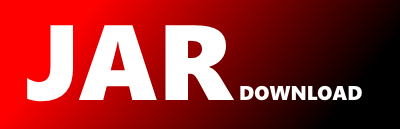
target.apidocs.com.google.api.services.drive.model.Permission.html Maven / Gradle / Ivy
Permission (Google Drive API v2-rev20240809-2.0.0)
com.google.api.services.drive.model
Class Permission
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.drive.model.Permission
-
public final class Permission
extends com.google.api.client.json.GenericJson
A permission for a file. A permission grants a user, group, domain, or the world access to a file
or a folder hierarchy. Some resource methods (such as `permissions.update`) require a
`permissionId`. Use the `permissions.list` method to retrieve the ID for a file, folder, or
shared drive.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Google Drive API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
static class
Permission.PermissionDetails
Model definition for PermissionPermissionDetails.
static class
Permission.TeamDrivePermissionDetails
Model definition for PermissionTeamDrivePermissionDetails.
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Permission()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Permission
clone()
List<String>
getAdditionalRoles()
Additional roles for this user.
String
getAuthKey()
Output only.
Boolean
getDeleted()
Output only.
String
getDomain()
Output only.
String
getEmailAddress()
Output only.
String
getEtag()
Output only.
com.google.api.client.util.DateTime
getExpirationDate()
The time at which this permission will expire (RFC 3339 date-time).
String
getId()
The ID of the user this permission refers to, and identical to the `permissionId` in the About
and Files resources.
String
getKind()
Output only.
String
getName()
Output only.
Boolean
getPendingOwner()
Whether the account associated with this permission is a pending owner.
List<Permission.PermissionDetails>
getPermissionDetails()
Output only.
String
getPhotoLink()
Output only.
String
getRole()
The primary role for this user.
String
getSelfLink()
Output only.
List<Permission.TeamDrivePermissionDetails>
getTeamDrivePermissionDetails()
Output only.
String
getType()
The account type.
String
getValue()
The email address or domain name for the entity.
String
getView()
Indicates the view for this permission.
Boolean
getWithLink()
Whether the link is required for this permission.
Permission
set(String fieldName,
Object value)
Permission
setAdditionalRoles(List<String> additionalRoles)
Additional roles for this user.
Permission
setAuthKey(String authKey)
Output only.
Permission
setDeleted(Boolean deleted)
Output only.
Permission
setDomain(String domain)
Output only.
Permission
setEmailAddress(String emailAddress)
Output only.
Permission
setEtag(String etag)
Output only.
Permission
setExpirationDate(com.google.api.client.util.DateTime expirationDate)
The time at which this permission will expire (RFC 3339 date-time).
Permission
setId(String id)
The ID of the user this permission refers to, and identical to the `permissionId` in the About
and Files resources.
Permission
setKind(String kind)
Output only.
Permission
setName(String name)
Output only.
Permission
setPendingOwner(Boolean pendingOwner)
Whether the account associated with this permission is a pending owner.
Permission
setPermissionDetails(List<Permission.PermissionDetails> permissionDetails)
Output only.
Permission
setPhotoLink(String photoLink)
Output only.
Permission
setRole(String role)
The primary role for this user.
Permission
setSelfLink(String selfLink)
Output only.
Permission
setTeamDrivePermissionDetails(List<Permission.TeamDrivePermissionDetails> teamDrivePermissionDetails)
Output only.
Permission
setType(String type)
The account type.
Permission
setValue(String value)
The email address or domain name for the entity.
Permission
setView(String view)
Indicates the view for this permission.
Permission
setWithLink(Boolean withLink)
Whether the link is required for this permission.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAdditionalRoles
public List<String> getAdditionalRoles()
Additional roles for this user. Only `commenter` is currently allowed, though more may be
supported in the future.
- Returns:
- value or
null
for none
-
setAdditionalRoles
public Permission setAdditionalRoles(List<String> additionalRoles)
Additional roles for this user. Only `commenter` is currently allowed, though more may be
supported in the future.
- Parameters:
additionalRoles
- additionalRoles or null
for none
-
getAuthKey
public String getAuthKey()
Output only. Deprecated.
- Returns:
- value or
null
for none
-
setAuthKey
public Permission setAuthKey(String authKey)
Output only. Deprecated.
- Parameters:
authKey
- authKey or null
for none
-
getDeleted
public Boolean getDeleted()
Output only. Whether the account associated with this permission has been deleted. This field
only pertains to user and group permissions.
- Returns:
- value or
null
for none
-
setDeleted
public Permission setDeleted(Boolean deleted)
Output only. Whether the account associated with this permission has been deleted. This field
only pertains to user and group permissions.
- Parameters:
deleted
- deleted or null
for none
-
getDomain
public String getDomain()
Output only. The domain name of the entity this permission refers to. This is an output-only
field which is present when the permission type is `user`, `group` or `domain`.
- Returns:
- value or
null
for none
-
setDomain
public Permission setDomain(String domain)
Output only. The domain name of the entity this permission refers to. This is an output-only
field which is present when the permission type is `user`, `group` or `domain`.
- Parameters:
domain
- domain or null
for none
-
getEmailAddress
public String getEmailAddress()
Output only. The email address of the user or group this permission refers to. This is an
output-only field which is present when the permission type is `user` or `group`.
- Returns:
- value or
null
for none
-
setEmailAddress
public Permission setEmailAddress(String emailAddress)
Output only. The email address of the user or group this permission refers to. This is an
output-only field which is present when the permission type is `user` or `group`.
- Parameters:
emailAddress
- emailAddress or null
for none
-
getEtag
public String getEtag()
Output only. The ETag of the permission.
- Returns:
- value or
null
for none
-
setEtag
public Permission setEtag(String etag)
Output only. The ETag of the permission.
- Parameters:
etag
- etag or null
for none
-
getExpirationDate
public com.google.api.client.util.DateTime getExpirationDate()
The time at which this permission will expire (RFC 3339 date-time). Expiration dates have the
following restrictions: - They can only be set on user and group permissions - The date must be
in the future - The date cannot be more than a year in the future - The date can only be set on
drive.permissions.update or drive.permissions.patch requests
- Returns:
- value or
null
for none
-
setExpirationDate
public Permission setExpirationDate(com.google.api.client.util.DateTime expirationDate)
The time at which this permission will expire (RFC 3339 date-time). Expiration dates have the
following restrictions: - They can only be set on user and group permissions - The date must be
in the future - The date cannot be more than a year in the future - The date can only be set on
drive.permissions.update or drive.permissions.patch requests
- Parameters:
expirationDate
- expirationDate or null
for none
-
getId
public String getId()
The ID of the user this permission refers to, and identical to the `permissionId` in the About
and Files resources. When making a `drive.permissions.insert` request, exactly one of the `id`
or `value` fields must be specified unless the permission type is `anyone`, in which case both
`id` and `value` are ignored.
- Returns:
- value or
null
for none
-
setId
public Permission setId(String id)
The ID of the user this permission refers to, and identical to the `permissionId` in the About
and Files resources. When making a `drive.permissions.insert` request, exactly one of the `id`
or `value` fields must be specified unless the permission type is `anyone`, in which case both
`id` and `value` are ignored.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
Output only. This is always `drive#permission`.
- Returns:
- value or
null
for none
-
setKind
public Permission setKind(String kind)
Output only. This is always `drive#permission`.
- Parameters:
kind
- kind or null
for none
-
getName
public String getName()
Output only. The name for this permission.
- Returns:
- value or
null
for none
-
setName
public Permission setName(String name)
Output only. The name for this permission.
- Parameters:
name
- name or null
for none
-
getPendingOwner
public Boolean getPendingOwner()
Whether the account associated with this permission is a pending owner. Only populated for
`user` type permissions for files that are not in a shared drive.
- Returns:
- value or
null
for none
-
setPendingOwner
public Permission setPendingOwner(Boolean pendingOwner)
Whether the account associated with this permission is a pending owner. Only populated for
`user` type permissions for files that are not in a shared drive.
- Parameters:
pendingOwner
- pendingOwner or null
for none
-
getPermissionDetails
public List<Permission.PermissionDetails> getPermissionDetails()
Output only. Details of whether the permissions on this shared drive item are inherited or
directly on this item. This is an output-only field which is present only for shared drive
items.
- Returns:
- value or
null
for none
-
setPermissionDetails
public Permission setPermissionDetails(List<Permission.PermissionDetails> permissionDetails)
Output only. Details of whether the permissions on this shared drive item are inherited or
directly on this item. This is an output-only field which is present only for shared drive
items.
- Parameters:
permissionDetails
- permissionDetails or null
for none
-
getPhotoLink
public String getPhotoLink()
Output only. A link to the profile photo, if available.
- Returns:
- value or
null
for none
-
setPhotoLink
public Permission setPhotoLink(String photoLink)
Output only. A link to the profile photo, if available.
- Parameters:
photoLink
- photoLink or null
for none
-
getRole
public String getRole()
The primary role for this user. While new values may be supported in the future, the following
are currently allowed: * `owner` * `organizer` * `fileOrganizer` * `writer` * `reader`
- Returns:
- value or
null
for none
-
setRole
public Permission setRole(String role)
The primary role for this user. While new values may be supported in the future, the following
are currently allowed: * `owner` * `organizer` * `fileOrganizer` * `writer` * `reader`
- Parameters:
role
- role or null
for none
-
getSelfLink
public String getSelfLink()
Output only. A link back to this permission.
- Returns:
- value or
null
for none
-
setSelfLink
public Permission setSelfLink(String selfLink)
Output only. A link back to this permission.
- Parameters:
selfLink
- selfLink or null
for none
-
getTeamDrivePermissionDetails
public List<Permission.TeamDrivePermissionDetails> getTeamDrivePermissionDetails()
Output only. Deprecated: Use `permissionDetails` instead.
- Returns:
- value or
null
for none
-
setTeamDrivePermissionDetails
public Permission setTeamDrivePermissionDetails(List<Permission.TeamDrivePermissionDetails> teamDrivePermissionDetails)
Output only. Deprecated: Use `permissionDetails` instead.
- Parameters:
teamDrivePermissionDetails
- teamDrivePermissionDetails or null
for none
-
getType
public String getType()
The account type. Allowed values are: * `user` * `group` * `domain` * `anyone`
- Returns:
- value or
null
for none
-
setType
public Permission setType(String type)
The account type. Allowed values are: * `user` * `group` * `domain` * `anyone`
- Parameters:
type
- type or null
for none
-
getValue
public String getValue()
The email address or domain name for the entity. This is used during inserts and is not
populated in responses. When making a `drive.permissions.insert` request, exactly one of the
`id` or `value` fields must be specified unless the permission type is `anyone`, in which case
both `id` and `value` are ignored.
- Returns:
- value or
null
for none
-
setValue
public Permission setValue(String value)
The email address or domain name for the entity. This is used during inserts and is not
populated in responses. When making a `drive.permissions.insert` request, exactly one of the
`id` or `value` fields must be specified unless the permission type is `anyone`, in which case
both `id` and `value` are ignored.
- Parameters:
value
- value or null
for none
-
getView
public String getView()
Indicates the view for this permission. Only populated for permissions that belong to a view.
`published` is the only supported value.
- Returns:
- value or
null
for none
-
setView
public Permission setView(String view)
Indicates the view for this permission. Only populated for permissions that belong to a view.
`published` is the only supported value.
- Parameters:
view
- view or null
for none
-
getWithLink
public Boolean getWithLink()
Whether the link is required for this permission.
- Returns:
- value or
null
for none
-
setWithLink
public Permission setWithLink(Boolean withLink)
Whether the link is required for this permission.
- Parameters:
withLink
- withLink or null
for none
-
set
public Permission set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Permission clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy