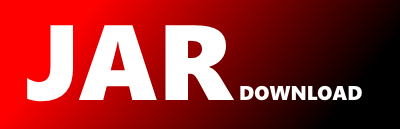
com.google.api.services.games.model.RoomParticipant Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2016-01-08 17:48:37 UTC)
* on 2016-02-09 at 02:21:42 UTC
* Modify at your own risk.
*/
package com.google.api.services.games.model;
/**
* This is a JSON template for a participant in a room.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Play Game Services API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class RoomParticipant extends com.google.api.client.json.GenericJson {
/**
* True if this participant was auto-matched with the requesting player.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean autoMatched;
/**
* Information about a player that has been anonymously auto-matched against the requesting
* player. (Either player or autoMatchedPlayer will be set.)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private AnonymousPlayer autoMatchedPlayer;
/**
* The capabilities which can be used when communicating with this participant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List capabilities;
/**
* Client address for the participant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RoomClientAddress clientAddress;
/**
* True if this participant is in the fully connected set of peers in the room.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean connected;
/**
* An identifier for the participant in the scope of the room. Cannot be used to identify a player
* across rooms or in other contexts.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* Uniquely identifies the type of this resource. Value is always the fixed string
* games#roomParticipant.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The reason the participant left the room; populated if the participant status is
* PARTICIPANT_LEFT. Possible values are: - "PLAYER_LEFT" - The player explicitly chose to leave
* the room. - "GAME_LEFT" - The game chose to remove the player from the room. - "ABANDONED" -
* The player switched to another application and abandoned the room. - "PEER_CONNECTION_FAILURE"
* - The client was unable to establish or maintain a connection to other peer(s) in the room. -
* "SERVER_ERROR" - The client received an error response when it tried to communicate with the
* server. - "TIMEOUT" - The client timed out while waiting for players to join and connect. -
* "PRESENCE_FAILURE" - The client's XMPP connection ended abruptly.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String leaveReason;
/**
* Information about the player. Not populated if this player was anonymously auto-matched against
* the requesting player. (Either player or autoMatchedPlayer will be set.)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Player player;
/**
* The status of the participant with respect to the room. Possible values are: -
* "PARTICIPANT_INVITED" - The participant has been invited to join the room, but has not yet
* responded. - "PARTICIPANT_JOINED" - The participant has joined the room (either after creating
* it or accepting an invitation.) - "PARTICIPANT_DECLINED" - The participant declined an
* invitation to join the room. - "PARTICIPANT_LEFT" - The participant joined the room and then
* left it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String status;
/**
* True if this participant was auto-matched with the requesting player.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAutoMatched() {
return autoMatched;
}
/**
* True if this participant was auto-matched with the requesting player.
* @param autoMatched autoMatched or {@code null} for none
*/
public RoomParticipant setAutoMatched(java.lang.Boolean autoMatched) {
this.autoMatched = autoMatched;
return this;
}
/**
* Information about a player that has been anonymously auto-matched against the requesting
* player. (Either player or autoMatchedPlayer will be set.)
* @return value or {@code null} for none
*/
public AnonymousPlayer getAutoMatchedPlayer() {
return autoMatchedPlayer;
}
/**
* Information about a player that has been anonymously auto-matched against the requesting
* player. (Either player or autoMatchedPlayer will be set.)
* @param autoMatchedPlayer autoMatchedPlayer or {@code null} for none
*/
public RoomParticipant setAutoMatchedPlayer(AnonymousPlayer autoMatchedPlayer) {
this.autoMatchedPlayer = autoMatchedPlayer;
return this;
}
/**
* The capabilities which can be used when communicating with this participant.
* @return value or {@code null} for none
*/
public java.util.List getCapabilities() {
return capabilities;
}
/**
* The capabilities which can be used when communicating with this participant.
* @param capabilities capabilities or {@code null} for none
*/
public RoomParticipant setCapabilities(java.util.List capabilities) {
this.capabilities = capabilities;
return this;
}
/**
* Client address for the participant.
* @return value or {@code null} for none
*/
public RoomClientAddress getClientAddress() {
return clientAddress;
}
/**
* Client address for the participant.
* @param clientAddress clientAddress or {@code null} for none
*/
public RoomParticipant setClientAddress(RoomClientAddress clientAddress) {
this.clientAddress = clientAddress;
return this;
}
/**
* True if this participant is in the fully connected set of peers in the room.
* @return value or {@code null} for none
*/
public java.lang.Boolean getConnected() {
return connected;
}
/**
* True if this participant is in the fully connected set of peers in the room.
* @param connected connected or {@code null} for none
*/
public RoomParticipant setConnected(java.lang.Boolean connected) {
this.connected = connected;
return this;
}
/**
* An identifier for the participant in the scope of the room. Cannot be used to identify a player
* across rooms or in other contexts.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* An identifier for the participant in the scope of the room. Cannot be used to identify a player
* across rooms or in other contexts.
* @param id id or {@code null} for none
*/
public RoomParticipant setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* Uniquely identifies the type of this resource. Value is always the fixed string
* games#roomParticipant.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Uniquely identifies the type of this resource. Value is always the fixed string
* games#roomParticipant.
* @param kind kind or {@code null} for none
*/
public RoomParticipant setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The reason the participant left the room; populated if the participant status is
* PARTICIPANT_LEFT. Possible values are: - "PLAYER_LEFT" - The player explicitly chose to leave
* the room. - "GAME_LEFT" - The game chose to remove the player from the room. - "ABANDONED" -
* The player switched to another application and abandoned the room. - "PEER_CONNECTION_FAILURE"
* - The client was unable to establish or maintain a connection to other peer(s) in the room. -
* "SERVER_ERROR" - The client received an error response when it tried to communicate with the
* server. - "TIMEOUT" - The client timed out while waiting for players to join and connect. -
* "PRESENCE_FAILURE" - The client's XMPP connection ended abruptly.
* @return value or {@code null} for none
*/
public java.lang.String getLeaveReason() {
return leaveReason;
}
/**
* The reason the participant left the room; populated if the participant status is
* PARTICIPANT_LEFT. Possible values are: - "PLAYER_LEFT" - The player explicitly chose to leave
* the room. - "GAME_LEFT" - The game chose to remove the player from the room. - "ABANDONED" -
* The player switched to another application and abandoned the room. - "PEER_CONNECTION_FAILURE"
* - The client was unable to establish or maintain a connection to other peer(s) in the room. -
* "SERVER_ERROR" - The client received an error response when it tried to communicate with the
* server. - "TIMEOUT" - The client timed out while waiting for players to join and connect. -
* "PRESENCE_FAILURE" - The client's XMPP connection ended abruptly.
* @param leaveReason leaveReason or {@code null} for none
*/
public RoomParticipant setLeaveReason(java.lang.String leaveReason) {
this.leaveReason = leaveReason;
return this;
}
/**
* Information about the player. Not populated if this player was anonymously auto-matched against
* the requesting player. (Either player or autoMatchedPlayer will be set.)
* @return value or {@code null} for none
*/
public Player getPlayer() {
return player;
}
/**
* Information about the player. Not populated if this player was anonymously auto-matched against
* the requesting player. (Either player or autoMatchedPlayer will be set.)
* @param player player or {@code null} for none
*/
public RoomParticipant setPlayer(Player player) {
this.player = player;
return this;
}
/**
* The status of the participant with respect to the room. Possible values are: -
* "PARTICIPANT_INVITED" - The participant has been invited to join the room, but has not yet
* responded. - "PARTICIPANT_JOINED" - The participant has joined the room (either after creating
* it or accepting an invitation.) - "PARTICIPANT_DECLINED" - The participant declined an
* invitation to join the room. - "PARTICIPANT_LEFT" - The participant joined the room and then
* left it.
* @return value or {@code null} for none
*/
public java.lang.String getStatus() {
return status;
}
/**
* The status of the participant with respect to the room. Possible values are: -
* "PARTICIPANT_INVITED" - The participant has been invited to join the room, but has not yet
* responded. - "PARTICIPANT_JOINED" - The participant has joined the room (either after creating
* it or accepting an invitation.) - "PARTICIPANT_DECLINED" - The participant declined an
* invitation to join the room. - "PARTICIPANT_LEFT" - The participant joined the room and then
* left it.
* @param status status or {@code null} for none
*/
public RoomParticipant setStatus(java.lang.String status) {
this.status = status;
return this;
}
@Override
public RoomParticipant set(String fieldName, Object value) {
return (RoomParticipant) super.set(fieldName, value);
}
@Override
public RoomParticipant clone() {
return (RoomParticipant) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy