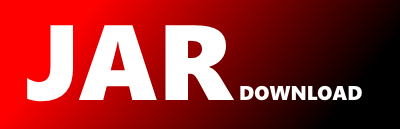
target.apidocs.com.google.api.services.gmail.Gmail.Users.Messages.html Maven / Gradle / Ivy
Gmail.Users.Messages (Gmail API v1-rev20240422-2.0.0)
com.google.api.services.gmail
Class Gmail.Users.Messages
- java.lang.Object
-
- com.google.api.services.gmail.Gmail.Users.Messages
-
- Enclosing class:
- Gmail.Users
public class Gmail.Users.Messages
extends Object
The "messages" collection of methods.
-
-
Nested Class Summary
Nested Classes
Modifier and Type
Class and Description
class
Gmail.Users.Messages.Attachments
The "attachments" collection of methods.
class
Gmail.Users.Messages.BatchDelete
class
Gmail.Users.Messages.BatchModify
class
Gmail.Users.Messages.Delete
class
Gmail.Users.Messages.Get
class
Gmail.Users.Messages.GmailImport
class
Gmail.Users.Messages.Insert
class
Gmail.Users.Messages.List
class
Gmail.Users.Messages.Modify
class
Gmail.Users.Messages.Send
class
Gmail.Users.Messages.Trash
class
Gmail.Users.Messages.Untrash
-
Constructor Summary
Constructors
Constructor and Description
Messages()
-
Method Summary
All Methods Instance Methods Concrete Methods
Modifier and Type
Method and Description
Gmail.Users.Messages.Attachments
attachments()
An accessor for creating requests from the Attachments collection.
Gmail.Users.Messages.BatchDelete
batchDelete(String userId,
BatchDeleteMessagesRequest content)
Deletes many messages by message ID.
Gmail.Users.Messages.BatchModify
batchModify(String userId,
BatchModifyMessagesRequest content)
Modifies the labels on the specified messages.
Gmail.Users.Messages.Delete
delete(String userId,
String id)
Immediately and permanently deletes the specified message.
Gmail.Users.Messages.Get
get(String userId,
String id)
Gets the specified message.
Gmail.Users.Messages.GmailImport
gmailImport(String userId,
Message content)
Imports a message into only this user's mailbox, with standard email delivery scanning and
classification similar to receiving via SMTP.
Gmail.Users.Messages.GmailImport
gmailImport(String userId,
Message content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
Imports a message into only this user's mailbox, with standard email delivery scanning and
classification similar to receiving via SMTP.
Gmail.Users.Messages.Insert
insert(String userId,
Message content)
Directly inserts a message into only this user's mailbox similar to `IMAP APPEND`, bypassing most
scanning and classification.
Gmail.Users.Messages.Insert
insert(String userId,
Message content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
Directly inserts a message into only this user's mailbox similar to `IMAP APPEND`, bypassing most
scanning and classification.
Gmail.Users.Messages.List
list(String userId)
Lists the messages in the user's mailbox.
Gmail.Users.Messages.Modify
modify(String userId,
String id,
ModifyMessageRequest content)
Modifies the labels on the specified message.
Gmail.Users.Messages.Send
send(String userId,
Message content)
Sends the specified message to the recipients in the `To`, `Cc`, and `Bcc` headers.
Gmail.Users.Messages.Send
send(String userId,
Message content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
Sends the specified message to the recipients in the `To`, `Cc`, and `Bcc` headers.
Gmail.Users.Messages.Trash
trash(String userId,
String id)
Moves the specified message to the trash.
Gmail.Users.Messages.Untrash
untrash(String userId,
String id)
Removes the specified message from the trash.
-
-
Method Detail
-
batchDelete
public Gmail.Users.Messages.BatchDelete batchDelete(String userId,
BatchDeleteMessagesRequest content)
throws IOException
Deletes many messages by message ID. Provides no guarantees that messages were not already
deleted or even existed at all.
Create a request for the method "messages.batchDelete".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
content
- the BatchDeleteMessagesRequest
- Returns:
- the request
- Throws:
IOException
-
batchModify
public Gmail.Users.Messages.BatchModify batchModify(String userId,
BatchModifyMessagesRequest content)
throws IOException
Modifies the labels on the specified messages.
Create a request for the method "messages.batchModify".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
content
- the BatchModifyMessagesRequest
- Returns:
- the request
- Throws:
IOException
-
delete
public Gmail.Users.Messages.Delete delete(String userId,
String id)
throws IOException
Immediately and permanently deletes the specified message. This operation cannot be undone.
Prefer `messages.trash` instead.
Create a request for the method "messages.delete".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
id
- The ID of the message to delete.
- Returns:
- the request
- Throws:
IOException
-
get
public Gmail.Users.Messages.Get get(String userId,
String id)
throws IOException
Gets the specified message.
Create a request for the method "messages.get".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
id
- The ID of the message to retrieve. This ID is usually retrieved using `messages.list`. The ID is
also contained in the result when a message is inserted (`messages.insert`) or imported
(`messages.import`).
- Returns:
- the request
- Throws:
IOException
-
gmailImport
public Gmail.Users.Messages.GmailImport gmailImport(String userId,
Message content)
throws IOException
Imports a message into only this user's mailbox, with standard email delivery scanning and
classification similar to receiving via SMTP. This method doesn't perform SPF checks, so it might
not work for some spam messages, such as those attempting to perform domain spoofing. This method
does not send a message.
Create a request for the method "messages.import".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
content
- the Message
- Returns:
- the request
- Throws:
IOException
-
gmailImport
public Gmail.Users.Messages.GmailImport gmailImport(String userId,
Message content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
throws IOException
Imports a message into only this user's mailbox, with standard email delivery scanning and
classification similar to receiving via SMTP. This method doesn't perform SPF checks, so it might
not work for some spam messages, such as those attempting to perform domain spoofing. This method
does not send a message.
Create a request for the method "messages.import".
This request holds the parameters needed by the the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
This method should be used for uploading media content.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
content
- the Message
media metadata or null
if none
mediaContent
- The media HTTP content.
- Returns:
- the request
- Throws:
IOException
- if the initialization of the request fails
-
insert
public Gmail.Users.Messages.Insert insert(String userId,
Message content)
throws IOException
Directly inserts a message into only this user's mailbox similar to `IMAP APPEND`, bypassing most
scanning and classification. Does not send a message.
Create a request for the method "messages.insert".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
content
- the Message
- Returns:
- the request
- Throws:
IOException
-
insert
public Gmail.Users.Messages.Insert insert(String userId,
Message content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
throws IOException
Directly inserts a message into only this user's mailbox similar to `IMAP APPEND`, bypassing most
scanning and classification. Does not send a message.
Create a request for the method "messages.insert".
This request holds the parameters needed by the the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
This method should be used for uploading media content.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
content
- the Message
media metadata or null
if none
mediaContent
- The media HTTP content.
- Returns:
- the request
- Throws:
IOException
- if the initialization of the request fails
-
list
public Gmail.Users.Messages.List list(String userId)
throws IOException
Lists the messages in the user's mailbox.
Create a request for the method "messages.list".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
- Returns:
- the request
- Throws:
IOException
-
modify
public Gmail.Users.Messages.Modify modify(String userId,
String id,
ModifyMessageRequest content)
throws IOException
Modifies the labels on the specified message.
Create a request for the method "messages.modify".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
id
- The ID of the message to modify.
content
- the ModifyMessageRequest
- Returns:
- the request
- Throws:
IOException
-
send
public Gmail.Users.Messages.Send send(String userId,
Message content)
throws IOException
Sends the specified message to the recipients in the `To`, `Cc`, and `Bcc` headers. For example
usage, see [Sending email](https://developers.google.com/gmail/api/guides/sending).
Create a request for the method "messages.send".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
content
- the Message
- Returns:
- the request
- Throws:
IOException
-
send
public Gmail.Users.Messages.Send send(String userId,
Message content,
com.google.api.client.http.AbstractInputStreamContent mediaContent)
throws IOException
Sends the specified message to the recipients in the `To`, `Cc`, and `Bcc` headers. For example
usage, see [Sending email](https://developers.google.com/gmail/api/guides/sending).
Create a request for the method "messages.send".
This request holds the parameters needed by the the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
This method should be used for uploading media content.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
content
- the Message
media metadata or null
if none
mediaContent
- The media HTTP content.
- Returns:
- the request
- Throws:
IOException
- if the initialization of the request fails
-
trash
public Gmail.Users.Messages.Trash trash(String userId,
String id)
throws IOException
Moves the specified message to the trash.
Create a request for the method "messages.trash".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
id
- The ID of the message to Trash.
- Returns:
- the request
- Throws:
IOException
-
untrash
public Gmail.Users.Messages.Untrash untrash(String userId,
String id)
throws IOException
Removes the specified message from the trash.
Create a request for the method "messages.untrash".
This request holds the parameters needed by the gmail server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
userId
- The user's email address. The special value `me` can be used to indicate the authenticated user.
[default: me]
id
- The ID of the message to remove from Trash.
- Returns:
- the request
- Throws:
IOException
-
attachments
public Gmail.Users.Messages.Attachments attachments()
An accessor for creating requests from the Attachments collection.
The typical use is:
Gmail gmail = new Gmail(...);
Gmail.Attachments.List request = gmail.attachments().list(parameters ...)
- Returns:
- the resource collection
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2024 Weber Informatics LLC | Privacy Policy