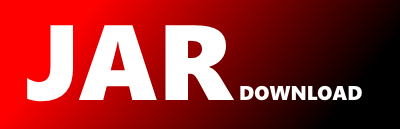
com.google.api.services.genomics.model.SearchReferenceSetsRequest Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-10-05 at 01:24:23 UTC
* Modify at your own risk.
*/
package com.google.api.services.genomics.model;
/**
* Model definition for SearchReferenceSetsRequest.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Genomics API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class SearchReferenceSetsRequest extends com.google.api.client.json.GenericJson {
/**
* If present, return reference sets for which a prefix of any of sourceAccessions match any of
* these strings. Accession numbers typically have a main number and a version, for example
* `NC_000001.11`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List accessions;
/**
* If present, return reference sets for which a substring of their `assemblyId` matches this
* string (case insensitive).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String assemblyId;
/**
* If present, return reference sets for which the md5checksum matches exactly.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List md5checksums;
/**
* The maximum number of results to return in a single page. If unspecified, defaults to 1024. The
* maximum value is 4096.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/**
* The continuation token, which is used to page through large result sets. To get the next page
* of results, set this parameter to the value of `nextPageToken` from the previous response.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/**
* If present, return reference sets for which a prefix of any of sourceAccessions match any of
* these strings. Accession numbers typically have a main number and a version, for example
* `NC_000001.11`.
* @return value or {@code null} for none
*/
public java.util.List getAccessions() {
return accessions;
}
/**
* If present, return reference sets for which a prefix of any of sourceAccessions match any of
* these strings. Accession numbers typically have a main number and a version, for example
* `NC_000001.11`.
* @param accessions accessions or {@code null} for none
*/
public SearchReferenceSetsRequest setAccessions(java.util.List accessions) {
this.accessions = accessions;
return this;
}
/**
* If present, return reference sets for which a substring of their `assemblyId` matches this
* string (case insensitive).
* @return value or {@code null} for none
*/
public java.lang.String getAssemblyId() {
return assemblyId;
}
/**
* If present, return reference sets for which a substring of their `assemblyId` matches this
* string (case insensitive).
* @param assemblyId assemblyId or {@code null} for none
*/
public SearchReferenceSetsRequest setAssemblyId(java.lang.String assemblyId) {
this.assemblyId = assemblyId;
return this;
}
/**
* If present, return reference sets for which the md5checksum matches exactly.
* @return value or {@code null} for none
*/
public java.util.List getMd5checksums() {
return md5checksums;
}
/**
* If present, return reference sets for which the md5checksum matches exactly.
* @param md5checksums md5checksums or {@code null} for none
*/
public SearchReferenceSetsRequest setMd5checksums(java.util.List md5checksums) {
this.md5checksums = md5checksums;
return this;
}
/**
* The maximum number of results to return in a single page. If unspecified, defaults to 1024. The
* maximum value is 4096.
* @return value or {@code null} for none
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return in a single page. If unspecified, defaults to 1024. The
* maximum value is 4096.
* @param pageSize pageSize or {@code null} for none
*/
public SearchReferenceSetsRequest setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next page
* of results, set this parameter to the value of `nextPageToken` from the previous response.
* @return value or {@code null} for none
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next page
* of results, set this parameter to the value of `nextPageToken` from the previous response.
* @param pageToken pageToken or {@code null} for none
*/
public SearchReferenceSetsRequest setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public SearchReferenceSetsRequest set(String fieldName, Object value) {
return (SearchReferenceSetsRequest) super.set(fieldName, value);
}
@Override
public SearchReferenceSetsRequest clone() {
return (SearchReferenceSetsRequest) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy