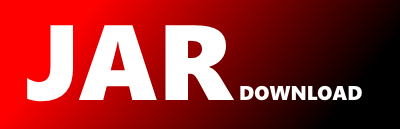
com.google.api.services.gmail.Gmail Maven / Gradle / Ivy
/*
* Copyright 2010 Google Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/google/apis-client-generator/
* (build: 2016-05-27 16:00:31 UTC)
* on 2016-06-24 at 22:52:46 UTC
* Modify at your own risk.
*/
package com.google.api.services.gmail;
/**
* Service definition for Gmail (v1).
*
*
* Access Gmail mailboxes including sending user email.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link GmailRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Gmail extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 15,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.15 of google-api-client to run version " +
"1.22.0 of the Gmail API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://www.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "gmail/v1/users/";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Gmail(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Gmail(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Users collection.
*
* The typical use is:
*
* {@code Gmail gmail = new Gmail(...);}
* {@code Gmail.Users.List request = gmail.users().list(parameters ...)}
*
*
* @return the resource collection
*/
public Users users() {
return new Users();
}
/**
* The "users" collection of methods.
*/
public class Users {
/**
* Gets the current user's Gmail profile.
*
* Create a request for the method "users.getProfile".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link GetProfile#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @return the request
*/
public GetProfile getProfile(java.lang.String userId) throws java.io.IOException {
GetProfile result = new GetProfile(userId);
initialize(result);
return result;
}
public class GetProfile extends GmailRequest {
private static final String REST_PATH = "{userId}/profile";
/**
* Gets the current user's Gmail profile.
*
* Create a request for the method "users.getProfile".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link GetProfile#execute()} method to invoke the remote operation.
* {@link
* GetProfile#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @since 1.13
*/
protected GetProfile(java.lang.String userId) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.Profile.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetProfile setAlt(java.lang.String alt) {
return (GetProfile) super.setAlt(alt);
}
@Override
public GetProfile setFields(java.lang.String fields) {
return (GetProfile) super.setFields(fields);
}
@Override
public GetProfile setKey(java.lang.String key) {
return (GetProfile) super.setKey(key);
}
@Override
public GetProfile setOauthToken(java.lang.String oauthToken) {
return (GetProfile) super.setOauthToken(oauthToken);
}
@Override
public GetProfile setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetProfile) super.setPrettyPrint(prettyPrint);
}
@Override
public GetProfile setQuotaUser(java.lang.String quotaUser) {
return (GetProfile) super.setQuotaUser(quotaUser);
}
@Override
public GetProfile setUserIp(java.lang.String userIp) {
return (GetProfile) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public GetProfile setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public GetProfile set(String parameterName, Object value) {
return (GetProfile) super.set(parameterName, value);
}
}
/**
* Stop receiving push notifications for the given user mailbox.
*
* Create a request for the method "users.stop".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Stop#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @return the request
*/
public Stop stop(java.lang.String userId) throws java.io.IOException {
Stop result = new Stop(userId);
initialize(result);
return result;
}
public class Stop extends GmailRequest {
private static final String REST_PATH = "{userId}/stop";
/**
* Stop receiving push notifications for the given user mailbox.
*
* Create a request for the method "users.stop".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Stop#execute()} method to invoke the remote operation. {@link
* Stop#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @since 1.13
*/
protected Stop(java.lang.String userId) {
super(Gmail.this, "POST", REST_PATH, null, Void.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Stop setAlt(java.lang.String alt) {
return (Stop) super.setAlt(alt);
}
@Override
public Stop setFields(java.lang.String fields) {
return (Stop) super.setFields(fields);
}
@Override
public Stop setKey(java.lang.String key) {
return (Stop) super.setKey(key);
}
@Override
public Stop setOauthToken(java.lang.String oauthToken) {
return (Stop) super.setOauthToken(oauthToken);
}
@Override
public Stop setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Stop) super.setPrettyPrint(prettyPrint);
}
@Override
public Stop setQuotaUser(java.lang.String quotaUser) {
return (Stop) super.setQuotaUser(quotaUser);
}
@Override
public Stop setUserIp(java.lang.String userIp) {
return (Stop) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Stop setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Stop set(String parameterName, Object value) {
return (Stop) super.set(parameterName, value);
}
}
/**
* Set up or update a push notification watch on the given user mailbox.
*
* Create a request for the method "users.watch".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Watch#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.WatchRequest}
* @return the request
*/
public Watch watch(java.lang.String userId, com.google.api.services.gmail.model.WatchRequest content) throws java.io.IOException {
Watch result = new Watch(userId, content);
initialize(result);
return result;
}
public class Watch extends GmailRequest {
private static final String REST_PATH = "{userId}/watch";
/**
* Set up or update a push notification watch on the given user mailbox.
*
* Create a request for the method "users.watch".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Watch#execute()} method to invoke the remote operation. {@link
* Watch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.WatchRequest}
* @since 1.13
*/
protected Watch(java.lang.String userId, com.google.api.services.gmail.model.WatchRequest content) {
super(Gmail.this, "POST", REST_PATH, content, com.google.api.services.gmail.model.WatchResponse.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public Watch setAlt(java.lang.String alt) {
return (Watch) super.setAlt(alt);
}
@Override
public Watch setFields(java.lang.String fields) {
return (Watch) super.setFields(fields);
}
@Override
public Watch setKey(java.lang.String key) {
return (Watch) super.setKey(key);
}
@Override
public Watch setOauthToken(java.lang.String oauthToken) {
return (Watch) super.setOauthToken(oauthToken);
}
@Override
public Watch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Watch) super.setPrettyPrint(prettyPrint);
}
@Override
public Watch setQuotaUser(java.lang.String quotaUser) {
return (Watch) super.setQuotaUser(quotaUser);
}
@Override
public Watch setUserIp(java.lang.String userIp) {
return (Watch) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Watch setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Watch set(String parameterName, Object value) {
return (Watch) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Drafts collection.
*
* The typical use is:
*
* {@code Gmail gmail = new Gmail(...);}
* {@code Gmail.Drafts.List request = gmail.drafts().list(parameters ...)}
*
*
* @return the resource collection
*/
public Drafts drafts() {
return new Drafts();
}
/**
* The "drafts" collection of methods.
*/
public class Drafts {
/**
* Creates a new draft with the DRAFT label.
*
* Create a request for the method "drafts.create".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Draft}
* @return the request
*/
public Create create(java.lang.String userId, com.google.api.services.gmail.model.Draft content) throws java.io.IOException {
Create result = new Create(userId, content);
initialize(result);
return result;
}
/**
* Creates a new draft with the DRAFT label.
*
* Create a request for the method "drafts.create".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Draft} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Create create(java.lang.String userId, com.google.api.services.gmail.model.Draft content, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Create result = new Create(userId, content, mediaContent);
initialize(result);
return result;
}
public class Create extends GmailRequest {
private static final String REST_PATH = "{userId}/drafts";
/**
* Creates a new draft with the DRAFT label.
*
* Create a request for the method "drafts.create".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Draft}
* @since 1.13
*/
protected Create(java.lang.String userId, com.google.api.services.gmail.model.Draft content) {
super(Gmail.this, "POST", REST_PATH, content, com.google.api.services.gmail.model.Draft.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
/**
* Creates a new draft with the DRAFT label.
*
* Create a request for the method "drafts.create".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Draft} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Create(java.lang.String userId, com.google.api.services.gmail.model.Draft content, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(Gmail.this, "POST", "/upload/" + getServicePath() + REST_PATH, content, com.google.api.services.gmail.model.Draft.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Create setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Immediately and permanently deletes the specified draft. Does not simply trash it.
*
* Create a request for the method "drafts.delete".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the draft to delete.
* @return the request
*/
public Delete delete(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Delete result = new Delete(userId, id);
initialize(result);
return result;
}
public class Delete extends GmailRequest {
private static final String REST_PATH = "{userId}/drafts/{id}";
/**
* Immediately and permanently deletes the specified draft. Does not simply trash it.
*
* Create a request for the method "drafts.delete".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the draft to delete.
* @since 1.13
*/
protected Delete(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "DELETE", REST_PATH, null, Void.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the draft to delete. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the draft to delete.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the draft to delete. */
public Delete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the specified draft.
*
* Create a request for the method "drafts.get".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the draft to retrieve.
* @return the request
*/
public Get get(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Get result = new Get(userId, id);
initialize(result);
return result;
}
public class Get extends GmailRequest {
private static final String REST_PATH = "{userId}/drafts/{id}";
/**
* Gets the specified draft.
*
* Create a request for the method "drafts.get".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the draft to retrieve.
* @since 1.13
*/
protected Get(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.Draft.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the draft to retrieve. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the draft to retrieve.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the draft to retrieve. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
/** The format to return the draft in. */
@com.google.api.client.util.Key
private java.lang.String format;
/** The format to return the draft in. [default: full]
*/
public java.lang.String getFormat() {
return format;
}
/** The format to return the draft in. */
public Get setFormat(java.lang.String format) {
this.format = format;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the drafts in the user's mailbox.
*
* Create a request for the method "drafts.list".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @return the request
*/
public List list(java.lang.String userId) throws java.io.IOException {
List result = new List(userId);
initialize(result);
return result;
}
public class List extends GmailRequest {
private static final String REST_PATH = "{userId}/drafts";
/**
* Lists the drafts in the user's mailbox.
*
* Create a request for the method "drafts.list".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @since 1.13
*/
protected List(java.lang.String userId) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.ListDraftsResponse.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** Include drafts from SPAM and TRASH in the results. */
@com.google.api.client.util.Key
private java.lang.Boolean includeSpamTrash;
/** Include drafts from SPAM and TRASH in the results. [default: false]
*/
public java.lang.Boolean getIncludeSpamTrash() {
return includeSpamTrash;
}
/** Include drafts from SPAM and TRASH in the results. */
public List setIncludeSpamTrash(java.lang.Boolean includeSpamTrash) {
this.includeSpamTrash = includeSpamTrash;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Include drafts from SPAM and TRASH in the results.
*
*/
public boolean isIncludeSpamTrash() {
if (includeSpamTrash == null || includeSpamTrash == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return includeSpamTrash;
}
/** Maximum number of drafts to return. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of drafts to return. [default: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of drafts to return. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Page token to retrieve a specific page of results in the list. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Page token to retrieve a specific page of results in the list.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Page token to retrieve a specific page of results in the list. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Only return draft messages matching the specified query. Supports the same query format
* as the Gmail search box. For example, "from:[email protected] rfc822msgid: is:unread".
*/
@com.google.api.client.util.Key
private java.lang.String q;
/** Only return draft messages matching the specified query. Supports the same query format as the
Gmail search box. For example, "from:[email protected] rfc822msgid: is:unread".
*/
public java.lang.String getQ() {
return q;
}
/**
* Only return draft messages matching the specified query. Supports the same query format
* as the Gmail search box. For example, "from:[email protected] rfc822msgid: is:unread".
*/
public List setQ(java.lang.String q) {
this.q = q;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Sends the specified, existing draft to the recipients in the To, Cc, and Bcc headers.
*
* Create a request for the method "drafts.send".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Send#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Draft}
* @return the request
*/
public Send send(java.lang.String userId, com.google.api.services.gmail.model.Draft content) throws java.io.IOException {
Send result = new Send(userId, content);
initialize(result);
return result;
}
/**
* Sends the specified, existing draft to the recipients in the To, Cc, and Bcc headers.
*
* Create a request for the method "drafts.send".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Send#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Draft} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Send send(java.lang.String userId, com.google.api.services.gmail.model.Draft content, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Send result = new Send(userId, content, mediaContent);
initialize(result);
return result;
}
public class Send extends GmailRequest {
private static final String REST_PATH = "{userId}/drafts/send";
/**
* Sends the specified, existing draft to the recipients in the To, Cc, and Bcc headers.
*
* Create a request for the method "drafts.send".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Send#execute()} method to invoke the remote operation. {@link
* Send#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Draft}
* @since 1.13
*/
protected Send(java.lang.String userId, com.google.api.services.gmail.model.Draft content) {
super(Gmail.this, "POST", REST_PATH, content, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
/**
* Sends the specified, existing draft to the recipients in the To, Cc, and Bcc headers.
*
* Create a request for the method "drafts.send".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Send#execute()} method to invoke the remote operation. {@link
* Send#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Draft} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Send(java.lang.String userId, com.google.api.services.gmail.model.Draft content, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(Gmail.this, "POST", "/upload/" + getServicePath() + REST_PATH, content, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Send setAlt(java.lang.String alt) {
return (Send) super.setAlt(alt);
}
@Override
public Send setFields(java.lang.String fields) {
return (Send) super.setFields(fields);
}
@Override
public Send setKey(java.lang.String key) {
return (Send) super.setKey(key);
}
@Override
public Send setOauthToken(java.lang.String oauthToken) {
return (Send) super.setOauthToken(oauthToken);
}
@Override
public Send setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Send) super.setPrettyPrint(prettyPrint);
}
@Override
public Send setQuotaUser(java.lang.String quotaUser) {
return (Send) super.setQuotaUser(quotaUser);
}
@Override
public Send setUserIp(java.lang.String userIp) {
return (Send) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Send setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Send set(String parameterName, Object value) {
return (Send) super.set(parameterName, value);
}
}
/**
* Replaces a draft's content.
*
* Create a request for the method "drafts.update".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the draft to update.
* @param content the {@link com.google.api.services.gmail.model.Draft}
* @return the request
*/
public Update update(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.Draft content) throws java.io.IOException {
Update result = new Update(userId, id, content);
initialize(result);
return result;
}
/**
* Replaces a draft's content.
*
* Create a request for the method "drafts.update".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]@param id The ID of the draft to update.
* @param content the {@link com.google.api.services.gmail.model.Draft} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Update update(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.Draft content, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Update result = new Update(userId, id, content, mediaContent);
initialize(result);
return result;
}
public class Update extends GmailRequest {
private static final String REST_PATH = "{userId}/drafts/{id}";
/**
* Replaces a draft's content.
*
* Create a request for the method "drafts.update".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the draft to update.
* @param content the {@link com.google.api.services.gmail.model.Draft}
* @since 1.13
*/
protected Update(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.Draft content) {
super(Gmail.this, "PUT", REST_PATH, content, com.google.api.services.gmail.model.Draft.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
/**
* Replaces a draft's content.
*
* Create a request for the method "drafts.update".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]@param id The ID of the draft to update.
* @param content the {@link com.google.api.services.gmail.model.Draft} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Update(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.Draft content, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(Gmail.this, "PUT", "/upload/" + getServicePath() + REST_PATH, content, com.google.api.services.gmail.model.Draft.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Update setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the draft to update. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the draft to update.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the draft to update. */
public Update setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the History collection.
*
* The typical use is:
*
* {@code Gmail gmail = new Gmail(...);}
* {@code Gmail.History.List request = gmail.history().list(parameters ...)}
*
*
* @return the resource collection
*/
public History history() {
return new History();
}
/**
* The "history" collection of methods.
*/
public class History {
/**
* Lists the history of all changes to the given mailbox. History results are returned in
* chronological order (increasing historyId).
*
* Create a request for the method "history.list".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @return the request
*/
public List list(java.lang.String userId) throws java.io.IOException {
List result = new List(userId);
initialize(result);
return result;
}
public class List extends GmailRequest {
private static final String REST_PATH = "{userId}/history";
/**
* Lists the history of all changes to the given mailbox. History results are returned in
* chronological order (increasing historyId).
*
* Create a request for the method "history.list".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @since 1.13
*/
protected List(java.lang.String userId) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.ListHistoryResponse.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** Only return messages with a label matching the ID. */
@com.google.api.client.util.Key
private java.lang.String labelId;
/** Only return messages with a label matching the ID.
*/
public java.lang.String getLabelId() {
return labelId;
}
/** Only return messages with a label matching the ID. */
public List setLabelId(java.lang.String labelId) {
this.labelId = labelId;
return this;
}
/** The maximum number of history records to return. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** The maximum number of history records to return. [default: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** The maximum number of history records to return. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Page token to retrieve a specific page of results in the list. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Page token to retrieve a specific page of results in the list.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Page token to retrieve a specific page of results in the list. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Required. Returns history records after the specified startHistoryId. The supplied
* startHistoryId should be obtained from the historyId of a message, thread, or previous
* list response. History IDs increase chronologically but are not contiguous with random
* gaps in between valid IDs. Supplying an invalid or out of date startHistoryId typically
* returns an HTTP 404 error code. A historyId is typically valid for at least a week, but
* in some rare circumstances may be valid for only a few hours. If you receive an HTTP 404
* error response, your application should perform a full sync. If you receive no
* nextPageToken in the response, there are no updates to retrieve and you can store the
* returned historyId for a future request.
*/
@com.google.api.client.util.Key
private java.math.BigInteger startHistoryId;
/** Required. Returns history records after the specified startHistoryId. The supplied startHistoryId
should be obtained from the historyId of a message, thread, or previous list response. History IDs
increase chronologically but are not contiguous with random gaps in between valid IDs. Supplying an
invalid or out of date startHistoryId typically returns an HTTP 404 error code. A historyId is
typically valid for at least a week, but in some rare circumstances may be valid for only a few
hours. If you receive an HTTP 404 error response, your application should perform a full sync. If
you receive no nextPageToken in the response, there are no updates to retrieve and you can store
the returned historyId for a future request.
*/
public java.math.BigInteger getStartHistoryId() {
return startHistoryId;
}
/**
* Required. Returns history records after the specified startHistoryId. The supplied
* startHistoryId should be obtained from the historyId of a message, thread, or previous
* list response. History IDs increase chronologically but are not contiguous with random
* gaps in between valid IDs. Supplying an invalid or out of date startHistoryId typically
* returns an HTTP 404 error code. A historyId is typically valid for at least a week, but
* in some rare circumstances may be valid for only a few hours. If you receive an HTTP 404
* error response, your application should perform a full sync. If you receive no
* nextPageToken in the response, there are no updates to retrieve and you can store the
* returned historyId for a future request.
*/
public List setStartHistoryId(java.math.BigInteger startHistoryId) {
this.startHistoryId = startHistoryId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Labels collection.
*
* The typical use is:
*
* {@code Gmail gmail = new Gmail(...);}
* {@code Gmail.Labels.List request = gmail.labels().list(parameters ...)}
*
*
* @return the resource collection
*/
public Labels labels() {
return new Labels();
}
/**
* The "labels" collection of methods.
*/
public class Labels {
/**
* Creates a new label.
*
* Create a request for the method "labels.create".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Label}
* @return the request
*/
public Create create(java.lang.String userId, com.google.api.services.gmail.model.Label content) throws java.io.IOException {
Create result = new Create(userId, content);
initialize(result);
return result;
}
public class Create extends GmailRequest {
private static final String REST_PATH = "{userId}/labels";
/**
* Creates a new label.
*
* Create a request for the method "labels.create".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Label}
* @since 1.13
*/
protected Create(java.lang.String userId, com.google.api.services.gmail.model.Label content) {
super(Gmail.this, "POST", REST_PATH, content, com.google.api.services.gmail.model.Label.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getLabelListVisibility(), "Label.getLabelListVisibility()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getMessageListVisibility(), "Label.getMessageListVisibility()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Label.getName()");
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUserIp(java.lang.String userIp) {
return (Create) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Create setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Immediately and permanently deletes the specified label and removes it from any messages and
* threads that it is applied to.
*
* Create a request for the method "labels.delete".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the label to delete.
* @return the request
*/
public Delete delete(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Delete result = new Delete(userId, id);
initialize(result);
return result;
}
public class Delete extends GmailRequest {
private static final String REST_PATH = "{userId}/labels/{id}";
/**
* Immediately and permanently deletes the specified label and removes it from any messages and
* threads that it is applied to.
*
* Create a request for the method "labels.delete".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the label to delete.
* @since 1.13
*/
protected Delete(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "DELETE", REST_PATH, null, Void.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the label to delete. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the label to delete.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the label to delete. */
public Delete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the specified label.
*
* Create a request for the method "labels.get".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the label to retrieve.
* @return the request
*/
public Get get(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Get result = new Get(userId, id);
initialize(result);
return result;
}
public class Get extends GmailRequest {
private static final String REST_PATH = "{userId}/labels/{id}";
/**
* Gets the specified label.
*
* Create a request for the method "labels.get".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the label to retrieve.
* @since 1.13
*/
protected Get(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.Label.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the label to retrieve. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the label to retrieve.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the label to retrieve. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists all labels in the user's mailbox.
*
* Create a request for the method "labels.list".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @return the request
*/
public List list(java.lang.String userId) throws java.io.IOException {
List result = new List(userId);
initialize(result);
return result;
}
public class List extends GmailRequest {
private static final String REST_PATH = "{userId}/labels";
/**
* Lists all labels in the user's mailbox.
*
* Create a request for the method "labels.list".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @since 1.13
*/
protected List(java.lang.String userId) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.ListLabelsResponse.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates the specified label. This method supports patch semantics.
*
* Create a request for the method "labels.patch".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the label to update.
* @param content the {@link com.google.api.services.gmail.model.Label}
* @return the request
*/
public Patch patch(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.Label content) throws java.io.IOException {
Patch result = new Patch(userId, id, content);
initialize(result);
return result;
}
public class Patch extends GmailRequest {
private static final String REST_PATH = "{userId}/labels/{id}";
/**
* Updates the specified label. This method supports patch semantics.
*
* Create a request for the method "labels.patch".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the label to update.
* @param content the {@link com.google.api.services.gmail.model.Label}
* @since 1.13
*/
protected Patch(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.Label content) {
super(Gmail.this, "PATCH", REST_PATH, content, com.google.api.services.gmail.model.Label.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUserIp(java.lang.String userIp) {
return (Patch) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Patch setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the label to update. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the label to update.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the label to update. */
public Patch setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
/**
* Updates the specified label.
*
* Create a request for the method "labels.update".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the label to update.
* @param content the {@link com.google.api.services.gmail.model.Label}
* @return the request
*/
public Update update(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.Label content) throws java.io.IOException {
Update result = new Update(userId, id, content);
initialize(result);
return result;
}
public class Update extends GmailRequest {
private static final String REST_PATH = "{userId}/labels/{id}";
/**
* Updates the specified label.
*
* Create a request for the method "labels.update".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the label to update.
* @param content the {@link com.google.api.services.gmail.model.Label}
* @since 1.13
*/
protected Update(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.Label content) {
super(Gmail.this, "PUT", REST_PATH, content, com.google.api.services.gmail.model.Label.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getId(), "Label.getId()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getLabelListVisibility(), "Label.getLabelListVisibility()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getMessageListVisibility(), "Label.getMessageListVisibility()");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getName(), "Label.getName()");
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUserIp(java.lang.String userIp) {
return (Update) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Update setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the label to update. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the label to update.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the label to update. */
public Update setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
}
/**
* An accessor for creating requests from the Messages collection.
*
* The typical use is:
*
* {@code Gmail gmail = new Gmail(...);}
* {@code Gmail.Messages.List request = gmail.messages().list(parameters ...)}
*
*
* @return the resource collection
*/
public Messages messages() {
return new Messages();
}
/**
* The "messages" collection of methods.
*/
public class Messages {
/**
* Deletes many messages by message ID. Provides no guarantees that messages were not already
* deleted or even existed at all.
*
* Create a request for the method "messages.batchDelete".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.BatchDeleteMessagesRequest}
* @return the request
*/
public BatchDelete batchDelete(java.lang.String userId, com.google.api.services.gmail.model.BatchDeleteMessagesRequest content) throws java.io.IOException {
BatchDelete result = new BatchDelete(userId, content);
initialize(result);
return result;
}
public class BatchDelete extends GmailRequest {
private static final String REST_PATH = "{userId}/messages/batchDelete";
/**
* Deletes many messages by message ID. Provides no guarantees that messages were not already
* deleted or even existed at all.
*
* Create a request for the method "messages.batchDelete".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link BatchDelete#execute()} method to invoke the remote operation.
* {@link
* BatchDelete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.BatchDeleteMessagesRequest}
* @since 1.13
*/
protected BatchDelete(java.lang.String userId, com.google.api.services.gmail.model.BatchDeleteMessagesRequest content) {
super(Gmail.this, "POST", REST_PATH, content, Void.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public BatchDelete setAlt(java.lang.String alt) {
return (BatchDelete) super.setAlt(alt);
}
@Override
public BatchDelete setFields(java.lang.String fields) {
return (BatchDelete) super.setFields(fields);
}
@Override
public BatchDelete setKey(java.lang.String key) {
return (BatchDelete) super.setKey(key);
}
@Override
public BatchDelete setOauthToken(java.lang.String oauthToken) {
return (BatchDelete) super.setOauthToken(oauthToken);
}
@Override
public BatchDelete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (BatchDelete) super.setPrettyPrint(prettyPrint);
}
@Override
public BatchDelete setQuotaUser(java.lang.String quotaUser) {
return (BatchDelete) super.setQuotaUser(quotaUser);
}
@Override
public BatchDelete setUserIp(java.lang.String userIp) {
return (BatchDelete) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public BatchDelete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public BatchDelete set(String parameterName, Object value) {
return (BatchDelete) super.set(parameterName, value);
}
}
/**
* Immediately and permanently deletes the specified message. This operation cannot be undone.
* Prefer messages.trash instead.
*
* Create a request for the method "messages.delete".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to delete.
* @return the request
*/
public Delete delete(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Delete result = new Delete(userId, id);
initialize(result);
return result;
}
public class Delete extends GmailRequest {
private static final String REST_PATH = "{userId}/messages/{id}";
/**
* Immediately and permanently deletes the specified message. This operation cannot be undone.
* Prefer messages.trash instead.
*
* Create a request for the method "messages.delete".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to delete.
* @since 1.13
*/
protected Delete(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "DELETE", REST_PATH, null, Void.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the message to delete. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the message to delete.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the message to delete. */
public Delete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the specified message.
*
* Create a request for the method "messages.get".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to retrieve.
* @return the request
*/
public Get get(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Get result = new Get(userId, id);
initialize(result);
return result;
}
public class Get extends GmailRequest {
private static final String REST_PATH = "{userId}/messages/{id}";
/**
* Gets the specified message.
*
* Create a request for the method "messages.get".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to retrieve.
* @since 1.13
*/
protected Get(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the message to retrieve. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the message to retrieve.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the message to retrieve. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
/** The format to return the message in. */
@com.google.api.client.util.Key
private java.lang.String format;
/** The format to return the message in. [default: full]
*/
public java.lang.String getFormat() {
return format;
}
/** The format to return the message in. */
public Get setFormat(java.lang.String format) {
this.format = format;
return this;
}
/** When given and format is METADATA, only include headers specified. */
@com.google.api.client.util.Key
private java.util.List metadataHeaders;
/** When given and format is METADATA, only include headers specified.
*/
public java.util.List getMetadataHeaders() {
return metadataHeaders;
}
/** When given and format is METADATA, only include headers specified. */
public Get setMetadataHeaders(java.util.List metadataHeaders) {
this.metadataHeaders = metadataHeaders;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Imports a message into only this user's mailbox, with standard email delivery scanning and
* classification similar to receiving via SMTP. Does not send a message.
*
* Create a request for the method "messages.import".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link GmailImport#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message}
* @return the request
*/
public GmailImport gmailImport(java.lang.String userId, com.google.api.services.gmail.model.Message content) throws java.io.IOException {
GmailImport result = new GmailImport(userId, content);
initialize(result);
return result;
}
/**
* Imports a message into only this user's mailbox, with standard email delivery scanning and
* classification similar to receiving via SMTP. Does not send a message.
*
* Create a request for the method "messages.import".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link GmailImport#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public GmailImport gmailImport(java.lang.String userId, com.google.api.services.gmail.model.Message content, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
GmailImport result = new GmailImport(userId, content, mediaContent);
initialize(result);
return result;
}
public class GmailImport extends GmailRequest {
private static final String REST_PATH = "{userId}/messages/import";
/**
* Imports a message into only this user's mailbox, with standard email delivery scanning and
* classification similar to receiving via SMTP. Does not send a message.
*
* Create a request for the method "messages.import".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link GmailImport#execute()} method to invoke the remote operation.
* {@link
* GmailImport#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message}
* @since 1.13
*/
protected GmailImport(java.lang.String userId, com.google.api.services.gmail.model.Message content) {
super(Gmail.this, "POST", REST_PATH, content, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
/**
* Imports a message into only this user's mailbox, with standard email delivery scanning and
* classification similar to receiving via SMTP. Does not send a message.
*
* Create a request for the method "messages.import".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link GmailImport#execute()} method to invoke the remote operation.
* {@link
* GmailImport#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected GmailImport(java.lang.String userId, com.google.api.services.gmail.model.Message content, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(Gmail.this, "POST", "/upload/" + getServicePath() + REST_PATH, content, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public GmailImport setAlt(java.lang.String alt) {
return (GmailImport) super.setAlt(alt);
}
@Override
public GmailImport setFields(java.lang.String fields) {
return (GmailImport) super.setFields(fields);
}
@Override
public GmailImport setKey(java.lang.String key) {
return (GmailImport) super.setKey(key);
}
@Override
public GmailImport setOauthToken(java.lang.String oauthToken) {
return (GmailImport) super.setOauthToken(oauthToken);
}
@Override
public GmailImport setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GmailImport) super.setPrettyPrint(prettyPrint);
}
@Override
public GmailImport setQuotaUser(java.lang.String quotaUser) {
return (GmailImport) super.setQuotaUser(quotaUser);
}
@Override
public GmailImport setUserIp(java.lang.String userIp) {
return (GmailImport) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public GmailImport setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/**
* Mark the email as permanently deleted (not TRASH) and only visible in Google Apps Vault
* to a Vault administrator. Only used for Google Apps for Work accounts.
*/
@com.google.api.client.util.Key
private java.lang.Boolean deleted;
/** Mark the email as permanently deleted (not TRASH) and only visible in Google Apps Vault to a Vault
administrator. Only used for Google Apps for Work accounts. [default: false]
*/
public java.lang.Boolean getDeleted() {
return deleted;
}
/**
* Mark the email as permanently deleted (not TRASH) and only visible in Google Apps Vault
* to a Vault administrator. Only used for Google Apps for Work accounts.
*/
public GmailImport setDeleted(java.lang.Boolean deleted) {
this.deleted = deleted;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Mark the email as permanently deleted (not TRASH) and only visible in Google Apps Vault to a Vault
administrator. Only used for Google Apps for Work accounts.
*
*/
public boolean isDeleted() {
if (deleted == null || deleted == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return deleted;
}
/** Source for Gmail's internal date of the message. */
@com.google.api.client.util.Key
private java.lang.String internalDateSource;
/** Source for Gmail's internal date of the message. [default: dateHeader]
*/
public java.lang.String getInternalDateSource() {
return internalDateSource;
}
/** Source for Gmail's internal date of the message. */
public GmailImport setInternalDateSource(java.lang.String internalDateSource) {
this.internalDateSource = internalDateSource;
return this;
}
/**
* Ignore the Gmail spam classifier decision and never mark this email as SPAM in the
* mailbox.
*/
@com.google.api.client.util.Key
private java.lang.Boolean neverMarkSpam;
/** Ignore the Gmail spam classifier decision and never mark this email as SPAM in the mailbox.
[default: false]
*/
public java.lang.Boolean getNeverMarkSpam() {
return neverMarkSpam;
}
/**
* Ignore the Gmail spam classifier decision and never mark this email as SPAM in the
* mailbox.
*/
public GmailImport setNeverMarkSpam(java.lang.Boolean neverMarkSpam) {
this.neverMarkSpam = neverMarkSpam;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Ignore the Gmail spam classifier decision and never mark this email as SPAM in the mailbox.
*
*/
public boolean isNeverMarkSpam() {
if (neverMarkSpam == null || neverMarkSpam == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return neverMarkSpam;
}
/**
* Process calendar invites in the email and add any extracted meetings to the Google
* Calendar for this user.
*/
@com.google.api.client.util.Key
private java.lang.Boolean processForCalendar;
/** Process calendar invites in the email and add any extracted meetings to the Google Calendar for
this user. [default: false]
*/
public java.lang.Boolean getProcessForCalendar() {
return processForCalendar;
}
/**
* Process calendar invites in the email and add any extracted meetings to the Google
* Calendar for this user.
*/
public GmailImport setProcessForCalendar(java.lang.Boolean processForCalendar) {
this.processForCalendar = processForCalendar;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Process calendar invites in the email and add any extracted meetings to the Google Calendar for
this user.
*
*/
public boolean isProcessForCalendar() {
if (processForCalendar == null || processForCalendar == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return processForCalendar;
}
@Override
public GmailImport set(String parameterName, Object value) {
return (GmailImport) super.set(parameterName, value);
}
}
/**
* Directly inserts a message into only this user's mailbox similar to IMAP APPEND, bypassing most
* scanning and classification. Does not send a message.
*
* Create a request for the method "messages.insert".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message}
* @return the request
*/
public Insert insert(java.lang.String userId, com.google.api.services.gmail.model.Message content) throws java.io.IOException {
Insert result = new Insert(userId, content);
initialize(result);
return result;
}
/**
* Directly inserts a message into only this user's mailbox similar to IMAP APPEND, bypassing most
* scanning and classification. Does not send a message.
*
* Create a request for the method "messages.insert".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Insert insert(java.lang.String userId, com.google.api.services.gmail.model.Message content, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Insert result = new Insert(userId, content, mediaContent);
initialize(result);
return result;
}
public class Insert extends GmailRequest {
private static final String REST_PATH = "{userId}/messages";
/**
* Directly inserts a message into only this user's mailbox similar to IMAP APPEND, bypassing most
* scanning and classification. Does not send a message.
*
* Create a request for the method "messages.insert".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message}
* @since 1.13
*/
protected Insert(java.lang.String userId, com.google.api.services.gmail.model.Message content) {
super(Gmail.this, "POST", REST_PATH, content, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getRaw(), "Message.getRaw()");
}
/**
* Directly inserts a message into only this user's mailbox similar to IMAP APPEND, bypassing most
* scanning and classification. Does not send a message.
*
* Create a request for the method "messages.insert".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Insert#execute()} method to invoke the remote operation. {@link
* Insert#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Insert(java.lang.String userId, com.google.api.services.gmail.model.Message content, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(Gmail.this, "POST", "/upload/" + getServicePath() + REST_PATH, content, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Insert setAlt(java.lang.String alt) {
return (Insert) super.setAlt(alt);
}
@Override
public Insert setFields(java.lang.String fields) {
return (Insert) super.setFields(fields);
}
@Override
public Insert setKey(java.lang.String key) {
return (Insert) super.setKey(key);
}
@Override
public Insert setOauthToken(java.lang.String oauthToken) {
return (Insert) super.setOauthToken(oauthToken);
}
@Override
public Insert setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Insert) super.setPrettyPrint(prettyPrint);
}
@Override
public Insert setQuotaUser(java.lang.String quotaUser) {
return (Insert) super.setQuotaUser(quotaUser);
}
@Override
public Insert setUserIp(java.lang.String userIp) {
return (Insert) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Insert setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/**
* Mark the email as permanently deleted (not TRASH) and only visible in Google Apps Vault
* to a Vault administrator. Only used for Google Apps for Work accounts.
*/
@com.google.api.client.util.Key
private java.lang.Boolean deleted;
/** Mark the email as permanently deleted (not TRASH) and only visible in Google Apps Vault to a Vault
administrator. Only used for Google Apps for Work accounts. [default: false]
*/
public java.lang.Boolean getDeleted() {
return deleted;
}
/**
* Mark the email as permanently deleted (not TRASH) and only visible in Google Apps Vault
* to a Vault administrator. Only used for Google Apps for Work accounts.
*/
public Insert setDeleted(java.lang.Boolean deleted) {
this.deleted = deleted;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Mark the email as permanently deleted (not TRASH) and only visible in Google Apps Vault to a Vault
administrator. Only used for Google Apps for Work accounts.
*
*/
public boolean isDeleted() {
if (deleted == null || deleted == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return deleted;
}
/** Source for Gmail's internal date of the message. */
@com.google.api.client.util.Key
private java.lang.String internalDateSource;
/** Source for Gmail's internal date of the message. [default: receivedTime]
*/
public java.lang.String getInternalDateSource() {
return internalDateSource;
}
/** Source for Gmail's internal date of the message. */
public Insert setInternalDateSource(java.lang.String internalDateSource) {
this.internalDateSource = internalDateSource;
return this;
}
@Override
public Insert set(String parameterName, Object value) {
return (Insert) super.set(parameterName, value);
}
}
/**
* Lists the messages in the user's mailbox.
*
* Create a request for the method "messages.list".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @return the request
*/
public List list(java.lang.String userId) throws java.io.IOException {
List result = new List(userId);
initialize(result);
return result;
}
public class List extends GmailRequest {
private static final String REST_PATH = "{userId}/messages";
/**
* Lists the messages in the user's mailbox.
*
* Create a request for the method "messages.list".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @since 1.13
*/
protected List(java.lang.String userId) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.ListMessagesResponse.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** Include messages from SPAM and TRASH in the results. */
@com.google.api.client.util.Key
private java.lang.Boolean includeSpamTrash;
/** Include messages from SPAM and TRASH in the results. [default: false]
*/
public java.lang.Boolean getIncludeSpamTrash() {
return includeSpamTrash;
}
/** Include messages from SPAM and TRASH in the results. */
public List setIncludeSpamTrash(java.lang.Boolean includeSpamTrash) {
this.includeSpamTrash = includeSpamTrash;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Include messages from SPAM and TRASH in the results.
*
*/
public boolean isIncludeSpamTrash() {
if (includeSpamTrash == null || includeSpamTrash == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return includeSpamTrash;
}
/** Only return messages with labels that match all of the specified label IDs. */
@com.google.api.client.util.Key
private java.util.List labelIds;
/** Only return messages with labels that match all of the specified label IDs.
*/
public java.util.List getLabelIds() {
return labelIds;
}
/** Only return messages with labels that match all of the specified label IDs. */
public List setLabelIds(java.util.List labelIds) {
this.labelIds = labelIds;
return this;
}
/** Maximum number of messages to return. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of messages to return. [default: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of messages to return. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Page token to retrieve a specific page of results in the list. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Page token to retrieve a specific page of results in the list.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Page token to retrieve a specific page of results in the list. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Only return messages matching the specified query. Supports the same query format as the
* Gmail search box. For example, "from:[email protected] rfc822msgid: is:unread".
*/
@com.google.api.client.util.Key
private java.lang.String q;
/** Only return messages matching the specified query. Supports the same query format as the Gmail
search box. For example, "from:[email protected] rfc822msgid: is:unread".
*/
public java.lang.String getQ() {
return q;
}
/**
* Only return messages matching the specified query. Supports the same query format as the
* Gmail search box. For example, "from:[email protected] rfc822msgid: is:unread".
*/
public List setQ(java.lang.String q) {
this.q = q;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Modifies the labels on the specified message.
*
* Create a request for the method "messages.modify".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Modify#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to modify.
* @param content the {@link com.google.api.services.gmail.model.ModifyMessageRequest}
* @return the request
*/
public Modify modify(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.ModifyMessageRequest content) throws java.io.IOException {
Modify result = new Modify(userId, id, content);
initialize(result);
return result;
}
public class Modify extends GmailRequest {
private static final String REST_PATH = "{userId}/messages/{id}/modify";
/**
* Modifies the labels on the specified message.
*
* Create a request for the method "messages.modify".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Modify#execute()} method to invoke the remote operation. {@link
* Modify#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to modify.
* @param content the {@link com.google.api.services.gmail.model.ModifyMessageRequest}
* @since 1.13
*/
protected Modify(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.ModifyMessageRequest content) {
super(Gmail.this, "POST", REST_PATH, content, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Modify setAlt(java.lang.String alt) {
return (Modify) super.setAlt(alt);
}
@Override
public Modify setFields(java.lang.String fields) {
return (Modify) super.setFields(fields);
}
@Override
public Modify setKey(java.lang.String key) {
return (Modify) super.setKey(key);
}
@Override
public Modify setOauthToken(java.lang.String oauthToken) {
return (Modify) super.setOauthToken(oauthToken);
}
@Override
public Modify setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Modify) super.setPrettyPrint(prettyPrint);
}
@Override
public Modify setQuotaUser(java.lang.String quotaUser) {
return (Modify) super.setQuotaUser(quotaUser);
}
@Override
public Modify setUserIp(java.lang.String userIp) {
return (Modify) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Modify setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the message to modify. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the message to modify.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the message to modify. */
public Modify setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Modify set(String parameterName, Object value) {
return (Modify) super.set(parameterName, value);
}
}
/**
* Sends the specified message to the recipients in the To, Cc, and Bcc headers.
*
* Create a request for the method "messages.send".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Send#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message}
* @return the request
*/
public Send send(java.lang.String userId, com.google.api.services.gmail.model.Message content) throws java.io.IOException {
Send result = new Send(userId, content);
initialize(result);
return result;
}
/**
* Sends the specified message to the recipients in the To, Cc, and Bcc headers.
*
* Create a request for the method "messages.send".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Send#execute()} method to invoke the remote operation.
*
*
* This method should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @return the request
* @throws java.io.IOException if the initialization of the request fails
*/
public Send send(java.lang.String userId, com.google.api.services.gmail.model.Message content, com.google.api.client.http.AbstractInputStreamContent mediaContent) throws java.io.IOException {
Send result = new Send(userId, content, mediaContent);
initialize(result);
return result;
}
public class Send extends GmailRequest {
private static final String REST_PATH = "{userId}/messages/send";
/**
* Sends the specified message to the recipients in the To, Cc, and Bcc headers.
*
* Create a request for the method "messages.send".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Send#execute()} method to invoke the remote operation. {@link
* Send#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message}
* @since 1.13
*/
protected Send(java.lang.String userId, com.google.api.services.gmail.model.Message content) {
super(Gmail.this, "POST", REST_PATH, content, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
checkRequiredParameter(content, "content");
checkRequiredParameter(content.getRaw(), "Message.getRaw()");
}
/**
* Sends the specified message to the recipients in the To, Cc, and Bcc headers.
*
* Create a request for the method "messages.send".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Send#execute()} method to invoke the remote operation. {@link
* Send#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
*
* This constructor should be used for uploading media content.
*
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param content the {@link com.google.api.services.gmail.model.Message} media metadata or {@code null} if none
* @param mediaContent The media HTTP content or {@code null} if none.
* @since 1.13
*/
protected Send(java.lang.String userId, com.google.api.services.gmail.model.Message content, com.google.api.client.http.AbstractInputStreamContent mediaContent) {
super(Gmail.this, "POST", "/upload/" + getServicePath() + REST_PATH, content, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
initializeMediaUpload(mediaContent);
}
@Override
public Send setAlt(java.lang.String alt) {
return (Send) super.setAlt(alt);
}
@Override
public Send setFields(java.lang.String fields) {
return (Send) super.setFields(fields);
}
@Override
public Send setKey(java.lang.String key) {
return (Send) super.setKey(key);
}
@Override
public Send setOauthToken(java.lang.String oauthToken) {
return (Send) super.setOauthToken(oauthToken);
}
@Override
public Send setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Send) super.setPrettyPrint(prettyPrint);
}
@Override
public Send setQuotaUser(java.lang.String quotaUser) {
return (Send) super.setQuotaUser(quotaUser);
}
@Override
public Send setUserIp(java.lang.String userIp) {
return (Send) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Send setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
@Override
public Send set(String parameterName, Object value) {
return (Send) super.set(parameterName, value);
}
}
/**
* Moves the specified message to the trash.
*
* Create a request for the method "messages.trash".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Trash#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to Trash.
* @return the request
*/
public Trash trash(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Trash result = new Trash(userId, id);
initialize(result);
return result;
}
public class Trash extends GmailRequest {
private static final String REST_PATH = "{userId}/messages/{id}/trash";
/**
* Moves the specified message to the trash.
*
* Create a request for the method "messages.trash".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Trash#execute()} method to invoke the remote operation. {@link
* Trash#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to Trash.
* @since 1.13
*/
protected Trash(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "POST", REST_PATH, null, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Trash setAlt(java.lang.String alt) {
return (Trash) super.setAlt(alt);
}
@Override
public Trash setFields(java.lang.String fields) {
return (Trash) super.setFields(fields);
}
@Override
public Trash setKey(java.lang.String key) {
return (Trash) super.setKey(key);
}
@Override
public Trash setOauthToken(java.lang.String oauthToken) {
return (Trash) super.setOauthToken(oauthToken);
}
@Override
public Trash setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Trash) super.setPrettyPrint(prettyPrint);
}
@Override
public Trash setQuotaUser(java.lang.String quotaUser) {
return (Trash) super.setQuotaUser(quotaUser);
}
@Override
public Trash setUserIp(java.lang.String userIp) {
return (Trash) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Trash setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the message to Trash. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the message to Trash.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the message to Trash. */
public Trash setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Trash set(String parameterName, Object value) {
return (Trash) super.set(parameterName, value);
}
}
/**
* Removes the specified message from the trash.
*
* Create a request for the method "messages.untrash".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Untrash#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to remove from Trash.
* @return the request
*/
public Untrash untrash(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Untrash result = new Untrash(userId, id);
initialize(result);
return result;
}
public class Untrash extends GmailRequest {
private static final String REST_PATH = "{userId}/messages/{id}/untrash";
/**
* Removes the specified message from the trash.
*
* Create a request for the method "messages.untrash".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Untrash#execute()} method to invoke the remote operation.
* {@link
* Untrash#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the message to remove from Trash.
* @since 1.13
*/
protected Untrash(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "POST", REST_PATH, null, com.google.api.services.gmail.model.Message.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Untrash setAlt(java.lang.String alt) {
return (Untrash) super.setAlt(alt);
}
@Override
public Untrash setFields(java.lang.String fields) {
return (Untrash) super.setFields(fields);
}
@Override
public Untrash setKey(java.lang.String key) {
return (Untrash) super.setKey(key);
}
@Override
public Untrash setOauthToken(java.lang.String oauthToken) {
return (Untrash) super.setOauthToken(oauthToken);
}
@Override
public Untrash setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Untrash) super.setPrettyPrint(prettyPrint);
}
@Override
public Untrash setQuotaUser(java.lang.String quotaUser) {
return (Untrash) super.setQuotaUser(quotaUser);
}
@Override
public Untrash setUserIp(java.lang.String userIp) {
return (Untrash) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Untrash setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the message to remove from Trash. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the message to remove from Trash.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the message to remove from Trash. */
public Untrash setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Untrash set(String parameterName, Object value) {
return (Untrash) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Attachments collection.
*
* The typical use is:
*
* {@code Gmail gmail = new Gmail(...);}
* {@code Gmail.Attachments.List request = gmail.attachments().list(parameters ...)}
*
*
* @return the resource collection
*/
public Attachments attachments() {
return new Attachments();
}
/**
* The "attachments" collection of methods.
*/
public class Attachments {
/**
* Gets the specified message attachment.
*
* Create a request for the method "attachments.get".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param messageId The ID of the message containing the attachment.
* @param id The ID of the attachment.
* @return the request
*/
public Get get(java.lang.String userId, java.lang.String messageId, java.lang.String id) throws java.io.IOException {
Get result = new Get(userId, messageId, id);
initialize(result);
return result;
}
public class Get extends GmailRequest {
private static final String REST_PATH = "{userId}/messages/{messageId}/attachments/{id}";
/**
* Gets the specified message attachment.
*
* Create a request for the method "attachments.get".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param messageId The ID of the message containing the attachment.
* @param id The ID of the attachment.
* @since 1.13
*/
protected Get(java.lang.String userId, java.lang.String messageId, java.lang.String id) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.MessagePartBody.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.messageId = com.google.api.client.util.Preconditions.checkNotNull(messageId, "Required parameter messageId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the
* authenticated user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the
* authenticated user.
*/
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the message containing the attachment. */
@com.google.api.client.util.Key
private java.lang.String messageId;
/** The ID of the message containing the attachment.
*/
public java.lang.String getMessageId() {
return messageId;
}
/** The ID of the message containing the attachment. */
public Get setMessageId(java.lang.String messageId) {
this.messageId = messageId;
return this;
}
/** The ID of the attachment. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the attachment.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the attachment. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the Threads collection.
*
* The typical use is:
*
* {@code Gmail gmail = new Gmail(...);}
* {@code Gmail.Threads.List request = gmail.threads().list(parameters ...)}
*
*
* @return the resource collection
*/
public Threads threads() {
return new Threads();
}
/**
* The "threads" collection of methods.
*/
public class Threads {
/**
* Immediately and permanently deletes the specified thread. This operation cannot be undone. Prefer
* threads.trash instead.
*
* Create a request for the method "threads.delete".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id ID of the Thread to delete.
* @return the request
*/
public Delete delete(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Delete result = new Delete(userId, id);
initialize(result);
return result;
}
public class Delete extends GmailRequest {
private static final String REST_PATH = "{userId}/threads/{id}";
/**
* Immediately and permanently deletes the specified thread. This operation cannot be undone.
* Prefer threads.trash instead.
*
* Create a request for the method "threads.delete".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id ID of the Thread to delete.
* @since 1.13
*/
protected Delete(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "DELETE", REST_PATH, null, Void.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUserIp(java.lang.String userIp) {
return (Delete) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Delete setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** ID of the Thread to delete. */
@com.google.api.client.util.Key
private java.lang.String id;
/** ID of the Thread to delete.
*/
public java.lang.String getId() {
return id;
}
/** ID of the Thread to delete. */
public Delete setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets the specified thread.
*
* Create a request for the method "threads.get".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the thread to retrieve.
* @return the request
*/
public Get get(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Get result = new Get(userId, id);
initialize(result);
return result;
}
public class Get extends GmailRequest {
private static final String REST_PATH = "{userId}/threads/{id}";
/**
* Gets the specified thread.
*
* Create a request for the method "threads.get".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the thread to retrieve.
* @since 1.13
*/
protected Get(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.Thread.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUserIp(java.lang.String userIp) {
return (Get) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Get setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the thread to retrieve. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the thread to retrieve.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the thread to retrieve. */
public Get setId(java.lang.String id) {
this.id = id;
return this;
}
/** The format to return the messages in. */
@com.google.api.client.util.Key
private java.lang.String format;
/** The format to return the messages in. [default: full]
*/
public java.lang.String getFormat() {
return format;
}
/** The format to return the messages in. */
public Get setFormat(java.lang.String format) {
this.format = format;
return this;
}
/** When given and format is METADATA, only include headers specified. */
@com.google.api.client.util.Key
private java.util.List metadataHeaders;
/** When given and format is METADATA, only include headers specified.
*/
public java.util.List getMetadataHeaders() {
return metadataHeaders;
}
/** When given and format is METADATA, only include headers specified. */
public Get setMetadataHeaders(java.util.List metadataHeaders) {
this.metadataHeaders = metadataHeaders;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the threads in the user's mailbox.
*
* Create a request for the method "threads.list".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @return the request
*/
public List list(java.lang.String userId) throws java.io.IOException {
List result = new List(userId);
initialize(result);
return result;
}
public class List extends GmailRequest {
private static final String REST_PATH = "{userId}/threads";
/**
* Lists the threads in the user's mailbox.
*
* Create a request for the method "threads.list".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @since 1.13
*/
protected List(java.lang.String userId) {
super(Gmail.this, "GET", REST_PATH, null, com.google.api.services.gmail.model.ListThreadsResponse.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUserIp(java.lang.String userIp) {
return (List) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public List setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** Include threads from SPAM and TRASH in the results. */
@com.google.api.client.util.Key
private java.lang.Boolean includeSpamTrash;
/** Include threads from SPAM and TRASH in the results. [default: false]
*/
public java.lang.Boolean getIncludeSpamTrash() {
return includeSpamTrash;
}
/** Include threads from SPAM and TRASH in the results. */
public List setIncludeSpamTrash(java.lang.Boolean includeSpamTrash) {
this.includeSpamTrash = includeSpamTrash;
return this;
}
/**
* Convenience method that returns only {@link Boolean#TRUE} or {@link Boolean#FALSE}.
*
*
* Boolean properties can have four possible values:
* {@code null}, {@link com.google.api.client.util.Data#NULL_BOOLEAN}, {@link Boolean#TRUE}
* or {@link Boolean#FALSE}.
*
*
*
* This method returns {@link Boolean#TRUE} if the default of the property is {@link Boolean#TRUE}
* and it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
* {@link Boolean#FALSE} is returned if the default of the property is {@link Boolean#FALSE} and
* it is {@code null} or {@link com.google.api.client.util.Data#NULL_BOOLEAN}.
*
*
*
* Include threads from SPAM and TRASH in the results.
*
*/
public boolean isIncludeSpamTrash() {
if (includeSpamTrash == null || includeSpamTrash == com.google.api.client.util.Data.NULL_BOOLEAN) {
return false;
}
return includeSpamTrash;
}
/** Only return threads with labels that match all of the specified label IDs. */
@com.google.api.client.util.Key
private java.util.List labelIds;
/** Only return threads with labels that match all of the specified label IDs.
*/
public java.util.List getLabelIds() {
return labelIds;
}
/** Only return threads with labels that match all of the specified label IDs. */
public List setLabelIds(java.util.List labelIds) {
this.labelIds = labelIds;
return this;
}
/** Maximum number of threads to return. */
@com.google.api.client.util.Key
private java.lang.Long maxResults;
/** Maximum number of threads to return. [default: 100]
*/
public java.lang.Long getMaxResults() {
return maxResults;
}
/** Maximum number of threads to return. */
public List setMaxResults(java.lang.Long maxResults) {
this.maxResults = maxResults;
return this;
}
/** Page token to retrieve a specific page of results in the list. */
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** Page token to retrieve a specific page of results in the list.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/** Page token to retrieve a specific page of results in the list. */
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
/**
* Only return threads matching the specified query. Supports the same query format as the
* Gmail search box. For example, "from:[email protected] rfc822msgid: is:unread".
*/
@com.google.api.client.util.Key
private java.lang.String q;
/** Only return threads matching the specified query. Supports the same query format as the Gmail
search box. For example, "from:[email protected] rfc822msgid: is:unread".
*/
public java.lang.String getQ() {
return q;
}
/**
* Only return threads matching the specified query. Supports the same query format as the
* Gmail search box. For example, "from:[email protected] rfc822msgid: is:unread".
*/
public List setQ(java.lang.String q) {
this.q = q;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Modifies the labels applied to the thread. This applies to all messages in the thread.
*
* Create a request for the method "threads.modify".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Modify#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the thread to modify.
* @param content the {@link com.google.api.services.gmail.model.ModifyThreadRequest}
* @return the request
*/
public Modify modify(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.ModifyThreadRequest content) throws java.io.IOException {
Modify result = new Modify(userId, id, content);
initialize(result);
return result;
}
public class Modify extends GmailRequest {
private static final String REST_PATH = "{userId}/threads/{id}/modify";
/**
* Modifies the labels applied to the thread. This applies to all messages in the thread.
*
* Create a request for the method "threads.modify".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Modify#execute()} method to invoke the remote operation. {@link
* Modify#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the thread to modify.
* @param content the {@link com.google.api.services.gmail.model.ModifyThreadRequest}
* @since 1.13
*/
protected Modify(java.lang.String userId, java.lang.String id, com.google.api.services.gmail.model.ModifyThreadRequest content) {
super(Gmail.this, "POST", REST_PATH, content, com.google.api.services.gmail.model.Thread.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Modify setAlt(java.lang.String alt) {
return (Modify) super.setAlt(alt);
}
@Override
public Modify setFields(java.lang.String fields) {
return (Modify) super.setFields(fields);
}
@Override
public Modify setKey(java.lang.String key) {
return (Modify) super.setKey(key);
}
@Override
public Modify setOauthToken(java.lang.String oauthToken) {
return (Modify) super.setOauthToken(oauthToken);
}
@Override
public Modify setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Modify) super.setPrettyPrint(prettyPrint);
}
@Override
public Modify setQuotaUser(java.lang.String quotaUser) {
return (Modify) super.setQuotaUser(quotaUser);
}
@Override
public Modify setUserIp(java.lang.String userIp) {
return (Modify) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Modify setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the thread to modify. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the thread to modify.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the thread to modify. */
public Modify setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Modify set(String parameterName, Object value) {
return (Modify) super.set(parameterName, value);
}
}
/**
* Moves the specified thread to the trash.
*
* Create a request for the method "threads.trash".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Trash#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the thread to Trash.
* @return the request
*/
public Trash trash(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Trash result = new Trash(userId, id);
initialize(result);
return result;
}
public class Trash extends GmailRequest {
private static final String REST_PATH = "{userId}/threads/{id}/trash";
/**
* Moves the specified thread to the trash.
*
* Create a request for the method "threads.trash".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Trash#execute()} method to invoke the remote operation. {@link
* Trash#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the thread to Trash.
* @since 1.13
*/
protected Trash(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "POST", REST_PATH, null, com.google.api.services.gmail.model.Thread.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Trash setAlt(java.lang.String alt) {
return (Trash) super.setAlt(alt);
}
@Override
public Trash setFields(java.lang.String fields) {
return (Trash) super.setFields(fields);
}
@Override
public Trash setKey(java.lang.String key) {
return (Trash) super.setKey(key);
}
@Override
public Trash setOauthToken(java.lang.String oauthToken) {
return (Trash) super.setOauthToken(oauthToken);
}
@Override
public Trash setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Trash) super.setPrettyPrint(prettyPrint);
}
@Override
public Trash setQuotaUser(java.lang.String quotaUser) {
return (Trash) super.setQuotaUser(quotaUser);
}
@Override
public Trash setUserIp(java.lang.String userIp) {
return (Trash) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Trash setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the thread to Trash. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the thread to Trash.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the thread to Trash. */
public Trash setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Trash set(String parameterName, Object value) {
return (Trash) super.set(parameterName, value);
}
}
/**
* Removes the specified thread from the trash.
*
* Create a request for the method "threads.untrash".
*
* This request holds the parameters needed by the gmail server. After setting any optional
* parameters, call the {@link Untrash#execute()} method to invoke the remote operation.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the thread to remove from Trash.
* @return the request
*/
public Untrash untrash(java.lang.String userId, java.lang.String id) throws java.io.IOException {
Untrash result = new Untrash(userId, id);
initialize(result);
return result;
}
public class Untrash extends GmailRequest {
private static final String REST_PATH = "{userId}/threads/{id}/untrash";
/**
* Removes the specified thread from the trash.
*
* Create a request for the method "threads.untrash".
*
* This request holds the parameters needed by the the gmail server. After setting any optional
* parameters, call the {@link Untrash#execute()} method to invoke the remote operation.
* {@link
* Untrash#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param userId The user's email address. The special value me can be used to indicate the authenticated user.
* [default: me]
* @param id The ID of the thread to remove from Trash.
* @since 1.13
*/
protected Untrash(java.lang.String userId, java.lang.String id) {
super(Gmail.this, "POST", REST_PATH, null, com.google.api.services.gmail.model.Thread.class);
this.userId = com.google.api.client.util.Preconditions.checkNotNull(userId, "Required parameter userId must be specified.");
this.id = com.google.api.client.util.Preconditions.checkNotNull(id, "Required parameter id must be specified.");
}
@Override
public Untrash setAlt(java.lang.String alt) {
return (Untrash) super.setAlt(alt);
}
@Override
public Untrash setFields(java.lang.String fields) {
return (Untrash) super.setFields(fields);
}
@Override
public Untrash setKey(java.lang.String key) {
return (Untrash) super.setKey(key);
}
@Override
public Untrash setOauthToken(java.lang.String oauthToken) {
return (Untrash) super.setOauthToken(oauthToken);
}
@Override
public Untrash setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Untrash) super.setPrettyPrint(prettyPrint);
}
@Override
public Untrash setQuotaUser(java.lang.String quotaUser) {
return (Untrash) super.setQuotaUser(quotaUser);
}
@Override
public Untrash setUserIp(java.lang.String userIp) {
return (Untrash) super.setUserIp(userIp);
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
@com.google.api.client.util.Key
private java.lang.String userId;
/** The user's email address. The special value me can be used to indicate the authenticated user.
[default: me]
*/
public java.lang.String getUserId() {
return userId;
}
/**
* The user's email address. The special value me can be used to indicate the authenticated
* user.
*/
public Untrash setUserId(java.lang.String userId) {
this.userId = userId;
return this;
}
/** The ID of the thread to remove from Trash. */
@com.google.api.client.util.Key
private java.lang.String id;
/** The ID of the thread to remove from Trash.
*/
public java.lang.String getId() {
return id;
}
/** The ID of the thread to remove from Trash. */
public Untrash setId(java.lang.String id) {
this.id = id;
return this;
}
@Override
public Untrash set(String parameterName, Object value) {
return (Untrash) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link Gmail}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
DEFAULT_ROOT_URL,
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
}
/** Builds a new instance of {@link Gmail}. */
@Override
public Gmail build() {
return new Gmail(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link GmailRequestInitializer}.
*
* @since 1.12
*/
public Builder setGmailRequestInitializer(
GmailRequestInitializer gmailRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(gmailRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
}
}