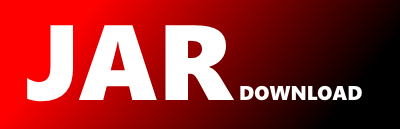
com.google.api.services.firebaserules.v1.FirebaseRulesAPI Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-09-12 at 23:11:01 UTC
* Modify at your own risk.
*/
package com.google.api.services.firebaserules.v1;
/**
* Service definition for FirebaseRulesAPI (v1).
*
*
* Creates and manages rules that determine when a Firebase Rules-enabled service should permit a request.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link FirebaseRulesAPIRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class FirebaseRulesAPI extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.22.0 of the Firebase Rules API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://firebaserules.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public FirebaseRulesAPI(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
FirebaseRulesAPI(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code FirebaseRulesAPI firebaserules = new FirebaseRulesAPI(...);}
* {@code FirebaseRulesAPI.Projects.List request = firebaserules.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* Test `Source` for syntactic and semantic correctness. Issues present in the rules, if any, will
* be returned to the caller with a description, severity, and source location.
*
* The test method will typically be executed with a developer provided `Source`, but if regression
* testing is desired, this method may be executed against a `Ruleset` resource name and the
* `Source` will be retrieved from the persisted `Ruleset`.
*
* The following is an example of `Source` that permits users to upload images to a bucket bearing
* their user id and matching the correct metadata:
*
* _*Example*_
*
* // Users are allowed to subscribe and unsubscribe to the blog. service firebase.storage {
* match /users/{userId}/images/{imageName} { allow write: if userId == request.userId
* && (imageName.endsWith('.png') || imageName.endsWith('.jpg')) &&
* resource.mimeType.startsWith('image/') } }
*
* Create a request for the method "projects.test".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link Test#execute()} method to invoke the remote operation.
*
* @param name Name of the project.
Format: `projects/{project_id}`
* @param content the {@link com.google.api.services.firebaserules.v1.model.TestRulesetRequest}
* @return the request
*/
public Test test(java.lang.String name, com.google.api.services.firebaserules.v1.model.TestRulesetRequest content) throws java.io.IOException {
Test result = new Test(name, content);
initialize(result);
return result;
}
public class Test extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}:test";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/.*$");
/**
* Test `Source` for syntactic and semantic correctness. Issues present in the rules, if any, will
* be returned to the caller with a description, severity, and source location.
*
* The test method will typically be executed with a developer provided `Source`, but if
* regression testing is desired, this method may be executed against a `Ruleset` resource name
* and the `Source` will be retrieved from the persisted `Ruleset`.
*
* The following is an example of `Source` that permits users to upload images to a bucket bearing
* their user id and matching the correct metadata:
*
* _*Example*_
*
* // Users are allowed to subscribe and unsubscribe to the blog. service firebase.storage
* { match /users/{userId}/images/{imageName} { allow write: if userId ==
* request.userId && (imageName.endsWith('.png') || imageName.endsWith('.jpg'))
* && resource.mimeType.startsWith('image/') } }
*
* Create a request for the method "projects.test".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link Test#execute()} method to invoke the remote operation.
* {@link Test#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Name of the project.
Format: `projects/{project_id}`
* @param content the {@link com.google.api.services.firebaserules.v1.model.TestRulesetRequest}
* @since 1.13
*/
protected Test(java.lang.String name, com.google.api.services.firebaserules.v1.model.TestRulesetRequest content) {
super(FirebaseRulesAPI.this, "POST", REST_PATH, content, com.google.api.services.firebaserules.v1.model.TestRulesetResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/.*$");
}
}
@Override
public Test set$Xgafv(java.lang.String $Xgafv) {
return (Test) super.set$Xgafv($Xgafv);
}
@Override
public Test setAccessToken(java.lang.String accessToken) {
return (Test) super.setAccessToken(accessToken);
}
@Override
public Test setAlt(java.lang.String alt) {
return (Test) super.setAlt(alt);
}
@Override
public Test setBearerToken(java.lang.String bearerToken) {
return (Test) super.setBearerToken(bearerToken);
}
@Override
public Test setCallback(java.lang.String callback) {
return (Test) super.setCallback(callback);
}
@Override
public Test setFields(java.lang.String fields) {
return (Test) super.setFields(fields);
}
@Override
public Test setKey(java.lang.String key) {
return (Test) super.setKey(key);
}
@Override
public Test setOauthToken(java.lang.String oauthToken) {
return (Test) super.setOauthToken(oauthToken);
}
@Override
public Test setPp(java.lang.Boolean pp) {
return (Test) super.setPp(pp);
}
@Override
public Test setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Test) super.setPrettyPrint(prettyPrint);
}
@Override
public Test setQuotaUser(java.lang.String quotaUser) {
return (Test) super.setQuotaUser(quotaUser);
}
@Override
public Test setUploadType(java.lang.String uploadType) {
return (Test) super.setUploadType(uploadType);
}
@Override
public Test setUploadProtocol(java.lang.String uploadProtocol) {
return (Test) super.setUploadProtocol(uploadProtocol);
}
/**
* Name of the project.
*
* Format: `projects/{project_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Name of the project.
Format: `projects/{project_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Name of the project.
*
* Format: `projects/{project_id}`
*/
public Test setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/.*$");
}
this.name = name;
return this;
}
@Override
public Test set(String parameterName, Object value) {
return (Test) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Releases collection.
*
* The typical use is:
*
* {@code FirebaseRulesAPI firebaserules = new FirebaseRulesAPI(...);}
* {@code FirebaseRulesAPI.Releases.List request = firebaserules.releases().list(parameters ...)}
*
*
* @return the resource collection
*/
public Releases releases() {
return new Releases();
}
/**
* The "releases" collection of methods.
*/
public class Releases {
/**
* Create a `Release`.
*
* Release names should reflect the developer's deployment practices. For example, the release name
* may include the environment name, application name, application version, or any other name
* meaningful to the developer. Once a `Release` refers to a `Ruleset`, the rules can be enforced by
* Firebase Rules-enabled services.
*
* More than one `Release` may be 'live' concurrently. Consider the following three `Release` names
* for `projects/foo` and the `Ruleset` to which they refer.
*
* Release Name | Ruleset Name --------------------------------|-------------
* projects/foo/releases/prod | projects/foo/rulesets/uuid123 projects/foo/releases/prod/beta |
* projects/foo/rulesets/uuid123 projects/foo/releases/prod/v23 | projects/foo/rulesets/uuid456
*
* The table reflects the `Ruleset` rollout in progress. The `prod` and `prod/beta` releases refer
* to the same `Ruleset`. However, `prod/v23` refers to a new `Ruleset`. The `Ruleset` reference for
* a `Release` may be updated using the UpdateRelease method, and the custom `Release` name may be
* referenced by specifying the `X-Firebase-Rules-Release-Name` header.
*
* Create a request for the method "releases.create".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param name Resource name for the project which owns this `Release`.
Format: `projects/{project_id}`
* @param content the {@link com.google.api.services.firebaserules.v1.model.Release}
* @return the request
*/
public Create create(java.lang.String name, com.google.api.services.firebaserules.v1.model.Release content) throws java.io.IOException {
Create result = new Create(name, content);
initialize(result);
return result;
}
public class Create extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}/releases";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]*$");
/**
* Create a `Release`.
*
* Release names should reflect the developer's deployment practices. For example, the release
* name may include the environment name, application name, application version, or any other name
* meaningful to the developer. Once a `Release` refers to a `Ruleset`, the rules can be enforced
* by Firebase Rules-enabled services.
*
* More than one `Release` may be 'live' concurrently. Consider the following three `Release`
* names for `projects/foo` and the `Ruleset` to which they refer.
*
* Release Name | Ruleset Name --------------------------------|-------------
* projects/foo/releases/prod | projects/foo/rulesets/uuid123 projects/foo/releases/prod/beta
* | projects/foo/rulesets/uuid123 projects/foo/releases/prod/v23 | projects/foo/rulesets/uuid456
*
* The table reflects the `Ruleset` rollout in progress. The `prod` and `prod/beta` releases refer
* to the same `Ruleset`. However, `prod/v23` refers to a new `Ruleset`. The `Ruleset` reference
* for a `Release` may be updated using the UpdateRelease method, and the custom `Release` name
* may be referenced by specifying the `X-Firebase-Rules-Release-Name` header.
*
* Create a request for the method "releases.create".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the project which owns this `Release`.
Format: `projects/{project_id}`
* @param content the {@link com.google.api.services.firebaserules.v1.model.Release}
* @since 1.13
*/
protected Create(java.lang.String name, com.google.api.services.firebaserules.v1.model.Release content) {
super(FirebaseRulesAPI.this, "POST", REST_PATH, content, com.google.api.services.firebaserules.v1.model.Release.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name for the project which owns this `Release`.
*
* Format: `projects/{project_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the project which owns this `Release`.
Format: `projects/{project_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name for the project which owns this `Release`.
*
* Format: `projects/{project_id}`
*/
public Create setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*$");
}
this.name = name;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Delete a `Release` by resource name.
*
* Create a request for the method "releases.delete".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Resource name for the `Release` to delete.
Format: `projects/{project_id}/releases/{release_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]*/releases/.*$");
/**
* Delete a `Release` by resource name.
*
* Create a request for the method "releases.delete".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the `Release` to delete.
Format: `projects/{project_id}/releases/{release_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(FirebaseRulesAPI.this, "DELETE", REST_PATH, null, com.google.api.services.firebaserules.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/releases/.*$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name for the `Release` to delete.
*
* Format: `projects/{project_id}/releases/{release_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the `Release` to delete.
Format: `projects/{project_id}/releases/{release_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name for the `Release` to delete.
*
* Format: `projects/{project_id}/releases/{release_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/releases/.*$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Get a `Release` by name.
*
* Create a request for the method "releases.get".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name of the `Release`.
Format: `projects/{project_id}/releases/{release_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]*/releases/.*$");
/**
* Get a `Release` by name.
*
* Create a request for the method "releases.get".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name of the `Release`.
Format: `projects/{project_id}/releases/{release_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(FirebaseRulesAPI.this, "GET", REST_PATH, null, com.google.api.services.firebaserules.v1.model.Release.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/releases/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name of the `Release`.
*
* Format: `projects/{project_id}/releases/{release_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name of the `Release`.
Format: `projects/{project_id}/releases/{release_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name of the `Release`.
*
* Format: `projects/{project_id}/releases/{release_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/releases/.*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List the `Release` values for a project. This list may optionally be filtered by `Release` name
* or `Ruleset` id or both.
*
* Create a request for the method "releases.list".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name Resource name for the project.
Format: `projects/{project_id}`
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}/releases";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]*$");
/**
* List the `Release` values for a project. This list may optionally be filtered by `Release` name
* or `Ruleset` id or both.
*
* Create a request for the method "releases.list".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the project.
Format: `projects/{project_id}`
* @since 1.13
*/
protected List(java.lang.String name) {
super(FirebaseRulesAPI.this, "GET", REST_PATH, null, com.google.api.services.firebaserules.v1.model.ListReleasesResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name for the project.
*
* Format: `projects/{project_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the project.
Format: `projects/{project_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name for the project.
*
* Format: `projects/{project_id}`
*/
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*$");
}
this.name = name;
return this;
}
/**
* Page size to load. Maximum of 100. Defaults to 10. Note: `page_size` is just a hint and
* the service may choose to load less than `page_size` due to the size of the output. To
* traverse all of the releases, caller should iterate until the `page_token` is empty.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Page size to load. Maximum of 100. Defaults to 10. Note: `page_size` is just a hint and the service
may choose to load less than `page_size` due to the size of the output. To traverse all of the
releases, caller should iterate until the `page_token` is empty.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Page size to load. Maximum of 100. Defaults to 10. Note: `page_size` is just a hint and
* the service may choose to load less than `page_size` due to the size of the output. To
* traverse all of the releases, caller should iterate until the `page_token` is empty.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* `Release` filter. The list method supports filters with restrictions on the `Release`
* `name` and also on the `Ruleset` `ruleset_name`.
*
* Example 1) A filter of 'name=prod*' might return `Release`s with names within
* 'projects/foo' prefixed with 'prod':
*
* Name | Ruleset Name ------------------------------|-------------
* projects/foo/releases/prod | projects/foo/rulesets/uuid1234
* projects/foo/releases/prod/v1 | projects/foo/rulesets/uuid1234
* projects/foo/releases/prod/v2 | projects/foo/rulesets/uuid8888
*
* Example 2) A filter of `name=prod* ruleset_name=uuid1234` would return only `Release`
* instances for 'projects/foo' with names prefixed with 'prod' referring to the same
* `Ruleset` name of 'uuid1234':
*
* Name | Ruleset Name ------------------------------|-------------
* projects/foo/releases/prod | projects/foo/rulesets/1234 projects/foo/releases/prod/v1
* | projects/foo/rulesets/1234
*
* In the examples, the filter parameters refer to the search filters for release and
* ruleset names are relative to the project releases and rulesets collections. Fully
* qualified prefixed may also be used. e.g. `name=projects/foo/releases/prod*
* ruleset_name=projects/foo/rulesets/uuid1`
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/**` Release` filter. The list method supports filters with restrictions on the Release` `name` and
` also on the `Ruleset` `ruleset_name`.
`
` Example 1) A filter of 'name=prod*' might return `Release`s with names within 'projects/foo'
` prefixed with 'prod':
`
` Name | Ruleset Name ------------------------------|-------------
` projects/foo/releases/prod | projects/foo/rulesets/uuid1234 projects/foo/releases/prod/v1 |
` projects/foo/rulesets/uuid1234 projects/foo/releases/prod/v2 | projects/foo/rulesets/uuid8888
`
` Example 2) A filter of `name=prod* ruleset_name=uuid1234` would return only Release` instances for
` 'projects/foo' with names prefixed with 'prod' referring to the same `Ruleset` name of 'uuid1234':
`
` Name | Ruleset Name ------------------------------|-------------
` projects/foo/releases/prod | projects/foo/rulesets/1234 projects/foo/releases/prod/v1 |
` projects/foo/rulesets/1234
`
` In the examples, the filter parameters refer to the search filters for release and ruleset names
` are relative to the project releases and rulesets collections. Fully qualified prefixed may also
` be used. e.g. name=projects/foo/releases/prod* ruleset_name=projects/foo/rulesets/uuid1`
`
*/
public java.lang.String getFilter() {
return filter;
}
/**
* `Release` filter. The list method supports filters with restrictions on the `Release`
* `name` and also on the `Ruleset` `ruleset_name`.
*
* Example 1) A filter of 'name=prod*' might return `Release`s with names within
* 'projects/foo' prefixed with 'prod':
*
* Name | Ruleset Name ------------------------------|-------------
* projects/foo/releases/prod | projects/foo/rulesets/uuid1234
* projects/foo/releases/prod/v1 | projects/foo/rulesets/uuid1234
* projects/foo/releases/prod/v2 | projects/foo/rulesets/uuid8888
*
* Example 2) A filter of `name=prod* ruleset_name=uuid1234` would return only `Release`
* instances for 'projects/foo' with names prefixed with 'prod' referring to the same
* `Ruleset` name of 'uuid1234':
*
* Name | Ruleset Name ------------------------------|-------------
* projects/foo/releases/prod | projects/foo/rulesets/1234 projects/foo/releases/prod/v1
* | projects/foo/rulesets/1234
*
* In the examples, the filter parameters refer to the search filters for release and
* ruleset names are relative to the project releases and rulesets collections. Fully
* qualified prefixed may also be used. e.g. `name=projects/foo/releases/prod*
* ruleset_name=projects/foo/rulesets/uuid1`
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/** Next page token for the next batch of `Release` instances. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Next page token for the next batch of `Release` instances.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Next page token for the next batch of `Release` instances. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Update a `Release`.
*
* Only updates to the `ruleset_name` field will be honored. `Release` rename is not supported. To
* create a `Release` use the CreateRelease method instead.
*
* Create a request for the method "releases.update".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param name Resource name for the `Release`.
`Release` names may be structured `app1/prod/v2` or flat
* `app1_prod_v2`
which affords developers a great deal of flexibility in mapping the name
to
* the style that best fits their existing development practices. For
example, a name could
* refer to an environment, an app, a version, or some
combination of three.
In the table
* below, for the project name `projects/foo`, the following
relative release paths show how
* flat and structured names might be chosen
to match a desired development / deployment
* strategy.
Use Case | Flat Name | Structured Name
* -------------|---------------------|----------------
Environments | releases/qa |
* releases/qa
Apps | releases/app1_qa | releases/app1/qa
Versions |
* releases/app1_v2_qa | releases/app1/v2/qa
The delimiter between the release name path
* elements can be almost anything
and it should work equally well with the release name list
* filter, but in
many ways the structured paths provide a clearer picture of the
* relationship between `Release` instances.
Format:
* `projects/{project_id}/releases/{release_id}`
* @param content the {@link com.google.api.services.firebaserules.v1.model.Release}
* @return the request
*/
public Update update(java.lang.String name, com.google.api.services.firebaserules.v1.model.Release content) throws java.io.IOException {
Update result = new Update(name, content);
initialize(result);
return result;
}
public class Update extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]*/releases/.*$");
/**
* Update a `Release`.
*
* Only updates to the `ruleset_name` field will be honored. `Release` rename is not supported. To
* create a `Release` use the CreateRelease method instead.
*
* Create a request for the method "releases.update".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the `Release`.
`Release` names may be structured `app1/prod/v2` or flat
* `app1_prod_v2`
which affords developers a great deal of flexibility in mapping the name
to
* the style that best fits their existing development practices. For
example, a name could
* refer to an environment, an app, a version, or some
combination of three.
In the table
* below, for the project name `projects/foo`, the following
relative release paths show how
* flat and structured names might be chosen
to match a desired development / deployment
* strategy.
Use Case | Flat Name | Structured Name
* -------------|---------------------|----------------
Environments | releases/qa |
* releases/qa
Apps | releases/app1_qa | releases/app1/qa
Versions |
* releases/app1_v2_qa | releases/app1/v2/qa
The delimiter between the release name path
* elements can be almost anything
and it should work equally well with the release name list
* filter, but in
many ways the structured paths provide a clearer picture of the
* relationship between `Release` instances.
Format:
* `projects/{project_id}/releases/{release_id}`
* @param content the {@link com.google.api.services.firebaserules.v1.model.Release}
* @since 1.13
*/
protected Update(java.lang.String name, com.google.api.services.firebaserules.v1.model.Release content) {
super(FirebaseRulesAPI.this, "PUT", REST_PATH, content, com.google.api.services.firebaserules.v1.model.Release.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/releases/.*$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setBearerToken(java.lang.String bearerToken) {
return (Update) super.setBearerToken(bearerToken);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPp(java.lang.Boolean pp) {
return (Update) super.setPp(pp);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name for the `Release`.
*
* `Release` names may be structured `app1/prod/v2` or flat `app1_prod_v2` which affords
* developers a great deal of flexibility in mapping the name to the style that best fits
* their existing development practices. For example, a name could refer to an environment,
* an app, a version, or some combination of three.
*
* In the table below, for the project name `projects/foo`, the following relative release
* paths show how flat and structured names might be chosen to match a desired development /
* deployment strategy.
*
* Use Case | Flat Name | Structured Name
* -------------|---------------------|---------------- Environments | releases/qa |
* releases/qa Apps | releases/app1_qa | releases/app1/qa Versions |
* releases/app1_v2_qa | releases/app1/v2/qa
*
* The delimiter between the release name path elements can be almost anything and it should
* work equally well with the release name list filter, but in many ways the structured
* paths provide a clearer picture of the relationship between `Release` instances.
*
* Format: `projects/{project_id}/releases/{release_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the `Release`.
`Release` names may be structured `app1/prod/v2` or flat `app1_prod_v2` which affords developers a
great deal of flexibility in mapping the name to the style that best fits their existing
development practices. For example, a name could refer to an environment, an app, a version, or
some combination of three.
In the table below, for the project name `projects/foo`, the following relative release paths show
how flat and structured names might be chosen to match a desired development / deployment strategy.
Use Case | Flat Name | Structured Name
-------------|---------------------|---------------- Environments | releases/qa |
releases/qa Apps | releases/app1_qa | releases/app1/qa Versions |
releases/app1_v2_qa | releases/app1/v2/qa
The delimiter between the release name path elements can be almost anything and it should work
equally well with the release name list filter, but in many ways the structured paths provide a
clearer picture of the relationship between `Release` instances.
Format: `projects/{project_id}/releases/{release_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name for the `Release`.
*
* `Release` names may be structured `app1/prod/v2` or flat `app1_prod_v2` which affords
* developers a great deal of flexibility in mapping the name to the style that best fits
* their existing development practices. For example, a name could refer to an environment,
* an app, a version, or some combination of three.
*
* In the table below, for the project name `projects/foo`, the following relative release
* paths show how flat and structured names might be chosen to match a desired development /
* deployment strategy.
*
* Use Case | Flat Name | Structured Name
* -------------|---------------------|---------------- Environments | releases/qa |
* releases/qa Apps | releases/app1_qa | releases/app1/qa Versions |
* releases/app1_v2_qa | releases/app1/v2/qa
*
* The delimiter between the release name path elements can be almost anything and it should
* work equally well with the release name list filter, but in many ways the structured
* paths provide a clearer picture of the relationship between `Release` instances.
*
* Format: `projects/{project_id}/releases/{release_id}`
*/
public Update setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/releases/.*$");
}
this.name = name;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Rulesets collection.
*
* The typical use is:
*
* {@code FirebaseRulesAPI firebaserules = new FirebaseRulesAPI(...);}
* {@code FirebaseRulesAPI.Rulesets.List request = firebaserules.rulesets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Rulesets rulesets() {
return new Rulesets();
}
/**
* The "rulesets" collection of methods.
*/
public class Rulesets {
/**
* Create a `Ruleset` from `Source`.
*
* The `Ruleset` is given a unique generated name which is returned to the caller. `Source`
* containing syntactic or semantics errors will result in an error response indicating the first
* error encountered. For a detailed view of `Source` issues, use TestRuleset.
*
* Create a request for the method "rulesets.create".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param name Resource name for Project which owns this `Ruleset`.
Format: `projects/{project_id}`
* @param content the {@link com.google.api.services.firebaserules.v1.model.Ruleset}
* @return the request
*/
public Create create(java.lang.String name, com.google.api.services.firebaserules.v1.model.Ruleset content) throws java.io.IOException {
Create result = new Create(name, content);
initialize(result);
return result;
}
public class Create extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}/rulesets";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]*$");
/**
* Create a `Ruleset` from `Source`.
*
* The `Ruleset` is given a unique generated name which is returned to the caller. `Source`
* containing syntactic or semantics errors will result in an error response indicating the first
* error encountered. For a detailed view of `Source` issues, use TestRuleset.
*
* Create a request for the method "rulesets.create".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for Project which owns this `Ruleset`.
Format: `projects/{project_id}`
* @param content the {@link com.google.api.services.firebaserules.v1.model.Ruleset}
* @since 1.13
*/
protected Create(java.lang.String name, com.google.api.services.firebaserules.v1.model.Ruleset content) {
super(FirebaseRulesAPI.this, "POST", REST_PATH, content, com.google.api.services.firebaserules.v1.model.Ruleset.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name for Project which owns this `Ruleset`.
*
* Format: `projects/{project_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for Project which owns this `Ruleset`.
Format: `projects/{project_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name for Project which owns this `Ruleset`.
*
* Format: `projects/{project_id}`
*/
public Create setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*$");
}
this.name = name;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Delete a `Ruleset` by resource name.
*
* If the `Ruleset` is referenced by a `Release` the operation will fail.
*
* Create a request for the method "rulesets.delete".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Resource name for the ruleset to delete.
Format: `projects/{project_id}/rulesets/{ruleset_id}`
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]*/rulesets/[^/]*$");
/**
* Delete a `Ruleset` by resource name.
*
* If the `Ruleset` is referenced by a `Release` the operation will fail.
*
* Create a request for the method "rulesets.delete".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the ruleset to delete.
Format: `projects/{project_id}/rulesets/{ruleset_id}`
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(FirebaseRulesAPI.this, "DELETE", REST_PATH, null, com.google.api.services.firebaserules.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/rulesets/[^/]*$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name for the ruleset to delete.
*
* Format: `projects/{project_id}/rulesets/{ruleset_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the ruleset to delete.
Format: `projects/{project_id}/rulesets/{ruleset_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name for the ruleset to delete.
*
* Format: `projects/{project_id}/rulesets/{ruleset_id}`
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/rulesets/[^/]*$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Get a `Ruleset` by name including the full `Source` contents.
*
* Create a request for the method "rulesets.get".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Resource name for the ruleset to get.
Format: `projects/{project_id}/rulesets/{ruleset_id}`
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]*/rulesets/[^/]*$");
/**
* Get a `Ruleset` by name including the full `Source` contents.
*
* Create a request for the method "rulesets.get".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the ruleset to get.
Format: `projects/{project_id}/rulesets/{ruleset_id}`
* @since 1.13
*/
protected Get(java.lang.String name) {
super(FirebaseRulesAPI.this, "GET", REST_PATH, null, com.google.api.services.firebaserules.v1.model.Ruleset.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/rulesets/[^/]*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name for the ruleset to get.
*
* Format: `projects/{project_id}/rulesets/{ruleset_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the ruleset to get.
Format: `projects/{project_id}/rulesets/{ruleset_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name for the ruleset to get.
*
* Format: `projects/{project_id}/rulesets/{ruleset_id}`
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*/rulesets/[^/]*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* List `Ruleset` metadata only and optionally filter the results by Ruleset name.
*
* The full `Source` contents of a `Ruleset` may be retrieved with GetRuleset.
*
* Create a request for the method "rulesets.list".
*
* This request holds the parameters needed by the firebaserules server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name Resource name for the project.
Format: `projects/{project_id}`
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends FirebaseRulesAPIRequest {
private static final String REST_PATH = "v1/{+name}/rulesets";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]*$");
/**
* List `Ruleset` metadata only and optionally filter the results by Ruleset name.
*
* The full `Source` contents of a `Ruleset` may be retrieved with GetRuleset.
*
* Create a request for the method "rulesets.list".
*
* This request holds the parameters needed by the the firebaserules server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Resource name for the project.
Format: `projects/{project_id}`
* @since 1.13
*/
protected List(java.lang.String name) {
super(FirebaseRulesAPI.this, "GET", REST_PATH, null, com.google.api.services.firebaserules.v1.model.ListRulesetsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Resource name for the project.
*
* Format: `projects/{project_id}`
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Resource name for the project.
Format: `projects/{project_id}`
*/
public java.lang.String getName() {
return name;
}
/**
* Resource name for the project.
*
* Format: `projects/{project_id}`
*/
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]*$");
}
this.name = name;
return this;
}
/**
* Page size to load. Maximum of 100. Defaults to 10. Note: `page_size` is just a hint and
* the service may choose to load less than `page_size` due to the size of the output. To
* traverse all of the releases, caller should iterate until the `page_token` is empty.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** Page size to load. Maximum of 100. Defaults to 10. Note: `page_size` is just a hint and the service
may choose to load less than `page_size` due to the size of the output. To traverse all of the
releases, caller should iterate until the `page_token` is empty.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* Page size to load. Maximum of 100. Defaults to 10. Note: `page_size` is just a hint and
* the service may choose to load less than `page_size` due to the size of the output. To
* traverse all of the releases, caller should iterate until the `page_token` is empty.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** Next page token for loading the next batch of `Ruleset` instances. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Next page token for loading the next batch of `Ruleset` instances.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Next page token for loading the next batch of `Ruleset` instances. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link FirebaseRulesAPI}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link FirebaseRulesAPI}. */
@Override
public FirebaseRulesAPI build() {
return new FirebaseRulesAPI(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link FirebaseRulesAPIRequestInitializer}.
*
* @since 1.12
*/
public Builder setFirebaseRulesAPIRequestInitializer(
FirebaseRulesAPIRequestInitializer firebaserulesapiRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(firebaserulesapiRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}