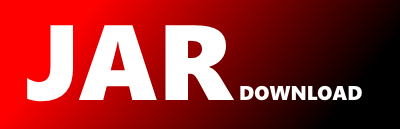
com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowCxV3WebhookRequest Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dialogflow.v3.model;
/**
* The request message for a webhook call. The request is sent as a JSON object and the field names
* will be presented in camel cases. You may see undocumented fields in an actual request. These
* fields are used internally by Dialogflow and should be ignored.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dialogflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudDialogflowCxV3WebhookRequest extends com.google.api.client.json.GenericJson {
/**
* Always present. The unique identifier of the DetectIntentResponse that will be returned to the
* API caller.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String detectIntentResponseId;
/**
* If DTMF was provided as input, this field will contain the DTMF digits.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String dtmfDigits;
/**
* Always present. Information about the fulfillment that triggered this webhook call.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3WebhookRequestFulfillmentInfo fulfillmentInfo;
/**
* Information about the last matched intent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3WebhookRequestIntentInfo intentInfo;
/**
* The language code specified in the original request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String languageCode;
/**
* Information about the language of the request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3LanguageInfo languageInfo;
/**
* The list of rich message responses to present to the user. Webhook can choose to append or
* replace this list in WebhookResponse.fulfillment_response;
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List messages;
static {
// hack to force ProGuard to consider GoogleCloudDialogflowCxV3ResponseMessage used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudDialogflowCxV3ResponseMessage.class);
}
/**
* Information about page status.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3PageInfo pageInfo;
/**
* Custom data set in QueryParameters.payload.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map payload;
/**
* The sentiment analysis result of the current user request. The field is filled when sentiment
* analysis is configured to be enabled for the request.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3WebhookRequestSentimentAnalysisResult sentimentAnalysisResult;
/**
* Information about session status.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudDialogflowCxV3SessionInfo sessionInfo;
/**
* If natural language text was provided as input, this field will contain a copy of the text.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String text;
/**
* If natural language speech audio was provided as input, this field will contain the transcript
* for the audio.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String transcript;
/**
* If an event was provided as input, this field will contain the name of the event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String triggerEvent;
/**
* If an intent was provided as input, this field will contain a copy of the intent identifier.
* Format: `projects//locations//agents//intents/`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String triggerIntent;
/**
* Always present. The unique identifier of the DetectIntentResponse that will be returned to the
* API caller.
* @return value or {@code null} for none
*/
public java.lang.String getDetectIntentResponseId() {
return detectIntentResponseId;
}
/**
* Always present. The unique identifier of the DetectIntentResponse that will be returned to the
* API caller.
* @param detectIntentResponseId detectIntentResponseId or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setDetectIntentResponseId(java.lang.String detectIntentResponseId) {
this.detectIntentResponseId = detectIntentResponseId;
return this;
}
/**
* If DTMF was provided as input, this field will contain the DTMF digits.
* @return value or {@code null} for none
*/
public java.lang.String getDtmfDigits() {
return dtmfDigits;
}
/**
* If DTMF was provided as input, this field will contain the DTMF digits.
* @param dtmfDigits dtmfDigits or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setDtmfDigits(java.lang.String dtmfDigits) {
this.dtmfDigits = dtmfDigits;
return this;
}
/**
* Always present. Information about the fulfillment that triggered this webhook call.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequestFulfillmentInfo getFulfillmentInfo() {
return fulfillmentInfo;
}
/**
* Always present. Information about the fulfillment that triggered this webhook call.
* @param fulfillmentInfo fulfillmentInfo or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setFulfillmentInfo(GoogleCloudDialogflowCxV3WebhookRequestFulfillmentInfo fulfillmentInfo) {
this.fulfillmentInfo = fulfillmentInfo;
return this;
}
/**
* Information about the last matched intent.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequestIntentInfo getIntentInfo() {
return intentInfo;
}
/**
* Information about the last matched intent.
* @param intentInfo intentInfo or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setIntentInfo(GoogleCloudDialogflowCxV3WebhookRequestIntentInfo intentInfo) {
this.intentInfo = intentInfo;
return this;
}
/**
* The language code specified in the original request.
* @return value or {@code null} for none
*/
public java.lang.String getLanguageCode() {
return languageCode;
}
/**
* The language code specified in the original request.
* @param languageCode languageCode or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setLanguageCode(java.lang.String languageCode) {
this.languageCode = languageCode;
return this;
}
/**
* Information about the language of the request.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3LanguageInfo getLanguageInfo() {
return languageInfo;
}
/**
* Information about the language of the request.
* @param languageInfo languageInfo or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setLanguageInfo(GoogleCloudDialogflowCxV3LanguageInfo languageInfo) {
this.languageInfo = languageInfo;
return this;
}
/**
* The list of rich message responses to present to the user. Webhook can choose to append or
* replace this list in WebhookResponse.fulfillment_response;
* @return value or {@code null} for none
*/
public java.util.List getMessages() {
return messages;
}
/**
* The list of rich message responses to present to the user. Webhook can choose to append or
* replace this list in WebhookResponse.fulfillment_response;
* @param messages messages or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setMessages(java.util.List messages) {
this.messages = messages;
return this;
}
/**
* Information about page status.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3PageInfo getPageInfo() {
return pageInfo;
}
/**
* Information about page status.
* @param pageInfo pageInfo or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setPageInfo(GoogleCloudDialogflowCxV3PageInfo pageInfo) {
this.pageInfo = pageInfo;
return this;
}
/**
* Custom data set in QueryParameters.payload.
* @return value or {@code null} for none
*/
public java.util.Map getPayload() {
return payload;
}
/**
* Custom data set in QueryParameters.payload.
* @param payload payload or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setPayload(java.util.Map payload) {
this.payload = payload;
return this;
}
/**
* The sentiment analysis result of the current user request. The field is filled when sentiment
* analysis is configured to be enabled for the request.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequestSentimentAnalysisResult getSentimentAnalysisResult() {
return sentimentAnalysisResult;
}
/**
* The sentiment analysis result of the current user request. The field is filled when sentiment
* analysis is configured to be enabled for the request.
* @param sentimentAnalysisResult sentimentAnalysisResult or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setSentimentAnalysisResult(GoogleCloudDialogflowCxV3WebhookRequestSentimentAnalysisResult sentimentAnalysisResult) {
this.sentimentAnalysisResult = sentimentAnalysisResult;
return this;
}
/**
* Information about session status.
* @return value or {@code null} for none
*/
public GoogleCloudDialogflowCxV3SessionInfo getSessionInfo() {
return sessionInfo;
}
/**
* Information about session status.
* @param sessionInfo sessionInfo or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setSessionInfo(GoogleCloudDialogflowCxV3SessionInfo sessionInfo) {
this.sessionInfo = sessionInfo;
return this;
}
/**
* If natural language text was provided as input, this field will contain a copy of the text.
* @return value or {@code null} for none
*/
public java.lang.String getText() {
return text;
}
/**
* If natural language text was provided as input, this field will contain a copy of the text.
* @param text text or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setText(java.lang.String text) {
this.text = text;
return this;
}
/**
* If natural language speech audio was provided as input, this field will contain the transcript
* for the audio.
* @return value or {@code null} for none
*/
public java.lang.String getTranscript() {
return transcript;
}
/**
* If natural language speech audio was provided as input, this field will contain the transcript
* for the audio.
* @param transcript transcript or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setTranscript(java.lang.String transcript) {
this.transcript = transcript;
return this;
}
/**
* If an event was provided as input, this field will contain the name of the event.
* @return value or {@code null} for none
*/
public java.lang.String getTriggerEvent() {
return triggerEvent;
}
/**
* If an event was provided as input, this field will contain the name of the event.
* @param triggerEvent triggerEvent or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setTriggerEvent(java.lang.String triggerEvent) {
this.triggerEvent = triggerEvent;
return this;
}
/**
* If an intent was provided as input, this field will contain a copy of the intent identifier.
* Format: `projects//locations//agents//intents/`.
* @return value or {@code null} for none
*/
public java.lang.String getTriggerIntent() {
return triggerIntent;
}
/**
* If an intent was provided as input, this field will contain a copy of the intent identifier.
* Format: `projects//locations//agents//intents/`.
* @param triggerIntent triggerIntent or {@code null} for none
*/
public GoogleCloudDialogflowCxV3WebhookRequest setTriggerIntent(java.lang.String triggerIntent) {
this.triggerIntent = triggerIntent;
return this;
}
@Override
public GoogleCloudDialogflowCxV3WebhookRequest set(String fieldName, Object value) {
return (GoogleCloudDialogflowCxV3WebhookRequest) super.set(fieldName, value);
}
@Override
public GoogleCloudDialogflowCxV3WebhookRequest clone() {
return (GoogleCloudDialogflowCxV3WebhookRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy