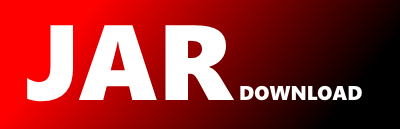
target.apidocs.com.google.api.services.drive.model.File.html Maven / Gradle / Ivy
File (Google Drive API v3-rev20241027-2.0.0)
com.google.api.services.drive.model
Class File
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.drive.model.File
-
public final class File
extends com.google.api.client.json.GenericJson
The metadata for a file. Some resource methods (such as `files.update`) require a `fileId`. Use
the `files.list` method to retrieve the ID for a file.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Google Drive API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
static class
File.Capabilities
Output only.
static class
File.ContentHints
Additional information about the content of the file.
static class
File.ImageMediaMetadata
Output only.
static class
File.LabelInfo
Output only.
static class
File.LinkShareMetadata
Contains details about the link URLs that clients are using to refer to this item.
static class
File.ShortcutDetails
Shortcut file details.
static class
File.VideoMediaMetadata
Output only.
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
File()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
File
clone()
Map<String,String>
getAppProperties()
A collection of arbitrary key-value pairs which are private to the requesting app.
File.Capabilities
getCapabilities()
Output only.
File.ContentHints
getContentHints()
Additional information about the content of the file.
List<ContentRestriction>
getContentRestrictions()
Restrictions for accessing the content of the file.
Boolean
getCopyRequiresWriterPermission()
Whether the options to copy, print, or download this file, should be disabled for readers and
commenters.
com.google.api.client.util.DateTime
getCreatedTime()
The time at which the file was created (RFC 3339 date-time).
String
getDescription()
A short description of the file.
String
getDriveId()
Output only.
Boolean
getExplicitlyTrashed()
Output only.
Map<String,String>
getExportLinks()
Output only.
String
getFileExtension()
Output only.
String
getFolderColorRgb()
The color for a folder or a shortcut to a folder as an RGB hex string.
String
getFullFileExtension()
Output only.
Boolean
getHasAugmentedPermissions()
Output only.
Boolean
getHasThumbnail()
Output only.
String
getHeadRevisionId()
Output only.
String
getIconLink()
Output only.
String
getId()
The ID of the file.
File.ImageMediaMetadata
getImageMediaMetadata()
Output only.
Boolean
getIsAppAuthorized()
Output only.
String
getKind()
Output only.
File.LabelInfo
getLabelInfo()
Output only.
User
getLastModifyingUser()
Output only.
File.LinkShareMetadata
getLinkShareMetadata()
Contains details about the link URLs that clients are using to refer to this item.
String
getMd5Checksum()
Output only.
String
getMimeType()
The MIME type of the file.
Boolean
getModifiedByMe()
Output only.
com.google.api.client.util.DateTime
getModifiedByMeTime()
The last time the file was modified by the user (RFC 3339 date-time).
com.google.api.client.util.DateTime
getModifiedTime()
he last time the file was modified by anyone (RFC 3339 date-time).
String
getName()
The name of the file.
String
getOriginalFilename()
The original filename of the uploaded content if available, or else the original value of the
`name` field.
Boolean
getOwnedByMe()
Output only.
List<User>
getOwners()
Output only.
List<String>
getParents()
The ID of the parent folder containing the file.
List<String>
getPermissionIds()
Output only.
List<Permission>
getPermissions()
Output only.
Map<String,String>
getProperties()
A collection of arbitrary key-value pairs which are visible to all apps.
Long
getQuotaBytesUsed()
Output only.
String
getResourceKey()
Output only.
String
getSha1Checksum()
Output only.
String
getSha256Checksum()
Output only.
Boolean
getShared()
Output only.
com.google.api.client.util.DateTime
getSharedWithMeTime()
The time at which the file was shared with the user, if applicable (RFC 3339 date-time).
User
getSharingUser()
Output only.
File.ShortcutDetails
getShortcutDetails()
Shortcut file details.
Long
getSize()
Output only.
List<String>
getSpaces()
Output only.
Boolean
getStarred()
Whether the user has starred the file.
String
getTeamDriveId()
Deprecated: Output only.
String
getThumbnailLink()
Output only.
Long
getThumbnailVersion()
Output only.
Boolean
getTrashed()
Whether the file has been trashed, either explicitly or from a trashed parent folder.
com.google.api.client.util.DateTime
getTrashedTime()
The time that the item was trashed (RFC 3339 date-time).
User
getTrashingUser()
Output only.
Long
getVersion()
Output only.
File.VideoMediaMetadata
getVideoMediaMetadata()
Output only.
Boolean
getViewedByMe()
Output only.
com.google.api.client.util.DateTime
getViewedByMeTime()
The last time the file was viewed by the user (RFC 3339 date-time).
Boolean
getViewersCanCopyContent()
Deprecated: Use `copyRequiresWriterPermission` instead.
String
getWebContentLink()
Output only.
String
getWebViewLink()
Output only.
Boolean
getWritersCanShare()
Whether users with only `writer` permission can modify the file's permissions.
File
set(String fieldName,
Object value)
File
setAppProperties(Map<String,String> appProperties)
A collection of arbitrary key-value pairs which are private to the requesting app.
File
setCapabilities(File.Capabilities capabilities)
Output only.
File
setContentHints(File.ContentHints contentHints)
Additional information about the content of the file.
File
setContentRestrictions(List<ContentRestriction> contentRestrictions)
Restrictions for accessing the content of the file.
File
setCopyRequiresWriterPermission(Boolean copyRequiresWriterPermission)
Whether the options to copy, print, or download this file, should be disabled for readers and
commenters.
File
setCreatedTime(com.google.api.client.util.DateTime createdTime)
The time at which the file was created (RFC 3339 date-time).
File
setDescription(String description)
A short description of the file.
File
setDriveId(String driveId)
Output only.
File
setExplicitlyTrashed(Boolean explicitlyTrashed)
Output only.
File
setExportLinks(Map<String,String> exportLinks)
Output only.
File
setFileExtension(String fileExtension)
Output only.
File
setFolderColorRgb(String folderColorRgb)
The color for a folder or a shortcut to a folder as an RGB hex string.
File
setFullFileExtension(String fullFileExtension)
Output only.
File
setHasAugmentedPermissions(Boolean hasAugmentedPermissions)
Output only.
File
setHasThumbnail(Boolean hasThumbnail)
Output only.
File
setHeadRevisionId(String headRevisionId)
Output only.
File
setIconLink(String iconLink)
Output only.
File
setId(String id)
The ID of the file.
File
setImageMediaMetadata(File.ImageMediaMetadata imageMediaMetadata)
Output only.
File
setIsAppAuthorized(Boolean isAppAuthorized)
Output only.
File
setKind(String kind)
Output only.
File
setLabelInfo(File.LabelInfo labelInfo)
Output only.
File
setLastModifyingUser(User lastModifyingUser)
Output only.
File
setLinkShareMetadata(File.LinkShareMetadata linkShareMetadata)
Contains details about the link URLs that clients are using to refer to this item.
File
setMd5Checksum(String md5Checksum)
Output only.
File
setMimeType(String mimeType)
The MIME type of the file.
File
setModifiedByMe(Boolean modifiedByMe)
Output only.
File
setModifiedByMeTime(com.google.api.client.util.DateTime modifiedByMeTime)
The last time the file was modified by the user (RFC 3339 date-time).
File
setModifiedTime(com.google.api.client.util.DateTime modifiedTime)
he last time the file was modified by anyone (RFC 3339 date-time).
File
setName(String name)
The name of the file.
File
setOriginalFilename(String originalFilename)
The original filename of the uploaded content if available, or else the original value of the
`name` field.
File
setOwnedByMe(Boolean ownedByMe)
Output only.
File
setOwners(List<User> owners)
Output only.
File
setParents(List<String> parents)
The ID of the parent folder containing the file.
File
setPermissionIds(List<String> permissionIds)
Output only.
File
setPermissions(List<Permission> permissions)
Output only.
File
setProperties(Map<String,String> properties)
A collection of arbitrary key-value pairs which are visible to all apps.
File
setQuotaBytesUsed(Long quotaBytesUsed)
Output only.
File
setResourceKey(String resourceKey)
Output only.
File
setSha1Checksum(String sha1Checksum)
Output only.
File
setSha256Checksum(String sha256Checksum)
Output only.
File
setShared(Boolean shared)
Output only.
File
setSharedWithMeTime(com.google.api.client.util.DateTime sharedWithMeTime)
The time at which the file was shared with the user, if applicable (RFC 3339 date-time).
File
setSharingUser(User sharingUser)
Output only.
File
setShortcutDetails(File.ShortcutDetails shortcutDetails)
Shortcut file details.
File
setSize(Long size)
Output only.
File
setSpaces(List<String> spaces)
Output only.
File
setStarred(Boolean starred)
Whether the user has starred the file.
File
setTeamDriveId(String teamDriveId)
Deprecated: Output only.
File
setThumbnailLink(String thumbnailLink)
Output only.
File
setThumbnailVersion(Long thumbnailVersion)
Output only.
File
setTrashed(Boolean trashed)
Whether the file has been trashed, either explicitly or from a trashed parent folder.
File
setTrashedTime(com.google.api.client.util.DateTime trashedTime)
The time that the item was trashed (RFC 3339 date-time).
File
setTrashingUser(User trashingUser)
Output only.
File
setVersion(Long version)
Output only.
File
setVideoMediaMetadata(File.VideoMediaMetadata videoMediaMetadata)
Output only.
File
setViewedByMe(Boolean viewedByMe)
Output only.
File
setViewedByMeTime(com.google.api.client.util.DateTime viewedByMeTime)
The last time the file was viewed by the user (RFC 3339 date-time).
File
setViewersCanCopyContent(Boolean viewersCanCopyContent)
Deprecated: Use `copyRequiresWriterPermission` instead.
File
setWebContentLink(String webContentLink)
Output only.
File
setWebViewLink(String webViewLink)
Output only.
File
setWritersCanShare(Boolean writersCanShare)
Whether users with only `writer` permission can modify the file's permissions.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAppProperties
public Map<String,String> getAppProperties()
A collection of arbitrary key-value pairs which are private to the requesting app. Entries with
null values are cleared in update and copy requests. These properties can only be retrieved
using an authenticated request. An authenticated request uses an access token obtained with a
OAuth 2 client ID. You cannot use an API key to retrieve private properties.
- Returns:
- value or
null
for none
-
setAppProperties
public File setAppProperties(Map<String,String> appProperties)
A collection of arbitrary key-value pairs which are private to the requesting app. Entries with
null values are cleared in update and copy requests. These properties can only be retrieved
using an authenticated request. An authenticated request uses an access token obtained with a
OAuth 2 client ID. You cannot use an API key to retrieve private properties.
- Parameters:
appProperties
- appProperties or null
for none
-
getCapabilities
public File.Capabilities getCapabilities()
Output only. Capabilities the current user has on this file. Each capability corresponds to a
fine-grained action that a user may take.
- Returns:
- value or
null
for none
-
setCapabilities
public File setCapabilities(File.Capabilities capabilities)
Output only. Capabilities the current user has on this file. Each capability corresponds to a
fine-grained action that a user may take.
- Parameters:
capabilities
- capabilities or null
for none
-
getContentHints
public File.ContentHints getContentHints()
Additional information about the content of the file. These fields are never populated in
responses.
- Returns:
- value or
null
for none
-
setContentHints
public File setContentHints(File.ContentHints contentHints)
Additional information about the content of the file. These fields are never populated in
responses.
- Parameters:
contentHints
- contentHints or null
for none
-
getContentRestrictions
public List<ContentRestriction> getContentRestrictions()
Restrictions for accessing the content of the file. Only populated if such a restriction
exists.
- Returns:
- value or
null
for none
-
setContentRestrictions
public File setContentRestrictions(List<ContentRestriction> contentRestrictions)
Restrictions for accessing the content of the file. Only populated if such a restriction
exists.
- Parameters:
contentRestrictions
- contentRestrictions or null
for none
-
getCopyRequiresWriterPermission
public Boolean getCopyRequiresWriterPermission()
Whether the options to copy, print, or download this file, should be disabled for readers and
commenters.
- Returns:
- value or
null
for none
-
setCopyRequiresWriterPermission
public File setCopyRequiresWriterPermission(Boolean copyRequiresWriterPermission)
Whether the options to copy, print, or download this file, should be disabled for readers and
commenters.
- Parameters:
copyRequiresWriterPermission
- copyRequiresWriterPermission or null
for none
-
getCreatedTime
public com.google.api.client.util.DateTime getCreatedTime()
The time at which the file was created (RFC 3339 date-time).
- Returns:
- value or
null
for none
-
setCreatedTime
public File setCreatedTime(com.google.api.client.util.DateTime createdTime)
The time at which the file was created (RFC 3339 date-time).
- Parameters:
createdTime
- createdTime or null
for none
-
getDescription
public String getDescription()
A short description of the file.
- Returns:
- value or
null
for none
-
setDescription
public File setDescription(String description)
A short description of the file.
- Parameters:
description
- description or null
for none
-
getDriveId
public String getDriveId()
Output only. ID of the shared drive the file resides in. Only populated for items in shared
drives.
- Returns:
- value or
null
for none
-
setDriveId
public File setDriveId(String driveId)
Output only. ID of the shared drive the file resides in. Only populated for items in shared
drives.
- Parameters:
driveId
- driveId or null
for none
-
getExplicitlyTrashed
public Boolean getExplicitlyTrashed()
Output only. Whether the file has been explicitly trashed, as opposed to recursively trashed
from a parent folder.
- Returns:
- value or
null
for none
-
setExplicitlyTrashed
public File setExplicitlyTrashed(Boolean explicitlyTrashed)
Output only. Whether the file has been explicitly trashed, as opposed to recursively trashed
from a parent folder.
- Parameters:
explicitlyTrashed
- explicitlyTrashed or null
for none
-
getExportLinks
public Map<String,String> getExportLinks()
Output only. Links for exporting Docs Editors files to specific formats.
- Returns:
- value or
null
for none
-
setExportLinks
public File setExportLinks(Map<String,String> exportLinks)
Output only. Links for exporting Docs Editors files to specific formats.
- Parameters:
exportLinks
- exportLinks or null
for none
-
getFileExtension
public String getFileExtension()
Output only. The final component of `fullFileExtension`. This is only available for files with
binary content in Google Drive.
- Returns:
- value or
null
for none
-
setFileExtension
public File setFileExtension(String fileExtension)
Output only. The final component of `fullFileExtension`. This is only available for files with
binary content in Google Drive.
- Parameters:
fileExtension
- fileExtension or null
for none
-
getFolderColorRgb
public String getFolderColorRgb()
The color for a folder or a shortcut to a folder as an RGB hex string. The supported colors are
published in the `folderColorPalette` field of the About resource. If an unsupported color is
specified, the closest color in the palette is used instead.
- Returns:
- value or
null
for none
-
setFolderColorRgb
public File setFolderColorRgb(String folderColorRgb)
The color for a folder or a shortcut to a folder as an RGB hex string. The supported colors are
published in the `folderColorPalette` field of the About resource. If an unsupported color is
specified, the closest color in the palette is used instead.
- Parameters:
folderColorRgb
- folderColorRgb or null
for none
-
getFullFileExtension
public String getFullFileExtension()
Output only. The full file extension extracted from the `name` field. May contain multiple
concatenated extensions, such as "tar.gz". This is only available for files with binary content
in Google Drive. This is automatically updated when the `name` field changes, however it is not
cleared if the new name does not contain a valid extension.
- Returns:
- value or
null
for none
-
setFullFileExtension
public File setFullFileExtension(String fullFileExtension)
Output only. The full file extension extracted from the `name` field. May contain multiple
concatenated extensions, such as "tar.gz". This is only available for files with binary content
in Google Drive. This is automatically updated when the `name` field changes, however it is not
cleared if the new name does not contain a valid extension.
- Parameters:
fullFileExtension
- fullFileExtension or null
for none
-
getHasAugmentedPermissions
public Boolean getHasAugmentedPermissions()
Output only. Whether there are permissions directly on this file. This field is only populated
for items in shared drives.
- Returns:
- value or
null
for none
-
setHasAugmentedPermissions
public File setHasAugmentedPermissions(Boolean hasAugmentedPermissions)
Output only. Whether there are permissions directly on this file. This field is only populated
for items in shared drives.
- Parameters:
hasAugmentedPermissions
- hasAugmentedPermissions or null
for none
-
getHasThumbnail
public Boolean getHasThumbnail()
Output only. Whether this file has a thumbnail. This does not indicate whether the requesting
app has access to the thumbnail. To check access, look for the presence of the thumbnailLink
field.
- Returns:
- value or
null
for none
-
setHasThumbnail
public File setHasThumbnail(Boolean hasThumbnail)
Output only. Whether this file has a thumbnail. This does not indicate whether the requesting
app has access to the thumbnail. To check access, look for the presence of the thumbnailLink
field.
- Parameters:
hasThumbnail
- hasThumbnail or null
for none
-
getHeadRevisionId
public String getHeadRevisionId()
Output only. The ID of the file's head revision. This is currently only available for files
with binary content in Google Drive.
- Returns:
- value or
null
for none
-
setHeadRevisionId
public File setHeadRevisionId(String headRevisionId)
Output only. The ID of the file's head revision. This is currently only available for files
with binary content in Google Drive.
- Parameters:
headRevisionId
- headRevisionId or null
for none
-
getIconLink
public String getIconLink()
Output only. A static, unauthenticated link to the file's icon.
- Returns:
- value or
null
for none
-
setIconLink
public File setIconLink(String iconLink)
Output only. A static, unauthenticated link to the file's icon.
- Parameters:
iconLink
- iconLink or null
for none
-
getId
public String getId()
The ID of the file.
- Returns:
- value or
null
for none
-
getImageMediaMetadata
public File.ImageMediaMetadata getImageMediaMetadata()
Output only. Additional metadata about image media, if available.
- Returns:
- value or
null
for none
-
setImageMediaMetadata
public File setImageMediaMetadata(File.ImageMediaMetadata imageMediaMetadata)
Output only. Additional metadata about image media, if available.
- Parameters:
imageMediaMetadata
- imageMediaMetadata or null
for none
-
getIsAppAuthorized
public Boolean getIsAppAuthorized()
Output only. Whether the file was created or opened by the requesting app.
- Returns:
- value or
null
for none
-
setIsAppAuthorized
public File setIsAppAuthorized(Boolean isAppAuthorized)
Output only. Whether the file was created or opened by the requesting app.
- Parameters:
isAppAuthorized
- isAppAuthorized or null
for none
-
getKind
public String getKind()
Output only. Identifies what kind of resource this is. Value: the fixed string `"drive#file"`.
- Returns:
- value or
null
for none
-
setKind
public File setKind(String kind)
Output only. Identifies what kind of resource this is. Value: the fixed string `"drive#file"`.
- Parameters:
kind
- kind or null
for none
-
getLabelInfo
public File.LabelInfo getLabelInfo()
Output only. An overview of the labels on the file.
- Returns:
- value or
null
for none
-
setLabelInfo
public File setLabelInfo(File.LabelInfo labelInfo)
Output only. An overview of the labels on the file.
- Parameters:
labelInfo
- labelInfo or null
for none
-
getLastModifyingUser
public User getLastModifyingUser()
Output only. The last user to modify the file. This field is only populated when the last
modification was performed by a signed-in user.
- Returns:
- value or
null
for none
-
setLastModifyingUser
public File setLastModifyingUser(User lastModifyingUser)
Output only. The last user to modify the file. This field is only populated when the last
modification was performed by a signed-in user.
- Parameters:
lastModifyingUser
- lastModifyingUser or null
for none
-
getLinkShareMetadata
public File.LinkShareMetadata getLinkShareMetadata()
Contains details about the link URLs that clients are using to refer to this item.
- Returns:
- value or
null
for none
-
setLinkShareMetadata
public File setLinkShareMetadata(File.LinkShareMetadata linkShareMetadata)
Contains details about the link URLs that clients are using to refer to this item.
- Parameters:
linkShareMetadata
- linkShareMetadata or null
for none
-
getMd5Checksum
public String getMd5Checksum()
Output only. The MD5 checksum for the content of the file. This is only applicable to files
with binary content in Google Drive.
- Returns:
- value or
null
for none
-
setMd5Checksum
public File setMd5Checksum(String md5Checksum)
Output only. The MD5 checksum for the content of the file. This is only applicable to files
with binary content in Google Drive.
- Parameters:
md5Checksum
- md5Checksum or null
for none
-
getMimeType
public String getMimeType()
The MIME type of the file. Google Drive attempts to automatically detect an appropriate value
from uploaded content, if no value is provided. The value cannot be changed unless a new
revision is uploaded. If a file is created with a Google Doc MIME type, the uploaded content is
imported, if possible. The supported import formats are published in the About resource.
- Returns:
- value or
null
for none
-
setMimeType
public File setMimeType(String mimeType)
The MIME type of the file. Google Drive attempts to automatically detect an appropriate value
from uploaded content, if no value is provided. The value cannot be changed unless a new
revision is uploaded. If a file is created with a Google Doc MIME type, the uploaded content is
imported, if possible. The supported import formats are published in the About resource.
- Parameters:
mimeType
- mimeType or null
for none
-
getModifiedByMe
public Boolean getModifiedByMe()
Output only. Whether the file has been modified by this user.
- Returns:
- value or
null
for none
-
setModifiedByMe
public File setModifiedByMe(Boolean modifiedByMe)
Output only. Whether the file has been modified by this user.
- Parameters:
modifiedByMe
- modifiedByMe or null
for none
-
getModifiedByMeTime
public com.google.api.client.util.DateTime getModifiedByMeTime()
The last time the file was modified by the user (RFC 3339 date-time).
- Returns:
- value or
null
for none
-
setModifiedByMeTime
public File setModifiedByMeTime(com.google.api.client.util.DateTime modifiedByMeTime)
The last time the file was modified by the user (RFC 3339 date-time).
- Parameters:
modifiedByMeTime
- modifiedByMeTime or null
for none
-
getModifiedTime
public com.google.api.client.util.DateTime getModifiedTime()
he last time the file was modified by anyone (RFC 3339 date-time). Note that setting
modifiedTime will also update modifiedByMeTime for the user.
- Returns:
- value or
null
for none
-
setModifiedTime
public File setModifiedTime(com.google.api.client.util.DateTime modifiedTime)
he last time the file was modified by anyone (RFC 3339 date-time). Note that setting
modifiedTime will also update modifiedByMeTime for the user.
- Parameters:
modifiedTime
- modifiedTime or null
for none
-
getName
public String getName()
The name of the file. This is not necessarily unique within a folder. Note that for immutable
items such as the top level folders of shared drives, My Drive root folder, and Application
Data folder the name is constant.
- Returns:
- value or
null
for none
-
setName
public File setName(String name)
The name of the file. This is not necessarily unique within a folder. Note that for immutable
items such as the top level folders of shared drives, My Drive root folder, and Application
Data folder the name is constant.
- Parameters:
name
- name or null
for none
-
getOriginalFilename
public String getOriginalFilename()
The original filename of the uploaded content if available, or else the original value of the
`name` field. This is only available for files with binary content in Google Drive.
- Returns:
- value or
null
for none
-
setOriginalFilename
public File setOriginalFilename(String originalFilename)
The original filename of the uploaded content if available, or else the original value of the
`name` field. This is only available for files with binary content in Google Drive.
- Parameters:
originalFilename
- originalFilename or null
for none
-
getOwnedByMe
public Boolean getOwnedByMe()
Output only. Whether the user owns the file. Not populated for items in shared drives.
- Returns:
- value or
null
for none
-
setOwnedByMe
public File setOwnedByMe(Boolean ownedByMe)
Output only. Whether the user owns the file. Not populated for items in shared drives.
- Parameters:
ownedByMe
- ownedByMe or null
for none
-
getOwners
public List<User> getOwners()
Output only. The owner of this file. Only certain legacy files may have more than one owner.
This field isn't populated for items in shared drives.
- Returns:
- value or
null
for none
-
setOwners
public File setOwners(List<User> owners)
Output only. The owner of this file. Only certain legacy files may have more than one owner.
This field isn't populated for items in shared drives.
- Parameters:
owners
- owners or null
for none
-
getParents
public List<String> getParents()
The ID of the parent folder containing the file. A file can only have one parent folder;
specifying multiple parents isn't supported. If not specified as part of a create request, the
file is placed directly in the user's My Drive folder. If not specified as part of a copy
request, the file inherits any discoverable parent of the source file. Update requests must use
the `addParents` and `removeParents` parameters to modify the parents list.
- Returns:
- value or
null
for none
-
setParents
public File setParents(List<String> parents)
The ID of the parent folder containing the file. A file can only have one parent folder;
specifying multiple parents isn't supported. If not specified as part of a create request, the
file is placed directly in the user's My Drive folder. If not specified as part of a copy
request, the file inherits any discoverable parent of the source file. Update requests must use
the `addParents` and `removeParents` parameters to modify the parents list.
- Parameters:
parents
- parents or null
for none
-
getPermissionIds
public List<String> getPermissionIds()
Output only. List of permission IDs for users with access to this file.
- Returns:
- value or
null
for none
-
setPermissionIds
public File setPermissionIds(List<String> permissionIds)
Output only. List of permission IDs for users with access to this file.
- Parameters:
permissionIds
- permissionIds or null
for none
-
getPermissions
public List<Permission> getPermissions()
Output only. The full list of permissions for the file. This is only available if the
requesting user can share the file. Not populated for items in shared drives.
- Returns:
- value or
null
for none
-
setPermissions
public File setPermissions(List<Permission> permissions)
Output only. The full list of permissions for the file. This is only available if the
requesting user can share the file. Not populated for items in shared drives.
- Parameters:
permissions
- permissions or null
for none
-
getProperties
public Map<String,String> getProperties()
A collection of arbitrary key-value pairs which are visible to all apps. Entries with null
values are cleared in update and copy requests.
- Returns:
- value or
null
for none
-
setProperties
public File setProperties(Map<String,String> properties)
A collection of arbitrary key-value pairs which are visible to all apps. Entries with null
values are cleared in update and copy requests.
- Parameters:
properties
- properties or null
for none
-
getQuotaBytesUsed
public Long getQuotaBytesUsed()
Output only. The number of storage quota bytes used by the file. This includes the head
revision as well as previous revisions with `keepForever` enabled.
- Returns:
- value or
null
for none
-
setQuotaBytesUsed
public File setQuotaBytesUsed(Long quotaBytesUsed)
Output only. The number of storage quota bytes used by the file. This includes the head
revision as well as previous revisions with `keepForever` enabled.
- Parameters:
quotaBytesUsed
- quotaBytesUsed or null
for none
-
getResourceKey
public String getResourceKey()
Output only. A key needed to access the item via a shared link.
- Returns:
- value or
null
for none
-
setResourceKey
public File setResourceKey(String resourceKey)
Output only. A key needed to access the item via a shared link.
- Parameters:
resourceKey
- resourceKey or null
for none
-
getSha1Checksum
public String getSha1Checksum()
Output only. The SHA1 checksum associated with this file, if available. This field is only
populated for files with content stored in Google Drive; it is not populated for Docs Editors
or shortcut files.
- Returns:
- value or
null
for none
-
setSha1Checksum
public File setSha1Checksum(String sha1Checksum)
Output only. The SHA1 checksum associated with this file, if available. This field is only
populated for files with content stored in Google Drive; it is not populated for Docs Editors
or shortcut files.
- Parameters:
sha1Checksum
- sha1Checksum or null
for none
-
getSha256Checksum
public String getSha256Checksum()
Output only. The SHA256 checksum associated with this file, if available. This field is only
populated for files with content stored in Google Drive; it is not populated for Docs Editors
or shortcut files.
- Returns:
- value or
null
for none
-
setSha256Checksum
public File setSha256Checksum(String sha256Checksum)
Output only. The SHA256 checksum associated with this file, if available. This field is only
populated for files with content stored in Google Drive; it is not populated for Docs Editors
or shortcut files.
- Parameters:
sha256Checksum
- sha256Checksum or null
for none
-
getShared
public Boolean getShared()
Output only. Whether the file has been shared. Not populated for items in shared drives.
- Returns:
- value or
null
for none
-
setShared
public File setShared(Boolean shared)
Output only. Whether the file has been shared. Not populated for items in shared drives.
- Parameters:
shared
- shared or null
for none
-
getSharedWithMeTime
public com.google.api.client.util.DateTime getSharedWithMeTime()
The time at which the file was shared with the user, if applicable (RFC 3339 date-time).
- Returns:
- value or
null
for none
-
setSharedWithMeTime
public File setSharedWithMeTime(com.google.api.client.util.DateTime sharedWithMeTime)
The time at which the file was shared with the user, if applicable (RFC 3339 date-time).
- Parameters:
sharedWithMeTime
- sharedWithMeTime or null
for none
-
getSharingUser
public User getSharingUser()
Output only. The user who shared the file with the requesting user, if applicable.
- Returns:
- value or
null
for none
-
setSharingUser
public File setSharingUser(User sharingUser)
Output only. The user who shared the file with the requesting user, if applicable.
- Parameters:
sharingUser
- sharingUser or null
for none
-
getShortcutDetails
public File.ShortcutDetails getShortcutDetails()
Shortcut file details. Only populated for shortcut files, which have the mimeType field set to
`application/vnd.google-apps.shortcut`. Can only be set on `files.create` requests.
- Returns:
- value or
null
for none
-
setShortcutDetails
public File setShortcutDetails(File.ShortcutDetails shortcutDetails)
Shortcut file details. Only populated for shortcut files, which have the mimeType field set to
`application/vnd.google-apps.shortcut`. Can only be set on `files.create` requests.
- Parameters:
shortcutDetails
- shortcutDetails or null
for none
-
getSize
public Long getSize()
Output only. Size in bytes of blobs and first party editor files. Won't be populated for files
that have no size, like shortcuts and folders.
- Returns:
- value or
null
for none
-
setSize
public File setSize(Long size)
Output only. Size in bytes of blobs and first party editor files. Won't be populated for files
that have no size, like shortcuts and folders.
- Parameters:
size
- size or null
for none
-
getSpaces
public List<String> getSpaces()
Output only. The list of spaces which contain the file. The currently supported values are
'drive', 'appDataFolder' and 'photos'.
- Returns:
- value or
null
for none
-
setSpaces
public File setSpaces(List<String> spaces)
Output only. The list of spaces which contain the file. The currently supported values are
'drive', 'appDataFolder' and 'photos'.
- Parameters:
spaces
- spaces or null
for none
-
getStarred
public Boolean getStarred()
Whether the user has starred the file.
- Returns:
- value or
null
for none
-
setStarred
public File setStarred(Boolean starred)
Whether the user has starred the file.
- Parameters:
starred
- starred or null
for none
-
getTeamDriveId
public String getTeamDriveId()
Deprecated: Output only. Use `driveId` instead.
- Returns:
- value or
null
for none
-
setTeamDriveId
public File setTeamDriveId(String teamDriveId)
Deprecated: Output only. Use `driveId` instead.
- Parameters:
teamDriveId
- teamDriveId or null
for none
-
getThumbnailLink
public String getThumbnailLink()
Output only. A short-lived link to the file's thumbnail, if available. Typically lasts on the
order of hours. Not intended for direct usage on web applications due to [Cross-Origin Resource
Sharing (CORS)](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) policies, consider
using a proxy server. Only populated when the requesting app can access the file's content. If
the file isn't shared publicly, the URL returned in `Files.thumbnailLink` must be fetched using
a credentialed request.
- Returns:
- value or
null
for none
-
setThumbnailLink
public File setThumbnailLink(String thumbnailLink)
Output only. A short-lived link to the file's thumbnail, if available. Typically lasts on the
order of hours. Not intended for direct usage on web applications due to [Cross-Origin Resource
Sharing (CORS)](https://developer.mozilla.org/en-US/docs/Web/HTTP/CORS) policies, consider
using a proxy server. Only populated when the requesting app can access the file's content. If
the file isn't shared publicly, the URL returned in `Files.thumbnailLink` must be fetched using
a credentialed request.
- Parameters:
thumbnailLink
- thumbnailLink or null
for none
-
getThumbnailVersion
public Long getThumbnailVersion()
Output only. The thumbnail version for use in thumbnail cache invalidation.
- Returns:
- value or
null
for none
-
setThumbnailVersion
public File setThumbnailVersion(Long thumbnailVersion)
Output only. The thumbnail version for use in thumbnail cache invalidation.
- Parameters:
thumbnailVersion
- thumbnailVersion or null
for none
-
getTrashed
public Boolean getTrashed()
Whether the file has been trashed, either explicitly or from a trashed parent folder. Only the
owner may trash a file, and other users cannot see files in the owner's trash.
- Returns:
- value or
null
for none
-
setTrashed
public File setTrashed(Boolean trashed)
Whether the file has been trashed, either explicitly or from a trashed parent folder. Only the
owner may trash a file, and other users cannot see files in the owner's trash.
- Parameters:
trashed
- trashed or null
for none
-
getTrashedTime
public com.google.api.client.util.DateTime getTrashedTime()
The time that the item was trashed (RFC 3339 date-time). Only populated for items in shared
drives.
- Returns:
- value or
null
for none
-
setTrashedTime
public File setTrashedTime(com.google.api.client.util.DateTime trashedTime)
The time that the item was trashed (RFC 3339 date-time). Only populated for items in shared
drives.
- Parameters:
trashedTime
- trashedTime or null
for none
-
getTrashingUser
public User getTrashingUser()
Output only. If the file has been explicitly trashed, the user who trashed it. Only populated
for items in shared drives.
- Returns:
- value or
null
for none
-
setTrashingUser
public File setTrashingUser(User trashingUser)
Output only. If the file has been explicitly trashed, the user who trashed it. Only populated
for items in shared drives.
- Parameters:
trashingUser
- trashingUser or null
for none
-
getVersion
public Long getVersion()
Output only. A monotonically increasing version number for the file. This reflects every change
made to the file on the server, even those not visible to the user.
- Returns:
- value or
null
for none
-
setVersion
public File setVersion(Long version)
Output only. A monotonically increasing version number for the file. This reflects every change
made to the file on the server, even those not visible to the user.
- Parameters:
version
- version or null
for none
-
getVideoMediaMetadata
public File.VideoMediaMetadata getVideoMediaMetadata()
Output only. Additional metadata about video media. This may not be available immediately upon
upload.
- Returns:
- value or
null
for none
-
setVideoMediaMetadata
public File setVideoMediaMetadata(File.VideoMediaMetadata videoMediaMetadata)
Output only. Additional metadata about video media. This may not be available immediately upon
upload.
- Parameters:
videoMediaMetadata
- videoMediaMetadata or null
for none
-
getViewedByMe
public Boolean getViewedByMe()
Output only. Whether the file has been viewed by this user.
- Returns:
- value or
null
for none
-
setViewedByMe
public File setViewedByMe(Boolean viewedByMe)
Output only. Whether the file has been viewed by this user.
- Parameters:
viewedByMe
- viewedByMe or null
for none
-
getViewedByMeTime
public com.google.api.client.util.DateTime getViewedByMeTime()
The last time the file was viewed by the user (RFC 3339 date-time).
- Returns:
- value or
null
for none
-
setViewedByMeTime
public File setViewedByMeTime(com.google.api.client.util.DateTime viewedByMeTime)
The last time the file was viewed by the user (RFC 3339 date-time).
- Parameters:
viewedByMeTime
- viewedByMeTime or null
for none
-
getViewersCanCopyContent
public Boolean getViewersCanCopyContent()
Deprecated: Use `copyRequiresWriterPermission` instead.
- Returns:
- value or
null
for none
-
setViewersCanCopyContent
public File setViewersCanCopyContent(Boolean viewersCanCopyContent)
Deprecated: Use `copyRequiresWriterPermission` instead.
- Parameters:
viewersCanCopyContent
- viewersCanCopyContent or null
for none
-
getWebContentLink
public String getWebContentLink()
Output only. A link for downloading the content of the file in a browser. This is only
available for files with binary content in Google Drive.
- Returns:
- value or
null
for none
-
setWebContentLink
public File setWebContentLink(String webContentLink)
Output only. A link for downloading the content of the file in a browser. This is only
available for files with binary content in Google Drive.
- Parameters:
webContentLink
- webContentLink or null
for none
-
getWebViewLink
public String getWebViewLink()
Output only. A link for opening the file in a relevant Google editor or viewer in a browser.
- Returns:
- value or
null
for none
-
setWebViewLink
public File setWebViewLink(String webViewLink)
Output only. A link for opening the file in a relevant Google editor or viewer in a browser.
- Parameters:
webViewLink
- webViewLink or null
for none
-
getWritersCanShare
public Boolean getWritersCanShare()
Whether users with only `writer` permission can modify the file's permissions. Not populated
for items in shared drives.
- Returns:
- value or
null
for none
-
setWritersCanShare
public File setWritersCanShare(Boolean writersCanShare)
Whether users with only `writer` permission can modify the file's permissions. Not populated
for items in shared drives.
- Parameters:
writersCanShare
- writersCanShare or null
for none
-
set
public File set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public File clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy