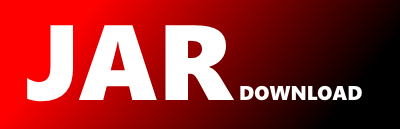
target.apidocs.com.google.api.services.drive.Drive.Files.html Maven / Gradle / Ivy
Drive.Files (Google Drive API v2-rev20240521-2.0.0)
com.google.api.services.drive
Class Drive.Files
- java.lang.Object
-
- com.google.api.services.drive.Drive.Files
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
class
Drive.Files.Copy
class
Drive.Files.Delete
class
Drive.Files.EmptyTrash
class
Drive.Files.Export
class
Drive.Files.GenerateIds
class
Drive.Files.Get
class
Drive.Files.Insert
class
Drive.Files.List
class
Drive.Files.ListLabels
class
Drive.Files.ModifyLabels
class
Drive.Files.Patch
class
Drive.Files.Touch
class
Drive.Files.Trash
class
Drive.Files.Untrash
class
Drive.Files.Update
class
Drive.Files.Watch
-
Constructor Summary
Constructors
Constructor and Description
Files()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Drive.Files.Copy
copy(String fileId,
File content)
Creates a copy of the specified file.
Drive.Files.Delete
delete(String fileId)
Permanently deletes a file owned by the user without moving it to the trash.
Drive.Files.EmptyTrash
emptyTrash()
Permanently deletes all of the user's trashed files.
Drive.Files.Export
export(String fileId,
String mimeType)
Exports a Google Workspace document to the requested MIME type and returns exported byte content.
Drive.Files.GenerateIds
generateIds()
Generates a set of file IDs which can be provided in insert or copy requests.
Drive.Files.Get
get(String fileId)
Gets a file's metadata or content by ID.
Drive.Files.Insert
insert(File content)
Inserts a new file.
Drive.Files.Insert
insert(File content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
Inserts a new file.
Drive.Files.List
list()
Lists the user's files.
Drive.Files.ListLabels
listLabels(String fileId)
Lists the labels on a file.
Drive.Files.ModifyLabels
modifyLabels(String fileId,
ModifyLabelsRequest content)
Modifies the set of labels applied to a file.
Drive.Files.Patch
patch(String fileId,
File content)
Updates a file's metadata and/or content.
Drive.Files.Touch
touch(String fileId)
Set the file's updated time to the current server time.
Drive.Files.Trash
trash(String fileId)
Moves a file to the trash.
Drive.Files.Untrash
untrash(String fileId)
Restores a file from the trash.
Drive.Files.Update
update(String fileId,
File content)
Updates a file's metadata and/or content.
Drive.Files.Update
update(String fileId,
File content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
Updates a file's metadata and/or content.
Drive.Files.Watch
watch(String fileId,
Channel content)
Subscribes to changes to a file.
-
-
Method Detail
-
copy
public Drive.Files.Copy copy(String fileId,
File content)
throws IOException
Creates a copy of the specified file.
Create a request for the method "files.copy".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID of the file to copy.
content
- the File
- Returns:
- the request
- Throws:
IOException
-
delete
public Drive.Files.Delete delete(String fileId)
throws IOException
Permanently deletes a file owned by the user without moving it to the trash. If the file belongs
to a shared drive, the user must be an `organizer` on the parent folder. If the target is a
folder, all descendants owned by the user are also deleted.
Create a request for the method "files.delete".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID of the file to delete.
- Returns:
- the request
- Throws:
IOException
-
emptyTrash
public Drive.Files.EmptyTrash emptyTrash()
throws IOException
Permanently deletes all of the user's trashed files.
Create a request for the method "files.emptyTrash".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Returns:
- the request
- Throws:
IOException
-
export
public Drive.Files.Export export(String fileId,
String mimeType)
throws IOException
Exports a Google Workspace document to the requested MIME type and returns exported byte content.
Note that the exported content is limited to 10MB.
Create a request for the method "files.export".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID of the file.
mimeType
- Required. The MIME type of the format requested for this export.
- Returns:
- the request
- Throws:
IOException
-
generateIds
public Drive.Files.GenerateIds generateIds()
throws IOException
Generates a set of file IDs which can be provided in insert or copy requests.
Create a request for the method "files.generateIds".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Returns:
- the request
- Throws:
IOException
-
get
public Drive.Files.Get get(String fileId)
throws IOException
Gets a file's metadata or content by ID. If you provide the URL parameter `alt=media`, then the
response includes the file contents in the response body. Downloading content with `alt=media`
only works if the file is stored in Drive. To download Google Docs, Sheets, and Slides use
[`files.export`](/drive/api/reference/rest/v2/files/export) instead. For more information, see
[Download & export files](/drive/api/guides/manage-downloads).
Create a request for the method "files.get".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID for the file in question.
- Returns:
- the request
- Throws:
IOException
-
insert
public Drive.Files.Insert insert(File content)
throws IOException
Inserts a new file. This method supports an upload* URI and accepts uploaded media with the
following characteristics: - *Maximum file size:* 5,120 GB - *Accepted Media MIME types:*`*`
Note: Specify a valid MIME type, rather than the literal `*` value. The literal `*` is only used
to indicate that any valid MIME type can be uploaded. For more information on uploading files,
see [Upload file data](/drive/api/guides/manage-uploads). Apps creating shortcuts with
`files.insert` must specify the MIME type `application/vnd.google-apps.shortcut`. Apps should
specify a file extension in the `title` property when inserting files with the API. For example,
an operation to insert a JPEG file should specify something like `"title": "cat.jpg"` in the
metadata. Subsequent `GET` requests include the read-only `fileExtension` property populated with
the extension originally specified in the `title` property. When a Google Drive user requests to
download a file, or when the file is downloaded through the sync client, Drive builds a full
filename (with extension) based on the title. In cases where the extension is missing, Drive
attempts to determine the extension based on the file's MIME type.
Create a request for the method "files.insert".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
content
- the File
- Returns:
- the request
- Throws:
IOException
-
insert
public Drive.Files.Insert insert(File content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
throws IOException
Inserts a new file. This method supports an upload* URI and accepts uploaded media with the
following characteristics: - *Maximum file size:* 5,120 GB - *Accepted Media MIME types:*`*`
Note: Specify a valid MIME type, rather than the literal `*` value. The literal `*` is only used
to indicate that any valid MIME type can be uploaded. For more information on uploading files,
see [Upload file data](/drive/api/guides/manage-uploads). Apps creating shortcuts with
`files.insert` must specify the MIME type `application/vnd.google-apps.shortcut`. Apps should
specify a file extension in the `title` property when inserting files with the API. For example,
an operation to insert a JPEG file should specify something like `"title": "cat.jpg"` in the
metadata. Subsequent `GET` requests include the read-only `fileExtension` property populated with
the extension originally specified in the `title` property. When a Google Drive user requests to
download a file, or when the file is downloaded through the sync client, Drive builds a full
filename (with extension) based on the title. In cases where the extension is missing, Drive
attempts to determine the extension based on the file's MIME type.
Create a request for the method "files.insert".
This request holds the parameters needed by the the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
This method should be used for uploading media content.
- Parameters:
content
- the File
media metadata or null
if none
mediaContent
- The media HTTP content.
- Returns:
- the request
- Throws:
IOException
- if the initialization of the request fails
-
list
public Drive.Files.List list()
throws IOException
Lists the user's files. This method accepts the `q` parameter, which is a search query combining
one or more search terms. For more information, see the [Search for files &
folders](/drive/api/guides/search-files) guide. *Note:* This method returns *all* files by
default, including trashed files. If you don't want trashed files to appear in the list, use the
`trashed=false` query parameter to remove trashed files from the results.
Create a request for the method "files.list".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Returns:
- the request
- Throws:
IOException
-
listLabels
public Drive.Files.ListLabels listLabels(String fileId)
throws IOException
Lists the labels on a file.
Create a request for the method "files.listLabels".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID for the file.
- Returns:
- the request
- Throws:
IOException
-
modifyLabels
public Drive.Files.ModifyLabels modifyLabels(String fileId,
ModifyLabelsRequest content)
throws IOException
Modifies the set of labels applied to a file. Returns a list of the labels that were added or
modified.
Create a request for the method "files.modifyLabels".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID of the file to which the labels belong.
content
- the ModifyLabelsRequest
- Returns:
- the request
- Throws:
IOException
-
patch
public Drive.Files.Patch patch(String fileId,
File content)
throws IOException
Updates a file's metadata and/or content. When calling this method, only populate fields in the
request that you want to modify. When updating fields, some fields might change automatically,
such as modifiedDate. This method supports patch semantics.
Create a request for the method "files.patch".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID of the file to update.
content
- the File
- Returns:
- the request
- Throws:
IOException
-
touch
public Drive.Files.Touch touch(String fileId)
throws IOException
Set the file's updated time to the current server time.
Create a request for the method "files.touch".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID of the file to update.
- Returns:
- the request
- Throws:
IOException
-
trash
public Drive.Files.Trash trash(String fileId)
throws IOException
Moves a file to the trash. The currently authenticated user must own the file or be at least a
`fileOrganizer` on the parent for shared drive files.
Create a request for the method "files.trash".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID of the file to trash.
- Returns:
- the request
- Throws:
IOException
-
untrash
public Drive.Files.Untrash untrash(String fileId)
throws IOException
Restores a file from the trash. The currently authenticated user must own the file or be at least
a `fileOrganizer` on the parent for shared drive files.
Create a request for the method "files.untrash".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID of the file to untrash.
- Returns:
- the request
- Throws:
IOException
-
update
public Drive.Files.Update update(String fileId,
File content)
throws IOException
Updates a file's metadata and/or content. When calling this method, only populate fields in the
request that you want to modify. When updating fields, some fields might be changed
automatically, such as `modifiedDate`. This method supports patch semantics. This method supports
an upload* URI and accepts uploaded media with the following characteristics: - *Maximum file
size:* 5,120 GB - *Accepted Media MIME types:*`*` Note: Specify a valid MIME type, rather than
the literal `*` value. The literal `*` is only used to indicate that any valid MIME type can be
uploaded. For more information on uploading files, see [Upload file
data](/drive/api/guides/manage-uploads).
Create a request for the method "files.update".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID of the file to update.
content
- the File
- Returns:
- the request
- Throws:
IOException
-
update
public Drive.Files.Update update(String fileId,
File content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
throws IOException
Updates a file's metadata and/or content. When calling this method, only populate fields in the
request that you want to modify. When updating fields, some fields might be changed
automatically, such as `modifiedDate`. This method supports patch semantics. This method supports
an upload* URI and accepts uploaded media with the following characteristics: - *Maximum file
size:* 5,120 GB - *Accepted Media MIME types:*`*` Note: Specify a valid MIME type, rather than
the literal `*` value. The literal `*` is only used to indicate that any valid MIME type can be
uploaded. For more information on uploading files, see [Upload file
data](/drive/api/guides/manage-uploads).
Create a request for the method "files.update".
This request holds the parameters needed by the the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
This method should be used for uploading media content.
- Parameters:
fileId
- The ID of the file to update.
content
- the File
media metadata or null
if none
mediaContent
- The media HTTP content.
- Returns:
- the request
- Throws:
IOException
- if the initialization of the request fails
-
watch
public Drive.Files.Watch watch(String fileId,
Channel content)
throws IOException
Subscribes to changes to a file.
Create a request for the method "files.watch".
This request holds the parameters needed by the drive server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
fileId
- The ID for the file in question.
content
- the Channel
- Returns:
- the request
- Throws:
IOException
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy