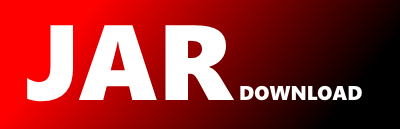
com.google.api.services.gan.model.Link Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-04-08 17:16:44 UTC)
* on 2016-04-27 at 00:52:02 UTC
* Modify at your own risk.
*/
package com.google.api.services.gan.model;
/**
* A LinkResource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Google Affiliate Network API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Link extends com.google.api.client.json.GenericJson {
/**
* The advertiser id for the advertiser who owns this link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long advertiserId;
/**
* Authorship
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String authorship;
/**
* Availability.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String availability;
/**
* Tracking url for clicks.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clickTrackingUrl;
/**
* Date that this link was created.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime createDate;
/**
* Description.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String description;
/**
* The destination URL for the link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String destinationUrl;
/**
* Duration
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String duration;
/**
* Date that this link becomes inactive.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime endDate;
/**
* The sum of fees paid to publishers divided by the total number of clicks over the past three
* months on this link. This value should be multiplied by 100 at the time of display.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money epcNinetyDayAverage;
/**
* The sum of fees paid to publishers divided by the total number of clicks over the past seven
* days on this link. This value should be multiplied by 100 at the time of display.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money epcSevenDayAverage;
/**
* The ID of this link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long id;
/**
* image alt text.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String imageAltText;
/**
* Tracking url for impressions.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String impressionTrackingUrl;
/**
* Flag for if this link is active.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean isActive;
/**
* The kind for one entity.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String kind;
/**
* The link type.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String linkType;
/**
* The logical name for this link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Promotion Type
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String promotionType;
/**
* Special offers on the link.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private SpecialOffers specialOffers;
/**
* Date that this link becomes active.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private com.google.api.client.util.DateTime startDate;
/**
* The advertiser id for the advertiser who owns this link.
* @return value or {@code null} for none
*/
public java.lang.Long getAdvertiserId() {
return advertiserId;
}
/**
* The advertiser id for the advertiser who owns this link.
* @param advertiserId advertiserId or {@code null} for none
*/
public Link setAdvertiserId(java.lang.Long advertiserId) {
this.advertiserId = advertiserId;
return this;
}
/**
* Authorship
* @return value or {@code null} for none
*/
public java.lang.String getAuthorship() {
return authorship;
}
/**
* Authorship
* @param authorship authorship or {@code null} for none
*/
public Link setAuthorship(java.lang.String authorship) {
this.authorship = authorship;
return this;
}
/**
* Availability.
* @return value or {@code null} for none
*/
public java.lang.String getAvailability() {
return availability;
}
/**
* Availability.
* @param availability availability or {@code null} for none
*/
public Link setAvailability(java.lang.String availability) {
this.availability = availability;
return this;
}
/**
* Tracking url for clicks.
* @return value or {@code null} for none
*/
public java.lang.String getClickTrackingUrl() {
return clickTrackingUrl;
}
/**
* Tracking url for clicks.
* @param clickTrackingUrl clickTrackingUrl or {@code null} for none
*/
public Link setClickTrackingUrl(java.lang.String clickTrackingUrl) {
this.clickTrackingUrl = clickTrackingUrl;
return this;
}
/**
* Date that this link was created.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getCreateDate() {
return createDate;
}
/**
* Date that this link was created.
* @param createDate createDate or {@code null} for none
*/
public Link setCreateDate(com.google.api.client.util.DateTime createDate) {
this.createDate = createDate;
return this;
}
/**
* Description.
* @return value or {@code null} for none
*/
public java.lang.String getDescription() {
return description;
}
/**
* Description.
* @param description description or {@code null} for none
*/
public Link setDescription(java.lang.String description) {
this.description = description;
return this;
}
/**
* The destination URL for the link.
* @return value or {@code null} for none
*/
public java.lang.String getDestinationUrl() {
return destinationUrl;
}
/**
* The destination URL for the link.
* @param destinationUrl destinationUrl or {@code null} for none
*/
public Link setDestinationUrl(java.lang.String destinationUrl) {
this.destinationUrl = destinationUrl;
return this;
}
/**
* Duration
* @return value or {@code null} for none
*/
public java.lang.String getDuration() {
return duration;
}
/**
* Duration
* @param duration duration or {@code null} for none
*/
public Link setDuration(java.lang.String duration) {
this.duration = duration;
return this;
}
/**
* Date that this link becomes inactive.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getEndDate() {
return endDate;
}
/**
* Date that this link becomes inactive.
* @param endDate endDate or {@code null} for none
*/
public Link setEndDate(com.google.api.client.util.DateTime endDate) {
this.endDate = endDate;
return this;
}
/**
* The sum of fees paid to publishers divided by the total number of clicks over the past three
* months on this link. This value should be multiplied by 100 at the time of display.
* @return value or {@code null} for none
*/
public Money getEpcNinetyDayAverage() {
return epcNinetyDayAverage;
}
/**
* The sum of fees paid to publishers divided by the total number of clicks over the past three
* months on this link. This value should be multiplied by 100 at the time of display.
* @param epcNinetyDayAverage epcNinetyDayAverage or {@code null} for none
*/
public Link setEpcNinetyDayAverage(Money epcNinetyDayAverage) {
this.epcNinetyDayAverage = epcNinetyDayAverage;
return this;
}
/**
* The sum of fees paid to publishers divided by the total number of clicks over the past seven
* days on this link. This value should be multiplied by 100 at the time of display.
* @return value or {@code null} for none
*/
public Money getEpcSevenDayAverage() {
return epcSevenDayAverage;
}
/**
* The sum of fees paid to publishers divided by the total number of clicks over the past seven
* days on this link. This value should be multiplied by 100 at the time of display.
* @param epcSevenDayAverage epcSevenDayAverage or {@code null} for none
*/
public Link setEpcSevenDayAverage(Money epcSevenDayAverage) {
this.epcSevenDayAverage = epcSevenDayAverage;
return this;
}
/**
* The ID of this link.
* @return value or {@code null} for none
*/
public java.lang.Long getId() {
return id;
}
/**
* The ID of this link.
* @param id id or {@code null} for none
*/
public Link setId(java.lang.Long id) {
this.id = id;
return this;
}
/**
* image alt text.
* @return value or {@code null} for none
*/
public java.lang.String getImageAltText() {
return imageAltText;
}
/**
* image alt text.
* @param imageAltText imageAltText or {@code null} for none
*/
public Link setImageAltText(java.lang.String imageAltText) {
this.imageAltText = imageAltText;
return this;
}
/**
* Tracking url for impressions.
* @return value or {@code null} for none
*/
public java.lang.String getImpressionTrackingUrl() {
return impressionTrackingUrl;
}
/**
* Tracking url for impressions.
* @param impressionTrackingUrl impressionTrackingUrl or {@code null} for none
*/
public Link setImpressionTrackingUrl(java.lang.String impressionTrackingUrl) {
this.impressionTrackingUrl = impressionTrackingUrl;
return this;
}
/**
* Flag for if this link is active.
* @return value or {@code null} for none
*/
public java.lang.Boolean getIsActive() {
return isActive;
}
/**
* Flag for if this link is active.
* @param isActive isActive or {@code null} for none
*/
public Link setIsActive(java.lang.Boolean isActive) {
this.isActive = isActive;
return this;
}
/**
* The kind for one entity.
* @return value or {@code null} for none
*/
public java.lang.String getKind() {
return kind;
}
/**
* The kind for one entity.
* @param kind kind or {@code null} for none
*/
public Link setKind(java.lang.String kind) {
this.kind = kind;
return this;
}
/**
* The link type.
* @return value or {@code null} for none
*/
public java.lang.String getLinkType() {
return linkType;
}
/**
* The link type.
* @param linkType linkType or {@code null} for none
*/
public Link setLinkType(java.lang.String linkType) {
this.linkType = linkType;
return this;
}
/**
* The logical name for this link.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The logical name for this link.
* @param name name or {@code null} for none
*/
public Link setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Promotion Type
* @return value or {@code null} for none
*/
public java.lang.String getPromotionType() {
return promotionType;
}
/**
* Promotion Type
* @param promotionType promotionType or {@code null} for none
*/
public Link setPromotionType(java.lang.String promotionType) {
this.promotionType = promotionType;
return this;
}
/**
* Special offers on the link.
* @return value or {@code null} for none
*/
public SpecialOffers getSpecialOffers() {
return specialOffers;
}
/**
* Special offers on the link.
* @param specialOffers specialOffers or {@code null} for none
*/
public Link setSpecialOffers(SpecialOffers specialOffers) {
this.specialOffers = specialOffers;
return this;
}
/**
* Date that this link becomes active.
* @return value or {@code null} for none
*/
public com.google.api.client.util.DateTime getStartDate() {
return startDate;
}
/**
* Date that this link becomes active.
* @param startDate startDate or {@code null} for none
*/
public Link setStartDate(com.google.api.client.util.DateTime startDate) {
this.startDate = startDate;
return this;
}
@Override
public Link set(String fieldName, Object value) {
return (Link) super.set(fieldName, value);
}
@Override
public Link clone() {
return (Link) super.clone();
}
/**
* Special offers on the link.
*/
public static final class SpecialOffers extends com.google.api.client.json.GenericJson {
/**
* Whether there is a free gift
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean freeGift;
/**
* Whether there is free shipping
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean freeShipping;
/**
* Minimum purchase amount for free shipping promotion
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money freeShippingMin;
/**
* Percent off on the purchase
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Double percentOff;
/**
* Minimum purchase amount for percent off promotion
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money percentOffMin;
/**
* Price cut on the purchase
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money priceCut;
/**
* Minimum purchase amount for price cut promotion
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Money priceCutMin;
/**
* List of promotion code associated with the link
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List promotionCodes;
/**
* Whether there is a free gift
* @return value or {@code null} for none
*/
public java.lang.Boolean getFreeGift() {
return freeGift;
}
/**
* Whether there is a free gift
* @param freeGift freeGift or {@code null} for none
*/
public SpecialOffers setFreeGift(java.lang.Boolean freeGift) {
this.freeGift = freeGift;
return this;
}
/**
* Whether there is free shipping
* @return value or {@code null} for none
*/
public java.lang.Boolean getFreeShipping() {
return freeShipping;
}
/**
* Whether there is free shipping
* @param freeShipping freeShipping or {@code null} for none
*/
public SpecialOffers setFreeShipping(java.lang.Boolean freeShipping) {
this.freeShipping = freeShipping;
return this;
}
/**
* Minimum purchase amount for free shipping promotion
* @return value or {@code null} for none
*/
public Money getFreeShippingMin() {
return freeShippingMin;
}
/**
* Minimum purchase amount for free shipping promotion
* @param freeShippingMin freeShippingMin or {@code null} for none
*/
public SpecialOffers setFreeShippingMin(Money freeShippingMin) {
this.freeShippingMin = freeShippingMin;
return this;
}
/**
* Percent off on the purchase
* @return value or {@code null} for none
*/
public java.lang.Double getPercentOff() {
return percentOff;
}
/**
* Percent off on the purchase
* @param percentOff percentOff or {@code null} for none
*/
public SpecialOffers setPercentOff(java.lang.Double percentOff) {
this.percentOff = percentOff;
return this;
}
/**
* Minimum purchase amount for percent off promotion
* @return value or {@code null} for none
*/
public Money getPercentOffMin() {
return percentOffMin;
}
/**
* Minimum purchase amount for percent off promotion
* @param percentOffMin percentOffMin or {@code null} for none
*/
public SpecialOffers setPercentOffMin(Money percentOffMin) {
this.percentOffMin = percentOffMin;
return this;
}
/**
* Price cut on the purchase
* @return value or {@code null} for none
*/
public Money getPriceCut() {
return priceCut;
}
/**
* Price cut on the purchase
* @param priceCut priceCut or {@code null} for none
*/
public SpecialOffers setPriceCut(Money priceCut) {
this.priceCut = priceCut;
return this;
}
/**
* Minimum purchase amount for price cut promotion
* @return value or {@code null} for none
*/
public Money getPriceCutMin() {
return priceCutMin;
}
/**
* Minimum purchase amount for price cut promotion
* @param priceCutMin priceCutMin or {@code null} for none
*/
public SpecialOffers setPriceCutMin(Money priceCutMin) {
this.priceCutMin = priceCutMin;
return this;
}
/**
* List of promotion code associated with the link
* @return value or {@code null} for none
*/
public java.util.List getPromotionCodes() {
return promotionCodes;
}
/**
* List of promotion code associated with the link
* @param promotionCodes promotionCodes or {@code null} for none
*/
public SpecialOffers setPromotionCodes(java.util.List promotionCodes) {
this.promotionCodes = promotionCodes;
return this;
}
@Override
public SpecialOffers set(String fieldName, Object value) {
return (SpecialOffers) super.set(fieldName, value);
}
@Override
public SpecialOffers clone() {
return (SpecialOffers) super.clone();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy