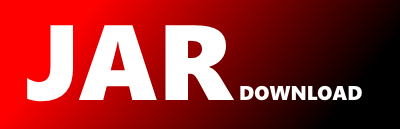
com.google.api.services.firebasedynamiclinks.v1.model.GooglePlayAnalytics Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-09-20 at 23:08:05 UTC
* Modify at your own risk.
*/
package com.google.api.services.firebasedynamiclinks.v1.model;
/**
* Parameters for Google Play Campaign Measurements. [Learn
* more](https://developers.google.com/analytics/devguides/collection/android/v4/campaigns#campaign-
* params)
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Firebase Dynamic Links API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GooglePlayAnalytics extends com.google.api.client.json.GenericJson {
/**
* [AdWords autotagging parameter](https://support.google.com/analytics/answer/1033981?hl=en);
* used to measure Google AdWords ads. This value is generated dynamically and should never be
* modified.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gclid;
/**
* Campaign name; used for keyword analysis to identify a specific product promotion or strategic
* campaign.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String utmCampaign;
/**
* Campaign content; used for A/B testing and content-targeted ads to differentiate ads or links
* that point to the same URL.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String utmContent;
/**
* Campaign medium; used to identify a medium such as email or cost-per-click.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String utmMedium;
/**
* Campaign source; used to identify a search engine, newsletter, or other source.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String utmSource;
/**
* Campaign term; used with paid search to supply the keywords for ads.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String utmTerm;
/**
* [AdWords autotagging parameter](https://support.google.com/analytics/answer/1033981?hl=en);
* used to measure Google AdWords ads. This value is generated dynamically and should never be
* modified.
* @return value or {@code null} for none
*/
public java.lang.String getGclid() {
return gclid;
}
/**
* [AdWords autotagging parameter](https://support.google.com/analytics/answer/1033981?hl=en);
* used to measure Google AdWords ads. This value is generated dynamically and should never be
* modified.
* @param gclid gclid or {@code null} for none
*/
public GooglePlayAnalytics setGclid(java.lang.String gclid) {
this.gclid = gclid;
return this;
}
/**
* Campaign name; used for keyword analysis to identify a specific product promotion or strategic
* campaign.
* @return value or {@code null} for none
*/
public java.lang.String getUtmCampaign() {
return utmCampaign;
}
/**
* Campaign name; used for keyword analysis to identify a specific product promotion or strategic
* campaign.
* @param utmCampaign utmCampaign or {@code null} for none
*/
public GooglePlayAnalytics setUtmCampaign(java.lang.String utmCampaign) {
this.utmCampaign = utmCampaign;
return this;
}
/**
* Campaign content; used for A/B testing and content-targeted ads to differentiate ads or links
* that point to the same URL.
* @return value or {@code null} for none
*/
public java.lang.String getUtmContent() {
return utmContent;
}
/**
* Campaign content; used for A/B testing and content-targeted ads to differentiate ads or links
* that point to the same URL.
* @param utmContent utmContent or {@code null} for none
*/
public GooglePlayAnalytics setUtmContent(java.lang.String utmContent) {
this.utmContent = utmContent;
return this;
}
/**
* Campaign medium; used to identify a medium such as email or cost-per-click.
* @return value or {@code null} for none
*/
public java.lang.String getUtmMedium() {
return utmMedium;
}
/**
* Campaign medium; used to identify a medium such as email or cost-per-click.
* @param utmMedium utmMedium or {@code null} for none
*/
public GooglePlayAnalytics setUtmMedium(java.lang.String utmMedium) {
this.utmMedium = utmMedium;
return this;
}
/**
* Campaign source; used to identify a search engine, newsletter, or other source.
* @return value or {@code null} for none
*/
public java.lang.String getUtmSource() {
return utmSource;
}
/**
* Campaign source; used to identify a search engine, newsletter, or other source.
* @param utmSource utmSource or {@code null} for none
*/
public GooglePlayAnalytics setUtmSource(java.lang.String utmSource) {
this.utmSource = utmSource;
return this;
}
/**
* Campaign term; used with paid search to supply the keywords for ads.
* @return value or {@code null} for none
*/
public java.lang.String getUtmTerm() {
return utmTerm;
}
/**
* Campaign term; used with paid search to supply the keywords for ads.
* @param utmTerm utmTerm or {@code null} for none
*/
public GooglePlayAnalytics setUtmTerm(java.lang.String utmTerm) {
this.utmTerm = utmTerm;
return this;
}
@Override
public GooglePlayAnalytics set(String fieldName, Object value) {
return (GooglePlayAnalytics) super.set(fieldName, value);
}
@Override
public GooglePlayAnalytics clone() {
return (GooglePlayAnalytics) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy