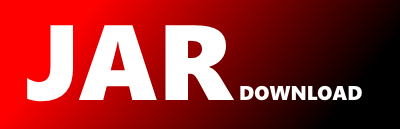
com.google.api.services.games.Games Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-01-14 17:53:03 UTC)
* on 2015-03-12 at 21:37:46 UTC
* Modify at your own risk.
*/
package com.google.api.services.games;
/**
* Service definition for Games (v1).
*
*
* The API for Google Play Game Services.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link GamesRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Games extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.18.0-rc of the Google Play Game Services API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "games/v1/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Games(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Games(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the AchievementDefinitions collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.AchievementDefinitions.List request = games.achievementDefinitions().list(parameters ...)}
*
*
* @return the resource collection
*/
public AchievementDefinitions achievementDefinitions() {
return new AchievementDefinitions();
}
/**
* The "achievementDefinitions" collection of methods.
*/
public class AchievementDefinitions {
/**
* Lists all the achievement definitions for your application.
*
* Create a request for the method "achievementDefinitions.list".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends GamesRequest {
private static final String REST_PATH = "achievements";
/**
* Lists all the achievement definitions for your application.
*
* Create a request for the method "achievementDefinitions.list".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.AchievementDefinitionsListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of achievement resources to return in the response, used for paging. For
* any response, the actual number of achievement resources returned may be less than the
* specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of achievement resources to return in the response, used for paging. For any
response, the actual number of achievement resources returned may be less than the specified
maxResults.
[minimum: 1] [maximum: 200]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of achievement resources to return in the response, used for paging. For
* any response, the actual number of achievement resources returned may be less than the
* specified maxResults.
*/
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Achievements collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Achievements.List request = games.achievements().list(parameters ...)}
*
*
* @return the resource collection
*/
public Achievements achievements() {
return new Achievements();
}
/**
* The "achievements" collection of methods.
*/
public class Achievements {
/**
* Increments the steps of the achievement with the given ID for the currently authenticated player.
*
* Create a request for the method "achievements.increment".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Increment#execute()} method to invoke the remote operation.
*
* @param achievementId The ID of the achievement used by this method.
* @param stepsToIncrement The number of steps to increment.
[minimum: 1]
* @return the request
*/
public Increment increment(java.lang.String achievementId, java.lang.Integer stepsToIncrement) throws java.io.IOException {
Increment result = new Increment(achievementId, stepsToIncrement);
initialize(result);
return result;
}
public class Increment extends GamesRequest {
private static final String REST_PATH = "achievements/{achievementId}/increment";
/**
* Increments the steps of the achievement with the given ID for the currently authenticated
* player.
*
* Create a request for the method "achievements.increment".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Increment#execute()} method to invoke the remote operation.
* {@link
* Increment#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param achievementId The ID of the achievement used by this method.
* @param stepsToIncrement The number of steps to increment.
[minimum: 1]
* @since 1.13
*/
protected Increment(java.lang.String achievementId, java.lang.Integer stepsToIncrement) {
super(Games.this, "POST", REST_PATH, null, com.google.api.services.games.model.AchievementIncrementResponse.class);
this.achievementId = com.google.api.client.util.Preconditions.checkNotNull(achievementId, "Required parameter achievementId must be specified.");
this.stepsToIncrement = com.google.api.client.util.Preconditions.checkNotNull(stepsToIncrement, "Required parameter stepsToIncrement must be specified.");
}
@Override
public Increment setAlt(java.lang.String alt) {
return (Increment) super.setAlt(alt);
}
@Override
public Increment setFields(java.lang.String fields) {
return (Increment) super.setFields(fields);
}
@Override
public Increment setKey(java.lang.String key) {
return (Increment) super.setKey(key);
}
@Override
public Increment setOauthToken(java.lang.String oauthToken) {
return (Increment) super.setOauthToken(oauthToken);
}
@Override
public Increment setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Increment) super.setPrettyPrint(prettyPrint);
}
@Override
public Increment setQuotaUser(java.lang.String quotaUser) {
return (Increment) super.setQuotaUser(quotaUser);
}
@Override
public Increment setUserIp(java.lang.String userIp) {
return (Increment) super.setUserIp(userIp);
}
/** The ID of the achievement used by this method. */
@com.google.api.client.util.Key
private java.lang.String achievementId;
/** The ID of the achievement used by this method.
*/
public java.lang.String getAchievementId() {
return achievementId;
}
/** The ID of the achievement used by this method. */
public Increment setAchievementId(java.lang.String achievementId) {
this.achievementId = achievementId;
return this;
}
/** The number of steps to increment. */
@com.google.api.client.util.Key
private java.lang.Integer stepsToIncrement;
/** The number of steps to increment.
[minimum: 1]
*/
public java.lang.Integer getStepsToIncrement() {
return stepsToIncrement;
}
/** The number of steps to increment. */
public Increment setStepsToIncrement(java.lang.Integer stepsToIncrement) {
this.stepsToIncrement = stepsToIncrement;
return this;
}
/**
* A randomly generated numeric ID for each request specified by the caller. This number is
* used at the server to ensure that the request is handled correctly across retries.
*/
@com.google.api.client.util.Key
private java.lang.Long requestId;
/** A randomly generated numeric ID for each request specified by the caller. This number is used at
the server to ensure that the request is handled correctly across retries.
*/
public java.lang.Long getRequestId() {
return requestId;
}
/**
* A randomly generated numeric ID for each request specified by the caller. This number is
* used at the server to ensure that the request is handled correctly across retries.
*/
public Increment setRequestId(java.lang.Long requestId) {
this.requestId = requestId;
return this;
}
@Override
public Increment set(String parameterName, Object value) {
return (Increment) super.set(parameterName, value);
}
}
/**
* Lists the progress for all your application's achievements for the currently authenticated
* player.
*
* Create a request for the method "achievements.list".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @return the request
*/
public List list(java.lang.String playerId) throws java.io.IOException {
List result = new List(playerId);
initialize(result);
return result;
}
public class List extends GamesRequest {
private static final String REST_PATH = "players/{playerId}/achievements";
/**
* Lists the progress for all your application's achievements for the currently authenticated
* player.
*
* Create a request for the method "achievements.list".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @since 1.13
*/
protected List(java.lang.String playerId) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.PlayerAchievementListResponse.class);
this.playerId = com.google.api.client.util.Preconditions.checkNotNull(playerId, "Required parameter playerId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
@com.google.api.client.util.Key
private java.lang.String playerId;
/** A player ID. A value of me may be used in place of the authenticated player's ID.
*/
public java.lang.String getPlayerId() {
return playerId;
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
public List setPlayerId(java.lang.String playerId) {
this.playerId = playerId;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Tells the server to return only achievements with the specified state. If this parameter
* isn't specified, all achievements are returned.
*/
@com.google.api.client.util.Key
private java.lang.String state;
/** Tells the server to return only achievements with the specified state. If this parameter isn't
specified, all achievements are returned.
*/
public java.lang.String getState() {
return state;
}
/**
* Tells the server to return only achievements with the specified state. If this parameter
* isn't specified, all achievements are returned.
*/
public List setState(java.lang.String state) {
this.state = state;
return this;
}
/**
* The maximum number of achievement resources to return in the response, used for paging. For
* any response, the actual number of achievement resources returned may be less than the
* specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of achievement resources to return in the response, used for paging. For any
response, the actual number of achievement resources returned may be less than the specified
maxResults.
[minimum: 1] [maximum: 200]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of achievement resources to return in the response, used for paging. For
* any response, the actual number of achievement resources returned may be less than the
* specified maxResults.
*/
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sets the state of the achievement with the given ID to REVEALED for the currently authenticated
* player.
*
* Create a request for the method "achievements.reveal".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Reveal#execute()} method to invoke the remote operation.
*
* @param achievementId The ID of the achievement used by this method.
* @return the request
*/
public Reveal reveal(java.lang.String achievementId) throws java.io.IOException {
Reveal result = new Reveal(achievementId);
initialize(result);
return result;
}
public class Reveal extends GamesRequest {
private static final String REST_PATH = "achievements/{achievementId}/reveal";
/**
* Sets the state of the achievement with the given ID to REVEALED for the currently authenticated
* player.
*
* Create a request for the method "achievements.reveal".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Reveal#execute()} method to invoke the remote operation. {@link
* Reveal#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param achievementId The ID of the achievement used by this method.
* @since 1.13
*/
protected Reveal(java.lang.String achievementId) {
super(Games.this, "POST", REST_PATH, null, com.google.api.services.games.model.AchievementRevealResponse.class);
this.achievementId = com.google.api.client.util.Preconditions.checkNotNull(achievementId, "Required parameter achievementId must be specified.");
}
@Override
public Reveal setAlt(java.lang.String alt) {
return (Reveal) super.setAlt(alt);
}
@Override
public Reveal setFields(java.lang.String fields) {
return (Reveal) super.setFields(fields);
}
@Override
public Reveal setKey(java.lang.String key) {
return (Reveal) super.setKey(key);
}
@Override
public Reveal setOauthToken(java.lang.String oauthToken) {
return (Reveal) super.setOauthToken(oauthToken);
}
@Override
public Reveal setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Reveal) super.setPrettyPrint(prettyPrint);
}
@Override
public Reveal setQuotaUser(java.lang.String quotaUser) {
return (Reveal) super.setQuotaUser(quotaUser);
}
@Override
public Reveal setUserIp(java.lang.String userIp) {
return (Reveal) super.setUserIp(userIp);
}
/** The ID of the achievement used by this method. */
@com.google.api.client.util.Key
private java.lang.String achievementId;
/** The ID of the achievement used by this method.
*/
public java.lang.String getAchievementId() {
return achievementId;
}
/** The ID of the achievement used by this method. */
public Reveal setAchievementId(java.lang.String achievementId) {
this.achievementId = achievementId;
return this;
}
@Override
public Reveal set(String parameterName, Object value) {
return (Reveal) super.set(parameterName, value);
}
}
/**
* Sets the steps for the currently authenticated player towards unlocking an achievement. If the
* steps parameter is less than the current number of steps that the player already gained for the
* achievement, the achievement is not modified.
*
* Create a request for the method "achievements.setStepsAtLeast".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link SetStepsAtLeast#execute()} method to invoke the remote operation.
*
* @param achievementId The ID of the achievement used by this method.
* @param steps The minimum value to set the steps to.
[minimum: 1]
* @return the request
*/
public SetStepsAtLeast setStepsAtLeast(java.lang.String achievementId, java.lang.Integer steps) throws java.io.IOException {
SetStepsAtLeast result = new SetStepsAtLeast(achievementId, steps);
initialize(result);
return result;
}
public class SetStepsAtLeast extends GamesRequest {
private static final String REST_PATH = "achievements/{achievementId}/setStepsAtLeast";
/**
* Sets the steps for the currently authenticated player towards unlocking an achievement. If the
* steps parameter is less than the current number of steps that the player already gained for the
* achievement, the achievement is not modified.
*
* Create a request for the method "achievements.setStepsAtLeast".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link SetStepsAtLeast#execute()} method to invoke the remote operation.
* {@link SetStepsAtLeast#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param achievementId The ID of the achievement used by this method.
* @param steps The minimum value to set the steps to.
[minimum: 1]
* @since 1.13
*/
protected SetStepsAtLeast(java.lang.String achievementId, java.lang.Integer steps) {
super(Games.this, "POST", REST_PATH, null, com.google.api.services.games.model.AchievementSetStepsAtLeastResponse.class);
this.achievementId = com.google.api.client.util.Preconditions.checkNotNull(achievementId, "Required parameter achievementId must be specified.");
this.steps = com.google.api.client.util.Preconditions.checkNotNull(steps, "Required parameter steps must be specified.");
}
@Override
public SetStepsAtLeast setAlt(java.lang.String alt) {
return (SetStepsAtLeast) super.setAlt(alt);
}
@Override
public SetStepsAtLeast setFields(java.lang.String fields) {
return (SetStepsAtLeast) super.setFields(fields);
}
@Override
public SetStepsAtLeast setKey(java.lang.String key) {
return (SetStepsAtLeast) super.setKey(key);
}
@Override
public SetStepsAtLeast setOauthToken(java.lang.String oauthToken) {
return (SetStepsAtLeast) super.setOauthToken(oauthToken);
}
@Override
public SetStepsAtLeast setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetStepsAtLeast) super.setPrettyPrint(prettyPrint);
}
@Override
public SetStepsAtLeast setQuotaUser(java.lang.String quotaUser) {
return (SetStepsAtLeast) super.setQuotaUser(quotaUser);
}
@Override
public SetStepsAtLeast setUserIp(java.lang.String userIp) {
return (SetStepsAtLeast) super.setUserIp(userIp);
}
/** The ID of the achievement used by this method. */
@com.google.api.client.util.Key
private java.lang.String achievementId;
/** The ID of the achievement used by this method.
*/
public java.lang.String getAchievementId() {
return achievementId;
}
/** The ID of the achievement used by this method. */
public SetStepsAtLeast setAchievementId(java.lang.String achievementId) {
this.achievementId = achievementId;
return this;
}
/** The minimum value to set the steps to. */
@com.google.api.client.util.Key
private java.lang.Integer steps;
/** The minimum value to set the steps to.
[minimum: 1]
*/
public java.lang.Integer getSteps() {
return steps;
}
/** The minimum value to set the steps to. */
public SetStepsAtLeast setSteps(java.lang.Integer steps) {
this.steps = steps;
return this;
}
@Override
public SetStepsAtLeast set(String parameterName, Object value) {
return (SetStepsAtLeast) super.set(parameterName, value);
}
}
/**
* Unlocks this achievement for the currently authenticated player.
*
* Create a request for the method "achievements.unlock".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Unlock#execute()} method to invoke the remote operation.
*
* @param achievementId The ID of the achievement used by this method.
* @return the request
*/
public Unlock unlock(java.lang.String achievementId) throws java.io.IOException {
Unlock result = new Unlock(achievementId);
initialize(result);
return result;
}
public class Unlock extends GamesRequest {
private static final String REST_PATH = "achievements/{achievementId}/unlock";
/**
* Unlocks this achievement for the currently authenticated player.
*
* Create a request for the method "achievements.unlock".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Unlock#execute()} method to invoke the remote operation. {@link
* Unlock#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param achievementId The ID of the achievement used by this method.
* @since 1.13
*/
protected Unlock(java.lang.String achievementId) {
super(Games.this, "POST", REST_PATH, null, com.google.api.services.games.model.AchievementUnlockResponse.class);
this.achievementId = com.google.api.client.util.Preconditions.checkNotNull(achievementId, "Required parameter achievementId must be specified.");
}
@Override
public Unlock setAlt(java.lang.String alt) {
return (Unlock) super.setAlt(alt);
}
@Override
public Unlock setFields(java.lang.String fields) {
return (Unlock) super.setFields(fields);
}
@Override
public Unlock setKey(java.lang.String key) {
return (Unlock) super.setKey(key);
}
@Override
public Unlock setOauthToken(java.lang.String oauthToken) {
return (Unlock) super.setOauthToken(oauthToken);
}
@Override
public Unlock setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Unlock) super.setPrettyPrint(prettyPrint);
}
@Override
public Unlock setQuotaUser(java.lang.String quotaUser) {
return (Unlock) super.setQuotaUser(quotaUser);
}
@Override
public Unlock setUserIp(java.lang.String userIp) {
return (Unlock) super.setUserIp(userIp);
}
/** The ID of the achievement used by this method. */
@com.google.api.client.util.Key
private java.lang.String achievementId;
/** The ID of the achievement used by this method.
*/
public java.lang.String getAchievementId() {
return achievementId;
}
/** The ID of the achievement used by this method. */
public Unlock setAchievementId(java.lang.String achievementId) {
this.achievementId = achievementId;
return this;
}
@Override
public Unlock set(String parameterName, Object value) {
return (Unlock) super.set(parameterName, value);
}
}
/**
* Updates multiple achievements for the currently authenticated player.
*
* Create a request for the method "achievements.updateMultiple".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link UpdateMultiple#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.games.model.AchievementUpdateMultipleRequest}
* @return the request
*/
public UpdateMultiple updateMultiple(com.google.api.services.games.model.AchievementUpdateMultipleRequest content) throws java.io.IOException {
UpdateMultiple result = new UpdateMultiple(content);
initialize(result);
return result;
}
public class UpdateMultiple extends GamesRequest {
private static final String REST_PATH = "achievements/updateMultiple";
/**
* Updates multiple achievements for the currently authenticated player.
*
* Create a request for the method "achievements.updateMultiple".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link UpdateMultiple#execute()} method to invoke the remote operation.
* {@link UpdateMultiple#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param content the {@link com.google.api.services.games.model.AchievementUpdateMultipleRequest}
* @since 1.13
*/
protected UpdateMultiple(com.google.api.services.games.model.AchievementUpdateMultipleRequest content) {
super(Games.this, "POST", REST_PATH, content, com.google.api.services.games.model.AchievementUpdateMultipleResponse.class);
}
@Override
public UpdateMultiple setAlt(java.lang.String alt) {
return (UpdateMultiple) super.setAlt(alt);
}
@Override
public UpdateMultiple setFields(java.lang.String fields) {
return (UpdateMultiple) super.setFields(fields);
}
@Override
public UpdateMultiple setKey(java.lang.String key) {
return (UpdateMultiple) super.setKey(key);
}
@Override
public UpdateMultiple setOauthToken(java.lang.String oauthToken) {
return (UpdateMultiple) super.setOauthToken(oauthToken);
}
@Override
public UpdateMultiple setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateMultiple) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateMultiple setQuotaUser(java.lang.String quotaUser) {
return (UpdateMultiple) super.setQuotaUser(quotaUser);
}
@Override
public UpdateMultiple setUserIp(java.lang.String userIp) {
return (UpdateMultiple) super.setUserIp(userIp);
}
@Override
public UpdateMultiple set(String parameterName, Object value) {
return (UpdateMultiple) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Applications collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Applications.List request = games.applications().list(parameters ...)}
*
*
* @return the resource collection
*/
public Applications applications() {
return new Applications();
}
/**
* The "applications" collection of methods.
*/
public class Applications {
/**
* Retrieves the metadata of the application with the given ID. If the requested application is not
* available for the specified platformType, the returned response will not include any instance
* data.
*
* Create a request for the method "applications.get".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param applicationId The application ID from the Google Play developer console.
* @return the request
*/
public Get get(java.lang.String applicationId) throws java.io.IOException {
Get result = new Get(applicationId);
initialize(result);
return result;
}
public class Get extends GamesRequest {
private static final String REST_PATH = "applications/{applicationId}";
/**
* Retrieves the metadata of the application with the given ID. If the requested application is
* not available for the specified platformType, the returned response will not include any
* instance data.
*
* Create a request for the method "applications.get".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param applicationId The application ID from the Google Play developer console.
* @since 1.13
*/
protected Get(java.lang.String applicationId) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.Application.class);
this.applicationId = com.google.api.client.util.Preconditions.checkNotNull(applicationId, "Required parameter applicationId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The application ID from the Google Play developer console. */
@com.google.api.client.util.Key
private java.lang.String applicationId;
/** The application ID from the Google Play developer console.
*/
public java.lang.String getApplicationId() {
return applicationId;
}
/** The application ID from the Google Play developer console. */
public Get setApplicationId(java.lang.String applicationId) {
this.applicationId = applicationId;
return this;
}
/** Restrict application details returned to the specific platform. */
@com.google.api.client.util.Key
private java.lang.String platformType;
/** Restrict application details returned to the specific platform.
*/
public java.lang.String getPlatformType() {
return platformType;
}
/** Restrict application details returned to the specific platform. */
public Get setPlatformType(java.lang.String platformType) {
this.platformType = platformType;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Indicate that the the currently authenticated user is playing your application.
*
* Create a request for the method "applications.played".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Played#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Played played() throws java.io.IOException {
Played result = new Played();
initialize(result);
return result;
}
public class Played extends GamesRequest {
private static final String REST_PATH = "applications/played";
/**
* Indicate that the the currently authenticated user is playing your application.
*
* Create a request for the method "applications.played".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Played#execute()} method to invoke the remote operation. {@link
* Played#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Played() {
super(Games.this, "POST", REST_PATH, null, Void.class);
}
@Override
public Played setAlt(java.lang.String alt) {
return (Played) super.setAlt(alt);
}
@Override
public Played setFields(java.lang.String fields) {
return (Played) super.setFields(fields);
}
@Override
public Played setKey(java.lang.String key) {
return (Played) super.setKey(key);
}
@Override
public Played setOauthToken(java.lang.String oauthToken) {
return (Played) super.setOauthToken(oauthToken);
}
@Override
public Played setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Played) super.setPrettyPrint(prettyPrint);
}
@Override
public Played setQuotaUser(java.lang.String quotaUser) {
return (Played) super.setQuotaUser(quotaUser);
}
@Override
public Played setUserIp(java.lang.String userIp) {
return (Played) super.setUserIp(userIp);
}
@Override
public Played set(String parameterName, Object value) {
return (Played) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Events collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Events.List request = games.events().list(parameters ...)}
*
*
* @return the resource collection
*/
public Events events() {
return new Events();
}
/**
* The "events" collection of methods.
*/
public class Events {
/**
* Returns a list showing the current progress on events in this application for the currently
* authenticated user.
*
* Create a request for the method "events.listByPlayer".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link ListByPlayer#execute()} method to invoke the remote operation.
*
* @return the request
*/
public ListByPlayer listByPlayer() throws java.io.IOException {
ListByPlayer result = new ListByPlayer();
initialize(result);
return result;
}
public class ListByPlayer extends GamesRequest {
private static final String REST_PATH = "events";
/**
* Returns a list showing the current progress on events in this application for the currently
* authenticated user.
*
* Create a request for the method "events.listByPlayer".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link ListByPlayer#execute()} method to invoke the remote operation.
* {@link
* ListByPlayer#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected ListByPlayer() {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.PlayerEventListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListByPlayer setAlt(java.lang.String alt) {
return (ListByPlayer) super.setAlt(alt);
}
@Override
public ListByPlayer setFields(java.lang.String fields) {
return (ListByPlayer) super.setFields(fields);
}
@Override
public ListByPlayer setKey(java.lang.String key) {
return (ListByPlayer) super.setKey(key);
}
@Override
public ListByPlayer setOauthToken(java.lang.String oauthToken) {
return (ListByPlayer) super.setOauthToken(oauthToken);
}
@Override
public ListByPlayer setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListByPlayer) super.setPrettyPrint(prettyPrint);
}
@Override
public ListByPlayer setQuotaUser(java.lang.String quotaUser) {
return (ListByPlayer) super.setQuotaUser(quotaUser);
}
@Override
public ListByPlayer setUserIp(java.lang.String userIp) {
return (ListByPlayer) super.setUserIp(userIp);
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public ListByPlayer setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of events to return in the response, used for paging. For any response,
* the actual number of events to return may be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of events to return in the response, used for paging. For any response, the
actual number of events to return may be less than the specified maxResults.
[minimum: 1] [maximum: 100]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of events to return in the response, used for paging. For any response,
* the actual number of events to return may be less than the specified maxResults.
*/
public ListByPlayer setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public ListByPlayer setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public ListByPlayer set(String parameterName, Object value) {
return (ListByPlayer) super.set(parameterName, value);
}
}
/**
* Returns a list of the event definitions in this application.
*
* Create a request for the method "events.listDefinitions".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link ListDefinitions#execute()} method to invoke the remote operation.
*
* @return the request
*/
public ListDefinitions listDefinitions() throws java.io.IOException {
ListDefinitions result = new ListDefinitions();
initialize(result);
return result;
}
public class ListDefinitions extends GamesRequest {
private static final String REST_PATH = "eventDefinitions";
/**
* Returns a list of the event definitions in this application.
*
* Create a request for the method "events.listDefinitions".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link ListDefinitions#execute()} method to invoke the remote operation.
* {@link ListDefinitions#initialize(com.google.api.client.googleapis.services.AbstractGoogleC
* lientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @since 1.13
*/
protected ListDefinitions() {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.EventDefinitionListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListDefinitions setAlt(java.lang.String alt) {
return (ListDefinitions) super.setAlt(alt);
}
@Override
public ListDefinitions setFields(java.lang.String fields) {
return (ListDefinitions) super.setFields(fields);
}
@Override
public ListDefinitions setKey(java.lang.String key) {
return (ListDefinitions) super.setKey(key);
}
@Override
public ListDefinitions setOauthToken(java.lang.String oauthToken) {
return (ListDefinitions) super.setOauthToken(oauthToken);
}
@Override
public ListDefinitions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListDefinitions) super.setPrettyPrint(prettyPrint);
}
@Override
public ListDefinitions setQuotaUser(java.lang.String quotaUser) {
return (ListDefinitions) super.setQuotaUser(quotaUser);
}
@Override
public ListDefinitions setUserIp(java.lang.String userIp) {
return (ListDefinitions) super.setUserIp(userIp);
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public ListDefinitions setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of event definitions to return in the response, used for paging. For any
* response, the actual number of event definitions to return may be less than the specified
* maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of event definitions to return in the response, used for paging. For any
response, the actual number of event definitions to return may be less than the specified
maxResults.
[minimum: 1] [maximum: 100]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of event definitions to return in the response, used for paging. For any
* response, the actual number of event definitions to return may be less than the specified
* maxResults.
*/
public ListDefinitions setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public ListDefinitions setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public ListDefinitions set(String parameterName, Object value) {
return (ListDefinitions) super.set(parameterName, value);
}
}
/**
* Records a batch of changes to the number of times events have occurred for the currently
* authenticated user of this application.
*
* Create a request for the method "events.record".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Record#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.games.model.EventRecordRequest}
* @return the request
*/
public Record record(com.google.api.services.games.model.EventRecordRequest content) throws java.io.IOException {
Record result = new Record(content);
initialize(result);
return result;
}
public class Record extends GamesRequest {
private static final String REST_PATH = "events";
/**
* Records a batch of changes to the number of times events have occurred for the currently
* authenticated user of this application.
*
* Create a request for the method "events.record".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Record#execute()} method to invoke the remote operation. {@link
* Record#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.games.model.EventRecordRequest}
* @since 1.13
*/
protected Record(com.google.api.services.games.model.EventRecordRequest content) {
super(Games.this, "POST", REST_PATH, content, com.google.api.services.games.model.EventUpdateResponse.class);
}
@Override
public Record setAlt(java.lang.String alt) {
return (Record) super.setAlt(alt);
}
@Override
public Record setFields(java.lang.String fields) {
return (Record) super.setFields(fields);
}
@Override
public Record setKey(java.lang.String key) {
return (Record) super.setKey(key);
}
@Override
public Record setOauthToken(java.lang.String oauthToken) {
return (Record) super.setOauthToken(oauthToken);
}
@Override
public Record setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Record) super.setPrettyPrint(prettyPrint);
}
@Override
public Record setQuotaUser(java.lang.String quotaUser) {
return (Record) super.setQuotaUser(quotaUser);
}
@Override
public Record setUserIp(java.lang.String userIp) {
return (Record) super.setUserIp(userIp);
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Record setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Record set(String parameterName, Object value) {
return (Record) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Leaderboards collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Leaderboards.List request = games.leaderboards().list(parameters ...)}
*
*
* @return the resource collection
*/
public Leaderboards leaderboards() {
return new Leaderboards();
}
/**
* The "leaderboards" collection of methods.
*/
public class Leaderboards {
/**
* Retrieves the metadata of the leaderboard with the given ID.
*
* Create a request for the method "leaderboards.get".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param leaderboardId The ID of the leaderboard.
* @return the request
*/
public Get get(java.lang.String leaderboardId) throws java.io.IOException {
Get result = new Get(leaderboardId);
initialize(result);
return result;
}
public class Get extends GamesRequest {
private static final String REST_PATH = "leaderboards/{leaderboardId}";
/**
* Retrieves the metadata of the leaderboard with the given ID.
*
* Create a request for the method "leaderboards.get".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param leaderboardId The ID of the leaderboard.
* @since 1.13
*/
protected Get(java.lang.String leaderboardId) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.Leaderboard.class);
this.leaderboardId = com.google.api.client.util.Preconditions.checkNotNull(leaderboardId, "Required parameter leaderboardId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the leaderboard. */
@com.google.api.client.util.Key
private java.lang.String leaderboardId;
/** The ID of the leaderboard.
*/
public java.lang.String getLeaderboardId() {
return leaderboardId;
}
/** The ID of the leaderboard. */
public Get setLeaderboardId(java.lang.String leaderboardId) {
this.leaderboardId = leaderboardId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all the leaderboard metadata for your application.
*
* Create a request for the method "leaderboards.list".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends GamesRequest {
private static final String REST_PATH = "leaderboards";
/**
* Lists all the leaderboard metadata for your application.
*
* Create a request for the method "leaderboards.list".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.LeaderboardListResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of leaderboards to return in the response. For any response, the actual
* number of leaderboards returned may be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of leaderboards to return in the response. For any response, the actual number
of leaderboards returned may be less than the specified maxResults.
[minimum: 1] [maximum: 200]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of leaderboards to return in the response. For any response, the actual
* number of leaderboards returned may be less than the specified maxResults.
*/
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Metagame collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Metagame.List request = games.metagame().list(parameters ...)}
*
*
* @return the resource collection
*/
public Metagame metagame() {
return new Metagame();
}
/**
* The "metagame" collection of methods.
*/
public class Metagame {
/**
* Return the metagame configuration data for the calling application.
*
* Create a request for the method "metagame.getMetagameConfig".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link GetMetagameConfig#execute()} method to invoke the remote operation.
*
* @return the request
*/
public GetMetagameConfig getMetagameConfig() throws java.io.IOException {
GetMetagameConfig result = new GetMetagameConfig();
initialize(result);
return result;
}
public class GetMetagameConfig extends GamesRequest {
private static final String REST_PATH = "metagameConfig";
/**
* Return the metagame configuration data for the calling application.
*
* Create a request for the method "metagame.getMetagameConfig".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link GetMetagameConfig#execute()} method to invoke the remote operation.
* {@link GetMetagameConfig#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @since 1.13
*/
protected GetMetagameConfig() {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.MetagameConfig.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetMetagameConfig setAlt(java.lang.String alt) {
return (GetMetagameConfig) super.setAlt(alt);
}
@Override
public GetMetagameConfig setFields(java.lang.String fields) {
return (GetMetagameConfig) super.setFields(fields);
}
@Override
public GetMetagameConfig setKey(java.lang.String key) {
return (GetMetagameConfig) super.setKey(key);
}
@Override
public GetMetagameConfig setOauthToken(java.lang.String oauthToken) {
return (GetMetagameConfig) super.setOauthToken(oauthToken);
}
@Override
public GetMetagameConfig setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetMetagameConfig) super.setPrettyPrint(prettyPrint);
}
@Override
public GetMetagameConfig setQuotaUser(java.lang.String quotaUser) {
return (GetMetagameConfig) super.setQuotaUser(quotaUser);
}
@Override
public GetMetagameConfig setUserIp(java.lang.String userIp) {
return (GetMetagameConfig) super.setUserIp(userIp);
}
@Override
public GetMetagameConfig set(String parameterName, Object value) {
return (GetMetagameConfig) super.set(parameterName, value);
}
}
/**
* List play data aggregated per category for the player corresponding to playerId.
*
* Create a request for the method "metagame.listCategoriesByPlayer".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link ListCategoriesByPlayer#execute()} method to invoke the remote
* operation.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @param collection The collection of categories for which data will be returned.
* @return the request
*/
public ListCategoriesByPlayer listCategoriesByPlayer(java.lang.String playerId, java.lang.String collection) throws java.io.IOException {
ListCategoriesByPlayer result = new ListCategoriesByPlayer(playerId, collection);
initialize(result);
return result;
}
public class ListCategoriesByPlayer extends GamesRequest {
private static final String REST_PATH = "players/{playerId}/categories/{collection}";
/**
* List play data aggregated per category for the player corresponding to playerId.
*
* Create a request for the method "metagame.listCategoriesByPlayer".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link ListCategoriesByPlayer#execute()} method to invoke the remote
* operation. {@link ListCategoriesByPlayer#initialize(com.google.api.client.googleapis.servic
* es.AbstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @param collection The collection of categories for which data will be returned.
* @since 1.13
*/
protected ListCategoriesByPlayer(java.lang.String playerId, java.lang.String collection) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.CategoryListResponse.class);
this.playerId = com.google.api.client.util.Preconditions.checkNotNull(playerId, "Required parameter playerId must be specified.");
this.collection = com.google.api.client.util.Preconditions.checkNotNull(collection, "Required parameter collection must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListCategoriesByPlayer setAlt(java.lang.String alt) {
return (ListCategoriesByPlayer) super.setAlt(alt);
}
@Override
public ListCategoriesByPlayer setFields(java.lang.String fields) {
return (ListCategoriesByPlayer) super.setFields(fields);
}
@Override
public ListCategoriesByPlayer setKey(java.lang.String key) {
return (ListCategoriesByPlayer) super.setKey(key);
}
@Override
public ListCategoriesByPlayer setOauthToken(java.lang.String oauthToken) {
return (ListCategoriesByPlayer) super.setOauthToken(oauthToken);
}
@Override
public ListCategoriesByPlayer setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListCategoriesByPlayer) super.setPrettyPrint(prettyPrint);
}
@Override
public ListCategoriesByPlayer setQuotaUser(java.lang.String quotaUser) {
return (ListCategoriesByPlayer) super.setQuotaUser(quotaUser);
}
@Override
public ListCategoriesByPlayer setUserIp(java.lang.String userIp) {
return (ListCategoriesByPlayer) super.setUserIp(userIp);
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
@com.google.api.client.util.Key
private java.lang.String playerId;
/** A player ID. A value of me may be used in place of the authenticated player's ID.
*/
public java.lang.String getPlayerId() {
return playerId;
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
public ListCategoriesByPlayer setPlayerId(java.lang.String playerId) {
this.playerId = playerId;
return this;
}
/** The collection of categories for which data will be returned. */
@com.google.api.client.util.Key
private java.lang.String collection;
/** The collection of categories for which data will be returned.
*/
public java.lang.String getCollection() {
return collection;
}
/** The collection of categories for which data will be returned. */
public ListCategoriesByPlayer setCollection(java.lang.String collection) {
this.collection = collection;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public ListCategoriesByPlayer setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of category resources to return in the response, used for paging. For
* any response, the actual number of category resources returned may be less than the
* specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of category resources to return in the response, used for paging. For any
response, the actual number of category resources returned may be less than the specified
maxResults.
[minimum: 1] [maximum: 100]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of category resources to return in the response, used for paging. For
* any response, the actual number of category resources returned may be less than the
* specified maxResults.
*/
public ListCategoriesByPlayer setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public ListCategoriesByPlayer setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public ListCategoriesByPlayer set(String parameterName, Object value) {
return (ListCategoriesByPlayer) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Players collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Players.List request = games.players().list(parameters ...)}
*
*
* @return the resource collection
*/
public Players players() {
return new Players();
}
/**
* The "players" collection of methods.
*/
public class Players {
/**
* Retrieves the Player resource with the given ID. To retrieve the player for the currently
* authenticated user, set playerId to me.
*
* Create a request for the method "players.get".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @return the request
*/
public Get get(java.lang.String playerId) throws java.io.IOException {
Get result = new Get(playerId);
initialize(result);
return result;
}
public class Get extends GamesRequest {
private static final String REST_PATH = "players/{playerId}";
/**
* Retrieves the Player resource with the given ID. To retrieve the player for the currently
* authenticated user, set playerId to me.
*
* Create a request for the method "players.get".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @since 1.13
*/
protected Get(java.lang.String playerId) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.Player.class);
this.playerId = com.google.api.client.util.Preconditions.checkNotNull(playerId, "Required parameter playerId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
@com.google.api.client.util.Key
private java.lang.String playerId;
/** A player ID. A value of me may be used in place of the authenticated player's ID.
*/
public java.lang.String getPlayerId() {
return playerId;
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
public Get setPlayerId(java.lang.String playerId) {
this.playerId = playerId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Get the collection of players for the currently authenticated user.
*
* Create a request for the method "players.list".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param collection Collection of players being retrieved
* @return the request
*/
public List list(java.lang.String collection) throws java.io.IOException {
List result = new List(collection);
initialize(result);
return result;
}
public class List extends GamesRequest {
private static final String REST_PATH = "players/me/players/{collection}";
/**
* Get the collection of players for the currently authenticated user.
*
* Create a request for the method "players.list".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param collection Collection of players being retrieved
* @since 1.13
*/
protected List(java.lang.String collection) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.PlayerListResponse.class);
this.collection = com.google.api.client.util.Preconditions.checkNotNull(collection, "Required parameter collection must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** Collection of players being retrieved */
@com.google.api.client.util.Key
private java.lang.String collection;
/** Collection of players being retrieved
*/
public java.lang.String getCollection() {
return collection;
}
/** Collection of players being retrieved */
public List setCollection(java.lang.String collection) {
this.collection = collection;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of player resources to return in the response, used for paging. For any
* response, the actual number of player resources returned may be less than the specified
* maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of player resources to return in the response, used for paging. For any
response, the actual number of player resources returned may be less than the specified maxResults.
[minimum: 1] [maximum: 15]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of player resources to return in the response, used for paging. For any
* response, the actual number of player resources returned may be less than the specified
* maxResults.
*/
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Pushtokens collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Pushtokens.List request = games.pushtokens().list(parameters ...)}
*
*
* @return the resource collection
*/
public Pushtokens pushtokens() {
return new Pushtokens();
}
/**
* The "pushtokens" collection of methods.
*/
public class Pushtokens {
/**
* Removes a push token for the current user and application. Removing a non-existent push token
* will report success.
*
* Create a request for the method "pushtokens.remove".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Remove#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.games.model.PushTokenId}
* @return the request
*/
public Remove remove(com.google.api.services.games.model.PushTokenId content) throws java.io.IOException {
Remove result = new Remove(content);
initialize(result);
return result;
}
public class Remove extends GamesRequest {
private static final String REST_PATH = "pushtokens/remove";
/**
* Removes a push token for the current user and application. Removing a non-existent push token
* will report success.
*
* Create a request for the method "pushtokens.remove".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Remove#execute()} method to invoke the remote operation. {@link
* Remove#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.games.model.PushTokenId}
* @since 1.13
*/
protected Remove(com.google.api.services.games.model.PushTokenId content) {
super(Games.this, "POST", REST_PATH, content, Void.class);
}
@Override
public Remove setAlt(java.lang.String alt) {
return (Remove) super.setAlt(alt);
}
@Override
public Remove setFields(java.lang.String fields) {
return (Remove) super.setFields(fields);
}
@Override
public Remove setKey(java.lang.String key) {
return (Remove) super.setKey(key);
}
@Override
public Remove setOauthToken(java.lang.String oauthToken) {
return (Remove) super.setOauthToken(oauthToken);
}
@Override
public Remove setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Remove) super.setPrettyPrint(prettyPrint);
}
@Override
public Remove setQuotaUser(java.lang.String quotaUser) {
return (Remove) super.setQuotaUser(quotaUser);
}
@Override
public Remove setUserIp(java.lang.String userIp) {
return (Remove) super.setUserIp(userIp);
}
@Override
public Remove set(String parameterName, Object value) {
return (Remove) super.set(parameterName, value);
}
}
/**
* Registers a push token for the current user and application.
*
* Create a request for the method "pushtokens.update".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.games.model.PushToken}
* @return the request
*/
public Update update(com.google.api.services.games.model.PushToken content) throws java.io.IOException {
Update result = new Update(content);
initialize(result);
return result;
}
public class Update extends GamesRequest {
private static final String REST_PATH = "pushtokens";
/**
* Registers a push token for the current user and application.
*
* Create a request for the method "pushtokens.update".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.games.model.PushToken}
* @since 1.13
*/
protected Update(com.google.api.services.games.model.PushToken content) {
super(Games.this, "PUT", REST_PATH, content, Void.class);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the QuestMilestones collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.QuestMilestones.List request = games.questMilestones().list(parameters ...)}
*
*
* @return the resource collection
*/
public QuestMilestones questMilestones() {
return new QuestMilestones();
}
/**
* The "questMilestones" collection of methods.
*/
public class QuestMilestones {
/**
* Report that a reward for the milestone corresponding to milestoneId for the quest corresponding
* to questId has been claimed by the currently authorized user.
*
* Create a request for the method "questMilestones.claim".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Claim#execute()} method to invoke the remote operation.
*
* @param questId The ID of the quest.
* @param milestoneId The ID of the milestone.
* @param requestId A numeric ID to ensure that the request is handled correctly across retries. Your client application
* must generate this ID randomly.
* @return the request
*/
public Claim claim(java.lang.String questId, java.lang.String milestoneId, java.lang.Long requestId) throws java.io.IOException {
Claim result = new Claim(questId, milestoneId, requestId);
initialize(result);
return result;
}
public class Claim extends GamesRequest {
private static final String REST_PATH = "quests/{questId}/milestones/{milestoneId}/claim";
/**
* Report that a reward for the milestone corresponding to milestoneId for the quest corresponding
* to questId has been claimed by the currently authorized user.
*
* Create a request for the method "questMilestones.claim".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Claim#execute()} method to invoke the remote operation. {@link
* Claim#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param questId The ID of the quest.
* @param milestoneId The ID of the milestone.
* @param requestId A numeric ID to ensure that the request is handled correctly across retries. Your client application
* must generate this ID randomly.
* @since 1.13
*/
protected Claim(java.lang.String questId, java.lang.String milestoneId, java.lang.Long requestId) {
super(Games.this, "PUT", REST_PATH, null, Void.class);
this.questId = com.google.api.client.util.Preconditions.checkNotNull(questId, "Required parameter questId must be specified.");
this.milestoneId = com.google.api.client.util.Preconditions.checkNotNull(milestoneId, "Required parameter milestoneId must be specified.");
this.requestId = com.google.api.client.util.Preconditions.checkNotNull(requestId, "Required parameter requestId must be specified.");
}
@Override
public Claim setAlt(java.lang.String alt) {
return (Claim) super.setAlt(alt);
}
@Override
public Claim setFields(java.lang.String fields) {
return (Claim) super.setFields(fields);
}
@Override
public Claim setKey(java.lang.String key) {
return (Claim) super.setKey(key);
}
@Override
public Claim setOauthToken(java.lang.String oauthToken) {
return (Claim) super.setOauthToken(oauthToken);
}
@Override
public Claim setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Claim) super.setPrettyPrint(prettyPrint);
}
@Override
public Claim setQuotaUser(java.lang.String quotaUser) {
return (Claim) super.setQuotaUser(quotaUser);
}
@Override
public Claim setUserIp(java.lang.String userIp) {
return (Claim) super.setUserIp(userIp);
}
/** The ID of the quest. */
@com.google.api.client.util.Key
private java.lang.String questId;
/** The ID of the quest.
*/
public java.lang.String getQuestId() {
return questId;
}
/** The ID of the quest. */
public Claim setQuestId(java.lang.String questId) {
this.questId = questId;
return this;
}
/** The ID of the milestone. */
@com.google.api.client.util.Key
private java.lang.String milestoneId;
/** The ID of the milestone.
*/
public java.lang.String getMilestoneId() {
return milestoneId;
}
/** The ID of the milestone. */
public Claim setMilestoneId(java.lang.String milestoneId) {
this.milestoneId = milestoneId;
return this;
}
/**
* A numeric ID to ensure that the request is handled correctly across retries. Your client
* application must generate this ID randomly.
*/
@com.google.api.client.util.Key
private java.lang.Long requestId;
/** A numeric ID to ensure that the request is handled correctly across retries. Your client
application must generate this ID randomly.
*/
public java.lang.Long getRequestId() {
return requestId;
}
/**
* A numeric ID to ensure that the request is handled correctly across retries. Your client
* application must generate this ID randomly.
*/
public Claim setRequestId(java.lang.Long requestId) {
this.requestId = requestId;
return this;
}
@Override
public Claim set(String parameterName, Object value) {
return (Claim) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Quests collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Quests.List request = games.quests().list(parameters ...)}
*
*
* @return the resource collection
*/
public Quests quests() {
return new Quests();
}
/**
* The "quests" collection of methods.
*/
public class Quests {
/**
* Indicates that the currently authorized user will participate in the quest.
*
* Create a request for the method "quests.accept".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Accept#execute()} method to invoke the remote operation.
*
* @param questId The ID of the quest.
* @return the request
*/
public Accept accept(java.lang.String questId) throws java.io.IOException {
Accept result = new Accept(questId);
initialize(result);
return result;
}
public class Accept extends GamesRequest {
private static final String REST_PATH = "quests/{questId}/accept";
/**
* Indicates that the currently authorized user will participate in the quest.
*
* Create a request for the method "quests.accept".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Accept#execute()} method to invoke the remote operation. {@link
* Accept#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param questId The ID of the quest.
* @since 1.13
*/
protected Accept(java.lang.String questId) {
super(Games.this, "POST", REST_PATH, null, com.google.api.services.games.model.Quest.class);
this.questId = com.google.api.client.util.Preconditions.checkNotNull(questId, "Required parameter questId must be specified.");
}
@Override
public Accept setAlt(java.lang.String alt) {
return (Accept) super.setAlt(alt);
}
@Override
public Accept setFields(java.lang.String fields) {
return (Accept) super.setFields(fields);
}
@Override
public Accept setKey(java.lang.String key) {
return (Accept) super.setKey(key);
}
@Override
public Accept setOauthToken(java.lang.String oauthToken) {
return (Accept) super.setOauthToken(oauthToken);
}
@Override
public Accept setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Accept) super.setPrettyPrint(prettyPrint);
}
@Override
public Accept setQuotaUser(java.lang.String quotaUser) {
return (Accept) super.setQuotaUser(quotaUser);
}
@Override
public Accept setUserIp(java.lang.String userIp) {
return (Accept) super.setUserIp(userIp);
}
/** The ID of the quest. */
@com.google.api.client.util.Key
private java.lang.String questId;
/** The ID of the quest.
*/
public java.lang.String getQuestId() {
return questId;
}
/** The ID of the quest. */
public Accept setQuestId(java.lang.String questId) {
this.questId = questId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Accept setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Accept set(String parameterName, Object value) {
return (Accept) super.set(parameterName, value);
}
}
/**
* Get a list of quests for your application and the currently authenticated player.
*
* Create a request for the method "quests.list".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @return the request
*/
public List list(java.lang.String playerId) throws java.io.IOException {
List result = new List(playerId);
initialize(result);
return result;
}
public class List extends GamesRequest {
private static final String REST_PATH = "players/{playerId}/quests";
/**
* Get a list of quests for your application and the currently authenticated player.
*
* Create a request for the method "quests.list".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @since 1.13
*/
protected List(java.lang.String playerId) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.QuestListResponse.class);
this.playerId = com.google.api.client.util.Preconditions.checkNotNull(playerId, "Required parameter playerId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
@com.google.api.client.util.Key
private java.lang.String playerId;
/** A player ID. A value of me may be used in place of the authenticated player's ID.
*/
public java.lang.String getPlayerId() {
return playerId;
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
public List setPlayerId(java.lang.String playerId) {
this.playerId = playerId;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of quest resources to return in the response, used for paging. For any
* response, the actual number of quest resources returned may be less than the specified
* maxResults. Acceptable values are 1 to 50, inclusive. (Default: 50).
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of quest resources to return in the response, used for paging. For any response,
the actual number of quest resources returned may be less than the specified maxResults. Acceptable
values are 1 to 50, inclusive. (Default: 50).
[minimum: 1] [maximum: 50]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of quest resources to return in the response, used for paging. For any
* response, the actual number of quest resources returned may be less than the specified
* maxResults. Acceptable values are 1 to 50, inclusive. (Default: 50).
*/
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Revisions collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Revisions.List request = games.revisions().list(parameters ...)}
*
*
* @return the resource collection
*/
public Revisions revisions() {
return new Revisions();
}
/**
* The "revisions" collection of methods.
*/
public class Revisions {
/**
* Checks whether the games client is out of date.
*
* Create a request for the method "revisions.check".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Check#execute()} method to invoke the remote operation.
*
* @param clientRevision The revision of the client SDK used by your application. Format:
[PLATFORM_TYPE]:[VERSION_NUMBER].
* Possible values of PLATFORM_TYPE are:
- "ANDROID" - Client is running the Android SDK.
-
* "IOS" - Client is running the iOS SDK.
- "WEB_APP" - Client is running as a Web App.
* @return the request
*/
public Check check(java.lang.String clientRevision) throws java.io.IOException {
Check result = new Check(clientRevision);
initialize(result);
return result;
}
public class Check extends GamesRequest {
private static final String REST_PATH = "revisions/check";
/**
* Checks whether the games client is out of date.
*
* Create a request for the method "revisions.check".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Check#execute()} method to invoke the remote operation. {@link
* Check#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param clientRevision The revision of the client SDK used by your application. Format:
[PLATFORM_TYPE]:[VERSION_NUMBER].
* Possible values of PLATFORM_TYPE are:
- "ANDROID" - Client is running the Android SDK.
-
* "IOS" - Client is running the iOS SDK.
- "WEB_APP" - Client is running as a Web App.
* @since 1.13
*/
protected Check(java.lang.String clientRevision) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.RevisionCheckResponse.class);
this.clientRevision = com.google.api.client.util.Preconditions.checkNotNull(clientRevision, "Required parameter clientRevision must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Check setAlt(java.lang.String alt) {
return (Check) super.setAlt(alt);
}
@Override
public Check setFields(java.lang.String fields) {
return (Check) super.setFields(fields);
}
@Override
public Check setKey(java.lang.String key) {
return (Check) super.setKey(key);
}
@Override
public Check setOauthToken(java.lang.String oauthToken) {
return (Check) super.setOauthToken(oauthToken);
}
@Override
public Check setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Check) super.setPrettyPrint(prettyPrint);
}
@Override
public Check setQuotaUser(java.lang.String quotaUser) {
return (Check) super.setQuotaUser(quotaUser);
}
@Override
public Check setUserIp(java.lang.String userIp) {
return (Check) super.setUserIp(userIp);
}
/**
* The revision of the client SDK used by your application. Format:
* [PLATFORM_TYPE]:[VERSION_NUMBER]. Possible values of PLATFORM_TYPE are:
*
* - "ANDROID" - Client is running the Android SDK. - "IOS" - Client is running the iOS SDK. -
* "WEB_APP" - Client is running as a Web App.
*/
@com.google.api.client.util.Key
private java.lang.String clientRevision;
/** The revision of the client SDK used by your application. Format: [PLATFORM_TYPE]:[VERSION_NUMBER].
Possible values of PLATFORM_TYPE are:
- "ANDROID" - Client is running the Android SDK. - "IOS" - Client is running the iOS SDK. -
"WEB_APP" - Client is running as a Web App.
*/
public java.lang.String getClientRevision() {
return clientRevision;
}
/**
* The revision of the client SDK used by your application. Format:
* [PLATFORM_TYPE]:[VERSION_NUMBER]. Possible values of PLATFORM_TYPE are:
*
* - "ANDROID" - Client is running the Android SDK. - "IOS" - Client is running the iOS SDK. -
* "WEB_APP" - Client is running as a Web App.
*/
public Check setClientRevision(java.lang.String clientRevision) {
this.clientRevision = clientRevision;
return this;
}
@Override
public Check set(String parameterName, Object value) {
return (Check) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Rooms collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Rooms.List request = games.rooms().list(parameters ...)}
*
*
* @return the resource collection
*/
public Rooms rooms() {
return new Rooms();
}
/**
* The "rooms" collection of methods.
*/
public class Rooms {
/**
* Create a room. For internal use by the Games SDK only. Calling this method directly is
* unsupported.
*
* Create a request for the method "rooms.create".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.games.model.RoomCreateRequest}
* @return the request
*/
public Create create(com.google.api.services.games.model.RoomCreateRequest content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends GamesRequest {
private static final String REST_PATH = "rooms/create";
/**
* Create a room. For internal use by the Games SDK only. Calling this method directly is
* unsupported.
*
* Create a request for the method "rooms.create".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.games.model.RoomCreateRequest}
* @since 1.13
*/
protected Create(com.google.api.services.games.model.RoomCreateRequest content) {
super(Games.this, "POST", REST_PATH, content, com.google.api.services.games.model.Room.class);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Create setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Decline an invitation to join a room. For internal use by the Games SDK only. Calling this method
* directly is unsupported.
*
* Create a request for the method "rooms.decline".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Decline#execute()} method to invoke the remote operation.
*
* @param roomId The ID of the room.
* @return the request
*/
public Decline decline(java.lang.String roomId) throws java.io.IOException {
Decline result = new Decline(roomId);
initialize(result);
return result;
}
public class Decline extends GamesRequest {
private static final String REST_PATH = "rooms/{roomId}/decline";
/**
* Decline an invitation to join a room. For internal use by the Games SDK only. Calling this
* method directly is unsupported.
*
* Create a request for the method "rooms.decline".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Decline#execute()} method to invoke the remote operation.
* {@link
* Decline#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param roomId The ID of the room.
* @since 1.13
*/
protected Decline(java.lang.String roomId) {
super(Games.this, "POST", REST_PATH, null, com.google.api.services.games.model.Room.class);
this.roomId = com.google.api.client.util.Preconditions.checkNotNull(roomId, "Required parameter roomId must be specified.");
}
@Override
public Decline setAlt(java.lang.String alt) {
return (Decline) super.setAlt(alt);
}
@Override
public Decline setFields(java.lang.String fields) {
return (Decline) super.setFields(fields);
}
@Override
public Decline setKey(java.lang.String key) {
return (Decline) super.setKey(key);
}
@Override
public Decline setOauthToken(java.lang.String oauthToken) {
return (Decline) super.setOauthToken(oauthToken);
}
@Override
public Decline setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Decline) super.setPrettyPrint(prettyPrint);
}
@Override
public Decline setQuotaUser(java.lang.String quotaUser) {
return (Decline) super.setQuotaUser(quotaUser);
}
@Override
public Decline setUserIp(java.lang.String userIp) {
return (Decline) super.setUserIp(userIp);
}
/** The ID of the room. */
@com.google.api.client.util.Key
private java.lang.String roomId;
/** The ID of the room.
*/
public java.lang.String getRoomId() {
return roomId;
}
/** The ID of the room. */
public Decline setRoomId(java.lang.String roomId) {
this.roomId = roomId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Decline setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Decline set(String parameterName, Object value) {
return (Decline) super.set(parameterName, value);
}
}
/**
* Dismiss an invitation to join a room. For internal use by the Games SDK only. Calling this method
* directly is unsupported.
*
* Create a request for the method "rooms.dismiss".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
*
* @param roomId The ID of the room.
* @return the request
*/
public Dismiss dismiss(java.lang.String roomId) throws java.io.IOException {
Dismiss result = new Dismiss(roomId);
initialize(result);
return result;
}
public class Dismiss extends GamesRequest {
private static final String REST_PATH = "rooms/{roomId}/dismiss";
/**
* Dismiss an invitation to join a room. For internal use by the Games SDK only. Calling this
* method directly is unsupported.
*
* Create a request for the method "rooms.dismiss".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
* {@link
* Dismiss#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param roomId The ID of the room.
* @since 1.13
*/
protected Dismiss(java.lang.String roomId) {
super(Games.this, "POST", REST_PATH, null, Void.class);
this.roomId = com.google.api.client.util.Preconditions.checkNotNull(roomId, "Required parameter roomId must be specified.");
}
@Override
public Dismiss setAlt(java.lang.String alt) {
return (Dismiss) super.setAlt(alt);
}
@Override
public Dismiss setFields(java.lang.String fields) {
return (Dismiss) super.setFields(fields);
}
@Override
public Dismiss setKey(java.lang.String key) {
return (Dismiss) super.setKey(key);
}
@Override
public Dismiss setOauthToken(java.lang.String oauthToken) {
return (Dismiss) super.setOauthToken(oauthToken);
}
@Override
public Dismiss setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Dismiss) super.setPrettyPrint(prettyPrint);
}
@Override
public Dismiss setQuotaUser(java.lang.String quotaUser) {
return (Dismiss) super.setQuotaUser(quotaUser);
}
@Override
public Dismiss setUserIp(java.lang.String userIp) {
return (Dismiss) super.setUserIp(userIp);
}
/** The ID of the room. */
@com.google.api.client.util.Key
private java.lang.String roomId;
/** The ID of the room.
*/
public java.lang.String getRoomId() {
return roomId;
}
/** The ID of the room. */
public Dismiss setRoomId(java.lang.String roomId) {
this.roomId = roomId;
return this;
}
@Override
public Dismiss set(String parameterName, Object value) {
return (Dismiss) super.set(parameterName, value);
}
}
/**
* Get the data for a room.
*
* Create a request for the method "rooms.get".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param roomId The ID of the room.
* @return the request
*/
public Get get(java.lang.String roomId) throws java.io.IOException {
Get result = new Get(roomId);
initialize(result);
return result;
}
public class Get extends GamesRequest {
private static final String REST_PATH = "rooms/{roomId}";
/**
* Get the data for a room.
*
* Create a request for the method "rooms.get".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param roomId The ID of the room.
* @since 1.13
*/
protected Get(java.lang.String roomId) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.Room.class);
this.roomId = com.google.api.client.util.Preconditions.checkNotNull(roomId, "Required parameter roomId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the room. */
@com.google.api.client.util.Key
private java.lang.String roomId;
/** The ID of the room.
*/
public java.lang.String getRoomId() {
return roomId;
}
/** The ID of the room. */
public Get setRoomId(java.lang.String roomId) {
this.roomId = roomId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Join a room. For internal use by the Games SDK only. Calling this method directly is unsupported.
*
* Create a request for the method "rooms.join".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Join#execute()} method to invoke the remote operation.
*
* @param roomId The ID of the room.
* @param content the {@link com.google.api.services.games.model.RoomJoinRequest}
* @return the request
*/
public Join join(java.lang.String roomId, com.google.api.services.games.model.RoomJoinRequest content) throws java.io.IOException {
Join result = new Join(roomId, content);
initialize(result);
return result;
}
public class Join extends GamesRequest {
private static final String REST_PATH = "rooms/{roomId}/join";
/**
* Join a room. For internal use by the Games SDK only. Calling this method directly is
* unsupported.
*
* Create a request for the method "rooms.join".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Join#execute()} method to invoke the remote operation. {@link
* Join#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param roomId The ID of the room.
* @param content the {@link com.google.api.services.games.model.RoomJoinRequest}
* @since 1.13
*/
protected Join(java.lang.String roomId, com.google.api.services.games.model.RoomJoinRequest content) {
super(Games.this, "POST", REST_PATH, content, com.google.api.services.games.model.Room.class);
this.roomId = com.google.api.client.util.Preconditions.checkNotNull(roomId, "Required parameter roomId must be specified.");
}
@Override
public Join setAlt(java.lang.String alt) {
return (Join) super.setAlt(alt);
}
@Override
public Join setFields(java.lang.String fields) {
return (Join) super.setFields(fields);
}
@Override
public Join setKey(java.lang.String key) {
return (Join) super.setKey(key);
}
@Override
public Join setOauthToken(java.lang.String oauthToken) {
return (Join) super.setOauthToken(oauthToken);
}
@Override
public Join setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Join) super.setPrettyPrint(prettyPrint);
}
@Override
public Join setQuotaUser(java.lang.String quotaUser) {
return (Join) super.setQuotaUser(quotaUser);
}
@Override
public Join setUserIp(java.lang.String userIp) {
return (Join) super.setUserIp(userIp);
}
/** The ID of the room. */
@com.google.api.client.util.Key
private java.lang.String roomId;
/** The ID of the room.
*/
public java.lang.String getRoomId() {
return roomId;
}
/** The ID of the room. */
public Join setRoomId(java.lang.String roomId) {
this.roomId = roomId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Join setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Join set(String parameterName, Object value) {
return (Join) super.set(parameterName, value);
}
}
/**
* Leave a room. For internal use by the Games SDK only. Calling this method directly is
* unsupported.
*
* Create a request for the method "rooms.leave".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Leave#execute()} method to invoke the remote operation.
*
* @param roomId The ID of the room.
* @param content the {@link com.google.api.services.games.model.RoomLeaveRequest}
* @return the request
*/
public Leave leave(java.lang.String roomId, com.google.api.services.games.model.RoomLeaveRequest content) throws java.io.IOException {
Leave result = new Leave(roomId, content);
initialize(result);
return result;
}
public class Leave extends GamesRequest {
private static final String REST_PATH = "rooms/{roomId}/leave";
/**
* Leave a room. For internal use by the Games SDK only. Calling this method directly is
* unsupported.
*
* Create a request for the method "rooms.leave".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Leave#execute()} method to invoke the remote operation. {@link
* Leave#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param roomId The ID of the room.
* @param content the {@link com.google.api.services.games.model.RoomLeaveRequest}
* @since 1.13
*/
protected Leave(java.lang.String roomId, com.google.api.services.games.model.RoomLeaveRequest content) {
super(Games.this, "POST", REST_PATH, content, com.google.api.services.games.model.Room.class);
this.roomId = com.google.api.client.util.Preconditions.checkNotNull(roomId, "Required parameter roomId must be specified.");
}
@Override
public Leave setAlt(java.lang.String alt) {
return (Leave) super.setAlt(alt);
}
@Override
public Leave setFields(java.lang.String fields) {
return (Leave) super.setFields(fields);
}
@Override
public Leave setKey(java.lang.String key) {
return (Leave) super.setKey(key);
}
@Override
public Leave setOauthToken(java.lang.String oauthToken) {
return (Leave) super.setOauthToken(oauthToken);
}
@Override
public Leave setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Leave) super.setPrettyPrint(prettyPrint);
}
@Override
public Leave setQuotaUser(java.lang.String quotaUser) {
return (Leave) super.setQuotaUser(quotaUser);
}
@Override
public Leave setUserIp(java.lang.String userIp) {
return (Leave) super.setUserIp(userIp);
}
/** The ID of the room. */
@com.google.api.client.util.Key
private java.lang.String roomId;
/** The ID of the room.
*/
public java.lang.String getRoomId() {
return roomId;
}
/** The ID of the room. */
public Leave setRoomId(java.lang.String roomId) {
this.roomId = roomId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Leave setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Leave set(String parameterName, Object value) {
return (Leave) super.set(parameterName, value);
}
}
/**
* Returns invitations to join rooms.
*
* Create a request for the method "rooms.list".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends GamesRequest {
private static final String REST_PATH = "rooms";
/**
* Returns invitations to join rooms.
*
* Create a request for the method "rooms.list".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.RoomList.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of rooms to return in the response, used for paging. For any response,
* the actual number of rooms to return may be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of rooms to return in the response, used for paging. For any response, the
actual number of rooms to return may be less than the specified maxResults.
[minimum: 1] [maximum: 500]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of rooms to return in the response, used for paging. For any response,
* the actual number of rooms to return may be less than the specified maxResults.
*/
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates sent by a client reporting the status of peers in a room. For internal use by the Games
* SDK only. Calling this method directly is unsupported.
*
* Create a request for the method "rooms.reportStatus".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link ReportStatus#execute()} method to invoke the remote operation.
*
* @param roomId The ID of the room.
* @param content the {@link com.google.api.services.games.model.RoomP2PStatuses}
* @return the request
*/
public ReportStatus reportStatus(java.lang.String roomId, com.google.api.services.games.model.RoomP2PStatuses content) throws java.io.IOException {
ReportStatus result = new ReportStatus(roomId, content);
initialize(result);
return result;
}
public class ReportStatus extends GamesRequest {
private static final String REST_PATH = "rooms/{roomId}/reportstatus";
/**
* Updates sent by a client reporting the status of peers in a room. For internal use by the Games
* SDK only. Calling this method directly is unsupported.
*
* Create a request for the method "rooms.reportStatus".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link ReportStatus#execute()} method to invoke the remote operation.
* {@link
* ReportStatus#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param roomId The ID of the room.
* @param content the {@link com.google.api.services.games.model.RoomP2PStatuses}
* @since 1.13
*/
protected ReportStatus(java.lang.String roomId, com.google.api.services.games.model.RoomP2PStatuses content) {
super(Games.this, "POST", REST_PATH, content, com.google.api.services.games.model.RoomStatus.class);
this.roomId = com.google.api.client.util.Preconditions.checkNotNull(roomId, "Required parameter roomId must be specified.");
}
@Override
public ReportStatus setAlt(java.lang.String alt) {
return (ReportStatus) super.setAlt(alt);
}
@Override
public ReportStatus setFields(java.lang.String fields) {
return (ReportStatus) super.setFields(fields);
}
@Override
public ReportStatus setKey(java.lang.String key) {
return (ReportStatus) super.setKey(key);
}
@Override
public ReportStatus setOauthToken(java.lang.String oauthToken) {
return (ReportStatus) super.setOauthToken(oauthToken);
}
@Override
public ReportStatus setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ReportStatus) super.setPrettyPrint(prettyPrint);
}
@Override
public ReportStatus setQuotaUser(java.lang.String quotaUser) {
return (ReportStatus) super.setQuotaUser(quotaUser);
}
@Override
public ReportStatus setUserIp(java.lang.String userIp) {
return (ReportStatus) super.setUserIp(userIp);
}
/** The ID of the room. */
@com.google.api.client.util.Key
private java.lang.String roomId;
/** The ID of the room.
*/
public java.lang.String getRoomId() {
return roomId;
}
/** The ID of the room. */
public ReportStatus setRoomId(java.lang.String roomId) {
this.roomId = roomId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public ReportStatus setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public ReportStatus set(String parameterName, Object value) {
return (ReportStatus) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Scores collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Scores.List request = games.scores().list(parameters ...)}
*
*
* @return the resource collection
*/
public Scores scores() {
return new Scores();
}
/**
* The "scores" collection of methods.
*/
public class Scores {
/**
* Get high scores, and optionally ranks, in leaderboards for the currently authenticated player.
* For a specific time span, leaderboardId can be set to ALL to retrieve data for all leaderboards
* in a given time span. NOTE: You cannot ask for 'ALL' leaderboards and 'ALL' timeSpans in the same
* request; only one parameter may be set to 'ALL'.
*
* Create a request for the method "scores.get".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @param leaderboardId The ID of the leaderboard. Can be set to 'ALL' to retrieve data for all leaderboards for this
* application.
* @param timeSpan The time span for the scores and ranks you're requesting.
* @return the request
*/
public Get get(java.lang.String playerId, java.lang.String leaderboardId, java.lang.String timeSpan) throws java.io.IOException {
Get result = new Get(playerId, leaderboardId, timeSpan);
initialize(result);
return result;
}
public class Get extends GamesRequest {
private static final String REST_PATH = "players/{playerId}/leaderboards/{leaderboardId}/scores/{timeSpan}";
/**
* Get high scores, and optionally ranks, in leaderboards for the currently authenticated player.
* For a specific time span, leaderboardId can be set to ALL to retrieve data for all leaderboards
* in a given time span. NOTE: You cannot ask for 'ALL' leaderboards and 'ALL' timeSpans in the
* same request; only one parameter may be set to 'ALL'.
*
* Create a request for the method "scores.get".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @param leaderboardId The ID of the leaderboard. Can be set to 'ALL' to retrieve data for all leaderboards for this
* application.
* @param timeSpan The time span for the scores and ranks you're requesting.
* @since 1.13
*/
protected Get(java.lang.String playerId, java.lang.String leaderboardId, java.lang.String timeSpan) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.PlayerLeaderboardScoreListResponse.class);
this.playerId = com.google.api.client.util.Preconditions.checkNotNull(playerId, "Required parameter playerId must be specified.");
this.leaderboardId = com.google.api.client.util.Preconditions.checkNotNull(leaderboardId, "Required parameter leaderboardId must be specified.");
this.timeSpan = com.google.api.client.util.Preconditions.checkNotNull(timeSpan, "Required parameter timeSpan must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
@com.google.api.client.util.Key
private java.lang.String playerId;
/** A player ID. A value of me may be used in place of the authenticated player's ID.
*/
public java.lang.String getPlayerId() {
return playerId;
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
public Get setPlayerId(java.lang.String playerId) {
this.playerId = playerId;
return this;
}
/**
* The ID of the leaderboard. Can be set to 'ALL' to retrieve data for all leaderboards for
* this application.
*/
@com.google.api.client.util.Key
private java.lang.String leaderboardId;
/** The ID of the leaderboard. Can be set to 'ALL' to retrieve data for all leaderboards for this
application.
*/
public java.lang.String getLeaderboardId() {
return leaderboardId;
}
/**
* The ID of the leaderboard. Can be set to 'ALL' to retrieve data for all leaderboards for
* this application.
*/
public Get setLeaderboardId(java.lang.String leaderboardId) {
this.leaderboardId = leaderboardId;
return this;
}
/** The time span for the scores and ranks you're requesting. */
@com.google.api.client.util.Key
private java.lang.String timeSpan;
/** The time span for the scores and ranks you're requesting.
*/
public java.lang.String getTimeSpan() {
return timeSpan;
}
/** The time span for the scores and ranks you're requesting. */
public Get setTimeSpan(java.lang.String timeSpan) {
this.timeSpan = timeSpan;
return this;
}
/** The types of ranks to return. If the parameter is omitted, no ranks will be returned. */
@com.google.api.client.util.Key
private java.lang.String includeRankType;
/** The types of ranks to return. If the parameter is omitted, no ranks will be returned.
*/
public java.lang.String getIncludeRankType() {
return includeRankType;
}
/** The types of ranks to return. If the parameter is omitted, no ranks will be returned. */
public Get setIncludeRankType(java.lang.String includeRankType) {
this.includeRankType = includeRankType;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* The maximum number of leaderboard scores to return in the response. For any response, the
* actual number of leaderboard scores returned may be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of leaderboard scores to return in the response. For any response, the actual
number of leaderboard scores returned may be less than the specified maxResults.
[minimum: 1] [maximum: 30]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of leaderboard scores to return in the response. For any response, the
* actual number of leaderboard scores returned may be less than the specified maxResults.
*/
public Get setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public Get setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the scores in a leaderboard, starting from the top.
*
* Create a request for the method "scores.list".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param leaderboardId The ID of the leaderboard.
* @param collection The collection of scores you're requesting.
* @param timeSpan The time span for the scores and ranks you're requesting.
* @return the request
*/
public List list(java.lang.String leaderboardId, java.lang.String collection, java.lang.String timeSpan) throws java.io.IOException {
List result = new List(leaderboardId, collection, timeSpan);
initialize(result);
return result;
}
public class List extends GamesRequest {
private static final String REST_PATH = "leaderboards/{leaderboardId}/scores/{collection}";
/**
* Lists the scores in a leaderboard, starting from the top.
*
* Create a request for the method "scores.list".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param leaderboardId The ID of the leaderboard.
* @param collection The collection of scores you're requesting.
* @param timeSpan The time span for the scores and ranks you're requesting.
* @since 1.13
*/
protected List(java.lang.String leaderboardId, java.lang.String collection, java.lang.String timeSpan) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.LeaderboardScores.class);
this.leaderboardId = com.google.api.client.util.Preconditions.checkNotNull(leaderboardId, "Required parameter leaderboardId must be specified.");
this.collection = com.google.api.client.util.Preconditions.checkNotNull(collection, "Required parameter collection must be specified.");
this.timeSpan = com.google.api.client.util.Preconditions.checkNotNull(timeSpan, "Required parameter timeSpan must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The ID of the leaderboard. */
@com.google.api.client.util.Key
private java.lang.String leaderboardId;
/** The ID of the leaderboard.
*/
public java.lang.String getLeaderboardId() {
return leaderboardId;
}
/** The ID of the leaderboard. */
public List setLeaderboardId(java.lang.String leaderboardId) {
this.leaderboardId = leaderboardId;
return this;
}
/** The collection of scores you're requesting. */
@com.google.api.client.util.Key
private java.lang.String collection;
/** The collection of scores you're requesting.
*/
public java.lang.String getCollection() {
return collection;
}
/** The collection of scores you're requesting. */
public List setCollection(java.lang.String collection) {
this.collection = collection;
return this;
}
/** The time span for the scores and ranks you're requesting. */
@com.google.api.client.util.Key
private java.lang.String timeSpan;
/** The time span for the scores and ranks you're requesting.
*/
public java.lang.String getTimeSpan() {
return timeSpan;
}
/** The time span for the scores and ranks you're requesting. */
public List setTimeSpan(java.lang.String timeSpan) {
this.timeSpan = timeSpan;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* The maximum number of leaderboard scores to return in the response. For any response, the
* actual number of leaderboard scores returned may be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of leaderboard scores to return in the response. For any response, the actual
number of leaderboard scores returned may be less than the specified maxResults.
[minimum: 1] [maximum: 30]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of leaderboard scores to return in the response. For any response, the
* actual number of leaderboard scores returned may be less than the specified maxResults.
*/
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Lists the scores in a leaderboard around (and including) a player's score.
*
* Create a request for the method "scores.listWindow".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link ListWindow#execute()} method to invoke the remote operation.
*
* @param leaderboardId The ID of the leaderboard.
* @param collection The collection of scores you're requesting.
* @param timeSpan The time span for the scores and ranks you're requesting.
* @return the request
*/
public ListWindow listWindow(java.lang.String leaderboardId, java.lang.String collection, java.lang.String timeSpan) throws java.io.IOException {
ListWindow result = new ListWindow(leaderboardId, collection, timeSpan);
initialize(result);
return result;
}
public class ListWindow extends GamesRequest {
private static final String REST_PATH = "leaderboards/{leaderboardId}/window/{collection}";
/**
* Lists the scores in a leaderboard around (and including) a player's score.
*
* Create a request for the method "scores.listWindow".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link ListWindow#execute()} method to invoke the remote operation.
* {@link
* ListWindow#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param leaderboardId The ID of the leaderboard.
* @param collection The collection of scores you're requesting.
* @param timeSpan The time span for the scores and ranks you're requesting.
* @since 1.13
*/
protected ListWindow(java.lang.String leaderboardId, java.lang.String collection, java.lang.String timeSpan) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.LeaderboardScores.class);
this.leaderboardId = com.google.api.client.util.Preconditions.checkNotNull(leaderboardId, "Required parameter leaderboardId must be specified.");
this.collection = com.google.api.client.util.Preconditions.checkNotNull(collection, "Required parameter collection must be specified.");
this.timeSpan = com.google.api.client.util.Preconditions.checkNotNull(timeSpan, "Required parameter timeSpan must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListWindow setAlt(java.lang.String alt) {
return (ListWindow) super.setAlt(alt);
}
@Override
public ListWindow setFields(java.lang.String fields) {
return (ListWindow) super.setFields(fields);
}
@Override
public ListWindow setKey(java.lang.String key) {
return (ListWindow) super.setKey(key);
}
@Override
public ListWindow setOauthToken(java.lang.String oauthToken) {
return (ListWindow) super.setOauthToken(oauthToken);
}
@Override
public ListWindow setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListWindow) super.setPrettyPrint(prettyPrint);
}
@Override
public ListWindow setQuotaUser(java.lang.String quotaUser) {
return (ListWindow) super.setQuotaUser(quotaUser);
}
@Override
public ListWindow setUserIp(java.lang.String userIp) {
return (ListWindow) super.setUserIp(userIp);
}
/** The ID of the leaderboard. */
@com.google.api.client.util.Key
private java.lang.String leaderboardId;
/** The ID of the leaderboard.
*/
public java.lang.String getLeaderboardId() {
return leaderboardId;
}
/** The ID of the leaderboard. */
public ListWindow setLeaderboardId(java.lang.String leaderboardId) {
this.leaderboardId = leaderboardId;
return this;
}
/** The collection of scores you're requesting. */
@com.google.api.client.util.Key
private java.lang.String collection;
/** The collection of scores you're requesting.
*/
public java.lang.String getCollection() {
return collection;
}
/** The collection of scores you're requesting. */
public ListWindow setCollection(java.lang.String collection) {
this.collection = collection;
return this;
}
/** The time span for the scores and ranks you're requesting. */
@com.google.api.client.util.Key
private java.lang.String timeSpan;
/** The time span for the scores and ranks you're requesting.
*/
public java.lang.String getTimeSpan() {
return timeSpan;
}
/** The time span for the scores and ranks you're requesting. */
public ListWindow setTimeSpan(java.lang.String timeSpan) {
this.timeSpan = timeSpan;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public ListWindow setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* True if the top scores should be returned when the player is not in the leaderboard.
* Defaults to true.
*/
@com.google.api.client.util.Key
private java.lang.Boolean returnTopIfAbsent;
/** True if the top scores should be returned when the player is not in the leaderboard. Defaults to
true.
*/
public java.lang.Boolean getReturnTopIfAbsent() {
return returnTopIfAbsent;
}
/**
* True if the top scores should be returned when the player is not in the leaderboard.
* Defaults to true.
*/
public ListWindow setReturnTopIfAbsent(java.lang.Boolean returnTopIfAbsent) {
this.returnTopIfAbsent = returnTopIfAbsent;
return this;
}
/**
* The preferred number of scores to return above the player's score. More scores may be
* returned if the player is at the bottom of the leaderboard; fewer may be returned if the
* player is at the top. Must be less than or equal to maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer resultsAbove;
/** The preferred number of scores to return above the player's score. More scores may be returned if
the player is at the bottom of the leaderboard; fewer may be returned if the player is at the top.
Must be less than or equal to maxResults.
*/
public java.lang.Integer getResultsAbove() {
return resultsAbove;
}
/**
* The preferred number of scores to return above the player's score. More scores may be
* returned if the player is at the bottom of the leaderboard; fewer may be returned if the
* player is at the top. Must be less than or equal to maxResults.
*/
public ListWindow setResultsAbove(java.lang.Integer resultsAbove) {
this.resultsAbove = resultsAbove;
return this;
}
/**
* The maximum number of leaderboard scores to return in the response. For any response, the
* actual number of leaderboard scores returned may be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of leaderboard scores to return in the response. For any response, the actual
number of leaderboard scores returned may be less than the specified maxResults.
[minimum: 1] [maximum: 30]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of leaderboard scores to return in the response. For any response, the
* actual number of leaderboard scores returned may be less than the specified maxResults.
*/
public ListWindow setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public ListWindow setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListWindow set(String parameterName, Object value) {
return (ListWindow) super.set(parameterName, value);
}
}
/**
* Submits a score to the specified leaderboard.
*
* Create a request for the method "scores.submit".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Submit#execute()} method to invoke the remote operation.
*
* @param leaderboardId The ID of the leaderboard.
* @param score The score you're submitting. The submitted score is ignored if it is worse than a previously
* submitted score, where worse depends on the leaderboard sort order. The meaning of the
* score value depends on the leaderboard format type. For fixed-point, the score represents
* the raw value. For time, the score represents elapsed time in milliseconds. For currency,
* the score represents a value in micro units.
* @return the request
*/
public Submit submit(java.lang.String leaderboardId, java.lang.Long score) throws java.io.IOException {
Submit result = new Submit(leaderboardId, score);
initialize(result);
return result;
}
public class Submit extends GamesRequest {
private static final String REST_PATH = "leaderboards/{leaderboardId}/scores";
private final java.util.regex.Pattern SCORETAG_PATTERN =
java.util.regex.Pattern.compile("[a-zA-Z0-9-._~]{0,64}");
/**
* Submits a score to the specified leaderboard.
*
* Create a request for the method "scores.submit".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Submit#execute()} method to invoke the remote operation. {@link
* Submit#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param leaderboardId The ID of the leaderboard.
* @param score The score you're submitting. The submitted score is ignored if it is worse than a previously
* submitted score, where worse depends on the leaderboard sort order. The meaning of the
* score value depends on the leaderboard format type. For fixed-point, the score represents
* the raw value. For time, the score represents elapsed time in milliseconds. For currency,
* the score represents a value in micro units.
* @since 1.13
*/
protected Submit(java.lang.String leaderboardId, java.lang.Long score) {
super(Games.this, "POST", REST_PATH, null, com.google.api.services.games.model.PlayerScoreResponse.class);
this.leaderboardId = com.google.api.client.util.Preconditions.checkNotNull(leaderboardId, "Required parameter leaderboardId must be specified.");
this.score = com.google.api.client.util.Preconditions.checkNotNull(score, "Required parameter score must be specified.");
}
@Override
public Submit setAlt(java.lang.String alt) {
return (Submit) super.setAlt(alt);
}
@Override
public Submit setFields(java.lang.String fields) {
return (Submit) super.setFields(fields);
}
@Override
public Submit setKey(java.lang.String key) {
return (Submit) super.setKey(key);
}
@Override
public Submit setOauthToken(java.lang.String oauthToken) {
return (Submit) super.setOauthToken(oauthToken);
}
@Override
public Submit setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Submit) super.setPrettyPrint(prettyPrint);
}
@Override
public Submit setQuotaUser(java.lang.String quotaUser) {
return (Submit) super.setQuotaUser(quotaUser);
}
@Override
public Submit setUserIp(java.lang.String userIp) {
return (Submit) super.setUserIp(userIp);
}
/** The ID of the leaderboard. */
@com.google.api.client.util.Key
private java.lang.String leaderboardId;
/** The ID of the leaderboard.
*/
public java.lang.String getLeaderboardId() {
return leaderboardId;
}
/** The ID of the leaderboard. */
public Submit setLeaderboardId(java.lang.String leaderboardId) {
this.leaderboardId = leaderboardId;
return this;
}
/**
* The score you're submitting. The submitted score is ignored if it is worse than a
* previously submitted score, where worse depends on the leaderboard sort order. The meaning
* of the score value depends on the leaderboard format type. For fixed-point, the score
* represents the raw value. For time, the score represents elapsed time in milliseconds. For
* currency, the score represents a value in micro units.
*/
@com.google.api.client.util.Key
private java.lang.Long score;
/** The score you're submitting. The submitted score is ignored if it is worse than a previously
submitted score, where worse depends on the leaderboard sort order. The meaning of the score value
depends on the leaderboard format type. For fixed-point, the score represents the raw value. For
time, the score represents elapsed time in milliseconds. For currency, the score represents a value
in micro units.
*/
public java.lang.Long getScore() {
return score;
}
/**
* The score you're submitting. The submitted score is ignored if it is worse than a
* previously submitted score, where worse depends on the leaderboard sort order. The meaning
* of the score value depends on the leaderboard format type. For fixed-point, the score
* represents the raw value. For time, the score represents elapsed time in milliseconds. For
* currency, the score represents a value in micro units.
*/
public Submit setScore(java.lang.Long score) {
this.score = score;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Submit setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* Additional information about the score you're submitting. Values must contain no more than
* 64 URI-safe characters as defined by section 2.3 of RFC 3986.
*/
@com.google.api.client.util.Key
private java.lang.String scoreTag;
/** Additional information about the score you're submitting. Values must contain no more than 64 URI-
safe characters as defined by section 2.3 of RFC 3986.
*/
public java.lang.String getScoreTag() {
return scoreTag;
}
/**
* Additional information about the score you're submitting. Values must contain no more than
* 64 URI-safe characters as defined by section 2.3 of RFC 3986.
*/
public Submit setScoreTag(java.lang.String scoreTag) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(SCORETAG_PATTERN.matcher(scoreTag).matches(),
"Parameter scoreTag must conform to the pattern " +
"[a-zA-Z0-9-._~]{0,64}");
}
this.scoreTag = scoreTag;
return this;
}
@Override
public Submit set(String parameterName, Object value) {
return (Submit) super.set(parameterName, value);
}
}
/**
* Submits multiple scores to leaderboards.
*
* Create a request for the method "scores.submitMultiple".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link SubmitMultiple#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.games.model.PlayerScoreSubmissionList}
* @return the request
*/
public SubmitMultiple submitMultiple(com.google.api.services.games.model.PlayerScoreSubmissionList content) throws java.io.IOException {
SubmitMultiple result = new SubmitMultiple(content);
initialize(result);
return result;
}
public class SubmitMultiple extends GamesRequest {
private static final String REST_PATH = "leaderboards/scores";
/**
* Submits multiple scores to leaderboards.
*
* Create a request for the method "scores.submitMultiple".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link SubmitMultiple#execute()} method to invoke the remote operation.
* {@link SubmitMultiple#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param content the {@link com.google.api.services.games.model.PlayerScoreSubmissionList}
* @since 1.13
*/
protected SubmitMultiple(com.google.api.services.games.model.PlayerScoreSubmissionList content) {
super(Games.this, "POST", REST_PATH, content, com.google.api.services.games.model.PlayerScoreListResponse.class);
}
@Override
public SubmitMultiple setAlt(java.lang.String alt) {
return (SubmitMultiple) super.setAlt(alt);
}
@Override
public SubmitMultiple setFields(java.lang.String fields) {
return (SubmitMultiple) super.setFields(fields);
}
@Override
public SubmitMultiple setKey(java.lang.String key) {
return (SubmitMultiple) super.setKey(key);
}
@Override
public SubmitMultiple setOauthToken(java.lang.String oauthToken) {
return (SubmitMultiple) super.setOauthToken(oauthToken);
}
@Override
public SubmitMultiple setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SubmitMultiple) super.setPrettyPrint(prettyPrint);
}
@Override
public SubmitMultiple setQuotaUser(java.lang.String quotaUser) {
return (SubmitMultiple) super.setQuotaUser(quotaUser);
}
@Override
public SubmitMultiple setUserIp(java.lang.String userIp) {
return (SubmitMultiple) super.setUserIp(userIp);
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public SubmitMultiple setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public SubmitMultiple set(String parameterName, Object value) {
return (SubmitMultiple) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Snapshots collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.Snapshots.List request = games.snapshots().list(parameters ...)}
*
*
* @return the resource collection
*/
public Snapshots snapshots() {
return new Snapshots();
}
/**
* The "snapshots" collection of methods.
*/
public class Snapshots {
/**
* Retrieves the metadata for a given snapshot ID.
*
* Create a request for the method "snapshots.get".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param snapshotId The ID of the snapshot.
* @return the request
*/
public Get get(java.lang.String snapshotId) throws java.io.IOException {
Get result = new Get(snapshotId);
initialize(result);
return result;
}
public class Get extends GamesRequest {
private static final String REST_PATH = "snapshots/{snapshotId}";
/**
* Retrieves the metadata for a given snapshot ID.
*
* Create a request for the method "snapshots.get".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param snapshotId The ID of the snapshot.
* @since 1.13
*/
protected Get(java.lang.String snapshotId) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.Snapshot.class);
this.snapshotId = com.google.api.client.util.Preconditions.checkNotNull(snapshotId, "Required parameter snapshotId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the snapshot. */
@com.google.api.client.util.Key
private java.lang.String snapshotId;
/** The ID of the snapshot.
*/
public java.lang.String getSnapshotId() {
return snapshotId;
}
/** The ID of the snapshot. */
public Get setSnapshotId(java.lang.String snapshotId) {
this.snapshotId = snapshotId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves a list of snapshots created by your application for the player corresponding to the
* player ID.
*
* Create a request for the method "snapshots.list".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @return the request
*/
public List list(java.lang.String playerId) throws java.io.IOException {
List result = new List(playerId);
initialize(result);
return result;
}
public class List extends GamesRequest {
private static final String REST_PATH = "players/{playerId}/snapshots";
/**
* Retrieves a list of snapshots created by your application for the player corresponding to the
* player ID.
*
* Create a request for the method "snapshots.list".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param playerId A player ID. A value of me may be used in place of the authenticated player's ID.
* @since 1.13
*/
protected List(java.lang.String playerId) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.SnapshotListResponse.class);
this.playerId = com.google.api.client.util.Preconditions.checkNotNull(playerId, "Required parameter playerId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
@com.google.api.client.util.Key
private java.lang.String playerId;
/** A player ID. A value of me may be used in place of the authenticated player's ID.
*/
public java.lang.String getPlayerId() {
return playerId;
}
/** A player ID. A value of me may be used in place of the authenticated player's ID. */
public List setPlayerId(java.lang.String playerId) {
this.playerId = playerId;
return this;
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of snapshot resources to return in the response, used for paging. For
* any response, the actual number of snapshot resources returned may be less than the
* specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of snapshot resources to return in the response, used for paging. For any
response, the actual number of snapshot resources returned may be less than the specified
maxResults.
[minimum: 1] [maximum: 25]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of snapshot resources to return in the response, used for paging. For
* any response, the actual number of snapshot resources returned may be less than the
* specified maxResults.
*/
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the TurnBasedMatches collection.
*
* The typical use is:
*
* {@code Games games = new Games(...);}
* {@code Games.TurnBasedMatches.List request = games.turnBasedMatches().list(parameters ...)}
*
*
* @return the resource collection
*/
public TurnBasedMatches turnBasedMatches() {
return new TurnBasedMatches();
}
/**
* The "turnBasedMatches" collection of methods.
*/
public class TurnBasedMatches {
/**
* Cancel a turn-based match.
*
* Create a request for the method "turnBasedMatches.cancel".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @return the request
*/
public Cancel cancel(java.lang.String matchId) throws java.io.IOException {
Cancel result = new Cancel(matchId);
initialize(result);
return result;
}
public class Cancel extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}/cancel";
/**
* Cancel a turn-based match.
*
* Create a request for the method "turnBasedMatches.cancel".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation. {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @since 1.13
*/
protected Cancel(java.lang.String matchId) {
super(Games.this, "PUT", REST_PATH, null, Void.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUserIp(java.lang.String userIp) {
return (Cancel) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public Cancel setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Create a turn-based match.
*
* Create a request for the method "turnBasedMatches.create".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.games.model.TurnBasedMatchCreateRequest}
* @return the request
*/
public Create create(com.google.api.services.games.model.TurnBasedMatchCreateRequest content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/create";
/**
* Create a turn-based match.
*
* Create a request for the method "turnBasedMatches.create".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.games.model.TurnBasedMatchCreateRequest}
* @since 1.13
*/
protected Create(com.google.api.services.games.model.TurnBasedMatchCreateRequest content) {
super(Games.this, "POST", REST_PATH, content, com.google.api.services.games.model.TurnBasedMatch.class);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Create setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Decline an invitation to play a turn-based match.
*
* Create a request for the method "turnBasedMatches.decline".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Decline#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @return the request
*/
public Decline decline(java.lang.String matchId) throws java.io.IOException {
Decline result = new Decline(matchId);
initialize(result);
return result;
}
public class Decline extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}/decline";
/**
* Decline an invitation to play a turn-based match.
*
* Create a request for the method "turnBasedMatches.decline".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Decline#execute()} method to invoke the remote operation.
* {@link
* Decline#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @since 1.13
*/
protected Decline(java.lang.String matchId) {
super(Games.this, "PUT", REST_PATH, null, com.google.api.services.games.model.TurnBasedMatch.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
}
@Override
public Decline setAlt(java.lang.String alt) {
return (Decline) super.setAlt(alt);
}
@Override
public Decline setFields(java.lang.String fields) {
return (Decline) super.setFields(fields);
}
@Override
public Decline setKey(java.lang.String key) {
return (Decline) super.setKey(key);
}
@Override
public Decline setOauthToken(java.lang.String oauthToken) {
return (Decline) super.setOauthToken(oauthToken);
}
@Override
public Decline setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Decline) super.setPrettyPrint(prettyPrint);
}
@Override
public Decline setQuotaUser(java.lang.String quotaUser) {
return (Decline) super.setQuotaUser(quotaUser);
}
@Override
public Decline setUserIp(java.lang.String userIp) {
return (Decline) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public Decline setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Decline setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Decline set(String parameterName, Object value) {
return (Decline) super.set(parameterName, value);
}
}
/**
* Dismiss a turn-based match from the match list. The match will no longer show up in the list and
* will not generate notifications.
*
* Create a request for the method "turnBasedMatches.dismiss".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @return the request
*/
public Dismiss dismiss(java.lang.String matchId) throws java.io.IOException {
Dismiss result = new Dismiss(matchId);
initialize(result);
return result;
}
public class Dismiss extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}/dismiss";
/**
* Dismiss a turn-based match from the match list. The match will no longer show up in the list
* and will not generate notifications.
*
* Create a request for the method "turnBasedMatches.dismiss".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Dismiss#execute()} method to invoke the remote operation.
* {@link
* Dismiss#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @since 1.13
*/
protected Dismiss(java.lang.String matchId) {
super(Games.this, "PUT", REST_PATH, null, Void.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
}
@Override
public Dismiss setAlt(java.lang.String alt) {
return (Dismiss) super.setAlt(alt);
}
@Override
public Dismiss setFields(java.lang.String fields) {
return (Dismiss) super.setFields(fields);
}
@Override
public Dismiss setKey(java.lang.String key) {
return (Dismiss) super.setKey(key);
}
@Override
public Dismiss setOauthToken(java.lang.String oauthToken) {
return (Dismiss) super.setOauthToken(oauthToken);
}
@Override
public Dismiss setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Dismiss) super.setPrettyPrint(prettyPrint);
}
@Override
public Dismiss setQuotaUser(java.lang.String quotaUser) {
return (Dismiss) super.setQuotaUser(quotaUser);
}
@Override
public Dismiss setUserIp(java.lang.String userIp) {
return (Dismiss) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public Dismiss setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
@Override
public Dismiss set(String parameterName, Object value) {
return (Dismiss) super.set(parameterName, value);
}
}
/**
* Finish a turn-based match. Each player should make this call once, after all results are in. Only
* the player whose turn it is may make the first call to Finish, and can pass in the final match
* state.
*
* Create a request for the method "turnBasedMatches.finish".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Finish#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @param content the {@link com.google.api.services.games.model.TurnBasedMatchResults}
* @return the request
*/
public Finish finish(java.lang.String matchId, com.google.api.services.games.model.TurnBasedMatchResults content) throws java.io.IOException {
Finish result = new Finish(matchId, content);
initialize(result);
return result;
}
public class Finish extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}/finish";
/**
* Finish a turn-based match. Each player should make this call once, after all results are in.
* Only the player whose turn it is may make the first call to Finish, and can pass in the final
* match state.
*
* Create a request for the method "turnBasedMatches.finish".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Finish#execute()} method to invoke the remote operation. {@link
* Finish#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @param content the {@link com.google.api.services.games.model.TurnBasedMatchResults}
* @since 1.13
*/
protected Finish(java.lang.String matchId, com.google.api.services.games.model.TurnBasedMatchResults content) {
super(Games.this, "PUT", REST_PATH, content, com.google.api.services.games.model.TurnBasedMatch.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
}
@Override
public Finish setAlt(java.lang.String alt) {
return (Finish) super.setAlt(alt);
}
@Override
public Finish setFields(java.lang.String fields) {
return (Finish) super.setFields(fields);
}
@Override
public Finish setKey(java.lang.String key) {
return (Finish) super.setKey(key);
}
@Override
public Finish setOauthToken(java.lang.String oauthToken) {
return (Finish) super.setOauthToken(oauthToken);
}
@Override
public Finish setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Finish) super.setPrettyPrint(prettyPrint);
}
@Override
public Finish setQuotaUser(java.lang.String quotaUser) {
return (Finish) super.setQuotaUser(quotaUser);
}
@Override
public Finish setUserIp(java.lang.String userIp) {
return (Finish) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public Finish setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Finish setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Finish set(String parameterName, Object value) {
return (Finish) super.set(parameterName, value);
}
}
/**
* Get the data for a turn-based match.
*
* Create a request for the method "turnBasedMatches.get".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @return the request
*/
public Get get(java.lang.String matchId) throws java.io.IOException {
Get result = new Get(matchId);
initialize(result);
return result;
}
public class Get extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}";
/**
* Get the data for a turn-based match.
*
* Create a request for the method "turnBasedMatches.get".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @since 1.13
*/
protected Get(java.lang.String matchId) {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.TurnBasedMatch.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public Get setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Get setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/** Get match data along with metadata. */
@com.google.api.client.util.Key
private java.lang.Boolean includeMatchData;
/** Get match data along with metadata.
*/
public java.lang.Boolean getIncludeMatchData() {
return includeMatchData;
}
/** Get match data along with metadata. */
public Get setIncludeMatchData(java.lang.Boolean includeMatchData) {
this.includeMatchData = includeMatchData;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Join a turn-based match.
*
* Create a request for the method "turnBasedMatches.join".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Join#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @return the request
*/
public Join join(java.lang.String matchId) throws java.io.IOException {
Join result = new Join(matchId);
initialize(result);
return result;
}
public class Join extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}/join";
/**
* Join a turn-based match.
*
* Create a request for the method "turnBasedMatches.join".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Join#execute()} method to invoke the remote operation. {@link
* Join#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @since 1.13
*/
protected Join(java.lang.String matchId) {
super(Games.this, "PUT", REST_PATH, null, com.google.api.services.games.model.TurnBasedMatch.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
}
@Override
public Join setAlt(java.lang.String alt) {
return (Join) super.setAlt(alt);
}
@Override
public Join setFields(java.lang.String fields) {
return (Join) super.setFields(fields);
}
@Override
public Join setKey(java.lang.String key) {
return (Join) super.setKey(key);
}
@Override
public Join setOauthToken(java.lang.String oauthToken) {
return (Join) super.setOauthToken(oauthToken);
}
@Override
public Join setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Join) super.setPrettyPrint(prettyPrint);
}
@Override
public Join setQuotaUser(java.lang.String quotaUser) {
return (Join) super.setQuotaUser(quotaUser);
}
@Override
public Join setUserIp(java.lang.String userIp) {
return (Join) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public Join setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Join setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Join set(String parameterName, Object value) {
return (Join) super.set(parameterName, value);
}
}
/**
* Leave a turn-based match when it is not the current player's turn, without canceling the match.
*
* Create a request for the method "turnBasedMatches.leave".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Leave#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @return the request
*/
public Leave leave(java.lang.String matchId) throws java.io.IOException {
Leave result = new Leave(matchId);
initialize(result);
return result;
}
public class Leave extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}/leave";
/**
* Leave a turn-based match when it is not the current player's turn, without canceling the match.
*
* Create a request for the method "turnBasedMatches.leave".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Leave#execute()} method to invoke the remote operation. {@link
* Leave#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @since 1.13
*/
protected Leave(java.lang.String matchId) {
super(Games.this, "PUT", REST_PATH, null, com.google.api.services.games.model.TurnBasedMatch.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
}
@Override
public Leave setAlt(java.lang.String alt) {
return (Leave) super.setAlt(alt);
}
@Override
public Leave setFields(java.lang.String fields) {
return (Leave) super.setFields(fields);
}
@Override
public Leave setKey(java.lang.String key) {
return (Leave) super.setKey(key);
}
@Override
public Leave setOauthToken(java.lang.String oauthToken) {
return (Leave) super.setOauthToken(oauthToken);
}
@Override
public Leave setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Leave) super.setPrettyPrint(prettyPrint);
}
@Override
public Leave setQuotaUser(java.lang.String quotaUser) {
return (Leave) super.setQuotaUser(quotaUser);
}
@Override
public Leave setUserIp(java.lang.String userIp) {
return (Leave) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public Leave setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Leave setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Leave set(String parameterName, Object value) {
return (Leave) super.set(parameterName, value);
}
}
/**
* Leave a turn-based match during the current player's turn, without canceling the match.
*
* Create a request for the method "turnBasedMatches.leaveTurn".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link LeaveTurn#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @param matchVersion The version of the match being updated.
* @return the request
*/
public LeaveTurn leaveTurn(java.lang.String matchId, java.lang.Integer matchVersion) throws java.io.IOException {
LeaveTurn result = new LeaveTurn(matchId, matchVersion);
initialize(result);
return result;
}
public class LeaveTurn extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}/leaveTurn";
/**
* Leave a turn-based match during the current player's turn, without canceling the match.
*
* Create a request for the method "turnBasedMatches.leaveTurn".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link LeaveTurn#execute()} method to invoke the remote operation.
* {@link
* LeaveTurn#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @param matchVersion The version of the match being updated.
* @since 1.13
*/
protected LeaveTurn(java.lang.String matchId, java.lang.Integer matchVersion) {
super(Games.this, "PUT", REST_PATH, null, com.google.api.services.games.model.TurnBasedMatch.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
this.matchVersion = com.google.api.client.util.Preconditions.checkNotNull(matchVersion, "Required parameter matchVersion must be specified.");
}
@Override
public LeaveTurn setAlt(java.lang.String alt) {
return (LeaveTurn) super.setAlt(alt);
}
@Override
public LeaveTurn setFields(java.lang.String fields) {
return (LeaveTurn) super.setFields(fields);
}
@Override
public LeaveTurn setKey(java.lang.String key) {
return (LeaveTurn) super.setKey(key);
}
@Override
public LeaveTurn setOauthToken(java.lang.String oauthToken) {
return (LeaveTurn) super.setOauthToken(oauthToken);
}
@Override
public LeaveTurn setPrettyPrint(java.lang.Boolean prettyPrint) {
return (LeaveTurn) super.setPrettyPrint(prettyPrint);
}
@Override
public LeaveTurn setQuotaUser(java.lang.String quotaUser) {
return (LeaveTurn) super.setQuotaUser(quotaUser);
}
@Override
public LeaveTurn setUserIp(java.lang.String userIp) {
return (LeaveTurn) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public LeaveTurn setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
/** The version of the match being updated. */
@com.google.api.client.util.Key
private java.lang.Integer matchVersion;
/** The version of the match being updated.
*/
public java.lang.Integer getMatchVersion() {
return matchVersion;
}
/** The version of the match being updated. */
public LeaveTurn setMatchVersion(java.lang.Integer matchVersion) {
this.matchVersion = matchVersion;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public LeaveTurn setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* The ID of another participant who should take their turn next. If not set, the match will
* wait for other player(s) to join via automatching; this is only valid if automatch criteria
* is set on the match with remaining slots for automatched players.
*/
@com.google.api.client.util.Key
private java.lang.String pendingParticipantId;
/** The ID of another participant who should take their turn next. If not set, the match will wait for
other player(s) to join via automatching; this is only valid if automatch criteria is set on the
match with remaining slots for automatched players.
*/
public java.lang.String getPendingParticipantId() {
return pendingParticipantId;
}
/**
* The ID of another participant who should take their turn next. If not set, the match will
* wait for other player(s) to join via automatching; this is only valid if automatch criteria
* is set on the match with remaining slots for automatched players.
*/
public LeaveTurn setPendingParticipantId(java.lang.String pendingParticipantId) {
this.pendingParticipantId = pendingParticipantId;
return this;
}
@Override
public LeaveTurn set(String parameterName, Object value) {
return (LeaveTurn) super.set(parameterName, value);
}
}
/**
* Returns turn-based matches the player is or was involved in.
*
* Create a request for the method "turnBasedMatches.list".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches";
/**
* Returns turn-based matches the player is or was involved in.
*
* Create a request for the method "turnBasedMatches.list".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.TurnBasedMatchList.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of completed or canceled matches to return in the response. If not set,
* all matches returned could be completed or canceled.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxCompletedMatches;
/** The maximum number of completed or canceled matches to return in the response. If not set, all
matches returned could be completed or canceled.
[minimum: 0] [maximum: 500]
*/
public java.lang.Integer getMaxCompletedMatches() {
return maxCompletedMatches;
}
/**
* The maximum number of completed or canceled matches to return in the response. If not set,
* all matches returned could be completed or canceled.
*/
public List setMaxCompletedMatches(java.lang.Integer maxCompletedMatches) {
this.maxCompletedMatches = maxCompletedMatches;
return this;
}
/**
* The maximum number of matches to return in the response, used for paging. For any response,
* the actual number of matches to return may be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of matches to return in the response, used for paging. For any response, the
actual number of matches to return may be less than the specified maxResults.
[minimum: 1] [maximum: 500]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of matches to return in the response, used for paging. For any response,
* the actual number of matches to return may be less than the specified maxResults.
*/
public List setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public List setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* True if match data should be returned in the response. Note that not all data will
* necessarily be returned if include_match_data is true; the server may decide to only return
* data for some of the matches to limit download size for the client. The remainder of the
* data for these matches will be retrievable on request.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeMatchData;
/** True if match data should be returned in the response. Note that not all data will necessarily be
returned if include_match_data is true; the server may decide to only return data for some of the
matches to limit download size for the client. The remainder of the data for these matches will be
retrievable on request.
*/
public java.lang.Boolean getIncludeMatchData() {
return includeMatchData;
}
/**
* True if match data should be returned in the response. Note that not all data will
* necessarily be returned if include_match_data is true; the server may decide to only return
* data for some of the matches to limit download size for the client. The remainder of the
* data for these matches will be retrievable on request.
*/
public List setIncludeMatchData(java.lang.Boolean includeMatchData) {
this.includeMatchData = includeMatchData;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Create a rematch of a match that was previously completed, with the same participants. This can
* be called by only one player on a match still in their list; the player must have called Finish
* first. Returns the newly created match; it will be the caller's turn.
*
* Create a request for the method "turnBasedMatches.rematch".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Rematch#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @return the request
*/
public Rematch rematch(java.lang.String matchId) throws java.io.IOException {
Rematch result = new Rematch(matchId);
initialize(result);
return result;
}
public class Rematch extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}/rematch";
/**
* Create a rematch of a match that was previously completed, with the same participants. This can
* be called by only one player on a match still in their list; the player must have called Finish
* first. Returns the newly created match; it will be the caller's turn.
*
* Create a request for the method "turnBasedMatches.rematch".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Rematch#execute()} method to invoke the remote operation.
* {@link
* Rematch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @since 1.13
*/
protected Rematch(java.lang.String matchId) {
super(Games.this, "POST", REST_PATH, null, com.google.api.services.games.model.TurnBasedMatchRematch.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
}
@Override
public Rematch setAlt(java.lang.String alt) {
return (Rematch) super.setAlt(alt);
}
@Override
public Rematch setFields(java.lang.String fields) {
return (Rematch) super.setFields(fields);
}
@Override
public Rematch setKey(java.lang.String key) {
return (Rematch) super.setKey(key);
}
@Override
public Rematch setOauthToken(java.lang.String oauthToken) {
return (Rematch) super.setOauthToken(oauthToken);
}
@Override
public Rematch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Rematch) super.setPrettyPrint(prettyPrint);
}
@Override
public Rematch setQuotaUser(java.lang.String quotaUser) {
return (Rematch) super.setQuotaUser(quotaUser);
}
@Override
public Rematch setUserIp(java.lang.String userIp) {
return (Rematch) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public Rematch setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
/**
* A randomly generated numeric ID for each request specified by the caller. This number is
* used at the server to ensure that the request is handled correctly across retries.
*/
@com.google.api.client.util.Key
private java.lang.Long requestId;
/** A randomly generated numeric ID for each request specified by the caller. This number is used at
the server to ensure that the request is handled correctly across retries.
*/
public java.lang.Long getRequestId() {
return requestId;
}
/**
* A randomly generated numeric ID for each request specified by the caller. This number is
* used at the server to ensure that the request is handled correctly across retries.
*/
public Rematch setRequestId(java.lang.Long requestId) {
this.requestId = requestId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Rematch setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public Rematch set(String parameterName, Object value) {
return (Rematch) super.set(parameterName, value);
}
}
/**
* Returns turn-based matches the player is or was involved in that changed since the last sync
* call, with the least recent changes coming first. Matches that should be removed from the local
* cache will have a status of MATCH_DELETED.
*
* Create a request for the method "turnBasedMatches.sync".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link Sync#execute()} method to invoke the remote operation.
*
* @return the request
*/
public Sync sync() throws java.io.IOException {
Sync result = new Sync();
initialize(result);
return result;
}
public class Sync extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/sync";
/**
* Returns turn-based matches the player is or was involved in that changed since the last sync
* call, with the least recent changes coming first. Matches that should be removed from the local
* cache will have a status of MATCH_DELETED.
*
* Create a request for the method "turnBasedMatches.sync".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link Sync#execute()} method to invoke the remote operation. {@link
* Sync#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected Sync() {
super(Games.this, "GET", REST_PATH, null, com.google.api.services.games.model.TurnBasedMatchSync.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Sync setAlt(java.lang.String alt) {
return (Sync) super.setAlt(alt);
}
@Override
public Sync setFields(java.lang.String fields) {
return (Sync) super.setFields(fields);
}
@Override
public Sync setKey(java.lang.String key) {
return (Sync) super.setKey(key);
}
@Override
public Sync setOauthToken(java.lang.String oauthToken) {
return (Sync) super.setOauthToken(oauthToken);
}
@Override
public Sync setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Sync) super.setPrettyPrint(prettyPrint);
}
@Override
public Sync setQuotaUser(java.lang.String quotaUser) {
return (Sync) super.setQuotaUser(quotaUser);
}
@Override
public Sync setUserIp(java.lang.String userIp) {
return (Sync) super.setUserIp(userIp);
}
/** The token returned by the previous request. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The token returned by the previous request.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The token returned by the previous request. */
public Sync setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of completed or canceled matches to return in the response. If not set,
* all matches returned could be completed or canceled.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxCompletedMatches;
/** The maximum number of completed or canceled matches to return in the response. If not set, all
matches returned could be completed or canceled.
[minimum: 0] [maximum: 500]
*/
public java.lang.Integer getMaxCompletedMatches() {
return maxCompletedMatches;
}
/**
* The maximum number of completed or canceled matches to return in the response. If not set,
* all matches returned could be completed or canceled.
*/
public Sync setMaxCompletedMatches(java.lang.Integer maxCompletedMatches) {
this.maxCompletedMatches = maxCompletedMatches;
return this;
}
/**
* The maximum number of matches to return in the response, used for paging. For any response,
* the actual number of matches to return may be less than the specified maxResults.
*/
@com.google.api.client.util.Key
private java.lang.Integer maxResults;
/** The maximum number of matches to return in the response, used for paging. For any response, the
actual number of matches to return may be less than the specified maxResults.
[minimum: 1] [maximum: 500]
*/
public java.lang.Integer getMaxResults() {
return maxResults;
}
/**
* The maximum number of matches to return in the response, used for paging. For any response,
* the actual number of matches to return may be less than the specified maxResults.
*/
public Sync setMaxResults(java.lang.Integer maxResults) {
this.maxResults = maxResults;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public Sync setLanguage(java.lang.String language) {
this.language = language;
return this;
}
/**
* True if match data should be returned in the response. Note that not all data will
* necessarily be returned if include_match_data is true; the server may decide to only return
* data for some of the matches to limit download size for the client. The remainder of the
* data for these matches will be retrievable on request.
*/
@com.google.api.client.util.Key
private java.lang.Boolean includeMatchData;
/** True if match data should be returned in the response. Note that not all data will necessarily be
returned if include_match_data is true; the server may decide to only return data for some of the
matches to limit download size for the client. The remainder of the data for these matches will be
retrievable on request.
*/
public java.lang.Boolean getIncludeMatchData() {
return includeMatchData;
}
/**
* True if match data should be returned in the response. Note that not all data will
* necessarily be returned if include_match_data is true; the server may decide to only return
* data for some of the matches to limit download size for the client. The remainder of the
* data for these matches will be retrievable on request.
*/
public Sync setIncludeMatchData(java.lang.Boolean includeMatchData) {
this.includeMatchData = includeMatchData;
return this;
}
@Override
public Sync set(String parameterName, Object value) {
return (Sync) super.set(parameterName, value);
}
}
/**
* Commit the results of a player turn.
*
* Create a request for the method "turnBasedMatches.takeTurn".
*
* This request holds the parameters needed by the games server. After setting any optional
* parameters, call the {@link TakeTurn#execute()} method to invoke the remote operation.
*
* @param matchId The ID of the match.
* @param content the {@link com.google.api.services.games.model.TurnBasedMatchTurn}
* @return the request
*/
public TakeTurn takeTurn(java.lang.String matchId, com.google.api.services.games.model.TurnBasedMatchTurn content) throws java.io.IOException {
TakeTurn result = new TakeTurn(matchId, content);
initialize(result);
return result;
}
public class TakeTurn extends GamesRequest {
private static final String REST_PATH = "turnbasedmatches/{matchId}/turn";
/**
* Commit the results of a player turn.
*
* Create a request for the method "turnBasedMatches.takeTurn".
*
* This request holds the parameters needed by the the games server. After setting any optional
* parameters, call the {@link TakeTurn#execute()} method to invoke the remote operation.
* {@link
* TakeTurn#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param matchId The ID of the match.
* @param content the {@link com.google.api.services.games.model.TurnBasedMatchTurn}
* @since 1.13
*/
protected TakeTurn(java.lang.String matchId, com.google.api.services.games.model.TurnBasedMatchTurn content) {
super(Games.this, "PUT", REST_PATH, content, com.google.api.services.games.model.TurnBasedMatch.class);
this.matchId = com.google.api.client.util.Preconditions.checkNotNull(matchId, "Required parameter matchId must be specified.");
}
@Override
public TakeTurn setAlt(java.lang.String alt) {
return (TakeTurn) super.setAlt(alt);
}
@Override
public TakeTurn setFields(java.lang.String fields) {
return (TakeTurn) super.setFields(fields);
}
@Override
public TakeTurn setKey(java.lang.String key) {
return (TakeTurn) super.setKey(key);
}
@Override
public TakeTurn setOauthToken(java.lang.String oauthToken) {
return (TakeTurn) super.setOauthToken(oauthToken);
}
@Override
public TakeTurn setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TakeTurn) super.setPrettyPrint(prettyPrint);
}
@Override
public TakeTurn setQuotaUser(java.lang.String quotaUser) {
return (TakeTurn) super.setQuotaUser(quotaUser);
}
@Override
public TakeTurn setUserIp(java.lang.String userIp) {
return (TakeTurn) super.setUserIp(userIp);
}
/** The ID of the match. */
@com.google.api.client.util.Key
private java.lang.String matchId;
/** The ID of the match.
*/
public java.lang.String getMatchId() {
return matchId;
}
/** The ID of the match. */
public TakeTurn setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
/** The preferred language to use for strings returned by this method. */
@com.google.api.client.util.Key
private java.lang.String language;
/** The preferred language to use for strings returned by this method.
*/
public java.lang.String getLanguage() {
return language;
}
/** The preferred language to use for strings returned by this method. */
public TakeTurn setLanguage(java.lang.String language) {
this.language = language;
return this;
}
@Override
public TakeTurn set(String parameterName, Object value) {
return (TakeTurn) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link Games}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link Games}. */
@Override
public Games build() {
return new Games(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link GamesRequestInitializer}.
*
* @since 1.12
*/
public Builder setGamesRequestInitializer(
GamesRequestInitializer gamesRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(gamesRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}