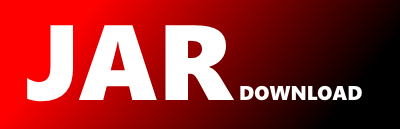
com.google.api.services.docs.v1.model.DocumentStyle Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-10-08 17:45:39 UTC)
* on 2019-04-02 at 01:09:20 UTC
* Modify at your own risk.
*/
package com.google.api.services.docs.v1.model;
/**
* The style of the document.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Docs API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class DocumentStyle extends com.google.api.client.json.GenericJson {
/**
* The background of the document.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Background background;
/**
* The ID of the default footer. If not set, there is no default footer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String defaultFooterId;
/**
* The ID of the default header. If not set, there is no default header.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String defaultHeaderId;
/**
* The ID of the footer used only for even pages. The value of use_even_page_header_footer
* determines whether to use the default_footer_id or this value for the footer on even pages. If
* not set, there is no even page footer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String evenPageFooterId;
/**
* The ID of the header used only for even pages. The value of use_even_page_header_footer
* determines whether to use the default_header_id or this value for the header on even pages. If
* not set, there is no even page header.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String evenPageHeaderId;
/**
* The ID of the footer used only for the first page. If not set then a unique footer for the
* first page does not exist. The value of use_first_page_header_footer determines whether to use
* the default_footer_id or this value for the footer on the first page. If not set, there is no
* first page footer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String firstPageFooterId;
/**
* The ID of the header used only for the first page. If not set then a unique header for the
* first page does not exist. The value of use_first_page_header_footer determines whether to use
* the default_header_id or this value for the header on the first page. If not set, there is no
* first page header.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String firstPageHeaderId;
/**
* The bottom page margin.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Dimension marginBottom;
/**
* The left page margin.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Dimension marginLeft;
/**
* The right page margin.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Dimension marginRight;
/**
* The top page margin.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Dimension marginTop;
/**
* The page number from which to start counting the number of pages.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageNumberStart;
/**
* The size of a page in the document.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Size pageSize;
/**
* Indicates whether to use the even page header / footer IDs for the even pages.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useEvenPageHeaderFooter;
/**
* Indicates whether to use the first page header / footer IDs for the first page.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean useFirstPageHeaderFooter;
/**
* The background of the document.
* @return value or {@code null} for none
*/
public Background getBackground() {
return background;
}
/**
* The background of the document.
* @param background background or {@code null} for none
*/
public DocumentStyle setBackground(Background background) {
this.background = background;
return this;
}
/**
* The ID of the default footer. If not set, there is no default footer.
* @return value or {@code null} for none
*/
public java.lang.String getDefaultFooterId() {
return defaultFooterId;
}
/**
* The ID of the default footer. If not set, there is no default footer.
* @param defaultFooterId defaultFooterId or {@code null} for none
*/
public DocumentStyle setDefaultFooterId(java.lang.String defaultFooterId) {
this.defaultFooterId = defaultFooterId;
return this;
}
/**
* The ID of the default header. If not set, there is no default header.
* @return value or {@code null} for none
*/
public java.lang.String getDefaultHeaderId() {
return defaultHeaderId;
}
/**
* The ID of the default header. If not set, there is no default header.
* @param defaultHeaderId defaultHeaderId or {@code null} for none
*/
public DocumentStyle setDefaultHeaderId(java.lang.String defaultHeaderId) {
this.defaultHeaderId = defaultHeaderId;
return this;
}
/**
* The ID of the footer used only for even pages. The value of use_even_page_header_footer
* determines whether to use the default_footer_id or this value for the footer on even pages. If
* not set, there is no even page footer.
* @return value or {@code null} for none
*/
public java.lang.String getEvenPageFooterId() {
return evenPageFooterId;
}
/**
* The ID of the footer used only for even pages. The value of use_even_page_header_footer
* determines whether to use the default_footer_id or this value for the footer on even pages. If
* not set, there is no even page footer.
* @param evenPageFooterId evenPageFooterId or {@code null} for none
*/
public DocumentStyle setEvenPageFooterId(java.lang.String evenPageFooterId) {
this.evenPageFooterId = evenPageFooterId;
return this;
}
/**
* The ID of the header used only for even pages. The value of use_even_page_header_footer
* determines whether to use the default_header_id or this value for the header on even pages. If
* not set, there is no even page header.
* @return value or {@code null} for none
*/
public java.lang.String getEvenPageHeaderId() {
return evenPageHeaderId;
}
/**
* The ID of the header used only for even pages. The value of use_even_page_header_footer
* determines whether to use the default_header_id or this value for the header on even pages. If
* not set, there is no even page header.
* @param evenPageHeaderId evenPageHeaderId or {@code null} for none
*/
public DocumentStyle setEvenPageHeaderId(java.lang.String evenPageHeaderId) {
this.evenPageHeaderId = evenPageHeaderId;
return this;
}
/**
* The ID of the footer used only for the first page. If not set then a unique footer for the
* first page does not exist. The value of use_first_page_header_footer determines whether to use
* the default_footer_id or this value for the footer on the first page. If not set, there is no
* first page footer.
* @return value or {@code null} for none
*/
public java.lang.String getFirstPageFooterId() {
return firstPageFooterId;
}
/**
* The ID of the footer used only for the first page. If not set then a unique footer for the
* first page does not exist. The value of use_first_page_header_footer determines whether to use
* the default_footer_id or this value for the footer on the first page. If not set, there is no
* first page footer.
* @param firstPageFooterId firstPageFooterId or {@code null} for none
*/
public DocumentStyle setFirstPageFooterId(java.lang.String firstPageFooterId) {
this.firstPageFooterId = firstPageFooterId;
return this;
}
/**
* The ID of the header used only for the first page. If not set then a unique header for the
* first page does not exist. The value of use_first_page_header_footer determines whether to use
* the default_header_id or this value for the header on the first page. If not set, there is no
* first page header.
* @return value or {@code null} for none
*/
public java.lang.String getFirstPageHeaderId() {
return firstPageHeaderId;
}
/**
* The ID of the header used only for the first page. If not set then a unique header for the
* first page does not exist. The value of use_first_page_header_footer determines whether to use
* the default_header_id or this value for the header on the first page. If not set, there is no
* first page header.
* @param firstPageHeaderId firstPageHeaderId or {@code null} for none
*/
public DocumentStyle setFirstPageHeaderId(java.lang.String firstPageHeaderId) {
this.firstPageHeaderId = firstPageHeaderId;
return this;
}
/**
* The bottom page margin.
* @return value or {@code null} for none
*/
public Dimension getMarginBottom() {
return marginBottom;
}
/**
* The bottom page margin.
* @param marginBottom marginBottom or {@code null} for none
*/
public DocumentStyle setMarginBottom(Dimension marginBottom) {
this.marginBottom = marginBottom;
return this;
}
/**
* The left page margin.
* @return value or {@code null} for none
*/
public Dimension getMarginLeft() {
return marginLeft;
}
/**
* The left page margin.
* @param marginLeft marginLeft or {@code null} for none
*/
public DocumentStyle setMarginLeft(Dimension marginLeft) {
this.marginLeft = marginLeft;
return this;
}
/**
* The right page margin.
* @return value or {@code null} for none
*/
public Dimension getMarginRight() {
return marginRight;
}
/**
* The right page margin.
* @param marginRight marginRight or {@code null} for none
*/
public DocumentStyle setMarginRight(Dimension marginRight) {
this.marginRight = marginRight;
return this;
}
/**
* The top page margin.
* @return value or {@code null} for none
*/
public Dimension getMarginTop() {
return marginTop;
}
/**
* The top page margin.
* @param marginTop marginTop or {@code null} for none
*/
public DocumentStyle setMarginTop(Dimension marginTop) {
this.marginTop = marginTop;
return this;
}
/**
* The page number from which to start counting the number of pages.
* @return value or {@code null} for none
*/
public java.lang.Integer getPageNumberStart() {
return pageNumberStart;
}
/**
* The page number from which to start counting the number of pages.
* @param pageNumberStart pageNumberStart or {@code null} for none
*/
public DocumentStyle setPageNumberStart(java.lang.Integer pageNumberStart) {
this.pageNumberStart = pageNumberStart;
return this;
}
/**
* The size of a page in the document.
* @return value or {@code null} for none
*/
public Size getPageSize() {
return pageSize;
}
/**
* The size of a page in the document.
* @param pageSize pageSize or {@code null} for none
*/
public DocumentStyle setPageSize(Size pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Indicates whether to use the even page header / footer IDs for the even pages.
* @return value or {@code null} for none
*/
public java.lang.Boolean getUseEvenPageHeaderFooter() {
return useEvenPageHeaderFooter;
}
/**
* Indicates whether to use the even page header / footer IDs for the even pages.
* @param useEvenPageHeaderFooter useEvenPageHeaderFooter or {@code null} for none
*/
public DocumentStyle setUseEvenPageHeaderFooter(java.lang.Boolean useEvenPageHeaderFooter) {
this.useEvenPageHeaderFooter = useEvenPageHeaderFooter;
return this;
}
/**
* Indicates whether to use the first page header / footer IDs for the first page.
* @return value or {@code null} for none
*/
public java.lang.Boolean getUseFirstPageHeaderFooter() {
return useFirstPageHeaderFooter;
}
/**
* Indicates whether to use the first page header / footer IDs for the first page.
* @param useFirstPageHeaderFooter useFirstPageHeaderFooter or {@code null} for none
*/
public DocumentStyle setUseFirstPageHeaderFooter(java.lang.Boolean useFirstPageHeaderFooter) {
this.useFirstPageHeaderFooter = useFirstPageHeaderFooter;
return this;
}
@Override
public DocumentStyle set(String fieldName, Object value) {
return (DocumentStyle) super.set(fieldName, value);
}
@Override
public DocumentStyle clone() {
return (DocumentStyle) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy