata - `response`: Version
*
* Create a request for the method "versions.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Flow to create an Version for. Format: `projects//locations//agents//flows/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Flow to create an Version for. Format:
* `projects//locations//agents//flows/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Flow to create an Version for. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Flow to create an Version for. Format:
* `projects//locations//agents//flows/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified Version.
*
* Create a request for the method "versions.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Version to delete. Format:
* `projects//locations//agents//flows//versions/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
/**
* Deletes the specified Version.
*
* Create a request for the method "versions.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Version to delete. Format:
* `projects//locations//agents//flows//versions/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Version to delete. Format:
* `projects//locations//agents//flows//versions/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Version to delete. Format:
`projects//locations//agents//flows//versions/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Version to delete. Format:
* `projects//locations//agents//flows//versions/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified Version.
*
* Create a request for the method "versions.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the Version. Format: `projects//locations//agents//flows//versions/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
/**
* Retrieves the specified Version.
*
* Create a request for the method "versions.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the Version. Format: `projects//locations//agents//flows//versions/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the Version. Format:
* `projects//locations//agents//flows//versions/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the Version. Format: `projects//locations//agents//flows//versions/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the Version. Format:
* `projects//locations//agents//flows//versions/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all versions in the specified Flow.
*
* Create a request for the method "versions.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The Flow to list all versions for. Format: `projects//locations//agents//flows/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/versions";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
/**
* Returns the list of all versions in the specified Flow.
*
* Create a request for the method "versions.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The Flow to list all versions for. Format: `projects//locations//agents//flows/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListVersionsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Flow to list all versions for. Format:
* `projects//locations//agents//flows/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The Flow to list all versions for. Format: `projects//locations//agents//flows/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The Flow to list all versions for. Format:
* `projects//locations//agents//flows/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most
* 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 20 and at most 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most
* 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Loads resources in the specified version to the draft flow. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: An empty [Struct
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#struct) -
* `response`: An [Empty message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#empty)
*
* Create a request for the method "versions.load".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Load#execute()} method to invoke the remote operation.
*
* @param name Required. The Version to be loaded to draft flow. Format:
* `projects//locations//agents//flows//versions/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3LoadVersionRequest}
* @return the request
*/
public Load load(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3LoadVersionRequest content) throws java.io.IOException {
Load result = new Load(name, content);
initialize(result);
return result;
}
public class Load extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:load";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
/**
* Loads resources in the specified version to the draft flow. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`: An empty
* [Struct message](https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#struct) - `response`: An [Empty
* message](https://developers.google.com/protocol-buffers/docs/reference/google.protobuf#empty)
*
* Create a request for the method "versions.load".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Load#execute()} method to invoke the remote operation.
* {@link Load#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The Version to be loaded to draft flow. Format:
* `projects//locations//agents//flows//versions/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3LoadVersionRequest}
* @since 1.13
*/
protected Load(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3LoadVersionRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
}
@Override
public Load set$Xgafv(java.lang.String $Xgafv) {
return (Load) super.set$Xgafv($Xgafv);
}
@Override
public Load setAccessToken(java.lang.String accessToken) {
return (Load) super.setAccessToken(accessToken);
}
@Override
public Load setAlt(java.lang.String alt) {
return (Load) super.setAlt(alt);
}
@Override
public Load setCallback(java.lang.String callback) {
return (Load) super.setCallback(callback);
}
@Override
public Load setFields(java.lang.String fields) {
return (Load) super.setFields(fields);
}
@Override
public Load setKey(java.lang.String key) {
return (Load) super.setKey(key);
}
@Override
public Load setOauthToken(java.lang.String oauthToken) {
return (Load) super.setOauthToken(oauthToken);
}
@Override
public Load setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Load) super.setPrettyPrint(prettyPrint);
}
@Override
public Load setQuotaUser(java.lang.String quotaUser) {
return (Load) super.setQuotaUser(quotaUser);
}
@Override
public Load setUploadType(java.lang.String uploadType) {
return (Load) super.setUploadType(uploadType);
}
@Override
public Load setUploadProtocol(java.lang.String uploadProtocol) {
return (Load) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The Version to be loaded to draft flow. Format:
* `projects//locations//agents//flows//versions/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The Version to be loaded to draft flow. Format:
`projects//locations//agents//flows//versions/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The Version to be loaded to draft flow. Format:
* `projects//locations//agents//flows//versions/`.
*/
public Load setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Load set(String parameterName, Object value) {
return (Load) super.set(parameterName, value);
}
}
/**
* Updates the specified Version.
*
* Create a request for the method "versions.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Format: projects//locations//agents//flows//versions/. Version ID is a self-increasing number
* generated by Dialogflow upon version creation.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
/**
* Updates the specified Version.
*
* Create a request for the method "versions.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Format: projects//locations//agents//flows//versions/. Version ID is a self-increasing number
* generated by Dialogflow upon version creation.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Version.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Format: projects//locations//agents//flows//versions/. Version ID is a self-
* increasing number generated by Dialogflow upon version creation.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Format: projects//locations//agents//flows//versions/. Version ID is a self-increasing number
generated by Dialogflow upon version creation.
*/
public java.lang.String getName() {
return name;
}
/**
* Format: projects//locations//agents//flows//versions/. Version ID is a self-
* increasing number generated by Dialogflow upon version creation.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/flows/[^/]+/versions/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. The mask to control which fields get updated. Currently only
* `description` and `display_name` can be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The mask to control which fields get updated. Currently only `description` and
`display_name` can be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The mask to control which fields get updated. Currently only
* `description` and `display_name` can be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Generators collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Generators.List request = dialogflow.generators().list(parameters ...)}
*
*
* @return the resource collection
*/
public Generators generators() {
return new Generators();
}
/**
* The "generators" collection of methods.
*/
public class Generators {
/**
* Creates a generator in the specified agent.
*
* Create a request for the method "generators.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to create a generator for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/generators";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Creates a generator in the specified agent.
*
* Create a request for the method "generators.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to create a generator for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to create a generator for. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to create a generator for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to create a generator for. Format:
* `projects//locations//agents/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language to create generators for the following fields: *
* `Generator.prompt_text.text` If not specified, the agent's default language is used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to create generators for the following fields: * `Generator.prompt_text.text` If not
specified, the agent's default language is used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to create generators for the following fields: *
* `Generator.prompt_text.text` If not specified, the agent's default language is used.
*/
public Create setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified generators.
*
* Create a request for the method "generators.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the generator to delete. Format: `projects//locations//agents//generators/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/generators/[^/]+$");
/**
* Deletes the specified generators.
*
* Create a request for the method "generators.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the generator to delete. Format: `projects//locations//agents//generators/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generators/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the generator to delete. Format:
* `projects//locations//agents//generators/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the generator to delete. Format: `projects//locations//agents//generators/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the generator to delete. Format:
* `projects//locations//agents//generators/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generators/[^/]+$");
}
this.name = name;
return this;
}
/**
* This field has no effect for generators not being used. For generators that are used
* by pages/flows/transition route groups: * If `force` is set to false, an error will
* be returned with message indicating the referenced resources. * If `force` is set to
* true, Dialogflow will remove the generator, as well as any references to the
* generator (i.e. Generator) in fulfillments.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** This field has no effect for generators not being used. For generators that are used by
pages/flows/transition route groups: * If `force` is set to false, an error will be returned with
message indicating the referenced resources. * If `force` is set to true, Dialogflow will remove
the generator, as well as any references to the generator (i.e. Generator) in fulfillments.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* This field has no effect for generators not being used. For generators that are used
* by pages/flows/transition route groups: * If `force` is set to false, an error will
* be returned with message indicating the referenced resources. * If `force` is set to
* true, Dialogflow will remove the generator, as well as any references to the
* generator (i.e. Generator) in fulfillments.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified generator.
*
* Create a request for the method "generators.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the generator. Format: `projects//locations//agents//generators/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/generators/[^/]+$");
/**
* Retrieves the specified generator.
*
* Create a request for the method "generators.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the generator. Format: `projects//locations//agents//generators/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generators/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the generator. Format:
* `projects//locations//agents//generators/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the generator. Format: `projects//locations//agents//generators/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the generator. Format:
* `projects//locations//agents//generators/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generators/[^/]+$");
}
this.name = name;
return this;
}
/** The language to list generators for. */
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to list generators for.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/** The language to list generators for. */
public Get setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all generators in the specified agent.
*
* Create a request for the method "generators.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to list all generators for. Format: `projects//locations//agents/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/generators";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Returns the list of all generators in the specified agent.
*
* Create a request for the method "generators.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to list all generators for. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListGeneratorsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to list all generators for. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to list all generators for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to list all generators for. Format:
* `projects//locations//agents/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/** The language to list generators for. */
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to list generators for.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/** The language to list generators for. */
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Update the specified generator.
*
* Create a request for the method "generators.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the generator. Must be set for the Generators.UpdateGenerator method.
* Generators.CreateGenerate populates the name automatically. Format:
* `projects//locations//agents//generators/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/generators/[^/]+$");
/**
* Update the specified generator.
*
* Create a request for the method "generators.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the generator. Must be set for the Generators.UpdateGenerator method.
* Generators.CreateGenerate populates the name automatically. Format:
* `projects//locations//agents//generators/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Generator.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generators/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the generator. Must be set for the
* Generators.UpdateGenerator method. Generators.CreateGenerate populates the name
* automatically. Format: `projects//locations//agents//generators/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the generator. Must be set for the Generators.UpdateGenerator method.
Generators.CreateGenerate populates the name automatically. Format:
`projects//locations//agents//generators/`.
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the generator. Must be set for the
* Generators.UpdateGenerator method. Generators.CreateGenerate populates the name
* automatically. Format: `projects//locations//agents//generators/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/generators/[^/]+$");
}
this.name = name;
return this;
}
/** The language to list generators for. */
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to list generators for.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/** The language to list generators for. */
public Patch setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated. If the mask is not present, all fields will be
updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Intents collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Intents.List request = dialogflow.intents().list(parameters ...)}
*
*
* @return the resource collection
*/
public Intents intents() {
return new Intents();
}
/**
* The "intents" collection of methods.
*/
public class Intents {
/**
* Creates an intent in the specified agent. Note: You should always train a flow prior to sending
* it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "intents.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to create an intent for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/intents";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Creates an intent in the specified agent. Note: You should always train a flow prior to sending
* it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "intents.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to create an intent for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to create an intent for. Format: `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to create an intent for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to create an intent for. Format: `projects//locations//agents/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language of the following fields in `intent`: *
* `Intent.training_phrases.parts.text` If not specified, the agent's default language
* is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `intent`: * `Intent.training_phrases.parts.text` If not
specified, the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `intent`: *
* `Intent.training_phrases.parts.text` If not specified, the agent's default language
* is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Create setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified intent. Note: You should always train a flow prior to sending it queries.
* See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "intents.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the intent to delete. Format: `projects//locations//agents//intents/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/intents/[^/]+$");
/**
* Deletes the specified intent. Note: You should always train a flow prior to sending it queries.
* See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "intents.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the intent to delete. Format: `projects//locations//agents//intents/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/intents/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the intent to delete. Format:
* `projects//locations//agents//intents/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the intent to delete. Format: `projects//locations//agents//intents/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the intent to delete. Format:
* `projects//locations//agents//intents/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/intents/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Exports the selected intents. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: ExportIntentsMetadata -
* `response`: ExportIntentsResponse
*
* Create a request for the method "intents.export".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the parent agent to export intents. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportIntentsRequest}
* @return the request
*/
public Export export(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportIntentsRequest content) throws java.io.IOException {
Export result = new Export(parent, content);
initialize(result);
return result;
}
public class Export extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/intents:export";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Exports the selected intents. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`:
* ExportIntentsMetadata - `response`: ExportIntentsResponse
*
* Create a request for the method "intents.export".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Export#execute()} method to invoke the remote operation.
* {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the parent agent to export intents. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportIntentsRequest}
* @since 1.13
*/
protected Export(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportIntentsRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the parent agent to export intents. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the parent agent to export intents. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the parent agent to export intents. Format:
* `projects//locations//agents/`.
*/
public Export setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Retrieves the specified intent.
*
* Create a request for the method "intents.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the intent. Format: `projects//locations//agents//intents/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/intents/[^/]+$");
/**
* Retrieves the specified intent.
*
* Create a request for the method "intents.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the intent. Format: `projects//locations//agents//intents/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/intents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the intent. Format: `projects//locations//agents//intents/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the intent. Format: `projects//locations//agents//intents/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the intent. Format: `projects//locations//agents//intents/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/intents/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language to retrieve the intent for. The following fields are language dependent:
* * `Intent.training_phrases.parts.text` If not specified, the agent's default language
* is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to retrieve the intent for. The following fields are language dependent: *
`Intent.training_phrases.parts.text` If not specified, the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to retrieve the intent for. The following fields are language dependent:
* * `Intent.training_phrases.parts.text` If not specified, the agent's default language
* is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Get setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Imports the specified intents into the agent. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: ImportIntentsMetadata -
* `response`: ImportIntentsResponse
*
* Create a request for the method "intents.import".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link DialogflowImport#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to import the intents into. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportIntentsRequest}
* @return the request
*/
public DialogflowImport dialogflowImport(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportIntentsRequest content) throws java.io.IOException {
DialogflowImport result = new DialogflowImport(parent, content);
initialize(result);
return result;
}
public class DialogflowImport extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/intents:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Imports the specified intents into the agent. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`:
* ImportIntentsMetadata - `response`: ImportIntentsResponse
*
* Create a request for the method "intents.import".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link DialogflowImport#execute()} method to invoke the remote
* operation. {@link DialogflowImport#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The agent to import the intents into. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportIntentsRequest}
* @since 1.13
*/
protected DialogflowImport(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportIntentsRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public DialogflowImport set$Xgafv(java.lang.String $Xgafv) {
return (DialogflowImport) super.set$Xgafv($Xgafv);
}
@Override
public DialogflowImport setAccessToken(java.lang.String accessToken) {
return (DialogflowImport) super.setAccessToken(accessToken);
}
@Override
public DialogflowImport setAlt(java.lang.String alt) {
return (DialogflowImport) super.setAlt(alt);
}
@Override
public DialogflowImport setCallback(java.lang.String callback) {
return (DialogflowImport) super.setCallback(callback);
}
@Override
public DialogflowImport setFields(java.lang.String fields) {
return (DialogflowImport) super.setFields(fields);
}
@Override
public DialogflowImport setKey(java.lang.String key) {
return (DialogflowImport) super.setKey(key);
}
@Override
public DialogflowImport setOauthToken(java.lang.String oauthToken) {
return (DialogflowImport) super.setOauthToken(oauthToken);
}
@Override
public DialogflowImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DialogflowImport) super.setPrettyPrint(prettyPrint);
}
@Override
public DialogflowImport setQuotaUser(java.lang.String quotaUser) {
return (DialogflowImport) super.setQuotaUser(quotaUser);
}
@Override
public DialogflowImport setUploadType(java.lang.String uploadType) {
return (DialogflowImport) super.setUploadType(uploadType);
}
@Override
public DialogflowImport setUploadProtocol(java.lang.String uploadProtocol) {
return (DialogflowImport) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to import the intents into. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to import the intents into. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to import the intents into. Format:
* `projects//locations//agents/`.
*/
public DialogflowImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public DialogflowImport set(String parameterName, Object value) {
return (DialogflowImport) super.set(parameterName, value);
}
}
/**
* Returns the list of all intents in the specified agent.
*
* Create a request for the method "intents.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to list all intents for. Format: `projects//locations//agents/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/intents";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Returns the list of all intents in the specified agent.
*
* Create a request for the method "intents.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to list all intents for. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListIntentsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to list all intents for. Format: `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to list all intents for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to list all intents for. Format: `projects//locations//agents/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/** The resource view to apply to the returned intent. */
@com.google.api.client.util.Key
private java.lang.String intentView;
/** The resource view to apply to the returned intent.
*/
public java.lang.String getIntentView() {
return intentView;
}
/** The resource view to apply to the returned intent. */
public List setIntentView(java.lang.String intentView) {
this.intentView = intentView;
return this;
}
/**
* The language to list intents for. The following fields are language dependent: *
* `Intent.training_phrases.parts.text` If not specified, the agent's default language
* is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to list intents for. The following fields are language dependent: *
`Intent.training_phrases.parts.text` If not specified, the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to list intents for. The following fields are language dependent: *
* `Intent.training_phrases.parts.text` If not specified, the agent's default language
* is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified intent. Note: You should always train a flow prior to sending it queries.
* See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "intents.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the intent. Required for the Intents.UpdateIntent method.
* Intents.CreateIntent populates the name automatically. Format:
* `projects//locations//agents//intents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/intents/[^/]+$");
/**
* Updates the specified intent. Note: You should always train a flow prior to sending it queries.
* See the [training documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "intents.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the intent. Required for the Intents.UpdateIntent method.
* Intents.CreateIntent populates the name automatically. Format:
* `projects//locations//agents//intents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Intent.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/intents/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the intent. Required for the Intents.UpdateIntent method.
* Intents.CreateIntent populates the name automatically. Format:
* `projects//locations//agents//intents/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the intent. Required for the Intents.UpdateIntent method.
Intents.CreateIntent populates the name automatically. Format:
`projects//locations//agents//intents/`.
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the intent. Required for the Intents.UpdateIntent method.
* Intents.CreateIntent populates the name automatically. Format:
* `projects//locations//agents//intents/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/intents/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language of the following fields in `intent`: *
* `Intent.training_phrases.parts.text` If not specified, the agent's default language
* is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `intent`: * `Intent.training_phrases.parts.text` If not
specified, the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `intent`: *
* `Intent.training_phrases.parts.text` If not specified, the agent's default language
* is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Patch setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated. If the mask is not present, all fields will be
updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Sessions collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Sessions.List request = dialogflow.sessions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Sessions sessions() {
return new Sessions();
}
/**
* The "sessions" collection of methods.
*/
public class Sessions {
/**
* Processes a natural language query and returns structured, actionable data as a result. This
* method is not idempotent, because it may cause session entity types to be updated, which in turn
* might affect results of future queries. Note: Always use agent versions for production traffic.
* See [Versions and environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*
* Create a request for the method "sessions.detectIntent".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link DetectIntent#execute()} method to invoke the remote operation.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest}
* @return the request
*/
public DetectIntent detectIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest content) throws java.io.IOException {
DetectIntent result = new DetectIntent(session, content);
initialize(result);
return result;
}
public class DetectIntent extends DialogflowRequest {
private static final String REST_PATH = "v3/{+session}:detectIntent";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
/**
* Processes a natural language query and returns structured, actionable data as a result. This
* method is not idempotent, because it may cause session entity types to be updated, which in
* turn might affect results of future queries. Note: Always use agent versions for production
* traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*
* Create a request for the method "sessions.detectIntent".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link DetectIntent#execute()} method to invoke the remote
* operation. {@link
* DetectIntent#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest}
* @since 1.13
*/
protected DetectIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
}
@Override
public DetectIntent set$Xgafv(java.lang.String $Xgafv) {
return (DetectIntent) super.set$Xgafv($Xgafv);
}
@Override
public DetectIntent setAccessToken(java.lang.String accessToken) {
return (DetectIntent) super.setAccessToken(accessToken);
}
@Override
public DetectIntent setAlt(java.lang.String alt) {
return (DetectIntent) super.setAlt(alt);
}
@Override
public DetectIntent setCallback(java.lang.String callback) {
return (DetectIntent) super.setCallback(callback);
}
@Override
public DetectIntent setFields(java.lang.String fields) {
return (DetectIntent) super.setFields(fields);
}
@Override
public DetectIntent setKey(java.lang.String key) {
return (DetectIntent) super.setKey(key);
}
@Override
public DetectIntent setOauthToken(java.lang.String oauthToken) {
return (DetectIntent) super.setOauthToken(oauthToken);
}
@Override
public DetectIntent setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DetectIntent) super.setPrettyPrint(prettyPrint);
}
@Override
public DetectIntent setQuotaUser(java.lang.String quotaUser) {
return (DetectIntent) super.setQuotaUser(quotaUser);
}
@Override
public DetectIntent setUploadType(java.lang.String uploadType) {
return (DetectIntent) super.setUploadType(uploadType);
}
@Override
public DetectIntent setUploadProtocol(java.lang.String uploadProtocol) {
return (DetectIntent) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose
* an appropriate `Session ID`. It can be a random number or some type of session
* identifiers (preferably hashed). The length of the `Session ID` must not exceed 36
* characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The name of the session this query is sent to. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment. It's up to the API
caller to choose an appropriate `Session ID`. It can be a random number or some type of session
identifiers (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
more information, see the [sessions
guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use agent
versions for production traffic. See [Versions and
environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
public java.lang.String getSession() {
return session;
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose
* an appropriate `Session ID`. It can be a random number or some type of session
* identifiers (preferably hashed). The length of the `Session ID` must not exceed 36
* characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
public DetectIntent setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public DetectIntent set(String parameterName, Object value) {
return (DetectIntent) super.set(parameterName, value);
}
}
/**
* Fulfills a matched intent returned by MatchIntent. Must be called after MatchIntent, with input
* from MatchIntentResponse. Otherwise, the behavior is undefined.
*
* Create a request for the method "sessions.fulfillIntent".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link FulfillIntent#execute()} method to invoke the remote operation.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentRequest}
* @return the request
*/
public FulfillIntent fulfillIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentRequest content) throws java.io.IOException {
FulfillIntent result = new FulfillIntent(session, content);
initialize(result);
return result;
}
public class FulfillIntent extends DialogflowRequest {
private static final String REST_PATH = "v3/{+session}:fulfillIntent";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
/**
* Fulfills a matched intent returned by MatchIntent. Must be called after MatchIntent, with input
* from MatchIntentResponse. Otherwise, the behavior is undefined.
*
* Create a request for the method "sessions.fulfillIntent".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link FulfillIntent#execute()} method to invoke the remote
* operation. {@link FulfillIntent#initialize(com.google.api.client.googleapis.services.Abstra
* ctGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentRequest}
* @since 1.13
*/
protected FulfillIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3FulfillIntentResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
}
@Override
public FulfillIntent set$Xgafv(java.lang.String $Xgafv) {
return (FulfillIntent) super.set$Xgafv($Xgafv);
}
@Override
public FulfillIntent setAccessToken(java.lang.String accessToken) {
return (FulfillIntent) super.setAccessToken(accessToken);
}
@Override
public FulfillIntent setAlt(java.lang.String alt) {
return (FulfillIntent) super.setAlt(alt);
}
@Override
public FulfillIntent setCallback(java.lang.String callback) {
return (FulfillIntent) super.setCallback(callback);
}
@Override
public FulfillIntent setFields(java.lang.String fields) {
return (FulfillIntent) super.setFields(fields);
}
@Override
public FulfillIntent setKey(java.lang.String key) {
return (FulfillIntent) super.setKey(key);
}
@Override
public FulfillIntent setOauthToken(java.lang.String oauthToken) {
return (FulfillIntent) super.setOauthToken(oauthToken);
}
@Override
public FulfillIntent setPrettyPrint(java.lang.Boolean prettyPrint) {
return (FulfillIntent) super.setPrettyPrint(prettyPrint);
}
@Override
public FulfillIntent setQuotaUser(java.lang.String quotaUser) {
return (FulfillIntent) super.setQuotaUser(quotaUser);
}
@Override
public FulfillIntent setUploadType(java.lang.String uploadType) {
return (FulfillIntent) super.setUploadType(uploadType);
}
@Override
public FulfillIntent setUploadProtocol(java.lang.String uploadProtocol) {
return (FulfillIntent) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose
* an appropriate `Session ID`. It can be a random number or some type of session
* identifiers (preferably hashed). The length of the `Session ID` must not exceed 36
* characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The name of the session this query is sent to. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment. It's up to the API
caller to choose an appropriate `Session ID`. It can be a random number or some type of session
identifiers (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
more information, see the [sessions
guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
public java.lang.String getSession() {
return session;
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose
* an appropriate `Session ID`. It can be a random number or some type of session
* identifiers (preferably hashed). The length of the `Session ID` must not exceed 36
* characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
public FulfillIntent setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public FulfillIntent set(String parameterName, Object value) {
return (FulfillIntent) super.set(parameterName, value);
}
}
/**
* Returns preliminary intent match results, doesn't change the session status.
*
* Create a request for the method "sessions.matchIntent".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link MatchIntent#execute()} method to invoke the remote operation.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentRequest}
* @return the request
*/
public MatchIntent matchIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentRequest content) throws java.io.IOException {
MatchIntent result = new MatchIntent(session, content);
initialize(result);
return result;
}
public class MatchIntent extends DialogflowRequest {
private static final String REST_PATH = "v3/{+session}:matchIntent";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
/**
* Returns preliminary intent match results, doesn't change the session status.
*
* Create a request for the method "sessions.matchIntent".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link MatchIntent#execute()} method to invoke the remote
* operation. {@link
* MatchIntent#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentRequest}
* @since 1.13
*/
protected MatchIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3MatchIntentResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
}
@Override
public MatchIntent set$Xgafv(java.lang.String $Xgafv) {
return (MatchIntent) super.set$Xgafv($Xgafv);
}
@Override
public MatchIntent setAccessToken(java.lang.String accessToken) {
return (MatchIntent) super.setAccessToken(accessToken);
}
@Override
public MatchIntent setAlt(java.lang.String alt) {
return (MatchIntent) super.setAlt(alt);
}
@Override
public MatchIntent setCallback(java.lang.String callback) {
return (MatchIntent) super.setCallback(callback);
}
@Override
public MatchIntent setFields(java.lang.String fields) {
return (MatchIntent) super.setFields(fields);
}
@Override
public MatchIntent setKey(java.lang.String key) {
return (MatchIntent) super.setKey(key);
}
@Override
public MatchIntent setOauthToken(java.lang.String oauthToken) {
return (MatchIntent) super.setOauthToken(oauthToken);
}
@Override
public MatchIntent setPrettyPrint(java.lang.Boolean prettyPrint) {
return (MatchIntent) super.setPrettyPrint(prettyPrint);
}
@Override
public MatchIntent setQuotaUser(java.lang.String quotaUser) {
return (MatchIntent) super.setQuotaUser(quotaUser);
}
@Override
public MatchIntent setUploadType(java.lang.String uploadType) {
return (MatchIntent) super.setUploadType(uploadType);
}
@Override
public MatchIntent setUploadProtocol(java.lang.String uploadProtocol) {
return (MatchIntent) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose
* an appropriate `Session ID`. It can be a random number or some type of session
* identifiers (preferably hashed). The length of the `Session ID` must not exceed 36
* characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The name of the session this query is sent to. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment. It's up to the API
caller to choose an appropriate `Session ID`. It can be a random number or some type of session
identifiers (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
more information, see the [sessions
guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
public java.lang.String getSession() {
return session;
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose
* an appropriate `Session ID`. It can be a random number or some type of session
* identifiers (preferably hashed). The length of the `Session ID` must not exceed 36
* characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session).
*/
public MatchIntent setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public MatchIntent set(String parameterName, Object value) {
return (MatchIntent) super.set(parameterName, value);
}
}
/**
* Processes a natural language query and returns structured, actionable data as a result through
* server-side streaming. Server-side streaming allows Dialogflow to send [partial
* responses](https://cloud.google.com/dialogflow/cx/docs/concept/fulfillment#partial-response)
* earlier in a single request.
*
* Create a request for the method "sessions.serverStreamingDetectIntent".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link ServerStreamingDetectIntent#execute()} method to invoke the remote
* operation.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest}
* @return the request
*/
public ServerStreamingDetectIntent serverStreamingDetectIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest content) throws java.io.IOException {
ServerStreamingDetectIntent result = new ServerStreamingDetectIntent(session, content);
initialize(result);
return result;
}
public class ServerStreamingDetectIntent extends DialogflowRequest {
private static final String REST_PATH = "v3/{+session}:serverStreamingDetectIntent";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
/**
* Processes a natural language query and returns structured, actionable data as a result through
* server-side streaming. Server-side streaming allows Dialogflow to send [partial
* responses](https://cloud.google.com/dialogflow/cx/docs/concept/fulfillment#partial-response)
* earlier in a single request.
*
* Create a request for the method "sessions.serverStreamingDetectIntent".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link ServerStreamingDetectIntent#execute()} method to invoke
* the remote operation. {@link ServerStreamingDetectIntent#initialize(com.google.api.client.g
* oogleapis.services.AbstractGoogleClientRequest)} must be called to initialize this instance
* immediately after invoking the constructor.
*
* @param session Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose an
* appropriate `Session ID`. It can be a random number or some type of session identifiers
* (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
* more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest}
* @since 1.13
*/
protected ServerStreamingDetectIntent(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DetectIntentResponse.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
}
@Override
public ServerStreamingDetectIntent set$Xgafv(java.lang.String $Xgafv) {
return (ServerStreamingDetectIntent) super.set$Xgafv($Xgafv);
}
@Override
public ServerStreamingDetectIntent setAccessToken(java.lang.String accessToken) {
return (ServerStreamingDetectIntent) super.setAccessToken(accessToken);
}
@Override
public ServerStreamingDetectIntent setAlt(java.lang.String alt) {
return (ServerStreamingDetectIntent) super.setAlt(alt);
}
@Override
public ServerStreamingDetectIntent setCallback(java.lang.String callback) {
return (ServerStreamingDetectIntent) super.setCallback(callback);
}
@Override
public ServerStreamingDetectIntent setFields(java.lang.String fields) {
return (ServerStreamingDetectIntent) super.setFields(fields);
}
@Override
public ServerStreamingDetectIntent setKey(java.lang.String key) {
return (ServerStreamingDetectIntent) super.setKey(key);
}
@Override
public ServerStreamingDetectIntent setOauthToken(java.lang.String oauthToken) {
return (ServerStreamingDetectIntent) super.setOauthToken(oauthToken);
}
@Override
public ServerStreamingDetectIntent setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ServerStreamingDetectIntent) super.setPrettyPrint(prettyPrint);
}
@Override
public ServerStreamingDetectIntent setQuotaUser(java.lang.String quotaUser) {
return (ServerStreamingDetectIntent) super.setQuotaUser(quotaUser);
}
@Override
public ServerStreamingDetectIntent setUploadType(java.lang.String uploadType) {
return (ServerStreamingDetectIntent) super.setUploadType(uploadType);
}
@Override
public ServerStreamingDetectIntent setUploadProtocol(java.lang.String uploadProtocol) {
return (ServerStreamingDetectIntent) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose
* an appropriate `Session ID`. It can be a random number or some type of session
* identifiers (preferably hashed). The length of the `Session ID` must not exceed 36
* characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The name of the session this query is sent to. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment. It's up to the API
caller to choose an appropriate `Session ID`. It can be a random number or some type of session
identifiers (preferably hashed). The length of the `Session ID` must not exceed 36 characters. For
more information, see the [sessions
guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use agent
versions for production traffic. See [Versions and
environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
public java.lang.String getSession() {
return session;
}
/**
* Required. The name of the session this query is sent to. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment. It's up to the API caller to choose
* an appropriate `Session ID`. It can be a random number or some type of session
* identifiers (preferably hashed). The length of the `Session ID` must not exceed 36
* characters. For more information, see the [sessions
* guide](https://cloud.google.com/dialogflow/cx/docs/concept/session). Note: Always use
* agent versions for production traffic. See [Versions and
* environments](https://cloud.google.com/dialogflow/cx/docs/concept/version).
*/
public ServerStreamingDetectIntent setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public ServerStreamingDetectIntent set(String parameterName, Object value) {
return (ServerStreamingDetectIntent) super.set(parameterName, value);
}
}
/**
* Updates the feedback received from the user for a single turn of the bot response.
*
* Create a request for the method "sessions.submitAnswerFeedback".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link SubmitAnswerFeedback#execute()} method to invoke the remote
* operation.
*
* @param session Required. The name of the session the feedback was sent to.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SubmitAnswerFeedbackRequest}
* @return the request
*/
public SubmitAnswerFeedback submitAnswerFeedback(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SubmitAnswerFeedbackRequest content) throws java.io.IOException {
SubmitAnswerFeedback result = new SubmitAnswerFeedback(session, content);
initialize(result);
return result;
}
public class SubmitAnswerFeedback extends DialogflowRequest {
private static final String REST_PATH = "v3/{+session}:submitAnswerFeedback";
private final java.util.regex.Pattern SESSION_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
/**
* Updates the feedback received from the user for a single turn of the bot response.
*
* Create a request for the method "sessions.submitAnswerFeedback".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link SubmitAnswerFeedback#execute()} method to invoke the
* remote operation. {@link SubmitAnswerFeedback#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param session Required. The name of the session the feedback was sent to.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SubmitAnswerFeedbackRequest}
* @since 1.13
*/
protected SubmitAnswerFeedback(java.lang.String session, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SubmitAnswerFeedbackRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3AnswerFeedback.class);
this.session = com.google.api.client.util.Preconditions.checkNotNull(session, "Required parameter session must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
}
@Override
public SubmitAnswerFeedback set$Xgafv(java.lang.String $Xgafv) {
return (SubmitAnswerFeedback) super.set$Xgafv($Xgafv);
}
@Override
public SubmitAnswerFeedback setAccessToken(java.lang.String accessToken) {
return (SubmitAnswerFeedback) super.setAccessToken(accessToken);
}
@Override
public SubmitAnswerFeedback setAlt(java.lang.String alt) {
return (SubmitAnswerFeedback) super.setAlt(alt);
}
@Override
public SubmitAnswerFeedback setCallback(java.lang.String callback) {
return (SubmitAnswerFeedback) super.setCallback(callback);
}
@Override
public SubmitAnswerFeedback setFields(java.lang.String fields) {
return (SubmitAnswerFeedback) super.setFields(fields);
}
@Override
public SubmitAnswerFeedback setKey(java.lang.String key) {
return (SubmitAnswerFeedback) super.setKey(key);
}
@Override
public SubmitAnswerFeedback setOauthToken(java.lang.String oauthToken) {
return (SubmitAnswerFeedback) super.setOauthToken(oauthToken);
}
@Override
public SubmitAnswerFeedback setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SubmitAnswerFeedback) super.setPrettyPrint(prettyPrint);
}
@Override
public SubmitAnswerFeedback setQuotaUser(java.lang.String quotaUser) {
return (SubmitAnswerFeedback) super.setQuotaUser(quotaUser);
}
@Override
public SubmitAnswerFeedback setUploadType(java.lang.String uploadType) {
return (SubmitAnswerFeedback) super.setUploadType(uploadType);
}
@Override
public SubmitAnswerFeedback setUploadProtocol(java.lang.String uploadProtocol) {
return (SubmitAnswerFeedback) super.setUploadProtocol(uploadProtocol);
}
/** Required. The name of the session the feedback was sent to. */
@com.google.api.client.util.Key
private java.lang.String session;
/** Required. The name of the session the feedback was sent to.
*/
public java.lang.String getSession() {
return session;
}
/** Required. The name of the session the feedback was sent to. */
public SubmitAnswerFeedback setSession(java.lang.String session) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SESSION_PATTERN.matcher(session).matches(),
"Parameter session must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
this.session = session;
return this;
}
@Override
public SubmitAnswerFeedback set(String parameterName, Object value) {
return (SubmitAnswerFeedback) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the EntityTypes collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.EntityTypes.List request = dialogflow.entityTypes().list(parameters ...)}
*
*
* @return the resource collection
*/
public EntityTypes entityTypes() {
return new EntityTypes();
}
/**
* The "entityTypes" collection of methods.
*/
public class EntityTypes {
/**
* Creates a session entity type.
*
* Create a request for the method "entityTypes.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The session to create a session entity type for. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/entityTypes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
/**
* Creates a session entity type.
*
* Create a request for the method "entityTypes.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The session to create a session entity type for. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The session to create a session entity type for. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The session to create a session entity type for. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The session to create a session entity type for. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified session entity type.
*
* Create a request for the method "entityTypes.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the session entity type to delete. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
/**
* Deletes the specified session entity type.
*
* Create a request for the method "entityTypes.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the session entity type to delete. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session entity type to delete. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the session entity type to delete. Format:
`projects//locations//agents//sessions//entityTypes/` or
`projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID` is not
specified, we assume default 'draft' environment.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the session entity type to delete. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified session entity type.
*
* Create a request for the method "entityTypes.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
/**
* Retrieves the specified session entity type.
*
* Create a request for the method "entityTypes.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the session entity type. Format:
`projects//locations//agents//sessions//entityTypes/` or
`projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID` is not
specified, we assume default 'draft' environment.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all session entity types in the specified session.
*
* Create a request for the method "entityTypes.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The session to list all session entity types from. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/entityTypes";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
/**
* Returns the list of all session entity types in the specified session.
*
* Create a request for the method "entityTypes.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The session to list all session entity types from. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListSessionEntityTypesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The session to list all session entity types from. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The session to list all session entity types from. Format:
`projects//locations//agents//sessions/` or `projects//locations//agents//environments//sessions/`.
If `Environment ID` is not specified, we assume default 'draft' environment.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The session to list all session entity types from. Format:
* `projects//locations//agents//sessions/` or
* `projects//locations//agents//environments//sessions/`. If `Environment ID` is not
* specified, we assume default 'draft' environment.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified session entity type.
*
* Create a request for the method "entityTypes.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Required. The unique identifier of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
/**
* Updates the specified session entity type.
*
* Create a request for the method "entityTypes.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The unique identifier of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID`
* is not specified, we assume default 'draft' environment.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SessionEntityType.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The unique identifier of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The unique identifier of the session entity type. Format:
`projects//locations//agents//sessions//entityTypes/` or
`projects//locations//agents//environments//sessions//entityTypes/`. If `Environment ID` is not
specified, we assume default 'draft' environment.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The unique identifier of the session entity type. Format:
* `projects//locations//agents//sessions//entityTypes/` or
* `projects//locations//agents//environments//sessions//entityTypes/`. If
* `Environment ID` is not specified, we assume default 'draft' environment.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/sessions/[^/]+/entityTypes/[^/]+$");
}
this.name = name;
return this;
}
/** The mask to control which fields get updated. */
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** The mask to control which fields get updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the TestCases collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.TestCases.List request = dialogflow.testCases().list(parameters ...)}
*
*
* @return the resource collection
*/
public TestCases testCases() {
return new TestCases();
}
/**
* The "testCases" collection of methods.
*/
public class TestCases {
/**
* Batch deletes test cases.
*
* Create a request for the method "testCases.batchDelete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to delete test cases from. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3BatchDeleteTestCasesRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3BatchDeleteTestCasesRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(parent, content);
initialize(result);
return result;
}
public class BatchDelete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/testCases:batchDelete";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Batch deletes test cases.
*
* Create a request for the method "testCases.batchDelete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link BatchDelete#execute()} method to invoke the remote
* operation. {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to delete test cases from. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3BatchDeleteTestCasesRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3BatchDeleteTestCasesRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public BatchDelete set$Xgafv(java.lang.String $Xgafv) {
return (BatchDelete) super.set$Xgafv($Xgafv);
}
@Override
public BatchDelete setAccessToken(java.lang.String accessToken) {
return (BatchDelete) super.setAccessToken(accessToken);
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setCallback(java.lang.String callback) {
return (BatchDelete) super.setCallback(callback);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUploadType(java.lang.String uploadType) {
return (BatchDelete) super.setUploadType(uploadType);
}
@Override
public BatchDelete setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchDelete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to delete test cases from. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to delete test cases from. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to delete test cases from. Format:
* `projects//locations//agents/`.
*/
public BatchDelete setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Kicks off a batch run of test cases. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`:
* BatchRunTestCasesMetadata - `response`: BatchRunTestCasesResponse
*
* Create a request for the method "testCases.batchRun".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link BatchRun#execute()} method to invoke the remote operation.
*
* @param parent Required. Agent name. Format: `projects//locations//agents/ `.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3BatchRunTestCasesRequest}
* @return the request
*/
public BatchRun batchRun(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3BatchRunTestCasesRequest content) throws java.io.IOException {
BatchRun result = new BatchRun(parent, content);
initialize(result);
return result;
}
public class BatchRun extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/testCases:batchRun";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Kicks off a batch run of test cases. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`:
* BatchRunTestCasesMetadata - `response`: BatchRunTestCasesResponse
*
* Create a request for the method "testCases.batchRun".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link BatchRun#execute()} method to invoke the remote operation.
* {@link
* BatchRun#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Agent name. Format: `projects//locations//agents/ `.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3BatchRunTestCasesRequest}
* @since 1.13
*/
protected BatchRun(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3BatchRunTestCasesRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public BatchRun set$Xgafv(java.lang.String $Xgafv) {
return (BatchRun) super.set$Xgafv($Xgafv);
}
@Override
public BatchRun setAccessToken(java.lang.String accessToken) {
return (BatchRun) super.setAccessToken(accessToken);
}
@Override
public BatchRun setAlt(java.lang.String alt) {
return (BatchRun) super.setAlt(alt);
}
@Override
public BatchRun setCallback(java.lang.String callback) {
return (BatchRun) super.setCallback(callback);
}
@Override
public BatchRun setFields(java.lang.String fields) {
return (BatchRun) super.setFields(fields);
}
@Override
public BatchRun setKey(java.lang.String key) {
return (BatchRun) super.setKey(key);
}
@Override
public BatchRun setOauthToken(java.lang.String oauthToken) {
return (BatchRun) super.setOauthToken(oauthToken);
}
@Override
public BatchRun setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchRun) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchRun setQuotaUser(java.lang.String quotaUser) {
return (BatchRun) super.setQuotaUser(quotaUser);
}
@Override
public BatchRun setUploadType(java.lang.String uploadType) {
return (BatchRun) super.setUploadType(uploadType);
}
@Override
public BatchRun setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchRun) super.setUploadProtocol(uploadProtocol);
}
/** Required. Agent name. Format: `projects//locations//agents/ `. */
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Agent name. Format: `projects//locations//agents/ `.
*/
public java.lang.String getParent() {
return parent;
}
/** Required. Agent name. Format: `projects//locations//agents/ `. */
public BatchRun setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public BatchRun set(String parameterName, Object value) {
return (BatchRun) super.set(parameterName, value);
}
}
/**
* Calculates the test coverage for an agent.
*
* Create a request for the method "testCases.calculateCoverage".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link CalculateCoverage#execute()} method to invoke the remote operation.
*
* @param agent Required. The agent to calculate coverage for. Format: `projects//locations//agents/`.
* @return the request
*/
public CalculateCoverage calculateCoverage(java.lang.String agent) throws java.io.IOException {
CalculateCoverage result = new CalculateCoverage(agent);
initialize(result);
return result;
}
public class CalculateCoverage extends DialogflowRequest {
private static final String REST_PATH = "v3/{+agent}/testCases:calculateCoverage";
private final java.util.regex.Pattern AGENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Calculates the test coverage for an agent.
*
* Create a request for the method "testCases.calculateCoverage".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link CalculateCoverage#execute()} method to invoke the remote
* operation. {@link CalculateCoverage#initialize(com.google.api.client.googleapis.services.Ab
* stractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param agent Required. The agent to calculate coverage for. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected CalculateCoverage(java.lang.String agent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3CalculateCoverageResponse.class);
this.agent = com.google.api.client.util.Preconditions.checkNotNull(agent, "Required parameter agent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(AGENT_PATTERN.matcher(agent).matches(),
"Parameter agent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public CalculateCoverage set$Xgafv(java.lang.String $Xgafv) {
return (CalculateCoverage) super.set$Xgafv($Xgafv);
}
@Override
public CalculateCoverage setAccessToken(java.lang.String accessToken) {
return (CalculateCoverage) super.setAccessToken(accessToken);
}
@Override
public CalculateCoverage setAlt(java.lang.String alt) {
return (CalculateCoverage) super.setAlt(alt);
}
@Override
public CalculateCoverage setCallback(java.lang.String callback) {
return (CalculateCoverage) super.setCallback(callback);
}
@Override
public CalculateCoverage setFields(java.lang.String fields) {
return (CalculateCoverage) super.setFields(fields);
}
@Override
public CalculateCoverage setKey(java.lang.String key) {
return (CalculateCoverage) super.setKey(key);
}
@Override
public CalculateCoverage setOauthToken(java.lang.String oauthToken) {
return (CalculateCoverage) super.setOauthToken(oauthToken);
}
@Override
public CalculateCoverage setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CalculateCoverage) super.setPrettyPrint(prettyPrint);
}
@Override
public CalculateCoverage setQuotaUser(java.lang.String quotaUser) {
return (CalculateCoverage) super.setQuotaUser(quotaUser);
}
@Override
public CalculateCoverage setUploadType(java.lang.String uploadType) {
return (CalculateCoverage) super.setUploadType(uploadType);
}
@Override
public CalculateCoverage setUploadProtocol(java.lang.String uploadProtocol) {
return (CalculateCoverage) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to calculate coverage for. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String agent;
/** Required. The agent to calculate coverage for. Format: `projects//locations//agents/`.
*/
public java.lang.String getAgent() {
return agent;
}
/**
* Required. The agent to calculate coverage for. Format:
* `projects//locations//agents/`.
*/
public CalculateCoverage setAgent(java.lang.String agent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(AGENT_PATTERN.matcher(agent).matches(),
"Parameter agent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.agent = agent;
return this;
}
/** Required. The type of coverage requested. */
@com.google.api.client.util.Key
private java.lang.String type;
/** Required. The type of coverage requested.
*/
public java.lang.String getType() {
return type;
}
/** Required. The type of coverage requested. */
public CalculateCoverage setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public CalculateCoverage set(String parameterName, Object value) {
return (CalculateCoverage) super.set(parameterName, value);
}
}
/**
* Creates a test case for the given agent.
*
* Create a request for the method "testCases.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to create the test case for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/testCases";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Creates a test case for the given agent.
*
* Create a request for the method "testCases.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to create the test case for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to create the test case for. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to create the test case for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to create the test case for. Format:
* `projects//locations//agents/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Exports the test cases under the agent to a Cloud Storage bucket or a local file. Filter can be
* applied to export a subset of test cases. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: ExportTestCasesMetadata
* - `response`: ExportTestCasesResponse
*
* Create a request for the method "testCases.export".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent where to export test cases from. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportTestCasesRequest}
* @return the request
*/
public Export export(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportTestCasesRequest content) throws java.io.IOException {
Export result = new Export(parent, content);
initialize(result);
return result;
}
public class Export extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/testCases:export";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Exports the test cases under the agent to a Cloud Storage bucket or a local file. Filter can be
* applied to export a subset of test cases. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`:
* ExportTestCasesMetadata - `response`: ExportTestCasesResponse
*
* Create a request for the method "testCases.export".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Export#execute()} method to invoke the remote operation.
* {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent where to export test cases from. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportTestCasesRequest}
* @since 1.13
*/
protected Export(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ExportTestCasesRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent where to export test cases from. Format:
* `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent where to export test cases from. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent where to export test cases from. Format:
* `projects//locations//agents/`.
*/
public Export setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Gets a test case.
*
* Create a request for the method "testCases.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the testcase. Format: `projects//locations//agents//testCases/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
/**
* Gets a test case.
*
* Create a request for the method "testCases.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the testcase. Format: `projects//locations//agents//testCases/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the testcase. Format:
* `projects//locations//agents//testCases/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the testcase. Format: `projects//locations//agents//testCases/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the testcase. Format:
* `projects//locations//agents//testCases/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Imports the test cases from a Cloud Storage bucket or a local file. It always creates new test
* cases and won't overwrite any existing ones. The provided ID in the imported test case is
* neglected. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: ImportTestCasesMetadata
* - `response`: ImportTestCasesResponse
*
* Create a request for the method "testCases.import".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link DialogflowImport#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to import test cases to. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportTestCasesRequest}
* @return the request
*/
public DialogflowImport dialogflowImport(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportTestCasesRequest content) throws java.io.IOException {
DialogflowImport result = new DialogflowImport(parent, content);
initialize(result);
return result;
}
public class DialogflowImport extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/testCases:import";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Imports the test cases from a Cloud Storage bucket or a local file. It always creates new test
* cases and won't overwrite any existing ones. The provided ID in the imported test case is
* neglected. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`:
* ImportTestCasesMetadata - `response`: ImportTestCasesResponse
*
* Create a request for the method "testCases.import".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link DialogflowImport#execute()} method to invoke the remote
* operation. {@link DialogflowImport#initialize(com.google.api.client.googleapis.services.Abs
* tractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param parent Required. The agent to import test cases to. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportTestCasesRequest}
* @since 1.13
*/
protected DialogflowImport(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ImportTestCasesRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public DialogflowImport set$Xgafv(java.lang.String $Xgafv) {
return (DialogflowImport) super.set$Xgafv($Xgafv);
}
@Override
public DialogflowImport setAccessToken(java.lang.String accessToken) {
return (DialogflowImport) super.setAccessToken(accessToken);
}
@Override
public DialogflowImport setAlt(java.lang.String alt) {
return (DialogflowImport) super.setAlt(alt);
}
@Override
public DialogflowImport setCallback(java.lang.String callback) {
return (DialogflowImport) super.setCallback(callback);
}
@Override
public DialogflowImport setFields(java.lang.String fields) {
return (DialogflowImport) super.setFields(fields);
}
@Override
public DialogflowImport setKey(java.lang.String key) {
return (DialogflowImport) super.setKey(key);
}
@Override
public DialogflowImport setOauthToken(java.lang.String oauthToken) {
return (DialogflowImport) super.setOauthToken(oauthToken);
}
@Override
public DialogflowImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (DialogflowImport) super.setPrettyPrint(prettyPrint);
}
@Override
public DialogflowImport setQuotaUser(java.lang.String quotaUser) {
return (DialogflowImport) super.setQuotaUser(quotaUser);
}
@Override
public DialogflowImport setUploadType(java.lang.String uploadType) {
return (DialogflowImport) super.setUploadType(uploadType);
}
@Override
public DialogflowImport setUploadProtocol(java.lang.String uploadProtocol) {
return (DialogflowImport) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to import test cases to. Format: `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to import test cases to. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to import test cases to. Format: `projects//locations//agents/`.
*/
public DialogflowImport setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public DialogflowImport set(String parameterName, Object value) {
return (DialogflowImport) super.set(parameterName, value);
}
}
/**
* Fetches a list of test cases for a given agent.
*
* Create a request for the method "testCases.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to list all pages for. Format: `projects//locations//agents/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/testCases";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Fetches a list of test cases for a given agent.
*
* Create a request for the method "testCases.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to list all pages for. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListTestCasesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to list all pages for. Format: `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to list all pages for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to list all pages for. Format: `projects//locations//agents/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 20. Note that when
* TestCaseView = FULL, the maximum page size allowed is 20. When TestCaseView = BASIC,
* the maximum page size allowed is 500.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 20. Note that when TestCaseView
= FULL, the maximum page size allowed is 20. When TestCaseView = BASIC, the maximum page size
allowed is 500.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 20. Note that when
* TestCaseView = FULL, the maximum page size allowed is 20. When TestCaseView = BASIC,
* the maximum page size allowed is 500.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/** Specifies whether response should include all fields or just the metadata. */
@com.google.api.client.util.Key
private java.lang.String view;
/** Specifies whether response should include all fields or just the metadata.
*/
public java.lang.String getView() {
return view;
}
/** Specifies whether response should include all fields or just the metadata. */
public List setView(java.lang.String view) {
this.view = view;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified test case.
*
* Create a request for the method "testCases.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the test case. TestCases.CreateTestCase will populate the name
* automatically. Otherwise use format: `projects//locations//agents/ /testCases/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
/**
* Updates the specified test case.
*
* Create a request for the method "testCases.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the test case. TestCases.CreateTestCase will populate the name
* automatically. Otherwise use format: `projects//locations//agents/ /testCases/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCase.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the test case. TestCases.CreateTestCase will populate the
* name automatically. Otherwise use format: `projects//locations//agents/ /testCases/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the test case. TestCases.CreateTestCase will populate the name
automatically. Otherwise use format: `projects//locations//agents/ /testCases/`.
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the test case. TestCases.CreateTestCase will populate the
* name automatically. Otherwise use format: `projects//locations//agents/ /testCases/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. The mask to specify which fields should be updated. The `creationTime` and
* `lastTestResult` cannot be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The mask to specify which fields should be updated. The `creationTime` and
`lastTestResult` cannot be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The mask to specify which fields should be updated. The `creationTime` and
* `lastTestResult` cannot be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Kicks off a test case run. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The returned
* `Operation` type has the following method-specific fields: - `metadata`: RunTestCaseMetadata -
* `response`: RunTestCaseResponse
*
* Create a request for the method "testCases.run".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Run#execute()} method to invoke the remote operation.
*
* @param name Required. Format of test case name to run: `projects//locations/ /agents//testCases/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RunTestCaseRequest}
* @return the request
*/
public Run run(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RunTestCaseRequest content) throws java.io.IOException {
Run result = new Run(name, content);
initialize(result);
return result;
}
public class Run extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:run";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
/**
* Kicks off a test case run. This method is a [long-running
* operation](https://cloud.google.com/dialogflow/cx/docs/how/long-running-operation). The
* returned `Operation` type has the following method-specific fields: - `metadata`:
* RunTestCaseMetadata - `response`: RunTestCaseResponse
*
* Create a request for the method "testCases.run".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Run#execute()} method to invoke the remote operation.
* {@link Run#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Format of test case name to run: `projects//locations/ /agents//testCases/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RunTestCaseRequest}
* @since 1.13
*/
protected Run(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3RunTestCaseRequest content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
}
}
@Override
public Run set$Xgafv(java.lang.String $Xgafv) {
return (Run) super.set$Xgafv($Xgafv);
}
@Override
public Run setAccessToken(java.lang.String accessToken) {
return (Run) super.setAccessToken(accessToken);
}
@Override
public Run setAlt(java.lang.String alt) {
return (Run) super.setAlt(alt);
}
@Override
public Run setCallback(java.lang.String callback) {
return (Run) super.setCallback(callback);
}
@Override
public Run setFields(java.lang.String fields) {
return (Run) super.setFields(fields);
}
@Override
public Run setKey(java.lang.String key) {
return (Run) super.setKey(key);
}
@Override
public Run setOauthToken(java.lang.String oauthToken) {
return (Run) super.setOauthToken(oauthToken);
}
@Override
public Run setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Run) super.setPrettyPrint(prettyPrint);
}
@Override
public Run setQuotaUser(java.lang.String quotaUser) {
return (Run) super.setQuotaUser(quotaUser);
}
@Override
public Run setUploadType(java.lang.String uploadType) {
return (Run) super.setUploadType(uploadType);
}
@Override
public Run setUploadProtocol(java.lang.String uploadProtocol) {
return (Run) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Format of test case name to run: `projects//locations/
* /agents//testCases/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Format of test case name to run: `projects//locations/ /agents//testCases/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Format of test case name to run: `projects//locations/
* /agents//testCases/`.
*/
public Run setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Run set(String parameterName, Object value) {
return (Run) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Results collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Results.List request = dialogflow.results().list(parameters ...)}
*
*
* @return the resource collection
*/
public Results results() {
return new Results();
}
/**
* The "results" collection of methods.
*/
public class Results {
/**
* Gets a test case result.
*
* Create a request for the method "results.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the testcase. Format: `projects//locations//agents//testCases//results/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+/results/[^/]+$");
/**
* Gets a test case result.
*
* Create a request for the method "results.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the testcase. Format: `projects//locations//agents//testCases//results/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TestCaseResult.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+/results/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the testcase. Format:
* `projects//locations//agents//testCases//results/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the testcase. Format: `projects//locations//agents//testCases//results/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the testcase. Format:
* `projects//locations//agents//testCases//results/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+/results/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Fetches the list of run results for the given test case. A maximum of 100 results are kept for
* each test case.
*
* Create a request for the method "results.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The test case to list results for. Format: `projects//locations//agents// testCases/`.
* Specify a `-` as a wildcard for TestCase ID to list results across multiple test cases.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/results";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
/**
* Fetches the list of run results for the given test case. A maximum of 100 results are kept for
* each test case.
*
* Create a request for the method "results.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The test case to list results for. Format: `projects//locations//agents// testCases/`.
* Specify a `-` as a wildcard for TestCase ID to list results across multiple test cases.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListTestCaseResultsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The test case to list results for. Format: `projects//locations//agents//
* testCases/`. Specify a `-` as a wildcard for TestCase ID to list results across
* multiple test cases.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The test case to list results for. Format: `projects//locations//agents// testCases/`.
Specify a `-` as a wildcard for TestCase ID to list results across multiple test cases.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The test case to list results for. Format: `projects//locations//agents//
* testCases/`. Specify a `-` as a wildcard for TestCase ID to list results across
* multiple test cases.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/testCases/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The filter expression used to filter test case results. See [API
* Filtering](https://aip.dev/160). The expression is case insensitive. Only 'AND' is
* supported for logical operators. The supported syntax is listed below in detail:
* [AND ] ... [AND latest] The supported fields and operators are: field operator
* `environment` `=`, `IN` (Use value `draft` for draft environment) `test_time` `>`,
* `<` `latest` only returns the latest test result in all results for each test case.
* Examples: * "environment=draft AND latest" matches the latest test result for each
* test case in the draft environment. * "environment IN (e1,e2)" matches any test
* case results with an environment resource name of either "e1" or "e2". * "test_time
* > 1602540713" matches any test case results with test time later than a unix
* timestamp in seconds 1602540713.
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** The filter expression used to filter test case results. See [API Filtering](https://aip.dev/160).
The expression is case insensitive. Only 'AND' is supported for logical operators. The supported
syntax is listed below in detail: [AND ] ... [AND latest] The supported fields and operators are:
field operator `environment` `=`, `IN` (Use value `draft` for draft environment) `test_time` `>`,
`<` `latest` only returns the latest test result in all results for each test case. Examples: *
"environment=draft AND latest" matches the latest test result for each test case in the draft
environment. * "environment IN (e1,e2)" matches any test case results with an environment resource
name of either "e1" or "e2". * "test_time > 1602540713" matches any test case results with test
time later than a unix timestamp in seconds 1602540713.
*/
public java.lang.String getFilter() {
return filter;
}
/**
* The filter expression used to filter test case results. See [API
* Filtering](https://aip.dev/160). The expression is case insensitive. Only 'AND' is
* supported for logical operators. The supported syntax is listed below in detail:
* [AND ] ... [AND latest] The supported fields and operators are: field operator
* `environment` `=`, `IN` (Use value `draft` for draft environment) `test_time` `>`,
* `<` `latest` only returns the latest test result in all results for each test case.
* Examples: * "environment=draft AND latest" matches the latest test result for each
* test case in the draft environment. * "environment IN (e1,e2)" matches any test
* case results with an environment resource name of either "e1" or "e2". * "test_time
* > 1602540713" matches any test case results with test time later than a unix
* timestamp in seconds 1602540713.
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the TransitionRouteGroups collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.TransitionRouteGroups.List request = dialogflow.transitionRouteGroups().list(parameters ...)}
*
*
* @return the resource collection
*/
public TransitionRouteGroups transitionRouteGroups() {
return new TransitionRouteGroups();
}
/**
* The "transitionRouteGroups" collection of methods.
*/
public class TransitionRouteGroups {
/**
* Creates an TransitionRouteGroup in the specified flow. Note: You should always train a flow prior
* to sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The flow to create an TransitionRouteGroup for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/` for agent-level
* groups.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/transitionRouteGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Creates an TransitionRouteGroup in the specified flow. Note: You should always train a flow
* prior to sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The flow to create an TransitionRouteGroup for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/` for agent-level
* groups.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The flow to create an TransitionRouteGroup for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/` for agent-
* level groups.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The flow to create an TransitionRouteGroup for. Format:
`projects//locations//agents//flows/` or `projects//locations//agents/` for agent-level groups.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The flow to create an TransitionRouteGroup for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/` for agent-
* level groups.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language of the following fields in `TransitionRouteGroup`: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `TransitionRouteGroup`: *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `TransitionRouteGroup`: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Create setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified TransitionRouteGroup. Note: You should always train a flow prior to sending
* it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the TransitionRouteGroup to delete. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/transitionRouteGroups/[^/]+$");
/**
* Deletes the specified TransitionRouteGroup. Note: You should always train a flow prior to
* sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the TransitionRouteGroup to delete. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/transitionRouteGroups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the TransitionRouteGroup to delete. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the TransitionRouteGroup to delete. Format:
`projects//locations//agents//flows//transitionRouteGroups/` or
`projects//locations//agents//transitionRouteGroups/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the TransitionRouteGroup to delete. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/transitionRouteGroups/[^/]+$");
}
this.name = name;
return this;
}
/**
* This field has no effect for transition route group that no page is using. If the
* transition route group is referenced by any page: * If `force` is set to false, an
* error will be returned with message indicating pages that reference the transition
* route group. * If `force` is set to true, Dialogflow will remove the transition route
* group, as well as any reference to it.
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** This field has no effect for transition route group that no page is using. If the transition route
group is referenced by any page: * If `force` is set to false, an error will be returned with
message indicating pages that reference the transition route group. * If `force` is set to true,
Dialogflow will remove the transition route group, as well as any reference to it.
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* This field has no effect for transition route group that no page is using. If the
* transition route group is referenced by any page: * If `force` is set to false, an
* error will be returned with message indicating pages that reference the transition
* route group. * If `force` is set to true, Dialogflow will remove the transition route
* group, as well as any reference to it.
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified TransitionRouteGroup.
*
* Create a request for the method "transitionRouteGroups.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the TransitionRouteGroup. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/transitionRouteGroups/[^/]+$");
/**
* Retrieves the specified TransitionRouteGroup.
*
* Create a request for the method "transitionRouteGroups.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the TransitionRouteGroup. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/transitionRouteGroups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the TransitionRouteGroup. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the TransitionRouteGroup. Format:
`projects//locations//agents//flows//transitionRouteGroups/` or
`projects//locations//agents//transitionRouteGroups/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the TransitionRouteGroup. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` or
* `projects//locations//agents//transitionRouteGroups/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/transitionRouteGroups/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language to retrieve the transition route group for. The following fields are
* language dependent: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to retrieve the transition route group for. The following fields are language
dependent: * `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to retrieve the transition route group for. The following fields are
* language dependent: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Get setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all transition route groups in the specified flow.
*
* Create a request for the method "transitionRouteGroups.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The flow to list all transition route groups for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/transitionRouteGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Returns the list of all transition route groups in the specified flow.
*
* Create a request for the method "transitionRouteGroups.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The flow to list all transition route groups for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListTransitionRouteGroupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The flow to list all transition route groups for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The flow to list all transition route groups for. Format:
`projects//locations//agents//flows/` or `projects//locations//agents/.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The flow to list all transition route groups for. Format:
* `projects//locations//agents//flows/` or `projects//locations//agents/.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The language to list transition route groups for. The following fields are language
* dependent: * `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language to list transition route groups for. The following fields are language dependent: *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language to list transition route groups for. The following fields are language
* dependent: * `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public List setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified TransitionRouteGroup. Note: You should always train a flow prior to sending
* it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the transition route group.
* TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` .
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/transitionRouteGroups/[^/]+$");
/**
* Updates the specified TransitionRouteGroup. Note: You should always train a flow prior to
* sending it queries. See the [training
* documentation](https://cloud.google.com/dialogflow/cx/docs/concept/training).
*
* Create a request for the method "transitionRouteGroups.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the transition route group.
* TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically. Format:
* `projects//locations//agents//flows//transitionRouteGroups/` .
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3TransitionRouteGroup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/transitionRouteGroups/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the transition route group.
* TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically.
* Format: `projects//locations//agents//flows//transitionRouteGroups/` .
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the transition route group.
TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically. Format:
`projects//locations//agents//flows//transitionRouteGroups/` .
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the transition route group.
* TransitionRouteGroups.CreateTransitionRouteGroup populates the name automatically.
* Format: `projects//locations//agents//flows//transitionRouteGroups/` .
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/transitionRouteGroups/[^/]+$");
}
this.name = name;
return this;
}
/**
* The language of the following fields in `TransitionRouteGroup`: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/** The language of the following fields in `TransitionRouteGroup`: *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
`TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not specified,
the agent's default language is used. [Many
languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are supported. Note:
languages must be enabled in the agent before they can be used.
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language of the following fields in `TransitionRouteGroup`: *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.messages` *
* `TransitionRouteGroup.transition_routes.trigger_fulfillment.conditional_cases` If not
* specified, the agent's default language is used. [Many
* languages](https://cloud.google.com/dialogflow/cx/docs/reference/language) are
* supported. Note: languages must be enabled in the agent before they can be used.
*/
public Patch setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/** The mask to control which fields get updated. */
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated.
*/
public String getUpdateMask() {
return updateMask;
}
/** The mask to control which fields get updated. */
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Webhooks collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Webhooks.List request = dialogflow.webhooks().list(parameters ...)}
*
*
* @return the resource collection
*/
public Webhooks webhooks() {
return new Webhooks();
}
/**
* The "webhooks" collection of methods.
*/
public class Webhooks {
/**
* Creates a webhook in the specified agent.
*
* Create a request for the method "webhooks.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to create a webhook for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/webhooks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Creates a webhook in the specified agent.
*
* Create a request for the method "webhooks.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to create a webhook for. Format: `projects//locations//agents/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to create a webhook for. Format: `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to create a webhook for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to create a webhook for. Format: `projects//locations//agents/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified webhook.
*
* Create a request for the method "webhooks.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the webhook to delete. Format: `projects//locations//agents//webhooks/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/webhooks/[^/]+$");
/**
* Deletes the specified webhook.
*
* Create a request for the method "webhooks.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the webhook to delete. Format: `projects//locations//agents//webhooks/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/webhooks/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the webhook to delete. Format:
* `projects//locations//agents//webhooks/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the webhook to delete. Format: `projects//locations//agents//webhooks/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the webhook to delete. Format:
* `projects//locations//agents//webhooks/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/webhooks/[^/]+$");
}
this.name = name;
return this;
}
/**
* This field has no effect for webhook not being used. For webhooks that are used by
* pages/flows/transition route groups: * If `force` is set to false, an error will be
* returned with message indicating the referenced resources. * If `force` is set to
* true, Dialogflow will remove the webhook, as well as any references to the webhook
* (i.e. Webhook and tagin fulfillments that point to this webhook will be removed).
*/
@com.google.api.client.util.Key
private java.lang.Boolean force;
/** This field has no effect for webhook not being used. For webhooks that are used by
pages/flows/transition route groups: * If `force` is set to false, an error will be returned with
message indicating the referenced resources. * If `force` is set to true, Dialogflow will remove
the webhook, as well as any references to the webhook (i.e. Webhook and tagin fulfillments that
point to this webhook will be removed).
*/
public java.lang.Boolean getForce() {
return force;
}
/**
* This field has no effect for webhook not being used. For webhooks that are used by
* pages/flows/transition route groups: * If `force` is set to false, an error will be
* returned with message indicating the referenced resources. * If `force` is set to
* true, Dialogflow will remove the webhook, as well as any references to the webhook
* (i.e. Webhook and tagin fulfillments that point to this webhook will be removed).
*/
public Delete setForce(java.lang.Boolean force) {
this.force = force;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified webhook.
*
* Create a request for the method "webhooks.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the webhook. Format: `projects//locations//agents//webhooks/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/webhooks/[^/]+$");
/**
* Retrieves the specified webhook.
*
* Create a request for the method "webhooks.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the webhook. Format: `projects//locations//agents//webhooks/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/webhooks/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the webhook. Format: `projects//locations//agents//webhooks/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the webhook. Format: `projects//locations//agents//webhooks/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the webhook. Format: `projects//locations//agents//webhooks/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/webhooks/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all webhooks in the specified agent.
*
* Create a request for the method "webhooks.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The agent to list all webhooks for. Format: `projects//locations//agents/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/webhooks";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
/**
* Returns the list of all webhooks in the specified agent.
*
* Create a request for the method "webhooks.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The agent to list all webhooks for. Format: `projects//locations//agents/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListWebhooksResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The agent to list all webhooks for. Format: `projects//locations//agents/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The agent to list all webhooks for. Format: `projects//locations//agents/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The agent to list all webhooks for. Format: `projects//locations//agents/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 100 and at most 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 100 and at most
* 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified webhook.
*
* Create a request for the method "webhooks.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name The unique identifier of the webhook. Required for the Webhooks.UpdateWebhook method.
* Webhooks.CreateWebhook populates the name automatically. Format:
* `projects//locations//agents//webhooks/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/agents/[^/]+/webhooks/[^/]+$");
/**
* Updates the specified webhook.
*
* Create a request for the method "webhooks.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The unique identifier of the webhook. Required for the Webhooks.UpdateWebhook method.
* Webhooks.CreateWebhook populates the name automatically. Format:
* `projects//locations//agents//webhooks/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Webhook.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/webhooks/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The unique identifier of the webhook. Required for the Webhooks.UpdateWebhook method.
* Webhooks.CreateWebhook populates the name automatically. Format:
* `projects//locations//agents//webhooks/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** The unique identifier of the webhook. Required for the Webhooks.UpdateWebhook method.
Webhooks.CreateWebhook populates the name automatically. Format:
`projects//locations//agents//webhooks/`.
*/
public java.lang.String getName() {
return name;
}
/**
* The unique identifier of the webhook. Required for the Webhooks.UpdateWebhook method.
* Webhooks.CreateWebhook populates the name automatically. Format:
* `projects//locations//agents//webhooks/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/agents/[^/]+/webhooks/[^/]+$");
}
this.name = name;
return this;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The mask to control which fields get updated. If the mask is not present, all fields will be
updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The mask to control which fields get updated. If the mask is not present, all fields
* will be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Operations.List request = dialogflow.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(Dialogflow.this, "POST", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleLongrunningListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the SecuritySettings collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.SecuritySettings.List request = dialogflow.securitySettings().list(parameters ...)}
*
*
* @return the resource collection
*/
public SecuritySettings securitySettings() {
return new SecuritySettings();
}
/**
* The "securitySettings" collection of methods.
*/
public class SecuritySettings {
/**
* Create security settings in the specified location.
*
* Create a request for the method "securitySettings.create".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The location to create an SecuritySettings for. Format: `projects//locations/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/securitySettings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Create security settings in the specified location.
*
* Create a request for the method "securitySettings.create".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The location to create an SecuritySettings for. Format: `projects//locations/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings content) {
super(Dialogflow.this, "POST", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The location to create an SecuritySettings for. Format:
* `projects//locations/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The location to create an SecuritySettings for. Format: `projects//locations/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The location to create an SecuritySettings for. Format:
* `projects//locations/`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes the specified SecuritySettings.
*
* Create a request for the method "securitySettings.delete".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the SecuritySettings to delete. Format:
* `projects//locations//securitySettings/`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/securitySettings/[^/]+$");
/**
* Deletes the specified SecuritySettings.
*
* Create a request for the method "securitySettings.delete".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the SecuritySettings to delete. Format:
* `projects//locations//securitySettings/`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Dialogflow.this, "DELETE", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/securitySettings/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the SecuritySettings to delete. Format:
* `projects//locations//securitySettings/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the SecuritySettings to delete. Format:
`projects//locations//securitySettings/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the SecuritySettings to delete. Format:
* `projects//locations//securitySettings/`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/securitySettings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves the specified SecuritySettings. The returned settings may be stale by up to 1 minute.
*
* Create a request for the method "securitySettings.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Resource name of the settings. Format: `projects//locations//securitySettings/`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/securitySettings/[^/]+$");
/**
* Retrieves the specified SecuritySettings. The returned settings may be stale by up to 1 minute.
*
* Create a request for the method "securitySettings.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Resource name of the settings. Format: `projects//locations//securitySettings/`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/securitySettings/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Resource name of the settings. Format:
* `projects//locations//securitySettings/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Resource name of the settings. Format: `projects//locations//securitySettings/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Resource name of the settings. Format:
* `projects//locations//securitySettings/`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/securitySettings/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Returns the list of all security settings in the specified location.
*
* Create a request for the method "securitySettings.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The location to list all security settings for. Format: `projects//locations/`.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+parent}/securitySettings";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+$");
/**
* Returns the list of all security settings in the specified location.
*
* Create a request for the method "securitySettings.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The location to list all security settings for. Format: `projects//locations/`.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3ListSecuritySettingsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The location to list all security settings for. Format:
* `projects//locations/`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The location to list all security settings for. Format: `projects//locations/`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The location to list all security settings for. Format:
* `projects//locations/`.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most 100.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of items to return in a single page. By default 20 and at most 100.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of items to return in a single page. By default 20 and at most 100.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The next_page_token value returned from a previous list request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The next_page_token value returned from a previous list request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The next_page_token value returned from a previous list request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified SecuritySettings.
*
* Create a request for the method "securitySettings.patch".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Resource name of the settings. Required for the SecuritySettingsService.UpdateSecuritySettings
* method. SecuritySettingsService.CreateSecuritySettings populates the name automatically.
* Format: `projects//locations//securitySettings/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/locations/[^/]+/securitySettings/[^/]+$");
/**
* Updates the specified SecuritySettings.
*
* Create a request for the method "securitySettings.patch".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name of the settings. Required for the SecuritySettingsService.UpdateSecuritySettings
* method. SecuritySettingsService.CreateSecuritySettings populates the name automatically.
* Format: `projects//locations//securitySettings/`.
* @param content the {@link com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings content) {
super(Dialogflow.this, "PATCH", REST_PATH, content, com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3SecuritySettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/securitySettings/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name of the settings. Required for the
* SecuritySettingsService.UpdateSecuritySettings method.
* SecuritySettingsService.CreateSecuritySettings populates the name automatically.
* Format: `projects//locations//securitySettings/`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name of the settings. Required for the SecuritySettingsService.UpdateSecuritySettings
method. SecuritySettingsService.CreateSecuritySettings populates the name automatically. Format:
`projects//locations//securitySettings/`.
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name of the settings. Required for the
* SecuritySettingsService.UpdateSecuritySettings method.
* SecuritySettingsService.CreateSecuritySettings populates the name automatically.
* Format: `projects//locations//securitySettings/`.
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/locations/[^/]+/securitySettings/[^/]+$");
}
this.name = name;
return this;
}
/**
* Required. The mask to control which fields get updated. If the mask is not present, all
* fields will be updated.
*/
@com.google.api.client.util.Key
private String updateMask;
/** Required. The mask to control which fields get updated. If the mask is not present, all fields will
be updated.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* Required. The mask to control which fields get updated. If the mask is not present, all
* fields will be updated.
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Dialogflow dialogflow = new Dialogflow(...);}
* {@code Dialogflow.Operations.List request = dialogflow.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @return the request
*/
public Cancel cancel(java.lang.String name) throws java.io.IOException {
Cancel result = new Cancel(name);
initialize(result);
return result;
}
public class Cancel extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/operations/[^/]+$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. If the server doesn't support this method,
* it returns `google.rpc.Code.UNIMPLEMENTED`. Clients can use Operations.GetOperation or other
* methods to check whether the cancellation succeeded or whether the operation completed despite
* cancellation. On successful cancellation, the operation is not deleted; instead, it becomes an
* operation with an Operation.error value with a google.rpc.Status.code of 1, corresponding to
* `Code.CANCELLED`.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @since 1.13
*/
protected Cancel(java.lang.String name) {
super(Dialogflow.this, "POST", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleProtobufEmpty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/operations/[^/]+$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the dialogflow server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation's parent resource.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends DialogflowRequest {
private static final String REST_PATH = "v3/{+name}/operations";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists operations that match the specified filter in the request. If the server doesn't support
* this method, it returns `UNIMPLEMENTED`.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the dialogflow server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation's parent resource.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Dialogflow.this, "GET", REST_PATH, null, com.google.api.services.dialogflow.v3.model.GoogleLongrunningListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation's parent resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation's parent resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation's parent resource. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+$");
}
this.name = name;
return this;
}
/** The standard list filter. */
@com.google.api.client.util.Key
private java.lang.String filter;
/** The standard list filter.
*/
public java.lang.String getFilter() {
return filter;
}
/** The standard list filter. */
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** The standard list page size. */
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The standard list page size.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/** The standard list page size. */
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link Dialogflow}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link Dialogflow}. */
@Override
public Dialogflow build() {
return new Dialogflow(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link DialogflowRequestInitializer}.
*
* @since 1.12
*/
public Builder setDialogflowRequestInitializer(
DialogflowRequestInitializer dialogflowRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(dialogflowRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}