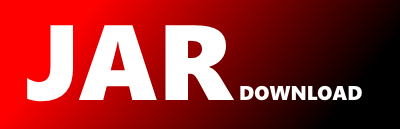
com.google.api.services.gan.model.Event Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-04-08 17:16:44 UTC)
* on 2016-04-27 at 00:52:02 UTC
* Modify at your own risk.
*/
package com.google.api.services.gan.model;
/**
* An EventResource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Affiliate Network API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Event extends com.google.api.client.json.GenericJson {
/**
* The ID of advertiser for this event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long advertiserId;
/**
* The name of the advertiser for this event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String advertiserName;
/**
* The charge ID for this event. Only returned for charge events.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String chargeId;
/**
* Charge type of the event (other|slotting_fee|monthly_minimum|tier_bonus|debit|credit). Only
* returned for charge events.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String chargeType;
/**
* Amount of money exchanged during the transaction. Only returned for charge and conversion
* events.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money commissionableSales;
/**
* Earnings by the publisher.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money earnings;
/**
* The date-time this event was initiated as a RFC 3339 date-time value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime eventDate;
/**
* The kind for one event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The ID of the member attached to this event. Only returned for conversion events.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String memberId;
/**
* The date-time this event was last modified as a RFC 3339 date-time value.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime modifyDate;
/**
* Fee that the advertiser paid to the Google Affiliate Network.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money networkFee;
/**
* The order ID for this event. Only returned for conversion events.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String orderId;
/**
* Products associated with the event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List products;
static {
// hack to force ProGuard to consider Products used, since otherwise it would be stripped out
// see https://github.com/google/google-api-java-client/issues/543
com.google.api.client.util.Data.nullOf(Products.class);
}
/**
* Fee that the advertiser paid to the publisher.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money publisherFee;
/**
* The ID of the publisher for this event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long publisherId;
/**
* The name of the publisher for this event.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String publisherName;
/**
* Status of the event (active|canceled). Only returned for charge and conversion events.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String status;
/**
* Type of the event (action|transaction|charge).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The ID of advertiser for this event.
* @return value or {@code null} for none
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/**
* The ID of advertiser for this event.
* @param advertiserId advertiserId or {@code null} for none
*/
public Event setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
/**
* The name of the advertiser for this event.
* @return value or {@code null} for none
*/
public java.lang.String getAdvertiserName() {
return advertiserName;
}
/**
* The name of the advertiser for this event.
* @param advertiserName advertiserName or {@code null} for none
*/
public Event setAdvertiserName(java.lang.String advertiserName) {
this.advertiserName = advertiserName;
return this;
}
/**
* The charge ID for this event. Only returned for charge events.
* @return value or {@code null} for none
*/
public java.lang.String getChargeId() {
return chargeId;
}
/**
* The charge ID for this event. Only returned for charge events.
* @param chargeId chargeId or {@code null} for none
*/
public Event setChargeId(java.lang.String chargeId) {
this.chargeId = chargeId;
return this;
}
/**
* Charge type of the event (other|slotting_fee|monthly_minimum|tier_bonus|debit|credit). Only
* returned for charge events.
* @return value or {@code null} for none
*/
public java.lang.String getChargeType() {
return chargeType;
}
/**
* Charge type of the event (other|slotting_fee|monthly_minimum|tier_bonus|debit|credit). Only
* returned for charge events.
* @param chargeType chargeType or {@code null} for none
*/
public Event setChargeType(java.lang.String chargeType) {
this.chargeType = chargeType;
return this;
}
/**
* Amount of money exchanged during the transaction. Only returned for charge and conversion
* events.
* @return value or {@code null} for none
*/
public Money getCommissionableSales() {
return commissionableSales;
}
/**
* Amount of money exchanged during the transaction. Only returned for charge and conversion
* events.
* @param commissionableSales commissionableSales or {@code null} for none
*/
public Event setCommissionableSales(Money commissionableSales) {
this.commissionableSales = commissionableSales;
return this;
}
/**
* Earnings by the publisher.
* @return value or {@code null} for none
*/
public Money getEarnings() {
return earnings;
}
/**
* Earnings by the publisher.
* @param earnings earnings or {@code null} for none
*/
public Event setEarnings(Money earnings) {
this.earnings = earnings;
return this;
}
/**
* The date-time this event was initiated as a RFC 3339 date-time value.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getEventDate() {
return eventDate;
}
/**
* The date-time this event was initiated as a RFC 3339 date-time value.
* @param eventDate eventDate or {@code null} for none
*/
public Event setEventDate(com.google.api.client.util.DateTime eventDate) {
this.eventDate = eventDate;
return this;
}
/**
* The kind for one event.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* The kind for one event.
* @param kind kind or {@code null} for none
*/
public Event setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The ID of the member attached to this event. Only returned for conversion events.
* @return value or {@code null} for none
*/
public java.lang.String getMemberId() {
return memberId;
}
/**
* The ID of the member attached to this event. Only returned for conversion events.
* @param memberId memberId or {@code null} for none
*/
public Event setMemberId(java.lang.String memberId) {
this.memberId = memberId;
return this;
}
/**
* The date-time this event was last modified as a RFC 3339 date-time value.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getModifyDate() {
return modifyDate;
}
/**
* The date-time this event was last modified as a RFC 3339 date-time value.
* @param modifyDate modifyDate or {@code null} for none
*/
public Event setModifyDate(com.google.api.client.util.DateTime modifyDate) {
this.modifyDate = modifyDate;
return this;
}
/**
* Fee that the advertiser paid to the Google Affiliate Network.
* @return value or {@code null} for none
*/
public Money getNetworkFee() {
return networkFee;
}
/**
* Fee that the advertiser paid to the Google Affiliate Network.
* @param networkFee networkFee or {@code null} for none
*/
public Event setNetworkFee(Money networkFee) {
this.networkFee = networkFee;
return this;
}
/**
* The order ID for this event. Only returned for conversion events.
* @return value or {@code null} for none
*/
public java.lang.String getOrderId() {
return orderId;
}
/**
* The order ID for this event. Only returned for conversion events.
* @param orderId orderId or {@code null} for none
*/
public Event setOrderId(java.lang.String orderId) {
this.orderId = orderId;
return this;
}
/**
* Products associated with the event.
* @return value or {@code null} for none
*/
public java.util.List getProducts() {
return products;
}
/**
* Products associated with the event.
* @param products products or {@code null} for none
*/
public Event setProducts(java.util.List products) {
this.products = products;
return this;
}
/**
* Fee that the advertiser paid to the publisher.
* @return value or {@code null} for none
*/
public Money getPublisherFee() {
return publisherFee;
}
/**
* Fee that the advertiser paid to the publisher.
* @param publisherFee publisherFee or {@code null} for none
*/
public Event setPublisherFee(Money publisherFee) {
this.publisherFee = publisherFee;
return this;
}
/**
* The ID of the publisher for this event.
* @return value or {@code null} for none
*/
public java.lang.Long getPublisherId() {
return publisherId;
}
/**
* The ID of the publisher for this event.
* @param publisherId publisherId or {@code null} for none
*/
public Event setPublisherId(java.lang.Long publisherId) {
this.publisherId = publisherId;
return this;
}
/**
* The name of the publisher for this event.
* @return value or {@code null} for none
*/
public java.lang.String getPublisherName() {
return publisherName;
}
/**
* The name of the publisher for this event.
* @param publisherName publisherName or {@code null} for none
*/
public Event setPublisherName(java.lang.String publisherName) {
this.publisherName = publisherName;
return this;
}
/**
* Status of the event (active|canceled). Only returned for charge and conversion events.
* @return value or {@code null} for none
*/
public java.lang.String getStatus() {
return status;
}
/**
* Status of the event (active|canceled). Only returned for charge and conversion events.
* @param status status or {@code null} for none
*/
public Event setStatus(java.lang.String status) {
this.status = status;
return this;
}
/**
* Type of the event (action|transaction|charge).
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* Type of the event (action|transaction|charge).
* @param type type or {@code null} for none
*/
public Event setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public Event set(String fieldName, Object value) {
return (Event) super.set(fieldName, value);
}
@Override
public Event clone() {
return (Event) super.clone();
}
/**
* Model definition for EventProducts.
*/
public static final class Products extends com.google.api.client.json.GenericJson {
/**
* Id of the category this product belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String categoryId;
/**
* Name of the category this product belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String categoryName;
/**
* Amount earned by the publisher on this product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money earnings;
/**
* Fee that the advertiser paid to the Google Affiliate Network for this product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money networkFee;
/**
* Fee that the advertiser paid to the publisehr for this product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money publisherFee;
/**
* Quantity of this product bought/exchanged.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long quantity;
/**
* Sku of this product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String sku;
/**
* Sku name of this product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String skuName;
/**
* Price per unit of this product.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money unitPrice;
/**
* Id of the category this product belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getCategoryId() {
return categoryId;
}
/**
* Id of the category this product belongs to.
* @param categoryId categoryId or {@code null} for none
*/
public Products setCategoryId(java.lang.String categoryId) {
this.categoryId = categoryId;
return this;
}
/**
* Name of the category this product belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getCategoryName() {
return categoryName;
}
/**
* Name of the category this product belongs to.
* @param categoryName categoryName or {@code null} for none
*/
public Products setCategoryName(java.lang.String categoryName) {
this.categoryName = categoryName;
return this;
}
/**
* Amount earned by the publisher on this product.
* @return value or {@code null} for none
*/
public Money getEarnings() {
return earnings;
}
/**
* Amount earned by the publisher on this product.
* @param earnings earnings or {@code null} for none
*/
public Products setEarnings(Money earnings) {
this.earnings = earnings;
return this;
}
/**
* Fee that the advertiser paid to the Google Affiliate Network for this product.
* @return value or {@code null} for none
*/
public Money getNetworkFee() {
return networkFee;
}
/**
* Fee that the advertiser paid to the Google Affiliate Network for this product.
* @param networkFee networkFee or {@code null} for none
*/
public Products setNetworkFee(Money networkFee) {
this.networkFee = networkFee;
return this;
}
/**
* Fee that the advertiser paid to the publisehr for this product.
* @return value or {@code null} for none
*/
public Money getPublisherFee() {
return publisherFee;
}
/**
* Fee that the advertiser paid to the publisehr for this product.
* @param publisherFee publisherFee or {@code null} for none
*/
public Products setPublisherFee(Money publisherFee) {
this.publisherFee = publisherFee;
return this;
}
/**
* Quantity of this product bought/exchanged.
* @return value or {@code null} for none
*/
public java.lang.Long getQuantity() {
return quantity;
}
/**
* Quantity of this product bought/exchanged.
* @param quantity quantity or {@code null} for none
*/
public Products setQuantity(java.lang.Long quantity) {
this.quantity = quantity;
return this;
}
/**
* Sku of this product.
* @return value or {@code null} for none
*/
public java.lang.String getSku() {
return sku;
}
/**
* Sku of this product.
* @param sku sku or {@code null} for none
*/
public Products setSku(java.lang.String sku) {
this.sku = sku;
return this;
}
/**
* Sku name of this product.
* @return value or {@code null} for none
*/
public java.lang.String getSkuName() {
return skuName;
}
/**
* Sku name of this product.
* @param skuName skuName or {@code null} for none
*/
public Products setSkuName(java.lang.String skuName) {
this.skuName = skuName;
return this;
}
/**
* Price per unit of this product.
* @return value or {@code null} for none
*/
public Money getUnitPrice() {
return unitPrice;
}
/**
* Price per unit of this product.
* @param unitPrice unitPrice or {@code null} for none
*/
public Products setUnitPrice(Money unitPrice) {
this.unitPrice = unitPrice;
return this;
}
@Override
public Products set(String fieldName, Object value) {
return (Products) super.set(fieldName, value);
}
@Override
public Products clone() {
return (Products) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy