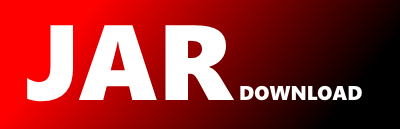
target.apidocs.com.google.api.services.gamesManagement.model.Player.html Maven / Gradle / Ivy
The newest version!
Player (Google Play Game Management v1management-rev20241209-2.0.0)
com.google.api.services.gamesManagement.model
Class Player
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.gamesManagement.model.Player
-
public final class Player
extends com.google.api.client.json.GenericJson
A Player resource.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Google Play Game Management. For a detailed
explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
static class
Player.Name
An object representation of the individual components of the player's name.
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Player()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Player
clone()
String
getAvatarImageUrl()
The base URL for the image that represents the player.
String
getBannerUrlLandscape()
The url to the landscape mode player banner image.
String
getBannerUrlPortrait()
The url to the portrait mode player banner image.
String
getDisplayName()
The name to display for the player.
GamesPlayerExperienceInfoResource
getExperienceInfo()
An object to represent Play Game experience information for the player.
String
getKind()
Uniquely identifies the type of this resource.
Player.Name
getName()
An object representation of the individual components of the player's name.
String
getOriginalPlayerId()
The player ID that was used for this player the first time they signed into the game in
question.
String
getPlayerId()
The ID of the player.
ProfileSettings
getProfileSettings()
The player's profile settings.
String
getTitle()
The player's title rewarded for their game activities.
Player
set(String fieldName,
Object value)
Player
setAvatarImageUrl(String avatarImageUrl)
The base URL for the image that represents the player.
Player
setBannerUrlLandscape(String bannerUrlLandscape)
The url to the landscape mode player banner image.
Player
setBannerUrlPortrait(String bannerUrlPortrait)
The url to the portrait mode player banner image.
Player
setDisplayName(String displayName)
The name to display for the player.
Player
setExperienceInfo(GamesPlayerExperienceInfoResource experienceInfo)
An object to represent Play Game experience information for the player.
Player
setKind(String kind)
Uniquely identifies the type of this resource.
Player
setName(Player.Name name)
An object representation of the individual components of the player's name.
Player
setOriginalPlayerId(String originalPlayerId)
The player ID that was used for this player the first time they signed into the game in
question.
Player
setPlayerId(String playerId)
The ID of the player.
Player
setProfileSettings(ProfileSettings profileSettings)
The player's profile settings.
Player
setTitle(String title)
The player's title rewarded for their game activities.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getAvatarImageUrl
public String getAvatarImageUrl()
The base URL for the image that represents the player.
- Returns:
- value or
null
for none
-
setAvatarImageUrl
public Player setAvatarImageUrl(String avatarImageUrl)
The base URL for the image that represents the player.
- Parameters:
avatarImageUrl
- avatarImageUrl or null
for none
-
getBannerUrlLandscape
public String getBannerUrlLandscape()
The url to the landscape mode player banner image.
- Returns:
- value or
null
for none
-
setBannerUrlLandscape
public Player setBannerUrlLandscape(String bannerUrlLandscape)
The url to the landscape mode player banner image.
- Parameters:
bannerUrlLandscape
- bannerUrlLandscape or null
for none
-
getBannerUrlPortrait
public String getBannerUrlPortrait()
The url to the portrait mode player banner image.
- Returns:
- value or
null
for none
-
setBannerUrlPortrait
public Player setBannerUrlPortrait(String bannerUrlPortrait)
The url to the portrait mode player banner image.
- Parameters:
bannerUrlPortrait
- bannerUrlPortrait or null
for none
-
getDisplayName
public String getDisplayName()
The name to display for the player.
- Returns:
- value or
null
for none
-
setDisplayName
public Player setDisplayName(String displayName)
The name to display for the player.
- Parameters:
displayName
- displayName or null
for none
-
getExperienceInfo
public GamesPlayerExperienceInfoResource getExperienceInfo()
An object to represent Play Game experience information for the player.
- Returns:
- value or
null
for none
-
setExperienceInfo
public Player setExperienceInfo(GamesPlayerExperienceInfoResource experienceInfo)
An object to represent Play Game experience information for the player.
- Parameters:
experienceInfo
- experienceInfo or null
for none
-
getKind
public String getKind()
Uniquely identifies the type of this resource. Value is always the fixed string
`gamesManagement#player`.
- Returns:
- value or
null
for none
-
setKind
public Player setKind(String kind)
Uniquely identifies the type of this resource. Value is always the fixed string
`gamesManagement#player`.
- Parameters:
kind
- kind or null
for none
-
getName
public Player.Name getName()
An object representation of the individual components of the player's name. For some players,
these fields may not be present.
- Returns:
- value or
null
for none
-
setName
public Player setName(Player.Name name)
An object representation of the individual components of the player's name. For some players,
these fields may not be present.
- Parameters:
name
- name or null
for none
-
getOriginalPlayerId
public String getOriginalPlayerId()
The player ID that was used for this player the first time they signed into the game in
question. This is only populated for calls to player.get for the requesting player, only if the
player ID has subsequently changed, and only to clients that support remapping player IDs.
- Returns:
- value or
null
for none
-
setOriginalPlayerId
public Player setOriginalPlayerId(String originalPlayerId)
The player ID that was used for this player the first time they signed into the game in
question. This is only populated for calls to player.get for the requesting player, only if the
player ID has subsequently changed, and only to clients that support remapping player IDs.
- Parameters:
originalPlayerId
- originalPlayerId or null
for none
-
getPlayerId
public String getPlayerId()
The ID of the player.
- Returns:
- value or
null
for none
-
setPlayerId
public Player setPlayerId(String playerId)
The ID of the player.
- Parameters:
playerId
- playerId or null
for none
-
getProfileSettings
public ProfileSettings getProfileSettings()
The player's profile settings. Controls whether or not the player's profile is visible to other
players.
- Returns:
- value or
null
for none
-
setProfileSettings
public Player setProfileSettings(ProfileSettings profileSettings)
The player's profile settings. Controls whether or not the player's profile is visible to other
players.
- Parameters:
profileSettings
- profileSettings or null
for none
-
getTitle
public String getTitle()
The player's title rewarded for their game activities.
- Returns:
- value or
null
for none
-
setTitle
public Player setTitle(String title)
The player's title rewarded for their game activities.
- Parameters:
title
- title or null
for none
-
set
public Player set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Player clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy