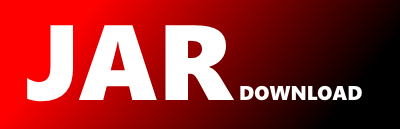
com.google.api.services.firebaseml.v2beta.model.GoogleCloudAiplatformV1beta1Tool Maven / Gradle / Ivy
The newest version!
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.firebaseml.v2beta.model;
/**
* Tool details that the model may use to generate response. A `Tool` is a piece of code that
* enables the system to interact with external systems to perform an action, or set of actions,
* outside of knowledge and scope of the model. A Tool object should contain exactly one type of
* Tool (e.g FunctionDeclaration, Retrieval or GoogleSearchRetrieval).
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Firebase ML API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class GoogleCloudAiplatformV1beta1Tool extends com.google.api.client.json.GenericJson {
/**
* Optional. CodeExecution tool type. Enables the model to execute code as part of generation.
* This field is only used by the Gemini Developer API services.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudAiplatformV1beta1ToolCodeExecution codeExecution;
/**
* Optional. Function tool type. One or more function declarations to be passed to the model along
* with the current user query. Model may decide to call a subset of these functions by populating
* FunctionCall in the response. User should provide a FunctionResponse for each function call in
* the next turn. Based on the function responses, Model will generate the final response back to
* the user. Maximum 128 function declarations can be provided.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List functionDeclarations;
static {
// hack to force ProGuard to consider GoogleCloudAiplatformV1beta1FunctionDeclaration used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(GoogleCloudAiplatformV1beta1FunctionDeclaration.class);
}
/**
* Optional. GoogleSearch tool type. Tool to support Google Search in Model. Powered by Google.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudAiplatformV1beta1ToolGoogleSearch googleSearch;
/**
* Optional. GoogleSearchRetrieval tool type. Specialized retrieval tool that is powered by Google
* search.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudAiplatformV1beta1GoogleSearchRetrieval googleSearchRetrieval;
/**
* Optional. Retrieval tool type. System will always execute the provided retrieval tool(s) to get
* external knowledge to answer the prompt. Retrieval results are presented to the model for
* generation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private GoogleCloudAiplatformV1beta1Retrieval retrieval;
/**
* Optional. CodeExecution tool type. Enables the model to execute code as part of generation.
* This field is only used by the Gemini Developer API services.
* @return value or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1ToolCodeExecution getCodeExecution() {
return codeExecution;
}
/**
* Optional. CodeExecution tool type. Enables the model to execute code as part of generation.
* This field is only used by the Gemini Developer API services.
* @param codeExecution codeExecution or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Tool setCodeExecution(GoogleCloudAiplatformV1beta1ToolCodeExecution codeExecution) {
this.codeExecution = codeExecution;
return this;
}
/**
* Optional. Function tool type. One or more function declarations to be passed to the model along
* with the current user query. Model may decide to call a subset of these functions by populating
* FunctionCall in the response. User should provide a FunctionResponse for each function call in
* the next turn. Based on the function responses, Model will generate the final response back to
* the user. Maximum 128 function declarations can be provided.
* @return value or {@code null} for none
*/
public java.util.List getFunctionDeclarations() {
return functionDeclarations;
}
/**
* Optional. Function tool type. One or more function declarations to be passed to the model along
* with the current user query. Model may decide to call a subset of these functions by populating
* FunctionCall in the response. User should provide a FunctionResponse for each function call in
* the next turn. Based on the function responses, Model will generate the final response back to
* the user. Maximum 128 function declarations can be provided.
* @param functionDeclarations functionDeclarations or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Tool setFunctionDeclarations(java.util.List functionDeclarations) {
this.functionDeclarations = functionDeclarations;
return this;
}
/**
* Optional. GoogleSearch tool type. Tool to support Google Search in Model. Powered by Google.
* @return value or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1ToolGoogleSearch getGoogleSearch() {
return googleSearch;
}
/**
* Optional. GoogleSearch tool type. Tool to support Google Search in Model. Powered by Google.
* @param googleSearch googleSearch or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Tool setGoogleSearch(GoogleCloudAiplatformV1beta1ToolGoogleSearch googleSearch) {
this.googleSearch = googleSearch;
return this;
}
/**
* Optional. GoogleSearchRetrieval tool type. Specialized retrieval tool that is powered by Google
* search.
* @return value or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1GoogleSearchRetrieval getGoogleSearchRetrieval() {
return googleSearchRetrieval;
}
/**
* Optional. GoogleSearchRetrieval tool type. Specialized retrieval tool that is powered by Google
* search.
* @param googleSearchRetrieval googleSearchRetrieval or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Tool setGoogleSearchRetrieval(GoogleCloudAiplatformV1beta1GoogleSearchRetrieval googleSearchRetrieval) {
this.googleSearchRetrieval = googleSearchRetrieval;
return this;
}
/**
* Optional. Retrieval tool type. System will always execute the provided retrieval tool(s) to get
* external knowledge to answer the prompt. Retrieval results are presented to the model for
* generation.
* @return value or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Retrieval getRetrieval() {
return retrieval;
}
/**
* Optional. Retrieval tool type. System will always execute the provided retrieval tool(s) to get
* external knowledge to answer the prompt. Retrieval results are presented to the model for
* generation.
* @param retrieval retrieval or {@code null} for none
*/
public GoogleCloudAiplatformV1beta1Tool setRetrieval(GoogleCloudAiplatformV1beta1Retrieval retrieval) {
this.retrieval = retrieval;
return this;
}
@Override
public GoogleCloudAiplatformV1beta1Tool set(String fieldName, Object value) {
return (GoogleCloudAiplatformV1beta1Tool) super.set(fieldName, value);
}
@Override
public GoogleCloudAiplatformV1beta1Tool clone() {
return (GoogleCloudAiplatformV1beta1Tool) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy