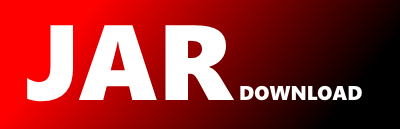
com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Experiment Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dialogflow.v3.model;
/**
* Represents an experiment in an environment.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dialogflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudDialogflowCxV3Experiment extends com.google.api.client.json.GenericJson {
/**
* Creation time of this experiment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String createTime;
/**
* The definition of the experiment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3ExperimentDefinition definition;
/**
* The human-readable description of the experiment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Required. The human-readable name of the experiment (unique in an environment). Limit of 64
* characters.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayName;
/**
* End time of this experiment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String endTime;
/**
* Maximum number of days to run the experiment/rollout. If auto-rollout is not enabled, default
* value and maximum will be 30 days. If auto-rollout is enabled, default value and maximum will
* be 6 days.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String experimentLength;
/**
* Last update time of this experiment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String lastUpdateTime;
/**
* The name of the experiment. Format: projects//locations//agents//environments//experiments/..
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Inference result of the experiment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3ExperimentResult result;
/**
* The configuration for auto rollout. If set, there should be exactly two variants in the
* experiment (control variant being the default version of the flow), the traffic allocation for
* the non-control variant will gradually increase to 100% when conditions are met, and eventually
* replace the control variant to become the default version of the flow.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3RolloutConfig rolloutConfig;
/**
* The reason why rollout has failed. Should only be set when state is ROLLOUT_FAILED.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rolloutFailureReason;
/**
* State of the auto rollout process.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3RolloutState rolloutState;
/**
* Start time of this experiment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String startTime;
/**
* The current state of the experiment. Transition triggered by Experiments.StartExperiment:
* DRAFT->RUNNING. Transition triggered by Experiments.CancelExperiment: DRAFT->DONE or
* RUNNING->DONE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/**
* The history of updates to the experiment variants.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List variantsHistory;
/**
* Creation time of this experiment.
* @return value or {@code null} for none
*/
public String getCreateTime() {
return createTime;
}
/**
* Creation time of this experiment.
* @param createTime createTime or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setCreateTime(String createTime) {
this.createTime = createTime;
return this;
}
/**
* The definition of the experiment.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3ExperimentDefinition getDefinition() {
return definition;
}
/**
* The definition of the experiment.
* @param definition definition or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setDefinition(GoogleCloudDialogflowCxV3ExperimentDefinition definition) {
this.definition = definition;
return this;
}
/**
* The human-readable description of the experiment.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* The human-readable description of the experiment.
* @param description description or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Required. The human-readable name of the experiment (unique in an environment). Limit of 64
* characters.
* @return value or {@code null} for none
*/
public java.lang.String getDisplayName() {
return displayName;
}
/**
* Required. The human-readable name of the experiment (unique in an environment). Limit of 64
* characters.
* @param displayName displayName or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setDisplayName(java.lang.String displayName) {
this.displayName = displayName;
return this;
}
/**
* End time of this experiment.
* @return value or {@code null} for none
*/
public String getEndTime() {
return endTime;
}
/**
* End time of this experiment.
* @param endTime endTime or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
/**
* Maximum number of days to run the experiment/rollout. If auto-rollout is not enabled, default
* value and maximum will be 30 days. If auto-rollout is enabled, default value and maximum will
* be 6 days.
* @return value or {@code null} for none
*/
public String getExperimentLength() {
return experimentLength;
}
/**
* Maximum number of days to run the experiment/rollout. If auto-rollout is not enabled, default
* value and maximum will be 30 days. If auto-rollout is enabled, default value and maximum will
* be 6 days.
* @param experimentLength experimentLength or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setExperimentLength(String experimentLength) {
this.experimentLength = experimentLength;
return this;
}
/**
* Last update time of this experiment.
* @return value or {@code null} for none
*/
public String getLastUpdateTime() {
return lastUpdateTime;
}
/**
* Last update time of this experiment.
* @param lastUpdateTime lastUpdateTime or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setLastUpdateTime(String lastUpdateTime) {
this.lastUpdateTime = lastUpdateTime;
return this;
}
/**
* The name of the experiment. Format: projects//locations//agents//environments//experiments/..
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The name of the experiment. Format: projects//locations//agents//environments//experiments/..
* @param name name or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Inference result of the experiment.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3ExperimentResult getResult() {
return result;
}
/**
* Inference result of the experiment.
* @param result result or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setResult(GoogleCloudDialogflowCxV3ExperimentResult result) {
this.result = result;
return this;
}
/**
* The configuration for auto rollout. If set, there should be exactly two variants in the
* experiment (control variant being the default version of the flow), the traffic allocation for
* the non-control variant will gradually increase to 100% when conditions are met, and eventually
* replace the control variant to become the default version of the flow.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3RolloutConfig getRolloutConfig() {
return rolloutConfig;
}
/**
* The configuration for auto rollout. If set, there should be exactly two variants in the
* experiment (control variant being the default version of the flow), the traffic allocation for
* the non-control variant will gradually increase to 100% when conditions are met, and eventually
* replace the control variant to become the default version of the flow.
* @param rolloutConfig rolloutConfig or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setRolloutConfig(GoogleCloudDialogflowCxV3RolloutConfig rolloutConfig) {
this.rolloutConfig = rolloutConfig;
return this;
}
/**
* The reason why rollout has failed. Should only be set when state is ROLLOUT_FAILED.
* @return value or {@code null} for none
*/
public java.lang.String getRolloutFailureReason() {
return rolloutFailureReason;
}
/**
* The reason why rollout has failed. Should only be set when state is ROLLOUT_FAILED.
* @param rolloutFailureReason rolloutFailureReason or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setRolloutFailureReason(java.lang.String rolloutFailureReason) {
this.rolloutFailureReason = rolloutFailureReason;
return this;
}
/**
* State of the auto rollout process.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3RolloutState getRolloutState() {
return rolloutState;
}
/**
* State of the auto rollout process.
* @param rolloutState rolloutState or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setRolloutState(GoogleCloudDialogflowCxV3RolloutState rolloutState) {
this.rolloutState = rolloutState;
return this;
}
/**
* Start time of this experiment.
* @return value or {@code null} for none
*/
public String getStartTime() {
return startTime;
}
/**
* Start time of this experiment.
* @param startTime startTime or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
/**
* The current state of the experiment. Transition triggered by Experiments.StartExperiment:
* DRAFT->RUNNING. Transition triggered by Experiments.CancelExperiment: DRAFT->DONE or
* RUNNING->DONE.
* @return value or {@code null} for none
*/
public java.lang.String getState() {
return state;
}
/**
* The current state of the experiment. Transition triggered by Experiments.StartExperiment:
* DRAFT->RUNNING. Transition triggered by Experiments.CancelExperiment: DRAFT->DONE or
* RUNNING->DONE.
* @param state state or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* The history of updates to the experiment variants.
* @return value or {@code null} for none
*/
public java.util.List getVariantsHistory() {
return variantsHistory;
}
/**
* The history of updates to the experiment variants.
* @param variantsHistory variantsHistory or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Experiment setVariantsHistory(java.util.List variantsHistory) {
this.variantsHistory = variantsHistory;
return this;
}
@Override
public GoogleCloudDialogflowCxV3Experiment set(String fieldName, Object value) {
return (GoogleCloudDialogflowCxV3Experiment) super.set(fieldName, value);
}
@Override
public GoogleCloudDialogflowCxV3Experiment clone() {
return (GoogleCloudDialogflowCxV3Experiment) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy