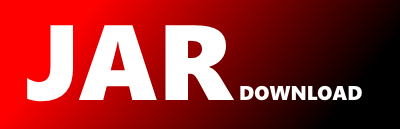
com.google.api.services.doubleclicksearch.model.ReportRequest Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-27 16:00:31 UTC)
* on 2016-08-03 at 20:19:09 UTC
* Modify at your own risk.
*/
package com.google.api.services.doubleclicksearch.model;
/**
* A request object used to create a DoubleClick Search report.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the DoubleClick Search API. For a detailed explanation
* see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ReportRequest extends com.google.api.client.json.GenericJson {
/**
* The columns to include in the report. This includes both DoubleClick Search columns and saved
* columns. For DoubleClick Search columns, only the columnName parameter is required. For saved
* columns only the savedColumnName parameter is required. Both columnName and savedColumnName
* cannot be set in the same stanza.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List columns;
static {
// hack to force ProGuard to consider ReportApiColumnSpec used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(ReportApiColumnSpec.class);
}
/**
* Format that the report should be returned in. Currently csv or tsv is supported.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String downloadFormat;
/**
* A list of filters to be applied to the report.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List filters;
static {
// hack to force ProGuard to consider Filters used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Filters.class);
}
/**
* Determines if removed entities should be included in the report. Defaults to false. Deprecated,
* please use includeRemovedEntities instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeDeletedEntities;
/**
* Determines if removed entities should be included in the report. Defaults to false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeRemovedEntities;
/**
* Asynchronous report only. The maximum number of rows per report file. A large report is split
* into many files based on this field. Acceptable values are 1000000 to 100000000, inclusive.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxRowsPerFile;
/**
* Synchronous report only. A list of columns and directions defining sorting to be performed on
* the report rows.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List orderBy;
static {
// hack to force ProGuard to consider OrderBy used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(OrderBy.class);
}
/**
* The reportScope is a set of IDs that are used to determine which subset of entities will be
* returned in the report. The full lineage of IDs from the lowest scoped level desired up through
* agency is required.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ReportScope reportScope;
/**
* Determines the type of rows that are returned in the report. For example, if you specify
* reportType: keyword, each row in the report will contain data about a keyword. See the Types of
* Reports reference for the columns that are available for each type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String reportType;
/**
* Synchronous report only. The maxinum number of rows to return; additional rows are dropped.
* Acceptable values are 0 to 10000, inclusive. Defaults to 10000.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer rowCount;
/**
* Synchronous report only. Zero-based index of the first row to return. Acceptable values are 0
* to 50000, inclusive. Defaults to 0.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer startRow;
/**
* Specifies the currency in which monetary will be returned. Possible values are: usd, agency
* (valid if the report is scoped to agency or lower), advertiser (valid if the report is scoped
* to * advertiser or lower), or account (valid if the report is scoped to engine account or
* lower).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String statisticsCurrency;
/**
* If metrics are requested in a report, this argument will be used to restrict the metrics to a
* specific time range.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TimeRange timeRange;
/**
* If true, the report would only be created if all the requested stat data are sourced from a
* single timezone. Defaults to false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean verifySingleTimeZone;
/**
* The columns to include in the report. This includes both DoubleClick Search columns and saved
* columns. For DoubleClick Search columns, only the columnName parameter is required. For saved
* columns only the savedColumnName parameter is required. Both columnName and savedColumnName
* cannot be set in the same stanza.
* @return value or {@code null} for none
*/
public java.util.List getColumns() {
return columns;
}
/**
* The columns to include in the report. This includes both DoubleClick Search columns and saved
* columns. For DoubleClick Search columns, only the columnName parameter is required. For saved
* columns only the savedColumnName parameter is required. Both columnName and savedColumnName
* cannot be set in the same stanza.
* @param columns columns or {@code null} for none
*/
public ReportRequest setColumns(java.util.List columns) {
this.columns = columns;
return this;
}
/**
* Format that the report should be returned in. Currently csv or tsv is supported.
* @return value or {@code null} for none
*/
public java.lang.String getDownloadFormat() {
return downloadFormat;
}
/**
* Format that the report should be returned in. Currently csv or tsv is supported.
* @param downloadFormat downloadFormat or {@code null} for none
*/
public ReportRequest setDownloadFormat(java.lang.String downloadFormat) {
this.downloadFormat = downloadFormat;
return this;
}
/**
* A list of filters to be applied to the report.
* @return value or {@code null} for none
*/
public java.util.List getFilters() {
return filters;
}
/**
* A list of filters to be applied to the report.
* @param filters filters or {@code null} for none
*/
public ReportRequest setFilters(java.util.List filters) {
this.filters = filters;
return this;
}
/**
* Determines if removed entities should be included in the report. Defaults to false. Deprecated,
* please use includeRemovedEntities instead.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIncludeDeletedEntities() {
return includeDeletedEntities;
}
/**
* Determines if removed entities should be included in the report. Defaults to false. Deprecated,
* please use includeRemovedEntities instead.
* @param includeDeletedEntities includeDeletedEntities or {@code null} for none
*/
public ReportRequest setIncludeDeletedEntities(java.lang.Boolean includeDeletedEntities) {
this.includeDeletedEntities = includeDeletedEntities;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Determines if removed entities should be included in the report. Defaults to false. Deprecated,
please use includeRemovedEntities instead.
*
*/
public boolean isIncludeDeletedEntities() {
if (includeDeletedEntities == null || includeDeletedEntities == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return includeDeletedEntities;
}
/**
* Determines if removed entities should be included in the report. Defaults to false.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIncludeRemovedEntities() {
return includeRemovedEntities;
}
/**
* Determines if removed entities should be included in the report. Defaults to false.
* @param includeRemovedEntities includeRemovedEntities or {@code null} for none
*/
public ReportRequest setIncludeRemovedEntities(java.lang.Boolean includeRemovedEntities) {
this.includeRemovedEntities = includeRemovedEntities;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Determines if removed entities should be included in the report. Defaults to false.
*
*/
public boolean isIncludeRemovedEntities() {
if (includeRemovedEntities == null || includeRemovedEntities == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return includeRemovedEntities;
}
/**
* Asynchronous report only. The maximum number of rows per report file. A large report is split
* into many files based on this field. Acceptable values are 1000000 to 100000000, inclusive.
* @return value or {@code null} for none
*/
public java.lang.Integer getMaxRowsPerFile() {
return maxRowsPerFile;
}
/**
* Asynchronous report only. The maximum number of rows per report file. A large report is split
* into many files based on this field. Acceptable values are 1000000 to 100000000, inclusive.
* @param maxRowsPerFile maxRowsPerFile or {@code null} for none
*/
public ReportRequest setMaxRowsPerFile(java.lang.Integer maxRowsPerFile) {
this.maxRowsPerFile = maxRowsPerFile;
return this;
}
/**
* Synchronous report only. A list of columns and directions defining sorting to be performed on
* the report rows.
* @return value or {@code null} for none
*/
public java.util.List getOrderBy() {
return orderBy;
}
/**
* Synchronous report only. A list of columns and directions defining sorting to be performed on
* the report rows.
* @param orderBy orderBy or {@code null} for none
*/
public ReportRequest setOrderBy(java.util.List orderBy) {
this.orderBy = orderBy;
return this;
}
/**
* The reportScope is a set of IDs that are used to determine which subset of entities will be
* returned in the report. The full lineage of IDs from the lowest scoped level desired up through
* agency is required.
* @return value or {@code null} for none
*/
public ReportScope getReportScope() {
return reportScope;
}
/**
* The reportScope is a set of IDs that are used to determine which subset of entities will be
* returned in the report. The full lineage of IDs from the lowest scoped level desired up through
* agency is required.
* @param reportScope reportScope or {@code null} for none
*/
public ReportRequest setReportScope(ReportScope reportScope) {
this.reportScope = reportScope;
return this;
}
/**
* Determines the type of rows that are returned in the report. For example, if you specify
* reportType: keyword, each row in the report will contain data about a keyword. See the Types of
* Reports reference for the columns that are available for each type.
* @return value or {@code null} for none
*/
public java.lang.String getReportType() {
return reportType;
}
/**
* Determines the type of rows that are returned in the report. For example, if you specify
* reportType: keyword, each row in the report will contain data about a keyword. See the Types of
* Reports reference for the columns that are available for each type.
* @param reportType reportType or {@code null} for none
*/
public ReportRequest setReportType(java.lang.String reportType) {
this.reportType = reportType;
return this;
}
/**
* Synchronous report only. The maxinum number of rows to return; additional rows are dropped.
* Acceptable values are 0 to 10000, inclusive. Defaults to 10000.
* @return value or {@code null} for none
*/
public java.lang.Integer getRowCount() {
return rowCount;
}
/**
* Synchronous report only. The maxinum number of rows to return; additional rows are dropped.
* Acceptable values are 0 to 10000, inclusive. Defaults to 10000.
* @param rowCount rowCount or {@code null} for none
*/
public ReportRequest setRowCount(java.lang.Integer rowCount) {
this.rowCount = rowCount;
return this;
}
/**
* Synchronous report only. Zero-based index of the first row to return. Acceptable values are 0
* to 50000, inclusive. Defaults to 0.
* @return value or {@code null} for none
*/
public java.lang.Integer getStartRow() {
return startRow;
}
/**
* Synchronous report only. Zero-based index of the first row to return. Acceptable values are 0
* to 50000, inclusive. Defaults to 0.
* @param startRow startRow or {@code null} for none
*/
public ReportRequest setStartRow(java.lang.Integer startRow) {
this.startRow = startRow;
return this;
}
/**
* Specifies the currency in which monetary will be returned. Possible values are: usd, agency
* (valid if the report is scoped to agency or lower), advertiser (valid if the report is scoped
* to * advertiser or lower), or account (valid if the report is scoped to engine account or
* lower).
* @return value or {@code null} for none
*/
public java.lang.String getStatisticsCurrency() {
return statisticsCurrency;
}
/**
* Specifies the currency in which monetary will be returned. Possible values are: usd, agency
* (valid if the report is scoped to agency or lower), advertiser (valid if the report is scoped
* to * advertiser or lower), or account (valid if the report is scoped to engine account or
* lower).
* @param statisticsCurrency statisticsCurrency or {@code null} for none
*/
public ReportRequest setStatisticsCurrency(java.lang.String statisticsCurrency) {
this.statisticsCurrency = statisticsCurrency;
return this;
}
/**
* If metrics are requested in a report, this argument will be used to restrict the metrics to a
* specific time range.
* @return value or {@code null} for none
*/
public TimeRange getTimeRange() {
return timeRange;
}
/**
* If metrics are requested in a report, this argument will be used to restrict the metrics to a
* specific time range.
* @param timeRange timeRange or {@code null} for none
*/
public ReportRequest setTimeRange(TimeRange timeRange) {
this.timeRange = timeRange;
return this;
}
/**
* If true, the report would only be created if all the requested stat data are sourced from a
* single timezone. Defaults to false.
* @return value or {@code null} for none
*/
public java.lang.Boolean getVerifySingleTimeZone() {
return verifySingleTimeZone;
}
/**
* If true, the report would only be created if all the requested stat data are sourced from a
* single timezone. Defaults to false.
* @param verifySingleTimeZone verifySingleTimeZone or {@code null} for none
*/
public ReportRequest setVerifySingleTimeZone(java.lang.Boolean verifySingleTimeZone) {
this.verifySingleTimeZone = verifySingleTimeZone;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* If true, the report would only be created if all the requested stat data are sourced from a single
timezone. Defaults to false.
*
*/
public boolean isVerifySingleTimeZone() {
if (verifySingleTimeZone == null || verifySingleTimeZone == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return verifySingleTimeZone;
}
@Override
public ReportRequest set(String fieldName, Object value) {
return (ReportRequest) super.set(fieldName, value);
}
@Override
public ReportRequest clone() {
return (ReportRequest) super.clone();
}
/**
* Model definition for ReportRequestFilters.
*/
public static final class Filters extends com.google.api.client.json.GenericJson {
/**
* Column to perform the filter on. This can be a DoubleClick Search column or a saved column.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ReportApiColumnSpec column;
/**
* Operator to use in the filter. See the filter reference for a list of available operators.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String operator;
/**
* A list of values to filter the column value against.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List values;
/**
* Column to perform the filter on. This can be a DoubleClick Search column or a saved column.
* @return value or {@code null} for none
*/
public ReportApiColumnSpec getColumn() {
return column;
}
/**
* Column to perform the filter on. This can be a DoubleClick Search column or a saved column.
* @param column column or {@code null} for none
*/
public Filters setColumn(ReportApiColumnSpec column) {
this.column = column;
return this;
}
/**
* Operator to use in the filter. See the filter reference for a list of available operators.
* @return value or {@code null} for none
*/
public java.lang.String getOperator() {
return operator;
}
/**
* Operator to use in the filter. See the filter reference for a list of available operators.
* @param operator operator or {@code null} for none
*/
public Filters setOperator(java.lang.String operator) {
this.operator = operator;
return this;
}
/**
* A list of values to filter the column value against.
* @return value or {@code null} for none
*/
public java.util.List getValues() {
return values;
}
/**
* A list of values to filter the column value against.
* @param values values or {@code null} for none
*/
public Filters setValues(java.util.List values) {
this.values = values;
return this;
}
@Override
public Filters set(String fieldName, Object value) {
return (Filters) super.set(fieldName, value);
}
@Override
public Filters clone() {
return (Filters) super.clone();
}
}
/**
* Model definition for ReportRequestOrderBy.
*/
public static final class OrderBy extends com.google.api.client.json.GenericJson {
/**
* Column to perform the sort on. This can be a DoubleClick Search-defined column or a saved
* column.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ReportApiColumnSpec column;
/**
* The sort direction, which is either ascending or descending.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sortOrder;
/**
* Column to perform the sort on. This can be a DoubleClick Search-defined column or a saved
* column.
* @return value or {@code null} for none
*/
public ReportApiColumnSpec getColumn() {
return column;
}
/**
* Column to perform the sort on. This can be a DoubleClick Search-defined column or a saved
* column.
* @param column column or {@code null} for none
*/
public OrderBy setColumn(ReportApiColumnSpec column) {
this.column = column;
return this;
}
/**
* The sort direction, which is either ascending or descending.
* @return value or {@code null} for none
*/
public java.lang.String getSortOrder() {
return sortOrder;
}
/**
* The sort direction, which is either ascending or descending.
* @param sortOrder sortOrder or {@code null} for none
*/
public OrderBy setSortOrder(java.lang.String sortOrder) {
this.sortOrder = sortOrder;
return this;
}
@Override
public OrderBy set(String fieldName, Object value) {
return (OrderBy) super.set(fieldName, value);
}
@Override
public OrderBy clone() {
return (OrderBy) super.clone();
}
}
/**
* The reportScope is a set of IDs that are used to determine which subset of entities will be
* returned in the report. The full lineage of IDs from the lowest scoped level desired up through
* agency is required.
*/
public static final class ReportScope extends com.google.api.client.json.GenericJson {
/**
* DS ad group ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long adGroupId;
/**
* DS ad ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long adId;
/**
* DS advertiser ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long advertiserId;
/**
* DS agency ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long agencyId;
/**
* DS campaign ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long campaignId;
/**
* DS engine account ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long engineAccountId;
/**
* DS keyword ID.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long keywordId;
/**
* DS ad group ID.
* @return value or {@code null} for none
*/
public java.lang.Long getAdGroupId() {
return adGroupId;
}
/**
* DS ad group ID.
* @param adGroupId adGroupId or {@code null} for none
*/
public ReportScope setAdGroupId(java.lang.Long adGroupId) {
this.adGroupId = adGroupId;
return this;
}
/**
* DS ad ID.
* @return value or {@code null} for none
*/
public java.lang.Long getAdId() {
return adId;
}
/**
* DS ad ID.
* @param adId adId or {@code null} for none
*/
public ReportScope setAdId(java.lang.Long adId) {
this.adId = adId;
return this;
}
/**
* DS advertiser ID.
* @return value or {@code null} for none
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/**
* DS advertiser ID.
* @param advertiserId advertiserId or {@code null} for none
*/
public ReportScope setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
/**
* DS agency ID.
* @return value or {@code null} for none
*/
public java.lang.Long getAgencyId() {
return agencyId;
}
/**
* DS agency ID.
* @param agencyId agencyId or {@code null} for none
*/
public ReportScope setAgencyId(java.lang.Long agencyId) {
this.agencyId = agencyId;
return this;
}
/**
* DS campaign ID.
* @return value or {@code null} for none
*/
public java.lang.Long getCampaignId() {
return campaignId;
}
/**
* DS campaign ID.
* @param campaignId campaignId or {@code null} for none
*/
public ReportScope setCampaignId(java.lang.Long campaignId) {
this.campaignId = campaignId;
return this;
}
/**
* DS engine account ID.
* @return value or {@code null} for none
*/
public java.lang.Long getEngineAccountId() {
return engineAccountId;
}
/**
* DS engine account ID.
* @param engineAccountId engineAccountId or {@code null} for none
*/
public ReportScope setEngineAccountId(java.lang.Long engineAccountId) {
this.engineAccountId = engineAccountId;
return this;
}
/**
* DS keyword ID.
* @return value or {@code null} for none
*/
public java.lang.Long getKeywordId() {
return keywordId;
}
/**
* DS keyword ID.
* @param keywordId keywordId or {@code null} for none
*/
public ReportScope setKeywordId(java.lang.Long keywordId) {
this.keywordId = keywordId;
return this;
}
@Override
public ReportScope set(String fieldName, Object value) {
return (ReportScope) super.set(fieldName, value);
}
@Override
public ReportScope clone() {
return (ReportScope) super.clone();
}
}
/**
* If metrics are requested in a report, this argument will be used to restrict the metrics to a
* specific time range.
*/
public static final class TimeRange extends com.google.api.client.json.GenericJson {
/**
* Inclusive UTC timestamp in RFC format, e.g., 2013-07-16T10:16:23.555Z. See additional
* references on how changed attribute reports work.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime changedAttributesSinceTimestamp;
/**
* Inclusive UTC timestamp in RFC format, e.g., 2013-07-16T10:16:23.555Z. See additional
* references on how changed metrics reports work.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime changedMetricsSinceTimestamp;
/**
* Inclusive date in YYYY-MM-DD format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String endDate;
/**
* Inclusive date in YYYY-MM-DD format.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String startDate;
/**
* Inclusive UTC timestamp in RFC format, e.g., 2013-07-16T10:16:23.555Z. See additional
* references on how changed attribute reports work.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getChangedAttributesSinceTimestamp() {
return changedAttributesSinceTimestamp;
}
/**
* Inclusive UTC timestamp in RFC format, e.g., 2013-07-16T10:16:23.555Z. See additional
* references on how changed attribute reports work.
* @param changedAttributesSinceTimestamp changedAttributesSinceTimestamp or {@code null} for none
*/
public TimeRange setChangedAttributesSinceTimestamp(com.google.api.client.util.DateTime changedAttributesSinceTimestamp) {
this.changedAttributesSinceTimestamp = changedAttributesSinceTimestamp;
return this;
}
/**
* Inclusive UTC timestamp in RFC format, e.g., 2013-07-16T10:16:23.555Z. See additional
* references on how changed metrics reports work.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getChangedMetricsSinceTimestamp() {
return changedMetricsSinceTimestamp;
}
/**
* Inclusive UTC timestamp in RFC format, e.g., 2013-07-16T10:16:23.555Z. See additional
* references on how changed metrics reports work.
* @param changedMetricsSinceTimestamp changedMetricsSinceTimestamp or {@code null} for none
*/
public TimeRange setChangedMetricsSinceTimestamp(com.google.api.client.util.DateTime changedMetricsSinceTimestamp) {
this.changedMetricsSinceTimestamp = changedMetricsSinceTimestamp;
return this;
}
/**
* Inclusive date in YYYY-MM-DD format.
* @return value or {@code null} for none
*/
public java.lang.String getEndDate() {
return endDate;
}
/**
* Inclusive date in YYYY-MM-DD format.
* @param endDate endDate or {@code null} for none
*/
public TimeRange setEndDate(java.lang.String endDate) {
this.endDate = endDate;
return this;
}
/**
* Inclusive date in YYYY-MM-DD format.
* @return value or {@code null} for none
*/
public java.lang.String getStartDate() {
return startDate;
}
/**
* Inclusive date in YYYY-MM-DD format.
* @param startDate startDate or {@code null} for none
*/
public TimeRange setStartDate(java.lang.String startDate) {
this.startDate = startDate;
return this;
}
@Override
public TimeRange set(String fieldName, Object value) {
return (TimeRange) super.set(fieldName, value);
}
@Override
public TimeRange clone() {
return (TimeRange) super.clone();
}
}
}