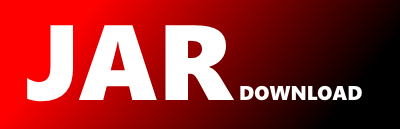
com.google.api.services.firebaseappdistribution.v1alpha.model.GoogleFirebaseAppdistroV1alphaRelease Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.firebaseappdistribution.v1alpha.model;
/**
* Proto defining a release object
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Firebase App Distribution API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleFirebaseAppdistroV1alphaRelease extends com.google.api.client.json.GenericJson {
/**
* Release build version
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String buildVersion;
/**
* Release version
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String displayVersion;
/**
* Timestamp when the release was created
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String distributedAt;
/**
* Release Id
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* Instance id of the release
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String instanceId;
/**
* Last activity timestamp
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String lastActivityAt;
/**
* Number of testers who have open invitations for the release
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer openInvitationCount;
/**
* unused.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private String receivedAt;
/**
* Release notes summary
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String releaseNotesSummary;
/**
* Count of testers added to the release
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer testerCount;
/**
* Number of testers who have installed the release
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer testerWithInstallCount;
/**
* Release build version
* @return value or {@code null} for none
*/
public java.lang.String getBuildVersion() {
return buildVersion;
}
/**
* Release build version
* @param buildVersion buildVersion or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setBuildVersion(java.lang.String buildVersion) {
this.buildVersion = buildVersion;
return this;
}
/**
* Release version
* @return value or {@code null} for none
*/
public java.lang.String getDisplayVersion() {
return displayVersion;
}
/**
* Release version
* @param displayVersion displayVersion or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setDisplayVersion(java.lang.String displayVersion) {
this.displayVersion = displayVersion;
return this;
}
/**
* Timestamp when the release was created
* @return value or {@code null} for none
*/
public String getDistributedAt() {
return distributedAt;
}
/**
* Timestamp when the release was created
* @param distributedAt distributedAt or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setDistributedAt(String distributedAt) {
this.distributedAt = distributedAt;
return this;
}
/**
* Release Id
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* Release Id
* @param id id or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Instance id of the release
* @return value or {@code null} for none
*/
public java.lang.String getInstanceId() {
return instanceId;
}
/**
* Instance id of the release
* @param instanceId instanceId or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setInstanceId(java.lang.String instanceId) {
this.instanceId = instanceId;
return this;
}
/**
* Last activity timestamp
* @return value or {@code null} for none
*/
public String getLastActivityAt() {
return lastActivityAt;
}
/**
* Last activity timestamp
* @param lastActivityAt lastActivityAt or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setLastActivityAt(String lastActivityAt) {
this.lastActivityAt = lastActivityAt;
return this;
}
/**
* Number of testers who have open invitations for the release
* @return value or {@code null} for none
*/
public java.lang.Integer getOpenInvitationCount() {
return openInvitationCount;
}
/**
* Number of testers who have open invitations for the release
* @param openInvitationCount openInvitationCount or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setOpenInvitationCount(java.lang.Integer openInvitationCount) {
this.openInvitationCount = openInvitationCount;
return this;
}
/**
* unused.
* @return value or {@code null} for none
*/
public String getReceivedAt() {
return receivedAt;
}
/**
* unused.
* @param receivedAt receivedAt or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setReceivedAt(String receivedAt) {
this.receivedAt = receivedAt;
return this;
}
/**
* Release notes summary
* @return value or {@code null} for none
*/
public java.lang.String getReleaseNotesSummary() {
return releaseNotesSummary;
}
/**
* Release notes summary
* @param releaseNotesSummary releaseNotesSummary or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setReleaseNotesSummary(java.lang.String releaseNotesSummary) {
this.releaseNotesSummary = releaseNotesSummary;
return this;
}
/**
* Count of testers added to the release
* @return value or {@code null} for none
*/
public java.lang.Integer getTesterCount() {
return testerCount;
}
/**
* Count of testers added to the release
* @param testerCount testerCount or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setTesterCount(java.lang.Integer testerCount) {
this.testerCount = testerCount;
return this;
}
/**
* Number of testers who have installed the release
* @return value or {@code null} for none
*/
public java.lang.Integer getTesterWithInstallCount() {
return testerWithInstallCount;
}
/**
* Number of testers who have installed the release
* @param testerWithInstallCount testerWithInstallCount or {@code null} for none
*/
public GoogleFirebaseAppdistroV1alphaRelease setTesterWithInstallCount(java.lang.Integer testerWithInstallCount) {
this.testerWithInstallCount = testerWithInstallCount;
return this;
}
@Override
public GoogleFirebaseAppdistroV1alphaRelease set(String fieldName, Object value) {
return (GoogleFirebaseAppdistroV1alphaRelease) super.set(fieldName, value);
}
@Override
public GoogleFirebaseAppdistroV1alphaRelease clone() {
return (GoogleFirebaseAppdistroV1alphaRelease) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy