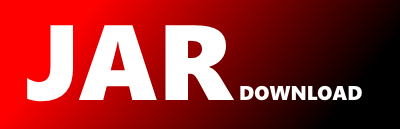
target.apidocs.com.google.api.services.gmail.model.Message.html Maven / Gradle / Ivy
Message (Gmail API v1-rev20230925-2.0.0)
com.google.api.services.gmail.model
Class Message
- java.lang.Object
-
- java.util.AbstractMap<String,Object>
-
- com.google.api.client.util.GenericData
-
- com.google.api.client.json.GenericJson
-
- com.google.api.services.gmail.model.Message
-
public final class Message
extends com.google.api.client.json.GenericJson
An email message.
This is the Java data model class that specifies how to parse/serialize into the JSON that is
transmitted over HTTP when working with the Gmail API. For a detailed explanation see:
https://developers.google.com/api-client-library/java/google-http-java-client/json
- Author:
- Google, Inc.
-
-
Nested Class Summary
-
Nested classes/interfaces inherited from class com.google.api.client.util.GenericData
com.google.api.client.util.GenericData.Flags
-
Nested classes/interfaces inherited from class java.util.AbstractMap
AbstractMap.SimpleEntry<K,V>, AbstractMap.SimpleImmutableEntry<K,V>
-
Constructor Summary
Constructors
Constructor and Description
Message()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Message
clone()
byte[]
decodeRaw()
The entire email message in an RFC 2822 formatted and base64url encoded string.
Message
encodeRaw(byte[] raw)
The entire email message in an RFC 2822 formatted and base64url encoded string.
BigInteger
getHistoryId()
The ID of the last history record that modified this message.
String
getId()
The immutable ID of the message.
Long
getInternalDate()
The internal message creation timestamp (epoch ms), which determines ordering in the inbox.
List<String>
getLabelIds()
List of IDs of labels applied to this message.
MessagePart
getPayload()
The parsed email structure in the message parts.
String
getRaw()
The entire email message in an RFC 2822 formatted and base64url encoded string.
Integer
getSizeEstimate()
Estimated size in bytes of the message.
String
getSnippet()
A short part of the message text.
String
getThreadId()
The ID of the thread the message belongs to.
Message
set(String fieldName,
Object value)
Message
setHistoryId(BigInteger historyId)
The ID of the last history record that modified this message.
Message
setId(String id)
The immutable ID of the message.
Message
setInternalDate(Long internalDate)
The internal message creation timestamp (epoch ms), which determines ordering in the inbox.
Message
setLabelIds(List<String> labelIds)
List of IDs of labels applied to this message.
Message
setPayload(MessagePart payload)
The parsed email structure in the message parts.
Message
setRaw(String raw)
The entire email message in an RFC 2822 formatted and base64url encoded string.
Message
setSizeEstimate(Integer sizeEstimate)
Estimated size in bytes of the message.
Message
setSnippet(String snippet)
A short part of the message text.
Message
setThreadId(String threadId)
The ID of the thread the message belongs to.
-
Methods inherited from class com.google.api.client.json.GenericJson
getFactory, setFactory, toPrettyString, toString
-
Methods inherited from class com.google.api.client.util.GenericData
entrySet, equals, get, getClassInfo, getUnknownKeys, hashCode, put, putAll, remove, setUnknownKeys
-
Methods inherited from class java.util.AbstractMap
clear, containsKey, containsValue, isEmpty, keySet, size, values
-
Methods inherited from class java.lang.Object
finalize, getClass, notify, notifyAll, wait, wait, wait
-
Methods inherited from interface java.util.Map
compute, computeIfAbsent, computeIfPresent, forEach, getOrDefault, merge, putIfAbsent, remove, replace, replace, replaceAll
-
-
Method Detail
-
getHistoryId
public BigInteger getHistoryId()
The ID of the last history record that modified this message.
- Returns:
- value or
null
for none
-
setHistoryId
public Message setHistoryId(BigInteger historyId)
The ID of the last history record that modified this message.
- Parameters:
historyId
- historyId or null
for none
-
getId
public String getId()
The immutable ID of the message.
- Returns:
- value or
null
for none
-
setId
public Message setId(String id)
The immutable ID of the message.
- Parameters:
id
- id or null
for none
-
getInternalDate
public Long getInternalDate()
The internal message creation timestamp (epoch ms), which determines ordering in the inbox. For
normal SMTP-received email, this represents the time the message was originally accepted by
Google, which is more reliable than the `Date` header. However, for API-migrated mail, it can
be configured by client to be based on the `Date` header.
- Returns:
- value or
null
for none
-
setInternalDate
public Message setInternalDate(Long internalDate)
The internal message creation timestamp (epoch ms), which determines ordering in the inbox. For
normal SMTP-received email, this represents the time the message was originally accepted by
Google, which is more reliable than the `Date` header. However, for API-migrated mail, it can
be configured by client to be based on the `Date` header.
- Parameters:
internalDate
- internalDate or null
for none
-
getLabelIds
public List<String> getLabelIds()
List of IDs of labels applied to this message.
- Returns:
- value or
null
for none
-
setLabelIds
public Message setLabelIds(List<String> labelIds)
List of IDs of labels applied to this message.
- Parameters:
labelIds
- labelIds or null
for none
-
getPayload
public MessagePart getPayload()
The parsed email structure in the message parts.
- Returns:
- value or
null
for none
-
setPayload
public Message setPayload(MessagePart payload)
The parsed email structure in the message parts.
- Parameters:
payload
- payload or null
for none
-
getRaw
public String getRaw()
The entire email message in an RFC 2822 formatted and base64url encoded string. Returned in
`messages.get` and `drafts.get` responses when the `format=RAW` parameter is supplied.
- Returns:
- value or
null
for none
- See Also:
decodeRaw()
-
decodeRaw
public byte[] decodeRaw()
The entire email message in an RFC 2822 formatted and base64url encoded string. Returned in
`messages.get` and `drafts.get` responses when the `format=RAW` parameter is supplied.
- Returns:
- Base64 decoded value or
null
for none
- Since:
- 1.14
- See Also:
getRaw()
-
setRaw
public Message setRaw(String raw)
The entire email message in an RFC 2822 formatted and base64url encoded string. Returned in
`messages.get` and `drafts.get` responses when the `format=RAW` parameter is supplied.
- Parameters:
raw
- raw or null
for none
- See Also:
#encodeRaw()
-
encodeRaw
public Message encodeRaw(byte[] raw)
The entire email message in an RFC 2822 formatted and base64url encoded string. Returned in
`messages.get` and `drafts.get` responses when the `format=RAW` parameter is supplied.
- Since:
- 1.14
- See Also:
The value is encoded Base64 or {@code null} for none.
-
getSizeEstimate
public Integer getSizeEstimate()
Estimated size in bytes of the message.
- Returns:
- value or
null
for none
-
setSizeEstimate
public Message setSizeEstimate(Integer sizeEstimate)
Estimated size in bytes of the message.
- Parameters:
sizeEstimate
- sizeEstimate or null
for none
-
getSnippet
public String getSnippet()
A short part of the message text.
- Returns:
- value or
null
for none
-
setSnippet
public Message setSnippet(String snippet)
A short part of the message text.
- Parameters:
snippet
- snippet or null
for none
-
getThreadId
public String getThreadId()
The ID of the thread the message belongs to. To add a message or draft to a thread, the
following criteria must be met: 1. The requested `threadId` must be specified on the `Message`
or `Draft.Message` you supply with your request. 2. The `References` and `In-Reply-To` headers
must be set in compliance with the [RFC 2822](https://tools.ietf.org/html/rfc2822) standard. 3.
The `Subject` headers must match.
- Returns:
- value or
null
for none
-
setThreadId
public Message setThreadId(String threadId)
The ID of the thread the message belongs to. To add a message or draft to a thread, the
following criteria must be met: 1. The requested `threadId` must be specified on the `Message`
or `Draft.Message` you supply with your request. 2. The `References` and `In-Reply-To` headers
must be set in compliance with the [RFC 2822](https://tools.ietf.org/html/rfc2822) standard. 3.
The `Subject` headers must match.
- Parameters:
threadId
- threadId or null
for none
-
set
public Message set(String fieldName,
Object value)
- Overrides:
set
in class com.google.api.client.json.GenericJson
-
clone
public Message clone()
- Overrides:
clone
in class com.google.api.client.json.GenericJson
Copyright © 2011–2023 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy