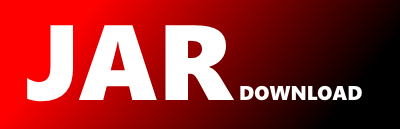
com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3DataStoreConnectionSignals Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dialogflow.v3.model;
/**
* Data store connection feature output signals. Might be only partially field if processing stop
* before the final answer. Reasons for this can be, but are not limited to: empty UCS search
* results, positive RAI check outcome, grounding failure, ...
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dialogflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudDialogflowCxV3DataStoreConnectionSignals extends com.google.api.client.json.GenericJson {
/**
* Optional. The final compiled answer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String answer;
/**
* Optional. Diagnostic info related to the answer generation model call.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3DataStoreConnectionSignalsAnswerGenerationModelCallSignals answerGenerationModelCallSignals;
/**
* Optional. Answer parts with relevant citations. Concatenation of texts should add up the
* `answer` (not counting whitespaces).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List answerParts;
/**
* Optional. Snippets cited by the answer generation model from the most to least relevant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List citedSnippets;
/**
* Optional. Grounding signals.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3DataStoreConnectionSignalsGroundingSignals groundingSignals;
/**
* Optional. Diagnostic info related to the rewriter model call.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3DataStoreConnectionSignalsRewriterModelCallSignals rewriterModelCallSignals;
/**
* Optional. Rewritten string query used for search.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rewrittenQuery;
/**
* Optional. Safety check result.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3DataStoreConnectionSignalsSafetySignals safetySignals;
/**
* Optional. Search snippets included in the answer generation prompt.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List searchSnippets;
/**
* Optional. The final compiled answer.
* @return value or {@code null} for none
*/
public java.lang.String getAnswer() {
return answer;
}
/**
* Optional. The final compiled answer.
* @param answer answer or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals setAnswer(java.lang.String answer) {
this.answer = answer;
return this;
}
/**
* Optional. Diagnostic info related to the answer generation model call.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignalsAnswerGenerationModelCallSignals getAnswerGenerationModelCallSignals() {
return answerGenerationModelCallSignals;
}
/**
* Optional. Diagnostic info related to the answer generation model call.
* @param answerGenerationModelCallSignals answerGenerationModelCallSignals or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals setAnswerGenerationModelCallSignals(GoogleCloudDialogflowCxV3DataStoreConnectionSignalsAnswerGenerationModelCallSignals answerGenerationModelCallSignals) {
this.answerGenerationModelCallSignals = answerGenerationModelCallSignals;
return this;
}
/**
* Optional. Answer parts with relevant citations. Concatenation of texts should add up the
* `answer` (not counting whitespaces).
* @return value or {@code null} for none
*/
public java.util.List getAnswerParts() {
return answerParts;
}
/**
* Optional. Answer parts with relevant citations. Concatenation of texts should add up the
* `answer` (not counting whitespaces).
* @param answerParts answerParts or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals setAnswerParts(java.util.List answerParts) {
this.answerParts = answerParts;
return this;
}
/**
* Optional. Snippets cited by the answer generation model from the most to least relevant.
* @return value or {@code null} for none
*/
public java.util.List getCitedSnippets() {
return citedSnippets;
}
/**
* Optional. Snippets cited by the answer generation model from the most to least relevant.
* @param citedSnippets citedSnippets or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals setCitedSnippets(java.util.List citedSnippets) {
this.citedSnippets = citedSnippets;
return this;
}
/**
* Optional. Grounding signals.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignalsGroundingSignals getGroundingSignals() {
return groundingSignals;
}
/**
* Optional. Grounding signals.
* @param groundingSignals groundingSignals or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals setGroundingSignals(GoogleCloudDialogflowCxV3DataStoreConnectionSignalsGroundingSignals groundingSignals) {
this.groundingSignals = groundingSignals;
return this;
}
/**
* Optional. Diagnostic info related to the rewriter model call.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignalsRewriterModelCallSignals getRewriterModelCallSignals() {
return rewriterModelCallSignals;
}
/**
* Optional. Diagnostic info related to the rewriter model call.
* @param rewriterModelCallSignals rewriterModelCallSignals or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals setRewriterModelCallSignals(GoogleCloudDialogflowCxV3DataStoreConnectionSignalsRewriterModelCallSignals rewriterModelCallSignals) {
this.rewriterModelCallSignals = rewriterModelCallSignals;
return this;
}
/**
* Optional. Rewritten string query used for search.
* @return value or {@code null} for none
*/
public java.lang.String getRewrittenQuery() {
return rewrittenQuery;
}
/**
* Optional. Rewritten string query used for search.
* @param rewrittenQuery rewrittenQuery or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals setRewrittenQuery(java.lang.String rewrittenQuery) {
this.rewrittenQuery = rewrittenQuery;
return this;
}
/**
* Optional. Safety check result.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignalsSafetySignals getSafetySignals() {
return safetySignals;
}
/**
* Optional. Safety check result.
* @param safetySignals safetySignals or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals setSafetySignals(GoogleCloudDialogflowCxV3DataStoreConnectionSignalsSafetySignals safetySignals) {
this.safetySignals = safetySignals;
return this;
}
/**
* Optional. Search snippets included in the answer generation prompt.
* @return value or {@code null} for none
*/
public java.util.List getSearchSnippets() {
return searchSnippets;
}
/**
* Optional. Search snippets included in the answer generation prompt.
* @param searchSnippets searchSnippets or {@code null} for none
*/
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals setSearchSnippets(java.util.List searchSnippets) {
this.searchSnippets = searchSnippets;
return this;
}
@Override
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals set(String fieldName, Object value) {
return (GoogleCloudDialogflowCxV3DataStoreConnectionSignals) super.set(fieldName, value);
}
@Override
public GoogleCloudDialogflowCxV3DataStoreConnectionSignals clone() {
return (GoogleCloudDialogflowCxV3DataStoreConnectionSignals) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy