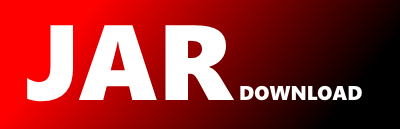
com.google.api.services.games.model.TurnBasedMatch Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://code.google.com/p/google-apis-client-generator/
* (build: 2015-01-14 17:53:03 UTC)
* on 2015-03-12 at 21:37:46 UTC
* Modify at your own risk.
*/
package com.google.api.services.games.model;
/**
* This is a JSON template for a turn-based match resource object.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Play Game Services API. For a detailed
* explanation see:
* http://code.google.com/p/google-http-java-client/wiki/JSON
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class TurnBasedMatch extends com.google.api.client.json.GenericJson {
/**
* The ID of the application being played.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String applicationId;
/**
* Criteria for auto-matching players into this match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TurnBasedAutoMatchingCriteria autoMatchingCriteria;
/**
* Details about the match creation.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TurnBasedMatchModification creationDetails;
/**
* The data / game state for this match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TurnBasedMatchData data;
/**
* This short description is generated by our servers based on turn state and is localized and
* worded relative to the player requesting the match. It is intended to be displayed when the
* match is shown in a list.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The ID of the participant that invited the user to the match. Not set if the user was not
* invited to the match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String inviterId;
/**
* Uniquely identifies the type of this resource. Value is always the fixed string
* games#turnBasedMatch.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* Details about the last update to the match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TurnBasedMatchModification lastUpdateDetails;
/**
* Globally unique ID for a turn-based match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String matchId;
/**
* The number of the match in a chain of rematches. Will be set to 1 for the first match and
* incremented by 1 for each rematch.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer matchNumber;
/**
* The version of this match: an increasing counter, used to avoid out-of-date updates to the
* match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer matchVersion;
/**
* The participants involved in the match, along with their statuses. Includes participants who
* have left or declined invitations.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List participants;
static {
// hack to force ProGuard to consider TurnBasedMatchParticipant used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(TurnBasedMatchParticipant.class);
}
/**
* The ID of the participant that is taking a turn.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String pendingParticipantId;
/**
* The data / game state for the previous match; set for the first turn of rematches only.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private TurnBasedMatchData previousMatchData;
/**
* The ID of a rematch of this match. Only set for completed matches that have been rematched.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rematchId;
/**
* The results reported for this match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List results;
static {
// hack to force ProGuard to consider ParticipantResult used, since otherwise it would be stripped out
// see http://code.google.com/p/google-api-java-client/issues/detail?id=528
com.google.api.client.util.Data.nullOf(ParticipantResult.class);
}
/**
* The status of the match. Possible values are: - "MATCH_AUTO_MATCHING" - One or more slots
* need to be filled by auto-matching; the match cannot be established until they are filled. -
* "MATCH_ACTIVE" - The match has started. - "MATCH_COMPLETE" - The match has finished. -
* "MATCH_CANCELED" - The match was canceled. - "MATCH_EXPIRED" - The match expired due to
* inactivity. - "MATCH_DELETED" - The match should no longer be shown on the client. Returned
* only for tombstones for matches when sync is called.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String status;
/**
* The status of the current user in the match. Derived from the match type, match status, the
* user's participant status, and the pending participant for the match. Possible values are: -
* "USER_INVITED" - The user has been invited to join the match and has not responded yet. -
* "USER_AWAITING_TURN" - The user is waiting for their turn. - "USER_TURN" - The user has an
* action to take in the match. - "USER_MATCH_COMPLETED" - The match has ended (it is completed,
* canceled, or expired.)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String userMatchStatus;
/**
* The variant / mode of the application being played; can be any integer value, or left blank.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer variant;
/**
* The ID of another participant in the match that can be used when describing the participants
* the user is playing with.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String withParticipantId;
/**
* The ID of the application being played.
* @return value or {@code null} for none
*/
public java.lang.String getApplicationId() {
return applicationId;
}
/**
* The ID of the application being played.
* @param applicationId applicationId or {@code null} for none
*/
public TurnBasedMatch setApplicationId(java.lang.String applicationId) {
this.applicationId = applicationId;
return this;
}
/**
* Criteria for auto-matching players into this match.
* @return value or {@code null} for none
*/
public TurnBasedAutoMatchingCriteria getAutoMatchingCriteria() {
return autoMatchingCriteria;
}
/**
* Criteria for auto-matching players into this match.
* @param autoMatchingCriteria autoMatchingCriteria or {@code null} for none
*/
public TurnBasedMatch setAutoMatchingCriteria(TurnBasedAutoMatchingCriteria autoMatchingCriteria) {
this.autoMatchingCriteria = autoMatchingCriteria;
return this;
}
/**
* Details about the match creation.
* @return value or {@code null} for none
*/
public TurnBasedMatchModification getCreationDetails() {
return creationDetails;
}
/**
* Details about the match creation.
* @param creationDetails creationDetails or {@code null} for none
*/
public TurnBasedMatch setCreationDetails(TurnBasedMatchModification creationDetails) {
this.creationDetails = creationDetails;
return this;
}
/**
* The data / game state for this match.
* @return value or {@code null} for none
*/
public TurnBasedMatchData getData() {
return data;
}
/**
* The data / game state for this match.
* @param data data or {@code null} for none
*/
public TurnBasedMatch setData(TurnBasedMatchData data) {
this.data = data;
return this;
}
/**
* This short description is generated by our servers based on turn state and is localized and
* worded relative to the player requesting the match. It is intended to be displayed when the
* match is shown in a list.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* This short description is generated by our servers based on turn state and is localized and
* worded relative to the player requesting the match. It is intended to be displayed when the
* match is shown in a list.
* @param description description or {@code null} for none
*/
public TurnBasedMatch setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The ID of the participant that invited the user to the match. Not set if the user was not
* invited to the match.
* @return value or {@code null} for none
*/
public java.lang.String getInviterId() {
return inviterId;
}
/**
* The ID of the participant that invited the user to the match. Not set if the user was not
* invited to the match.
* @param inviterId inviterId or {@code null} for none
*/
public TurnBasedMatch setInviterId(java.lang.String inviterId) {
this.inviterId = inviterId;
return this;
}
/**
* Uniquely identifies the type of this resource. Value is always the fixed string
* games#turnBasedMatch.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* Uniquely identifies the type of this resource. Value is always the fixed string
* games#turnBasedMatch.
* @param kind kind or {@code null} for none
*/
public TurnBasedMatch setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* Details about the last update to the match.
* @return value or {@code null} for none
*/
public TurnBasedMatchModification getLastUpdateDetails() {
return lastUpdateDetails;
}
/**
* Details about the last update to the match.
* @param lastUpdateDetails lastUpdateDetails or {@code null} for none
*/
public TurnBasedMatch setLastUpdateDetails(TurnBasedMatchModification lastUpdateDetails) {
this.lastUpdateDetails = lastUpdateDetails;
return this;
}
/**
* Globally unique ID for a turn-based match.
* @return value or {@code null} for none
*/
public java.lang.String getMatchId() {
return matchId;
}
/**
* Globally unique ID for a turn-based match.
* @param matchId matchId or {@code null} for none
*/
public TurnBasedMatch setMatchId(java.lang.String matchId) {
this.matchId = matchId;
return this;
}
/**
* The number of the match in a chain of rematches. Will be set to 1 for the first match and
* incremented by 1 for each rematch.
* @return value or {@code null} for none
*/
public java.lang.Integer getMatchNumber() {
return matchNumber;
}
/**
* The number of the match in a chain of rematches. Will be set to 1 for the first match and
* incremented by 1 for each rematch.
* @param matchNumber matchNumber or {@code null} for none
*/
public TurnBasedMatch setMatchNumber(java.lang.Integer matchNumber) {
this.matchNumber = matchNumber;
return this;
}
/**
* The version of this match: an increasing counter, used to avoid out-of-date updates to the
* match.
* @return value or {@code null} for none
*/
public java.lang.Integer getMatchVersion() {
return matchVersion;
}
/**
* The version of this match: an increasing counter, used to avoid out-of-date updates to the
* match.
* @param matchVersion matchVersion or {@code null} for none
*/
public TurnBasedMatch setMatchVersion(java.lang.Integer matchVersion) {
this.matchVersion = matchVersion;
return this;
}
/**
* The participants involved in the match, along with their statuses. Includes participants who
* have left or declined invitations.
* @return value or {@code null} for none
*/
public java.util.List getParticipants() {
return participants;
}
/**
* The participants involved in the match, along with their statuses. Includes participants who
* have left or declined invitations.
* @param participants participants or {@code null} for none
*/
public TurnBasedMatch setParticipants(java.util.List participants) {
this.participants = participants;
return this;
}
/**
* The ID of the participant that is taking a turn.
* @return value or {@code null} for none
*/
public java.lang.String getPendingParticipantId() {
return pendingParticipantId;
}
/**
* The ID of the participant that is taking a turn.
* @param pendingParticipantId pendingParticipantId or {@code null} for none
*/
public TurnBasedMatch setPendingParticipantId(java.lang.String pendingParticipantId) {
this.pendingParticipantId = pendingParticipantId;
return this;
}
/**
* The data / game state for the previous match; set for the first turn of rematches only.
* @return value or {@code null} for none
*/
public TurnBasedMatchData getPreviousMatchData() {
return previousMatchData;
}
/**
* The data / game state for the previous match; set for the first turn of rematches only.
* @param previousMatchData previousMatchData or {@code null} for none
*/
public TurnBasedMatch setPreviousMatchData(TurnBasedMatchData previousMatchData) {
this.previousMatchData = previousMatchData;
return this;
}
/**
* The ID of a rematch of this match. Only set for completed matches that have been rematched.
* @return value or {@code null} for none
*/
public java.lang.String getRematchId() {
return rematchId;
}
/**
* The ID of a rematch of this match. Only set for completed matches that have been rematched.
* @param rematchId rematchId or {@code null} for none
*/
public TurnBasedMatch setRematchId(java.lang.String rematchId) {
this.rematchId = rematchId;
return this;
}
/**
* The results reported for this match.
* @return value or {@code null} for none
*/
public java.util.List getResults() {
return results;
}
/**
* The results reported for this match.
* @param results results or {@code null} for none
*/
public TurnBasedMatch setResults(java.util.List results) {
this.results = results;
return this;
}
/**
* The status of the match. Possible values are: - "MATCH_AUTO_MATCHING" - One or more slots
* need to be filled by auto-matching; the match cannot be established until they are filled. -
* "MATCH_ACTIVE" - The match has started. - "MATCH_COMPLETE" - The match has finished. -
* "MATCH_CANCELED" - The match was canceled. - "MATCH_EXPIRED" - The match expired due to
* inactivity. - "MATCH_DELETED" - The match should no longer be shown on the client. Returned
* only for tombstones for matches when sync is called.
* @return value or {@code null} for none
*/
public java.lang.String getStatus() {
return status;
}
/**
* The status of the match. Possible values are: - "MATCH_AUTO_MATCHING" - One or more slots
* need to be filled by auto-matching; the match cannot be established until they are filled. -
* "MATCH_ACTIVE" - The match has started. - "MATCH_COMPLETE" - The match has finished. -
* "MATCH_CANCELED" - The match was canceled. - "MATCH_EXPIRED" - The match expired due to
* inactivity. - "MATCH_DELETED" - The match should no longer be shown on the client. Returned
* only for tombstones for matches when sync is called.
* @param status status or {@code null} for none
*/
public TurnBasedMatch setStatus(java.lang.String status) {
this.status = status;
return this;
}
/**
* The status of the current user in the match. Derived from the match type, match status, the
* user's participant status, and the pending participant for the match. Possible values are: -
* "USER_INVITED" - The user has been invited to join the match and has not responded yet. -
* "USER_AWAITING_TURN" - The user is waiting for their turn. - "USER_TURN" - The user has an
* action to take in the match. - "USER_MATCH_COMPLETED" - The match has ended (it is completed,
* canceled, or expired.)
* @return value or {@code null} for none
*/
public java.lang.String getUserMatchStatus() {
return userMatchStatus;
}
/**
* The status of the current user in the match. Derived from the match type, match status, the
* user's participant status, and the pending participant for the match. Possible values are: -
* "USER_INVITED" - The user has been invited to join the match and has not responded yet. -
* "USER_AWAITING_TURN" - The user is waiting for their turn. - "USER_TURN" - The user has an
* action to take in the match. - "USER_MATCH_COMPLETED" - The match has ended (it is completed,
* canceled, or expired.)
* @param userMatchStatus userMatchStatus or {@code null} for none
*/
public TurnBasedMatch setUserMatchStatus(java.lang.String userMatchStatus) {
this.userMatchStatus = userMatchStatus;
return this;
}
/**
* The variant / mode of the application being played; can be any integer value, or left blank.
* @return value or {@code null} for none
*/
public java.lang.Integer getVariant() {
return variant;
}
/**
* The variant / mode of the application being played; can be any integer value, or left blank.
* @param variant variant or {@code null} for none
*/
public TurnBasedMatch setVariant(java.lang.Integer variant) {
this.variant = variant;
return this;
}
/**
* The ID of another participant in the match that can be used when describing the participants
* the user is playing with.
* @return value or {@code null} for none
*/
public java.lang.String getWithParticipantId() {
return withParticipantId;
}
/**
* The ID of another participant in the match that can be used when describing the participants
* the user is playing with.
* @param withParticipantId withParticipantId or {@code null} for none
*/
public TurnBasedMatch setWithParticipantId(java.lang.String withParticipantId) {
this.withParticipantId = withParticipantId;
return this;
}
@Override
public TurnBasedMatch set(String fieldName, Object value) {
return (TurnBasedMatch) super.set(fieldName, value);
}
@Override
public TurnBasedMatch clone() {
return (TurnBasedMatch) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy