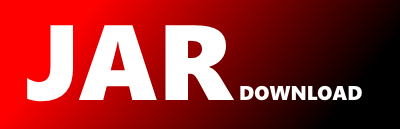
com.google.api.services.file.v1.model.PerformanceConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.file.v1.model;
/**
* Used for setting the performance configuration. If the user doesn't specify PerformanceConfig,
* automatically provision the default performance settings as described in
* https://cloud.google.com/filestore/docs/performance. Larger instances will be linearly set to
* more IOPS. If the instance's capacity is increased or decreased, its performance will be
* automatically adjusted upwards or downwards accordingly (respectively).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Filestore API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class PerformanceConfig extends com.google.api.client.json.GenericJson {
/**
* Choose a fixed provisioned IOPS value for the instance, which will remain constant regardless
* of instance capacity. Value must be a multiple of 1000. If the chosen value is outside the
* supported range for the instance's capacity during instance creation, instance creation will
* fail with an `InvalidArgument` error. Similarly, if an instance capacity update would result in
* a value outside the supported range, the update will fail with an `InvalidArgument` error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private FixedIOPS fixedIops;
/**
* Provision IOPS dynamically based on the capacity of the instance. Provisioned read IOPS will be
* calculated by multiplying the capacity of the instance in TiB by the `iops_per_tb` value. For
* example, for a 2 TiB instance with an `iops_per_tb` value of 17000 the provisioned read IOPS
* will be 34000. If the calculated value is outside the supported range for the instance's
* capacity during instance creation, instance creation will fail with an `InvalidArgument` error.
* Similarly, if an instance capacity update would result in a value outside the supported range,
* the update will fail with an `InvalidArgument` error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private IOPSPerTB iopsPerTb;
/**
* Choose a fixed provisioned IOPS value for the instance, which will remain constant regardless
* of instance capacity. Value must be a multiple of 1000. If the chosen value is outside the
* supported range for the instance's capacity during instance creation, instance creation will
* fail with an `InvalidArgument` error. Similarly, if an instance capacity update would result in
* a value outside the supported range, the update will fail with an `InvalidArgument` error.
* @return value or {@code null} for none
*/
public FixedIOPS getFixedIops() {
return fixedIops;
}
/**
* Choose a fixed provisioned IOPS value for the instance, which will remain constant regardless
* of instance capacity. Value must be a multiple of 1000. If the chosen value is outside the
* supported range for the instance's capacity during instance creation, instance creation will
* fail with an `InvalidArgument` error. Similarly, if an instance capacity update would result in
* a value outside the supported range, the update will fail with an `InvalidArgument` error.
* @param fixedIops fixedIops or {@code null} for none
*/
public PerformanceConfig setFixedIops(FixedIOPS fixedIops) {
this.fixedIops = fixedIops;
return this;
}
/**
* Provision IOPS dynamically based on the capacity of the instance. Provisioned read IOPS will be
* calculated by multiplying the capacity of the instance in TiB by the `iops_per_tb` value. For
* example, for a 2 TiB instance with an `iops_per_tb` value of 17000 the provisioned read IOPS
* will be 34000. If the calculated value is outside the supported range for the instance's
* capacity during instance creation, instance creation will fail with an `InvalidArgument` error.
* Similarly, if an instance capacity update would result in a value outside the supported range,
* the update will fail with an `InvalidArgument` error.
* @return value or {@code null} for none
*/
public IOPSPerTB getIopsPerTb() {
return iopsPerTb;
}
/**
* Provision IOPS dynamically based on the capacity of the instance. Provisioned read IOPS will be
* calculated by multiplying the capacity of the instance in TiB by the `iops_per_tb` value. For
* example, for a 2 TiB instance with an `iops_per_tb` value of 17000 the provisioned read IOPS
* will be 34000. If the calculated value is outside the supported range for the instance's
* capacity during instance creation, instance creation will fail with an `InvalidArgument` error.
* Similarly, if an instance capacity update would result in a value outside the supported range,
* the update will fail with an `InvalidArgument` error.
* @param iopsPerTb iopsPerTb or {@code null} for none
*/
public PerformanceConfig setIopsPerTb(IOPSPerTB iopsPerTb) {
this.iopsPerTb = iopsPerTb;
return this;
}
@Override
public PerformanceConfig set(String fieldName, Object value) {
return (PerformanceConfig) super.set(fieldName, value);
}
@Override
public PerformanceConfig clone() {
return (PerformanceConfig) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy