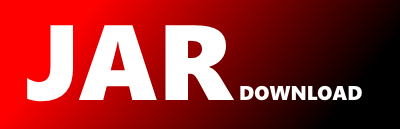
com.google.api.services.docs.v1.model.Document Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-10-08 17:45:39 UTC)
* on 2019-04-15 at 23:33:03 UTC
* Modify at your own risk.
*/
package com.google.api.services.docs.v1.model;
/**
* A Google Docs document.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Docs API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Document extends com.google.api.client.json.GenericJson {
/**
* The main body of the document.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Body body;
/**
* The ID of the document.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String documentId;
/**
* The style of the document.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private DocumentStyle documentStyle;
/**
* The footers in the document, keyed by footer ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map footers;
/**
* The footnotes in the document, keyed by footnote ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map footnotes;
/**
* The headers in the document, keyed by header ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map headers;
/**
* The inline objects in the document, keyed by object ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map inlineObjects;
/**
* The lists in the document, keyed by list ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map lists;
static {
// hack to force ProGuard to consider List used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(List.class);
}
/**
* The named ranges in the document, keyed by name.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map namedRanges;
/**
* The named styles of the document.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NamedStyles namedStyles;
/**
* The positioned objects in the document, keyed by object ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map positionedObjects;
static {
// hack to force ProGuard to consider PositionedObject used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(PositionedObject.class);
}
/**
* The revision ID of the document. Can be used in update requests to specify which revision of a
* document to apply updates to and how the request should behave if the document has been edited
* since that revision. Only populated if the user has edit access to the document.
*
* The format of the revision ID may change over time, so it should be treated opaquely. A
* returned revision ID is only guaranteed to be valid for 24 hours after it has been returned and
* cannot be shared across users. If the revision ID is unchanged between calls, then the document
* has not changed. Conversely, a changed ID (for the same document and user) usually means the
* document has been updated; however, a changed ID can also be due to internal factors such as ID
* format changes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String revisionId;
/**
* The suggested changes to the style of the document, keyed by suggestion ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map suggestedDocumentStyleChanges;
/**
* The suggested changes to the named styles of the document, keyed by suggestion ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map suggestedNamedStylesChanges;
static {
// hack to force ProGuard to consider SuggestedNamedStyles used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(SuggestedNamedStyles.class);
}
/**
* The suggestions view mode applied to the document.
*
* Note: When editing a document, changes must be based on a document with SUGGESTIONS_INLINE.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String suggestionsViewMode;
/**
* The title of the document.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* The main body of the document.
* @return value or {@code null} for none
*/
public Body getBody() {
return body;
}
/**
* The main body of the document.
* @param body body or {@code null} for none
*/
public Document setBody(Body body) {
this.body = body;
return this;
}
/**
* The ID of the document.
* @return value or {@code null} for none
*/
public java.lang.String getDocumentId() {
return documentId;
}
/**
* The ID of the document.
* @param documentId documentId or {@code null} for none
*/
public Document setDocumentId(java.lang.String documentId) {
this.documentId = documentId;
return this;
}
/**
* The style of the document.
* @return value or {@code null} for none
*/
public DocumentStyle getDocumentStyle() {
return documentStyle;
}
/**
* The style of the document.
* @param documentStyle documentStyle or {@code null} for none
*/
public Document setDocumentStyle(DocumentStyle documentStyle) {
this.documentStyle = documentStyle;
return this;
}
/**
* The footers in the document, keyed by footer ID.
* @return value or {@code null} for none
*/
public java.util.Map getFooters() {
return footers;
}
/**
* The footers in the document, keyed by footer ID.
* @param footers footers or {@code null} for none
*/
public Document setFooters(java.util.Map footers) {
this.footers = footers;
return this;
}
/**
* The footnotes in the document, keyed by footnote ID.
* @return value or {@code null} for none
*/
public java.util.Map getFootnotes() {
return footnotes;
}
/**
* The footnotes in the document, keyed by footnote ID.
* @param footnotes footnotes or {@code null} for none
*/
public Document setFootnotes(java.util.Map footnotes) {
this.footnotes = footnotes;
return this;
}
/**
* The headers in the document, keyed by header ID.
* @return value or {@code null} for none
*/
public java.util.Map getHeaders() {
return headers;
}
/**
* The headers in the document, keyed by header ID.
* @param headers headers or {@code null} for none
*/
public Document setHeaders(java.util.Map headers) {
this.headers = headers;
return this;
}
/**
* The inline objects in the document, keyed by object ID.
* @return value or {@code null} for none
*/
public java.util.Map getInlineObjects() {
return inlineObjects;
}
/**
* The inline objects in the document, keyed by object ID.
* @param inlineObjects inlineObjects or {@code null} for none
*/
public Document setInlineObjects(java.util.Map inlineObjects) {
this.inlineObjects = inlineObjects;
return this;
}
/**
* The lists in the document, keyed by list ID.
* @return value or {@code null} for none
*/
public java.util.Map getLists() {
return lists;
}
/**
* The lists in the document, keyed by list ID.
* @param lists lists or {@code null} for none
*/
public Document setLists(java.util.Map lists) {
this.lists = lists;
return this;
}
/**
* The named ranges in the document, keyed by name.
* @return value or {@code null} for none
*/
public java.util.Map getNamedRanges() {
return namedRanges;
}
/**
* The named ranges in the document, keyed by name.
* @param namedRanges namedRanges or {@code null} for none
*/
public Document setNamedRanges(java.util.Map namedRanges) {
this.namedRanges = namedRanges;
return this;
}
/**
* The named styles of the document.
* @return value or {@code null} for none
*/
public NamedStyles getNamedStyles() {
return namedStyles;
}
/**
* The named styles of the document.
* @param namedStyles namedStyles or {@code null} for none
*/
public Document setNamedStyles(NamedStyles namedStyles) {
this.namedStyles = namedStyles;
return this;
}
/**
* The positioned objects in the document, keyed by object ID.
* @return value or {@code null} for none
*/
public java.util.Map getPositionedObjects() {
return positionedObjects;
}
/**
* The positioned objects in the document, keyed by object ID.
* @param positionedObjects positionedObjects or {@code null} for none
*/
public Document setPositionedObjects(java.util.Map positionedObjects) {
this.positionedObjects = positionedObjects;
return this;
}
/**
* The revision ID of the document. Can be used in update requests to specify which revision of a
* document to apply updates to and how the request should behave if the document has been edited
* since that revision. Only populated if the user has edit access to the document.
*
* The format of the revision ID may change over time, so it should be treated opaquely. A
* returned revision ID is only guaranteed to be valid for 24 hours after it has been returned and
* cannot be shared across users. If the revision ID is unchanged between calls, then the document
* has not changed. Conversely, a changed ID (for the same document and user) usually means the
* document has been updated; however, a changed ID can also be due to internal factors such as ID
* format changes.
* @return value or {@code null} for none
*/
public java.lang.String getRevisionId() {
return revisionId;
}
/**
* The revision ID of the document. Can be used in update requests to specify which revision of a
* document to apply updates to and how the request should behave if the document has been edited
* since that revision. Only populated if the user has edit access to the document.
*
* The format of the revision ID may change over time, so it should be treated opaquely. A
* returned revision ID is only guaranteed to be valid for 24 hours after it has been returned and
* cannot be shared across users. If the revision ID is unchanged between calls, then the document
* has not changed. Conversely, a changed ID (for the same document and user) usually means the
* document has been updated; however, a changed ID can also be due to internal factors such as ID
* format changes.
* @param revisionId revisionId or {@code null} for none
*/
public Document setRevisionId(java.lang.String revisionId) {
this.revisionId = revisionId;
return this;
}
/**
* The suggested changes to the style of the document, keyed by suggestion ID.
* @return value or {@code null} for none
*/
public java.util.Map getSuggestedDocumentStyleChanges() {
return suggestedDocumentStyleChanges;
}
/**
* The suggested changes to the style of the document, keyed by suggestion ID.
* @param suggestedDocumentStyleChanges suggestedDocumentStyleChanges or {@code null} for none
*/
public Document setSuggestedDocumentStyleChanges(java.util.Map suggestedDocumentStyleChanges) {
this.suggestedDocumentStyleChanges = suggestedDocumentStyleChanges;
return this;
}
/**
* The suggested changes to the named styles of the document, keyed by suggestion ID.
* @return value or {@code null} for none
*/
public java.util.Map getSuggestedNamedStylesChanges() {
return suggestedNamedStylesChanges;
}
/**
* The suggested changes to the named styles of the document, keyed by suggestion ID.
* @param suggestedNamedStylesChanges suggestedNamedStylesChanges or {@code null} for none
*/
public Document setSuggestedNamedStylesChanges(java.util.Map suggestedNamedStylesChanges) {
this.suggestedNamedStylesChanges = suggestedNamedStylesChanges;
return this;
}
/**
* The suggestions view mode applied to the document.
*
* Note: When editing a document, changes must be based on a document with SUGGESTIONS_INLINE.
* @return value or {@code null} for none
*/
public java.lang.String getSuggestionsViewMode() {
return suggestionsViewMode;
}
/**
* The suggestions view mode applied to the document.
*
* Note: When editing a document, changes must be based on a document with SUGGESTIONS_INLINE.
* @param suggestionsViewMode suggestionsViewMode or {@code null} for none
*/
public Document setSuggestionsViewMode(java.lang.String suggestionsViewMode) {
this.suggestionsViewMode = suggestionsViewMode;
return this;
}
/**
* The title of the document.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* The title of the document.
* @param title title or {@code null} for none
*/
public Document setTitle(java.lang.String title) {
this.title = title;
return this;
}
@Override
public Document set(String fieldName, Object value) {
return (Document) super.set(fieldName, value);
}
@Override
public Document clone() {
return (Document) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy