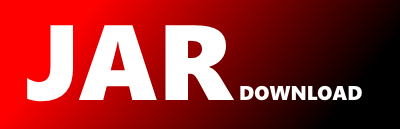
com.google.api.services.genomics.Genomics Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-27 16:00:31 UTC)
* on 2016-07-28 at 21:58:52 UTC
* Modify at your own risk.
*/
package com.google.api.services.genomics;
/**
* Service definition for Genomics (v1).
*
*
* Stores, processes, explores and shares genomic data.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link GenomicsRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Genomics extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.22.0 of the Genomics API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://genomics.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Genomics(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Genomics(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Annotations collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Annotations.List request = genomics.annotations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Annotations annotations() {
return new Annotations();
}
/**
* The "annotations" collection of methods.
*/
public class Annotations {
/**
* Creates one or more new annotations atomically. All annotations must belong to the same
* annotation set. Caller must have WRITE permission for this annotation set. For optimal
* performance, batch positionally adjacent annotations together. If the request has a systemic
* issue, such as an attempt to write to an inaccessible annotation set, the entire RPC will fail
* accordingly. For lesser data issues, when possible an error will be isolated to the corresponding
* batch entry in the response; the remaining well formed annotations will be created normally. For
* details on the requirements for each individual annotation resource, see CreateAnnotation.
*
* Create a request for the method "annotations.batchCreate".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link BatchCreate#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.BatchCreateAnnotationsRequest}
* @return the request
*/
public BatchCreate batchCreate(com.google.api.services.genomics.model.BatchCreateAnnotationsRequest content) throws java.io.IOException {
BatchCreate result = new BatchCreate(content);
initialize(result);
return result;
}
public class BatchCreate extends GenomicsRequest {
private static final String REST_PATH = "v1/annotations:batchCreate";
/**
* Creates one or more new annotations atomically. All annotations must belong to the same
* annotation set. Caller must have WRITE permission for this annotation set. For optimal
* performance, batch positionally adjacent annotations together. If the request has a systemic
* issue, such as an attempt to write to an inaccessible annotation set, the entire RPC will fail
* accordingly. For lesser data issues, when possible an error will be isolated to the
* corresponding batch entry in the response; the remaining well formed annotations will be
* created normally. For details on the requirements for each individual annotation resource, see
* CreateAnnotation.
*
* Create a request for the method "annotations.batchCreate".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link BatchCreate#execute()} method to invoke the remote
* operation. {@link
* BatchCreate#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.BatchCreateAnnotationsRequest}
* @since 1.13
*/
protected BatchCreate(com.google.api.services.genomics.model.BatchCreateAnnotationsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.BatchCreateAnnotationsResponse.class);
}
@Override
public BatchCreate set$Xgafv(java.lang.String $Xgafv) {
return (BatchCreate) super.set$Xgafv($Xgafv);
}
@Override
public BatchCreate setAccessToken(java.lang.String accessToken) {
return (BatchCreate) super.setAccessToken(accessToken);
}
@Override
public BatchCreate setAlt(java.lang.String alt) {
return (BatchCreate) super.setAlt(alt);
}
@Override
public BatchCreate setBearerToken(java.lang.String bearerToken) {
return (BatchCreate) super.setBearerToken(bearerToken);
}
@Override
public BatchCreate setCallback(java.lang.String callback) {
return (BatchCreate) super.setCallback(callback);
}
@Override
public BatchCreate setFields(java.lang.String fields) {
return (BatchCreate) super.setFields(fields);
}
@Override
public BatchCreate setKey(java.lang.String key) {
return (BatchCreate) super.setKey(key);
}
@Override
public BatchCreate setOauthToken(java.lang.String oauthToken) {
return (BatchCreate) super.setOauthToken(oauthToken);
}
@Override
public BatchCreate setPp(java.lang.Boolean pp) {
return (BatchCreate) super.setPp(pp);
}
@Override
public BatchCreate setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchCreate) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchCreate setQuotaUser(java.lang.String quotaUser) {
return (BatchCreate) super.setQuotaUser(quotaUser);
}
@Override
public BatchCreate setUploadType(java.lang.String uploadType) {
return (BatchCreate) super.setUploadType(uploadType);
}
@Override
public BatchCreate setUploadProtocol(java.lang.String uploadProtocol) {
return (BatchCreate) super.setUploadProtocol(uploadProtocol);
}
@Override
public BatchCreate set(String parameterName, Object value) {
return (BatchCreate) super.set(parameterName, value);
}
}
/**
* Creates a new annotation. Caller must have WRITE permission for the associated annotation set.
* The following fields are required: * annotationSetId * referenceName or referenceId ###
* Transcripts For annotations of type TRANSCRIPT, the following fields of transcript must be
* provided: * exons.start * exons.end All other fields may be optionally specified, unless
* documented as being server-generated (for example, the `id` field). The annotated range must be
* no longer than 100Mbp (mega base pairs). See the Annotation resource for additional restrictions
* on each field.
*
* Create a request for the method "annotations.create".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.Annotation}
* @return the request
*/
public Create create(com.google.api.services.genomics.model.Annotation content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends GenomicsRequest {
private static final String REST_PATH = "v1/annotations";
/**
* Creates a new annotation. Caller must have WRITE permission for the associated annotation set.
* The following fields are required: * annotationSetId * referenceName or referenceId ###
* Transcripts For annotations of type TRANSCRIPT, the following fields of transcript must be
* provided: * exons.start * exons.end All other fields may be optionally specified, unless
* documented as being server-generated (for example, the `id` field). The annotated range must be
* no longer than 100Mbp (mega base pairs). See the Annotation resource for additional
* restrictions on each field.
*
* Create a request for the method "annotations.create".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.Annotation}
* @since 1.13
*/
protected Create(com.google.api.services.genomics.model.Annotation content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Annotation.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an annotation. Caller must have WRITE permission for the associated annotation set.
*
* Create a request for the method "annotations.delete".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param annotationId The ID of the annotation to be deleted.
* @return the request
*/
public Delete delete(java.lang.String annotationId) throws java.io.IOException {
Delete result = new Delete(annotationId);
initialize(result);
return result;
}
public class Delete extends GenomicsRequest {
private static final String REST_PATH = "v1/annotations/{annotationId}";
/**
* Deletes an annotation. Caller must have WRITE permission for the associated annotation set.
*
* Create a request for the method "annotations.delete".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param annotationId The ID of the annotation to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String annotationId) {
super(Genomics.this, "DELETE", REST_PATH, null, com.google.api.services.genomics.model.Empty.class);
this.annotationId = com.google.api.client.util.Preconditions.checkNotNull(annotationId, "Required parameter annotationId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the annotation to be deleted. */
@com.google.api.client.util.Key
private java.lang.String annotationId;
/** The ID of the annotation to be deleted.
*/
public java.lang.String getAnnotationId() {
return annotationId;
}
/** The ID of the annotation to be deleted. */
public Delete setAnnotationId(java.lang.String annotationId) {
this.annotationId = annotationId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an annotation. Caller must have READ permission for the associated annotation set.
*
* Create a request for the method "annotations.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param annotationId The ID of the annotation to be retrieved.
* @return the request
*/
public Get get(java.lang.String annotationId) throws java.io.IOException {
Get result = new Get(annotationId);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/annotations/{annotationId}";
/**
* Gets an annotation. Caller must have READ permission for the associated annotation set.
*
* Create a request for the method "annotations.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param annotationId The ID of the annotation to be retrieved.
* @since 1.13
*/
protected Get(java.lang.String annotationId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.Annotation.class);
this.annotationId = com.google.api.client.util.Preconditions.checkNotNull(annotationId, "Required parameter annotationId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the annotation to be retrieved. */
@com.google.api.client.util.Key
private java.lang.String annotationId;
/** The ID of the annotation to be retrieved.
*/
public java.lang.String getAnnotationId() {
return annotationId;
}
/** The ID of the annotation to be retrieved. */
public Get setAnnotationId(java.lang.String annotationId) {
this.annotationId = annotationId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Searches for annotations that match the given criteria. Results are ordered by genomic coordinate
* (by reference sequence, then position). Annotations with equivalent genomic coordinates are
* returned in an unspecified order. This order is consistent, such that two queries for the same
* content (regardless of page size) yield annotations in the same order across their respective
* streams of paginated responses. Caller must have READ permission for the queried annotation sets.
*
* Create a request for the method "annotations.search".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.SearchAnnotationsRequest}
* @return the request
*/
public Search search(com.google.api.services.genomics.model.SearchAnnotationsRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends GenomicsRequest {
private static final String REST_PATH = "v1/annotations/search";
/**
* Searches for annotations that match the given criteria. Results are ordered by genomic
* coordinate (by reference sequence, then position). Annotations with equivalent genomic
* coordinates are returned in an unspecified order. This order is consistent, such that two
* queries for the same content (regardless of page size) yield annotations in the same order
* across their respective streams of paginated responses. Caller must have READ permission for
* the queried annotation sets.
*
* Create a request for the method "annotations.search".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.SearchAnnotationsRequest}
* @since 1.13
*/
protected Search(com.google.api.services.genomics.model.SearchAnnotationsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.SearchAnnotationsResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setBearerToken(java.lang.String bearerToken) {
return (Search) super.setBearerToken(bearerToken);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPp(java.lang.Boolean pp) {
return (Search) super.setPp(pp);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* Updates an annotation. Caller must have WRITE permission for the associated dataset.
*
* Create a request for the method "annotations.update".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param annotationId The ID of the annotation to be updated.
* @param content the {@link com.google.api.services.genomics.model.Annotation}
* @return the request
*/
public Update update(java.lang.String annotationId, com.google.api.services.genomics.model.Annotation content) throws java.io.IOException {
Update result = new Update(annotationId, content);
initialize(result);
return result;
}
public class Update extends GenomicsRequest {
private static final String REST_PATH = "v1/annotations/{annotationId}";
/**
* Updates an annotation. Caller must have WRITE permission for the associated dataset.
*
* Create a request for the method "annotations.update".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param annotationId The ID of the annotation to be updated.
* @param content the {@link com.google.api.services.genomics.model.Annotation}
* @since 1.13
*/
protected Update(java.lang.String annotationId, com.google.api.services.genomics.model.Annotation content) {
super(Genomics.this, "PUT", REST_PATH, content, com.google.api.services.genomics.model.Annotation.class);
this.annotationId = com.google.api.client.util.Preconditions.checkNotNull(annotationId, "Required parameter annotationId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setBearerToken(java.lang.String bearerToken) {
return (Update) super.setBearerToken(bearerToken);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPp(java.lang.Boolean pp) {
return (Update) super.setPp(pp);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the annotation to be updated. */
@com.google.api.client.util.Key
private java.lang.String annotationId;
/** The ID of the annotation to be updated.
*/
public java.lang.String getAnnotationId() {
return annotationId;
}
/** The ID of the annotation to be updated. */
public Update setAnnotationId(java.lang.String annotationId) {
this.annotationId = annotationId;
return this;
}
/**
* An optional mask specifying which fields to update. Mutable fields are name, variant,
* transcript, and info. If unspecified, all mutable fields will be updated.
*/
@com.google.api.client.util.Key
private java.lang.String updateMask;
/** An optional mask specifying which fields to update. Mutable fields are name, variant, transcript,
and info. If unspecified, all mutable fields will be updated.
*/
public java.lang.String getUpdateMask() {
return updateMask;
}
/**
* An optional mask specifying which fields to update. Mutable fields are name, variant,
* transcript, and info. If unspecified, all mutable fields will be updated.
*/
public Update setUpdateMask(java.lang.String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Annotationsets collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Annotationsets.List request = genomics.annotationsets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Annotationsets annotationsets() {
return new Annotationsets();
}
/**
* The "annotationsets" collection of methods.
*/
public class Annotationsets {
/**
* Creates a new annotation set. Caller must have WRITE permission for the associated dataset. The
* following fields are required: * datasetId * referenceSetId All other fields may be optionally
* specified, unless documented as being server-generated (for example, the `id` field).
*
* Create a request for the method "annotationsets.create".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.AnnotationSet}
* @return the request
*/
public Create create(com.google.api.services.genomics.model.AnnotationSet content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends GenomicsRequest {
private static final String REST_PATH = "v1/annotationsets";
/**
* Creates a new annotation set. Caller must have WRITE permission for the associated dataset. The
* following fields are required: * datasetId * referenceSetId All other fields may be optionally
* specified, unless documented as being server-generated (for example, the `id` field).
*
* Create a request for the method "annotationsets.create".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.AnnotationSet}
* @since 1.13
*/
protected Create(com.google.api.services.genomics.model.AnnotationSet content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.AnnotationSet.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an annotation set. Caller must have WRITE permission for the associated annotation set.
*
* Create a request for the method "annotationsets.delete".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param annotationSetId The ID of the annotation set to be deleted.
* @return the request
*/
public Delete delete(java.lang.String annotationSetId) throws java.io.IOException {
Delete result = new Delete(annotationSetId);
initialize(result);
return result;
}
public class Delete extends GenomicsRequest {
private static final String REST_PATH = "v1/annotationsets/{annotationSetId}";
/**
* Deletes an annotation set. Caller must have WRITE permission for the associated annotation set.
*
* Create a request for the method "annotationsets.delete".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param annotationSetId The ID of the annotation set to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String annotationSetId) {
super(Genomics.this, "DELETE", REST_PATH, null, com.google.api.services.genomics.model.Empty.class);
this.annotationSetId = com.google.api.client.util.Preconditions.checkNotNull(annotationSetId, "Required parameter annotationSetId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the annotation set to be deleted. */
@com.google.api.client.util.Key
private java.lang.String annotationSetId;
/** The ID of the annotation set to be deleted.
*/
public java.lang.String getAnnotationSetId() {
return annotationSetId;
}
/** The ID of the annotation set to be deleted. */
public Delete setAnnotationSetId(java.lang.String annotationSetId) {
this.annotationSetId = annotationSetId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets an annotation set. Caller must have READ permission for the associated dataset.
*
* Create a request for the method "annotationsets.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param annotationSetId The ID of the annotation set to be retrieved.
* @return the request
*/
public Get get(java.lang.String annotationSetId) throws java.io.IOException {
Get result = new Get(annotationSetId);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/annotationsets/{annotationSetId}";
/**
* Gets an annotation set. Caller must have READ permission for the associated dataset.
*
* Create a request for the method "annotationsets.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param annotationSetId The ID of the annotation set to be retrieved.
* @since 1.13
*/
protected Get(java.lang.String annotationSetId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.AnnotationSet.class);
this.annotationSetId = com.google.api.client.util.Preconditions.checkNotNull(annotationSetId, "Required parameter annotationSetId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the annotation set to be retrieved. */
@com.google.api.client.util.Key
private java.lang.String annotationSetId;
/** The ID of the annotation set to be retrieved.
*/
public java.lang.String getAnnotationSetId() {
return annotationSetId;
}
/** The ID of the annotation set to be retrieved. */
public Get setAnnotationSetId(java.lang.String annotationSetId) {
this.annotationSetId = annotationSetId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Searches for annotation sets that match the given criteria. Annotation sets are returned in an
* unspecified order. This order is consistent, such that two queries for the same content
* (regardless of page size) yield annotation sets in the same order across their respective streams
* of paginated responses. Caller must have READ permission for the queried datasets.
*
* Create a request for the method "annotationsets.search".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.SearchAnnotationSetsRequest}
* @return the request
*/
public Search search(com.google.api.services.genomics.model.SearchAnnotationSetsRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends GenomicsRequest {
private static final String REST_PATH = "v1/annotationsets/search";
/**
* Searches for annotation sets that match the given criteria. Annotation sets are returned in an
* unspecified order. This order is consistent, such that two queries for the same content
* (regardless of page size) yield annotation sets in the same order across their respective
* streams of paginated responses. Caller must have READ permission for the queried datasets.
*
* Create a request for the method "annotationsets.search".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.SearchAnnotationSetsRequest}
* @since 1.13
*/
protected Search(com.google.api.services.genomics.model.SearchAnnotationSetsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.SearchAnnotationSetsResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setBearerToken(java.lang.String bearerToken) {
return (Search) super.setBearerToken(bearerToken);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPp(java.lang.Boolean pp) {
return (Search) super.setPp(pp);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* Updates an annotation set. The update must respect all mutability restrictions and other
* invariants described on the annotation set resource. Caller must have WRITE permission for the
* associated dataset.
*
* Create a request for the method "annotationsets.update".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param annotationSetId The ID of the annotation set to be updated.
* @param content the {@link com.google.api.services.genomics.model.AnnotationSet}
* @return the request
*/
public Update update(java.lang.String annotationSetId, com.google.api.services.genomics.model.AnnotationSet content) throws java.io.IOException {
Update result = new Update(annotationSetId, content);
initialize(result);
return result;
}
public class Update extends GenomicsRequest {
private static final String REST_PATH = "v1/annotationsets/{annotationSetId}";
/**
* Updates an annotation set. The update must respect all mutability restrictions and other
* invariants described on the annotation set resource. Caller must have WRITE permission for the
* associated dataset.
*
* Create a request for the method "annotationsets.update".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Update#execute()} method to invoke the remote operation.
* {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param annotationSetId The ID of the annotation set to be updated.
* @param content the {@link com.google.api.services.genomics.model.AnnotationSet}
* @since 1.13
*/
protected Update(java.lang.String annotationSetId, com.google.api.services.genomics.model.AnnotationSet content) {
super(Genomics.this, "PUT", REST_PATH, content, com.google.api.services.genomics.model.AnnotationSet.class);
this.annotationSetId = com.google.api.client.util.Preconditions.checkNotNull(annotationSetId, "Required parameter annotationSetId must be specified.");
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setBearerToken(java.lang.String bearerToken) {
return (Update) super.setBearerToken(bearerToken);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPp(java.lang.Boolean pp) {
return (Update) super.setPp(pp);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the annotation set to be updated. */
@com.google.api.client.util.Key
private java.lang.String annotationSetId;
/** The ID of the annotation set to be updated.
*/
public java.lang.String getAnnotationSetId() {
return annotationSetId;
}
/** The ID of the annotation set to be updated. */
public Update setAnnotationSetId(java.lang.String annotationSetId) {
this.annotationSetId = annotationSetId;
return this;
}
/**
* An optional mask specifying which fields to update. Mutable fields are name, source_uri,
* and info. If unspecified, all mutable fields will be updated.
*/
@com.google.api.client.util.Key
private java.lang.String updateMask;
/** An optional mask specifying which fields to update. Mutable fields are name, source_uri, and info.
If unspecified, all mutable fields will be updated.
*/
public java.lang.String getUpdateMask() {
return updateMask;
}
/**
* An optional mask specifying which fields to update. Mutable fields are name, source_uri,
* and info. If unspecified, all mutable fields will be updated.
*/
public Update setUpdateMask(java.lang.String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Callsets collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Callsets.List request = genomics.callsets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Callsets callsets() {
return new Callsets();
}
/**
* The "callsets" collection of methods.
*/
public class Callsets {
/**
* Creates a new call set. For the definitions of call sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "callsets.create".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.CallSet}
* @return the request
*/
public Create create(com.google.api.services.genomics.model.CallSet content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends GenomicsRequest {
private static final String REST_PATH = "v1/callsets";
/**
* Creates a new call set. For the definitions of call sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "callsets.create".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.CallSet}
* @since 1.13
*/
protected Create(com.google.api.services.genomics.model.CallSet content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.CallSet.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a call set. For the definitions of call sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "callsets.delete".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param callSetId The ID of the call set to be deleted.
* @return the request
*/
public Delete delete(java.lang.String callSetId) throws java.io.IOException {
Delete result = new Delete(callSetId);
initialize(result);
return result;
}
public class Delete extends GenomicsRequest {
private static final String REST_PATH = "v1/callsets/{callSetId}";
/**
* Deletes a call set. For the definitions of call sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "callsets.delete".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param callSetId The ID of the call set to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String callSetId) {
super(Genomics.this, "DELETE", REST_PATH, null, com.google.api.services.genomics.model.Empty.class);
this.callSetId = com.google.api.client.util.Preconditions.checkNotNull(callSetId, "Required parameter callSetId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the call set to be deleted. */
@com.google.api.client.util.Key
private java.lang.String callSetId;
/** The ID of the call set to be deleted.
*/
public java.lang.String getCallSetId() {
return callSetId;
}
/** The ID of the call set to be deleted. */
public Delete setCallSetId(java.lang.String callSetId) {
this.callSetId = callSetId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a call set by ID. For the definitions of call sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "callsets.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param callSetId The ID of the call set.
* @return the request
*/
public Get get(java.lang.String callSetId) throws java.io.IOException {
Get result = new Get(callSetId);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/callsets/{callSetId}";
/**
* Gets a call set by ID. For the definitions of call sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "callsets.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param callSetId The ID of the call set.
* @since 1.13
*/
protected Get(java.lang.String callSetId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.CallSet.class);
this.callSetId = com.google.api.client.util.Preconditions.checkNotNull(callSetId, "Required parameter callSetId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the call set. */
@com.google.api.client.util.Key
private java.lang.String callSetId;
/** The ID of the call set.
*/
public java.lang.String getCallSetId() {
return callSetId;
}
/** The ID of the call set. */
public Get setCallSetId(java.lang.String callSetId) {
this.callSetId = callSetId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Updates a call set. For the definitions of call sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) This method supports patch semantics.
*
* Create a request for the method "callsets.patch".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param callSetId The ID of the call set to be updated.
* @param content the {@link com.google.api.services.genomics.model.CallSet}
* @return the request
*/
public Patch patch(java.lang.String callSetId, com.google.api.services.genomics.model.CallSet content) throws java.io.IOException {
Patch result = new Patch(callSetId, content);
initialize(result);
return result;
}
public class Patch extends GenomicsRequest {
private static final String REST_PATH = "v1/callsets/{callSetId}";
/**
* Updates a call set. For the definitions of call sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) This method supports patch semantics.
*
* Create a request for the method "callsets.patch".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param callSetId The ID of the call set to be updated.
* @param content the {@link com.google.api.services.genomics.model.CallSet}
* @since 1.13
*/
protected Patch(java.lang.String callSetId, com.google.api.services.genomics.model.CallSet content) {
super(Genomics.this, "PATCH", REST_PATH, content, com.google.api.services.genomics.model.CallSet.class);
this.callSetId = com.google.api.client.util.Preconditions.checkNotNull(callSetId, "Required parameter callSetId must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setBearerToken(java.lang.String bearerToken) {
return (Patch) super.setBearerToken(bearerToken);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPp(java.lang.Boolean pp) {
return (Patch) super.setPp(pp);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the call set to be updated. */
@com.google.api.client.util.Key
private java.lang.String callSetId;
/** The ID of the call set to be updated.
*/
public java.lang.String getCallSetId() {
return callSetId;
}
/** The ID of the call set to be updated. */
public Patch setCallSetId(java.lang.String callSetId) {
this.callSetId = callSetId;
return this;
}
/**
* An optional mask specifying which fields to update. At this time, the only mutable field is
* name. The only acceptable value is "name". If unspecified, all mutable fields will be
* updated.
*/
@com.google.api.client.util.Key
private java.lang.String updateMask;
/** An optional mask specifying which fields to update. At this time, the only mutable field is name.
The only acceptable value is "name". If unspecified, all mutable fields will be updated.
*/
public java.lang.String getUpdateMask() {
return updateMask;
}
/**
* An optional mask specifying which fields to update. At this time, the only mutable field is
* name. The only acceptable value is "name". If unspecified, all mutable fields will be
* updated.
*/
public Patch setUpdateMask(java.lang.String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Gets a list of call sets matching the criteria. For the definitions of call sets and other
* genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics) Implements [GlobalAllianceApi.searchCallSets](https://github.co
* m/ga4gh/schemas/blob/v0.5.1/src/main/resources/avro/variantmethods.avdl#L178).
*
* Create a request for the method "callsets.search".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.SearchCallSetsRequest}
* @return the request
*/
public Search search(com.google.api.services.genomics.model.SearchCallSetsRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends GenomicsRequest {
private static final String REST_PATH = "v1/callsets/search";
/**
* Gets a list of call sets matching the criteria. For the definitions of call sets and other
* genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics) Implements [GlobalAllianceApi.searchCallSets](https://github.
* com/ga4gh/schemas/blob/v0.5.1/src/main/resources/avro/variantmethods.avdl#L178).
*
* Create a request for the method "callsets.search".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.SearchCallSetsRequest}
* @since 1.13
*/
protected Search(com.google.api.services.genomics.model.SearchCallSetsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.SearchCallSetsResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setBearerToken(java.lang.String bearerToken) {
return (Search) super.setBearerToken(bearerToken);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPp(java.lang.Boolean pp) {
return (Search) super.setPp(pp);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Datasets collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Datasets.List request = genomics.datasets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Datasets datasets() {
return new Datasets();
}
/**
* The "datasets" collection of methods.
*/
public class Datasets {
/**
* Creates a new dataset. For the definitions of datasets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "datasets.create".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.Dataset}
* @return the request
*/
public Create create(com.google.api.services.genomics.model.Dataset content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends GenomicsRequest {
private static final String REST_PATH = "v1/datasets";
/**
* Creates a new dataset. For the definitions of datasets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "datasets.create".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.Dataset}
* @since 1.13
*/
protected Create(com.google.api.services.genomics.model.Dataset content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Dataset.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a dataset and all of its contents (all read group sets, reference sets, variant sets,
* call sets, annotation sets, etc.) This is reversible (up to one week after the deletion) via the
* datasets.undelete operation. For the definitions of datasets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "datasets.delete".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param datasetId The ID of the dataset to be deleted.
* @return the request
*/
public Delete delete(java.lang.String datasetId) throws java.io.IOException {
Delete result = new Delete(datasetId);
initialize(result);
return result;
}
public class Delete extends GenomicsRequest {
private static final String REST_PATH = "v1/datasets/{datasetId}";
/**
* Deletes a dataset and all of its contents (all read group sets, reference sets, variant sets,
* call sets, annotation sets, etc.) This is reversible (up to one week after the deletion) via
* the datasets.undelete operation. For the definitions of datasets and other genomics resources,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "datasets.delete".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param datasetId The ID of the dataset to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String datasetId) {
super(Genomics.this, "DELETE", REST_PATH, null, com.google.api.services.genomics.model.Empty.class);
this.datasetId = com.google.api.client.util.Preconditions.checkNotNull(datasetId, "Required parameter datasetId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the dataset to be deleted. */
@com.google.api.client.util.Key
private java.lang.String datasetId;
/** The ID of the dataset to be deleted.
*/
public java.lang.String getDatasetId() {
return datasetId;
}
/** The ID of the dataset to be deleted. */
public Delete setDatasetId(java.lang.String datasetId) {
this.datasetId = datasetId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a dataset by ID. For the definitions of datasets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "datasets.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param datasetId The ID of the dataset.
* @return the request
*/
public Get get(java.lang.String datasetId) throws java.io.IOException {
Get result = new Get(datasetId);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/datasets/{datasetId}";
/**
* Gets a dataset by ID. For the definitions of datasets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "datasets.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param datasetId The ID of the dataset.
* @since 1.13
*/
protected Get(java.lang.String datasetId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.Dataset.class);
this.datasetId = com.google.api.client.util.Preconditions.checkNotNull(datasetId, "Required parameter datasetId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the dataset. */
@com.google.api.client.util.Key
private java.lang.String datasetId;
/** The ID of the dataset.
*/
public java.lang.String getDatasetId() {
return datasetId;
}
/** The ID of the dataset. */
public Get setDatasetId(java.lang.String datasetId) {
this.datasetId = datasetId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Gets the access control policy for the dataset. This is empty if the policy or resource does not
* exist. See Getting a Policy for more information. For the definitions of datasets and other
* genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics)
*
* Create a request for the method "datasets.getIamPolicy".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which policy is being specified. Format is `datasets/`.
* @param content the {@link com.google.api.services.genomics.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.genomics.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends GenomicsRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^datasets/[^/]*$");
/**
* Gets the access control policy for the dataset. This is empty if the policy or resource does
* not exist. See Getting a Policy for more information. For the definitions of datasets and other
* genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics)
*
* Create a request for the method "datasets.getIamPolicy".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which policy is being specified. Format is `datasets/`.
* @param content the {@link com.google.api.services.genomics.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.genomics.model.GetIamPolicyRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^datasets/[^/]*$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setBearerToken(java.lang.String bearerToken) {
return (GetIamPolicy) super.setBearerToken(bearerToken);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPp(java.lang.Boolean pp) {
return (GetIamPolicy) super.setPp(pp);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/** REQUIRED: The resource for which policy is being specified. Format is `datasets/`. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which policy is being specified. Format is `datasets/`.
*/
public java.lang.String getResource() {
return resource;
}
/** REQUIRED: The resource for which policy is being specified. Format is `datasets/`. */
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^datasets/[^/]*$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Lists datasets within a project. For the definitions of datasets and other genomics resources,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "datasets.list".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @return the request
*/
public List list() throws java.io.IOException {
List result = new List();
initialize(result);
return result;
}
public class List extends GenomicsRequest {
private static final String REST_PATH = "v1/datasets";
/**
* Lists datasets within a project. For the definitions of datasets and other genomics resources,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "datasets.list".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @since 1.13
*/
protected List() {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.ListDatasetsResponse.class);
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The project to list datasets for. */
@com.google.api.client.util.Key
private java.lang.String projectId;
/** Required. The project to list datasets for.
*/
public java.lang.String getProjectId() {
return projectId;
}
/** Required. The project to list datasets for. */
public List setProjectId(java.lang.String projectId) {
this.projectId = projectId;
return this;
}
/**
* The maximum number of results to return in a single page. If unspecified, defaults to 50.
* The maximum value is 1024.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return in a single page. If unspecified, defaults to 50. The
maximum value is 1024.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return in a single page. If unspecified, defaults to 50.
* The maximum value is 1024.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of `nextPageToken` from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, which is used to page through large result sets. To get the next page of
results, set this parameter to the value of `nextPageToken` from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of `nextPageToken` from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a dataset. For the definitions of datasets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) This method supports patch semantics.
*
* Create a request for the method "datasets.patch".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param datasetId The ID of the dataset to be updated.
* @param content the {@link com.google.api.services.genomics.model.Dataset}
* @return the request
*/
public Patch patch(java.lang.String datasetId, com.google.api.services.genomics.model.Dataset content) throws java.io.IOException {
Patch result = new Patch(datasetId, content);
initialize(result);
return result;
}
public class Patch extends GenomicsRequest {
private static final String REST_PATH = "v1/datasets/{datasetId}";
/**
* Updates a dataset. For the definitions of datasets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) This method supports patch semantics.
*
* Create a request for the method "datasets.patch".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param datasetId The ID of the dataset to be updated.
* @param content the {@link com.google.api.services.genomics.model.Dataset}
* @since 1.13
*/
protected Patch(java.lang.String datasetId, com.google.api.services.genomics.model.Dataset content) {
super(Genomics.this, "PATCH", REST_PATH, content, com.google.api.services.genomics.model.Dataset.class);
this.datasetId = com.google.api.client.util.Preconditions.checkNotNull(datasetId, "Required parameter datasetId must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setBearerToken(java.lang.String bearerToken) {
return (Patch) super.setBearerToken(bearerToken);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPp(java.lang.Boolean pp) {
return (Patch) super.setPp(pp);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the dataset to be updated. */
@com.google.api.client.util.Key
private java.lang.String datasetId;
/** The ID of the dataset to be updated.
*/
public java.lang.String getDatasetId() {
return datasetId;
}
/** The ID of the dataset to be updated. */
public Patch setDatasetId(java.lang.String datasetId) {
this.datasetId = datasetId;
return this;
}
/**
* An optional mask specifying which fields to update. At this time, the only mutable field is
* name. The only acceptable value is "name". If unspecified, all mutable fields will be
* updated.
*/
@com.google.api.client.util.Key
private java.lang.String updateMask;
/** An optional mask specifying which fields to update. At this time, the only mutable field is name.
The only acceptable value is "name". If unspecified, all mutable fields will be updated.
*/
public java.lang.String getUpdateMask() {
return updateMask;
}
/**
* An optional mask specifying which fields to update. At this time, the only mutable field is
* name. The only acceptable value is "name". If unspecified, all mutable fields will be
* updated.
*/
public Patch setUpdateMask(java.lang.String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Sets the access control policy on the specified dataset. Replaces any existing policy. For the
* definitions of datasets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) See Setting a Policy
* for more information.
*
* Create a request for the method "datasets.setIamPolicy".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which policy is being specified. Format is `datasets/`.
* @param content the {@link com.google.api.services.genomics.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.genomics.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends GenomicsRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^datasets/[^/]*$");
/**
* Sets the access control policy on the specified dataset. Replaces any existing policy. For the
* definitions of datasets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) See Setting a
* Policy for more information.
*
* Create a request for the method "datasets.setIamPolicy".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote
* operation. {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which policy is being specified. Format is `datasets/`.
* @param content the {@link com.google.api.services.genomics.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.genomics.model.SetIamPolicyRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^datasets/[^/]*$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setBearerToken(java.lang.String bearerToken) {
return (SetIamPolicy) super.setBearerToken(bearerToken);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPp(java.lang.Boolean pp) {
return (SetIamPolicy) super.setPp(pp);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/** REQUIRED: The resource for which policy is being specified. Format is `datasets/`. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which policy is being specified. Format is `datasets/`.
*/
public java.lang.String getResource() {
return resource;
}
/** REQUIRED: The resource for which policy is being specified. Format is `datasets/`. */
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^datasets/[^/]*$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the specified resource. See Testing Permissions for more
* information. For the definitions of datasets and other genomics resources, see [Fundamentals of
* Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics)
*
* Create a request for the method "datasets.testIamPermissions".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which policy is being specified. Format is `datasets/`.
* @param content the {@link com.google.api.services.genomics.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.genomics.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends GenomicsRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^datasets/[^/]*$");
/**
* Returns permissions that a caller has on the specified resource. See Testing Permissions for
* more information. For the definitions of datasets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "datasets.testIamPermissions".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which policy is being specified. Format is `datasets/`.
* @param content the {@link com.google.api.services.genomics.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.genomics.model.TestIamPermissionsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^datasets/[^/]*$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setBearerToken(java.lang.String bearerToken) {
return (TestIamPermissions) super.setBearerToken(bearerToken);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPp(java.lang.Boolean pp) {
return (TestIamPermissions) super.setPp(pp);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/** REQUIRED: The resource for which policy is being specified. Format is `datasets/`. */
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which policy is being specified. Format is `datasets/`.
*/
public java.lang.String getResource() {
return resource;
}
/** REQUIRED: The resource for which policy is being specified. Format is `datasets/`. */
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^datasets/[^/]*$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Undeletes a dataset by restoring a dataset which was deleted via this API. For the definitions of
* datasets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) This operation is
* only possible for a week after the deletion occurred.
*
* Create a request for the method "datasets.undelete".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
*
* @param datasetId The ID of the dataset to be undeleted.
* @param content the {@link com.google.api.services.genomics.model.UndeleteDatasetRequest}
* @return the request
*/
public Undelete undelete(java.lang.String datasetId, com.google.api.services.genomics.model.UndeleteDatasetRequest content) throws java.io.IOException {
Undelete result = new Undelete(datasetId, content);
initialize(result);
return result;
}
public class Undelete extends GenomicsRequest {
private static final String REST_PATH = "v1/datasets/{datasetId}:undelete";
/**
* Undeletes a dataset by restoring a dataset which was deleted via this API. For the definitions
* of datasets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) This operation is
* only possible for a week after the deletion occurred.
*
* Create a request for the method "datasets.undelete".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Undelete#execute()} method to invoke the remote operation.
* {@link
* Undelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param datasetId The ID of the dataset to be undeleted.
* @param content the {@link com.google.api.services.genomics.model.UndeleteDatasetRequest}
* @since 1.13
*/
protected Undelete(java.lang.String datasetId, com.google.api.services.genomics.model.UndeleteDatasetRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Dataset.class);
this.datasetId = com.google.api.client.util.Preconditions.checkNotNull(datasetId, "Required parameter datasetId must be specified.");
}
@Override
public Undelete set$Xgafv(java.lang.String $Xgafv) {
return (Undelete) super.set$Xgafv($Xgafv);
}
@Override
public Undelete setAccessToken(java.lang.String accessToken) {
return (Undelete) super.setAccessToken(accessToken);
}
@Override
public Undelete setAlt(java.lang.String alt) {
return (Undelete) super.setAlt(alt);
}
@Override
public Undelete setBearerToken(java.lang.String bearerToken) {
return (Undelete) super.setBearerToken(bearerToken);
}
@Override
public Undelete setCallback(java.lang.String callback) {
return (Undelete) super.setCallback(callback);
}
@Override
public Undelete setFields(java.lang.String fields) {
return (Undelete) super.setFields(fields);
}
@Override
public Undelete setKey(java.lang.String key) {
return (Undelete) super.setKey(key);
}
@Override
public Undelete setOauthToken(java.lang.String oauthToken) {
return (Undelete) super.setOauthToken(oauthToken);
}
@Override
public Undelete setPp(java.lang.Boolean pp) {
return (Undelete) super.setPp(pp);
}
@Override
public Undelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Undelete) super.setPrettyPrint(prettyPrint);
}
@Override
public Undelete setQuotaUser(java.lang.String quotaUser) {
return (Undelete) super.setQuotaUser(quotaUser);
}
@Override
public Undelete setUploadType(java.lang.String uploadType) {
return (Undelete) super.setUploadType(uploadType);
}
@Override
public Undelete setUploadProtocol(java.lang.String uploadProtocol) {
return (Undelete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the dataset to be undeleted. */
@com.google.api.client.util.Key
private java.lang.String datasetId;
/** The ID of the dataset to be undeleted.
*/
public java.lang.String getDatasetId() {
return datasetId;
}
/** The ID of the dataset to be undeleted. */
public Undelete setDatasetId(java.lang.String datasetId) {
this.datasetId = datasetId;
return this;
}
@Override
public Undelete set(String parameterName, Object value) {
return (Undelete) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Operations.List request = genomics.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. Clients may use Operations.GetOperation or
* Operations.ListOperations to check whether the cancellation succeeded or the operation completed
* despite cancellation.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.genomics.model.CancelOperationRequest}
* @return the request
*/
public Cancel cancel(java.lang.String name, com.google.api.services.genomics.model.CancelOperationRequest content) throws java.io.IOException {
Cancel result = new Cancel(name, content);
initialize(result);
return result;
}
public class Cancel extends GenomicsRequest {
private static final String REST_PATH = "v1/{+name}:cancel";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Starts asynchronous cancellation on a long-running operation. The server makes a best effort to
* cancel the operation, but success is not guaranteed. Clients may use Operations.GetOperation or
* Operations.ListOperations to check whether the cancellation succeeded or the operation
* completed despite cancellation.
*
* Create a request for the method "operations.cancel".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Cancel#execute()} method to invoke the remote operation.
* {@link
* Cancel#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource to be cancelled.
* @param content the {@link com.google.api.services.genomics.model.CancelOperationRequest}
* @since 1.13
*/
protected Cancel(java.lang.String name, com.google.api.services.genomics.model.CancelOperationRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public Cancel set$Xgafv(java.lang.String $Xgafv) {
return (Cancel) super.set$Xgafv($Xgafv);
}
@Override
public Cancel setAccessToken(java.lang.String accessToken) {
return (Cancel) super.setAccessToken(accessToken);
}
@Override
public Cancel setAlt(java.lang.String alt) {
return (Cancel) super.setAlt(alt);
}
@Override
public Cancel setBearerToken(java.lang.String bearerToken) {
return (Cancel) super.setBearerToken(bearerToken);
}
@Override
public Cancel setCallback(java.lang.String callback) {
return (Cancel) super.setCallback(callback);
}
@Override
public Cancel setFields(java.lang.String fields) {
return (Cancel) super.setFields(fields);
}
@Override
public Cancel setKey(java.lang.String key) {
return (Cancel) super.setKey(key);
}
@Override
public Cancel setOauthToken(java.lang.String oauthToken) {
return (Cancel) super.setOauthToken(oauthToken);
}
@Override
public Cancel setPp(java.lang.Boolean pp) {
return (Cancel) super.setPp(pp);
}
@Override
public Cancel setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Cancel) super.setPrettyPrint(prettyPrint);
}
@Override
public Cancel setQuotaUser(java.lang.String quotaUser) {
return (Cancel) super.setQuotaUser(quotaUser);
}
@Override
public Cancel setUploadType(java.lang.String uploadType) {
return (Cancel) super.setUploadType(uploadType);
}
@Override
public Cancel setUploadProtocol(java.lang.String uploadProtocol) {
return (Cancel) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource to be cancelled. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource to be cancelled.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource to be cancelled. */
public Cancel setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Cancel set(String parameterName, Object value) {
return (Cancel) super.set(parameterName, value);
}
}
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations/.*$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.Operation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations/.*$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists operations that match the specified filter in the request.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param name The name of the operation collection.
* @return the request
*/
public List list(java.lang.String name) throws java.io.IOException {
List result = new List(name);
initialize(result);
return result;
}
public class List extends GenomicsRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^operations$");
/**
* Lists operations that match the specified filter in the request.
*
* Create a request for the method "operations.list".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation collection.
* @since 1.13
*/
protected List(java.lang.String name) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.ListOperationsResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation collection. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation collection.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation collection. */
public List setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^operations$");
}
this.name = name;
return this;
}
/**
* A string for filtering Operations. The following filter fields are supported: * projectId:
* Required. Corresponds to OperationMetadata.projectId. * createTime: The time this job was
* created, in seconds from the [epoch](http://en.wikipedia.org/wiki/Unix_time). Can use `>=`
* and/or `= 1432140000` * `projectId = my-project AND createTime >= 1432140000 AND createTime
* <= 1432150000 AND status = RUNNING`
*/
@com.google.api.client.util.Key
private java.lang.String filter;
/** A string for filtering Operations. The following filter fields are supported: * projectId:
Required. Corresponds to OperationMetadata.projectId. * createTime: The time this job was created,
in seconds from the [epoch](http://en.wikipedia.org/wiki/Unix_time). Can use `>=` and/or `=
1432140000` * `projectId = my-project AND createTime >= 1432140000 AND createTime <= 1432150000 AND
status = RUNNING`
*/
public java.lang.String getFilter() {
return filter;
}
/**
* A string for filtering Operations. The following filter fields are supported: * projectId:
* Required. Corresponds to OperationMetadata.projectId. * createTime: The time this job was
* created, in seconds from the [epoch](http://en.wikipedia.org/wiki/Unix_time). Can use `>=`
* and/or `= 1432140000` * `projectId = my-project AND createTime >= 1432140000 AND createTime
* <= 1432150000 AND status = RUNNING`
*/
public List setFilter(java.lang.String filter) {
this.filter = filter;
return this;
}
/**
* The maximum number of results to return. If unspecified, defaults to 256. The maximum value
* is 2048.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return. If unspecified, defaults to 256. The maximum value is
2048.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return. If unspecified, defaults to 256. The maximum value
* is 2048.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/** The standard list page token. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The standard list page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** The standard list page token. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Readgroupsets collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Readgroupsets.List request = genomics.readgroupsets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Readgroupsets readgroupsets() {
return new Readgroupsets();
}
/**
* The "readgroupsets" collection of methods.
*/
public class Readgroupsets {
/**
* Deletes a read group set. For the definitions of read group sets and other genomics resources,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "readgroupsets.delete".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param readGroupSetId The ID of the read group set to be deleted. The caller must have WRITE permissions to the dataset
* associated with this read group set.
* @return the request
*/
public Delete delete(java.lang.String readGroupSetId) throws java.io.IOException {
Delete result = new Delete(readGroupSetId);
initialize(result);
return result;
}
public class Delete extends GenomicsRequest {
private static final String REST_PATH = "v1/readgroupsets/{readGroupSetId}";
/**
* Deletes a read group set. For the definitions of read group sets and other genomics resources,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "readgroupsets.delete".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param readGroupSetId The ID of the read group set to be deleted. The caller must have WRITE permissions to the dataset
* associated with this read group set.
* @since 1.13
*/
protected Delete(java.lang.String readGroupSetId) {
super(Genomics.this, "DELETE", REST_PATH, null, com.google.api.services.genomics.model.Empty.class);
this.readGroupSetId = com.google.api.client.util.Preconditions.checkNotNull(readGroupSetId, "Required parameter readGroupSetId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* The ID of the read group set to be deleted. The caller must have WRITE permissions to the
* dataset associated with this read group set.
*/
@com.google.api.client.util.Key
private java.lang.String readGroupSetId;
/** The ID of the read group set to be deleted. The caller must have WRITE permissions to the dataset
associated with this read group set.
*/
public java.lang.String getReadGroupSetId() {
return readGroupSetId;
}
/**
* The ID of the read group set to be deleted. The caller must have WRITE permissions to the
* dataset associated with this read group set.
*/
public Delete setReadGroupSetId(java.lang.String readGroupSetId) {
this.readGroupSetId = readGroupSetId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Exports a read group set to a BAM file in Google Cloud Storage. For the definitions of read group
* sets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Note that currently
* there may be some differences between exported BAM files and the original BAM file at the time of
* import. See ImportReadGroupSets for caveats.
*
* Create a request for the method "readgroupsets.export".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param readGroupSetId Required. The ID of the read group set to export. The caller must have READ access to this read
* group set.
* @param content the {@link com.google.api.services.genomics.model.ExportReadGroupSetRequest}
* @return the request
*/
public Export export(java.lang.String readGroupSetId, com.google.api.services.genomics.model.ExportReadGroupSetRequest content) throws java.io.IOException {
Export result = new Export(readGroupSetId, content);
initialize(result);
return result;
}
public class Export extends GenomicsRequest {
private static final String REST_PATH = "v1/readgroupsets/{readGroupSetId}:export";
/**
* Exports a read group set to a BAM file in Google Cloud Storage. For the definitions of read
* group sets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Note that
* currently there may be some differences between exported BAM files and the original BAM file at
* the time of import. See ImportReadGroupSets for caveats.
*
* Create a request for the method "readgroupsets.export".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Export#execute()} method to invoke the remote operation.
* {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param readGroupSetId Required. The ID of the read group set to export. The caller must have READ access to this read
* group set.
* @param content the {@link com.google.api.services.genomics.model.ExportReadGroupSetRequest}
* @since 1.13
*/
protected Export(java.lang.String readGroupSetId, com.google.api.services.genomics.model.ExportReadGroupSetRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Operation.class);
this.readGroupSetId = com.google.api.client.util.Preconditions.checkNotNull(readGroupSetId, "Required parameter readGroupSetId must be specified.");
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setBearerToken(java.lang.String bearerToken) {
return (Export) super.setBearerToken(bearerToken);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPp(java.lang.Boolean pp) {
return (Export) super.setPp(pp);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The ID of the read group set to export. The caller must have READ access to this
* read group set.
*/
@com.google.api.client.util.Key
private java.lang.String readGroupSetId;
/** Required. The ID of the read group set to export. The caller must have READ access to this read
group set.
*/
public java.lang.String getReadGroupSetId() {
return readGroupSetId;
}
/**
* Required. The ID of the read group set to export. The caller must have READ access to this
* read group set.
*/
public Export setReadGroupSetId(java.lang.String readGroupSetId) {
this.readGroupSetId = readGroupSetId;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Gets a read group set by ID. For the definitions of read group sets and other genomics resources,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "readgroupsets.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param readGroupSetId The ID of the read group set.
* @return the request
*/
public Get get(java.lang.String readGroupSetId) throws java.io.IOException {
Get result = new Get(readGroupSetId);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/readgroupsets/{readGroupSetId}";
/**
* Gets a read group set by ID. For the definitions of read group sets and other genomics
* resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics)
*
* Create a request for the method "readgroupsets.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param readGroupSetId The ID of the read group set.
* @since 1.13
*/
protected Get(java.lang.String readGroupSetId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.ReadGroupSet.class);
this.readGroupSetId = com.google.api.client.util.Preconditions.checkNotNull(readGroupSetId, "Required parameter readGroupSetId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the read group set. */
@com.google.api.client.util.Key
private java.lang.String readGroupSetId;
/** The ID of the read group set.
*/
public java.lang.String getReadGroupSetId() {
return readGroupSetId;
}
/** The ID of the read group set. */
public Get setReadGroupSetId(java.lang.String readGroupSetId) {
this.readGroupSetId = readGroupSetId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates read group sets by asynchronously importing the provided information. For the definitions
* of read group sets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) The caller must have
* WRITE permissions to the dataset. ## Notes on [BAM](https://samtools.github.io/hts-
* specs/SAMv1.pdf) import - Tags will be converted to strings - tag types are not preserved -
* Comments (`@CO`) in the input file header will not be preserved - Original header order of
* references (`@SQ`) will not be preserved - Any reverse stranded unmapped reads will be reverse
* complemented, and their qualities (also the "BQ" and "OQ" tags, if any) will be reversed -
* Unmapped reads will be stripped of positional information (reference name and position)
*
* Create a request for the method "readgroupsets.import".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link GenomicsImport#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.ImportReadGroupSetsRequest}
* @return the request
*/
public GenomicsImport genomicsImport(com.google.api.services.genomics.model.ImportReadGroupSetsRequest content) throws java.io.IOException {
GenomicsImport result = new GenomicsImport(content);
initialize(result);
return result;
}
public class GenomicsImport extends GenomicsRequest {
private static final String REST_PATH = "v1/readgroupsets:import";
/**
* Creates read group sets by asynchronously importing the provided information. For the
* definitions of read group sets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) The caller must
* have WRITE permissions to the dataset. ## Notes on [BAM](https://samtools.github.io/hts-
* specs/SAMv1.pdf) import - Tags will be converted to strings - tag types are not preserved -
* Comments (`@CO`) in the input file header will not be preserved - Original header order of
* references (`@SQ`) will not be preserved - Any reverse stranded unmapped reads will be reverse
* complemented, and their qualities (also the "BQ" and "OQ" tags, if any) will be reversed -
* Unmapped reads will be stripped of positional information (reference name and position)
*
* Create a request for the method "readgroupsets.import".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link GenomicsImport#execute()} method to invoke the remote
* operation. {@link GenomicsImport#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.ImportReadGroupSetsRequest}
* @since 1.13
*/
protected GenomicsImport(com.google.api.services.genomics.model.ImportReadGroupSetsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Operation.class);
}
@Override
public GenomicsImport set$Xgafv(java.lang.String $Xgafv) {
return (GenomicsImport) super.set$Xgafv($Xgafv);
}
@Override
public GenomicsImport setAccessToken(java.lang.String accessToken) {
return (GenomicsImport) super.setAccessToken(accessToken);
}
@Override
public GenomicsImport setAlt(java.lang.String alt) {
return (GenomicsImport) super.setAlt(alt);
}
@Override
public GenomicsImport setBearerToken(java.lang.String bearerToken) {
return (GenomicsImport) super.setBearerToken(bearerToken);
}
@Override
public GenomicsImport setCallback(java.lang.String callback) {
return (GenomicsImport) super.setCallback(callback);
}
@Override
public GenomicsImport setFields(java.lang.String fields) {
return (GenomicsImport) super.setFields(fields);
}
@Override
public GenomicsImport setKey(java.lang.String key) {
return (GenomicsImport) super.setKey(key);
}
@Override
public GenomicsImport setOauthToken(java.lang.String oauthToken) {
return (GenomicsImport) super.setOauthToken(oauthToken);
}
@Override
public GenomicsImport setPp(java.lang.Boolean pp) {
return (GenomicsImport) super.setPp(pp);
}
@Override
public GenomicsImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenomicsImport) super.setPrettyPrint(prettyPrint);
}
@Override
public GenomicsImport setQuotaUser(java.lang.String quotaUser) {
return (GenomicsImport) super.setQuotaUser(quotaUser);
}
@Override
public GenomicsImport setUploadType(java.lang.String uploadType) {
return (GenomicsImport) super.setUploadType(uploadType);
}
@Override
public GenomicsImport setUploadProtocol(java.lang.String uploadProtocol) {
return (GenomicsImport) super.setUploadProtocol(uploadProtocol);
}
@Override
public GenomicsImport set(String parameterName, Object value) {
return (GenomicsImport) super.set(parameterName, value);
}
}
/**
* Updates a read group set. For the definitions of read group sets and other genomics resources,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) This method supports patch semantics.
*
* Create a request for the method "readgroupsets.patch".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param readGroupSetId The ID of the read group set to be updated. The caller must have WRITE permissions to the dataset
* associated with this read group set.
* @param content the {@link com.google.api.services.genomics.model.ReadGroupSet}
* @return the request
*/
public Patch patch(java.lang.String readGroupSetId, com.google.api.services.genomics.model.ReadGroupSet content) throws java.io.IOException {
Patch result = new Patch(readGroupSetId, content);
initialize(result);
return result;
}
public class Patch extends GenomicsRequest {
private static final String REST_PATH = "v1/readgroupsets/{readGroupSetId}";
/**
* Updates a read group set. For the definitions of read group sets and other genomics resources,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) This method supports patch semantics.
*
* Create a request for the method "readgroupsets.patch".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param readGroupSetId The ID of the read group set to be updated. The caller must have WRITE permissions to the dataset
* associated with this read group set.
* @param content the {@link com.google.api.services.genomics.model.ReadGroupSet}
* @since 1.13
*/
protected Patch(java.lang.String readGroupSetId, com.google.api.services.genomics.model.ReadGroupSet content) {
super(Genomics.this, "PATCH", REST_PATH, content, com.google.api.services.genomics.model.ReadGroupSet.class);
this.readGroupSetId = com.google.api.client.util.Preconditions.checkNotNull(readGroupSetId, "Required parameter readGroupSetId must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setBearerToken(java.lang.String bearerToken) {
return (Patch) super.setBearerToken(bearerToken);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPp(java.lang.Boolean pp) {
return (Patch) super.setPp(pp);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* The ID of the read group set to be updated. The caller must have WRITE permissions to the
* dataset associated with this read group set.
*/
@com.google.api.client.util.Key
private java.lang.String readGroupSetId;
/** The ID of the read group set to be updated. The caller must have WRITE permissions to the dataset
associated with this read group set.
*/
public java.lang.String getReadGroupSetId() {
return readGroupSetId;
}
/**
* The ID of the read group set to be updated. The caller must have WRITE permissions to the
* dataset associated with this read group set.
*/
public Patch setReadGroupSetId(java.lang.String readGroupSetId) {
this.readGroupSetId = readGroupSetId;
return this;
}
/**
* An optional mask specifying which fields to update. Supported fields: * name. *
* referenceSetId. Leaving `updateMask` unset is equivalent to specifying all mutable fields.
*/
@com.google.api.client.util.Key
private java.lang.String updateMask;
/** An optional mask specifying which fields to update. Supported fields: * name. * referenceSetId.
Leaving `updateMask` unset is equivalent to specifying all mutable fields.
*/
public java.lang.String getUpdateMask() {
return updateMask;
}
/**
* An optional mask specifying which fields to update. Supported fields: * name. *
* referenceSetId. Leaving `updateMask` unset is equivalent to specifying all mutable fields.
*/
public Patch setUpdateMask(java.lang.String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Searches for read group sets matching the criteria. For the definitions of read group sets and
* other genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics) Implements [GlobalAllianceApi.searchReadGroupSets](https://gith
* ub.com/ga4gh/schemas/blob/v0.5.1/src/main/resources/avro/readmethods.avdl#L135).
*
* Create a request for the method "readgroupsets.search".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.SearchReadGroupSetsRequest}
* @return the request
*/
public Search search(com.google.api.services.genomics.model.SearchReadGroupSetsRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends GenomicsRequest {
private static final String REST_PATH = "v1/readgroupsets/search";
/**
* Searches for read group sets matching the criteria. For the definitions of read group sets and
* other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Implements [Global
* AllianceApi.searchReadGroupSets](https://github.com/ga4gh/schemas/blob/v0.5.1/src/main/resource
* s/avro/readmethods.avdl#L135).
*
* Create a request for the method "readgroupsets.search".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.SearchReadGroupSetsRequest}
* @since 1.13
*/
protected Search(com.google.api.services.genomics.model.SearchReadGroupSetsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.SearchReadGroupSetsResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setBearerToken(java.lang.String bearerToken) {
return (Search) super.setBearerToken(bearerToken);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPp(java.lang.Boolean pp) {
return (Search) super.setPp(pp);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Coveragebuckets collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Coveragebuckets.List request = genomics.coveragebuckets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Coveragebuckets coveragebuckets() {
return new Coveragebuckets();
}
/**
* The "coveragebuckets" collection of methods.
*/
public class Coveragebuckets {
/**
* Lists fixed width coverage buckets for a read group set, each of which correspond to a range of a
* reference sequence. Each bucket summarizes coverage information across its corresponding genomic
* range. For the definitions of read group sets and other genomics resources, see [Fundamentals of
* Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Coverage is
* defined as the number of reads which are aligned to a given base in the reference sequence.
* Coverage buckets are available at several precomputed bucket widths, enabling retrieval of
* various coverage 'zoom levels'. The caller must have READ permissions for the target read group
* set.
*
* Create a request for the method "coveragebuckets.list".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param readGroupSetId Required. The ID of the read group set over which coverage is requested.
* @return the request
*/
public List list(java.lang.String readGroupSetId) throws java.io.IOException {
List result = new List(readGroupSetId);
initialize(result);
return result;
}
public class List extends GenomicsRequest {
private static final String REST_PATH = "v1/readgroupsets/{readGroupSetId}/coveragebuckets";
/**
* Lists fixed width coverage buckets for a read group set, each of which correspond to a range of
* a reference sequence. Each bucket summarizes coverage information across its corresponding
* genomic range. For the definitions of read group sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) Coverage is defined as the number of reads which are aligned to a given base in the
* reference sequence. Coverage buckets are available at several precomputed bucket widths,
* enabling retrieval of various coverage 'zoom levels'. The caller must have READ permissions for
* the target read group set.
*
* Create a request for the method "coveragebuckets.list".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param readGroupSetId Required. The ID of the read group set over which coverage is requested.
* @since 1.13
*/
protected List(java.lang.String readGroupSetId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.ListCoverageBucketsResponse.class);
this.readGroupSetId = com.google.api.client.util.Preconditions.checkNotNull(readGroupSetId, "Required parameter readGroupSetId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the read group set over which coverage is requested. */
@com.google.api.client.util.Key
private java.lang.String readGroupSetId;
/** Required. The ID of the read group set over which coverage is requested.
*/
public java.lang.String getReadGroupSetId() {
return readGroupSetId;
}
/** Required. The ID of the read group set over which coverage is requested. */
public List setReadGroupSetId(java.lang.String readGroupSetId) {
this.readGroupSetId = readGroupSetId;
return this;
}
/**
* The name of the reference to query, within the reference set associated with this query.
* Optional.
*/
@com.google.api.client.util.Key
private java.lang.String referenceName;
/** The name of the reference to query, within the reference set associated with this query. Optional.
*/
public java.lang.String getReferenceName() {
return referenceName;
}
/**
* The name of the reference to query, within the reference set associated with this query.
* Optional.
*/
public List setReferenceName(java.lang.String referenceName) {
this.referenceName = referenceName;
return this;
}
/**
* The start position of the range on the reference, 0-based inclusive. If specified,
* `referenceName` must also be specified. Defaults to 0.
*/
@com.google.api.client.util.Key
private java.lang.Long start;
/** The start position of the range on the reference, 0-based inclusive. If specified, `referenceName`
must also be specified. Defaults to 0.
*/
public java.lang.Long getStart() {
return start;
}
/**
* The start position of the range on the reference, 0-based inclusive. If specified,
* `referenceName` must also be specified. Defaults to 0.
*/
public List setStart(java.lang.Long start) {
this.start = start;
return this;
}
/**
* The end position of the range on the reference, 0-based exclusive. If specified,
* `referenceName` must also be specified. If unset or 0, defaults to the length of the
* reference.
*/
@com.google.api.client.util.Key
private java.lang.Long end;
/** The end position of the range on the reference, 0-based exclusive. If specified, `referenceName`
must also be specified. If unset or 0, defaults to the length of the reference.
*/
public java.lang.Long getEnd() {
return end;
}
/**
* The end position of the range on the reference, 0-based exclusive. If specified,
* `referenceName` must also be specified. If unset or 0, defaults to the length of the
* reference.
*/
public List setEnd(java.lang.Long end) {
this.end = end;
return this;
}
/**
* The desired width of each reported coverage bucket in base pairs. This will be rounded
* down to the nearest precomputed bucket width; the value of which is returned as
* `bucketWidth` in the response. Defaults to infinity (each bucket spans an entire
* reference sequence) or the length of the target range, if specified. The smallest
* precomputed `bucketWidth` is currently 2048 base pairs; this is subject to change.
*/
@com.google.api.client.util.Key
private java.lang.Long targetBucketWidth;
/** The desired width of each reported coverage bucket in base pairs. This will be rounded down to the
nearest precomputed bucket width; the value of which is returned as `bucketWidth` in the response.
Defaults to infinity (each bucket spans an entire reference sequence) or the length of the target
range, if specified. The smallest precomputed `bucketWidth` is currently 2048 base pairs; this is
subject to change.
*/
public java.lang.Long getTargetBucketWidth() {
return targetBucketWidth;
}
/**
* The desired width of each reported coverage bucket in base pairs. This will be rounded
* down to the nearest precomputed bucket width; the value of which is returned as
* `bucketWidth` in the response. Defaults to infinity (each bucket spans an entire
* reference sequence) or the length of the target range, if specified. The smallest
* precomputed `bucketWidth` is currently 2048 base pairs; this is subject to change.
*/
public List setTargetBucketWidth(java.lang.Long targetBucketWidth) {
this.targetBucketWidth = targetBucketWidth;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of `nextPageToken` from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, which is used to page through large result sets. To get the next page of
results, set this parameter to the value of `nextPageToken` from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of `nextPageToken` from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of results to return in a single page. If unspecified, defaults to
* 1024. The maximum value is 2048.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of results to return in a single page. If unspecified, defaults to 1024. The
maximum value is 2048.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of results to return in a single page. If unspecified, defaults to
* 1024. The maximum value is 2048.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Reads collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Reads.List request = genomics.reads().list(parameters ...)}
*
*
* @return the resource collection
*/
public Reads reads() {
return new Reads();
}
/**
* The "reads" collection of methods.
*/
public class Reads {
/**
* Gets a list of reads for one or more read group sets. For the definitions of read group sets and
* other genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics) Reads search operates over a genomic coordinate space of
* reference sequence & position defined over the reference sequences to which the requested read
* group sets are aligned. If a target positional range is specified, search returns all reads whose
* alignment to the reference genome overlap the range. A query which specifies only read group set
* IDs yields all reads in those read group sets, including unmapped reads. All reads returned
* (including reads on subsequent pages) are ordered by genomic coordinate (by reference sequence,
* then position). Reads with equivalent genomic coordinates are returned in an unspecified order.
* This order is consistent, such that two queries for the same content (regardless of page size)
* yield reads in the same order across their respective streams of paginated responses. Implements
* [GlobalAllianceApi.searchReads](https://github.com/ga4gh/schemas/blob/v0.5.1/src/main/resources/a
* vro/readmethods.avdl#L85).
*
* Create a request for the method "reads.search".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.SearchReadsRequest}
* @return the request
*/
public Search search(com.google.api.services.genomics.model.SearchReadsRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends GenomicsRequest {
private static final String REST_PATH = "v1/reads/search";
/**
* Gets a list of reads for one or more read group sets. For the definitions of read group sets
* and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Reads search
* operates over a genomic coordinate space of reference sequence & position defined over the
* reference sequences to which the requested read group sets are aligned. If a target positional
* range is specified, search returns all reads whose alignment to the reference genome overlap
* the range. A query which specifies only read group set IDs yields all reads in those read group
* sets, including unmapped reads. All reads returned (including reads on subsequent pages) are
* ordered by genomic coordinate (by reference sequence, then position). Reads with equivalent
* genomic coordinates are returned in an unspecified order. This order is consistent, such that
* two queries for the same content (regardless of page size) yield reads in the same order across
* their respective streams of paginated responses. Implements [GlobalAllianceApi.searchReads](htt
* ps://github.com/ga4gh/schemas/blob/v0.5.1/src/main/resources/avro/readmethods.avdl#L85).
*
* Create a request for the method "reads.search".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.SearchReadsRequest}
* @since 1.13
*/
protected Search(com.google.api.services.genomics.model.SearchReadsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.SearchReadsResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setBearerToken(java.lang.String bearerToken) {
return (Search) super.setBearerToken(bearerToken);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPp(java.lang.Boolean pp) {
return (Search) super.setPp(pp);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* Returns a stream of all the reads matching the search request, ordered by reference name,
* position, and ID.
*
* Create a request for the method "reads.stream".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Stream#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.StreamReadsRequest}
* @return the request
*/
public Stream stream(com.google.api.services.genomics.model.StreamReadsRequest content) throws java.io.IOException {
Stream result = new Stream(content);
initialize(result);
return result;
}
public class Stream extends GenomicsRequest {
private static final String REST_PATH = "v1/reads:stream";
/**
* Returns a stream of all the reads matching the search request, ordered by reference name,
* position, and ID.
*
* Create a request for the method "reads.stream".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Stream#execute()} method to invoke the remote operation.
* {@link
* Stream#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.StreamReadsRequest}
* @since 1.13
*/
protected Stream(com.google.api.services.genomics.model.StreamReadsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.StreamReadsResponse.class);
}
@Override
public Stream set$Xgafv(java.lang.String $Xgafv) {
return (Stream) super.set$Xgafv($Xgafv);
}
@Override
public Stream setAccessToken(java.lang.String accessToken) {
return (Stream) super.setAccessToken(accessToken);
}
@Override
public Stream setAlt(java.lang.String alt) {
return (Stream) super.setAlt(alt);
}
@Override
public Stream setBearerToken(java.lang.String bearerToken) {
return (Stream) super.setBearerToken(bearerToken);
}
@Override
public Stream setCallback(java.lang.String callback) {
return (Stream) super.setCallback(callback);
}
@Override
public Stream setFields(java.lang.String fields) {
return (Stream) super.setFields(fields);
}
@Override
public Stream setKey(java.lang.String key) {
return (Stream) super.setKey(key);
}
@Override
public Stream setOauthToken(java.lang.String oauthToken) {
return (Stream) super.setOauthToken(oauthToken);
}
@Override
public Stream setPp(java.lang.Boolean pp) {
return (Stream) super.setPp(pp);
}
@Override
public Stream setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Stream) super.setPrettyPrint(prettyPrint);
}
@Override
public Stream setQuotaUser(java.lang.String quotaUser) {
return (Stream) super.setQuotaUser(quotaUser);
}
@Override
public Stream setUploadType(java.lang.String uploadType) {
return (Stream) super.setUploadType(uploadType);
}
@Override
public Stream setUploadProtocol(java.lang.String uploadProtocol) {
return (Stream) super.setUploadProtocol(uploadProtocol);
}
@Override
public Stream set(String parameterName, Object value) {
return (Stream) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the References collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.References.List request = genomics.references().list(parameters ...)}
*
*
* @return the resource collection
*/
public References references() {
return new References();
}
/**
* The "references" collection of methods.
*/
public class References {
/**
* Gets a reference. For the definitions of references and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) Implements [GlobalAllianceApi.getReference](https://github.com/ga4gh/schemas/blob/v0.5.
* 1/src/main/resources/avro/referencemethods.avdl#L158).
*
* Create a request for the method "references.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param referenceId The ID of the reference.
* @return the request
*/
public Get get(java.lang.String referenceId) throws java.io.IOException {
Get result = new Get(referenceId);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/references/{referenceId}";
/**
* Gets a reference. For the definitions of references and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) Implements [GlobalAllianceApi.getReference](https://github.com/ga4gh/schemas/blob/v0.
* 5.1/src/main/resources/avro/referencemethods.avdl#L158).
*
* Create a request for the method "references.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param referenceId The ID of the reference.
* @since 1.13
*/
protected Get(java.lang.String referenceId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.Reference.class);
this.referenceId = com.google.api.client.util.Preconditions.checkNotNull(referenceId, "Required parameter referenceId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the reference. */
@com.google.api.client.util.Key
private java.lang.String referenceId;
/** The ID of the reference.
*/
public java.lang.String getReferenceId() {
return referenceId;
}
/** The ID of the reference. */
public Get setReferenceId(java.lang.String referenceId) {
this.referenceId = referenceId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Searches for references which match the given criteria. For the definitions of references and
* other genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics) Implements [GlobalAllianceApi.searchReferences](https://github.
* com/ga4gh/schemas/blob/v0.5.1/src/main/resources/avro/referencemethods.avdl#L146).
*
* Create a request for the method "references.search".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.SearchReferencesRequest}
* @return the request
*/
public Search search(com.google.api.services.genomics.model.SearchReferencesRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends GenomicsRequest {
private static final String REST_PATH = "v1/references/search";
/**
* Searches for references which match the given criteria. For the definitions of references and
* other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Implements [Global
* AllianceApi.searchReferences](https://github.com/ga4gh/schemas/blob/v0.5.1/src/main/resources/a
* vro/referencemethods.avdl#L146).
*
* Create a request for the method "references.search".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.SearchReferencesRequest}
* @since 1.13
*/
protected Search(com.google.api.services.genomics.model.SearchReferencesRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.SearchReferencesResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setBearerToken(java.lang.String bearerToken) {
return (Search) super.setBearerToken(bearerToken);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPp(java.lang.Boolean pp) {
return (Search) super.setPp(pp);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Bases collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Bases.List request = genomics.bases().list(parameters ...)}
*
*
* @return the resource collection
*/
public Bases bases() {
return new Bases();
}
/**
* The "bases" collection of methods.
*/
public class Bases {
/**
* Lists the bases in a reference, optionally restricted to a range. For the definitions of
* references and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Implements [GlobalAl
* lianceApi.getReferenceBases](https://github.com/ga4gh/schemas/blob/v0.5.1/src/main/resources/avro
* /referencemethods.avdl#L221).
*
* Create a request for the method "bases.list".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param referenceId The ID of the reference.
* @return the request
*/
public List list(java.lang.String referenceId) throws java.io.IOException {
List result = new List(referenceId);
initialize(result);
return result;
}
public class List extends GenomicsRequest {
private static final String REST_PATH = "v1/references/{referenceId}/bases";
/**
* Lists the bases in a reference, optionally restricted to a range. For the definitions of
* references and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Implements [Global
* AllianceApi.getReferenceBases](https://github.com/ga4gh/schemas/blob/v0.5.1/src/main/resources/
* avro/referencemethods.avdl#L221).
*
* Create a request for the method "bases.list".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link List#execute()} method to invoke the remote operation.
* {@link List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param referenceId The ID of the reference.
* @since 1.13
*/
protected List(java.lang.String referenceId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.ListBasesResponse.class);
this.referenceId = com.google.api.client.util.Preconditions.checkNotNull(referenceId, "Required parameter referenceId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setBearerToken(java.lang.String bearerToken) {
return (List) super.setBearerToken(bearerToken);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPp(java.lang.Boolean pp) {
return (List) super.setPp(pp);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the reference. */
@com.google.api.client.util.Key
private java.lang.String referenceId;
/** The ID of the reference.
*/
public java.lang.String getReferenceId() {
return referenceId;
}
/** The ID of the reference. */
public List setReferenceId(java.lang.String referenceId) {
this.referenceId = referenceId;
return this;
}
/** The start position (0-based) of this query. Defaults to 0. */
@com.google.api.client.util.Key
private java.lang.Long start;
/** The start position (0-based) of this query. Defaults to 0.
*/
public java.lang.Long getStart() {
return start;
}
/** The start position (0-based) of this query. Defaults to 0. */
public List setStart(java.lang.Long start) {
this.start = start;
return this;
}
/**
* The end position (0-based, exclusive) of this query. Defaults to the length of this
* reference.
*/
@com.google.api.client.util.Key
private java.lang.Long end;
/** The end position (0-based, exclusive) of this query. Defaults to the length of this reference.
*/
public java.lang.Long getEnd() {
return end;
}
/**
* The end position (0-based, exclusive) of this query. Defaults to the length of this
* reference.
*/
public List setEnd(java.lang.Long end) {
this.end = end;
return this;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of `nextPageToken` from the previous
* response.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** The continuation token, which is used to page through large result sets. To get the next page of
results, set this parameter to the value of `nextPageToken` from the previous response.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* The continuation token, which is used to page through large result sets. To get the next
* page of results, set this parameter to the value of `nextPageToken` from the previous
* response.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* The maximum number of bases to return in a single page. If unspecified, defaults to
* 200Kbp (kilo base pairs). The maximum value is 10Mbp (mega base pairs).
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of bases to return in a single page. If unspecified, defaults to 200Kbp (kilo
base pairs). The maximum value is 10Mbp (mega base pairs).
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of bases to return in a single page. If unspecified, defaults to
* 200Kbp (kilo base pairs). The maximum value is 10Mbp (mega base pairs).
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Referencesets collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Referencesets.List request = genomics.referencesets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Referencesets referencesets() {
return new Referencesets();
}
/**
* The "referencesets" collection of methods.
*/
public class Referencesets {
/**
* Gets a reference set. For the definitions of references and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) Implements [GlobalAllianceApi.getReferenceSet](https://github.com/ga4gh/schemas/blob/v0
* .5.1/src/main/resources/avro/referencemethods.avdl#L83).
*
* Create a request for the method "referencesets.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param referenceSetId The ID of the reference set.
* @return the request
*/
public Get get(java.lang.String referenceSetId) throws java.io.IOException {
Get result = new Get(referenceSetId);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/referencesets/{referenceSetId}";
/**
* Gets a reference set. For the definitions of references and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) Implements [GlobalAllianceApi.getReferenceSet](https://github.com/ga4gh/schemas/blob/
* v0.5.1/src/main/resources/avro/referencemethods.avdl#L83).
*
* Create a request for the method "referencesets.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param referenceSetId The ID of the reference set.
* @since 1.13
*/
protected Get(java.lang.String referenceSetId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.ReferenceSet.class);
this.referenceSetId = com.google.api.client.util.Preconditions.checkNotNull(referenceSetId, "Required parameter referenceSetId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the reference set. */
@com.google.api.client.util.Key
private java.lang.String referenceSetId;
/** The ID of the reference set.
*/
public java.lang.String getReferenceSetId() {
return referenceSetId;
}
/** The ID of the reference set. */
public Get setReferenceSetId(java.lang.String referenceSetId) {
this.referenceSetId = referenceSetId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Searches for reference sets which match the given criteria. For the definitions of references and
* other genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics) Implements [GlobalAllianceApi.searchReferenceSets](https://gith
* ub.com/ga4gh/schemas/blob/v0.5.1/src/main/resources/avro/referencemethods.avdl#L71)
*
* Create a request for the method "referencesets.search".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.SearchReferenceSetsRequest}
* @return the request
*/
public Search search(com.google.api.services.genomics.model.SearchReferenceSetsRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends GenomicsRequest {
private static final String REST_PATH = "v1/referencesets/search";
/**
* Searches for reference sets which match the given criteria. For the definitions of references
* and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Implements [Global
* AllianceApi.searchReferenceSets](https://github.com/ga4gh/schemas/blob/v0.5.1/src/main/resource
* s/avro/referencemethods.avdl#L71)
*
* Create a request for the method "referencesets.search".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.SearchReferenceSetsRequest}
* @since 1.13
*/
protected Search(com.google.api.services.genomics.model.SearchReferenceSetsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.SearchReferenceSetsResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setBearerToken(java.lang.String bearerToken) {
return (Search) super.setBearerToken(bearerToken);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPp(java.lang.Boolean pp) {
return (Search) super.setPp(pp);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Variants collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Variants.List request = genomics.variants().list(parameters ...)}
*
*
* @return the resource collection
*/
public Variants variants() {
return new Variants();
}
/**
* The "variants" collection of methods.
*/
public class Variants {
/**
* Creates a new variant. For the definitions of variants and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "variants.create".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.Variant}
* @return the request
*/
public Create create(com.google.api.services.genomics.model.Variant content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends GenomicsRequest {
private static final String REST_PATH = "v1/variants";
/**
* Creates a new variant. For the definitions of variants and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "variants.create".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.Variant}
* @since 1.13
*/
protected Create(com.google.api.services.genomics.model.Variant content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Variant.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a variant. For the definitions of variants and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "variants.delete".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param variantId The ID of the variant to be deleted.
* @return the request
*/
public Delete delete(java.lang.String variantId) throws java.io.IOException {
Delete result = new Delete(variantId);
initialize(result);
return result;
}
public class Delete extends GenomicsRequest {
private static final String REST_PATH = "v1/variants/{variantId}";
/**
* Deletes a variant. For the definitions of variants and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "variants.delete".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param variantId The ID of the variant to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String variantId) {
super(Genomics.this, "DELETE", REST_PATH, null, com.google.api.services.genomics.model.Empty.class);
this.variantId = com.google.api.client.util.Preconditions.checkNotNull(variantId, "Required parameter variantId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the variant to be deleted. */
@com.google.api.client.util.Key
private java.lang.String variantId;
/** The ID of the variant to be deleted.
*/
public java.lang.String getVariantId() {
return variantId;
}
/** The ID of the variant to be deleted. */
public Delete setVariantId(java.lang.String variantId) {
this.variantId = variantId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a variant by ID. For the definitions of variants and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "variants.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param variantId The ID of the variant.
* @return the request
*/
public Get get(java.lang.String variantId) throws java.io.IOException {
Get result = new Get(variantId);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/variants/{variantId}";
/**
* Gets a variant by ID. For the definitions of variants and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "variants.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param variantId The ID of the variant.
* @since 1.13
*/
protected Get(java.lang.String variantId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.Variant.class);
this.variantId = com.google.api.client.util.Preconditions.checkNotNull(variantId, "Required parameter variantId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the variant. */
@com.google.api.client.util.Key
private java.lang.String variantId;
/** The ID of the variant.
*/
public java.lang.String getVariantId() {
return variantId;
}
/** The ID of the variant. */
public Get setVariantId(java.lang.String variantId) {
this.variantId = variantId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Creates variant data by asynchronously importing the provided information. For the definitions of
* variant sets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) The variants for
* import will be merged with any existing variant that matches its reference sequence, start, end,
* reference bases, and alternative bases. If no such variant exists, a new one will be created.
* When variants are merged, the call information from the new variant is added to the existing
* variant, and Variant info fields are merged as specified in infoMergeConfig. As a special case,
* for single-sample VCF files, QUAL and FILTER fields will be moved to the call level; these are
* sometimes interpreted in a call-specific context. Imported VCF headers are appended to the
* metadata already in a variant set.
*
* Create a request for the method "variants.import".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link GenomicsImport#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.ImportVariantsRequest}
* @return the request
*/
public GenomicsImport genomicsImport(com.google.api.services.genomics.model.ImportVariantsRequest content) throws java.io.IOException {
GenomicsImport result = new GenomicsImport(content);
initialize(result);
return result;
}
public class GenomicsImport extends GenomicsRequest {
private static final String REST_PATH = "v1/variants:import";
/**
* Creates variant data by asynchronously importing the provided information. For the definitions
* of variant sets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) The variants for
* import will be merged with any existing variant that matches its reference sequence, start,
* end, reference bases, and alternative bases. If no such variant exists, a new one will be
* created. When variants are merged, the call information from the new variant is added to the
* existing variant, and Variant info fields are merged as specified in infoMergeConfig. As a
* special case, for single-sample VCF files, QUAL and FILTER fields will be moved to the call
* level; these are sometimes interpreted in a call-specific context. Imported VCF headers are
* appended to the metadata already in a variant set.
*
* Create a request for the method "variants.import".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link GenomicsImport#execute()} method to invoke the remote
* operation. {@link GenomicsImport#initialize(com.google.api.client.googleapis.services.Abstr
* actGoogleClientRequest)} must be called to initialize this instance immediately after invoking
* the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.ImportVariantsRequest}
* @since 1.13
*/
protected GenomicsImport(com.google.api.services.genomics.model.ImportVariantsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Operation.class);
}
@Override
public GenomicsImport set$Xgafv(java.lang.String $Xgafv) {
return (GenomicsImport) super.set$Xgafv($Xgafv);
}
@Override
public GenomicsImport setAccessToken(java.lang.String accessToken) {
return (GenomicsImport) super.setAccessToken(accessToken);
}
@Override
public GenomicsImport setAlt(java.lang.String alt) {
return (GenomicsImport) super.setAlt(alt);
}
@Override
public GenomicsImport setBearerToken(java.lang.String bearerToken) {
return (GenomicsImport) super.setBearerToken(bearerToken);
}
@Override
public GenomicsImport setCallback(java.lang.String callback) {
return (GenomicsImport) super.setCallback(callback);
}
@Override
public GenomicsImport setFields(java.lang.String fields) {
return (GenomicsImport) super.setFields(fields);
}
@Override
public GenomicsImport setKey(java.lang.String key) {
return (GenomicsImport) super.setKey(key);
}
@Override
public GenomicsImport setOauthToken(java.lang.String oauthToken) {
return (GenomicsImport) super.setOauthToken(oauthToken);
}
@Override
public GenomicsImport setPp(java.lang.Boolean pp) {
return (GenomicsImport) super.setPp(pp);
}
@Override
public GenomicsImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GenomicsImport) super.setPrettyPrint(prettyPrint);
}
@Override
public GenomicsImport setQuotaUser(java.lang.String quotaUser) {
return (GenomicsImport) super.setQuotaUser(quotaUser);
}
@Override
public GenomicsImport setUploadType(java.lang.String uploadType) {
return (GenomicsImport) super.setUploadType(uploadType);
}
@Override
public GenomicsImport setUploadProtocol(java.lang.String uploadProtocol) {
return (GenomicsImport) super.setUploadProtocol(uploadProtocol);
}
@Override
public GenomicsImport set(String parameterName, Object value) {
return (GenomicsImport) super.set(parameterName, value);
}
}
/**
* Merges the given variants with existing variants. For the definitions of variants and other
* genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics) Each variant will be merged with an existing variant that
* matches its reference sequence, start, end, reference bases, and alternative bases. If no such
* variant exists, a new one will be created. When variants are merged, the call information from
* the new variant is added to the existing variant. Variant info fields are merged as specified in
* the infoMergeConfig field of the MergeVariantsRequest. Please exercise caution when using this
* method! It is easy to introduce mistakes in existing variants and difficult to back out of them.
* For example, suppose you were trying to merge a new variant with an existing one and both
* variants contain calls that belong to callsets with the same callset ID. // Existing variant -
* irrelevant fields trimmed for clarity { "variantSetId": "10473108253681171589", "referenceName":
* "1", "start": "10582", "referenceBases": "G", "alternateBases": [ "A" ], "calls": [ {
* "callSetId": "10473108253681171589-0", "callSetName": "CALLSET0", "genotype": [ 0, 1 ], } ] } //
* New variant with conflicting call information { "variantSetId": "10473108253681171589",
* "referenceName": "1", "start": "10582", "referenceBases": "G", "alternateBases": [ "A" ],
* "calls": [ { "callSetId": "10473108253681171589-0", "callSetName": "CALLSET0", "genotype": [ 1, 1
* ], } ] } The resulting merged variant would overwrite the existing calls with those from the new
* variant: { "variantSetId": "10473108253681171589", "referenceName": "1", "start": "10582",
* "referenceBases": "G", "alternateBases": [ "A" ], "calls": [ { "callSetId":
* "10473108253681171589-0", "callSetName": "CALLSET0", "genotype": [ 1, 1 ], } ] } This may be the
* desired outcome, but it is up to the user to determine if if that is indeed the case.
*
* Create a request for the method "variants.merge".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Merge#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.MergeVariantsRequest}
* @return the request
*/
public Merge merge(com.google.api.services.genomics.model.MergeVariantsRequest content) throws java.io.IOException {
Merge result = new Merge(content);
initialize(result);
return result;
}
public class Merge extends GenomicsRequest {
private static final String REST_PATH = "v1/variants:merge";
/**
* Merges the given variants with existing variants. For the definitions of variants and other
* genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics) Each variant will be merged with an existing variant that
* matches its reference sequence, start, end, reference bases, and alternative bases. If no such
* variant exists, a new one will be created. When variants are merged, the call information from
* the new variant is added to the existing variant. Variant info fields are merged as specified
* in the infoMergeConfig field of the MergeVariantsRequest. Please exercise caution when using
* this method! It is easy to introduce mistakes in existing variants and difficult to back out of
* them. For example, suppose you were trying to merge a new variant with an existing one and both
* variants contain calls that belong to callsets with the same callset ID. // Existing variant -
* irrelevant fields trimmed for clarity { "variantSetId": "10473108253681171589",
* "referenceName": "1", "start": "10582", "referenceBases": "G", "alternateBases": [ "A" ],
* "calls": [ { "callSetId": "10473108253681171589-0", "callSetName": "CALLSET0", "genotype": [ 0,
* 1 ], } ] } // New variant with conflicting call information { "variantSetId":
* "10473108253681171589", "referenceName": "1", "start": "10582", "referenceBases": "G",
* "alternateBases": [ "A" ], "calls": [ { "callSetId": "10473108253681171589-0", "callSetName":
* "CALLSET0", "genotype": [ 1, 1 ], } ] } The resulting merged variant would overwrite the
* existing calls with those from the new variant: { "variantSetId": "10473108253681171589",
* "referenceName": "1", "start": "10582", "referenceBases": "G", "alternateBases": [ "A" ],
* "calls": [ { "callSetId": "10473108253681171589-0", "callSetName": "CALLSET0", "genotype": [ 1,
* 1 ], } ] } This may be the desired outcome, but it is up to the user to determine if if that is
* indeed the case.
*
* Create a request for the method "variants.merge".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Merge#execute()} method to invoke the remote operation.
* {@link
* Merge#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.MergeVariantsRequest}
* @since 1.13
*/
protected Merge(com.google.api.services.genomics.model.MergeVariantsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Empty.class);
}
@Override
public Merge set$Xgafv(java.lang.String $Xgafv) {
return (Merge) super.set$Xgafv($Xgafv);
}
@Override
public Merge setAccessToken(java.lang.String accessToken) {
return (Merge) super.setAccessToken(accessToken);
}
@Override
public Merge setAlt(java.lang.String alt) {
return (Merge) super.setAlt(alt);
}
@Override
public Merge setBearerToken(java.lang.String bearerToken) {
return (Merge) super.setBearerToken(bearerToken);
}
@Override
public Merge setCallback(java.lang.String callback) {
return (Merge) super.setCallback(callback);
}
@Override
public Merge setFields(java.lang.String fields) {
return (Merge) super.setFields(fields);
}
@Override
public Merge setKey(java.lang.String key) {
return (Merge) super.setKey(key);
}
@Override
public Merge setOauthToken(java.lang.String oauthToken) {
return (Merge) super.setOauthToken(oauthToken);
}
@Override
public Merge setPp(java.lang.Boolean pp) {
return (Merge) super.setPp(pp);
}
@Override
public Merge setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Merge) super.setPrettyPrint(prettyPrint);
}
@Override
public Merge setQuotaUser(java.lang.String quotaUser) {
return (Merge) super.setQuotaUser(quotaUser);
}
@Override
public Merge setUploadType(java.lang.String uploadType) {
return (Merge) super.setUploadType(uploadType);
}
@Override
public Merge setUploadProtocol(java.lang.String uploadProtocol) {
return (Merge) super.setUploadProtocol(uploadProtocol);
}
@Override
public Merge set(String parameterName, Object value) {
return (Merge) super.set(parameterName, value);
}
}
/**
* Updates a variant. For the definitions of variants and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) This method supports patch semantics. Returns the modified variant without its calls.
*
* Create a request for the method "variants.patch".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param variantId The ID of the variant to be updated.
* @param content the {@link com.google.api.services.genomics.model.Variant}
* @return the request
*/
public Patch patch(java.lang.String variantId, com.google.api.services.genomics.model.Variant content) throws java.io.IOException {
Patch result = new Patch(variantId, content);
initialize(result);
return result;
}
public class Patch extends GenomicsRequest {
private static final String REST_PATH = "v1/variants/{variantId}";
/**
* Updates a variant. For the definitions of variants and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) This method supports patch semantics. Returns the modified variant without its calls.
*
* Create a request for the method "variants.patch".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param variantId The ID of the variant to be updated.
* @param content the {@link com.google.api.services.genomics.model.Variant}
* @since 1.13
*/
protected Patch(java.lang.String variantId, com.google.api.services.genomics.model.Variant content) {
super(Genomics.this, "PATCH", REST_PATH, content, com.google.api.services.genomics.model.Variant.class);
this.variantId = com.google.api.client.util.Preconditions.checkNotNull(variantId, "Required parameter variantId must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setBearerToken(java.lang.String bearerToken) {
return (Patch) super.setBearerToken(bearerToken);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPp(java.lang.Boolean pp) {
return (Patch) super.setPp(pp);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the variant to be updated. */
@com.google.api.client.util.Key
private java.lang.String variantId;
/** The ID of the variant to be updated.
*/
public java.lang.String getVariantId() {
return variantId;
}
/** The ID of the variant to be updated. */
public Patch setVariantId(java.lang.String variantId) {
this.variantId = variantId;
return this;
}
/**
* An optional mask specifying which fields to update. At this time, mutable fields are names
* and info. Acceptable values are "names" and "info". If unspecified, all mutable fields will
* be updated.
*/
@com.google.api.client.util.Key
private java.lang.String updateMask;
/** An optional mask specifying which fields to update. At this time, mutable fields are names and
info. Acceptable values are "names" and "info". If unspecified, all mutable fields will be updated.
*/
public java.lang.String getUpdateMask() {
return updateMask;
}
/**
* An optional mask specifying which fields to update. At this time, mutable fields are names
* and info. Acceptable values are "names" and "info". If unspecified, all mutable fields will
* be updated.
*/
public Patch setUpdateMask(java.lang.String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Gets a list of variants matching the criteria. For the definitions of variants and other genomics
* resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-
* of-google-genomics) Implements [GlobalAllianceApi.searchVariants](https://github.com/ga4gh/schema
* s/blob/v0.5.1/src/main/resources/avro/variantmethods.avdl#L126).
*
* Create a request for the method "variants.search".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.SearchVariantsRequest}
* @return the request
*/
public Search search(com.google.api.services.genomics.model.SearchVariantsRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends GenomicsRequest {
private static final String REST_PATH = "v1/variants/search";
/**
* Gets a list of variants matching the criteria. For the definitions of variants and other
* genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics) Implements [GlobalAllianceApi.searchVariants](https://github.
* com/ga4gh/schemas/blob/v0.5.1/src/main/resources/avro/variantmethods.avdl#L126).
*
* Create a request for the method "variants.search".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.SearchVariantsRequest}
* @since 1.13
*/
protected Search(com.google.api.services.genomics.model.SearchVariantsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.SearchVariantsResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setBearerToken(java.lang.String bearerToken) {
return (Search) super.setBearerToken(bearerToken);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPp(java.lang.Boolean pp) {
return (Search) super.setPp(pp);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
/**
* Returns a stream of all the variants matching the search request, ordered by reference name,
* position, and ID.
*
* Create a request for the method "variants.stream".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Stream#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.StreamVariantsRequest}
* @return the request
*/
public Stream stream(com.google.api.services.genomics.model.StreamVariantsRequest content) throws java.io.IOException {
Stream result = new Stream(content);
initialize(result);
return result;
}
public class Stream extends GenomicsRequest {
private static final String REST_PATH = "v1/variants:stream";
/**
* Returns a stream of all the variants matching the search request, ordered by reference name,
* position, and ID.
*
* Create a request for the method "variants.stream".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Stream#execute()} method to invoke the remote operation.
* {@link
* Stream#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.StreamVariantsRequest}
* @since 1.13
*/
protected Stream(com.google.api.services.genomics.model.StreamVariantsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.StreamVariantsResponse.class);
}
@Override
public Stream set$Xgafv(java.lang.String $Xgafv) {
return (Stream) super.set$Xgafv($Xgafv);
}
@Override
public Stream setAccessToken(java.lang.String accessToken) {
return (Stream) super.setAccessToken(accessToken);
}
@Override
public Stream setAlt(java.lang.String alt) {
return (Stream) super.setAlt(alt);
}
@Override
public Stream setBearerToken(java.lang.String bearerToken) {
return (Stream) super.setBearerToken(bearerToken);
}
@Override
public Stream setCallback(java.lang.String callback) {
return (Stream) super.setCallback(callback);
}
@Override
public Stream setFields(java.lang.String fields) {
return (Stream) super.setFields(fields);
}
@Override
public Stream setKey(java.lang.String key) {
return (Stream) super.setKey(key);
}
@Override
public Stream setOauthToken(java.lang.String oauthToken) {
return (Stream) super.setOauthToken(oauthToken);
}
@Override
public Stream setPp(java.lang.Boolean pp) {
return (Stream) super.setPp(pp);
}
@Override
public Stream setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Stream) super.setPrettyPrint(prettyPrint);
}
@Override
public Stream setQuotaUser(java.lang.String quotaUser) {
return (Stream) super.setQuotaUser(quotaUser);
}
@Override
public Stream setUploadType(java.lang.String uploadType) {
return (Stream) super.setUploadType(uploadType);
}
@Override
public Stream setUploadProtocol(java.lang.String uploadProtocol) {
return (Stream) super.setUploadProtocol(uploadProtocol);
}
@Override
public Stream set(String parameterName, Object value) {
return (Stream) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Variantsets collection.
*
* The typical use is:
*
* {@code Genomics genomics = new Genomics(...);}
* {@code Genomics.Variantsets.List request = genomics.variantsets().list(parameters ...)}
*
*
* @return the resource collection
*/
public Variantsets variantsets() {
return new Variantsets();
}
/**
* The "variantsets" collection of methods.
*/
public class Variantsets {
/**
* Creates a new variant set. For the definitions of variant sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) The provided variant set must have a valid `datasetId` set - all other fields are
* optional. Note that the `id` field will be ignored, as this is assigned by the server.
*
* Create a request for the method "variantsets.create".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.VariantSet}
* @return the request
*/
public Create create(com.google.api.services.genomics.model.VariantSet content) throws java.io.IOException {
Create result = new Create(content);
initialize(result);
return result;
}
public class Create extends GenomicsRequest {
private static final String REST_PATH = "v1/variantsets";
/**
* Creates a new variant set. For the definitions of variant sets and other genomics resources,
* see [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics) The provided variant set must have a valid `datasetId` set - all other fields are
* optional. Note that the `id` field will be ignored, as this is assigned by the server.
*
* Create a request for the method "variantsets.create".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Create#execute()} method to invoke the remote operation.
* {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.VariantSet}
* @since 1.13
*/
protected Create(com.google.api.services.genomics.model.VariantSet content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.VariantSet.class);
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setBearerToken(java.lang.String bearerToken) {
return (Create) super.setBearerToken(bearerToken);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPp(java.lang.Boolean pp) {
return (Create) super.setPp(pp);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a variant set including all variants, call sets, and calls within. This is not
* reversible. For the definitions of variant sets and other genomics resources, see [Fundamentals
* of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics)
*
* Create a request for the method "variantsets.delete".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param variantSetId The ID of the variant set to be deleted.
* @return the request
*/
public Delete delete(java.lang.String variantSetId) throws java.io.IOException {
Delete result = new Delete(variantSetId);
initialize(result);
return result;
}
public class Delete extends GenomicsRequest {
private static final String REST_PATH = "v1/variantsets/{variantSetId}";
/**
* Deletes a variant set including all variants, call sets, and calls within. This is not
* reversible. For the definitions of variant sets and other genomics resources, see [Fundamentals
* of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics)
*
* Create a request for the method "variantsets.delete".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Delete#execute()} method to invoke the remote operation.
* {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param variantSetId The ID of the variant set to be deleted.
* @since 1.13
*/
protected Delete(java.lang.String variantSetId) {
super(Genomics.this, "DELETE", REST_PATH, null, com.google.api.services.genomics.model.Empty.class);
this.variantSetId = com.google.api.client.util.Preconditions.checkNotNull(variantSetId, "Required parameter variantSetId must be specified.");
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setBearerToken(java.lang.String bearerToken) {
return (Delete) super.setBearerToken(bearerToken);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPp(java.lang.Boolean pp) {
return (Delete) super.setPp(pp);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the variant set to be deleted. */
@com.google.api.client.util.Key
private java.lang.String variantSetId;
/** The ID of the variant set to be deleted.
*/
public java.lang.String getVariantSetId() {
return variantSetId;
}
/** The ID of the variant set to be deleted. */
public Delete setVariantSetId(java.lang.String variantSetId) {
this.variantSetId = variantSetId;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Exports variant set data to an external destination. For the definitions of variant sets and
* other genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics)
*
* Create a request for the method "variantsets.export".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Export#execute()} method to invoke the remote operation.
*
* @param variantSetId Required. The ID of the variant set that contains variant data which should be exported. The caller
* must have READ access to this variant set.
* @param content the {@link com.google.api.services.genomics.model.ExportVariantSetRequest}
* @return the request
*/
public Export export(java.lang.String variantSetId, com.google.api.services.genomics.model.ExportVariantSetRequest content) throws java.io.IOException {
Export result = new Export(variantSetId, content);
initialize(result);
return result;
}
public class Export extends GenomicsRequest {
private static final String REST_PATH = "v1/variantsets/{variantSetId}:export";
/**
* Exports variant set data to an external destination. For the definitions of variant sets and
* other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics)
*
* Create a request for the method "variantsets.export".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Export#execute()} method to invoke the remote operation.
* {@link
* Export#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param variantSetId Required. The ID of the variant set that contains variant data which should be exported. The caller
* must have READ access to this variant set.
* @param content the {@link com.google.api.services.genomics.model.ExportVariantSetRequest}
* @since 1.13
*/
protected Export(java.lang.String variantSetId, com.google.api.services.genomics.model.ExportVariantSetRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.Operation.class);
this.variantSetId = com.google.api.client.util.Preconditions.checkNotNull(variantSetId, "Required parameter variantSetId must be specified.");
}
@Override
public Export set$Xgafv(java.lang.String $Xgafv) {
return (Export) super.set$Xgafv($Xgafv);
}
@Override
public Export setAccessToken(java.lang.String accessToken) {
return (Export) super.setAccessToken(accessToken);
}
@Override
public Export setAlt(java.lang.String alt) {
return (Export) super.setAlt(alt);
}
@Override
public Export setBearerToken(java.lang.String bearerToken) {
return (Export) super.setBearerToken(bearerToken);
}
@Override
public Export setCallback(java.lang.String callback) {
return (Export) super.setCallback(callback);
}
@Override
public Export setFields(java.lang.String fields) {
return (Export) super.setFields(fields);
}
@Override
public Export setKey(java.lang.String key) {
return (Export) super.setKey(key);
}
@Override
public Export setOauthToken(java.lang.String oauthToken) {
return (Export) super.setOauthToken(oauthToken);
}
@Override
public Export setPp(java.lang.Boolean pp) {
return (Export) super.setPp(pp);
}
@Override
public Export setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Export) super.setPrettyPrint(prettyPrint);
}
@Override
public Export setQuotaUser(java.lang.String quotaUser) {
return (Export) super.setQuotaUser(quotaUser);
}
@Override
public Export setUploadType(java.lang.String uploadType) {
return (Export) super.setUploadType(uploadType);
}
@Override
public Export setUploadProtocol(java.lang.String uploadProtocol) {
return (Export) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The ID of the variant set that contains variant data which should be exported.
* The caller must have READ access to this variant set.
*/
@com.google.api.client.util.Key
private java.lang.String variantSetId;
/** Required. The ID of the variant set that contains variant data which should be exported. The caller
must have READ access to this variant set.
*/
public java.lang.String getVariantSetId() {
return variantSetId;
}
/**
* Required. The ID of the variant set that contains variant data which should be exported.
* The caller must have READ access to this variant set.
*/
public Export setVariantSetId(java.lang.String variantSetId) {
this.variantSetId = variantSetId;
return this;
}
@Override
public Export set(String parameterName, Object value) {
return (Export) super.set(parameterName, value);
}
}
/**
* Gets a variant set by ID. For the definitions of variant sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "variantsets.get".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param variantSetId Required. The ID of the variant set.
* @return the request
*/
public Get get(java.lang.String variantSetId) throws java.io.IOException {
Get result = new Get(variantSetId);
initialize(result);
return result;
}
public class Get extends GenomicsRequest {
private static final String REST_PATH = "v1/variantsets/{variantSetId}";
/**
* Gets a variant set by ID. For the definitions of variant sets and other genomics resources, see
* [Fundamentals of Google Genomics](https://cloud.google.com/genomics/fundamentals-of-google-
* genomics)
*
* Create a request for the method "variantsets.get".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Get#execute()} method to invoke the remote operation.
* {@link Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param variantSetId Required. The ID of the variant set.
* @since 1.13
*/
protected Get(java.lang.String variantSetId) {
super(Genomics.this, "GET", REST_PATH, null, com.google.api.services.genomics.model.VariantSet.class);
this.variantSetId = com.google.api.client.util.Preconditions.checkNotNull(variantSetId, "Required parameter variantSetId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setBearerToken(java.lang.String bearerToken) {
return (Get) super.setBearerToken(bearerToken);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPp(java.lang.Boolean pp) {
return (Get) super.setPp(pp);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** Required. The ID of the variant set. */
@com.google.api.client.util.Key
private java.lang.String variantSetId;
/** Required. The ID of the variant set.
*/
public java.lang.String getVariantSetId() {
return variantSetId;
}
/** Required. The ID of the variant set. */
public Get setVariantSetId(java.lang.String variantSetId) {
this.variantSetId = variantSetId;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Updates a variant set using patch semantics. For the definitions of variant sets and other
* genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics)
*
* Create a request for the method "variantsets.patch".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param variantSetId The ID of the variant to be updated (must already exist).
* @param content the {@link com.google.api.services.genomics.model.VariantSet}
* @return the request
*/
public Patch patch(java.lang.String variantSetId, com.google.api.services.genomics.model.VariantSet content) throws java.io.IOException {
Patch result = new Patch(variantSetId, content);
initialize(result);
return result;
}
public class Patch extends GenomicsRequest {
private static final String REST_PATH = "v1/variantsets/{variantSetId}";
/**
* Updates a variant set using patch semantics. For the definitions of variant sets and other
* genomics resources, see [Fundamentals of Google Genomics](https://cloud.google.com/genomics
* /fundamentals-of-google-genomics)
*
* Create a request for the method "variantsets.patch".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Patch#execute()} method to invoke the remote operation.
* {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param variantSetId The ID of the variant to be updated (must already exist).
* @param content the {@link com.google.api.services.genomics.model.VariantSet}
* @since 1.13
*/
protected Patch(java.lang.String variantSetId, com.google.api.services.genomics.model.VariantSet content) {
super(Genomics.this, "PATCH", REST_PATH, content, com.google.api.services.genomics.model.VariantSet.class);
this.variantSetId = com.google.api.client.util.Preconditions.checkNotNull(variantSetId, "Required parameter variantSetId must be specified.");
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setBearerToken(java.lang.String bearerToken) {
return (Patch) super.setBearerToken(bearerToken);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPp(java.lang.Boolean pp) {
return (Patch) super.setPp(pp);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/** The ID of the variant to be updated (must already exist). */
@com.google.api.client.util.Key
private java.lang.String variantSetId;
/** The ID of the variant to be updated (must already exist).
*/
public java.lang.String getVariantSetId() {
return variantSetId;
}
/** The ID of the variant to be updated (must already exist). */
public Patch setVariantSetId(java.lang.String variantSetId) {
this.variantSetId = variantSetId;
return this;
}
/**
* An optional mask specifying which fields to update. Supported fields: * metadata. * name. *
* description. Leaving `updateMask` unset is equivalent to specifying all mutable fields.
*/
@com.google.api.client.util.Key
private java.lang.String updateMask;
/** An optional mask specifying which fields to update. Supported fields: * metadata. * name. *
description. Leaving `updateMask` unset is equivalent to specifying all mutable fields.
*/
public java.lang.String getUpdateMask() {
return updateMask;
}
/**
* An optional mask specifying which fields to update. Supported fields: * metadata. * name. *
* description. Leaving `updateMask` unset is equivalent to specifying all mutable fields.
*/
public Patch setUpdateMask(java.lang.String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Returns a list of all variant sets matching search criteria. For the definitions of variant sets
* and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Implements [GlobalAl
* lianceApi.searchVariantSets](https://github.com/ga4gh/schemas/blob/v0.5.1/src/main/resources/avro
* /variantmethods.avdl#L49).
*
* Create a request for the method "variantsets.search".
*
* This request holds the parameters needed by the genomics server. After setting any optional
* parameters, call the {@link Search#execute()} method to invoke the remote operation.
*
* @param content the {@link com.google.api.services.genomics.model.SearchVariantSetsRequest}
* @return the request
*/
public Search search(com.google.api.services.genomics.model.SearchVariantSetsRequest content) throws java.io.IOException {
Search result = new Search(content);
initialize(result);
return result;
}
public class Search extends GenomicsRequest {
private static final String REST_PATH = "v1/variantsets/search";
/**
* Returns a list of all variant sets matching search criteria. For the definitions of variant
* sets and other genomics resources, see [Fundamentals of Google
* Genomics](https://cloud.google.com/genomics/fundamentals-of-google-genomics) Implements [Global
* AllianceApi.searchVariantSets](https://github.com/ga4gh/schemas/blob/v0.5.1/src/main/resources/
* avro/variantmethods.avdl#L49).
*
* Create a request for the method "variantsets.search".
*
* This request holds the parameters needed by the the genomics server. After setting any
* optional parameters, call the {@link Search#execute()} method to invoke the remote operation.
* {@link
* Search#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param content the {@link com.google.api.services.genomics.model.SearchVariantSetsRequest}
* @since 1.13
*/
protected Search(com.google.api.services.genomics.model.SearchVariantSetsRequest content) {
super(Genomics.this, "POST", REST_PATH, content, com.google.api.services.genomics.model.SearchVariantSetsResponse.class);
}
@Override
public Search set$Xgafv(java.lang.String $Xgafv) {
return (Search) super.set$Xgafv($Xgafv);
}
@Override
public Search setAccessToken(java.lang.String accessToken) {
return (Search) super.setAccessToken(accessToken);
}
@Override
public Search setAlt(java.lang.String alt) {
return (Search) super.setAlt(alt);
}
@Override
public Search setBearerToken(java.lang.String bearerToken) {
return (Search) super.setBearerToken(bearerToken);
}
@Override
public Search setCallback(java.lang.String callback) {
return (Search) super.setCallback(callback);
}
@Override
public Search setFields(java.lang.String fields) {
return (Search) super.setFields(fields);
}
@Override
public Search setKey(java.lang.String key) {
return (Search) super.setKey(key);
}
@Override
public Search setOauthToken(java.lang.String oauthToken) {
return (Search) super.setOauthToken(oauthToken);
}
@Override
public Search setPp(java.lang.Boolean pp) {
return (Search) super.setPp(pp);
}
@Override
public Search setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Search) super.setPrettyPrint(prettyPrint);
}
@Override
public Search setQuotaUser(java.lang.String quotaUser) {
return (Search) super.setQuotaUser(quotaUser);
}
@Override
public Search setUploadType(java.lang.String uploadType) {
return (Search) super.setUploadType(uploadType);
}
@Override
public Search setUploadProtocol(java.lang.String uploadProtocol) {
return (Search) super.setUploadProtocol(uploadProtocol);
}
@Override
public Search set(String parameterName, Object value) {
return (Search) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link Genomics}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link Genomics}. */
@Override
public Genomics build() {
return new Genomics(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link GenomicsRequestInitializer}.
*
* @since 1.12
*/
public Builder setGenomicsRequestInitializer(
GenomicsRequestInitializer genomicsRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(genomicsRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}