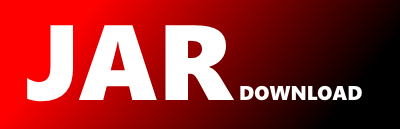
com.google.api.services.firebaseml.v2beta.model.GoogleCloudAiplatformV1beta1Schema Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.firebaseml.v2beta.model;
/**
* Schema is used to define the format of input/output data. Represents a select subset of an
* [OpenAPI 3.0 schema object](https://spec.openapis.org/oas/v3.0.3#schema-object). More fields may
* be added in the future as needed.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Firebase ML API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudAiplatformV1beta1Schema extends com.google.api.client.json.GenericJson {
/**
* Optional. The value should be validated against any (one or more) of the subschemas in the
* list.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List anyOf;
/**
* Optional. Default value of the data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("default")
private java.lang.Object default__;
/**
* Optional. The description of the data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* Optional. Possible values of the element of primitive type with enum format. Examples: 1. We
* can define direction as : {type:STRING, format:enum, enum:["EAST", NORTH", "SOUTH", "WEST"]} 2.
* We can define apartment number as : {type:INTEGER, format:enum, enum:["101", "201", "301"]}
* The value may be {@code null}.
*/
@com.google.api.client.util.Key("enum")
private java.util.List enum__;
/**
* Optional. Example of the object. Will only populated when the object is the root.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Object example;
/**
* Optional. The format of the data. Supported formats: for NUMBER type: "float", "double" for
* INTEGER type: "int32", "int64" for STRING type: "email", "byte", etc
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String format;
/**
* Optional. SCHEMA FIELDS FOR TYPE ARRAY Schema of the elements of Type.ARRAY.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudAiplatformV1beta1Schema items;
/**
* Optional. Maximum number of the elements for Type.ARRAY.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long maxItems;
/**
* Optional. Maximum length of the Type.STRING
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long maxLength;
/**
* Optional. Maximum number of the properties for Type.OBJECT.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long maxProperties;
/**
* Optional. Maximum value of the Type.INTEGER and Type.NUMBER
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double maximum;
/**
* Optional. Minimum number of the elements for Type.ARRAY.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long minItems;
/**
* Optional. SCHEMA FIELDS FOR TYPE STRING Minimum length of the Type.STRING
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long minLength;
/**
* Optional. Minimum number of the properties for Type.OBJECT.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long minProperties;
/**
* Optional. SCHEMA FIELDS FOR TYPE INTEGER and NUMBER Minimum value of the Type.INTEGER and
* Type.NUMBER
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double minimum;
/**
* Optional. Indicates if the value may be null.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean nullable;
/**
* Optional. Pattern of the Type.STRING to restrict a string to a regular expression.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String pattern;
/**
* Optional. SCHEMA FIELDS FOR TYPE OBJECT Properties of Type.OBJECT.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map properties;
/**
* Optional. The order of the properties. Not a standard field in open api spec. Only used to
* support the order of the properties.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List propertyOrdering;
/**
* Optional. Required properties of Type.OBJECT.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List required;
/**
* Optional. The title of the Schema.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String title;
/**
* Optional. The type of the data.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* Optional. The value should be validated against any (one or more) of the subschemas in the
* list.
* @return value or {@code null} for none
*/
public java.util.List getAnyOf() {
return anyOf;
}
/**
* Optional. The value should be validated against any (one or more) of the subschemas in the
* list.
* @param anyOf anyOf or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setAnyOf(java.util.List anyOf) {
this.anyOf = anyOf;
return this;
}
/**
* Optional. Default value of the data.
* @return value or {@code null} for none
*/
public java.lang.Object getDefault() {
return default__;
}
/**
* Optional. Default value of the data.
* @param default__ default__ or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setDefault(java.lang.Object default__) {
this.default__ = default__;
return this;
}
/**
* Optional. The description of the data.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Optional. The description of the data.
* @param description description or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* Optional. Possible values of the element of primitive type with enum format. Examples: 1. We
* can define direction as : {type:STRING, format:enum, enum:["EAST", NORTH", "SOUTH", "WEST"]} 2.
* We can define apartment number as : {type:INTEGER, format:enum, enum:["101", "201", "301"]}
* @return value or {@code null} for none
*/
public java.util.List getEnum() {
return enum__;
}
/**
* Optional. Possible values of the element of primitive type with enum format. Examples: 1. We
* can define direction as : {type:STRING, format:enum, enum:["EAST", NORTH", "SOUTH", "WEST"]} 2.
* We can define apartment number as : {type:INTEGER, format:enum, enum:["101", "201", "301"]}
* @param enum__ enum__ or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setEnum(java.util.List enum__) {
this.enum__ = enum__;
return this;
}
/**
* Optional. Example of the object. Will only populated when the object is the root.
* @return value or {@code null} for none
*/
public java.lang.Object getExample() {
return example;
}
/**
* Optional. Example of the object. Will only populated when the object is the root.
* @param example example or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setExample(java.lang.Object example) {
this.example = example;
return this;
}
/**
* Optional. The format of the data. Supported formats: for NUMBER type: "float", "double" for
* INTEGER type: "int32", "int64" for STRING type: "email", "byte", etc
* @return value or {@code null} for none
*/
public java.lang.String getFormat() {
return format;
}
/**
* Optional. The format of the data. Supported formats: for NUMBER type: "float", "double" for
* INTEGER type: "int32", "int64" for STRING type: "email", "byte", etc
* @param format format or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setFormat(java.lang.String format) {
this.format = format;
return this;
}
/**
* Optional. SCHEMA FIELDS FOR TYPE ARRAY Schema of the elements of Type.ARRAY.
* @return value or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema getItems() {
return items;
}
/**
* Optional. SCHEMA FIELDS FOR TYPE ARRAY Schema of the elements of Type.ARRAY.
* @param items items or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setItems(GoogleCloudAiplatformV1beta1Schema items) {
this.items = items;
return this;
}
/**
* Optional. Maximum number of the elements for Type.ARRAY.
* @return value or {@code null} for none
*/
public java.lang.Long getMaxItems() {
return maxItems;
}
/**
* Optional. Maximum number of the elements for Type.ARRAY.
* @param maxItems maxItems or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setMaxItems(java.lang.Long maxItems) {
this.maxItems = maxItems;
return this;
}
/**
* Optional. Maximum length of the Type.STRING
* @return value or {@code null} for none
*/
public java.lang.Long getMaxLength() {
return maxLength;
}
/**
* Optional. Maximum length of the Type.STRING
* @param maxLength maxLength or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setMaxLength(java.lang.Long maxLength) {
this.maxLength = maxLength;
return this;
}
/**
* Optional. Maximum number of the properties for Type.OBJECT.
* @return value or {@code null} for none
*/
public java.lang.Long getMaxProperties() {
return maxProperties;
}
/**
* Optional. Maximum number of the properties for Type.OBJECT.
* @param maxProperties maxProperties or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setMaxProperties(java.lang.Long maxProperties) {
this.maxProperties = maxProperties;
return this;
}
/**
* Optional. Maximum value of the Type.INTEGER and Type.NUMBER
* @return value or {@code null} for none
*/
public java.lang.Double getMaximum() {
return maximum;
}
/**
* Optional. Maximum value of the Type.INTEGER and Type.NUMBER
* @param maximum maximum or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setMaximum(java.lang.Double maximum) {
this.maximum = maximum;
return this;
}
/**
* Optional. Minimum number of the elements for Type.ARRAY.
* @return value or {@code null} for none
*/
public java.lang.Long getMinItems() {
return minItems;
}
/**
* Optional. Minimum number of the elements for Type.ARRAY.
* @param minItems minItems or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setMinItems(java.lang.Long minItems) {
this.minItems = minItems;
return this;
}
/**
* Optional. SCHEMA FIELDS FOR TYPE STRING Minimum length of the Type.STRING
* @return value or {@code null} for none
*/
public java.lang.Long getMinLength() {
return minLength;
}
/**
* Optional. SCHEMA FIELDS FOR TYPE STRING Minimum length of the Type.STRING
* @param minLength minLength or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setMinLength(java.lang.Long minLength) {
this.minLength = minLength;
return this;
}
/**
* Optional. Minimum number of the properties for Type.OBJECT.
* @return value or {@code null} for none
*/
public java.lang.Long getMinProperties() {
return minProperties;
}
/**
* Optional. Minimum number of the properties for Type.OBJECT.
* @param minProperties minProperties or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setMinProperties(java.lang.Long minProperties) {
this.minProperties = minProperties;
return this;
}
/**
* Optional. SCHEMA FIELDS FOR TYPE INTEGER and NUMBER Minimum value of the Type.INTEGER and
* Type.NUMBER
* @return value or {@code null} for none
*/
public java.lang.Double getMinimum() {
return minimum;
}
/**
* Optional. SCHEMA FIELDS FOR TYPE INTEGER and NUMBER Minimum value of the Type.INTEGER and
* Type.NUMBER
* @param minimum minimum or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setMinimum(java.lang.Double minimum) {
this.minimum = minimum;
return this;
}
/**
* Optional. Indicates if the value may be null.
* @return value or {@code null} for none
*/
public java.lang.Boolean getNullable() {
return nullable;
}
/**
* Optional. Indicates if the value may be null.
* @param nullable nullable or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setNullable(java.lang.Boolean nullable) {
this.nullable = nullable;
return this;
}
/**
* Optional. Pattern of the Type.STRING to restrict a string to a regular expression.
* @return value or {@code null} for none
*/
public java.lang.String getPattern() {
return pattern;
}
/**
* Optional. Pattern of the Type.STRING to restrict a string to a regular expression.
* @param pattern pattern or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setPattern(java.lang.String pattern) {
this.pattern = pattern;
return this;
}
/**
* Optional. SCHEMA FIELDS FOR TYPE OBJECT Properties of Type.OBJECT.
* @return value or {@code null} for none
*/
public java.util.Map getProperties() {
return properties;
}
/**
* Optional. SCHEMA FIELDS FOR TYPE OBJECT Properties of Type.OBJECT.
* @param properties properties or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setProperties(java.util.Map properties) {
this.properties = properties;
return this;
}
/**
* Optional. The order of the properties. Not a standard field in open api spec. Only used to
* support the order of the properties.
* @return value or {@code null} for none
*/
public java.util.List getPropertyOrdering() {
return propertyOrdering;
}
/**
* Optional. The order of the properties. Not a standard field in open api spec. Only used to
* support the order of the properties.
* @param propertyOrdering propertyOrdering or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setPropertyOrdering(java.util.List propertyOrdering) {
this.propertyOrdering = propertyOrdering;
return this;
}
/**
* Optional. Required properties of Type.OBJECT.
* @return value or {@code null} for none
*/
public java.util.List getRequired() {
return required;
}
/**
* Optional. Required properties of Type.OBJECT.
* @param required required or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setRequired(java.util.List required) {
this.required = required;
return this;
}
/**
* Optional. The title of the Schema.
* @return value or {@code null} for none
*/
public java.lang.String getTitle() {
return title;
}
/**
* Optional. The title of the Schema.
* @param title title or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setTitle(java.lang.String title) {
this.title = title;
return this;
}
/**
* Optional. The type of the data.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Optional. The type of the data.
* @param type type or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Schema setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public GoogleCloudAiplatformV1beta1Schema set(String fieldName, Object value) {
return (GoogleCloudAiplatformV1beta1Schema) super.set(fieldName, value);
}
@Override
public GoogleCloudAiplatformV1beta1Schema clone() {
return (GoogleCloudAiplatformV1beta1Schema) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy