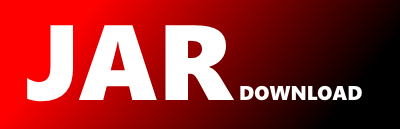
com.google.api.services.drive.model.Change Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.drive.model;
/**
* A change to a file or shared drive.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Drive API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Change extends com.google.api.client.json.GenericJson {
/**
* The type of the change. Possible values are `file` and `drive`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String changeType;
/**
* The updated state of the shared drive. Present if the changeType is drive, the user is still a
* member of the shared drive, and the shared drive has not been deleted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Drive drive;
/**
* The ID of the shared drive associated with this change.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String driveId;
/**
* The updated state of the file. Present if the type is file and the file has not been removed
* from this list of changes.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private File file;
/**
* The ID of the file which has changed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fileId;
/**
* Identifies what kind of resource this is. Value: the fixed string `"drive#change"`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Whether the file or shared drive has been removed from this list of changes, for example by
* deletion or loss of access.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean removed;
/**
* Deprecated: Use `drive` instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TeamDrive teamDrive;
/**
* Deprecated: Use `driveId` instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String teamDriveId;
/**
* The time of this change (RFC 3339 date-time).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime time;
/**
* Deprecated: Use `changeType` instead.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The type of the change. Possible values are `file` and `drive`.
* @return value or {@code null} for none
*/
public java.lang.String getChangeType() {
return changeType;
}
/**
* The type of the change. Possible values are `file` and `drive`.
* @param changeType changeType or {@code null} for none
*/
public Change setChangeType(java.lang.String changeType) {
this.changeType = changeType;
return this;
}
/**
* The updated state of the shared drive. Present if the changeType is drive, the user is still a
* member of the shared drive, and the shared drive has not been deleted.
* @return value or {@code null} for none
*/
public Drive getDrive() {
return drive;
}
/**
* The updated state of the shared drive. Present if the changeType is drive, the user is still a
* member of the shared drive, and the shared drive has not been deleted.
* @param drive drive or {@code null} for none
*/
public Change setDrive(Drive drive) {
this.drive = drive;
return this;
}
/**
* The ID of the shared drive associated with this change.
* @return value or {@code null} for none
*/
public java.lang.String getDriveId() {
return driveId;
}
/**
* The ID of the shared drive associated with this change.
* @param driveId driveId or {@code null} for none
*/
public Change setDriveId(java.lang.String driveId) {
this.driveId = driveId;
return this;
}
/**
* The updated state of the file. Present if the type is file and the file has not been removed
* from this list of changes.
* @return value or {@code null} for none
*/
public File getFile() {
return file;
}
/**
* The updated state of the file. Present if the type is file and the file has not been removed
* from this list of changes.
* @param file file or {@code null} for none
*/
public Change setFile(File file) {
this.file = file;
return this;
}
/**
* The ID of the file which has changed.
* @return value or {@code null} for none
*/
public java.lang.String getFileId() {
return fileId;
}
/**
* The ID of the file which has changed.
* @param fileId fileId or {@code null} for none
*/
public Change setFileId(java.lang.String fileId) {
this.fileId = fileId;
return this;
}
/**
* Identifies what kind of resource this is. Value: the fixed string `"drive#change"`.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Identifies what kind of resource this is. Value: the fixed string `"drive#change"`.
* @param kind kind or {@code null} for none
*/
public Change setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Whether the file or shared drive has been removed from this list of changes, for example by
* deletion or loss of access.
* @return value or {@code null} for none
*/
public java.lang.Boolean getRemoved() {
return removed;
}
/**
* Whether the file or shared drive has been removed from this list of changes, for example by
* deletion or loss of access.
* @param removed removed or {@code null} for none
*/
public Change setRemoved(java.lang.Boolean removed) {
this.removed = removed;
return this;
}
/**
* Deprecated: Use `drive` instead.
* @return value or {@code null} for none
*/
public TeamDrive getTeamDrive() {
return teamDrive;
}
/**
* Deprecated: Use `drive` instead.
* @param teamDrive teamDrive or {@code null} for none
*/
public Change setTeamDrive(TeamDrive teamDrive) {
this.teamDrive = teamDrive;
return this;
}
/**
* Deprecated: Use `driveId` instead.
* @return value or {@code null} for none
*/
public java.lang.String getTeamDriveId() {
return teamDriveId;
}
/**
* Deprecated: Use `driveId` instead.
* @param teamDriveId teamDriveId or {@code null} for none
*/
public Change setTeamDriveId(java.lang.String teamDriveId) {
this.teamDriveId = teamDriveId;
return this;
}
/**
* The time of this change (RFC 3339 date-time).
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getTime() {
return time;
}
/**
* The time of this change (RFC 3339 date-time).
* @param time time or {@code null} for none
*/
public Change setTime(com.google.api.client.util.DateTime time) {
this.time = time;
return this;
}
/**
* Deprecated: Use `changeType` instead.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Deprecated: Use `changeType` instead.
* @param type type or {@code null} for none
*/
public Change setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public Change set(String fieldName, Object value) {
return (Change) super.set(fieldName, value);
}
@Override
public Change clone() {
return (Change) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy