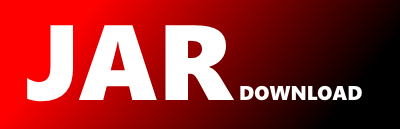
com.google.api.services.genomics.model.Transcript Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-10-05 at 01:24:23 UTC
* Modify at your own risk.
*/
package com.google.api.services.genomics.model;
/**
* A transcript represents the assertion that a particular region of the reference genome may be
* transcribed as RNA.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Genomics API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Transcript extends com.google.api.client.json.GenericJson {
/**
* The range of the coding sequence for this transcript, if any. To determine the exact ranges of
* coding sequence, intersect this range with those of the exons, if any. If there are any exons,
* the codingSequence must start and end within them. Note that in some cases, the reference
* genome will not exactly match the observed mRNA transcript e.g. due to variance in the source
* genome from reference. In these cases, exon.frame will not necessarily match the expected
* reference reading frame and coding exon reference bases cannot necessarily be concatenated to
* produce the original transcript mRNA.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private CodingSequence codingSequence;
/**
* The exons that compose this transcript. This field should be unset for genomes where transcript
* splicing does not occur, for example prokaryotes. Introns are regions of the transcript that
* are not included in the spliced RNA product. Though not explicitly modeled here, intron ranges
* can be deduced; all regions of this transcript that are not exons are introns. Exonic sequences
* do not necessarily code for a translational product (amino acids). Only the regions of exons
* bounded by the codingSequence correspond to coding DNA sequence. Exons are ordered by start
* position and may not overlap.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List exons;
/**
* The annotation ID of the gene from which this transcript is transcribed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String geneId;
/**
* The range of the coding sequence for this transcript, if any. To determine the exact ranges of
* coding sequence, intersect this range with those of the exons, if any. If there are any exons,
* the codingSequence must start and end within them. Note that in some cases, the reference
* genome will not exactly match the observed mRNA transcript e.g. due to variance in the source
* genome from reference. In these cases, exon.frame will not necessarily match the expected
* reference reading frame and coding exon reference bases cannot necessarily be concatenated to
* produce the original transcript mRNA.
* @return value or {@code null} for none
*/
public CodingSequence getCodingSequence() {
return codingSequence;
}
/**
* The range of the coding sequence for this transcript, if any. To determine the exact ranges of
* coding sequence, intersect this range with those of the exons, if any. If there are any exons,
* the codingSequence must start and end within them. Note that in some cases, the reference
* genome will not exactly match the observed mRNA transcript e.g. due to variance in the source
* genome from reference. In these cases, exon.frame will not necessarily match the expected
* reference reading frame and coding exon reference bases cannot necessarily be concatenated to
* produce the original transcript mRNA.
* @param codingSequence codingSequence or {@code null} for none
*/
public Transcript setCodingSequence(CodingSequence codingSequence) {
this.codingSequence = codingSequence;
return this;
}
/**
* The exons that compose this transcript. This field should be unset for genomes where transcript
* splicing does not occur, for example prokaryotes. Introns are regions of the transcript that
* are not included in the spliced RNA product. Though not explicitly modeled here, intron ranges
* can be deduced; all regions of this transcript that are not exons are introns. Exonic sequences
* do not necessarily code for a translational product (amino acids). Only the regions of exons
* bounded by the codingSequence correspond to coding DNA sequence. Exons are ordered by start
* position and may not overlap.
* @return value or {@code null} for none
*/
public java.util.List getExons() {
return exons;
}
/**
* The exons that compose this transcript. This field should be unset for genomes where transcript
* splicing does not occur, for example prokaryotes. Introns are regions of the transcript that
* are not included in the spliced RNA product. Though not explicitly modeled here, intron ranges
* can be deduced; all regions of this transcript that are not exons are introns. Exonic sequences
* do not necessarily code for a translational product (amino acids). Only the regions of exons
* bounded by the codingSequence correspond to coding DNA sequence. Exons are ordered by start
* position and may not overlap.
* @param exons exons or {@code null} for none
*/
public Transcript setExons(java.util.List exons) {
this.exons = exons;
return this;
}
/**
* The annotation ID of the gene from which this transcript is transcribed.
* @return value or {@code null} for none
*/
public java.lang.String getGeneId() {
return geneId;
}
/**
* The annotation ID of the gene from which this transcript is transcribed.
* @param geneId geneId or {@code null} for none
*/
public Transcript setGeneId(java.lang.String geneId) {
this.geneId = geneId;
return this;
}
@Override
public Transcript set(String fieldName, Object value) {
return (Transcript) super.set(fieldName, value);
}
@Override
public Transcript clone() {
return (Transcript) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy