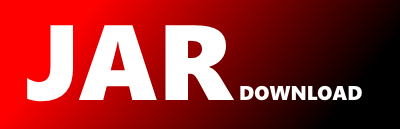
com.google.api.services.dialogflow.v3.model.GoogleCloudDialogflowV2Context Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dialogflow.v3.model;
/**
* Dialogflow contexts are similar to natural language context. If a person says to you "they are
* orange", you need context in order to understand what "they" is referring to. Similarly, for
* Dialogflow to handle an end-user expression like that, it needs to be provided with context in
* order to correctly match an intent. Using contexts, you can control the flow of a conversation.
* You can configure contexts for an intent by setting input and output contexts, which are
* identified by string names. When an intent is matched, any configured output contexts for that
* intent become active. While any contexts are active, Dialogflow is more likely to match intents
* that are configured with input contexts that correspond to the currently active contexts. For
* more information about context, see the [Contexts
* guide](https://cloud.google.com/dialogflow/docs/contexts-overview).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Dialogflow API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudDialogflowV2Context extends com.google.api.client.json.GenericJson {
/**
* Optional. The number of conversational query requests after which the context expires. The
* default is `0`. If set to `0`, the context expires immediately. Contexts expire automatically
* after 20 minutes if there are no matching queries.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer lifespanCount;
/**
* Required. The unique identifier of the context. Format: `projects//agent/sessions//contexts/`,
* or `projects//agent/environments//users//sessions//contexts/`. The `Context ID` is always
* converted to lowercase, may only contain characters in `a-zA-Z0-9_-%` and may be at most 250
* bytes long. If `Environment ID` is not specified, we assume default 'draft' environment. If
* `User ID` is not specified, we assume default '-' user. The following context names are
* reserved for internal use by Dialogflow. You should not use these contexts or create contexts
* with these names: * `__system_counters__` * `*_id_dialog_context` * `*_dialog_params_size`
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Optional. The collection of parameters associated with this context. Depending on your protocol
* or client library language, this is a map, associative array, symbol table, dictionary, or JSON
* object composed of a collection of (MapKey, MapValue) pairs: * MapKey type: string * MapKey
* value: parameter name * MapValue type: If parameter's entity type is a composite entity then
* use map, otherwise, depending on the parameter value type, it could be one of string, number,
* boolean, null, list or map. * MapValue value: If parameter's entity type is a composite entity
* then use map from composite entity property names to property values, otherwise, use parameter
* value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map parameters;
/**
* Optional. The number of conversational query requests after which the context expires. The
* default is `0`. If set to `0`, the context expires immediately. Contexts expire automatically
* after 20 minutes if there are no matching queries.
* @return value or {@code null} for none
*/
public java.lang.Integer getLifespanCount() {
return lifespanCount;
}
/**
* Optional. The number of conversational query requests after which the context expires. The
* default is `0`. If set to `0`, the context expires immediately. Contexts expire automatically
* after 20 minutes if there are no matching queries.
* @param lifespanCount lifespanCount or {@code null} for none
*/
public GoogleCloudDialogflowV2Context setLifespanCount(java.lang.Integer lifespanCount) {
this.lifespanCount = lifespanCount;
return this;
}
/**
* Required. The unique identifier of the context. Format: `projects//agent/sessions//contexts/`,
* or `projects//agent/environments//users//sessions//contexts/`. The `Context ID` is always
* converted to lowercase, may only contain characters in `a-zA-Z0-9_-%` and may be at most 250
* bytes long. If `Environment ID` is not specified, we assume default 'draft' environment. If
* `User ID` is not specified, we assume default '-' user. The following context names are
* reserved for internal use by Dialogflow. You should not use these contexts or create contexts
* with these names: * `__system_counters__` * `*_id_dialog_context` * `*_dialog_params_size`
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The unique identifier of the context. Format: `projects//agent/sessions//contexts/`,
* or `projects//agent/environments//users//sessions//contexts/`. The `Context ID` is always
* converted to lowercase, may only contain characters in `a-zA-Z0-9_-%` and may be at most 250
* bytes long. If `Environment ID` is not specified, we assume default 'draft' environment. If
* `User ID` is not specified, we assume default '-' user. The following context names are
* reserved for internal use by Dialogflow. You should not use these contexts or create contexts
* with these names: * `__system_counters__` * `*_id_dialog_context` * `*_dialog_params_size`
* @param name name or {@code null} for none
*/
public GoogleCloudDialogflowV2Context setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Optional. The collection of parameters associated with this context. Depending on your protocol
* or client library language, this is a map, associative array, symbol table, dictionary, or JSON
* object composed of a collection of (MapKey, MapValue) pairs: * MapKey type: string * MapKey
* value: parameter name * MapValue type: If parameter's entity type is a composite entity then
* use map, otherwise, depending on the parameter value type, it could be one of string, number,
* boolean, null, list or map. * MapValue value: If parameter's entity type is a composite entity
* then use map from composite entity property names to property values, otherwise, use parameter
* value.
* @return value or {@code null} for none
*/
public java.util.Map getParameters() {
return parameters;
}
/**
* Optional. The collection of parameters associated with this context. Depending on your protocol
* or client library language, this is a map, associative array, symbol table, dictionary, or JSON
* object composed of a collection of (MapKey, MapValue) pairs: * MapKey type: string * MapKey
* value: parameter name * MapValue type: If parameter's entity type is a composite entity then
* use map, otherwise, depending on the parameter value type, it could be one of string, number,
* boolean, null, list or map. * MapValue value: If parameter's entity type is a composite entity
* then use map from composite entity property names to property values, otherwise, use parameter
* value.
* @param parameters parameters or {@code null} for none
*/
public GoogleCloudDialogflowV2Context setParameters(java.util.Map parameters) {
this.parameters = parameters;
return this;
}
@Override
public GoogleCloudDialogflowV2Context set(String fieldName, Object value) {
return (GoogleCloudDialogflowV2Context) super.set(fieldName, value);
}
@Override
public GoogleCloudDialogflowV2Context clone() {
return (GoogleCloudDialogflowV2Context) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy