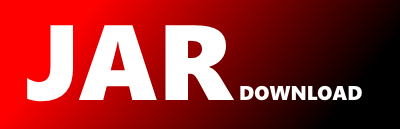
com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3WebhookGenericWebService Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dialogflow.v3.model;
/**
* Represents configuration for a generic web service.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dialogflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudDialogflowCxV3WebhookGenericWebService extends com.google.api.client.json.GenericJson {
/**
* Optional. Specifies a list of allowed custom CA certificates (in DER format) for HTTPS
* verification. This overrides the default SSL trust store. If this is empty or unspecified,
* Dialogflow will use Google's default trust store to verify certificates. N.B. Make sure the
* HTTPS server certificates are signed with "subject alt name". For instance a certificate can be
* self-signed using the following command, ``` openssl x509 -req -days 200 -in example.com.csr \
* -signkey example.com.key \ -out example.com.crt \ -extfile <(printf
* "\nsubjectAltName='DNS:www.example.com'") ```
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List allowedCaCerts;
/**
* Optional. HTTP method for the flexible webhook calls. Standard webhook always uses POST.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String httpMethod;
/**
* Optional. The OAuth configuration of the webhook. If specified, Dialogflow will initiate the
* OAuth client credential flow to exchange an access token from the 3rd party platform and put it
* in the auth header.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3WebhookGenericWebServiceOAuthConfig oauthConfig;
/**
* Optional. Maps the values extracted from specific fields of the flexible webhook response into
* session parameters. - Key: session parameter name - Value: field path in the webhook response
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map parameterMapping;
/**
* The password for HTTP Basic authentication.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String password;
/**
* Optional. Defines a custom JSON object as request body to send to flexible webhook.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String requestBody;
/**
* The HTTP request headers to send together with webhook requests.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map requestHeaders;
/**
* Optional. Indicate the auth token type generated from the [Diglogflow service
* agent](https://cloud.google.com/iam/docs/service-agents#dialogflow-service-agent). The
* generated token is sent in the Authorization header.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String serviceAgentAuth;
/**
* Required. The webhook URI for receiving POST requests. It must use https protocol.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String uri;
/**
* The user name for HTTP Basic authentication.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String username;
/**
* Optional. Type of the webhook.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String webhookType;
/**
* Optional. Specifies a list of allowed custom CA certificates (in DER format) for HTTPS
* verification. This overrides the default SSL trust store. If this is empty or unspecified,
* Dialogflow will use Google's default trust store to verify certificates. N.B. Make sure the
* HTTPS server certificates are signed with "subject alt name". For instance a certificate can be
* self-signed using the following command, ``` openssl x509 -req -days 200 -in example.com.csr \
* -signkey example.com.key \ -out example.com.crt \ -extfile <(printf
* "\nsubjectAltName='DNS:www.example.com'") ```
* @return value or {@code null} for none
*/
public java.util.List getAllowedCaCerts() {
return allowedCaCerts;
}
/**
* Optional. Specifies a list of allowed custom CA certificates (in DER format) for HTTPS
* verification. This overrides the default SSL trust store. If this is empty or unspecified,
* Dialogflow will use Google's default trust store to verify certificates. N.B. Make sure the
* HTTPS server certificates are signed with "subject alt name". For instance a certificate can be
* self-signed using the following command, ``` openssl x509 -req -days 200 -in example.com.csr \
* -signkey example.com.key \ -out example.com.crt \ -extfile <(printf
* "\nsubjectAltName='DNS:www.example.com'") ```
* @param allowedCaCerts allowedCaCerts or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setAllowedCaCerts(java.util.List allowedCaCerts) {
this.allowedCaCerts = allowedCaCerts;
return this;
}
/**
* Optional. HTTP method for the flexible webhook calls. Standard webhook always uses POST.
* @return value or {@code null} for none
*/
public java.lang.String getHttpMethod() {
return httpMethod;
}
/**
* Optional. HTTP method for the flexible webhook calls. Standard webhook always uses POST.
* @param httpMethod httpMethod or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setHttpMethod(java.lang.String httpMethod) {
this.httpMethod = httpMethod;
return this;
}
/**
* Optional. The OAuth configuration of the webhook. If specified, Dialogflow will initiate the
* OAuth client credential flow to exchange an access token from the 3rd party platform and put it
* in the auth header.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebServiceOAuthConfig getOauthConfig() {
return oauthConfig;
}
/**
* Optional. The OAuth configuration of the webhook. If specified, Dialogflow will initiate the
* OAuth client credential flow to exchange an access token from the 3rd party platform and put it
* in the auth header.
* @param oauthConfig oauthConfig or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setOauthConfig(GoogleCloudDialogflowCxV3WebhookGenericWebServiceOAuthConfig oauthConfig) {
this.oauthConfig = oauthConfig;
return this;
}
/**
* Optional. Maps the values extracted from specific fields of the flexible webhook response into
* session parameters. - Key: session parameter name - Value: field path in the webhook response
* @return value or {@code null} for none
*/
public java.util.Map getParameterMapping() {
return parameterMapping;
}
/**
* Optional. Maps the values extracted from specific fields of the flexible webhook response into
* session parameters. - Key: session parameter name - Value: field path in the webhook response
* @param parameterMapping parameterMapping or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setParameterMapping(java.util.Map parameterMapping) {
this.parameterMapping = parameterMapping;
return this;
}
/**
* The password for HTTP Basic authentication.
* @return value or {@code null} for none
*/
public java.lang.String getPassword() {
return password;
}
/**
* The password for HTTP Basic authentication.
* @param password password or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setPassword(java.lang.String password) {
this.password = password;
return this;
}
/**
* Optional. Defines a custom JSON object as request body to send to flexible webhook.
* @return value or {@code null} for none
*/
public java.lang.String getRequestBody() {
return requestBody;
}
/**
* Optional. Defines a custom JSON object as request body to send to flexible webhook.
* @param requestBody requestBody or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setRequestBody(java.lang.String requestBody) {
this.requestBody = requestBody;
return this;
}
/**
* The HTTP request headers to send together with webhook requests.
* @return value or {@code null} for none
*/
public java.util.Map getRequestHeaders() {
return requestHeaders;
}
/**
* The HTTP request headers to send together with webhook requests.
* @param requestHeaders requestHeaders or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setRequestHeaders(java.util.Map requestHeaders) {
this.requestHeaders = requestHeaders;
return this;
}
/**
* Optional. Indicate the auth token type generated from the [Diglogflow service
* agent](https://cloud.google.com/iam/docs/service-agents#dialogflow-service-agent). The
* generated token is sent in the Authorization header.
* @return value or {@code null} for none
*/
public java.lang.String getServiceAgentAuth() {
return serviceAgentAuth;
}
/**
* Optional. Indicate the auth token type generated from the [Diglogflow service
* agent](https://cloud.google.com/iam/docs/service-agents#dialogflow-service-agent). The
* generated token is sent in the Authorization header.
* @param serviceAgentAuth serviceAgentAuth or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setServiceAgentAuth(java.lang.String serviceAgentAuth) {
this.serviceAgentAuth = serviceAgentAuth;
return this;
}
/**
* Required. The webhook URI for receiving POST requests. It must use https protocol.
* @return value or {@code null} for none
*/
public java.lang.String getUri() {
return uri;
}
/**
* Required. The webhook URI for receiving POST requests. It must use https protocol.
* @param uri uri or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setUri(java.lang.String uri) {
this.uri = uri;
return this;
}
/**
* The user name for HTTP Basic authentication.
* @return value or {@code null} for none
*/
public java.lang.String getUsername() {
return username;
}
/**
* The user name for HTTP Basic authentication.
* @param username username or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setUsername(java.lang.String username) {
this.username = username;
return this;
}
/**
* Optional. Type of the webhook.
* @return value or {@code null} for none
*/
public java.lang.String getWebhookType() {
return webhookType;
}
/**
* Optional. Type of the webhook.
* @param webhookType webhookType or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookGenericWebService setWebhookType(java.lang.String webhookType) {
this.webhookType = webhookType;
return this;
}
@Override
public GoogleCloudDialogflowCxV3WebhookGenericWebService set(String fieldName, Object value) {
return (GoogleCloudDialogflowCxV3WebhookGenericWebService) super.set(fieldName, value);
}
@Override
public GoogleCloudDialogflowCxV3WebhookGenericWebService clone() {
return (GoogleCloudDialogflowCxV3WebhookGenericWebService) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy