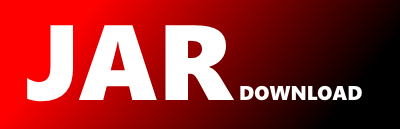
com.google.api.services.gkebackup.v1.model.RestoreConfig Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.gkebackup.v1.model;
/**
* Configuration of a restore. Next id: 12
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup for GKE API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class RestoreConfig extends com.google.api.client.json.GenericJson {
/**
* Restore all namespaced resources in the Backup if set to "True". Specifying this field to
* "False" is an error.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean allNamespaces;
/**
* Optional. Defines the behavior for handling the situation where cluster-scoped resources being
* restored already exist in the target cluster. This MUST be set to a value other than
* CLUSTER_RESOURCE_CONFLICT_POLICY_UNSPECIFIED if cluster_resource_restore_scope is not empty.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String clusterResourceConflictPolicy;
/**
* Optional. Identifies the cluster-scoped resources to restore from the Backup. Not specifying it
* means NO cluster resource will be restored.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private ClusterResourceRestoreScope clusterResourceRestoreScope;
/**
* A list of selected namespaces excluded from restoration. All namespaces except those in this
* list will be restored.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Namespaces excludedNamespaces;
/**
* Optional. Defines the behavior for handling the situation where sets of namespaced resources
* being restored already exist in the target cluster. This MUST be set to a value other than
* NAMESPACED_RESOURCE_RESTORE_MODE_UNSPECIFIED.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String namespacedResourceRestoreMode;
/**
* Do not restore any namespaced resources if set to "True". Specifying this field to "False" is
* not allowed.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean noNamespaces;
/**
* A list of selected ProtectedApplications to restore. The listed ProtectedApplications and all
* the resources to which they refer will be restored.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NamespacedNames selectedApplications;
/**
* A list of selected Namespaces to restore from the Backup. The listed Namespaces and all
* resources contained in them will be restored.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private Namespaces selectedNamespaces;
/**
* Optional. A list of transformation rules to be applied against Kubernetes resources as they are
* selected for restoration from a Backup. Rules are executed in order defined - this order
* matters, as changes made by a rule may impact the filtering logic of subsequent rules. An empty
* list means no substitution will occur.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List substitutionRules;
/**
* Optional. A list of transformation rules to be applied against Kubernetes resources as they are
* selected for restoration from a Backup. Rules are executed in order defined - this order
* matters, as changes made by a rule may impact the filtering logic of subsequent rules. An empty
* list means no transformation will occur.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List transformationRules;
/**
* Optional. Specifies the mechanism to be used to restore volume data. Default:
* VOLUME_DATA_RESTORE_POLICY_UNSPECIFIED (will be treated as NO_VOLUME_DATA_RESTORATION).
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String volumeDataRestorePolicy;
/**
* Restore all namespaced resources in the Backup if set to "True". Specifying this field to
* "False" is an error.
* @return value or {@code null} for none
*/
public java.lang.Boolean getAllNamespaces() {
return allNamespaces;
}
/**
* Restore all namespaced resources in the Backup if set to "True". Specifying this field to
* "False" is an error.
* @param allNamespaces allNamespaces or {@code null} for none
*/
public RestoreConfig setAllNamespaces(java.lang.Boolean allNamespaces) {
this.allNamespaces = allNamespaces;
return this;
}
/**
* Optional. Defines the behavior for handling the situation where cluster-scoped resources being
* restored already exist in the target cluster. This MUST be set to a value other than
* CLUSTER_RESOURCE_CONFLICT_POLICY_UNSPECIFIED if cluster_resource_restore_scope is not empty.
* @return value or {@code null} for none
*/
public java.lang.String getClusterResourceConflictPolicy() {
return clusterResourceConflictPolicy;
}
/**
* Optional. Defines the behavior for handling the situation where cluster-scoped resources being
* restored already exist in the target cluster. This MUST be set to a value other than
* CLUSTER_RESOURCE_CONFLICT_POLICY_UNSPECIFIED if cluster_resource_restore_scope is not empty.
* @param clusterResourceConflictPolicy clusterResourceConflictPolicy or {@code null} for none
*/
public RestoreConfig setClusterResourceConflictPolicy(java.lang.String clusterResourceConflictPolicy) {
this.clusterResourceConflictPolicy = clusterResourceConflictPolicy;
return this;
}
/**
* Optional. Identifies the cluster-scoped resources to restore from the Backup. Not specifying it
* means NO cluster resource will be restored.
* @return value or {@code null} for none
*/
public ClusterResourceRestoreScope getClusterResourceRestoreScope() {
return clusterResourceRestoreScope;
}
/**
* Optional. Identifies the cluster-scoped resources to restore from the Backup. Not specifying it
* means NO cluster resource will be restored.
* @param clusterResourceRestoreScope clusterResourceRestoreScope or {@code null} for none
*/
public RestoreConfig setClusterResourceRestoreScope(ClusterResourceRestoreScope clusterResourceRestoreScope) {
this.clusterResourceRestoreScope = clusterResourceRestoreScope;
return this;
}
/**
* A list of selected namespaces excluded from restoration. All namespaces except those in this
* list will be restored.
* @return value or {@code null} for none
*/
public Namespaces getExcludedNamespaces() {
return excludedNamespaces;
}
/**
* A list of selected namespaces excluded from restoration. All namespaces except those in this
* list will be restored.
* @param excludedNamespaces excludedNamespaces or {@code null} for none
*/
public RestoreConfig setExcludedNamespaces(Namespaces excludedNamespaces) {
this.excludedNamespaces = excludedNamespaces;
return this;
}
/**
* Optional. Defines the behavior for handling the situation where sets of namespaced resources
* being restored already exist in the target cluster. This MUST be set to a value other than
* NAMESPACED_RESOURCE_RESTORE_MODE_UNSPECIFIED.
* @return value or {@code null} for none
*/
public java.lang.String getNamespacedResourceRestoreMode() {
return namespacedResourceRestoreMode;
}
/**
* Optional. Defines the behavior for handling the situation where sets of namespaced resources
* being restored already exist in the target cluster. This MUST be set to a value other than
* NAMESPACED_RESOURCE_RESTORE_MODE_UNSPECIFIED.
* @param namespacedResourceRestoreMode namespacedResourceRestoreMode or {@code null} for none
*/
public RestoreConfig setNamespacedResourceRestoreMode(java.lang.String namespacedResourceRestoreMode) {
this.namespacedResourceRestoreMode = namespacedResourceRestoreMode;
return this;
}
/**
* Do not restore any namespaced resources if set to "True". Specifying this field to "False" is
* not allowed.
* @return value or {@code null} for none
*/
public java.lang.Boolean getNoNamespaces() {
return noNamespaces;
}
/**
* Do not restore any namespaced resources if set to "True". Specifying this field to "False" is
* not allowed.
* @param noNamespaces noNamespaces or {@code null} for none
*/
public RestoreConfig setNoNamespaces(java.lang.Boolean noNamespaces) {
this.noNamespaces = noNamespaces;
return this;
}
/**
* A list of selected ProtectedApplications to restore. The listed ProtectedApplications and all
* the resources to which they refer will be restored.
* @return value or {@code null} for none
*/
public NamespacedNames getSelectedApplications() {
return selectedApplications;
}
/**
* A list of selected ProtectedApplications to restore. The listed ProtectedApplications and all
* the resources to which they refer will be restored.
* @param selectedApplications selectedApplications or {@code null} for none
*/
public RestoreConfig setSelectedApplications(NamespacedNames selectedApplications) {
this.selectedApplications = selectedApplications;
return this;
}
/**
* A list of selected Namespaces to restore from the Backup. The listed Namespaces and all
* resources contained in them will be restored.
* @return value or {@code null} for none
*/
public Namespaces getSelectedNamespaces() {
return selectedNamespaces;
}
/**
* A list of selected Namespaces to restore from the Backup. The listed Namespaces and all
* resources contained in them will be restored.
* @param selectedNamespaces selectedNamespaces or {@code null} for none
*/
public RestoreConfig setSelectedNamespaces(Namespaces selectedNamespaces) {
this.selectedNamespaces = selectedNamespaces;
return this;
}
/**
* Optional. A list of transformation rules to be applied against Kubernetes resources as they are
* selected for restoration from a Backup. Rules are executed in order defined - this order
* matters, as changes made by a rule may impact the filtering logic of subsequent rules. An empty
* list means no substitution will occur.
* @return value or {@code null} for none
*/
public java.util.List getSubstitutionRules() {
return substitutionRules;
}
/**
* Optional. A list of transformation rules to be applied against Kubernetes resources as they are
* selected for restoration from a Backup. Rules are executed in order defined - this order
* matters, as changes made by a rule may impact the filtering logic of subsequent rules. An empty
* list means no substitution will occur.
* @param substitutionRules substitutionRules or {@code null} for none
*/
public RestoreConfig setSubstitutionRules(java.util.List substitutionRules) {
this.substitutionRules = substitutionRules;
return this;
}
/**
* Optional. A list of transformation rules to be applied against Kubernetes resources as they are
* selected for restoration from a Backup. Rules are executed in order defined - this order
* matters, as changes made by a rule may impact the filtering logic of subsequent rules. An empty
* list means no transformation will occur.
* @return value or {@code null} for none
*/
public java.util.List getTransformationRules() {
return transformationRules;
}
/**
* Optional. A list of transformation rules to be applied against Kubernetes resources as they are
* selected for restoration from a Backup. Rules are executed in order defined - this order
* matters, as changes made by a rule may impact the filtering logic of subsequent rules. An empty
* list means no transformation will occur.
* @param transformationRules transformationRules or {@code null} for none
*/
public RestoreConfig setTransformationRules(java.util.List transformationRules) {
this.transformationRules = transformationRules;
return this;
}
/**
* Optional. Specifies the mechanism to be used to restore volume data. Default:
* VOLUME_DATA_RESTORE_POLICY_UNSPECIFIED (will be treated as NO_VOLUME_DATA_RESTORATION).
* @return value or {@code null} for none
*/
public java.lang.String getVolumeDataRestorePolicy() {
return volumeDataRestorePolicy;
}
/**
* Optional. Specifies the mechanism to be used to restore volume data. Default:
* VOLUME_DATA_RESTORE_POLICY_UNSPECIFIED (will be treated as NO_VOLUME_DATA_RESTORATION).
* @param volumeDataRestorePolicy volumeDataRestorePolicy or {@code null} for none
*/
public RestoreConfig setVolumeDataRestorePolicy(java.lang.String volumeDataRestorePolicy) {
this.volumeDataRestorePolicy = volumeDataRestorePolicy;
return this;
}
@Override
public RestoreConfig set(String fieldName, Object value) {
return (RestoreConfig) super.set(fieldName, value);
}
@Override
public RestoreConfig clone() {
return (RestoreConfig) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy