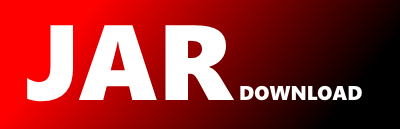
com.google.api.services.docs.v1.model.ParagraphStyle Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2018-10-08 17:45:39 UTC)
* on 2019-02-19 at 20:44:23 UTC
* Modify at your own risk.
*/
package com.google.api.services.docs.v1.model;
/**
* Styles that apply to a whole paragraph.
*
* Inherited paragraph styles are represented as unset fields in this message. A paragraph style's
* parent depends on where the paragraph style is defined:
*
* * The ParagraphStyle on a Paragraph inherits from the paragraph's corresponding named style
* type. * The ParagraphStyle on a named style inherits from the normal text named style. *
* The ParagraphStyle of the normal text named style inherits from the default paragraph style
* in the Docs editor. * The ParagraphStyle on a Paragraph element that is contained in a
* table may inherit its paragraph style from the table style.
*
* If the paragraph style does not inherit from a parent, unsetting fields will revert the style to
* a value matching the defaults in the Docs editor.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Docs API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ParagraphStyle extends com.google.api.client.json.GenericJson {
/**
* The text alignment for this paragraph.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String alignment;
/**
* Whether to avoid widows and orphans for the paragraph. If unset, the value is inherited from
* the parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean avoidWidowAndOrphan;
/**
* The border between this paragraph and the next and previous paragraphs. If unset, the value is
* inherited from the parent.
*
* The between border is rendered when the adjacent paragraph has the same border and indent
* properties.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ParagraphBorder borderBetween;
/**
* The border at the bottom of this paragraph. If unset, the value is inherited from the parent.
*
* The bottom border is rendered when the paragraph below has different border and indent
* properties.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ParagraphBorder borderBottom;
/**
* The border to the left of this paragraph. If unset, the value is inherited from the parent.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ParagraphBorder borderLeft;
/**
* The border to the right of this paragraph. If unset, the value is inherited from the parent.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ParagraphBorder borderRight;
/**
* The border at the top of this paragraph. If unset, the value is inherited from the parent.
*
* The top border is rendered when the paragraph above has different border and indent properties.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ParagraphBorder borderTop;
/**
* The text direction of this paragraph. If unset, the value defaults to LEFT_TO_RIGHT since
* paragraph direction is not inherited.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String direction;
/**
* The heading ID of the paragraph. If empty, then this paragraph is not a heading. This property
* is read-only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String headingId;
/**
* The amount of indentation for the paragraph on the side that corresponds to the end of the
* text, based on the current paragraph direction. If unset, the value is inherited from the
* parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Dimension indentEnd;
/**
* The amount of indentation for the first line of the paragraph. If unset, the value is inherited
* from the parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Dimension indentFirstLine;
/**
* The amount of indentation for the paragraph on the side that corresponds to the start of the
* text, based on the current paragraph direction. If unset, the value is inherited from the
* parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Dimension indentStart;
/**
* Whether all lines of the paragraph should be laid out on the same page or column if possible.
* If unset, the value is inherited from the parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean keepLinesTogether;
/**
* Whether at least a part of this paragraph should be laid out on the same page or column as the
* next paragraph if possible. If unset, the value is inherited from the parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean keepWithNext;
/**
* The amount of space between lines, as a percentage of normal, where normal is represented as
* 100.0. If unset, the value is inherited from the parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Float lineSpacing;
/**
* The named style type of the paragraph.
*
* Since updating the named style type affects other properties within ParagraphStyle, the named
* style type is applied before the other properties are updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String namedStyleType;
/**
* The shading of the paragraph. If unset, the value is inherited from the parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Shading shading;
/**
* The amount of extra space above the paragraph. If unset, the value is inherited from the
* parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Dimension spaceAbove;
/**
* The amount of extra space below the paragraph. If unset, the value is inherited from the
* parent.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Dimension spaceBelow;
/**
* The spacing mode for the paragraph.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String spacingMode;
/**
* A list of the tab stops for this paragraph. The list of tab stops is not inherited. This
* property is read-only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List tabStops;
/**
* The text alignment for this paragraph.
* @return value or {@code null} for none
*/
public java.lang.String getAlignment() {
return alignment;
}
/**
* The text alignment for this paragraph.
* @param alignment alignment or {@code null} for none
*/
public ParagraphStyle setAlignment(java.lang.String alignment) {
this.alignment = alignment;
return this;
}
/**
* Whether to avoid widows and orphans for the paragraph. If unset, the value is inherited from
* the parent.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAvoidWidowAndOrphan() {
return avoidWidowAndOrphan;
}
/**
* Whether to avoid widows and orphans for the paragraph. If unset, the value is inherited from
* the parent.
* @param avoidWidowAndOrphan avoidWidowAndOrphan or {@code null} for none
*/
public ParagraphStyle setAvoidWidowAndOrphan(java.lang.Boolean avoidWidowAndOrphan) {
this.avoidWidowAndOrphan = avoidWidowAndOrphan;
return this;
}
/**
* The border between this paragraph and the next and previous paragraphs. If unset, the value is
* inherited from the parent.
*
* The between border is rendered when the adjacent paragraph has the same border and indent
* properties.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @return value or {@code null} for none
*/
public ParagraphBorder getBorderBetween() {
return borderBetween;
}
/**
* The border between this paragraph and the next and previous paragraphs. If unset, the value is
* inherited from the parent.
*
* The between border is rendered when the adjacent paragraph has the same border and indent
* properties.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @param borderBetween borderBetween or {@code null} for none
*/
public ParagraphStyle setBorderBetween(ParagraphBorder borderBetween) {
this.borderBetween = borderBetween;
return this;
}
/**
* The border at the bottom of this paragraph. If unset, the value is inherited from the parent.
*
* The bottom border is rendered when the paragraph below has different border and indent
* properties.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @return value or {@code null} for none
*/
public ParagraphBorder getBorderBottom() {
return borderBottom;
}
/**
* The border at the bottom of this paragraph. If unset, the value is inherited from the parent.
*
* The bottom border is rendered when the paragraph below has different border and indent
* properties.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @param borderBottom borderBottom or {@code null} for none
*/
public ParagraphStyle setBorderBottom(ParagraphBorder borderBottom) {
this.borderBottom = borderBottom;
return this;
}
/**
* The border to the left of this paragraph. If unset, the value is inherited from the parent.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @return value or {@code null} for none
*/
public ParagraphBorder getBorderLeft() {
return borderLeft;
}
/**
* The border to the left of this paragraph. If unset, the value is inherited from the parent.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @param borderLeft borderLeft or {@code null} for none
*/
public ParagraphStyle setBorderLeft(ParagraphBorder borderLeft) {
this.borderLeft = borderLeft;
return this;
}
/**
* The border to the right of this paragraph. If unset, the value is inherited from the parent.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @return value or {@code null} for none
*/
public ParagraphBorder getBorderRight() {
return borderRight;
}
/**
* The border to the right of this paragraph. If unset, the value is inherited from the parent.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @param borderRight borderRight or {@code null} for none
*/
public ParagraphStyle setBorderRight(ParagraphBorder borderRight) {
this.borderRight = borderRight;
return this;
}
/**
* The border at the top of this paragraph. If unset, the value is inherited from the parent.
*
* The top border is rendered when the paragraph above has different border and indent properties.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @return value or {@code null} for none
*/
public ParagraphBorder getBorderTop() {
return borderTop;
}
/**
* The border at the top of this paragraph. If unset, the value is inherited from the parent.
*
* The top border is rendered when the paragraph above has different border and indent properties.
*
* Paragraph borders cannot be partially updated. When making changes to a paragraph border the
* new border must be specified in its entirety.
* @param borderTop borderTop or {@code null} for none
*/
public ParagraphStyle setBorderTop(ParagraphBorder borderTop) {
this.borderTop = borderTop;
return this;
}
/**
* The text direction of this paragraph. If unset, the value defaults to LEFT_TO_RIGHT since
* paragraph direction is not inherited.
* @return value or {@code null} for none
*/
public java.lang.String getDirection() {
return direction;
}
/**
* The text direction of this paragraph. If unset, the value defaults to LEFT_TO_RIGHT since
* paragraph direction is not inherited.
* @param direction direction or {@code null} for none
*/
public ParagraphStyle setDirection(java.lang.String direction) {
this.direction = direction;
return this;
}
/**
* The heading ID of the paragraph. If empty, then this paragraph is not a heading. This property
* is read-only.
* @return value or {@code null} for none
*/
public java.lang.String getHeadingId() {
return headingId;
}
/**
* The heading ID of the paragraph. If empty, then this paragraph is not a heading. This property
* is read-only.
* @param headingId headingId or {@code null} for none
*/
public ParagraphStyle setHeadingId(java.lang.String headingId) {
this.headingId = headingId;
return this;
}
/**
* The amount of indentation for the paragraph on the side that corresponds to the end of the
* text, based on the current paragraph direction. If unset, the value is inherited from the
* parent.
* @return value or {@code null} for none
*/
public Dimension getIndentEnd() {
return indentEnd;
}
/**
* The amount of indentation for the paragraph on the side that corresponds to the end of the
* text, based on the current paragraph direction. If unset, the value is inherited from the
* parent.
* @param indentEnd indentEnd or {@code null} for none
*/
public ParagraphStyle setIndentEnd(Dimension indentEnd) {
this.indentEnd = indentEnd;
return this;
}
/**
* The amount of indentation for the first line of the paragraph. If unset, the value is inherited
* from the parent.
* @return value or {@code null} for none
*/
public Dimension getIndentFirstLine() {
return indentFirstLine;
}
/**
* The amount of indentation for the first line of the paragraph. If unset, the value is inherited
* from the parent.
* @param indentFirstLine indentFirstLine or {@code null} for none
*/
public ParagraphStyle setIndentFirstLine(Dimension indentFirstLine) {
this.indentFirstLine = indentFirstLine;
return this;
}
/**
* The amount of indentation for the paragraph on the side that corresponds to the start of the
* text, based on the current paragraph direction. If unset, the value is inherited from the
* parent.
* @return value or {@code null} for none
*/
public Dimension getIndentStart() {
return indentStart;
}
/**
* The amount of indentation for the paragraph on the side that corresponds to the start of the
* text, based on the current paragraph direction. If unset, the value is inherited from the
* parent.
* @param indentStart indentStart or {@code null} for none
*/
public ParagraphStyle setIndentStart(Dimension indentStart) {
this.indentStart = indentStart;
return this;
}
/**
* Whether all lines of the paragraph should be laid out on the same page or column if possible.
* If unset, the value is inherited from the parent.
* @return value or {@code null} for none
*/
public java.lang.Boolean getKeepLinesTogether() {
return keepLinesTogether;
}
/**
* Whether all lines of the paragraph should be laid out on the same page or column if possible.
* If unset, the value is inherited from the parent.
* @param keepLinesTogether keepLinesTogether or {@code null} for none
*/
public ParagraphStyle setKeepLinesTogether(java.lang.Boolean keepLinesTogether) {
this.keepLinesTogether = keepLinesTogether;
return this;
}
/**
* Whether at least a part of this paragraph should be laid out on the same page or column as the
* next paragraph if possible. If unset, the value is inherited from the parent.
* @return value or {@code null} for none
*/
public java.lang.Boolean getKeepWithNext() {
return keepWithNext;
}
/**
* Whether at least a part of this paragraph should be laid out on the same page or column as the
* next paragraph if possible. If unset, the value is inherited from the parent.
* @param keepWithNext keepWithNext or {@code null} for none
*/
public ParagraphStyle setKeepWithNext(java.lang.Boolean keepWithNext) {
this.keepWithNext = keepWithNext;
return this;
}
/**
* The amount of space between lines, as a percentage of normal, where normal is represented as
* 100.0. If unset, the value is inherited from the parent.
* @return value or {@code null} for none
*/
public java.lang.Float getLineSpacing() {
return lineSpacing;
}
/**
* The amount of space between lines, as a percentage of normal, where normal is represented as
* 100.0. If unset, the value is inherited from the parent.
* @param lineSpacing lineSpacing or {@code null} for none
*/
public ParagraphStyle setLineSpacing(java.lang.Float lineSpacing) {
this.lineSpacing = lineSpacing;
return this;
}
/**
* The named style type of the paragraph.
*
* Since updating the named style type affects other properties within ParagraphStyle, the named
* style type is applied before the other properties are updated.
* @return value or {@code null} for none
*/
public java.lang.String getNamedStyleType() {
return namedStyleType;
}
/**
* The named style type of the paragraph.
*
* Since updating the named style type affects other properties within ParagraphStyle, the named
* style type is applied before the other properties are updated.
* @param namedStyleType namedStyleType or {@code null} for none
*/
public ParagraphStyle setNamedStyleType(java.lang.String namedStyleType) {
this.namedStyleType = namedStyleType;
return this;
}
/**
* The shading of the paragraph. If unset, the value is inherited from the parent.
* @return value or {@code null} for none
*/
public Shading getShading() {
return shading;
}
/**
* The shading of the paragraph. If unset, the value is inherited from the parent.
* @param shading shading or {@code null} for none
*/
public ParagraphStyle setShading(Shading shading) {
this.shading = shading;
return this;
}
/**
* The amount of extra space above the paragraph. If unset, the value is inherited from the
* parent.
* @return value or {@code null} for none
*/
public Dimension getSpaceAbove() {
return spaceAbove;
}
/**
* The amount of extra space above the paragraph. If unset, the value is inherited from the
* parent.
* @param spaceAbove spaceAbove or {@code null} for none
*/
public ParagraphStyle setSpaceAbove(Dimension spaceAbove) {
this.spaceAbove = spaceAbove;
return this;
}
/**
* The amount of extra space below the paragraph. If unset, the value is inherited from the
* parent.
* @return value or {@code null} for none
*/
public Dimension getSpaceBelow() {
return spaceBelow;
}
/**
* The amount of extra space below the paragraph. If unset, the value is inherited from the
* parent.
* @param spaceBelow spaceBelow or {@code null} for none
*/
public ParagraphStyle setSpaceBelow(Dimension spaceBelow) {
this.spaceBelow = spaceBelow;
return this;
}
/**
* The spacing mode for the paragraph.
* @return value or {@code null} for none
*/
public java.lang.String getSpacingMode() {
return spacingMode;
}
/**
* The spacing mode for the paragraph.
* @param spacingMode spacingMode or {@code null} for none
*/
public ParagraphStyle setSpacingMode(java.lang.String spacingMode) {
this.spacingMode = spacingMode;
return this;
}
/**
* A list of the tab stops for this paragraph. The list of tab stops is not inherited. This
* property is read-only.
* @return value or {@code null} for none
*/
public java.util.List getTabStops() {
return tabStops;
}
/**
* A list of the tab stops for this paragraph. The list of tab stops is not inherited. This
* property is read-only.
* @param tabStops tabStops or {@code null} for none
*/
public ParagraphStyle setTabStops(java.util.List tabStops) {
this.tabStops = tabStops;
return this;
}
@Override
public ParagraphStyle set(String fieldName, Object value) {
return (ParagraphStyle) super.set(fieldName, value);
}
@Override
public ParagraphStyle clone() {
return (ParagraphStyle) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy