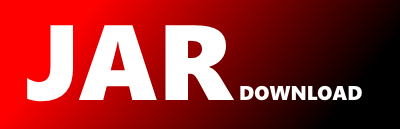
target.apidocs.com.google.api.services.drive.model.TeamDrive.html Maven / Gradle / Ivy
TeamDrive (Google Drive API v3-rev20240123-2.0.0)
com.google.api.services.drive.model
Class TeamDrive
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.drive.model.TeamDrive
-
public final class TeamDrive
extends com.google.api.client.json.GenericJson
Deprecated: use the drive collection instead.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Google Drive API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
static class
TeamDrive.BackgroundImageFile
An image file and cropping parameters from which a background image for this Team Drive is set.
static class
TeamDrive.Capabilities
Capabilities the current user has on this Team Drive.
static class
TeamDrive.Restrictions
A set of restrictions that apply to this Team Drive or items inside this Team Drive.
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
TeamDrive()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
TeamDrive
clone()
TeamDrive.BackgroundImageFile
getBackgroundImageFile()
An image file and cropping parameters from which a background image for this Team Drive is set.
String
getBackgroundImageLink()
A short-lived link to this Team Drive's background image.
TeamDrive.Capabilities
getCapabilities()
Capabilities the current user has on this Team Drive.
String
getColorRgb()
The color of this Team Drive as an RGB hex string.
com.google.api.client.util.DateTime
getCreatedTime()
The time at which the Team Drive was created (RFC 3339 date-time).
String
getId()
The ID of this Team Drive which is also the ID of the top level folder of this Team Drive.
String
getKind()
Identifies what kind of resource this is.
String
getName()
The name of this Team Drive.
String
getOrgUnitId()
The organizational unit of this shared drive.
TeamDrive.Restrictions
getRestrictions()
A set of restrictions that apply to this Team Drive or items inside this Team Drive.
String
getThemeId()
The ID of the theme from which the background image and color will be set.
TeamDrive
set(String fieldName,
Object value)
TeamDrive
setBackgroundImageFile(TeamDrive.BackgroundImageFile backgroundImageFile)
An image file and cropping parameters from which a background image for this Team Drive is set.
TeamDrive
setBackgroundImageLink(String backgroundImageLink)
A short-lived link to this Team Drive's background image.
TeamDrive
setCapabilities(TeamDrive.Capabilities capabilities)
Capabilities the current user has on this Team Drive.
TeamDrive
setColorRgb(String colorRgb)
The color of this Team Drive as an RGB hex string.
TeamDrive
setCreatedTime(com.google.api.client.util.DateTime createdTime)
The time at which the Team Drive was created (RFC 3339 date-time).
TeamDrive
setId(String id)
The ID of this Team Drive which is also the ID of the top level folder of this Team Drive.
TeamDrive
setKind(String kind)
Identifies what kind of resource this is.
TeamDrive
setName(String name)
The name of this Team Drive.
TeamDrive
setOrgUnitId(String orgUnitId)
The organizational unit of this shared drive.
TeamDrive
setRestrictions(TeamDrive.Restrictions restrictions)
A set of restrictions that apply to this Team Drive or items inside this Team Drive.
TeamDrive
setThemeId(String themeId)
The ID of the theme from which the background image and color will be set.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getBackgroundImageFile
public TeamDrive.BackgroundImageFile getBackgroundImageFile()
An image file and cropping parameters from which a background image for this Team Drive is set.
This is a write only field; it can only be set on `drive.teamdrives.update` requests that don't
set `themeId`. When specified, all fields of the `backgroundImageFile` must be set.
- Returns:
- value or
null
for none
-
setBackgroundImageFile
public TeamDrive setBackgroundImageFile(TeamDrive.BackgroundImageFile backgroundImageFile)
An image file and cropping parameters from which a background image for this Team Drive is set.
This is a write only field; it can only be set on `drive.teamdrives.update` requests that don't
set `themeId`. When specified, all fields of the `backgroundImageFile` must be set.
- Parameters:
backgroundImageFile
- backgroundImageFile or null
for none
-
getBackgroundImageLink
public String getBackgroundImageLink()
A short-lived link to this Team Drive's background image.
- Returns:
- value or
null
for none
-
setBackgroundImageLink
public TeamDrive setBackgroundImageLink(String backgroundImageLink)
A short-lived link to this Team Drive's background image.
- Parameters:
backgroundImageLink
- backgroundImageLink or null
for none
-
getCapabilities
public TeamDrive.Capabilities getCapabilities()
Capabilities the current user has on this Team Drive.
- Returns:
- value or
null
for none
-
setCapabilities
public TeamDrive setCapabilities(TeamDrive.Capabilities capabilities)
Capabilities the current user has on this Team Drive.
- Parameters:
capabilities
- capabilities or null
for none
-
getColorRgb
public String getColorRgb()
The color of this Team Drive as an RGB hex string. It can only be set on a
`drive.teamdrives.update` request that does not set `themeId`.
- Returns:
- value or
null
for none
-
setColorRgb
public TeamDrive setColorRgb(String colorRgb)
The color of this Team Drive as an RGB hex string. It can only be set on a
`drive.teamdrives.update` request that does not set `themeId`.
- Parameters:
colorRgb
- colorRgb or null
for none
-
getCreatedTime
public com.google.api.client.util.DateTime getCreatedTime()
The time at which the Team Drive was created (RFC 3339 date-time).
- Returns:
- value or
null
for none
-
setCreatedTime
public TeamDrive setCreatedTime(com.google.api.client.util.DateTime createdTime)
The time at which the Team Drive was created (RFC 3339 date-time).
- Parameters:
createdTime
- createdTime or null
for none
-
getId
public String getId()
The ID of this Team Drive which is also the ID of the top level folder of this Team Drive.
- Returns:
- value or
null
for none
-
setId
public TeamDrive setId(String id)
The ID of this Team Drive which is also the ID of the top level folder of this Team Drive.
- Parameters:
id
- id or null
for none
-
getKind
public String getKind()
Identifies what kind of resource this is. Value: the fixed string `"drive#teamDrive"`.
- Returns:
- value or
null
for none
-
setKind
public TeamDrive setKind(String kind)
Identifies what kind of resource this is. Value: the fixed string `"drive#teamDrive"`.
- Parameters:
kind
- kind or null
for none
-
getName
public String getName()
The name of this Team Drive.
- Returns:
- value or
null
for none
-
setName
public TeamDrive setName(String name)
The name of this Team Drive.
- Parameters:
name
- name or null
for none
-
getOrgUnitId
public String getOrgUnitId()
The organizational unit of this shared drive. This field is only populated on `drives.list`
responses when the `useDomainAdminAccess` parameter is set to `true`.
- Returns:
- value or
null
for none
-
setOrgUnitId
public TeamDrive setOrgUnitId(String orgUnitId)
The organizational unit of this shared drive. This field is only populated on `drives.list`
responses when the `useDomainAdminAccess` parameter is set to `true`.
- Parameters:
orgUnitId
- orgUnitId or null
for none
-
getRestrictions
public TeamDrive.Restrictions getRestrictions()
A set of restrictions that apply to this Team Drive or items inside this Team Drive.
- Returns:
- value or
null
for none
-
setRestrictions
public TeamDrive setRestrictions(TeamDrive.Restrictions restrictions)
A set of restrictions that apply to this Team Drive or items inside this Team Drive.
- Parameters:
restrictions
- restrictions or null
for none
-
getThemeId
public String getThemeId()
The ID of the theme from which the background image and color will be set. The set of possible
`teamDriveThemes` can be retrieved from a `drive.about.get` response. When not specified on a
`drive.teamdrives.create` request, a random theme is chosen from which the background image and
color are set. This is a write-only field; it can only be set on requests that don't set
`colorRgb` or `backgroundImageFile`.
- Returns:
- value or
null
for none
-
setThemeId
public TeamDrive setThemeId(String themeId)
The ID of the theme from which the background image and color will be set. The set of possible
`teamDriveThemes` can be retrieved from a `drive.about.get` response. When not specified on a
`drive.teamdrives.create` request, a random theme is chosen from which the background image and
color are set. This is a write-only field; it can only be set on requests that don't set
`colorRgb` or `backgroundImageFile`.
- Parameters:
themeId
- themeId or null
for none
-
set
public TeamDrive set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public TeamDrive clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy