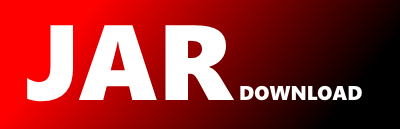
com.google.api.services.iam.v2.Iam Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.iam.v2;
/**
* Service definition for Iam (v2).
*
*
* Manages identity and access control for Google Cloud resources, including the creation of service accounts, which you can use to authenticate to Google and make API calls. Enabling this API also enables the IAM Service Account Credentials API (iamcredentials.googleapis.com). However, disabling this API doesn't disable the IAM Service Account Credentials API.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link IamRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class Iam extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Identity and Access Management (IAM) API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://iam.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://iam.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Iam(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
Iam(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Policies collection.
*
* The typical use is:
*
* {@code Iam iam = new Iam(...);}
* {@code Iam.Policies.List request = iam.policies().list(parameters ...)}
*
*
* @return the resource collection
*/
public Policies policies() {
return new Policies();
}
/**
* The "policies" collection of methods.
*/
public class Policies {
/**
* Creates a policy.
*
* Create a request for the method "policies.createPolicy".
*
* This request holds the parameters needed by the iam server. After setting any optional
* parameters, call the {@link CreatePolicy#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource that the policy is attached to, along with the kind of policy to create.
* Format: `policies/{attachment_point}/denypolicies` The attachment point is identified by
* its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies`. For
* organizations and folders, use the numeric ID in the full resource name. For projects, you
* can use the alphanumeric or the numeric ID.
* @param content the {@link com.google.api.services.iam.v2.model.GoogleIamV2Policy}
* @return the request
*/
public CreatePolicy createPolicy(java.lang.String parent, com.google.api.services.iam.v2.model.GoogleIamV2Policy content) throws java.io.IOException {
CreatePolicy result = new CreatePolicy(parent, content);
initialize(result);
return result;
}
public class CreatePolicy extends IamRequest {
private static final String REST_PATH = "v2/{+parent}";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^policies/[^/]+/[^/]+$");
/**
* Creates a policy.
*
* Create a request for the method "policies.createPolicy".
*
* This request holds the parameters needed by the the iam server. After setting any optional
* parameters, call the {@link CreatePolicy#execute()} method to invoke the remote operation.
* {@link
* CreatePolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource that the policy is attached to, along with the kind of policy to create.
* Format: `policies/{attachment_point}/denypolicies` The attachment point is identified by
* its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies`. For
* organizations and folders, use the numeric ID in the full resource name. For projects, you
* can use the alphanumeric or the numeric ID.
* @param content the {@link com.google.api.services.iam.v2.model.GoogleIamV2Policy}
* @since 1.13
*/
protected CreatePolicy(java.lang.String parent, com.google.api.services.iam.v2.model.GoogleIamV2Policy content) {
super(Iam.this, "POST", REST_PATH, content, com.google.api.services.iam.v2.model.GoogleLongrunningOperation.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^policies/[^/]+/[^/]+$");
}
}
@Override
public CreatePolicy set$Xgafv(java.lang.String $Xgafv) {
return (CreatePolicy) super.set$Xgafv($Xgafv);
}
@Override
public CreatePolicy setAccessToken(java.lang.String accessToken) {
return (CreatePolicy) super.setAccessToken(accessToken);
}
@Override
public CreatePolicy setAlt(java.lang.String alt) {
return (CreatePolicy) super.setAlt(alt);
}
@Override
public CreatePolicy setCallback(java.lang.String callback) {
return (CreatePolicy) super.setCallback(callback);
}
@Override
public CreatePolicy setFields(java.lang.String fields) {
return (CreatePolicy) super.setFields(fields);
}
@Override
public CreatePolicy setKey(java.lang.String key) {
return (CreatePolicy) super.setKey(key);
}
@Override
public CreatePolicy setOauthToken(java.lang.String oauthToken) {
return (CreatePolicy) super.setOauthToken(oauthToken);
}
@Override
public CreatePolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (CreatePolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public CreatePolicy setQuotaUser(java.lang.String quotaUser) {
return (CreatePolicy) super.setQuotaUser(quotaUser);
}
@Override
public CreatePolicy setUploadType(java.lang.String uploadType) {
return (CreatePolicy) super.setUploadType(uploadType);
}
@Override
public CreatePolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (CreatePolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource that the policy is attached to, along with the kind of policy to
* create. Format: `policies/{attachment_point}/denypolicies` The attachment point is
* identified by its URL-encoded full resource name, which means that the forward-slash
* character, `/`, must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies`. For
* organizations and folders, use the numeric ID in the full resource name. For projects, you
* can use the alphanumeric or the numeric ID.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource that the policy is attached to, along with the kind of policy to create.
Format: `policies/{attachment_point}/denypolicies` The attachment point is identified by its URL-
encoded full resource name, which means that the forward-slash character, `/`, must be written as
`%2F`. For example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
project/denypolicies`. For organizations and folders, use the numeric ID in the full resource name.
For projects, you can use the alphanumeric or the numeric ID.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource that the policy is attached to, along with the kind of policy to
* create. Format: `policies/{attachment_point}/denypolicies` The attachment point is
* identified by its URL-encoded full resource name, which means that the forward-slash
* character, `/`, must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies`. For
* organizations and folders, use the numeric ID in the full resource name. For projects, you
* can use the alphanumeric or the numeric ID.
*/
public CreatePolicy setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^policies/[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The ID to use for this policy, which will become the final component of the policy's
* resource name. The ID must contain 3 to 63 characters. It can contain lowercase letters and
* numbers, as well as dashes (`-`) and periods (`.`). The first character must be a lowercase
* letter.
*/
@com.google.api.client.util.Key
private java.lang.String policyId;
/** The ID to use for this policy, which will become the final component of the policy's resource name.
The ID must contain 3 to 63 characters. It can contain lowercase letters and numbers, as well as
dashes (`-`) and periods (`.`). The first character must be a lowercase letter.
*/
public java.lang.String getPolicyId() {
return policyId;
}
/**
* The ID to use for this policy, which will become the final component of the policy's
* resource name. The ID must contain 3 to 63 characters. It can contain lowercase letters and
* numbers, as well as dashes (`-`) and periods (`.`). The first character must be a lowercase
* letter.
*/
public CreatePolicy setPolicyId(java.lang.String policyId) {
this.policyId = policyId;
return this;
}
@Override
public CreatePolicy set(String parameterName, Object value) {
return (CreatePolicy) super.set(parameterName, value);
}
}
/**
* Deletes a policy. This action is permanent.
*
* Create a request for the method "policies.delete".
*
* This request holds the parameters needed by the iam server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the policy to delete. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource
* name, which means that the forward-slash character, `/`, must be written as `%2F`. For
* example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
* project/denypolicies/my-policy`. For organizations and folders, use the numeric ID in the
* full resource name. For projects, you can use the alphanumeric or the numeric ID.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends IamRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^policies/[^/]+/[^/]+/[^/]+$");
/**
* Deletes a policy. This action is permanent.
*
* Create a request for the method "policies.delete".
*
* This request holds the parameters needed by the the iam server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the policy to delete. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource
* name, which means that the forward-slash character, `/`, must be written as `%2F`. For
* example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
* project/denypolicies/my-policy`. For organizations and folders, use the numeric ID in the
* full resource name. For projects, you can use the alphanumeric or the numeric ID.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(Iam.this, "DELETE", REST_PATH, null, com.google.api.services.iam.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^policies/[^/]+/[^/]+/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the policy to delete. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource
* name, which means that the forward-slash character, `/`, must be written as `%2F`. For
* example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
* project/denypolicies/my-policy`. For organizations and folders, use the numeric ID in the
* full resource name. For projects, you can use the alphanumeric or the numeric ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the policy to delete. Format:
`policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource name,
which means that the forward-slash character, `/`, must be written as `%2F`. For example,
`policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies/my-policy`. For
organizations and folders, use the numeric ID in the full resource name. For projects, you can use
the alphanumeric or the numeric ID.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the policy to delete. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource
* name, which means that the forward-slash character, `/`, must be written as `%2F`. For
* example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
* project/denypolicies/my-policy`. For organizations and folders, use the numeric ID in the
* full resource name. For projects, you can use the alphanumeric or the numeric ID.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^policies/[^/]+/[^/]+/[^/]+$");
}
this.name = name;
return this;
}
/**
* Optional. The expected `etag` of the policy to delete. If the value does not match the
* value that is stored in IAM, the request fails with a `409` error code and `ABORTED`
* status. If you omit this field, the policy is deleted regardless of its current `etag`.
*/
@com.google.api.client.util.Key
private java.lang.String etag;
/** Optional. The expected `etag` of the policy to delete. If the value does not match the value that
is stored in IAM, the request fails with a `409` error code and `ABORTED` status. If you omit this
field, the policy is deleted regardless of its current `etag`.
*/
public java.lang.String getEtag() {
return etag;
}
/**
* Optional. The expected `etag` of the policy to delete. If the value does not match the
* value that is stored in IAM, the request fails with a `409` error code and `ABORTED`
* status. If you omit this field, the policy is deleted regardless of its current `etag`.
*/
public Delete setEtag(java.lang.String etag) {
this.etag = etag;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Gets a policy.
*
* Create a request for the method "policies.get".
*
* This request holds the parameters needed by the iam server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the policy to retrieve. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource
* name, which means that the forward-slash character, `/`, must be written as `%2F`. For
* example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
* project/denypolicies/my-policy`. For organizations and folders, use the numeric ID in the
* full resource name. For projects, you can use the alphanumeric or the numeric ID.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends IamRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^policies/[^/]+/[^/]+/[^/]+$");
/**
* Gets a policy.
*
* Create a request for the method "policies.get".
*
* This request holds the parameters needed by the the iam server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. The resource name of the policy to retrieve. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource
* name, which means that the forward-slash character, `/`, must be written as `%2F`. For
* example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
* project/denypolicies/my-policy`. For organizations and folders, use the numeric ID in the
* full resource name. For projects, you can use the alphanumeric or the numeric ID.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Iam.this, "GET", REST_PATH, null, com.google.api.services.iam.v2.model.GoogleIamV2Policy.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^policies/[^/]+/[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name of the policy to retrieve. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource
* name, which means that the forward-slash character, `/`, must be written as `%2F`. For
* example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
* project/denypolicies/my-policy`. For organizations and folders, use the numeric ID in the
* full resource name. For projects, you can use the alphanumeric or the numeric ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the policy to retrieve. Format:
`policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource name,
which means that the forward-slash character, `/`, must be written as `%2F`. For example,
`policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies/my-policy`. For
organizations and folders, use the numeric ID in the full resource name. For projects, you can use
the alphanumeric or the numeric ID.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name of the policy to retrieve. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` Use the URL-encoded full resource
* name, which means that the forward-slash character, `/`, must be written as `%2F`. For
* example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
* project/denypolicies/my-policy`. For organizations and folders, use the numeric ID in the
* full resource name. For projects, you can use the alphanumeric or the numeric ID.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^policies/[^/]+/[^/]+/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Retrieves the policies of the specified kind that are attached to a resource. The response lists
* only policy metadata. In particular, policy rules are omitted.
*
* Create a request for the method "policies.listPolicies".
*
* This request holds the parameters needed by the iam server. After setting any optional
* parameters, call the {@link ListPolicies#execute()} method to invoke the remote operation.
*
* @param parent Required. The resource that the policy is attached to, along with the kind of policy to list.
* Format: `policies/{attachment_point}/denypolicies` The attachment point is identified by
* its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies`. For
* organizations and folders, use the numeric ID in the full resource name. For projects, you
* can use the alphanumeric or the numeric ID.
* @return the request
*/
public ListPolicies listPolicies(java.lang.String parent) throws java.io.IOException {
ListPolicies result = new ListPolicies(parent);
initialize(result);
return result;
}
public class ListPolicies extends IamRequest {
private static final String REST_PATH = "v2/{+parent}";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^policies/[^/]+/[^/]+$");
/**
* Retrieves the policies of the specified kind that are attached to a resource. The response
* lists only policy metadata. In particular, policy rules are omitted.
*
* Create a request for the method "policies.listPolicies".
*
* This request holds the parameters needed by the the iam server. After setting any optional
* parameters, call the {@link ListPolicies#execute()} method to invoke the remote operation.
* {@link
* ListPolicies#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. The resource that the policy is attached to, along with the kind of policy to list.
* Format: `policies/{attachment_point}/denypolicies` The attachment point is identified by
* its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies`. For
* organizations and folders, use the numeric ID in the full resource name. For projects, you
* can use the alphanumeric or the numeric ID.
* @since 1.13
*/
protected ListPolicies(java.lang.String parent) {
super(Iam.this, "GET", REST_PATH, null, com.google.api.services.iam.v2.model.GoogleIamV2ListPoliciesResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^policies/[^/]+/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public ListPolicies set$Xgafv(java.lang.String $Xgafv) {
return (ListPolicies) super.set$Xgafv($Xgafv);
}
@Override
public ListPolicies setAccessToken(java.lang.String accessToken) {
return (ListPolicies) super.setAccessToken(accessToken);
}
@Override
public ListPolicies setAlt(java.lang.String alt) {
return (ListPolicies) super.setAlt(alt);
}
@Override
public ListPolicies setCallback(java.lang.String callback) {
return (ListPolicies) super.setCallback(callback);
}
@Override
public ListPolicies setFields(java.lang.String fields) {
return (ListPolicies) super.setFields(fields);
}
@Override
public ListPolicies setKey(java.lang.String key) {
return (ListPolicies) super.setKey(key);
}
@Override
public ListPolicies setOauthToken(java.lang.String oauthToken) {
return (ListPolicies) super.setOauthToken(oauthToken);
}
@Override
public ListPolicies setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ListPolicies) super.setPrettyPrint(prettyPrint);
}
@Override
public ListPolicies setQuotaUser(java.lang.String quotaUser) {
return (ListPolicies) super.setQuotaUser(quotaUser);
}
@Override
public ListPolicies setUploadType(java.lang.String uploadType) {
return (ListPolicies) super.setUploadType(uploadType);
}
@Override
public ListPolicies setUploadProtocol(java.lang.String uploadProtocol) {
return (ListPolicies) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource that the policy is attached to, along with the kind of policy to
* list. Format: `policies/{attachment_point}/denypolicies` The attachment point is identified
* by its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies`. For
* organizations and folders, use the numeric ID in the full resource name. For projects, you
* can use the alphanumeric or the numeric ID.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. The resource that the policy is attached to, along with the kind of policy to list.
Format: `policies/{attachment_point}/denypolicies` The attachment point is identified by its URL-
encoded full resource name, which means that the forward-slash character, `/`, must be written as
`%2F`. For example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
project/denypolicies`. For organizations and folders, use the numeric ID in the full resource name.
For projects, you can use the alphanumeric or the numeric ID.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. The resource that the policy is attached to, along with the kind of policy to
* list. Format: `policies/{attachment_point}/denypolicies` The attachment point is identified
* by its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies`. For
* organizations and folders, use the numeric ID in the full resource name. For projects, you
* can use the alphanumeric or the numeric ID.
*/
public ListPolicies setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^policies/[^/]+/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of policies to return. IAM ignores this value and uses the value 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of policies to return. IAM ignores this value and uses the value 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of policies to return. IAM ignores this value and uses the value 1000.
*/
public ListPolicies setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token received in a ListPoliciesResponse. Provide this token to retrieve the next
* page.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token received in a ListPoliciesResponse. Provide this token to retrieve the next page.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token received in a ListPoliciesResponse. Provide this token to retrieve the next
* page.
*/
public ListPolicies setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public ListPolicies set(String parameterName, Object value) {
return (ListPolicies) super.set(parameterName, value);
}
}
/**
* Updates the specified policy. You can update only the rules and the display name for the policy.
* To update a policy, you should use a read-modify-write loop: 1. Use GetPolicy to read the current
* version of the policy. 2. Modify the policy as needed. 3. Use `UpdatePolicy` to write the updated
* policy. This pattern helps prevent conflicts between concurrent updates.
*
* Create a request for the method "policies.update".
*
* This request holds the parameters needed by the iam server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation.
*
* @param name Immutable. The resource name of the `Policy`, which must be unique. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` The attachment point is identified
* by its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies/my-
* deny-policy`. For organizations and folders, use the numeric ID in the full resource name.
* For projects, requests can use the alphanumeric or the numeric ID. Responses always
* contain the numeric ID.
* @param content the {@link com.google.api.services.iam.v2.model.GoogleIamV2Policy}
* @return the request
*/
public Update update(java.lang.String name, com.google.api.services.iam.v2.model.GoogleIamV2Policy content) throws java.io.IOException {
Update result = new Update(name, content);
initialize(result);
return result;
}
public class Update extends IamRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^policies/[^/]+/[^/]+/[^/]+$");
/**
* Updates the specified policy. You can update only the rules and the display name for the
* policy. To update a policy, you should use a read-modify-write loop: 1. Use GetPolicy to read
* the current version of the policy. 2. Modify the policy as needed. 3. Use `UpdatePolicy` to
* write the updated policy. This pattern helps prevent conflicts between concurrent updates.
*
* Create a request for the method "policies.update".
*
* This request holds the parameters needed by the the iam server. After setting any optional
* parameters, call the {@link Update#execute()} method to invoke the remote operation. {@link
* Update#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Immutable. The resource name of the `Policy`, which must be unique. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` The attachment point is identified
* by its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies/my-
* deny-policy`. For organizations and folders, use the numeric ID in the full resource name.
* For projects, requests can use the alphanumeric or the numeric ID. Responses always
* contain the numeric ID.
* @param content the {@link com.google.api.services.iam.v2.model.GoogleIamV2Policy}
* @since 1.13
*/
protected Update(java.lang.String name, com.google.api.services.iam.v2.model.GoogleIamV2Policy content) {
super(Iam.this, "PUT", REST_PATH, content, com.google.api.services.iam.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^policies/[^/]+/[^/]+/[^/]+$");
}
}
@Override
public Update set$Xgafv(java.lang.String $Xgafv) {
return (Update) super.set$Xgafv($Xgafv);
}
@Override
public Update setAccessToken(java.lang.String accessToken) {
return (Update) super.setAccessToken(accessToken);
}
@Override
public Update setAlt(java.lang.String alt) {
return (Update) super.setAlt(alt);
}
@Override
public Update setCallback(java.lang.String callback) {
return (Update) super.setCallback(callback);
}
@Override
public Update setFields(java.lang.String fields) {
return (Update) super.setFields(fields);
}
@Override
public Update setKey(java.lang.String key) {
return (Update) super.setKey(key);
}
@Override
public Update setOauthToken(java.lang.String oauthToken) {
return (Update) super.setOauthToken(oauthToken);
}
@Override
public Update setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Update) super.setPrettyPrint(prettyPrint);
}
@Override
public Update setQuotaUser(java.lang.String quotaUser) {
return (Update) super.setQuotaUser(quotaUser);
}
@Override
public Update setUploadType(java.lang.String uploadType) {
return (Update) super.setUploadType(uploadType);
}
@Override
public Update setUploadProtocol(java.lang.String uploadProtocol) {
return (Update) super.setUploadProtocol(uploadProtocol);
}
/**
* Immutable. The resource name of the `Policy`, which must be unique. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` The attachment point is identified
* by its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies/my-deny-
* policy`. For organizations and folders, use the numeric ID in the full resource name. For
* projects, requests can use the alphanumeric or the numeric ID. Responses always contain the
* numeric ID.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Immutable. The resource name of the `Policy`, which must be unique. Format:
`policies/{attachment_point}/denypolicies/{policy_id}` The attachment point is identified by its
URL-encoded full resource name, which means that the forward-slash character, `/`, must be written
as `%2F`. For example, `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-
project/denypolicies/my-deny-policy`. For organizations and folders, use the numeric ID in the full
resource name. For projects, requests can use the alphanumeric or the numeric ID. Responses always
contain the numeric ID.
*/
public java.lang.String getName() {
return name;
}
/**
* Immutable. The resource name of the `Policy`, which must be unique. Format:
* `policies/{attachment_point}/denypolicies/{policy_id}` The attachment point is identified
* by its URL-encoded full resource name, which means that the forward-slash character, `/`,
* must be written as `%2F`. For example,
* `policies/cloudresourcemanager.googleapis.com%2Fprojects%2Fmy-project/denypolicies/my-deny-
* policy`. For organizations and folders, use the numeric ID in the full resource name. For
* projects, requests can use the alphanumeric or the numeric ID. Responses always contain the
* numeric ID.
*/
public Update setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^policies/[^/]+/[^/]+/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Update set(String parameterName, Object value) {
return (Update) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the Operations collection.
*
* The typical use is:
*
* {@code Iam iam = new Iam(...);}
* {@code Iam.Operations.List request = iam.operations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Operations operations() {
return new Operations();
}
/**
* The "operations" collection of methods.
*/
public class Operations {
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the iam server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name The name of the operation resource.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends IamRequest {
private static final String REST_PATH = "v2/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^policies/[^/]+/[^/]+/[^/]+/operations/[^/]+$");
/**
* Gets the latest state of a long-running operation. Clients can use this method to poll the
* operation result at intervals as recommended by the API service.
*
* Create a request for the method "operations.get".
*
* This request holds the parameters needed by the the iam server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name The name of the operation resource.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(Iam.this, "GET", REST_PATH, null, com.google.api.services.iam.v2.model.GoogleLongrunningOperation.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^policies/[^/]+/[^/]+/[^/]+/operations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/** The name of the operation resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** The name of the operation resource.
*/
public java.lang.String getName() {
return name;
}
/** The name of the operation resource. */
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^policies/[^/]+/[^/]+/[^/]+/operations/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
}
}
/**
* Builder for {@link Iam}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link Iam}. */
@Override
public Iam build() {
return new Iam(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link IamRequestInitializer}.
*
* @since 1.12
*/
public Builder setIamRequestInitializer(
IamRequestInitializer iamRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(iamRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}