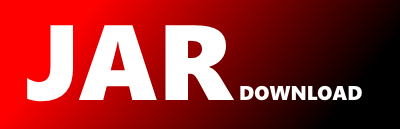
target.apidocs.com.google.api.services.healthcare.v1.CloudHealthcare.Projects.Locations.Datasets.html Maven / Gradle / Ivy
CloudHealthcare.Projects.Locations.Datasets (Cloud Healthcare API v1-rev20241205-2.0.0)
com.google.api.services.healthcare.v1
Class CloudHealthcare.Projects.Locations.Datasets
- java.lang.Object
-
- com.google.api.services.healthcare.v1.CloudHealthcare.Projects.Locations.Datasets
-
- Enclosing class:
- CloudHealthcare.Projects.Locations
public class CloudHealthcare.Projects.Locations.Datasets
extends Object
The "datasets" collection of methods.
-
-
Nested Class Summary
-
Constructor Summary
Constructors
Constructor and Description
Datasets()
-
Method Summary
-
-
Method Detail
-
create
public CloudHealthcare.Projects.Locations.Datasets.Create create(String parent,
Dataset content)
throws IOException
Creates a new health dataset. Results are returned through the Operation interface which returns
either an `Operation.response` which contains a Dataset or `Operation.error`. The metadata field
type is OperationMetadata.
Create a request for the method "datasets.create".
This request holds the parameters needed by the healthcare server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
parent
- Required. The name of the project where the server creates the dataset. For example,
`projects/{project_id}/locations/{location_id}`.
content
- the Dataset
- Returns:
- the request
- Throws:
IOException
-
deidentify
public CloudHealthcare.Projects.Locations.Datasets.Deidentify deidentify(String sourceDataset,
DeidentifyDatasetRequest content)
throws IOException
Creates a new dataset containing de-identified data from the source dataset. The metadata field
type is OperationMetadata. If the request is successful, the response field type is
DeidentifySummary. If errors occur, error is set. The LRO result may still be successful if de-
identification fails for some DICOM instances. The new de-identified dataset will not contain
these failed resources. Failed resource totals are tracked in Operation.metadata. Error details
are also logged to Cloud Logging. For more information, see [Viewing error logs in Cloud
Logging](https://cloud.google.com/healthcare/docs/how-tos/logging).
Create a request for the method "datasets.deidentify".
This request holds the parameters needed by the healthcare server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
sourceDataset
- Required. Source dataset resource name. For example,
`projects/{project_id}/locations/{location_id}/datasets/{dataset_id}`.
content
- the DeidentifyDatasetRequest
- Returns:
- the request
- Throws:
IOException
-
delete
public CloudHealthcare.Projects.Locations.Datasets.Delete delete(String name)
throws IOException
Deletes the specified health dataset and all data contained in the dataset. Deleting a dataset
does not affect the sources from which the dataset was imported (if any).
Create a request for the method "datasets.delete".
This request holds the parameters needed by the healthcare server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Required. The name of the dataset to delete. For example,
`projects/{project_id}/locations/{location_id}/datasets/{dataset_id}`.
- Returns:
- the request
- Throws:
IOException
-
get
public CloudHealthcare.Projects.Locations.Datasets.Get get(String name)
throws IOException
Gets any metadata associated with a dataset.
Create a request for the method "datasets.get".
This request holds the parameters needed by the healthcare server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Required. The name of the dataset to read. For example,
`projects/{project_id}/locations/{location_id}/datasets/{dataset_id}`.
- Returns:
- the request
- Throws:
IOException
-
getIamPolicy
public CloudHealthcare.Projects.Locations.Datasets.GetIamPolicy getIamPolicy(String resource)
throws IOException
Gets the access control policy for a resource. Returns an empty policy if the resource exists and
does not have a policy set.
Create a request for the method "datasets.getIamPolicy".
This request holds the parameters needed by the healthcare server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
resource
- REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
this field.
- Returns:
- the request
- Throws:
IOException
-
list
public CloudHealthcare.Projects.Locations.Datasets.List list(String parent)
throws IOException
Lists the health datasets in the current project.
Create a request for the method "datasets.list".
This request holds the parameters needed by the healthcare server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
parent
- Required. The name of the project whose datasets should be listed. For example,
`projects/{project_id}/locations/{location_id}`.
- Returns:
- the request
- Throws:
IOException
-
patch
public CloudHealthcare.Projects.Locations.Datasets.Patch patch(String name,
Dataset content)
throws IOException
Updates dataset metadata.
Create a request for the method "datasets.patch".
This request holds the parameters needed by the healthcare server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
name
- Identifier. Resource name of the dataset, of the form
`projects/{project_id}/locations/{location_id}/datasets/{dataset_id}`.
content
- the Dataset
- Returns:
- the request
- Throws:
IOException
-
setIamPolicy
public CloudHealthcare.Projects.Locations.Datasets.SetIamPolicy setIamPolicy(String resource,
SetIamPolicyRequest content)
throws IOException
Sets the access control policy on the specified resource. Replaces any existing policy. Can
return `NOT_FOUND`, `INVALID_ARGUMENT`, and `PERMISSION_DENIED` errors.
Create a request for the method "datasets.setIamPolicy".
This request holds the parameters needed by the healthcare server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
resource
- REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
this field.
content
- the SetIamPolicyRequest
- Returns:
- the request
- Throws:
IOException
-
testIamPermissions
public CloudHealthcare.Projects.Locations.Datasets.TestIamPermissions testIamPermissions(String resource,
TestIamPermissionsRequest content)
throws IOException
Returns permissions that a caller has on the specified resource. If the resource does not exist,
this will return an empty set of permissions, not a `NOT_FOUND` error. Note: This operation is
designed to be used for building permission-aware UIs and command-line tools, not for
authorization checking. This operation may "fail open" without warning.
Create a request for the method "datasets.testIamPermissions".
This request holds the parameters needed by the healthcare server. After setting any optional
parameters, call the AbstractGoogleClientRequest.execute()
method to invoke the remote operation.
- Parameters:
resource
- REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
this field.
content
- the TestIamPermissionsRequest
- Returns:
- the request
- Throws:
IOException
-
consentStores
public CloudHealthcare.Projects.Locations.Datasets.ConsentStores consentStores()
An accessor for creating requests from the ConsentStores collection.
The typical use is:
CloudHealthcare healthcare = new CloudHealthcare(...);
CloudHealthcare.ConsentStores.List request = healthcare.consentStores().list(parameters ...)
- Returns:
- the resource collection
-
dataMapperWorkspaces
public CloudHealthcare.Projects.Locations.Datasets.DataMapperWorkspaces dataMapperWorkspaces()
An accessor for creating requests from the DataMapperWorkspaces collection.
The typical use is:
CloudHealthcare healthcare = new CloudHealthcare(...);
CloudHealthcare.DataMapperWorkspaces.List request = healthcare.dataMapperWorkspaces().list(parameters ...)
- Returns:
- the resource collection
-
dicomStores
public CloudHealthcare.Projects.Locations.Datasets.DicomStores dicomStores()
An accessor for creating requests from the DicomStores collection.
The typical use is:
CloudHealthcare healthcare = new CloudHealthcare(...);
CloudHealthcare.DicomStores.List request = healthcare.dicomStores().list(parameters ...)
- Returns:
- the resource collection
-
fhirStores
public CloudHealthcare.Projects.Locations.Datasets.FhirStores fhirStores()
An accessor for creating requests from the FhirStores collection.
The typical use is:
CloudHealthcare healthcare = new CloudHealthcare(...);
CloudHealthcare.FhirStores.List request = healthcare.fhirStores().list(parameters ...)
- Returns:
- the resource collection
-
hl7V2Stores
public CloudHealthcare.Projects.Locations.Datasets.Hl7V2Stores hl7V2Stores()
An accessor for creating requests from the Hl7V2Stores collection.
The typical use is:
CloudHealthcare healthcare = new CloudHealthcare(...);
CloudHealthcare.Hl7V2Stores.List request = healthcare.hl7V2Stores().list(parameters ...)
- Returns:
- the resource collection
-
operations
public CloudHealthcare.Projects.Locations.Datasets.Operations operations()
An accessor for creating requests from the Operations collection.
The typical use is:
CloudHealthcare healthcare = new CloudHealthcare(...);
CloudHealthcare.Operations.List request = healthcare.operations().list(parameters ...)
- Returns:
- the resource collection
Copyright © 2011–2024 Google. All rights reserved.
© 2015 - 2025 Weber Informatics LLC | Privacy Policy