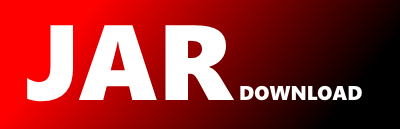
com.google.api.services.gkebackup.v1.model.ClusterResourceRestoreScope Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.gkebackup.v1.model;
/**
* Defines the scope of cluster-scoped resources to restore. Some group kinds are not reasonable
* choices for a restore, and will cause an error if selected here. Any scope selection that would
* restore "all valid" resources automatically excludes these group kinds. -
* gkebackup.gke.io/BackupJob - gkebackup.gke.io/RestoreJob - metrics.k8s.io/NodeMetrics -
* migration.k8s.io/StorageState - migration.k8s.io/StorageVersionMigration - Node -
* snapshot.storage.k8s.io/VolumeSnapshotContent - storage.k8s.io/CSINode Some group kinds are
* driven by restore configuration elsewhere, and will cause an error if selected here. - Namespace
* - PersistentVolume
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup for GKE API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class ClusterResourceRestoreScope extends com.google.api.client.json.GenericJson {
/**
* Optional. If True, all valid cluster-scoped resources will be restored. Mutually exclusive to
* any other field in the message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allGroupKinds;
/**
* Optional. A list of cluster-scoped resource group kinds to NOT restore from the backup. If
* specified, all valid cluster-scoped resources will be restored except for those specified in
* the list. Mutually exclusive to any other field in the message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List excludedGroupKinds;
/**
* Optional. If True, no cluster-scoped resources will be restored. This has the same restore
* scope as if the message is not defined. Mutually exclusive to any other field in the message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean noGroupKinds;
/**
* Optional. A list of cluster-scoped resource group kinds to restore from the backup. If
* specified, only the selected resources will be restored. Mutually exclusive to any other field
* in the message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List selectedGroupKinds;
/**
* Optional. If True, all valid cluster-scoped resources will be restored. Mutually exclusive to
* any other field in the message.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAllGroupKinds() {
return allGroupKinds;
}
/**
* Optional. If True, all valid cluster-scoped resources will be restored. Mutually exclusive to
* any other field in the message.
* @param allGroupKinds allGroupKinds or {@code null} for none
*/
public ClusterResourceRestoreScope setAllGroupKinds(java.lang.Boolean allGroupKinds) {
this.allGroupKinds = allGroupKinds;
return this;
}
/**
* Optional. A list of cluster-scoped resource group kinds to NOT restore from the backup. If
* specified, all valid cluster-scoped resources will be restored except for those specified in
* the list. Mutually exclusive to any other field in the message.
* @return value or {@code null} for none
*/
public java.util.List getExcludedGroupKinds() {
return excludedGroupKinds;
}
/**
* Optional. A list of cluster-scoped resource group kinds to NOT restore from the backup. If
* specified, all valid cluster-scoped resources will be restored except for those specified in
* the list. Mutually exclusive to any other field in the message.
* @param excludedGroupKinds excludedGroupKinds or {@code null} for none
*/
public ClusterResourceRestoreScope setExcludedGroupKinds(java.util.List excludedGroupKinds) {
this.excludedGroupKinds = excludedGroupKinds;
return this;
}
/**
* Optional. If True, no cluster-scoped resources will be restored. This has the same restore
* scope as if the message is not defined. Mutually exclusive to any other field in the message.
* @return value or {@code null} for none
*/
public java.lang.Boolean getNoGroupKinds() {
return noGroupKinds;
}
/**
* Optional. If True, no cluster-scoped resources will be restored. This has the same restore
* scope as if the message is not defined. Mutually exclusive to any other field in the message.
* @param noGroupKinds noGroupKinds or {@code null} for none
*/
public ClusterResourceRestoreScope setNoGroupKinds(java.lang.Boolean noGroupKinds) {
this.noGroupKinds = noGroupKinds;
return this;
}
/**
* Optional. A list of cluster-scoped resource group kinds to restore from the backup. If
* specified, only the selected resources will be restored. Mutually exclusive to any other field
* in the message.
* @return value or {@code null} for none
*/
public java.util.List getSelectedGroupKinds() {
return selectedGroupKinds;
}
/**
* Optional. A list of cluster-scoped resource group kinds to restore from the backup. If
* specified, only the selected resources will be restored. Mutually exclusive to any other field
* in the message.
* @param selectedGroupKinds selectedGroupKinds or {@code null} for none
*/
public ClusterResourceRestoreScope setSelectedGroupKinds(java.util.List selectedGroupKinds) {
this.selectedGroupKinds = selectedGroupKinds;
return this;
}
@Override
public ClusterResourceRestoreScope set(String fieldName, Object value) {
return (ClusterResourceRestoreScope) super.set(fieldName, value);
}
@Override
public ClusterResourceRestoreScope clone() {
return (ClusterResourceRestoreScope) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy