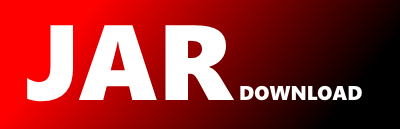
com.google.api.services.gan.model.CcOffer Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-04-08 17:16:44 UTC)
* on 2016-04-27 at 00:52:02 UTC
* Modify at your own risk.
*/
package com.google.api.services.gan.model;
/**
* A credit card offer. There are many possible result fields. We provide two different views of the
* data, or "projections." The "full" projection includes every result field. And the "summary"
* projection, which is the default, includes a smaller subset of the fields. The fields included in
* the summary projection are marked as such in their descriptions.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Affiliate Network API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class CcOffer extends com.google.api.client.json.GenericJson {
/**
* More marketing copy about the card's benefits. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List additionalCardBenefits;
/**
* Any extra fees levied on card holders.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String additionalCardHolderFee;
/**
* The youngest a recipient of this card may be.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double ageMinimum;
/**
* Text describing the details of the age minimum restriction.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String ageMinimumDetails;
/**
* The ongoing annual fee, in dollars.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double annualFee;
/**
* Text describing the annual fee, including any difference for the first year. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String annualFeeDisplay;
/**
* The largest number of units you may accumulate in a year.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double annualRewardMaximum;
/**
* Possible categories for this card, eg "Low Interest" or "Good." A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List approvedCategories;
/**
* Text describing the purchase APR. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String aprDisplay;
/**
* Text describing how the balance is computed. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String balanceComputationMethod;
/**
* Text describing the terms for balance transfers. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String balanceTransferTerms;
/**
* For cards with rewards programs, extra circumstances whereby additional rewards may be granted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List bonusRewards;
static {
// hack to force ProGuard to consider BonusRewards used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(BonusRewards.class);
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String carRentalInsurance;
/**
* A list of what the issuer thinks are the most important benefits of the card. Usually
* summarizes the rewards program, if there is one. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List cardBenefits;
/**
* The issuer's name for the card, including any trademark or service mark designators. A summary
* field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cardName;
/**
* What kind of credit card this is, for example secured or unsecured.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cardType;
/**
* Text describing the terms for cash advances. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String cashAdvanceTerms;
/**
* The high end for credit limits the issuer imposes on recipients of this card.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double creditLimitMax;
/**
* The low end for credit limits the issuer imposes on recipients of this card.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double creditLimitMin;
/**
* Text describing the credit ratings required for recipients of this card, for example
* "Excellent/Good." A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String creditRatingDisplay;
/**
* Fees for defaulting on your payments.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List defaultFees;
static {
// hack to force ProGuard to consider DefaultFees used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(DefaultFees.class);
}
/**
* A notice that, if present, is referenced via an asterisk by many of the other summary fields.
* If this field is present, it will always start with an asterisk ("*"), and must be prominently
* displayed with the offer. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String disclaimer;
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String emergencyInsurance;
/**
* Whether this card is only available to existing customers of the issuer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean existingCustomerOnly;
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String extendedWarranty;
/**
* The annual fee for the first year, if different from the ongoing fee. Optional.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double firstYearAnnualFee;
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String flightAccidentInsurance;
/**
* Fee for each transaction involving a foreign currency.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String foreignCurrencyTransactionFee;
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fraudLiability;
/**
* Text describing the grace period before finance charges apply. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String gracePeriodDisplay;
/**
* The link to the image of the card that is shown on Connect Commerce. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imageUrl;
/**
* Fee for setting up the card.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String initialSetupAndProcessingFee;
/**
* Text describing the terms for introductory period balance transfers. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String introBalanceTransferTerms;
/**
* Text describing the terms for introductory period cash advances. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String introCashAdvanceTerms;
/**
* Text describing the terms for introductory period purchases. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String introPurchaseTerms;
/**
* Name of card issuer. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String issuer;
/**
* The Google Affiliate Network ID of the advertiser making this offer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String issuerId;
/**
* The generic link to the issuer's site.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String issuerWebsite;
/**
* The kind for one credit card offer. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The link to the issuer's page for this card. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String landingPageUrl;
/**
* Text describing how much a late payment will cost, eg "up to $35." A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String latePaymentFee;
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String luggageInsurance;
/**
* The highest interest rate the issuer charges on this card. Expressed as an absolute number, not
* as a percentage.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double maxPurchaseRate;
/**
* The lowest interest rate the issuer charges on this card. Expressed as an absolute number, not
* as a percentage.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double minPurchaseRate;
/**
* Text describing how much missing the grace period will cost.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String minimumFinanceCharge;
/**
* Which network (eg Visa) the card belongs to. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String network;
/**
* This offer's ID. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String offerId;
/**
* Whether a cash reward program lets you get cash back sooner than end of year or other longish
* period.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean offersImmediateCashReward;
/**
* Fee for exceeding the card's charge limit.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String overLimitFee;
/**
* Categories in which the issuer does not wish the card to be displayed. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List prohibitedCategories;
/**
* Text describing any additional details for the purchase rate. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String purchaseRateAdditionalDetails;
/**
* Fixed or variable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String purchaseRateType;
/**
* Text describing the fee for a payment that doesn't clear. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String returnedPaymentFee;
/**
* The company that redeems the rewards, if different from the issuer.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rewardPartner;
/**
* For cards with rewards programs, the unit of reward. For example, miles, cash back, points.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rewardUnit;
/**
* For cards with rewards programs, detailed rules about how the program works.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List rewards;
static {
// hack to force ProGuard to consider Rewards used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Rewards.class);
}
/**
* Whether accumulated rewards ever expire.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean rewardsExpire;
/**
* For airline miles rewards, tells whether blackout dates apply to the miles.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean rewardsHaveBlackoutDates;
/**
* Fee for requesting a copy of your statement.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String statementCopyFee;
/**
* The link to ping to register a click on this offer. A summary field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String trackingUrl;
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String travelInsurance;
/**
* When variable rates were last updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String variableRatesLastUpdated;
/**
* How often variable rates are updated.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String variableRatesUpdateFrequency;
/**
* More marketing copy about the card's benefits. A summary field.
* @return value or {@code null} for none
*/
public java.util.List getAdditionalCardBenefits() {
return additionalCardBenefits;
}
/**
* More marketing copy about the card's benefits. A summary field.
* @param additionalCardBenefits additionalCardBenefits or {@code null} for none
*/
public CcOffer setAdditionalCardBenefits(java.util.List additionalCardBenefits) {
this.additionalCardBenefits = additionalCardBenefits;
return this;
}
/**
* Any extra fees levied on card holders.
* @return value or {@code null} for none
*/
public java.lang.String getAdditionalCardHolderFee() {
return additionalCardHolderFee;
}
/**
* Any extra fees levied on card holders.
* @param additionalCardHolderFee additionalCardHolderFee or {@code null} for none
*/
public CcOffer setAdditionalCardHolderFee(java.lang.String additionalCardHolderFee) {
this.additionalCardHolderFee = additionalCardHolderFee;
return this;
}
/**
* The youngest a recipient of this card may be.
* @return value or {@code null} for none
*/
public java.lang.Double getAgeMinimum() {
return ageMinimum;
}
/**
* The youngest a recipient of this card may be.
* @param ageMinimum ageMinimum or {@code null} for none
*/
public CcOffer setAgeMinimum(java.lang.Double ageMinimum) {
this.ageMinimum = ageMinimum;
return this;
}
/**
* Text describing the details of the age minimum restriction.
* @return value or {@code null} for none
*/
public java.lang.String getAgeMinimumDetails() {
return ageMinimumDetails;
}
/**
* Text describing the details of the age minimum restriction.
* @param ageMinimumDetails ageMinimumDetails or {@code null} for none
*/
public CcOffer setAgeMinimumDetails(java.lang.String ageMinimumDetails) {
this.ageMinimumDetails = ageMinimumDetails;
return this;
}
/**
* The ongoing annual fee, in dollars.
* @return value or {@code null} for none
*/
public java.lang.Double getAnnualFee() {
return annualFee;
}
/**
* The ongoing annual fee, in dollars.
* @param annualFee annualFee or {@code null} for none
*/
public CcOffer setAnnualFee(java.lang.Double annualFee) {
this.annualFee = annualFee;
return this;
}
/**
* Text describing the annual fee, including any difference for the first year. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getAnnualFeeDisplay() {
return annualFeeDisplay;
}
/**
* Text describing the annual fee, including any difference for the first year. A summary field.
* @param annualFeeDisplay annualFeeDisplay or {@code null} for none
*/
public CcOffer setAnnualFeeDisplay(java.lang.String annualFeeDisplay) {
this.annualFeeDisplay = annualFeeDisplay;
return this;
}
/**
* The largest number of units you may accumulate in a year.
* @return value or {@code null} for none
*/
public java.lang.Double getAnnualRewardMaximum() {
return annualRewardMaximum;
}
/**
* The largest number of units you may accumulate in a year.
* @param annualRewardMaximum annualRewardMaximum or {@code null} for none
*/
public CcOffer setAnnualRewardMaximum(java.lang.Double annualRewardMaximum) {
this.annualRewardMaximum = annualRewardMaximum;
return this;
}
/**
* Possible categories for this card, eg "Low Interest" or "Good." A summary field.
* @return value or {@code null} for none
*/
public java.util.List getApprovedCategories() {
return approvedCategories;
}
/**
* Possible categories for this card, eg "Low Interest" or "Good." A summary field.
* @param approvedCategories approvedCategories or {@code null} for none
*/
public CcOffer setApprovedCategories(java.util.List approvedCategories) {
this.approvedCategories = approvedCategories;
return this;
}
/**
* Text describing the purchase APR. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getAprDisplay() {
return aprDisplay;
}
/**
* Text describing the purchase APR. A summary field.
* @param aprDisplay aprDisplay or {@code null} for none
*/
public CcOffer setAprDisplay(java.lang.String aprDisplay) {
this.aprDisplay = aprDisplay;
return this;
}
/**
* Text describing how the balance is computed. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getBalanceComputationMethod() {
return balanceComputationMethod;
}
/**
* Text describing how the balance is computed. A summary field.
* @param balanceComputationMethod balanceComputationMethod or {@code null} for none
*/
public CcOffer setBalanceComputationMethod(java.lang.String balanceComputationMethod) {
this.balanceComputationMethod = balanceComputationMethod;
return this;
}
/**
* Text describing the terms for balance transfers. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getBalanceTransferTerms() {
return balanceTransferTerms;
}
/**
* Text describing the terms for balance transfers. A summary field.
* @param balanceTransferTerms balanceTransferTerms or {@code null} for none
*/
public CcOffer setBalanceTransferTerms(java.lang.String balanceTransferTerms) {
this.balanceTransferTerms = balanceTransferTerms;
return this;
}
/**
* For cards with rewards programs, extra circumstances whereby additional rewards may be granted.
* @return value or {@code null} for none
*/
public java.util.List getBonusRewards() {
return bonusRewards;
}
/**
* For cards with rewards programs, extra circumstances whereby additional rewards may be granted.
* @param bonusRewards bonusRewards or {@code null} for none
*/
public CcOffer setBonusRewards(java.util.List bonusRewards) {
this.bonusRewards = bonusRewards;
return this;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @return value or {@code null} for none
*/
public java.lang.String getCarRentalInsurance() {
return carRentalInsurance;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @param carRentalInsurance carRentalInsurance or {@code null} for none
*/
public CcOffer setCarRentalInsurance(java.lang.String carRentalInsurance) {
this.carRentalInsurance = carRentalInsurance;
return this;
}
/**
* A list of what the issuer thinks are the most important benefits of the card. Usually
* summarizes the rewards program, if there is one. A summary field.
* @return value or {@code null} for none
*/
public java.util.List getCardBenefits() {
return cardBenefits;
}
/**
* A list of what the issuer thinks are the most important benefits of the card. Usually
* summarizes the rewards program, if there is one. A summary field.
* @param cardBenefits cardBenefits or {@code null} for none
*/
public CcOffer setCardBenefits(java.util.List cardBenefits) {
this.cardBenefits = cardBenefits;
return this;
}
/**
* The issuer's name for the card, including any trademark or service mark designators. A summary
* field.
* @return value or {@code null} for none
*/
public java.lang.String getCardName() {
return cardName;
}
/**
* The issuer's name for the card, including any trademark or service mark designators. A summary
* field.
* @param cardName cardName or {@code null} for none
*/
public CcOffer setCardName(java.lang.String cardName) {
this.cardName = cardName;
return this;
}
/**
* What kind of credit card this is, for example secured or unsecured.
* @return value or {@code null} for none
*/
public java.lang.String getCardType() {
return cardType;
}
/**
* What kind of credit card this is, for example secured or unsecured.
* @param cardType cardType or {@code null} for none
*/
public CcOffer setCardType(java.lang.String cardType) {
this.cardType = cardType;
return this;
}
/**
* Text describing the terms for cash advances. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getCashAdvanceTerms() {
return cashAdvanceTerms;
}
/**
* Text describing the terms for cash advances. A summary field.
* @param cashAdvanceTerms cashAdvanceTerms or {@code null} for none
*/
public CcOffer setCashAdvanceTerms(java.lang.String cashAdvanceTerms) {
this.cashAdvanceTerms = cashAdvanceTerms;
return this;
}
/**
* The high end for credit limits the issuer imposes on recipients of this card.
* @return value or {@code null} for none
*/
public java.lang.Double getCreditLimitMax() {
return creditLimitMax;
}
/**
* The high end for credit limits the issuer imposes on recipients of this card.
* @param creditLimitMax creditLimitMax or {@code null} for none
*/
public CcOffer setCreditLimitMax(java.lang.Double creditLimitMax) {
this.creditLimitMax = creditLimitMax;
return this;
}
/**
* The low end for credit limits the issuer imposes on recipients of this card.
* @return value or {@code null} for none
*/
public java.lang.Double getCreditLimitMin() {
return creditLimitMin;
}
/**
* The low end for credit limits the issuer imposes on recipients of this card.
* @param creditLimitMin creditLimitMin or {@code null} for none
*/
public CcOffer setCreditLimitMin(java.lang.Double creditLimitMin) {
this.creditLimitMin = creditLimitMin;
return this;
}
/**
* Text describing the credit ratings required for recipients of this card, for example
* "Excellent/Good." A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getCreditRatingDisplay() {
return creditRatingDisplay;
}
/**
* Text describing the credit ratings required for recipients of this card, for example
* "Excellent/Good." A summary field.
* @param creditRatingDisplay creditRatingDisplay or {@code null} for none
*/
public CcOffer setCreditRatingDisplay(java.lang.String creditRatingDisplay) {
this.creditRatingDisplay = creditRatingDisplay;
return this;
}
/**
* Fees for defaulting on your payments.
* @return value or {@code null} for none
*/
public java.util.List getDefaultFees() {
return defaultFees;
}
/**
* Fees for defaulting on your payments.
* @param defaultFees defaultFees or {@code null} for none
*/
public CcOffer setDefaultFees(java.util.List defaultFees) {
this.defaultFees = defaultFees;
return this;
}
/**
* A notice that, if present, is referenced via an asterisk by many of the other summary fields.
* If this field is present, it will always start with an asterisk ("*"), and must be prominently
* displayed with the offer. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getDisclaimer() {
return disclaimer;
}
/**
* A notice that, if present, is referenced via an asterisk by many of the other summary fields.
* If this field is present, it will always start with an asterisk ("*"), and must be prominently
* displayed with the offer. A summary field.
* @param disclaimer disclaimer or {@code null} for none
*/
public CcOffer setDisclaimer(java.lang.String disclaimer) {
this.disclaimer = disclaimer;
return this;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @return value or {@code null} for none
*/
public java.lang.String getEmergencyInsurance() {
return emergencyInsurance;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @param emergencyInsurance emergencyInsurance or {@code null} for none
*/
public CcOffer setEmergencyInsurance(java.lang.String emergencyInsurance) {
this.emergencyInsurance = emergencyInsurance;
return this;
}
/**
* Whether this card is only available to existing customers of the issuer.
* @return value or {@code null} for none
*/
public java.lang.Boolean getExistingCustomerOnly() {
return existingCustomerOnly;
}
/**
* Whether this card is only available to existing customers of the issuer.
* @param existingCustomerOnly existingCustomerOnly or {@code null} for none
*/
public CcOffer setExistingCustomerOnly(java.lang.Boolean existingCustomerOnly) {
this.existingCustomerOnly = existingCustomerOnly;
return this;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @return value or {@code null} for none
*/
public java.lang.String getExtendedWarranty() {
return extendedWarranty;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @param extendedWarranty extendedWarranty or {@code null} for none
*/
public CcOffer setExtendedWarranty(java.lang.String extendedWarranty) {
this.extendedWarranty = extendedWarranty;
return this;
}
/**
* The annual fee for the first year, if different from the ongoing fee. Optional.
* @return value or {@code null} for none
*/
public java.lang.Double getFirstYearAnnualFee() {
return firstYearAnnualFee;
}
/**
* The annual fee for the first year, if different from the ongoing fee. Optional.
* @param firstYearAnnualFee firstYearAnnualFee or {@code null} for none
*/
public CcOffer setFirstYearAnnualFee(java.lang.Double firstYearAnnualFee) {
this.firstYearAnnualFee = firstYearAnnualFee;
return this;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @return value or {@code null} for none
*/
public java.lang.String getFlightAccidentInsurance() {
return flightAccidentInsurance;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @param flightAccidentInsurance flightAccidentInsurance or {@code null} for none
*/
public CcOffer setFlightAccidentInsurance(java.lang.String flightAccidentInsurance) {
this.flightAccidentInsurance = flightAccidentInsurance;
return this;
}
/**
* Fee for each transaction involving a foreign currency.
* @return value or {@code null} for none
*/
public java.lang.String getForeignCurrencyTransactionFee() {
return foreignCurrencyTransactionFee;
}
/**
* Fee for each transaction involving a foreign currency.
* @param foreignCurrencyTransactionFee foreignCurrencyTransactionFee or {@code null} for none
*/
public CcOffer setForeignCurrencyTransactionFee(java.lang.String foreignCurrencyTransactionFee) {
this.foreignCurrencyTransactionFee = foreignCurrencyTransactionFee;
return this;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @return value or {@code null} for none
*/
public java.lang.String getFraudLiability() {
return fraudLiability;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @param fraudLiability fraudLiability or {@code null} for none
*/
public CcOffer setFraudLiability(java.lang.String fraudLiability) {
this.fraudLiability = fraudLiability;
return this;
}
/**
* Text describing the grace period before finance charges apply. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getGracePeriodDisplay() {
return gracePeriodDisplay;
}
/**
* Text describing the grace period before finance charges apply. A summary field.
* @param gracePeriodDisplay gracePeriodDisplay or {@code null} for none
*/
public CcOffer setGracePeriodDisplay(java.lang.String gracePeriodDisplay) {
this.gracePeriodDisplay = gracePeriodDisplay;
return this;
}
/**
* The link to the image of the card that is shown on Connect Commerce. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getImageUrl() {
return imageUrl;
}
/**
* The link to the image of the card that is shown on Connect Commerce. A summary field.
* @param imageUrl imageUrl or {@code null} for none
*/
public CcOffer setImageUrl(java.lang.String imageUrl) {
this.imageUrl = imageUrl;
return this;
}
/**
* Fee for setting up the card.
* @return value or {@code null} for none
*/
public java.lang.String getInitialSetupAndProcessingFee() {
return initialSetupAndProcessingFee;
}
/**
* Fee for setting up the card.
* @param initialSetupAndProcessingFee initialSetupAndProcessingFee or {@code null} for none
*/
public CcOffer setInitialSetupAndProcessingFee(java.lang.String initialSetupAndProcessingFee) {
this.initialSetupAndProcessingFee = initialSetupAndProcessingFee;
return this;
}
/**
* Text describing the terms for introductory period balance transfers. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getIntroBalanceTransferTerms() {
return introBalanceTransferTerms;
}
/**
* Text describing the terms for introductory period balance transfers. A summary field.
* @param introBalanceTransferTerms introBalanceTransferTerms or {@code null} for none
*/
public CcOffer setIntroBalanceTransferTerms(java.lang.String introBalanceTransferTerms) {
this.introBalanceTransferTerms = introBalanceTransferTerms;
return this;
}
/**
* Text describing the terms for introductory period cash advances. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getIntroCashAdvanceTerms() {
return introCashAdvanceTerms;
}
/**
* Text describing the terms for introductory period cash advances. A summary field.
* @param introCashAdvanceTerms introCashAdvanceTerms or {@code null} for none
*/
public CcOffer setIntroCashAdvanceTerms(java.lang.String introCashAdvanceTerms) {
this.introCashAdvanceTerms = introCashAdvanceTerms;
return this;
}
/**
* Text describing the terms for introductory period purchases. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getIntroPurchaseTerms() {
return introPurchaseTerms;
}
/**
* Text describing the terms for introductory period purchases. A summary field.
* @param introPurchaseTerms introPurchaseTerms or {@code null} for none
*/
public CcOffer setIntroPurchaseTerms(java.lang.String introPurchaseTerms) {
this.introPurchaseTerms = introPurchaseTerms;
return this;
}
/**
* Name of card issuer. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getIssuer() {
return issuer;
}
/**
* Name of card issuer. A summary field.
* @param issuer issuer or {@code null} for none
*/
public CcOffer setIssuer(java.lang.String issuer) {
this.issuer = issuer;
return this;
}
/**
* The Google Affiliate Network ID of the advertiser making this offer.
* @return value or {@code null} for none
*/
public java.lang.String getIssuerId() {
return issuerId;
}
/**
* The Google Affiliate Network ID of the advertiser making this offer.
* @param issuerId issuerId or {@code null} for none
*/
public CcOffer setIssuerId(java.lang.String issuerId) {
this.issuerId = issuerId;
return this;
}
/**
* The generic link to the issuer's site.
* @return value or {@code null} for none
*/
public java.lang.String getIssuerWebsite() {
return issuerWebsite;
}
/**
* The generic link to the issuer's site.
* @param issuerWebsite issuerWebsite or {@code null} for none
*/
public CcOffer setIssuerWebsite(java.lang.String issuerWebsite) {
this.issuerWebsite = issuerWebsite;
return this;
}
/**
* The kind for one credit card offer. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* The kind for one credit card offer. A summary field.
* @param kind kind or {@code null} for none
*/
public CcOffer setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The link to the issuer's page for this card. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getLandingPageUrl() {
return landingPageUrl;
}
/**
* The link to the issuer's page for this card. A summary field.
* @param landingPageUrl landingPageUrl or {@code null} for none
*/
public CcOffer setLandingPageUrl(java.lang.String landingPageUrl) {
this.landingPageUrl = landingPageUrl;
return this;
}
/**
* Text describing how much a late payment will cost, eg "up to $35." A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getLatePaymentFee() {
return latePaymentFee;
}
/**
* Text describing how much a late payment will cost, eg "up to $35." A summary field.
* @param latePaymentFee latePaymentFee or {@code null} for none
*/
public CcOffer setLatePaymentFee(java.lang.String latePaymentFee) {
this.latePaymentFee = latePaymentFee;
return this;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @return value or {@code null} for none
*/
public java.lang.String getLuggageInsurance() {
return luggageInsurance;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @param luggageInsurance luggageInsurance or {@code null} for none
*/
public CcOffer setLuggageInsurance(java.lang.String luggageInsurance) {
this.luggageInsurance = luggageInsurance;
return this;
}
/**
* The highest interest rate the issuer charges on this card. Expressed as an absolute number, not
* as a percentage.
* @return value or {@code null} for none
*/
public java.lang.Double getMaxPurchaseRate() {
return maxPurchaseRate;
}
/**
* The highest interest rate the issuer charges on this card. Expressed as an absolute number, not
* as a percentage.
* @param maxPurchaseRate maxPurchaseRate or {@code null} for none
*/
public CcOffer setMaxPurchaseRate(java.lang.Double maxPurchaseRate) {
this.maxPurchaseRate = maxPurchaseRate;
return this;
}
/**
* The lowest interest rate the issuer charges on this card. Expressed as an absolute number, not
* as a percentage.
* @return value or {@code null} for none
*/
public java.lang.Double getMinPurchaseRate() {
return minPurchaseRate;
}
/**
* The lowest interest rate the issuer charges on this card. Expressed as an absolute number, not
* as a percentage.
* @param minPurchaseRate minPurchaseRate or {@code null} for none
*/
public CcOffer setMinPurchaseRate(java.lang.Double minPurchaseRate) {
this.minPurchaseRate = minPurchaseRate;
return this;
}
/**
* Text describing how much missing the grace period will cost.
* @return value or {@code null} for none
*/
public java.lang.String getMinimumFinanceCharge() {
return minimumFinanceCharge;
}
/**
* Text describing how much missing the grace period will cost.
* @param minimumFinanceCharge minimumFinanceCharge or {@code null} for none
*/
public CcOffer setMinimumFinanceCharge(java.lang.String minimumFinanceCharge) {
this.minimumFinanceCharge = minimumFinanceCharge;
return this;
}
/**
* Which network (eg Visa) the card belongs to. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getNetwork() {
return network;
}
/**
* Which network (eg Visa) the card belongs to. A summary field.
* @param network network or {@code null} for none
*/
public CcOffer setNetwork(java.lang.String network) {
this.network = network;
return this;
}
/**
* This offer's ID. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getOfferId() {
return offerId;
}
/**
* This offer's ID. A summary field.
* @param offerId offerId or {@code null} for none
*/
public CcOffer setOfferId(java.lang.String offerId) {
this.offerId = offerId;
return this;
}
/**
* Whether a cash reward program lets you get cash back sooner than end of year or other longish
* period.
* @return value or {@code null} for none
*/
public java.lang.Boolean getOffersImmediateCashReward() {
return offersImmediateCashReward;
}
/**
* Whether a cash reward program lets you get cash back sooner than end of year or other longish
* period.
* @param offersImmediateCashReward offersImmediateCashReward or {@code null} for none
*/
public CcOffer setOffersImmediateCashReward(java.lang.Boolean offersImmediateCashReward) {
this.offersImmediateCashReward = offersImmediateCashReward;
return this;
}
/**
* Fee for exceeding the card's charge limit.
* @return value or {@code null} for none
*/
public java.lang.String getOverLimitFee() {
return overLimitFee;
}
/**
* Fee for exceeding the card's charge limit.
* @param overLimitFee overLimitFee or {@code null} for none
*/
public CcOffer setOverLimitFee(java.lang.String overLimitFee) {
this.overLimitFee = overLimitFee;
return this;
}
/**
* Categories in which the issuer does not wish the card to be displayed. A summary field.
* @return value or {@code null} for none
*/
public java.util.List getProhibitedCategories() {
return prohibitedCategories;
}
/**
* Categories in which the issuer does not wish the card to be displayed. A summary field.
* @param prohibitedCategories prohibitedCategories or {@code null} for none
*/
public CcOffer setProhibitedCategories(java.util.List prohibitedCategories) {
this.prohibitedCategories = prohibitedCategories;
return this;
}
/**
* Text describing any additional details for the purchase rate. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getPurchaseRateAdditionalDetails() {
return purchaseRateAdditionalDetails;
}
/**
* Text describing any additional details for the purchase rate. A summary field.
* @param purchaseRateAdditionalDetails purchaseRateAdditionalDetails or {@code null} for none
*/
public CcOffer setPurchaseRateAdditionalDetails(java.lang.String purchaseRateAdditionalDetails) {
this.purchaseRateAdditionalDetails = purchaseRateAdditionalDetails;
return this;
}
/**
* Fixed or variable.
* @return value or {@code null} for none
*/
public java.lang.String getPurchaseRateType() {
return purchaseRateType;
}
/**
* Fixed or variable.
* @param purchaseRateType purchaseRateType or {@code null} for none
*/
public CcOffer setPurchaseRateType(java.lang.String purchaseRateType) {
this.purchaseRateType = purchaseRateType;
return this;
}
/**
* Text describing the fee for a payment that doesn't clear. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getReturnedPaymentFee() {
return returnedPaymentFee;
}
/**
* Text describing the fee for a payment that doesn't clear. A summary field.
* @param returnedPaymentFee returnedPaymentFee or {@code null} for none
*/
public CcOffer setReturnedPaymentFee(java.lang.String returnedPaymentFee) {
this.returnedPaymentFee = returnedPaymentFee;
return this;
}
/**
* The company that redeems the rewards, if different from the issuer.
* @return value or {@code null} for none
*/
public java.lang.String getRewardPartner() {
return rewardPartner;
}
/**
* The company that redeems the rewards, if different from the issuer.
* @param rewardPartner rewardPartner or {@code null} for none
*/
public CcOffer setRewardPartner(java.lang.String rewardPartner) {
this.rewardPartner = rewardPartner;
return this;
}
/**
* For cards with rewards programs, the unit of reward. For example, miles, cash back, points.
* @return value or {@code null} for none
*/
public java.lang.String getRewardUnit() {
return rewardUnit;
}
/**
* For cards with rewards programs, the unit of reward. For example, miles, cash back, points.
* @param rewardUnit rewardUnit or {@code null} for none
*/
public CcOffer setRewardUnit(java.lang.String rewardUnit) {
this.rewardUnit = rewardUnit;
return this;
}
/**
* For cards with rewards programs, detailed rules about how the program works.
* @return value or {@code null} for none
*/
public java.util.List getRewards() {
return rewards;
}
/**
* For cards with rewards programs, detailed rules about how the program works.
* @param rewards rewards or {@code null} for none
*/
public CcOffer setRewards(java.util.List rewards) {
this.rewards = rewards;
return this;
}
/**
* Whether accumulated rewards ever expire.
* @return value or {@code null} for none
*/
public java.lang.Boolean getRewardsExpire() {
return rewardsExpire;
}
/**
* Whether accumulated rewards ever expire.
* @param rewardsExpire rewardsExpire or {@code null} for none
*/
public CcOffer setRewardsExpire(java.lang.Boolean rewardsExpire) {
this.rewardsExpire = rewardsExpire;
return this;
}
/**
* For airline miles rewards, tells whether blackout dates apply to the miles.
* @return value or {@code null} for none
*/
public java.lang.Boolean getRewardsHaveBlackoutDates() {
return rewardsHaveBlackoutDates;
}
/**
* For airline miles rewards, tells whether blackout dates apply to the miles.
* @param rewardsHaveBlackoutDates rewardsHaveBlackoutDates or {@code null} for none
*/
public CcOffer setRewardsHaveBlackoutDates(java.lang.Boolean rewardsHaveBlackoutDates) {
this.rewardsHaveBlackoutDates = rewardsHaveBlackoutDates;
return this;
}
/**
* Fee for requesting a copy of your statement.
* @return value or {@code null} for none
*/
public java.lang.String getStatementCopyFee() {
return statementCopyFee;
}
/**
* Fee for requesting a copy of your statement.
* @param statementCopyFee statementCopyFee or {@code null} for none
*/
public CcOffer setStatementCopyFee(java.lang.String statementCopyFee) {
this.statementCopyFee = statementCopyFee;
return this;
}
/**
* The link to ping to register a click on this offer. A summary field.
* @return value or {@code null} for none
*/
public java.lang.String getTrackingUrl() {
return trackingUrl;
}
/**
* The link to ping to register a click on this offer. A summary field.
* @param trackingUrl trackingUrl or {@code null} for none
*/
public CcOffer setTrackingUrl(java.lang.String trackingUrl) {
this.trackingUrl = trackingUrl;
return this;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @return value or {@code null} for none
*/
public java.lang.String getTravelInsurance() {
return travelInsurance;
}
/**
* If you get coverage when you use the card for the given activity, this field describes it.
* @param travelInsurance travelInsurance or {@code null} for none
*/
public CcOffer setTravelInsurance(java.lang.String travelInsurance) {
this.travelInsurance = travelInsurance;
return this;
}
/**
* When variable rates were last updated.
* @return value or {@code null} for none
*/
public java.lang.String getVariableRatesLastUpdated() {
return variableRatesLastUpdated;
}
/**
* When variable rates were last updated.
* @param variableRatesLastUpdated variableRatesLastUpdated or {@code null} for none
*/
public CcOffer setVariableRatesLastUpdated(java.lang.String variableRatesLastUpdated) {
this.variableRatesLastUpdated = variableRatesLastUpdated;
return this;
}
/**
* How often variable rates are updated.
* @return value or {@code null} for none
*/
public java.lang.String getVariableRatesUpdateFrequency() {
return variableRatesUpdateFrequency;
}
/**
* How often variable rates are updated.
* @param variableRatesUpdateFrequency variableRatesUpdateFrequency or {@code null} for none
*/
public CcOffer setVariableRatesUpdateFrequency(java.lang.String variableRatesUpdateFrequency) {
this.variableRatesUpdateFrequency = variableRatesUpdateFrequency;
return this;
}
@Override
public CcOffer set(String fieldName, Object value) {
return (CcOffer) super.set(fieldName, value);
}
@Override
public CcOffer clone() {
return (CcOffer) super.clone();
}
/**
* Model definition for CcOfferBonusRewards.
*/
public static final class BonusRewards extends com.google.api.client.json.GenericJson {
/**
* How many units of reward will be granted.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double amount;
/**
* The circumstances under which this rule applies, for example, booking a flight via Orbitz.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String details;
/**
* How many units of reward will be granted.
* @return value or {@code null} for none
*/
public java.lang.Double getAmount() {
return amount;
}
/**
* How many units of reward will be granted.
* @param amount amount or {@code null} for none
*/
public BonusRewards setAmount(java.lang.Double amount) {
this.amount = amount;
return this;
}
/**
* The circumstances under which this rule applies, for example, booking a flight via Orbitz.
* @return value or {@code null} for none
*/
public java.lang.String getDetails() {
return details;
}
/**
* The circumstances under which this rule applies, for example, booking a flight via Orbitz.
* @param details details or {@code null} for none
*/
public BonusRewards setDetails(java.lang.String details) {
this.details = details;
return this;
}
@Override
public BonusRewards set(String fieldName, Object value) {
return (BonusRewards) super.set(fieldName, value);
}
@Override
public BonusRewards clone() {
return (BonusRewards) super.clone();
}
}
/**
* Model definition for CcOfferDefaultFees.
*/
public static final class DefaultFees extends com.google.api.client.json.GenericJson {
/**
* The type of charge, for example Purchases.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String category;
/**
* The highest rate the issuer may charge for defaulting on debt in this category. Expressed as an
* absolute number, not as a percentage.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double maxRate;
/**
* The lowest rate the issuer may charge for defaulting on debt in this category. Expressed as an
* absolute number, not as a percentage.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double minRate;
/**
* Fixed or variable.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String rateType;
/**
* The type of charge, for example Purchases.
* @return value or {@code null} for none
*/
public java.lang.String getCategory() {
return category;
}
/**
* The type of charge, for example Purchases.
* @param category category or {@code null} for none
*/
public DefaultFees setCategory(java.lang.String category) {
this.category = category;
return this;
}
/**
* The highest rate the issuer may charge for defaulting on debt in this category. Expressed as an
* absolute number, not as a percentage.
* @return value or {@code null} for none
*/
public java.lang.Double getMaxRate() {
return maxRate;
}
/**
* The highest rate the issuer may charge for defaulting on debt in this category. Expressed as an
* absolute number, not as a percentage.
* @param maxRate maxRate or {@code null} for none
*/
public DefaultFees setMaxRate(java.lang.Double maxRate) {
this.maxRate = maxRate;
return this;
}
/**
* The lowest rate the issuer may charge for defaulting on debt in this category. Expressed as an
* absolute number, not as a percentage.
* @return value or {@code null} for none
*/
public java.lang.Double getMinRate() {
return minRate;
}
/**
* The lowest rate the issuer may charge for defaulting on debt in this category. Expressed as an
* absolute number, not as a percentage.
* @param minRate minRate or {@code null} for none
*/
public DefaultFees setMinRate(java.lang.Double minRate) {
this.minRate = minRate;
return this;
}
/**
* Fixed or variable.
* @return value or {@code null} for none
*/
public java.lang.String getRateType() {
return rateType;
}
/**
* Fixed or variable.
* @param rateType rateType or {@code null} for none
*/
public DefaultFees setRateType(java.lang.String rateType) {
this.rateType = rateType;
return this;
}
@Override
public DefaultFees set(String fieldName, Object value) {
return (DefaultFees) super.set(fieldName, value);
}
@Override
public DefaultFees clone() {
return (DefaultFees) super.clone();
}
}
/**
* Model definition for CcOfferRewards.
*/
public static final class Rewards extends com.google.api.client.json.GenericJson {
/**
* Other limits, for example, if this rule only applies during an introductory period.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String additionalDetails;
/**
* The number of units rewarded per purchase dollar.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double amount;
/**
* The kind of purchases covered by this rule.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String category;
/**
* How long rewards granted by this rule last.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double expirationMonths;
/**
* The maximum purchase amount in the given category for this rule to apply.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double maxRewardTier;
/**
* The minimum purchase amount in the given category before this rule applies.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double minRewardTier;
/**
* Other limits, for example, if this rule only applies during an introductory period.
* @return value or {@code null} for none
*/
public java.lang.String getAdditionalDetails() {
return additionalDetails;
}
/**
* Other limits, for example, if this rule only applies during an introductory period.
* @param additionalDetails additionalDetails or {@code null} for none
*/
public Rewards setAdditionalDetails(java.lang.String additionalDetails) {
this.additionalDetails = additionalDetails;
return this;
}
/**
* The number of units rewarded per purchase dollar.
* @return value or {@code null} for none
*/
public java.lang.Double getAmount() {
return amount;
}
/**
* The number of units rewarded per purchase dollar.
* @param amount amount or {@code null} for none
*/
public Rewards setAmount(java.lang.Double amount) {
this.amount = amount;
return this;
}
/**
* The kind of purchases covered by this rule.
* @return value or {@code null} for none
*/
public java.lang.String getCategory() {
return category;
}
/**
* The kind of purchases covered by this rule.
* @param category category or {@code null} for none
*/
public Rewards setCategory(java.lang.String category) {
this.category = category;
return this;
}
/**
* How long rewards granted by this rule last.
* @return value or {@code null} for none
*/
public java.lang.Double getExpirationMonths() {
return expirationMonths;
}
/**
* How long rewards granted by this rule last.
* @param expirationMonths expirationMonths or {@code null} for none
*/
public Rewards setExpirationMonths(java.lang.Double expirationMonths) {
this.expirationMonths = expirationMonths;
return this;
}
/**
* The maximum purchase amount in the given category for this rule to apply.
* @return value or {@code null} for none
*/
public java.lang.Double getMaxRewardTier() {
return maxRewardTier;
}
/**
* The maximum purchase amount in the given category for this rule to apply.
* @param maxRewardTier maxRewardTier or {@code null} for none
*/
public Rewards setMaxRewardTier(java.lang.Double maxRewardTier) {
this.maxRewardTier = maxRewardTier;
return this;
}
/**
* The minimum purchase amount in the given category before this rule applies.
* @return value or {@code null} for none
*/
public java.lang.Double getMinRewardTier() {
return minRewardTier;
}
/**
* The minimum purchase amount in the given category before this rule applies.
* @param minRewardTier minRewardTier or {@code null} for none
*/
public Rewards setMinRewardTier(java.lang.Double minRewardTier) {
this.minRewardTier = minRewardTier;
return this;
}
@Override
public Rewards set(String fieldName, Object value) {
return (Rewards) super.set(fieldName, value);
}
@Override
public Rewards clone() {
return (Rewards) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy