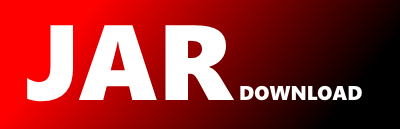
com.google.api.services.genomics.model.Read Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-10-05 at 01:24:23 UTC
* Modify at your own risk.
*/
package com.google.api.services.genomics.model;
/**
* A read alignment describes a linear alignment of a string of DNA to a reference sequence, in
* addition to metadata about the fragment (the molecule of DNA sequenced) and the read (the bases
* which were read by the sequencer). A read is equivalent to a line in a SAM file. A read belongs
* to exactly one read group and exactly one read group set. For more genomics resource definitions,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) ### Reverse-stranded reads Mapped reads (reads having a non-null `alignment`) can be
* aligned to either the forward or the reverse strand of their associated reference. Strandedness
* of a mapped read is encoded by `alignment.position.reverseStrand`. If we consider the reference
* to be a forward-stranded coordinate space of `[0, reference.length)` with `0` as the left-most
* position and `reference.length` as the right-most position, reads are always aligned left to
* right. That is, `alignment.position.position` always refers to the left-most reference coordinate
* and `alignment.cigar` describes the alignment of this read to the reference from left to right.
* All per-base fields such as `alignedSequence` and `alignedQuality` share this same left-to-right
* orientation; this is true of reads which are aligned to either strand. For reverse-stranded
* reads, this means that `alignedSequence` is the reverse complement of the bases that were
* originally reported by the sequencing machine. ### Generating a reference-aligned sequence string
* When interacting with mapped reads, it's often useful to produce a string representing the local
* alignment of the read to reference. The following pseudocode demonstrates one way of doing this:
* out = "" offset = 0 for c in read.alignment.cigar { switch c.operation { case "ALIGNMENT_MATCH",
* "SEQUENCE_MATCH", "SEQUENCE_MISMATCH": out +=
* read.alignedSequence[offset:offset+c.operationLength] offset += c.operationLength break case
* "CLIP_SOFT", "INSERT": offset += c.operationLength break case "PAD": out += repeat("*",
* c.operationLength) break case "DELETE": out += repeat("-", c.operationLength) break case "SKIP":
* out += repeat(" ", c.operationLength) break case "CLIP_HARD": break } } return out ### Converting
* to SAM's CIGAR string The following pseudocode generates a SAM CIGAR string from the `cigar`
* field. Note that this is a lossy conversion (`cigar.referenceSequence` is lost). cigarMap = {
* "ALIGNMENT_MATCH": "M", "INSERT": "I", "DELETE": "D", "SKIP": "N", "CLIP_SOFT": "S", "CLIP_HARD":
* "H", "PAD": "P", "SEQUENCE_MATCH": "=", "SEQUENCE_MISMATCH": "X", } cigarStr = "" for c in
* read.alignment.cigar { cigarStr += c.operationLength + cigarMap[c.operation] } return cigarStr
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Genomics API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Read extends com.google.api.client.json.GenericJson {
/**
* The quality of the read sequence contained in this alignment record (equivalent to QUAL in
* SAM). `alignedSequence` and `alignedQuality` may be shorter than the full read sequence and
* quality. This will occur if the alignment is part of a chimeric alignment, or if the read was
* trimmed. When this occurs, the CIGAR for this read will begin/end with a hard clip operator
* that will indicate the length of the excised sequence.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List alignedQuality;
/**
* The bases of the read sequence contained in this alignment record, **without CIGAR operations
* applied** (equivalent to SEQ in SAM). `alignedSequence` and `alignedQuality` may be shorter
* than the full read sequence and quality. This will occur if the alignment is part of a chimeric
* alignment, or if the read was trimmed. When this occurs, the CIGAR for this read will begin/end
* with a hard clip operator that will indicate the length of the excised sequence.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String alignedSequence;
/**
* The linear alignment for this alignment record. This field is null for unmapped reads.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private LinearAlignment alignment;
/**
* The fragment is a PCR or optical duplicate (SAM flag 0x400).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean duplicateFragment;
/**
* Whether this read did not pass filters, such as platform or vendor quality controls (SAM flag
* 0x200).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean failedVendorQualityChecks;
/**
* The observed length of the fragment, equivalent to TLEN in SAM.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer fragmentLength;
/**
* The fragment name. Equivalent to QNAME (query template name) in SAM.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String fragmentName;
/**
* The server-generated read ID, unique across all reads. This is different from the
* `fragmentName`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* A map of additional read alignment information. This must be of the form map (string key
* mapping to a list of string values).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map> info;
/**
* The mapping of the primary alignment of the `(readNumber+1)%numberReads` read in the fragment.
* It replaces mate position and mate strand in SAM.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Position nextMatePosition;
/**
* The number of reads in the fragment (extension to SAM flag 0x1).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer numberReads;
/**
* The orientation and the distance between reads from the fragment are consistent with the
* sequencing protocol (SAM flag 0x2).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean properPlacement;
/**
* The ID of the read group this read belongs to. A read belongs to exactly one read group. This
* is a server-generated ID which is distinct from SAM's RG tag (for that value, see
* ReadGroup.name).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String readGroupId;
/**
* The ID of the read group set this read belongs to. A read belongs to exactly one read group
* set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String readGroupSetId;
/**
* The read number in sequencing. 0-based and less than numberReads. This field replaces SAM flag
* 0x40 and 0x80.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer readNumber;
/**
* Whether this alignment is secondary. Equivalent to SAM flag 0x100. A secondary alignment
* represents an alternative to the primary alignment for this read. Aligners may return secondary
* alignments if a read can map ambiguously to multiple coordinates in the genome. By convention,
* each read has one and only one alignment where both `secondaryAlignment` and
* `supplementaryAlignment` are false.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean secondaryAlignment;
/**
* Whether this alignment is supplementary. Equivalent to SAM flag 0x800. Supplementary alignments
* are used in the representation of a chimeric alignment. In a chimeric alignment, a read is
* split into multiple linear alignments that map to different reference contigs. The first linear
* alignment in the read will be designated as the representative alignment; the remaining linear
* alignments will be designated as supplementary alignments. These alignments may have different
* mapping quality scores. In each linear alignment in a chimeric alignment, the read will be hard
* clipped. The `alignedSequence` and `alignedQuality` fields in the alignment record will only
* represent the bases for its respective linear alignment.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean supplementaryAlignment;
/**
* The quality of the read sequence contained in this alignment record (equivalent to QUAL in
* SAM). `alignedSequence` and `alignedQuality` may be shorter than the full read sequence and
* quality. This will occur if the alignment is part of a chimeric alignment, or if the read was
* trimmed. When this occurs, the CIGAR for this read will begin/end with a hard clip operator
* that will indicate the length of the excised sequence.
* @return value or {@code null} for none
*/
public java.util.List getAlignedQuality() {
return alignedQuality;
}
/**
* The quality of the read sequence contained in this alignment record (equivalent to QUAL in
* SAM). `alignedSequence` and `alignedQuality` may be shorter than the full read sequence and
* quality. This will occur if the alignment is part of a chimeric alignment, or if the read was
* trimmed. When this occurs, the CIGAR for this read will begin/end with a hard clip operator
* that will indicate the length of the excised sequence.
* @param alignedQuality alignedQuality or {@code null} for none
*/
public Read setAlignedQuality(java.util.List alignedQuality) {
this.alignedQuality = alignedQuality;
return this;
}
/**
* The bases of the read sequence contained in this alignment record, **without CIGAR operations
* applied** (equivalent to SEQ in SAM). `alignedSequence` and `alignedQuality` may be shorter
* than the full read sequence and quality. This will occur if the alignment is part of a chimeric
* alignment, or if the read was trimmed. When this occurs, the CIGAR for this read will begin/end
* with a hard clip operator that will indicate the length of the excised sequence.
* @return value or {@code null} for none
*/
public java.lang.String getAlignedSequence() {
return alignedSequence;
}
/**
* The bases of the read sequence contained in this alignment record, **without CIGAR operations
* applied** (equivalent to SEQ in SAM). `alignedSequence` and `alignedQuality` may be shorter
* than the full read sequence and quality. This will occur if the alignment is part of a chimeric
* alignment, or if the read was trimmed. When this occurs, the CIGAR for this read will begin/end
* with a hard clip operator that will indicate the length of the excised sequence.
* @param alignedSequence alignedSequence or {@code null} for none
*/
public Read setAlignedSequence(java.lang.String alignedSequence) {
this.alignedSequence = alignedSequence;
return this;
}
/**
* The linear alignment for this alignment record. This field is null for unmapped reads.
* @return value or {@code null} for none
*/
public LinearAlignment getAlignment() {
return alignment;
}
/**
* The linear alignment for this alignment record. This field is null for unmapped reads.
* @param alignment alignment or {@code null} for none
*/
public Read setAlignment(LinearAlignment alignment) {
this.alignment = alignment;
return this;
}
/**
* The fragment is a PCR or optical duplicate (SAM flag 0x400).
* @return value or {@code null} for none
*/
public java.lang.Boolean getDuplicateFragment() {
return duplicateFragment;
}
/**
* The fragment is a PCR or optical duplicate (SAM flag 0x400).
* @param duplicateFragment duplicateFragment or {@code null} for none
*/
public Read setDuplicateFragment(java.lang.Boolean duplicateFragment) {
this.duplicateFragment = duplicateFragment;
return this;
}
/**
* Whether this read did not pass filters, such as platform or vendor quality controls (SAM flag
* 0x200).
* @return value or {@code null} for none
*/
public java.lang.Boolean getFailedVendorQualityChecks() {
return failedVendorQualityChecks;
}
/**
* Whether this read did not pass filters, such as platform or vendor quality controls (SAM flag
* 0x200).
* @param failedVendorQualityChecks failedVendorQualityChecks or {@code null} for none
*/
public Read setFailedVendorQualityChecks(java.lang.Boolean failedVendorQualityChecks) {
this.failedVendorQualityChecks = failedVendorQualityChecks;
return this;
}
/**
* The observed length of the fragment, equivalent to TLEN in SAM.
* @return value or {@code null} for none
*/
public java.lang.Integer getFragmentLength() {
return fragmentLength;
}
/**
* The observed length of the fragment, equivalent to TLEN in SAM.
* @param fragmentLength fragmentLength or {@code null} for none
*/
public Read setFragmentLength(java.lang.Integer fragmentLength) {
this.fragmentLength = fragmentLength;
return this;
}
/**
* The fragment name. Equivalent to QNAME (query template name) in SAM.
* @return value or {@code null} for none
*/
public java.lang.String getFragmentName() {
return fragmentName;
}
/**
* The fragment name. Equivalent to QNAME (query template name) in SAM.
* @param fragmentName fragmentName or {@code null} for none
*/
public Read setFragmentName(java.lang.String fragmentName) {
this.fragmentName = fragmentName;
return this;
}
/**
* The server-generated read ID, unique across all reads. This is different from the
* `fragmentName`.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The server-generated read ID, unique across all reads. This is different from the
* `fragmentName`.
* @param id id or {@code null} for none
*/
public Read setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* A map of additional read alignment information. This must be of the form map (string key
* mapping to a list of string values).
* @return value or {@code null} for none
*/
public java.util.Map> getInfo() {
return info;
}
/**
* A map of additional read alignment information. This must be of the form map (string key
* mapping to a list of string values).
* @param info info or {@code null} for none
*/
public Read setInfo(java.util.Map> info) {
this.info = info;
return this;
}
/**
* The mapping of the primary alignment of the `(readNumber+1)%numberReads` read in the fragment.
* It replaces mate position and mate strand in SAM.
* @return value or {@code null} for none
*/
public Position getNextMatePosition() {
return nextMatePosition;
}
/**
* The mapping of the primary alignment of the `(readNumber+1)%numberReads` read in the fragment.
* It replaces mate position and mate strand in SAM.
* @param nextMatePosition nextMatePosition or {@code null} for none
*/
public Read setNextMatePosition(Position nextMatePosition) {
this.nextMatePosition = nextMatePosition;
return this;
}
/**
* The number of reads in the fragment (extension to SAM flag 0x1).
* @return value or {@code null} for none
*/
public java.lang.Integer getNumberReads() {
return numberReads;
}
/**
* The number of reads in the fragment (extension to SAM flag 0x1).
* @param numberReads numberReads or {@code null} for none
*/
public Read setNumberReads(java.lang.Integer numberReads) {
this.numberReads = numberReads;
return this;
}
/**
* The orientation and the distance between reads from the fragment are consistent with the
* sequencing protocol (SAM flag 0x2).
* @return value or {@code null} for none
*/
public java.lang.Boolean getProperPlacement() {
return properPlacement;
}
/**
* The orientation and the distance between reads from the fragment are consistent with the
* sequencing protocol (SAM flag 0x2).
* @param properPlacement properPlacement or {@code null} for none
*/
public Read setProperPlacement(java.lang.Boolean properPlacement) {
this.properPlacement = properPlacement;
return this;
}
/**
* The ID of the read group this read belongs to. A read belongs to exactly one read group. This
* is a server-generated ID which is distinct from SAM's RG tag (for that value, see
* ReadGroup.name).
* @return value or {@code null} for none
*/
public java.lang.String getReadGroupId() {
return readGroupId;
}
/**
* The ID of the read group this read belongs to. A read belongs to exactly one read group. This
* is a server-generated ID which is distinct from SAM's RG tag (for that value, see
* ReadGroup.name).
* @param readGroupId readGroupId or {@code null} for none
*/
public Read setReadGroupId(java.lang.String readGroupId) {
this.readGroupId = readGroupId;
return this;
}
/**
* The ID of the read group set this read belongs to. A read belongs to exactly one read group
* set.
* @return value or {@code null} for none
*/
public java.lang.String getReadGroupSetId() {
return readGroupSetId;
}
/**
* The ID of the read group set this read belongs to. A read belongs to exactly one read group
* set.
* @param readGroupSetId readGroupSetId or {@code null} for none
*/
public Read setReadGroupSetId(java.lang.String readGroupSetId) {
this.readGroupSetId = readGroupSetId;
return this;
}
/**
* The read number in sequencing. 0-based and less than numberReads. This field replaces SAM flag
* 0x40 and 0x80.
* @return value or {@code null} for none
*/
public java.lang.Integer getReadNumber() {
return readNumber;
}
/**
* The read number in sequencing. 0-based and less than numberReads. This field replaces SAM flag
* 0x40 and 0x80.
* @param readNumber readNumber or {@code null} for none
*/
public Read setReadNumber(java.lang.Integer readNumber) {
this.readNumber = readNumber;
return this;
}
/**
* Whether this alignment is secondary. Equivalent to SAM flag 0x100. A secondary alignment
* represents an alternative to the primary alignment for this read. Aligners may return secondary
* alignments if a read can map ambiguously to multiple coordinates in the genome. By convention,
* each read has one and only one alignment where both `secondaryAlignment` and
* `supplementaryAlignment` are false.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSecondaryAlignment() {
return secondaryAlignment;
}
/**
* Whether this alignment is secondary. Equivalent to SAM flag 0x100. A secondary alignment
* represents an alternative to the primary alignment for this read. Aligners may return secondary
* alignments if a read can map ambiguously to multiple coordinates in the genome. By convention,
* each read has one and only one alignment where both `secondaryAlignment` and
* `supplementaryAlignment` are false.
* @param secondaryAlignment secondaryAlignment or {@code null} for none
*/
public Read setSecondaryAlignment(java.lang.Boolean secondaryAlignment) {
this.secondaryAlignment = secondaryAlignment;
return this;
}
/**
* Whether this alignment is supplementary. Equivalent to SAM flag 0x800. Supplementary alignments
* are used in the representation of a chimeric alignment. In a chimeric alignment, a read is
* split into multiple linear alignments that map to different reference contigs. The first linear
* alignment in the read will be designated as the representative alignment; the remaining linear
* alignments will be designated as supplementary alignments. These alignments may have different
* mapping quality scores. In each linear alignment in a chimeric alignment, the read will be hard
* clipped. The `alignedSequence` and `alignedQuality` fields in the alignment record will only
* represent the bases for its respective linear alignment.
* @return value or {@code null} for none
*/
public java.lang.Boolean getSupplementaryAlignment() {
return supplementaryAlignment;
}
/**
* Whether this alignment is supplementary. Equivalent to SAM flag 0x800. Supplementary alignments
* are used in the representation of a chimeric alignment. In a chimeric alignment, a read is
* split into multiple linear alignments that map to different reference contigs. The first linear
* alignment in the read will be designated as the representative alignment; the remaining linear
* alignments will be designated as supplementary alignments. These alignments may have different
* mapping quality scores. In each linear alignment in a chimeric alignment, the read will be hard
* clipped. The `alignedSequence` and `alignedQuality` fields in the alignment record will only
* represent the bases for its respective linear alignment.
* @param supplementaryAlignment supplementaryAlignment or {@code null} for none
*/
public Read setSupplementaryAlignment(java.lang.Boolean supplementaryAlignment) {
this.supplementaryAlignment = supplementaryAlignment;
return this;
}
@Override
public Read set(String fieldName, Object value) {
return (Read) super.set(fieldName, value);
}
@Override
public Read clone() {
return (Read) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy