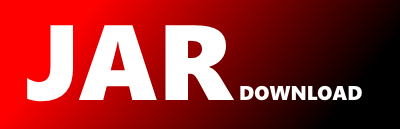
com.google.api.services.dlp.v2.model.GooglePrivacyDlpV2TimespanConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.dlp.v2.model;
/**
* Configuration of the timespan of the items to include in scanning. Currently only supported when
* inspecting Cloud Storage and BigQuery.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Sensitive Data Protection (DLP). For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GooglePrivacyDlpV2TimespanConfig extends com.google.api.client.json.GenericJson {
/**
* When the job is started by a JobTrigger we will automatically figure out a valid start_time to
* avoid scanning files that have not been modified since the last time the JobTrigger executed.
* This will be based on the time of the execution of the last run of the JobTrigger or the
* timespan end_time used in the last run of the JobTrigger. **For BigQuery** Inspect jobs
* triggered by automatic population will scan data that is at least three hours old when the job
* starts. This is because streaming buffer rows are not read during inspection and reading up to
* the current timestamp will result in skipped rows. See the [known
* issue](https://cloud.google.com/sensitive-data-protection/docs/known-issues#recently-streamed-
* data) related to this operation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean enableAutoPopulationOfTimespanConfig;
/**
* Exclude files, tables, or rows newer than this value. If not set, no upper time limit is
* applied.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String endTime;
/**
* Exclude files, tables, or rows older than this value. If not set, no lower time limit is
* applied.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String startTime;
/**
* Specification of the field containing the timestamp of scanned items. Used for data sources
* like Datastore and BigQuery. *For BigQuery* If this value is not specified and the table was
* modified between the given start and end times, the entire table will be scanned. If this value
* is specified, then rows are filtered based on the given start and end times. Rows with a `NULL`
* value in the provided BigQuery column are skipped. Valid data types of the provided BigQuery
* column are: `INTEGER`, `DATE`, `TIMESTAMP`, and `DATETIME`. If your BigQuery table is
* [partitioned at ingestion time](https://cloud.google.com/bigquery/docs/partitioned-
* tables#ingestion_time), you can use any of the following pseudo-columns as your timestamp
* field. When used with Cloud DLP, these pseudo-column names are case sensitive. - _PARTITIONTIME
* - _PARTITIONDATE - _PARTITION_LOAD_TIME *For Datastore* If this value is specified, then
* entities are filtered based on the given start and end times. If an entity does not contain the
* provided timestamp property or contains empty or invalid values, then it is included. Valid
* data types of the provided timestamp property are: `TIMESTAMP`. See the [known
* issue](https://cloud.google.com/sensitive-data-protection/docs/known-issues#bq-timespan)
* related to this operation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GooglePrivacyDlpV2FieldId timestampField;
/**
* When the job is started by a JobTrigger we will automatically figure out a valid start_time to
* avoid scanning files that have not been modified since the last time the JobTrigger executed.
* This will be based on the time of the execution of the last run of the JobTrigger or the
* timespan end_time used in the last run of the JobTrigger. **For BigQuery** Inspect jobs
* triggered by automatic population will scan data that is at least three hours old when the job
* starts. This is because streaming buffer rows are not read during inspection and reading up to
* the current timestamp will result in skipped rows. See the [known
* issue](https://cloud.google.com/sensitive-data-protection/docs/known-issues#recently-streamed-
* data) related to this operation.
* @return value or {@code null} for none
*/
public java.lang.Boolean getEnableAutoPopulationOfTimespanConfig() {
return enableAutoPopulationOfTimespanConfig;
}
/**
* When the job is started by a JobTrigger we will automatically figure out a valid start_time to
* avoid scanning files that have not been modified since the last time the JobTrigger executed.
* This will be based on the time of the execution of the last run of the JobTrigger or the
* timespan end_time used in the last run of the JobTrigger. **For BigQuery** Inspect jobs
* triggered by automatic population will scan data that is at least three hours old when the job
* starts. This is because streaming buffer rows are not read during inspection and reading up to
* the current timestamp will result in skipped rows. See the [known
* issue](https://cloud.google.com/sensitive-data-protection/docs/known-issues#recently-streamed-
* data) related to this operation.
* @param enableAutoPopulationOfTimespanConfig enableAutoPopulationOfTimespanConfig or {@code null} for none
*/
public GooglePrivacyDlpV2TimespanConfig setEnableAutoPopulationOfTimespanConfig(java.lang.Boolean enableAutoPopulationOfTimespanConfig) {
this.enableAutoPopulationOfTimespanConfig = enableAutoPopulationOfTimespanConfig;
return this;
}
/**
* Exclude files, tables, or rows newer than this value. If not set, no upper time limit is
* applied.
* @return value or {@code null} for none
*/
public String getEndTime() {
return endTime;
}
/**
* Exclude files, tables, or rows newer than this value. If not set, no upper time limit is
* applied.
* @param endTime endTime or {@code null} for none
*/
public GooglePrivacyDlpV2TimespanConfig setEndTime(String endTime) {
this.endTime = endTime;
return this;
}
/**
* Exclude files, tables, or rows older than this value. If not set, no lower time limit is
* applied.
* @return value or {@code null} for none
*/
public String getStartTime() {
return startTime;
}
/**
* Exclude files, tables, or rows older than this value. If not set, no lower time limit is
* applied.
* @param startTime startTime or {@code null} for none
*/
public GooglePrivacyDlpV2TimespanConfig setStartTime(String startTime) {
this.startTime = startTime;
return this;
}
/**
* Specification of the field containing the timestamp of scanned items. Used for data sources
* like Datastore and BigQuery. *For BigQuery* If this value is not specified and the table was
* modified between the given start and end times, the entire table will be scanned. If this value
* is specified, then rows are filtered based on the given start and end times. Rows with a `NULL`
* value in the provided BigQuery column are skipped. Valid data types of the provided BigQuery
* column are: `INTEGER`, `DATE`, `TIMESTAMP`, and `DATETIME`. If your BigQuery table is
* [partitioned at ingestion time](https://cloud.google.com/bigquery/docs/partitioned-
* tables#ingestion_time), you can use any of the following pseudo-columns as your timestamp
* field. When used with Cloud DLP, these pseudo-column names are case sensitive. - _PARTITIONTIME
* - _PARTITIONDATE - _PARTITION_LOAD_TIME *For Datastore* If this value is specified, then
* entities are filtered based on the given start and end times. If an entity does not contain the
* provided timestamp property or contains empty or invalid values, then it is included. Valid
* data types of the provided timestamp property are: `TIMESTAMP`. See the [known
* issue](https://cloud.google.com/sensitive-data-protection/docs/known-issues#bq-timespan)
* related to this operation.
* @return value or {@code null} for none
*/
public GooglePrivacyDlpV2FieldId getTimestampField() {
return timestampField;
}
/**
* Specification of the field containing the timestamp of scanned items. Used for data sources
* like Datastore and BigQuery. *For BigQuery* If this value is not specified and the table was
* modified between the given start and end times, the entire table will be scanned. If this value
* is specified, then rows are filtered based on the given start and end times. Rows with a `NULL`
* value in the provided BigQuery column are skipped. Valid data types of the provided BigQuery
* column are: `INTEGER`, `DATE`, `TIMESTAMP`, and `DATETIME`. If your BigQuery table is
* [partitioned at ingestion time](https://cloud.google.com/bigquery/docs/partitioned-
* tables#ingestion_time), you can use any of the following pseudo-columns as your timestamp
* field. When used with Cloud DLP, these pseudo-column names are case sensitive. - _PARTITIONTIME
* - _PARTITIONDATE - _PARTITION_LOAD_TIME *For Datastore* If this value is specified, then
* entities are filtered based on the given start and end times. If an entity does not contain the
* provided timestamp property or contains empty or invalid values, then it is included. Valid
* data types of the provided timestamp property are: `TIMESTAMP`. See the [known
* issue](https://cloud.google.com/sensitive-data-protection/docs/known-issues#bq-timespan)
* related to this operation.
* @param timestampField timestampField or {@code null} for none
*/
public GooglePrivacyDlpV2TimespanConfig setTimestampField(GooglePrivacyDlpV2FieldId timestampField) {
this.timestampField = timestampField;
return this;
}
@Override
public GooglePrivacyDlpV2TimespanConfig set(String fieldName, Object value) {
return (GooglePrivacyDlpV2TimespanConfig) super.set(fieldName, value);
}
@Override
public GooglePrivacyDlpV2TimespanConfig clone() {
return (GooglePrivacyDlpV2TimespanConfig) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy