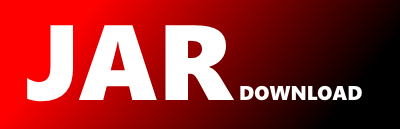
com.google.api.services.genomics.model.VariantCall Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-07-08 17:28:43 UTC)
* on 2016-10-05 at 01:24:23 UTC
* Modify at your own risk.
*/
package com.google.api.services.genomics.model;
/**
* A call represents the determination of genotype with respect to a particular variant. It may
* include associated information such as quality and phasing. For example, a call might assign a
* probability of 0.32 to the occurrence of a SNP named rs1234 in a call set with the name NA12345.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Genomics API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class VariantCall extends com.google.api.client.json.GenericJson {
/**
* The ID of the call set this variant call belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String callSetId;
/**
* The name of the call set this variant call belongs to.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String callSetName;
/**
* The genotype of this variant call. Each value represents either the value of the
* `referenceBases` field or a 1-based index into `alternateBases`. If a variant had a
* `referenceBases` value of `T` and an `alternateBases` value of `["A", "C"]`, and the `genotype`
* was `[2, 1]`, that would mean the call represented the heterozygous value `CA` for this
* variant. If the `genotype` was instead `[0, 1]`, the represented value would be `TA`. Ordering
* of the genotype values is important if the `phaseset` is present. If a genotype is not called
* (that is, a `.` is present in the GT string) -1 is returned.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List genotype;
/**
* The genotype likelihoods for this variant call. Each array entry represents how likely a
* specific genotype is for this call. The value ordering is defined by the GL tag in the VCF
* spec. If Phred-scaled genotype likelihood scores (PL) are available and log10(P) genotype
* likelihood scores (GL) are not, PL scores are converted to GL scores. If both are available, PL
* scores are stored in `info`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List genotypeLikelihood;
/**
* A map of additional variant call information. This must be of the form map (string key mapping
* to a list of string values).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map> info;
/**
* If this field is present, this variant call's genotype ordering implies the phase of the bases
* and is consistent with any other variant calls in the same reference sequence which have the
* same phaseset value. When importing data from VCF, if the genotype data was phased but no phase
* set was specified this field will be set to `*`.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String phaseset;
/**
* The ID of the call set this variant call belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getCallSetId() {
return callSetId;
}
/**
* The ID of the call set this variant call belongs to.
* @param callSetId callSetId or {@code null} for none
*/
public VariantCall setCallSetId(java.lang.String callSetId) {
this.callSetId = callSetId;
return this;
}
/**
* The name of the call set this variant call belongs to.
* @return value or {@code null} for none
*/
public java.lang.String getCallSetName() {
return callSetName;
}
/**
* The name of the call set this variant call belongs to.
* @param callSetName callSetName or {@code null} for none
*/
public VariantCall setCallSetName(java.lang.String callSetName) {
this.callSetName = callSetName;
return this;
}
/**
* The genotype of this variant call. Each value represents either the value of the
* `referenceBases` field or a 1-based index into `alternateBases`. If a variant had a
* `referenceBases` value of `T` and an `alternateBases` value of `["A", "C"]`, and the `genotype`
* was `[2, 1]`, that would mean the call represented the heterozygous value `CA` for this
* variant. If the `genotype` was instead `[0, 1]`, the represented value would be `TA`. Ordering
* of the genotype values is important if the `phaseset` is present. If a genotype is not called
* (that is, a `.` is present in the GT string) -1 is returned.
* @return value or {@code null} for none
*/
public java.util.List getGenotype() {
return genotype;
}
/**
* The genotype of this variant call. Each value represents either the value of the
* `referenceBases` field or a 1-based index into `alternateBases`. If a variant had a
* `referenceBases` value of `T` and an `alternateBases` value of `["A", "C"]`, and the `genotype`
* was `[2, 1]`, that would mean the call represented the heterozygous value `CA` for this
* variant. If the `genotype` was instead `[0, 1]`, the represented value would be `TA`. Ordering
* of the genotype values is important if the `phaseset` is present. If a genotype is not called
* (that is, a `.` is present in the GT string) -1 is returned.
* @param genotype genotype or {@code null} for none
*/
public VariantCall setGenotype(java.util.List genotype) {
this.genotype = genotype;
return this;
}
/**
* The genotype likelihoods for this variant call. Each array entry represents how likely a
* specific genotype is for this call. The value ordering is defined by the GL tag in the VCF
* spec. If Phred-scaled genotype likelihood scores (PL) are available and log10(P) genotype
* likelihood scores (GL) are not, PL scores are converted to GL scores. If both are available, PL
* scores are stored in `info`.
* @return value or {@code null} for none
*/
public java.util.List getGenotypeLikelihood() {
return genotypeLikelihood;
}
/**
* The genotype likelihoods for this variant call. Each array entry represents how likely a
* specific genotype is for this call. The value ordering is defined by the GL tag in the VCF
* spec. If Phred-scaled genotype likelihood scores (PL) are available and log10(P) genotype
* likelihood scores (GL) are not, PL scores are converted to GL scores. If both are available, PL
* scores are stored in `info`.
* @param genotypeLikelihood genotypeLikelihood or {@code null} for none
*/
public VariantCall setGenotypeLikelihood(java.util.List genotypeLikelihood) {
this.genotypeLikelihood = genotypeLikelihood;
return this;
}
/**
* A map of additional variant call information. This must be of the form map (string key mapping
* to a list of string values).
* @return value or {@code null} for none
*/
public java.util.Map> getInfo() {
return info;
}
/**
* A map of additional variant call information. This must be of the form map (string key mapping
* to a list of string values).
* @param info info or {@code null} for none
*/
public VariantCall setInfo(java.util.Map> info) {
this.info = info;
return this;
}
/**
* If this field is present, this variant call's genotype ordering implies the phase of the bases
* and is consistent with any other variant calls in the same reference sequence which have the
* same phaseset value. When importing data from VCF, if the genotype data was phased but no phase
* set was specified this field will be set to `*`.
* @return value or {@code null} for none
*/
public java.lang.String getPhaseset() {
return phaseset;
}
/**
* If this field is present, this variant call's genotype ordering implies the phase of the bases
* and is consistent with any other variant calls in the same reference sequence which have the
* same phaseset value. When importing data from VCF, if the genotype data was phased but no phase
* set was specified this field will be set to `*`.
* @param phaseset phaseset or {@code null} for none
*/
public VariantCall setPhaseset(java.lang.String phaseset) {
this.phaseset = phaseset;
return this;
}
@Override
public VariantCall set(String fieldName, Object value) {
return (VariantCall) super.set(fieldName, value);
}
@Override
public VariantCall clone() {
return (VariantCall) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy