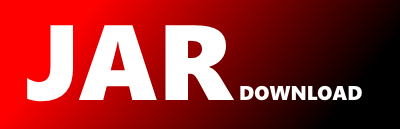
com.google.api.services.gmail.model.Message Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.gmail.model;
/**
* An email message.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Gmail API. For a detailed explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Message extends com.google.api.client.json.GenericJson {
/**
* The ID of the last history record that modified this message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.math.BigInteger historyId;
/**
* The immutable ID of the message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String id;
/**
* The internal message creation timestamp (epoch ms), which determines ordering in the inbox. For
* normal SMTP-received email, this represents the time the message was originally accepted by
* Google, which is more reliable than the `Date` header. However, for API-migrated mail, it can
* be configured by client to be based on the `Date` header.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key @com.google.api.client.json.JsonString
private java.lang.Long internalDate;
/**
* List of IDs of labels applied to this message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.List labelIds;
/**
* The parsed email structure in the message parts.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private MessagePart payload;
/**
* The entire email message in an RFC 2822 formatted and base64url encoded string. Returned in
* `messages.get` and `drafts.get` responses when the `format=RAW` parameter is supplied.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String raw;
/**
* Estimated size in bytes of the message.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.Integer sizeEstimate;
/**
* A short part of the message text.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String snippet;
/**
* The ID of the thread the message belongs to. To add a message or draft to a thread, the
* following criteria must be met: 1. The requested `threadId` must be specified on the `Message`
* or `Draft.Message` you supply with your request. 2. The `References` and `In-Reply-To` headers
* must be set in compliance with the [RFC 2822](https://tools.ietf.org/html/rfc2822) standard. 3.
* The `Subject` headers must match.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String threadId;
/**
* The ID of the last history record that modified this message.
* @return value or {@code null} for none
*/
public java.math.BigInteger getHistoryId() {
return historyId;
}
/**
* The ID of the last history record that modified this message.
* @param historyId historyId or {@code null} for none
*/
public Message setHistoryId(java.math.BigInteger historyId) {
this.historyId = historyId;
return this;
}
/**
* The immutable ID of the message.
* @return value or {@code null} for none
*/
public java.lang.String getId() {
return id;
}
/**
* The immutable ID of the message.
* @param id id or {@code null} for none
*/
public Message setId(java.lang.String id) {
this.id = id;
return this;
}
/**
* The internal message creation timestamp (epoch ms), which determines ordering in the inbox. For
* normal SMTP-received email, this represents the time the message was originally accepted by
* Google, which is more reliable than the `Date` header. However, for API-migrated mail, it can
* be configured by client to be based on the `Date` header.
* @return value or {@code null} for none
*/
public java.lang.Long getInternalDate() {
return internalDate;
}
/**
* The internal message creation timestamp (epoch ms), which determines ordering in the inbox. For
* normal SMTP-received email, this represents the time the message was originally accepted by
* Google, which is more reliable than the `Date` header. However, for API-migrated mail, it can
* be configured by client to be based on the `Date` header.
* @param internalDate internalDate or {@code null} for none
*/
public Message setInternalDate(java.lang.Long internalDate) {
this.internalDate = internalDate;
return this;
}
/**
* List of IDs of labels applied to this message.
* @return value or {@code null} for none
*/
public java.util.List getLabelIds() {
return labelIds;
}
/**
* List of IDs of labels applied to this message.
* @param labelIds labelIds or {@code null} for none
*/
public Message setLabelIds(java.util.List labelIds) {
this.labelIds = labelIds;
return this;
}
/**
* The parsed email structure in the message parts.
* @return value or {@code null} for none
*/
public MessagePart getPayload() {
return payload;
}
/**
* The parsed email structure in the message parts.
* @param payload payload or {@code null} for none
*/
public Message setPayload(MessagePart payload) {
this.payload = payload;
return this;
}
/**
* The entire email message in an RFC 2822 formatted and base64url encoded string. Returned in
* `messages.get` and `drafts.get` responses when the `format=RAW` parameter is supplied.
* @see #decodeRaw()
* @return value or {@code null} for none
*/
public java.lang.String getRaw() {
return raw;
}
/**
* The entire email message in an RFC 2822 formatted and base64url encoded string. Returned in
* `messages.get` and `drafts.get` responses when the `format=RAW` parameter is supplied.
* @see #getRaw()
* @return Base64 decoded value or {@code null} for none
*
* @since 1.14
*/
public byte[] decodeRaw() {
return com.google.api.client.util.Base64.decodeBase64(raw);
}
/**
* The entire email message in an RFC 2822 formatted and base64url encoded string. Returned in
* `messages.get` and `drafts.get` responses when the `format=RAW` parameter is supplied.
* @see #encodeRaw()
* @param raw raw or {@code null} for none
*/
public Message setRaw(java.lang.String raw) {
this.raw = raw;
return this;
}
/**
* The entire email message in an RFC 2822 formatted and base64url encoded string. Returned in
* `messages.get` and `drafts.get` responses when the `format=RAW` parameter is supplied.
* @see #setRaw()
*
*
* The value is encoded Base64 or {@code null} for none.
*
*
* @since 1.14
*/
public Message encodeRaw(byte[] raw) {
this.raw = com.google.api.client.util.Base64.encodeBase64URLSafeString(raw);
return this;
}
/**
* Estimated size in bytes of the message.
* @return value or {@code null} for none
*/
public java.lang.Integer getSizeEstimate() {
return sizeEstimate;
}
/**
* Estimated size in bytes of the message.
* @param sizeEstimate sizeEstimate or {@code null} for none
*/
public Message setSizeEstimate(java.lang.Integer sizeEstimate) {
this.sizeEstimate = sizeEstimate;
return this;
}
/**
* A short part of the message text.
* @return value or {@code null} for none
*/
public java.lang.String getSnippet() {
return snippet;
}
/**
* A short part of the message text.
* @param snippet snippet or {@code null} for none
*/
public Message setSnippet(java.lang.String snippet) {
this.snippet = snippet;
return this;
}
/**
* The ID of the thread the message belongs to. To add a message or draft to a thread, the
* following criteria must be met: 1. The requested `threadId` must be specified on the `Message`
* or `Draft.Message` you supply with your request. 2. The `References` and `In-Reply-To` headers
* must be set in compliance with the [RFC 2822](https://tools.ietf.org/html/rfc2822) standard. 3.
* The `Subject` headers must match.
* @return value or {@code null} for none
*/
public java.lang.String getThreadId() {
return threadId;
}
/**
* The ID of the thread the message belongs to. To add a message or draft to a thread, the
* following criteria must be met: 1. The requested `threadId` must be specified on the `Message`
* or `Draft.Message` you supply with your request. 2. The `References` and `In-Reply-To` headers
* must be set in compliance with the [RFC 2822](https://tools.ietf.org/html/rfc2822) standard. 3.
* The `Subject` headers must match.
* @param threadId threadId or {@code null} for none
*/
public Message setThreadId(java.lang.String threadId) {
this.threadId = threadId;
return this;
}
@Override
public Message set(String fieldName, Object value) {
return (Message) super.set(fieldName, value);
}
@Override
public Message clone() {
return (Message) super.clone();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy