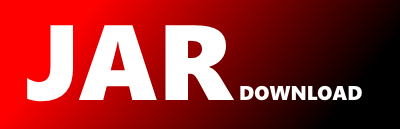
target.apidocs.com.google.api.services.gmail.model.Label.html Maven / Gradle / Ivy
Label (Gmail API v1-rev20230925-2.0.0)
com.google.api.services.gmail.model
Class Label
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.gmail.model.Label
-
public final class Label
extends com.google.api.client.json.GenericJson
Labels are used to categorize messages and threads within the user's mailbox. The maximum number
of labels supported for a user's mailbox is 10,000.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Gmail API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Label()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Label
clone()
LabelColor
getColor()
The color to assign to the label.
String
getId()
The immutable ID of the label.
String
getLabelListVisibility()
The visibility of the label in the label list in the Gmail web interface.
String
getMessageListVisibility()
The visibility of messages with this label in the message list in the Gmail web interface.
Integer
getMessagesTotal()
The total number of messages with the label.
Integer
getMessagesUnread()
The number of unread messages with the label.
String
getName()
The display name of the label.
Integer
getThreadsTotal()
The total number of threads with the label.
Integer
getThreadsUnread()
The number of unread threads with the label.
String
getType()
The owner type for the label.
Label
set(String fieldName,
Object value)
Label
setColor(LabelColor color)
The color to assign to the label.
Label
setId(String id)
The immutable ID of the label.
Label
setLabelListVisibility(String labelListVisibility)
The visibility of the label in the label list in the Gmail web interface.
Label
setMessageListVisibility(String messageListVisibility)
The visibility of messages with this label in the message list in the Gmail web interface.
Label
setMessagesTotal(Integer messagesTotal)
The total number of messages with the label.
Label
setMessagesUnread(Integer messagesUnread)
The number of unread messages with the label.
Label
setName(String name)
The display name of the label.
Label
setThreadsTotal(Integer threadsTotal)
The total number of threads with the label.
Label
setThreadsUnread(Integer threadsUnread)
The number of unread threads with the label.
Label
setType(String type)
The owner type for the label.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getColor
public LabelColor getColor()
The color to assign to the label. Color is only available for labels that have their `type` set
to `user`.
- Returns:
- value or
null
for none
-
setColor
public Label setColor(LabelColor color)
The color to assign to the label. Color is only available for labels that have their `type` set
to `user`.
- Parameters:
color
- color or null
for none
-
getId
public String getId()
The immutable ID of the label.
- Returns:
- value or
null
for none
-
setId
public Label setId(String id)
The immutable ID of the label.
- Parameters:
id
- id or null
for none
-
getLabelListVisibility
public String getLabelListVisibility()
The visibility of the label in the label list in the Gmail web interface.
- Returns:
- value or
null
for none
-
setLabelListVisibility
public Label setLabelListVisibility(String labelListVisibility)
The visibility of the label in the label list in the Gmail web interface.
- Parameters:
labelListVisibility
- labelListVisibility or null
for none
-
getMessageListVisibility
public String getMessageListVisibility()
The visibility of messages with this label in the message list in the Gmail web interface.
- Returns:
- value or
null
for none
-
setMessageListVisibility
public Label setMessageListVisibility(String messageListVisibility)
The visibility of messages with this label in the message list in the Gmail web interface.
- Parameters:
messageListVisibility
- messageListVisibility or null
for none
-
getMessagesTotal
public Integer getMessagesTotal()
The total number of messages with the label.
- Returns:
- value or
null
for none
-
setMessagesTotal
public Label setMessagesTotal(Integer messagesTotal)
The total number of messages with the label.
- Parameters:
messagesTotal
- messagesTotal or null
for none
-
getMessagesUnread
public Integer getMessagesUnread()
The number of unread messages with the label.
- Returns:
- value or
null
for none
-
setMessagesUnread
public Label setMessagesUnread(Integer messagesUnread)
The number of unread messages with the label.
- Parameters:
messagesUnread
- messagesUnread or null
for none
-
getName
public String getName()
The display name of the label.
- Returns:
- value or
null
for none
-
setName
public Label setName(String name)
The display name of the label.
- Parameters:
name
- name or null
for none
-
getThreadsTotal
public Integer getThreadsTotal()
The total number of threads with the label.
- Returns:
- value or
null
for none
-
setThreadsTotal
public Label setThreadsTotal(Integer threadsTotal)
The total number of threads with the label.
- Parameters:
threadsTotal
- threadsTotal or null
for none
-
getThreadsUnread
public Integer getThreadsUnread()
The number of unread threads with the label.
- Returns:
- value or
null
for none
-
setThreadsUnread
public Label setThreadsUnread(Integer threadsUnread)
The number of unread threads with the label.
- Parameters:
threadsUnread
- threadsUnread or null
for none
-
getType
public String getType()
The owner type for the label. User labels are created by the user and can be modified and
deleted by the user and can be applied to any message or thread. System labels are internally
created and cannot be added, modified, or deleted. System labels may be able to be applied to
or removed from messages and threads under some circumstances but this is not guaranteed. For
example, users can apply and remove the `INBOX` and `UNREAD` labels from messages and threads,
but cannot apply or remove the `DRAFTS` or `SENT` labels from messages or threads.
- Returns:
- value or
null
for none
-
setType
public Label setType(String type)
The owner type for the label. User labels are created by the user and can be modified and
deleted by the user and can be applied to any message or thread. System labels are internally
created and cannot be added, modified, or deleted. System labels may be able to be applied to
or removed from messages and threads under some circumstances but this is not guaranteed. For
example, users can apply and remove the `INBOX` and `UNREAD` labels from messages and threads,
but cannot apply or remove the `DRAFTS` or `SENT` labels from messages or threads.
- Parameters:
type
- type or null
for none
-
set
public Label set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Label clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2023 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy