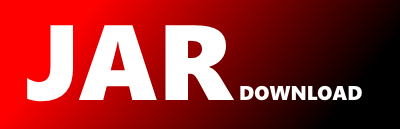
com.google.api.services.games.model.Room Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-01-14 17:53:03 UTC)
* on 2015-03-12 at 21:37:46 UTC
* Modify at your own risk.
*/
package com.google.api.services.games.model;
/**
* This is a JSON template for a room resource object.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Play Game Services API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Room extends com.google.api.client.json.GenericJson {
/**
* The ID of the application being played.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String applicationId;
/**
* Criteria for auto-matching players into this room.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RoomAutoMatchingCriteria autoMatchingCriteria;
/**
* Auto-matching status for this room. Not set if the room is not currently in the auto-matching
* queue.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RoomAutoMatchStatus autoMatchingStatus;
/**
* Details about the room creation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RoomModification creationDetails;
/**
* This short description is generated by our servers and worded relative to the player requesting
* the room. It is intended to be displayed when the room is shown in a list (that is, an
* invitation to a room.)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The ID of the participant that invited the user to the room. Not set if the user was not
* invited to the room.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String inviterId;
/**
* Uniquely identifies the type of this resource. Value is always the fixed string games#room.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Details about the last update to the room.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private RoomModification lastUpdateDetails;
/**
* The participants involved in the room, along with their statuses. Includes participants who
* have left or declined invitations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List participants;
static {
// hack to force ProGuard to consider RoomParticipant used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(RoomParticipant.class);
}
/**
* Globally unique ID for a room.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String roomId;
/**
* The version of the room status: an increasing counter, used by the client to ignore out-of-
* order updates to room status.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer roomStatusVersion;
/**
* The status of the room. Possible values are: - "ROOM_INVITING" - One or more players have
* been invited and not responded. - "ROOM_AUTO_MATCHING" - One or more slots need to be filled
* by auto-matching. - "ROOM_CONNECTING" - Players have joined and are connecting to each other
* (either before or after auto-matching). - "ROOM_ACTIVE" - All players have joined and
* connected to each other. - "ROOM_DELETED" - The room should no longer be shown on the client.
* Returned in sync calls when a player joins a room (as a tombstone), or for rooms where all
* joined participants have left.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String status;
/**
* The variant / mode of the application being played; can be any integer value, or left blank.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer variant;
/**
* The ID of the application being played.
* @return value or {@code null} for none
*/
public java.lang.String getApplicationId() {
return applicationId;
}
/**
* The ID of the application being played.
* @param applicationId applicationId or {@code null} for none
*/
public Room setApplicationId(java.lang.String applicationId) {
this.applicationId = applicationId;
return this;
}
/**
* Criteria for auto-matching players into this room.
* @return value or {@code null} for none
*/
public RoomAutoMatchingCriteria getAutoMatchingCriteria() {
return autoMatchingCriteria;
}
/**
* Criteria for auto-matching players into this room.
* @param autoMatchingCriteria autoMatchingCriteria or {@code null} for none
*/
public Room setAutoMatchingCriteria(RoomAutoMatchingCriteria autoMatchingCriteria) {
this.autoMatchingCriteria = autoMatchingCriteria;
return this;
}
/**
* Auto-matching status for this room. Not set if the room is not currently in the auto-matching
* queue.
* @return value or {@code null} for none
*/
public RoomAutoMatchStatus getAutoMatchingStatus() {
return autoMatchingStatus;
}
/**
* Auto-matching status for this room. Not set if the room is not currently in the auto-matching
* queue.
* @param autoMatchingStatus autoMatchingStatus or {@code null} for none
*/
public Room setAutoMatchingStatus(RoomAutoMatchStatus autoMatchingStatus) {
this.autoMatchingStatus = autoMatchingStatus;
return this;
}
/**
* Details about the room creation.
* @return value or {@code null} for none
*/
public RoomModification getCreationDetails() {
return creationDetails;
}
/**
* Details about the room creation.
* @param creationDetails creationDetails or {@code null} for none
*/
public Room setCreationDetails(RoomModification creationDetails) {
this.creationDetails = creationDetails;
return this;
}
/**
* This short description is generated by our servers and worded relative to the player requesting
* the room. It is intended to be displayed when the room is shown in a list (that is, an
* invitation to a room.)
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* This short description is generated by our servers and worded relative to the player requesting
* the room. It is intended to be displayed when the room is shown in a list (that is, an
* invitation to a room.)
* @param description description or {@code null} for none
*/
public Room setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The ID of the participant that invited the user to the room. Not set if the user was not
* invited to the room.
* @return value or {@code null} for none
*/
public java.lang.String getInviterId() {
return inviterId;
}
/**
* The ID of the participant that invited the user to the room. Not set if the user was not
* invited to the room.
* @param inviterId inviterId or {@code null} for none
*/
public Room setInviterId(java.lang.String inviterId) {
this.inviterId = inviterId;
return this;
}
/**
* Uniquely identifies the type of this resource. Value is always the fixed string games#room.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Uniquely identifies the type of this resource. Value is always the fixed string games#room.
* @param kind kind or {@code null} for none
*/
public Room setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Details about the last update to the room.
* @return value or {@code null} for none
*/
public RoomModification getLastUpdateDetails() {
return lastUpdateDetails;
}
/**
* Details about the last update to the room.
* @param lastUpdateDetails lastUpdateDetails or {@code null} for none
*/
public Room setLastUpdateDetails(RoomModification lastUpdateDetails) {
this.lastUpdateDetails = lastUpdateDetails;
return this;
}
/**
* The participants involved in the room, along with their statuses. Includes participants who
* have left or declined invitations.
* @return value or {@code null} for none
*/
public java.util.List getParticipants() {
return participants;
}
/**
* The participants involved in the room, along with their statuses. Includes participants who
* have left or declined invitations.
* @param participants participants or {@code null} for none
*/
public Room setParticipants(java.util.List participants) {
this.participants = participants;
return this;
}
/**
* Globally unique ID for a room.
* @return value or {@code null} for none
*/
public java.lang.String getRoomId() {
return roomId;
}
/**
* Globally unique ID for a room.
* @param roomId roomId or {@code null} for none
*/
public Room setRoomId(java.lang.String roomId) {
this.roomId = roomId;
return this;
}
/**
* The version of the room status: an increasing counter, used by the client to ignore out-of-
* order updates to room status.
* @return value or {@code null} for none
*/
public java.lang.Integer getRoomStatusVersion() {
return roomStatusVersion;
}
/**
* The version of the room status: an increasing counter, used by the client to ignore out-of-
* order updates to room status.
* @param roomStatusVersion roomStatusVersion or {@code null} for none
*/
public Room setRoomStatusVersion(java.lang.Integer roomStatusVersion) {
this.roomStatusVersion = roomStatusVersion;
return this;
}
/**
* The status of the room. Possible values are: - "ROOM_INVITING" - One or more players have
* been invited and not responded. - "ROOM_AUTO_MATCHING" - One or more slots need to be filled
* by auto-matching. - "ROOM_CONNECTING" - Players have joined and are connecting to each other
* (either before or after auto-matching). - "ROOM_ACTIVE" - All players have joined and
* connected to each other. - "ROOM_DELETED" - The room should no longer be shown on the client.
* Returned in sync calls when a player joins a room (as a tombstone), or for rooms where all
* joined participants have left.
* @return value or {@code null} for none
*/
public java.lang.String getStatus() {
return status;
}
/**
* The status of the room. Possible values are: - "ROOM_INVITING" - One or more players have
* been invited and not responded. - "ROOM_AUTO_MATCHING" - One or more slots need to be filled
* by auto-matching. - "ROOM_CONNECTING" - Players have joined and are connecting to each other
* (either before or after auto-matching). - "ROOM_ACTIVE" - All players have joined and
* connected to each other. - "ROOM_DELETED" - The room should no longer be shown on the client.
* Returned in sync calls when a player joins a room (as a tombstone), or for rooms where all
* joined participants have left.
* @param status status or {@code null} for none
*/
public Room setStatus(java.lang.String status) {
this.status = status;
return this;
}
/**
* The variant / mode of the application being played; can be any integer value, or left blank.
* @return value or {@code null} for none
*/
public java.lang.Integer getVariant() {
return variant;
}
/**
* The variant / mode of the application being played; can be any integer value, or left blank.
* @param variant variant or {@code null} for none
*/
public Room setVariant(java.lang.Integer variant) {
this.variant = variant;
return this;
}
@Override
public Room set(String fieldName, Object value) {
return (Room) super.set(fieldName, value);
}
@Override
public Room clone() {
return (Room) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy