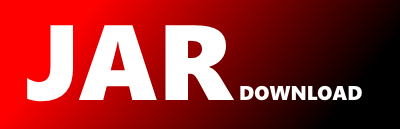
com.google.api.services.gkebackup.v1.model.RetentionPolicy Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.gkebackup.v1.model;
/**
* RetentionPolicy defines a Backup retention policy for a BackupPlan.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Backup for GKE API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class RetentionPolicy extends com.google.api.client.json.GenericJson {
/**
* Optional. Minimum age for Backups created via this BackupPlan (in days). This field MUST be an
* integer value between 0-90 (inclusive). A Backup created under this BackupPlan will NOT be
* deletable until it reaches Backup's (create_time + backup_delete_lock_days). Updating this
* field of a BackupPlan does NOT affect existing Backups under it. Backups created AFTER a
* successful update will inherit the new value. Default: 0 (no delete blocking)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer backupDeleteLockDays;
/**
* Optional. The default maximum age of a Backup created via this BackupPlan. This field MUST be
* an integer value >= 0 and <= 365. If specified, a Backup created under this BackupPlan will be
* automatically deleted after its age reaches (create_time + backup_retain_days). If not
* specified, Backups created under this BackupPlan will NOT be subject to automatic deletion.
* Updating this field does NOT affect existing Backups under it. Backups created AFTER a
* successful update will automatically pick up the new value. NOTE: backup_retain_days must be >=
* backup_delete_lock_days. If cron_schedule is defined, then this must be <= 360 * the creation
* interval. If rpo_config is defined, then this must be <= 360 * target_rpo_minutes /
* (1440minutes/day). Default: 0 (no automatic deletion)
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer backupRetainDays;
/**
* Optional. This flag denotes whether the retention policy of this BackupPlan is locked. If set
* to True, no further update is allowed on this policy, including the `locked` field itself.
* Default: False
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Boolean locked;
/**
* Optional. Minimum age for Backups created via this BackupPlan (in days). This field MUST be an
* integer value between 0-90 (inclusive). A Backup created under this BackupPlan will NOT be
* deletable until it reaches Backup's (create_time + backup_delete_lock_days). Updating this
* field of a BackupPlan does NOT affect existing Backups under it. Backups created AFTER a
* successful update will inherit the new value. Default: 0 (no delete blocking)
* @return value or {@code null} for none
*/
public java.lang.Integer getBackupDeleteLockDays() {
return backupDeleteLockDays;
}
/**
* Optional. Minimum age for Backups created via this BackupPlan (in days). This field MUST be an
* integer value between 0-90 (inclusive). A Backup created under this BackupPlan will NOT be
* deletable until it reaches Backup's (create_time + backup_delete_lock_days). Updating this
* field of a BackupPlan does NOT affect existing Backups under it. Backups created AFTER a
* successful update will inherit the new value. Default: 0 (no delete blocking)
* @param backupDeleteLockDays backupDeleteLockDays or {@code null} for none
*/
public RetentionPolicy setBackupDeleteLockDays(java.lang.Integer backupDeleteLockDays) {
this.backupDeleteLockDays = backupDeleteLockDays;
return this;
}
/**
* Optional. The default maximum age of a Backup created via this BackupPlan. This field MUST be
* an integer value >= 0 and <= 365. If specified, a Backup created under this BackupPlan will be
* automatically deleted after its age reaches (create_time + backup_retain_days). If not
* specified, Backups created under this BackupPlan will NOT be subject to automatic deletion.
* Updating this field does NOT affect existing Backups under it. Backups created AFTER a
* successful update will automatically pick up the new value. NOTE: backup_retain_days must be >=
* backup_delete_lock_days. If cron_schedule is defined, then this must be <= 360 * the creation
* interval. If rpo_config is defined, then this must be <= 360 * target_rpo_minutes /
* (1440minutes/day). Default: 0 (no automatic deletion)
* @return value or {@code null} for none
*/
public java.lang.Integer getBackupRetainDays() {
return backupRetainDays;
}
/**
* Optional. The default maximum age of a Backup created via this BackupPlan. This field MUST be
* an integer value >= 0 and <= 365. If specified, a Backup created under this BackupPlan will be
* automatically deleted after its age reaches (create_time + backup_retain_days). If not
* specified, Backups created under this BackupPlan will NOT be subject to automatic deletion.
* Updating this field does NOT affect existing Backups under it. Backups created AFTER a
* successful update will automatically pick up the new value. NOTE: backup_retain_days must be >=
* backup_delete_lock_days. If cron_schedule is defined, then this must be <= 360 * the creation
* interval. If rpo_config is defined, then this must be <= 360 * target_rpo_minutes /
* (1440minutes/day). Default: 0 (no automatic deletion)
* @param backupRetainDays backupRetainDays or {@code null} for none
*/
public RetentionPolicy setBackupRetainDays(java.lang.Integer backupRetainDays) {
this.backupRetainDays = backupRetainDays;
return this;
}
/**
* Optional. This flag denotes whether the retention policy of this BackupPlan is locked. If set
* to True, no further update is allowed on this policy, including the `locked` field itself.
* Default: False
* @return value or {@code null} for none
*/
public java.lang.Boolean getLocked() {
return locked;
}
/**
* Optional. This flag denotes whether the retention policy of this BackupPlan is locked. If set
* to True, no further update is allowed on this policy, including the `locked` field itself.
* Default: False
* @param locked locked or {@code null} for none
*/
public RetentionPolicy setLocked(java.lang.Boolean locked) {
this.locked = locked;
return this;
}
@Override
public RetentionPolicy set(String fieldName, Object value) {
return (RetentionPolicy) super.set(fieldName, value);
}
@Override
public RetentionPolicy clone() {
return (RetentionPolicy) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy