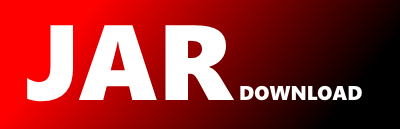
com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3Match Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dialogflow.v3.model;
/**
* Represents one match result of MatchIntent.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dialogflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudDialogflowCxV3Match extends com.google.api.client.json.GenericJson {
/**
* The confidence of this match. Values range from 0.0 (completely uncertain) to 1.0 (completely
* certain). This value is for informational purpose only and is only used to help match the best
* intent within the classification threshold. This value may change for the same end-user
* expression at any time due to a model retraining or change in implementation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float confidence;
/**
* The event that matched the query. Filled for `EVENT`, `NO_MATCH` and `NO_INPUT` match types.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String event;
/**
* The Intent that matched the query. Some, not all fields are filled in this message, including
* but not limited to: `name` and `display_name`. Only filled for `INTENT` match type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3Intent intent;
/**
* Type of this Match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String matchType;
/**
* The collection of parameters extracted from the query. Depending on your protocol or client
* library language, this is a map, associative array, symbol table, dictionary, or JSON object
* composed of a collection of (MapKey, MapValue) pairs: * MapKey type: string * MapKey value:
* parameter name * MapValue type: If parameter's entity type is a composite entity then use map,
* otherwise, depending on the parameter value type, it could be one of string, number, boolean,
* null, list or map. * MapValue value: If parameter's entity type is a composite entity then use
* map from composite entity property names to property values, otherwise, use parameter value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map parameters;
/**
* Final text input which was matched during MatchIntent. This value can be different from
* original input sent in request because of spelling correction or other processing.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String resolvedInput;
/**
* The confidence of this match. Values range from 0.0 (completely uncertain) to 1.0 (completely
* certain). This value is for informational purpose only and is only used to help match the best
* intent within the classification threshold. This value may change for the same end-user
* expression at any time due to a model retraining or change in implementation.
* @return value or {@code null} for none
*/
public java.lang.Float getConfidence() {
return confidence;
}
/**
* The confidence of this match. Values range from 0.0 (completely uncertain) to 1.0 (completely
* certain). This value is for informational purpose only and is only used to help match the best
* intent within the classification threshold. This value may change for the same end-user
* expression at any time due to a model retraining or change in implementation.
* @param confidence confidence or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Match setConfidence(java.lang.Float confidence) {
this.confidence = confidence;
return this;
}
/**
* The event that matched the query. Filled for `EVENT`, `NO_MATCH` and `NO_INPUT` match types.
* @return value or {@code null} for none
*/
public java.lang.String getEvent() {
return event;
}
/**
* The event that matched the query. Filled for `EVENT`, `NO_MATCH` and `NO_INPUT` match types.
* @param event event or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Match setEvent(java.lang.String event) {
this.event = event;
return this;
}
/**
* The Intent that matched the query. Some, not all fields are filled in this message, including
* but not limited to: `name` and `display_name`. Only filled for `INTENT` match type.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Intent getIntent() {
return intent;
}
/**
* The Intent that matched the query. Some, not all fields are filled in this message, including
* but not limited to: `name` and `display_name`. Only filled for `INTENT` match type.
* @param intent intent or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Match setIntent(GoogleCloudDialogflowCxV3Intent intent) {
this.intent = intent;
return this;
}
/**
* Type of this Match.
* @return value or {@code null} for none
*/
public java.lang.String getMatchType() {
return matchType;
}
/**
* Type of this Match.
* @param matchType matchType or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Match setMatchType(java.lang.String matchType) {
this.matchType = matchType;
return this;
}
/**
* The collection of parameters extracted from the query. Depending on your protocol or client
* library language, this is a map, associative array, symbol table, dictionary, or JSON object
* composed of a collection of (MapKey, MapValue) pairs: * MapKey type: string * MapKey value:
* parameter name * MapValue type: If parameter's entity type is a composite entity then use map,
* otherwise, depending on the parameter value type, it could be one of string, number, boolean,
* null, list or map. * MapValue value: If parameter's entity type is a composite entity then use
* map from composite entity property names to property values, otherwise, use parameter value.
* @return value or {@code null} for none
*/
public java.util.Map getParameters() {
return parameters;
}
/**
* The collection of parameters extracted from the query. Depending on your protocol or client
* library language, this is a map, associative array, symbol table, dictionary, or JSON object
* composed of a collection of (MapKey, MapValue) pairs: * MapKey type: string * MapKey value:
* parameter name * MapValue type: If parameter's entity type is a composite entity then use map,
* otherwise, depending on the parameter value type, it could be one of string, number, boolean,
* null, list or map. * MapValue value: If parameter's entity type is a composite entity then use
* map from composite entity property names to property values, otherwise, use parameter value.
* @param parameters parameters or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Match setParameters(java.util.Map parameters) {
this.parameters = parameters;
return this;
}
/**
* Final text input which was matched during MatchIntent. This value can be different from
* original input sent in request because of spelling correction or other processing.
* @return value or {@code null} for none
*/
public java.lang.String getResolvedInput() {
return resolvedInput;
}
/**
* Final text input which was matched during MatchIntent. This value can be different from
* original input sent in request because of spelling correction or other processing.
* @param resolvedInput resolvedInput or {@code null} for none
*/
public GoogleCloudDialogflowCxV3Match setResolvedInput(java.lang.String resolvedInput) {
this.resolvedInput = resolvedInput;
return this;
}
@Override
public GoogleCloudDialogflowCxV3Match set(String fieldName, Object value) {
return (GoogleCloudDialogflowCxV3Match) super.set(fieldName, value);
}
@Override
public GoogleCloudDialogflowCxV3Match clone() {
return (GoogleCloudDialogflowCxV3Match) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy