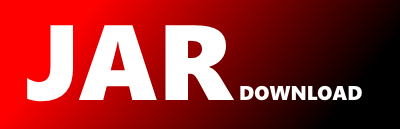
com.google.api.services.firebaseappdistribution.v1alpha.FirebaseAppDistribution Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.firebaseappdistribution.v1alpha;
/**
* Service definition for FirebaseAppDistribution (v1alpha).
*
*
*
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link FirebaseAppDistributionRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class FirebaseAppDistribution extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Firebase App Distribution API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://firebaseappdistribution.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://firebaseappdistribution.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public FirebaseAppDistribution(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
FirebaseAppDistribution(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Apps collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.Apps.List request = firebaseappdistribution.apps().list(parameters ...)}
*
*
* @return the resource collection
*/
public Apps apps() {
return new Apps();
}
/**
* The "apps" collection of methods.
*/
public class Apps {
/**
* Get the app, if it exists
*
* Create a request for the method "apps.get".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @return the request
*/
public Get get(java.lang.String mobilesdkAppId) throws java.io.IOException {
Get result = new Get(mobilesdkAppId);
initialize(result);
return result;
}
public class Get extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/apps/{mobilesdkAppId}";
/**
* Get the app, if it exists
*
* Create a request for the method "apps.get".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @since 1.13
*/
protected Get(java.lang.String mobilesdkAppId) {
super(FirebaseAppDistribution.this, "GET", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaApp.class);
this.mobilesdkAppId = com.google.api.client.util.Preconditions.checkNotNull(mobilesdkAppId, "Required parameter mobilesdkAppId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
@com.google.api.client.util.Key
private java.lang.String mobilesdkAppId;
/** Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
Example: 1:581234567376:android:aa0a3c7b135e90289
*/
public java.lang.String getMobilesdkAppId() {
return mobilesdkAppId;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
public Get setMobilesdkAppId(java.lang.String mobilesdkAppId) {
this.mobilesdkAppId = mobilesdkAppId;
return this;
}
/**
* App view. When unset or set to BASIC, returns an App with everything set except for
* aab_state. When set to FULL, returns an App with aab_state set.
*/
@com.google.api.client.util.Key
private java.lang.String appView;
/** App view. When unset or set to BASIC, returns an App with everything set except for aab_state. When
set to FULL, returns an App with aab_state set.
*/
public java.lang.String getAppView() {
return appView;
}
/**
* App view. When unset or set to BASIC, returns an App with everything set except for
* aab_state. When set to FULL, returns an App with aab_state set.
*/
public Get setAppView(java.lang.String appView) {
this.appView = appView;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Get a JWT token
*
* Create a request for the method "apps.getJwt".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link GetJwt#execute()} method to invoke the remote operation.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @return the request
*/
public GetJwt getJwt(java.lang.String mobilesdkAppId) throws java.io.IOException {
GetJwt result = new GetJwt(mobilesdkAppId);
initialize(result);
return result;
}
public class GetJwt extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/apps/{mobilesdkAppId}/jwt";
/**
* Get a JWT token
*
* Create a request for the method "apps.getJwt".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link GetJwt#execute()} method to invoke the remote
* operation. {@link
* GetJwt#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @since 1.13
*/
protected GetJwt(java.lang.String mobilesdkAppId) {
super(FirebaseAppDistribution.this, "GET", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaJwt.class);
this.mobilesdkAppId = com.google.api.client.util.Preconditions.checkNotNull(mobilesdkAppId, "Required parameter mobilesdkAppId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetJwt set$Xgafv(java.lang.String $Xgafv) {
return (GetJwt) super.set$Xgafv($Xgafv);
}
@Override
public GetJwt setAccessToken(java.lang.String accessToken) {
return (GetJwt) super.setAccessToken(accessToken);
}
@Override
public GetJwt setAlt(java.lang.String alt) {
return (GetJwt) super.setAlt(alt);
}
@Override
public GetJwt setCallback(java.lang.String callback) {
return (GetJwt) super.setCallback(callback);
}
@Override
public GetJwt setFields(java.lang.String fields) {
return (GetJwt) super.setFields(fields);
}
@Override
public GetJwt setKey(java.lang.String key) {
return (GetJwt) super.setKey(key);
}
@Override
public GetJwt setOauthToken(java.lang.String oauthToken) {
return (GetJwt) super.setOauthToken(oauthToken);
}
@Override
public GetJwt setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetJwt) super.setPrettyPrint(prettyPrint);
}
@Override
public GetJwt setQuotaUser(java.lang.String quotaUser) {
return (GetJwt) super.setQuotaUser(quotaUser);
}
@Override
public GetJwt setUploadType(java.lang.String uploadType) {
return (GetJwt) super.setUploadType(uploadType);
}
@Override
public GetJwt setUploadProtocol(java.lang.String uploadProtocol) {
return (GetJwt) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
@com.google.api.client.util.Key
private java.lang.String mobilesdkAppId;
/** Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
Example: 1:581234567376:android:aa0a3c7b135e90289
*/
public java.lang.String getMobilesdkAppId() {
return mobilesdkAppId;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
public GetJwt setMobilesdkAppId(java.lang.String mobilesdkAppId) {
this.mobilesdkAppId = mobilesdkAppId;
return this;
}
@Override
public GetJwt set(String parameterName, Object value) {
return (GetJwt) super.set(parameterName, value);
}
}
/**
* Provision app distribution for an existing Firebase app, enabling it to subsequently be used by
* appdistro.
*
* Create a request for the method "apps.provisionApp".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link ProvisionApp#execute()} method to invoke the remote
* operation.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @return the request
*/
public ProvisionApp provisionApp(java.lang.String mobilesdkAppId) throws java.io.IOException {
ProvisionApp result = new ProvisionApp(mobilesdkAppId);
initialize(result);
return result;
}
public class ProvisionApp extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/apps/{mobilesdkAppId}";
/**
* Provision app distribution for an existing Firebase app, enabling it to subsequently be used by
* appdistro.
*
* Create a request for the method "apps.provisionApp".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link ProvisionApp#execute()} method to invoke the
* remote operation. {@link
* ProvisionApp#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @since 1.13
*/
protected ProvisionApp(java.lang.String mobilesdkAppId) {
super(FirebaseAppDistribution.this, "POST", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaProvisionAppResponse.class);
this.mobilesdkAppId = com.google.api.client.util.Preconditions.checkNotNull(mobilesdkAppId, "Required parameter mobilesdkAppId must be specified.");
}
@Override
public ProvisionApp set$Xgafv(java.lang.String $Xgafv) {
return (ProvisionApp) super.set$Xgafv($Xgafv);
}
@Override
public ProvisionApp setAccessToken(java.lang.String accessToken) {
return (ProvisionApp) super.setAccessToken(accessToken);
}
@Override
public ProvisionApp setAlt(java.lang.String alt) {
return (ProvisionApp) super.setAlt(alt);
}
@Override
public ProvisionApp setCallback(java.lang.String callback) {
return (ProvisionApp) super.setCallback(callback);
}
@Override
public ProvisionApp setFields(java.lang.String fields) {
return (ProvisionApp) super.setFields(fields);
}
@Override
public ProvisionApp setKey(java.lang.String key) {
return (ProvisionApp) super.setKey(key);
}
@Override
public ProvisionApp setOauthToken(java.lang.String oauthToken) {
return (ProvisionApp) super.setOauthToken(oauthToken);
}
@Override
public ProvisionApp setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ProvisionApp) super.setPrettyPrint(prettyPrint);
}
@Override
public ProvisionApp setQuotaUser(java.lang.String quotaUser) {
return (ProvisionApp) super.setQuotaUser(quotaUser);
}
@Override
public ProvisionApp setUploadType(java.lang.String uploadType) {
return (ProvisionApp) super.setUploadType(uploadType);
}
@Override
public ProvisionApp setUploadProtocol(java.lang.String uploadProtocol) {
return (ProvisionApp) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
@com.google.api.client.util.Key
private java.lang.String mobilesdkAppId;
/** Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
Example: 1:581234567376:android:aa0a3c7b135e90289
*/
public java.lang.String getMobilesdkAppId() {
return mobilesdkAppId;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
public ProvisionApp setMobilesdkAppId(java.lang.String mobilesdkAppId) {
this.mobilesdkAppId = mobilesdkAppId;
return this;
}
@Override
public ProvisionApp set(String parameterName, Object value) {
return (ProvisionApp) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the ReleaseByHash collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.ReleaseByHash.List request = firebaseappdistribution.releaseByHash().list(parameters ...)}
*
*
* @return the resource collection
*/
public ReleaseByHash releaseByHash() {
return new ReleaseByHash();
}
/**
* The "release_by_hash" collection of methods.
*/
public class ReleaseByHash {
/**
* GET Release by binary upload hash
*
* Create a request for the method "release_by_hash.get".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @param uploadHash The hash for the upload
* @return the request
*/
public Get get(java.lang.String mobilesdkAppId, java.lang.String uploadHash) throws java.io.IOException {
Get result = new Get(mobilesdkAppId, uploadHash);
initialize(result);
return result;
}
public class Get extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/apps/{mobilesdkAppId}/release_by_hash/{uploadHash}";
/**
* GET Release by binary upload hash
*
* Create a request for the method "release_by_hash.get".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @param uploadHash The hash for the upload
* @since 1.13
*/
protected Get(java.lang.String mobilesdkAppId, java.lang.String uploadHash) {
super(FirebaseAppDistribution.this, "GET", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaGetReleaseByUploadHashResponse.class);
this.mobilesdkAppId = com.google.api.client.util.Preconditions.checkNotNull(mobilesdkAppId, "Required parameter mobilesdkAppId must be specified.");
this.uploadHash = com.google.api.client.util.Preconditions.checkNotNull(uploadHash, "Required parameter uploadHash must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
@com.google.api.client.util.Key
private java.lang.String mobilesdkAppId;
/** Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
Example: 1:581234567376:android:aa0a3c7b135e90289
*/
public java.lang.String getMobilesdkAppId() {
return mobilesdkAppId;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
public Get setMobilesdkAppId(java.lang.String mobilesdkAppId) {
this.mobilesdkAppId = mobilesdkAppId;
return this;
}
/** The hash for the upload */
@com.google.api.client.util.Key
private java.lang.String uploadHash;
/** The hash for the upload
*/
public java.lang.String getUploadHash() {
return uploadHash;
}
/** The hash for the upload */
public Get setUploadHash(java.lang.String uploadHash) {
this.uploadHash = uploadHash;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Releases collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.Releases.List request = firebaseappdistribution.releases().list(parameters ...)}
*
*
* @return the resource collection
*/
public Releases releases() {
return new Releases();
}
/**
* The "releases" collection of methods.
*/
public class Releases {
/**
* Enable access on a release for testers.
*
* Create a request for the method "releases.enable_access".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link EnableAccess#execute()} method to invoke the remote
* operation.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @param releaseId Release identifier
* @param content the {@link com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaEnableAccessOnReleaseRequest}
* @return the request
*/
public EnableAccess enableAccess(java.lang.String mobilesdkAppId, java.lang.String releaseId, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaEnableAccessOnReleaseRequest content) throws java.io.IOException {
EnableAccess result = new EnableAccess(mobilesdkAppId, releaseId, content);
initialize(result);
return result;
}
public class EnableAccess extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/apps/{mobilesdkAppId}/releases/{releaseId}/enable_access";
/**
* Enable access on a release for testers.
*
* Create a request for the method "releases.enable_access".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link EnableAccess#execute()} method to invoke the
* remote operation. {@link
* EnableAccess#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @param releaseId Release identifier
* @param content the {@link com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaEnableAccessOnReleaseRequest}
* @since 1.13
*/
protected EnableAccess(java.lang.String mobilesdkAppId, java.lang.String releaseId, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaEnableAccessOnReleaseRequest content) {
super(FirebaseAppDistribution.this, "POST", REST_PATH, content, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaEnableAccessOnReleaseResponse.class);
this.mobilesdkAppId = com.google.api.client.util.Preconditions.checkNotNull(mobilesdkAppId, "Required parameter mobilesdkAppId must be specified.");
this.releaseId = com.google.api.client.util.Preconditions.checkNotNull(releaseId, "Required parameter releaseId must be specified.");
}
@Override
public EnableAccess set$Xgafv(java.lang.String $Xgafv) {
return (EnableAccess) super.set$Xgafv($Xgafv);
}
@Override
public EnableAccess setAccessToken(java.lang.String accessToken) {
return (EnableAccess) super.setAccessToken(accessToken);
}
@Override
public EnableAccess setAlt(java.lang.String alt) {
return (EnableAccess) super.setAlt(alt);
}
@Override
public EnableAccess setCallback(java.lang.String callback) {
return (EnableAccess) super.setCallback(callback);
}
@Override
public EnableAccess setFields(java.lang.String fields) {
return (EnableAccess) super.setFields(fields);
}
@Override
public EnableAccess setKey(java.lang.String key) {
return (EnableAccess) super.setKey(key);
}
@Override
public EnableAccess setOauthToken(java.lang.String oauthToken) {
return (EnableAccess) super.setOauthToken(oauthToken);
}
@Override
public EnableAccess setPrettyPrint(java.lang.Boolean prettyPrint) {
return (EnableAccess) super.setPrettyPrint(prettyPrint);
}
@Override
public EnableAccess setQuotaUser(java.lang.String quotaUser) {
return (EnableAccess) super.setQuotaUser(quotaUser);
}
@Override
public EnableAccess setUploadType(java.lang.String uploadType) {
return (EnableAccess) super.setUploadType(uploadType);
}
@Override
public EnableAccess setUploadProtocol(java.lang.String uploadProtocol) {
return (EnableAccess) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
@com.google.api.client.util.Key
private java.lang.String mobilesdkAppId;
/** Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
Example: 1:581234567376:android:aa0a3c7b135e90289
*/
public java.lang.String getMobilesdkAppId() {
return mobilesdkAppId;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
public EnableAccess setMobilesdkAppId(java.lang.String mobilesdkAppId) {
this.mobilesdkAppId = mobilesdkAppId;
return this;
}
/** Release identifier */
@com.google.api.client.util.Key
private java.lang.String releaseId;
/** Release identifier
*/
public java.lang.String getReleaseId() {
return releaseId;
}
/** Release identifier */
public EnableAccess setReleaseId(java.lang.String releaseId) {
this.releaseId = releaseId;
return this;
}
@Override
public EnableAccess set(String parameterName, Object value) {
return (EnableAccess) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Notes collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.Notes.List request = firebaseappdistribution.notes().list(parameters ...)}
*
*
* @return the resource collection
*/
public Notes notes() {
return new Notes();
}
/**
* The "notes" collection of methods.
*/
public class Notes {
/**
* Create release notes on a release.
*
* Create a request for the method "notes.create".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @param releaseId Release identifier
* @param content the {@link com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaCreateReleaseNotesRequest}
* @return the request
*/
public Create create(java.lang.String mobilesdkAppId, java.lang.String releaseId, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaCreateReleaseNotesRequest content) throws java.io.IOException {
Create result = new Create(mobilesdkAppId, releaseId, content);
initialize(result);
return result;
}
public class Create extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/apps/{mobilesdkAppId}/releases/{releaseId}/notes";
/**
* Create release notes on a release.
*
* Create a request for the method "notes.create".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @param releaseId Release identifier
* @param content the {@link com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaCreateReleaseNotesRequest}
* @since 1.13
*/
protected Create(java.lang.String mobilesdkAppId, java.lang.String releaseId, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaCreateReleaseNotesRequest content) {
super(FirebaseAppDistribution.this, "POST", REST_PATH, content, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaCreateReleaseNotesResponse.class);
this.mobilesdkAppId = com.google.api.client.util.Preconditions.checkNotNull(mobilesdkAppId, "Required parameter mobilesdkAppId must be specified.");
this.releaseId = com.google.api.client.util.Preconditions.checkNotNull(releaseId, "Required parameter releaseId must be specified.");
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
@com.google.api.client.util.Key
private java.lang.String mobilesdkAppId;
/** Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
Example: 1:581234567376:android:aa0a3c7b135e90289
*/
public java.lang.String getMobilesdkAppId() {
return mobilesdkAppId;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
public Create setMobilesdkAppId(java.lang.String mobilesdkAppId) {
this.mobilesdkAppId = mobilesdkAppId;
return this;
}
/** Release identifier */
@com.google.api.client.util.Key
private java.lang.String releaseId;
/** Release identifier
*/
public java.lang.String getReleaseId() {
return releaseId;
}
/** Release identifier */
public Create setReleaseId(java.lang.String releaseId) {
this.releaseId = releaseId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Testers collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.Testers.List request = firebaseappdistribution.testers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Testers testers() {
return new Testers();
}
/**
* The "testers" collection of methods.
*/
public class Testers {
/**
* Get UDIDs of tester iOS devices in a project
*
* Create a request for the method "testers.getTesterUdids".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link GetTesterUdids#execute()} method to invoke the remote
* operation.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @return the request
*/
public GetTesterUdids getTesterUdids(java.lang.String mobilesdkAppId) throws java.io.IOException {
GetTesterUdids result = new GetTesterUdids(mobilesdkAppId);
initialize(result);
return result;
}
public class GetTesterUdids extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/apps/{mobilesdkAppId}/testers:getTesterUdids";
/**
* Get UDIDs of tester iOS devices in a project
*
* Create a request for the method "testers.getTesterUdids".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link GetTesterUdids#execute()} method to invoke the
* remote operation. {@link GetTesterUdids#initialize(com.google.api.client.googleapis.service
* s.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @since 1.13
*/
protected GetTesterUdids(java.lang.String mobilesdkAppId) {
super(FirebaseAppDistribution.this, "GET", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaGetTesterUdidsResponse.class);
this.mobilesdkAppId = com.google.api.client.util.Preconditions.checkNotNull(mobilesdkAppId, "Required parameter mobilesdkAppId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetTesterUdids set$Xgafv(java.lang.String $Xgafv) {
return (GetTesterUdids) super.set$Xgafv($Xgafv);
}
@Override
public GetTesterUdids setAccessToken(java.lang.String accessToken) {
return (GetTesterUdids) super.setAccessToken(accessToken);
}
@Override
public GetTesterUdids setAlt(java.lang.String alt) {
return (GetTesterUdids) super.setAlt(alt);
}
@Override
public GetTesterUdids setCallback(java.lang.String callback) {
return (GetTesterUdids) super.setCallback(callback);
}
@Override
public GetTesterUdids setFields(java.lang.String fields) {
return (GetTesterUdids) super.setFields(fields);
}
@Override
public GetTesterUdids setKey(java.lang.String key) {
return (GetTesterUdids) super.setKey(key);
}
@Override
public GetTesterUdids setOauthToken(java.lang.String oauthToken) {
return (GetTesterUdids) super.setOauthToken(oauthToken);
}
@Override
public GetTesterUdids setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetTesterUdids) super.setPrettyPrint(prettyPrint);
}
@Override
public GetTesterUdids setQuotaUser(java.lang.String quotaUser) {
return (GetTesterUdids) super.setQuotaUser(quotaUser);
}
@Override
public GetTesterUdids setUploadType(java.lang.String uploadType) {
return (GetTesterUdids) super.setUploadType(uploadType);
}
@Override
public GetTesterUdids setUploadProtocol(java.lang.String uploadProtocol) {
return (GetTesterUdids) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
@com.google.api.client.util.Key
private java.lang.String mobilesdkAppId;
/** Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
Example: 1:581234567376:android:aa0a3c7b135e90289
*/
public java.lang.String getMobilesdkAppId() {
return mobilesdkAppId;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
public GetTesterUdids setMobilesdkAppId(java.lang.String mobilesdkAppId) {
this.mobilesdkAppId = mobilesdkAppId;
return this;
}
/**
* The name of the project, which is the parent of testers Format:
* `projects/{project_number}`
*/
@com.google.api.client.util.Key
private java.lang.String project;
/** The name of the project, which is the parent of testers Format: `projects/{project_number}`
*/
public java.lang.String getProject() {
return project;
}
/**
* The name of the project, which is the parent of testers Format:
* `projects/{project_number}`
*/
public GetTesterUdids setProject(java.lang.String project) {
this.project = project;
return this;
}
@Override
public GetTesterUdids set(String parameterName, Object value) {
return (GetTesterUdids) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the UploadStatus collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.UploadStatus.List request = firebaseappdistribution.uploadStatus().list(parameters ...)}
*
*
* @return the resource collection
*/
public UploadStatus uploadStatus() {
return new UploadStatus();
}
/**
* The "upload_status" collection of methods.
*/
public class UploadStatus {
/**
* GET Binary upload status by token
*
* Create a request for the method "upload_status.get".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @param uploadToken The token for the upload
* @return the request
*/
public Get get(java.lang.String mobilesdkAppId, java.lang.String uploadToken) throws java.io.IOException {
Get result = new Get(mobilesdkAppId, uploadToken);
initialize(result);
return result;
}
public class Get extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/apps/{mobilesdkAppId}/upload_status/{uploadToken}";
/**
* GET Binary upload status by token
*
* Create a request for the method "upload_status.get".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param mobilesdkAppId Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
* Example: 1:581234567376:android:aa0a3c7b135e90289
* @param uploadToken The token for the upload
* @since 1.13
*/
protected Get(java.lang.String mobilesdkAppId, java.lang.String uploadToken) {
super(FirebaseAppDistribution.this, "GET", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaGetUploadStatusResponse.class);
this.mobilesdkAppId = com.google.api.client.util.Preconditions.checkNotNull(mobilesdkAppId, "Required parameter mobilesdkAppId must be specified.");
this.uploadToken = com.google.api.client.util.Preconditions.checkNotNull(uploadToken, "Required parameter uploadToken must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
@com.google.api.client.util.Key
private java.lang.String mobilesdkAppId;
/** Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
Example: 1:581234567376:android:aa0a3c7b135e90289
*/
public java.lang.String getMobilesdkAppId() {
return mobilesdkAppId;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
public Get setMobilesdkAppId(java.lang.String mobilesdkAppId) {
this.mobilesdkAppId = mobilesdkAppId;
return this;
}
/** The token for the upload */
@com.google.api.client.util.Key
private java.lang.String uploadToken;
/** The token for the upload
*/
public java.lang.String getUploadToken() {
return uploadToken;
}
/** The token for the upload */
public Get setUploadToken(java.lang.String uploadToken) {
this.uploadToken = uploadToken;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.Projects.List request = firebaseappdistribution.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Apps collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.Apps.List request = firebaseappdistribution.apps().list(parameters ...)}
*
*
* @return the resource collection
*/
public Apps apps() {
return new Apps();
}
/**
* The "apps" collection of methods.
*/
public class Apps {
/**
* Gets configuration for automated tests.
*
* Create a request for the method "apps.getTestConfig".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link GetTestConfig#execute()} method to invoke the remote
* operation.
*
* @param name Required. The name of the `TestConfig` resource to retrieve. Format:
* `projects/{project_number}/apps/{app_id}/testConfig`
* @return the request
*/
public GetTestConfig getTestConfig(java.lang.String name) throws java.io.IOException {
GetTestConfig result = new GetTestConfig(name);
initialize(result);
return result;
}
public class GetTestConfig extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/apps/[^/]+/testConfig$");
/**
* Gets configuration for automated tests.
*
* Create a request for the method "apps.getTestConfig".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link GetTestConfig#execute()} method to invoke the
* remote operation. {@link GetTestConfig#initialize(com.google.api.client.googleapis.services
* .AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param name Required. The name of the `TestConfig` resource to retrieve. Format:
* `projects/{project_number}/apps/{app_id}/testConfig`
* @since 1.13
*/
protected GetTestConfig(java.lang.String name) {
super(FirebaseAppDistribution.this, "GET", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaTestConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/testConfig$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetTestConfig set$Xgafv(java.lang.String $Xgafv) {
return (GetTestConfig) super.set$Xgafv($Xgafv);
}
@Override
public GetTestConfig setAccessToken(java.lang.String accessToken) {
return (GetTestConfig) super.setAccessToken(accessToken);
}
@Override
public GetTestConfig setAlt(java.lang.String alt) {
return (GetTestConfig) super.setAlt(alt);
}
@Override
public GetTestConfig setCallback(java.lang.String callback) {
return (GetTestConfig) super.setCallback(callback);
}
@Override
public GetTestConfig setFields(java.lang.String fields) {
return (GetTestConfig) super.setFields(fields);
}
@Override
public GetTestConfig setKey(java.lang.String key) {
return (GetTestConfig) super.setKey(key);
}
@Override
public GetTestConfig setOauthToken(java.lang.String oauthToken) {
return (GetTestConfig) super.setOauthToken(oauthToken);
}
@Override
public GetTestConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetTestConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public GetTestConfig setQuotaUser(java.lang.String quotaUser) {
return (GetTestConfig) super.setQuotaUser(quotaUser);
}
@Override
public GetTestConfig setUploadType(java.lang.String uploadType) {
return (GetTestConfig) super.setUploadType(uploadType);
}
@Override
public GetTestConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (GetTestConfig) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the `TestConfig` resource to retrieve. Format:
* `projects/{project_number}/apps/{app_id}/testConfig`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the `TestConfig` resource to retrieve. Format:
`projects/{project_number}/apps/{app_id}/testConfig`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the `TestConfig` resource to retrieve. Format:
* `projects/{project_number}/apps/{app_id}/testConfig`
*/
public GetTestConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/testConfig$");
}
this.name = name;
return this;
}
@Override
public GetTestConfig set(String parameterName, Object value) {
return (GetTestConfig) super.set(parameterName, value);
}
}
/**
* Updates a release.
*
* Create a request for the method "apps.updateTestConfig".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link UpdateTestConfig#execute()} method to invoke the remote
* operation.
*
* @param name Identifier. The name of the test configuration resource. Format:
* `projects/{project_number}/apps/{app_id}/testConfig`
* @param content the {@link com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaTestConfig}
* @return the request
*/
public UpdateTestConfig updateTestConfig(java.lang.String name, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaTestConfig content) throws java.io.IOException {
UpdateTestConfig result = new UpdateTestConfig(name, content);
initialize(result);
return result;
}
public class UpdateTestConfig extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/apps/[^/]+/testConfig$");
/**
* Updates a release.
*
* Create a request for the method "apps.updateTestConfig".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link UpdateTestConfig#execute()} method to invoke
* the remote operation. {@link UpdateTestConfig#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Identifier. The name of the test configuration resource. Format:
* `projects/{project_number}/apps/{app_id}/testConfig`
* @param content the {@link com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaTestConfig}
* @since 1.13
*/
protected UpdateTestConfig(java.lang.String name, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaTestConfig content) {
super(FirebaseAppDistribution.this, "PATCH", REST_PATH, content, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaTestConfig.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/testConfig$");
}
}
@Override
public UpdateTestConfig set$Xgafv(java.lang.String $Xgafv) {
return (UpdateTestConfig) super.set$Xgafv($Xgafv);
}
@Override
public UpdateTestConfig setAccessToken(java.lang.String accessToken) {
return (UpdateTestConfig) super.setAccessToken(accessToken);
}
@Override
public UpdateTestConfig setAlt(java.lang.String alt) {
return (UpdateTestConfig) super.setAlt(alt);
}
@Override
public UpdateTestConfig setCallback(java.lang.String callback) {
return (UpdateTestConfig) super.setCallback(callback);
}
@Override
public UpdateTestConfig setFields(java.lang.String fields) {
return (UpdateTestConfig) super.setFields(fields);
}
@Override
public UpdateTestConfig setKey(java.lang.String key) {
return (UpdateTestConfig) super.setKey(key);
}
@Override
public UpdateTestConfig setOauthToken(java.lang.String oauthToken) {
return (UpdateTestConfig) super.setOauthToken(oauthToken);
}
@Override
public UpdateTestConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateTestConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateTestConfig setQuotaUser(java.lang.String quotaUser) {
return (UpdateTestConfig) super.setQuotaUser(quotaUser);
}
@Override
public UpdateTestConfig setUploadType(java.lang.String uploadType) {
return (UpdateTestConfig) super.setUploadType(uploadType);
}
@Override
public UpdateTestConfig setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateTestConfig) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. The name of the test configuration resource. Format:
* `projects/{project_number}/apps/{app_id}/testConfig`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. The name of the test configuration resource. Format:
`projects/{project_number}/apps/{app_id}/testConfig`
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. The name of the test configuration resource. Format:
* `projects/{project_number}/apps/{app_id}/testConfig`
*/
public UpdateTestConfig setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/testConfig$");
}
this.name = name;
return this;
}
/** Optional. The list of fields to update. */
@com.google.api.client.util.Key
private String updateMask;
/** Optional. The list of fields to update.
*/
public String getUpdateMask() {
return updateMask;
}
/** Optional. The list of fields to update. */
public UpdateTestConfig setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateTestConfig set(String parameterName, Object value) {
return (UpdateTestConfig) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Releases collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.Releases.List request = firebaseappdistribution.releases().list(parameters ...)}
*
*
* @return the resource collection
*/
public Releases releases() {
return new Releases();
}
/**
* The "releases" collection of methods.
*/
public class Releases {
/**
* An accessor for creating requests from the Tests collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.Tests.List request = firebaseappdistribution.tests().list(parameters ...)}
*
*
* @return the resource collection
*/
public Tests tests() {
return new Tests();
}
/**
* The "tests" collection of methods.
*/
public class Tests {
/**
* Run automated test(s) on release.
*
* Create a request for the method "tests.create".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the release resource, which is the parent of the test Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}`
* @param content the {@link com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaReleaseTest}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaReleaseTest content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/{+parent}/tests";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/apps/[^/]+/releases/[^/]+$");
/**
* Run automated test(s) on release.
*
* Create a request for the method "tests.create".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link Create#execute()} method to invoke the remote
* operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the release resource, which is the parent of the test Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}`
* @param content the {@link com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaReleaseTest}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaReleaseTest content) {
super(FirebaseAppDistribution.this, "POST", REST_PATH, content, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaReleaseTest.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/releases/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the release resource, which is the parent of the test Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the release resource, which is the parent of the test Format:
`projects/{project_number}/apps/{app_id}/releases/{release_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the release resource, which is the parent of the test Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}`
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/releases/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The ID to use for the test, which will become the final component of the
* tests's resource name. This value should be 4-63 characters, and valid characters are
* /a-z-/. If it is not provided one will be automatically generated.
*/
@com.google.api.client.util.Key
private java.lang.String releaseTestId;
/** Optional. The ID to use for the test, which will become the final component of the tests's resource
name. This value should be 4-63 characters, and valid characters are /a-z-/. If it is not provided
one will be automatically generated.
*/
public java.lang.String getReleaseTestId() {
return releaseTestId;
}
/**
* Optional. The ID to use for the test, which will become the final component of the
* tests's resource name. This value should be 4-63 characters, and valid characters are
* /a-z-/. If it is not provided one will be automatically generated.
*/
public Create setReleaseTestId(java.lang.String releaseTestId) {
this.releaseTestId = releaseTestId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Get results for automated test run on release.
*
* Create a request for the method "tests.get".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The name of the release test resource. Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}/tests/{test_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/apps/[^/]+/releases/[^/]+/tests/[^/]+$");
/**
* Get results for automated test run on release.
*
* Create a request for the method "tests.get".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link Get#execute()} method to invoke the remote
* operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The name of the release test resource. Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}/tests/{test_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(FirebaseAppDistribution.this, "GET", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaReleaseTest.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/releases/[^/]+/tests/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the release test resource. Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}/tests/{test_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The name of the release test resource. Format:
`projects/{project_number}/apps/{app_id}/releases/{release_id}/tests/{test_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The name of the release test resource. Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}/tests/{test_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/releases/[^/]+/tests/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List results for automated tests run on release.
*
* Create a request for the method "tests.list".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. The name of the release resource, which is the parent of the tests Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}`
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/{+parent}/tests";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/apps/[^/]+/releases/[^/]+$");
/**
* List results for automated tests run on release.
*
* Create a request for the method "tests.list".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link List#execute()} method to invoke the remote
* operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The name of the release resource, which is the parent of the tests Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}`
* @since 1.13
*/
protected List(java.lang.String parent) {
super(FirebaseAppDistribution.this, "GET", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaListReleaseTestsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/releases/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The name of the release resource, which is the parent of the tests Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}`
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The name of the release resource, which is the parent of the tests Format:
`projects/{project_number}/apps/{app_id}/releases/{release_id}`
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The name of the release resource, which is the parent of the tests Format:
* `projects/{project_number}/apps/{app_id}/releases/{release_id}`
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/apps/[^/]+/releases/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Optional. The maximum number of tests to return. The service may return fewer than
* this value.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Optional. The maximum number of tests to return. The service may return fewer than this value.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Optional. The maximum number of tests to return. The service may return fewer than
* this value.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* Optional. A page token, received from a previous `ListReleaseTests` call. Provide
* this to retrieve the subsequent page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Optional. A page token, received from a previous `ListReleaseTests` call. Provide this to retrieve
the subsequent page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* Optional. A page token, received from a previous `ListReleaseTests` call. Provide
* this to retrieve the subsequent page.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
}
/**
* An accessor for creating requests from the Testers collection.
*
* The typical use is:
*
* {@code FirebaseAppDistribution firebaseappdistribution = new FirebaseAppDistribution(...);}
* {@code FirebaseAppDistribution.Testers.List request = firebaseappdistribution.testers().list(parameters ...)}
*
*
* @return the resource collection
*/
public Testers testers() {
return new Testers();
}
/**
* The "testers" collection of methods.
*/
public class Testers {
/**
* Get UDIDs of tester iOS devices in a project
*
* Create a request for the method "testers.getUdids".
*
* This request holds the parameters needed by the firebaseappdistribution server. After setting
* any optional parameters, call the {@link GetUdids#execute()} method to invoke the remote
* operation.
*
* @param project The name of the project, which is the parent of testers Format: `projects/{project_number}`
* @return the request
*/
public GetUdids getUdids(java.lang.String project) throws java.io.IOException {
GetUdids result = new GetUdids(project);
initialize(result);
return result;
}
public class GetUdids extends FirebaseAppDistributionRequest {
private static final String REST_PATH = "v1alpha/{+project}/testers:udids";
private final java.util.regex.Pattern PROJECT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Get UDIDs of tester iOS devices in a project
*
* Create a request for the method "testers.getUdids".
*
* This request holds the parameters needed by the the firebaseappdistribution server. After
* setting any optional parameters, call the {@link GetUdids#execute()} method to invoke the
* remote operation. {@link
* GetUdids#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param project The name of the project, which is the parent of testers Format: `projects/{project_number}`
* @since 1.13
*/
protected GetUdids(java.lang.String project) {
super(FirebaseAppDistribution.this, "GET", REST_PATH, null, com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaGetTesterUdidsResponse.class);
this.project = com.google.api.client.util.Preconditions.checkNotNull(project, "Required parameter project must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetUdids set$Xgafv(java.lang.String $Xgafv) {
return (GetUdids) super.set$Xgafv($Xgafv);
}
@Override
public GetUdids setAccessToken(java.lang.String accessToken) {
return (GetUdids) super.setAccessToken(accessToken);
}
@Override
public GetUdids setAlt(java.lang.String alt) {
return (GetUdids) super.setAlt(alt);
}
@Override
public GetUdids setCallback(java.lang.String callback) {
return (GetUdids) super.setCallback(callback);
}
@Override
public GetUdids setFields(java.lang.String fields) {
return (GetUdids) super.setFields(fields);
}
@Override
public GetUdids setKey(java.lang.String key) {
return (GetUdids) super.setKey(key);
}
@Override
public GetUdids setOauthToken(java.lang.String oauthToken) {
return (GetUdids) super.setOauthToken(oauthToken);
}
@Override
public GetUdids setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetUdids) super.setPrettyPrint(prettyPrint);
}
@Override
public GetUdids setQuotaUser(java.lang.String quotaUser) {
return (GetUdids) super.setQuotaUser(quotaUser);
}
@Override
public GetUdids setUploadType(java.lang.String uploadType) {
return (GetUdids) super.setUploadType(uploadType);
}
@Override
public GetUdids setUploadProtocol(java.lang.String uploadProtocol) {
return (GetUdids) super.setUploadProtocol(uploadProtocol);
}
/**
* The name of the project, which is the parent of testers Format:
* `projects/{project_number}`
*/
@com.google.api.client.util.Key
private java.lang.String project;
/** The name of the project, which is the parent of testers Format: `projects/{project_number}`
*/
public java.lang.String getProject() {
return project;
}
/**
* The name of the project, which is the parent of testers Format:
* `projects/{project_number}`
*/
public GetUdids setProject(java.lang.String project) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PROJECT_PATTERN.matcher(project).matches(),
"Parameter project must conform to the pattern " +
"^projects/[^/]+$");
}
this.project = project;
return this;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
@com.google.api.client.util.Key
private java.lang.String mobilesdkAppId;
/** Unique id for a Firebase app of the format: {version}:{project_number}:{platform}:{hash(bundle_id)}
Example: 1:581234567376:android:aa0a3c7b135e90289
*/
public java.lang.String getMobilesdkAppId() {
return mobilesdkAppId;
}
/**
* Unique id for a Firebase app of the format:
* {version}:{project_number}:{platform}:{hash(bundle_id)} Example:
* 1:581234567376:android:aa0a3c7b135e90289
*/
public GetUdids setMobilesdkAppId(java.lang.String mobilesdkAppId) {
this.mobilesdkAppId = mobilesdkAppId;
return this;
}
@Override
public GetUdids set(String parameterName, Object value) {
return (GetUdids) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link FirebaseAppDistribution}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link FirebaseAppDistribution}. */
@Override
public FirebaseAppDistribution build() {
return new FirebaseAppDistribution(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link FirebaseAppDistributionRequestInitializer}.
*
* @since 1.12
*/
public Builder setFirebaseAppDistributionRequestInitializer(
FirebaseAppDistributionRequestInitializer firebaseappdistributionRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(firebaseappdistributionRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}