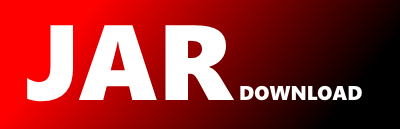
com.google.api.services.iap.v1.CloudIAP Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.iap.v1;
/**
* Service definition for CloudIAP (v1).
*
*
* Controls access to cloud applications running on Google Cloud Platform.
*
*
*
* For more information about this service, see the
* API Documentation
*
*
*
* This service uses {@link CloudIAPRequestInitializer} to initialize global parameters via its
* {@link Builder}.
*
*
* @since 1.3
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public class CloudIAP extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient {
// Note: Leave this static initializer at the top of the file.
static {
com.google.api.client.util.Preconditions.checkState(
(com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION == 1 &&
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION >= 32 ||
(com.google.api.client.googleapis.GoogleUtils.MINOR_VERSION == 31 &&
com.google.api.client.googleapis.GoogleUtils.BUGFIX_VERSION >= 1))) ||
com.google.api.client.googleapis.GoogleUtils.MAJOR_VERSION >= 2,
"You are currently running with version %s of google-api-client. " +
"You need at least version 1.31.1 of google-api-client to run version " +
"2.0.0 of the Cloud Identity-Aware Proxy API library.", com.google.api.client.googleapis.GoogleUtils.VERSION);
}
/**
* The default encoded root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_ROOT_URL = "https://iap.googleapis.com/";
/**
* The default encoded mTLS root URL of the service. This is determined when the library is generated
* and normally should not be changed.
*
* @since 1.31
*/
public static final String DEFAULT_MTLS_ROOT_URL = "https://iap.mtls.googleapis.com/";
/**
* The default encoded service path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.7
*/
public static final String DEFAULT_SERVICE_PATH = "";
/**
* The default encoded batch path of the service. This is determined when the library is
* generated and normally should not be changed.
*
* @since 1.23
*/
public static final String DEFAULT_BATCH_PATH = "batch";
/**
* The default encoded base URL of the service. This is determined when the library is generated
* and normally should not be changed.
*/
public static final String DEFAULT_BASE_URL = DEFAULT_ROOT_URL + DEFAULT_SERVICE_PATH;
/**
* Constructor.
*
*
* Use {@link Builder} if you need to specify any of the optional parameters.
*
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public CloudIAP(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
this(new Builder(transport, jsonFactory, httpRequestInitializer));
}
/**
* @param builder builder
*/
CloudIAP(Builder builder) {
super(builder);
}
@Override
protected void initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest> httpClientRequest) throws java.io.IOException {
super.initialize(httpClientRequest);
}
/**
* An accessor for creating requests from the Projects collection.
*
* The typical use is:
*
* {@code CloudIAP iap = new CloudIAP(...);}
* {@code CloudIAP.Projects.List request = iap.projects().list(parameters ...)}
*
*
* @return the resource collection
*/
public Projects projects() {
return new Projects();
}
/**
* The "projects" collection of methods.
*/
public class Projects {
/**
* An accessor for creating requests from the Brands collection.
*
* The typical use is:
*
* {@code CloudIAP iap = new CloudIAP(...);}
* {@code CloudIAP.Brands.List request = iap.brands().list(parameters ...)}
*
*
* @return the resource collection
*/
public Brands brands() {
return new Brands();
}
/**
* The "brands" collection of methods.
*/
public class Brands {
/**
* Constructs a new OAuth brand for the project if one does not exist. The created brand is
* "internal only", meaning that OAuth clients created under it only accept requests from users who
* belong to the same Google Workspace organization as the project. The brand is created in an un-
* reviewed status. NOTE: The "internal only" status can be manually changed in the Google Cloud
* Console. Requires that a brand does not already exist for the project, and that the specified
* support email is owned by the caller.
*
* Create a request for the method "brands.create".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. GCP Project number/id under which the brand is to be created. In the following format:
* projects/{project_number/id}.
* @param content the {@link com.google.api.services.iap.v1.model.Brand}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.iap.v1.model.Brand content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+parent}/brands";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Constructs a new OAuth brand for the project if one does not exist. The created brand is
* "internal only", meaning that OAuth clients created under it only accept requests from users
* who belong to the same Google Workspace organization as the project. The brand is created in an
* un-reviewed status. NOTE: The "internal only" status can be manually changed in the Google
* Cloud Console. Requires that a brand does not already exist for the project, and that the
* specified support email is owned by the caller.
*
* Create a request for the method "brands.create".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. GCP Project number/id under which the brand is to be created. In the following format:
* projects/{project_number/id}.
* @param content the {@link com.google.api.services.iap.v1.model.Brand}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.iap.v1.model.Brand content) {
super(CloudIAP.this, "POST", REST_PATH, content, com.google.api.services.iap.v1.model.Brand.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. GCP Project number/id under which the brand is to be created. In the following
* format: projects/{project_number/id}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. GCP Project number/id under which the brand is to be created. In the following format:
projects/{project_number/id}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. GCP Project number/id under which the brand is to be created. In the following
* format: projects/{project_number/id}.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Retrieves the OAuth brand of the project.
*
* Create a request for the method "brands.get".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the brand to be fetched. In the following format:
* projects/{project_number/id}/brands/{brand}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/brands/[^/]+$");
/**
* Retrieves the OAuth brand of the project.
*
* Create a request for the method "brands.get".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the brand to be fetched. In the following format:
* projects/{project_number/id}/brands/{brand}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIAP.this, "GET", REST_PATH, null, com.google.api.services.iap.v1.model.Brand.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the brand to be fetched. In the following format:
* projects/{project_number/id}/brands/{brand}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the brand to be fetched. In the following format:
projects/{project_number/id}/brands/{brand}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the brand to be fetched. In the following format:
* projects/{project_number/id}/brands/{brand}.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the existing brands for the project.
*
* Create a request for the method "brands.list".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. GCP Project number/id. In the following format: projects/{project_number/id}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+parent}/brands";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+$");
/**
* Lists the existing brands for the project.
*
* Create a request for the method "brands.list".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. GCP Project number/id. In the following format: projects/{project_number/id}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudIAP.this, "GET", REST_PATH, null, com.google.api.services.iap.v1.model.ListBrandsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. GCP Project number/id. In the following format: projects/{project_number/id}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. GCP Project number/id. In the following format: projects/{project_number/id}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. GCP Project number/id. In the following format: projects/{project_number/id}.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* An accessor for creating requests from the IdentityAwareProxyClients collection.
*
* The typical use is:
*
* {@code CloudIAP iap = new CloudIAP(...);}
* {@code CloudIAP.IdentityAwareProxyClients.List request = iap.identityAwareProxyClients().list(parameters ...)}
*
*
* @return the resource collection
*/
public IdentityAwareProxyClients identityAwareProxyClients() {
return new IdentityAwareProxyClients();
}
/**
* The "identityAwareProxyClients" collection of methods.
*/
public class IdentityAwareProxyClients {
/**
* Creates an Identity Aware Proxy (IAP) OAuth client. The client is owned by IAP. Requires that the
* brand for the project exists and that it is set for internal-only use.
*
* Create a request for the method "identityAwareProxyClients.create".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Path to create the client in. In the following format:
* projects/{project_number/id}/brands/{brand}. The project must belong to a G Suite account.
* @param content the {@link com.google.api.services.iap.v1.model.IdentityAwareProxyClient}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.iap.v1.model.IdentityAwareProxyClient content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+parent}/identityAwareProxyClients";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/brands/[^/]+$");
/**
* Creates an Identity Aware Proxy (IAP) OAuth client. The client is owned by IAP. Requires that
* the brand for the project exists and that it is set for internal-only use.
*
* Create a request for the method "identityAwareProxyClients.create".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Path to create the client in. In the following format:
* projects/{project_number/id}/brands/{brand}. The project must belong to a G Suite account.
* @param content the {@link com.google.api.services.iap.v1.model.IdentityAwareProxyClient}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.iap.v1.model.IdentityAwareProxyClient content) {
super(CloudIAP.this, "POST", REST_PATH, content, com.google.api.services.iap.v1.model.IdentityAwareProxyClient.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Path to create the client in. In the following format:
* projects/{project_number/id}/brands/{brand}. The project must belong to a G Suite
* account.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Path to create the client in. In the following format:
projects/{project_number/id}/brands/{brand}. The project must belong to a G Suite account.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Path to create the client in. In the following format:
* projects/{project_number/id}/brands/{brand}. The project must belong to a G Suite
* account.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+$");
}
this.parent = parent;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes an Identity Aware Proxy (IAP) OAuth client. Useful for removing obsolete clients,
* managing the number of clients in a given project, and cleaning up after tests. Requires that the
* client is owned by IAP.
*
* Create a request for the method "identityAwareProxyClients.delete".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the Identity Aware Proxy client to be deleted. In the following format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/brands/[^/]+/identityAwareProxyClients/[^/]+$");
/**
* Deletes an Identity Aware Proxy (IAP) OAuth client. Useful for removing obsolete clients,
* managing the number of clients in a given project, and cleaning up after tests. Requires that
* the client is owned by IAP.
*
* Create a request for the method "identityAwareProxyClients.delete".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the Identity Aware Proxy client to be deleted. In the following format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIAP.this, "DELETE", REST_PATH, null, com.google.api.services.iap.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+/identityAwareProxyClients/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the Identity Aware Proxy client to be deleted. In the following
* format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the Identity Aware Proxy client to be deleted. In the following format:
projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the Identity Aware Proxy client to be deleted. In the following
* format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+/identityAwareProxyClients/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves an Identity Aware Proxy (IAP) OAuth client. Requires that the client is owned by IAP.
*
* Create a request for the method "identityAwareProxyClients.get".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the Identity Aware Proxy client to be fetched. In the following format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/brands/[^/]+/identityAwareProxyClients/[^/]+$");
/**
* Retrieves an Identity Aware Proxy (IAP) OAuth client. Requires that the client is owned by IAP.
*
* Create a request for the method "identityAwareProxyClients.get".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the Identity Aware Proxy client to be fetched. In the following format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIAP.this, "GET", REST_PATH, null, com.google.api.services.iap.v1.model.IdentityAwareProxyClient.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+/identityAwareProxyClients/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the Identity Aware Proxy client to be fetched. In the following
* format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the Identity Aware Proxy client to be fetched. In the following format:
projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the Identity Aware Proxy client to be fetched. In the following
* format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+/identityAwareProxyClients/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the existing clients for the brand.
*
* Create a request for the method "identityAwareProxyClients.list".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Full brand path. In the following format: projects/{project_number/id}/brands/{brand}.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+parent}/identityAwareProxyClients";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/brands/[^/]+$");
/**
* Lists the existing clients for the brand.
*
* Create a request for the method "identityAwareProxyClients.list".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Full brand path. In the following format: projects/{project_number/id}/brands/{brand}.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudIAP.this, "GET", REST_PATH, null, com.google.api.services.iap.v1.model.ListIdentityAwareProxyClientsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Full brand path. In the following format:
* projects/{project_number/id}/brands/{brand}.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Full brand path. In the following format: projects/{project_number/id}/brands/{brand}.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Full brand path. In the following format:
* projects/{project_number/id}/brands/{brand}.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of clients to return. The service may return fewer than this value.
* If unspecified, at most 100 clients will be returned. The maximum value is 1000; values
* above 1000 will be coerced to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of clients to return. The service may return fewer than this value. If
unspecified, at most 100 clients will be returned. The maximum value is 1000; values above 1000
will be coerced to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of clients to return. The service may return fewer than this value.
* If unspecified, at most 100 clients will be returned. The maximum value is 1000; values
* above 1000 will be coerced to 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListIdentityAwareProxyClients` call. Provide
* this to retrieve the subsequent page. When paginating, all other parameters provided to
* `ListIdentityAwareProxyClients` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListIdentityAwareProxyClients` call. Provide this to
retrieve the subsequent page. When paginating, all other parameters provided to
`ListIdentityAwareProxyClients` must match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListIdentityAwareProxyClients` call. Provide
* this to retrieve the subsequent page. When paginating, all other parameters provided to
* `ListIdentityAwareProxyClients` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Resets an Identity Aware Proxy (IAP) OAuth client secret. Useful if the secret was compromised.
* Requires that the client is owned by IAP.
*
* Create a request for the method "identityAwareProxyClients.resetSecret".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link ResetSecret#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the Identity Aware Proxy client to that will have its secret reset. In the
* following format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
* @param content the {@link com.google.api.services.iap.v1.model.ResetIdentityAwareProxyClientSecretRequest}
* @return the request
*/
public ResetSecret resetSecret(java.lang.String name, com.google.api.services.iap.v1.model.ResetIdentityAwareProxyClientSecretRequest content) throws java.io.IOException {
ResetSecret result = new ResetSecret(name, content);
initialize(result);
return result;
}
public class ResetSecret extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}:resetSecret";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/brands/[^/]+/identityAwareProxyClients/[^/]+$");
/**
* Resets an Identity Aware Proxy (IAP) OAuth client secret. Useful if the secret was compromised.
* Requires that the client is owned by IAP.
*
* Create a request for the method "identityAwareProxyClients.resetSecret".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link ResetSecret#execute()} method to invoke the remote operation.
* {@link
* ResetSecret#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the Identity Aware Proxy client to that will have its secret reset. In the
* following format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
* @param content the {@link com.google.api.services.iap.v1.model.ResetIdentityAwareProxyClientSecretRequest}
* @since 1.13
*/
protected ResetSecret(java.lang.String name, com.google.api.services.iap.v1.model.ResetIdentityAwareProxyClientSecretRequest content) {
super(CloudIAP.this, "POST", REST_PATH, content, com.google.api.services.iap.v1.model.IdentityAwareProxyClient.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+/identityAwareProxyClients/[^/]+$");
}
}
@Override
public ResetSecret set$Xgafv(java.lang.String $Xgafv) {
return (ResetSecret) super.set$Xgafv($Xgafv);
}
@Override
public ResetSecret setAccessToken(java.lang.String accessToken) {
return (ResetSecret) super.setAccessToken(accessToken);
}
@Override
public ResetSecret setAlt(java.lang.String alt) {
return (ResetSecret) super.setAlt(alt);
}
@Override
public ResetSecret setCallback(java.lang.String callback) {
return (ResetSecret) super.setCallback(callback);
}
@Override
public ResetSecret setFields(java.lang.String fields) {
return (ResetSecret) super.setFields(fields);
}
@Override
public ResetSecret setKey(java.lang.String key) {
return (ResetSecret) super.setKey(key);
}
@Override
public ResetSecret setOauthToken(java.lang.String oauthToken) {
return (ResetSecret) super.setOauthToken(oauthToken);
}
@Override
public ResetSecret setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ResetSecret) super.setPrettyPrint(prettyPrint);
}
@Override
public ResetSecret setQuotaUser(java.lang.String quotaUser) {
return (ResetSecret) super.setQuotaUser(quotaUser);
}
@Override
public ResetSecret setUploadType(java.lang.String uploadType) {
return (ResetSecret) super.setUploadType(uploadType);
}
@Override
public ResetSecret setUploadProtocol(java.lang.String uploadProtocol) {
return (ResetSecret) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the Identity Aware Proxy client to that will have its secret reset.
* In the following format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the Identity Aware Proxy client to that will have its secret reset. In the
following format:
projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the Identity Aware Proxy client to that will have its secret reset.
* In the following format:
* projects/{project_number/id}/brands/{brand}/identityAwareProxyClients/{client_id}.
*/
public ResetSecret setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/brands/[^/]+/identityAwareProxyClients/[^/]+$");
}
this.name = name;
return this;
}
@Override
public ResetSecret set(String parameterName, Object value) {
return (ResetSecret) super.set(parameterName, value);
}
}
}
}
/**
* An accessor for creating requests from the IapTunnel collection.
*
* The typical use is:
*
* {@code CloudIAP iap = new CloudIAP(...);}
* {@code CloudIAP.IapTunnel.List request = iap.iapTunnel().list(parameters ...)}
*
*
* @return the resource collection
*/
public IapTunnel iapTunnel() {
return new IapTunnel();
}
/**
* The "iap_tunnel" collection of methods.
*/
public class IapTunnel {
/**
* An accessor for creating requests from the Locations collection.
*
* The typical use is:
*
* {@code CloudIAP iap = new CloudIAP(...);}
* {@code CloudIAP.Locations.List request = iap.locations().list(parameters ...)}
*
*
* @return the resource collection
*/
public Locations locations() {
return new Locations();
}
/**
* The "locations" collection of methods.
*/
public class Locations {
/**
* An accessor for creating requests from the DestGroups collection.
*
* The typical use is:
*
* {@code CloudIAP iap = new CloudIAP(...);}
* {@code CloudIAP.DestGroups.List request = iap.destGroups().list(parameters ...)}
*
*
* @return the resource collection
*/
public DestGroups destGroups() {
return new DestGroups();
}
/**
* The "destGroups" collection of methods.
*/
public class DestGroups {
/**
* Creates a new TunnelDestGroup.
*
* Create a request for the method "destGroups.create".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation.
*
* @param parent Required. Google Cloud Project ID and location. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}`.
* @param content the {@link com.google.api.services.iap.v1.model.TunnelDestGroup}
* @return the request
*/
public Create create(java.lang.String parent, com.google.api.services.iap.v1.model.TunnelDestGroup content) throws java.io.IOException {
Create result = new Create(parent, content);
initialize(result);
return result;
}
public class Create extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+parent}/destGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/iap_tunnel/locations/[^/]+$");
/**
* Creates a new TunnelDestGroup.
*
* Create a request for the method "destGroups.create".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link Create#execute()} method to invoke the remote operation. {@link
* Create#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Google Cloud Project ID and location. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}`.
* @param content the {@link com.google.api.services.iap.v1.model.TunnelDestGroup}
* @since 1.13
*/
protected Create(java.lang.String parent, com.google.api.services.iap.v1.model.TunnelDestGroup content) {
super(CloudIAP.this, "POST", REST_PATH, content, com.google.api.services.iap.v1.model.TunnelDestGroup.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+$");
}
}
@Override
public Create set$Xgafv(java.lang.String $Xgafv) {
return (Create) super.set$Xgafv($Xgafv);
}
@Override
public Create setAccessToken(java.lang.String accessToken) {
return (Create) super.setAccessToken(accessToken);
}
@Override
public Create setAlt(java.lang.String alt) {
return (Create) super.setAlt(alt);
}
@Override
public Create setCallback(java.lang.String callback) {
return (Create) super.setCallback(callback);
}
@Override
public Create setFields(java.lang.String fields) {
return (Create) super.setFields(fields);
}
@Override
public Create setKey(java.lang.String key) {
return (Create) super.setKey(key);
}
@Override
public Create setOauthToken(java.lang.String oauthToken) {
return (Create) super.setOauthToken(oauthToken);
}
@Override
public Create setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Create) super.setPrettyPrint(prettyPrint);
}
@Override
public Create setQuotaUser(java.lang.String quotaUser) {
return (Create) super.setQuotaUser(quotaUser);
}
@Override
public Create setUploadType(java.lang.String uploadType) {
return (Create) super.setUploadType(uploadType);
}
@Override
public Create setUploadProtocol(java.lang.String uploadProtocol) {
return (Create) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Google Cloud Project ID and location. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}`.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Google Cloud Project ID and location. In the following format:
`projects/{project_number/id}/iap_tunnel/locations/{location}`.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Google Cloud Project ID and location. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}`.
*/
public Create setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* Required. The ID to use for the TunnelDestGroup, which becomes the final component of
* the resource name. This value must be 4-63 characters, and valid characters are
* `[a-z]-`.
*/
@com.google.api.client.util.Key
private java.lang.String tunnelDestGroupId;
/** Required. The ID to use for the TunnelDestGroup, which becomes the final component of the resource
name. This value must be 4-63 characters, and valid characters are `[a-z]-`.
*/
public java.lang.String getTunnelDestGroupId() {
return tunnelDestGroupId;
}
/**
* Required. The ID to use for the TunnelDestGroup, which becomes the final component of
* the resource name. This value must be 4-63 characters, and valid characters are
* `[a-z]-`.
*/
public Create setTunnelDestGroupId(java.lang.String tunnelDestGroupId) {
this.tunnelDestGroupId = tunnelDestGroupId;
return this;
}
@Override
public Create set(String parameterName, Object value) {
return (Create) super.set(parameterName, value);
}
}
/**
* Deletes a TunnelDestGroup.
*
* Create a request for the method "destGroups.delete".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the TunnelDestGroup to delete. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
* @return the request
*/
public Delete delete(java.lang.String name) throws java.io.IOException {
Delete result = new Delete(name);
initialize(result);
return result;
}
public class Delete extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/iap_tunnel/locations/[^/]+/destGroups/[^/]+$");
/**
* Deletes a TunnelDestGroup.
*
* Create a request for the method "destGroups.delete".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link Delete#execute()} method to invoke the remote operation. {@link
* Delete#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the TunnelDestGroup to delete. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
* @since 1.13
*/
protected Delete(java.lang.String name) {
super(CloudIAP.this, "DELETE", REST_PATH, null, com.google.api.services.iap.v1.model.Empty.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+/destGroups/[^/]+$");
}
}
@Override
public Delete set$Xgafv(java.lang.String $Xgafv) {
return (Delete) super.set$Xgafv($Xgafv);
}
@Override
public Delete setAccessToken(java.lang.String accessToken) {
return (Delete) super.setAccessToken(accessToken);
}
@Override
public Delete setAlt(java.lang.String alt) {
return (Delete) super.setAlt(alt);
}
@Override
public Delete setCallback(java.lang.String callback) {
return (Delete) super.setCallback(callback);
}
@Override
public Delete setFields(java.lang.String fields) {
return (Delete) super.setFields(fields);
}
@Override
public Delete setKey(java.lang.String key) {
return (Delete) super.setKey(key);
}
@Override
public Delete setOauthToken(java.lang.String oauthToken) {
return (Delete) super.setOauthToken(oauthToken);
}
@Override
public Delete setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Delete) super.setPrettyPrint(prettyPrint);
}
@Override
public Delete setQuotaUser(java.lang.String quotaUser) {
return (Delete) super.setQuotaUser(quotaUser);
}
@Override
public Delete setUploadType(java.lang.String uploadType) {
return (Delete) super.setUploadType(uploadType);
}
@Override
public Delete setUploadProtocol(java.lang.String uploadProtocol) {
return (Delete) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the TunnelDestGroup to delete. In the following format: `projects/{
* project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the TunnelDestGroup to delete. In the following format:
`projects/{project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the TunnelDestGroup to delete. In the following format: `projects/{
* project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
*/
public Delete setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+/destGroups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Delete set(String parameterName, Object value) {
return (Delete) super.set(parameterName, value);
}
}
/**
* Retrieves an existing TunnelDestGroup.
*
* Create a request for the method "destGroups.get".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation.
*
* @param name Required. Name of the TunnelDestGroup to be fetched. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
* @return the request
*/
public Get get(java.lang.String name) throws java.io.IOException {
Get result = new Get(name);
initialize(result);
return result;
}
public class Get extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/iap_tunnel/locations/[^/]+/destGroups/[^/]+$");
/**
* Retrieves an existing TunnelDestGroup.
*
* Create a request for the method "destGroups.get".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link Get#execute()} method to invoke the remote operation. {@link
* Get#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param name Required. Name of the TunnelDestGroup to be fetched. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
* @since 1.13
*/
protected Get(java.lang.String name) {
super(CloudIAP.this, "GET", REST_PATH, null, com.google.api.services.iap.v1.model.TunnelDestGroup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+/destGroups/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public Get set$Xgafv(java.lang.String $Xgafv) {
return (Get) super.set$Xgafv($Xgafv);
}
@Override
public Get setAccessToken(java.lang.String accessToken) {
return (Get) super.setAccessToken(accessToken);
}
@Override
public Get setAlt(java.lang.String alt) {
return (Get) super.setAlt(alt);
}
@Override
public Get setCallback(java.lang.String callback) {
return (Get) super.setCallback(callback);
}
@Override
public Get setFields(java.lang.String fields) {
return (Get) super.setFields(fields);
}
@Override
public Get setKey(java.lang.String key) {
return (Get) super.setKey(key);
}
@Override
public Get setOauthToken(java.lang.String oauthToken) {
return (Get) super.setOauthToken(oauthToken);
}
@Override
public Get setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Get) super.setPrettyPrint(prettyPrint);
}
@Override
public Get setQuotaUser(java.lang.String quotaUser) {
return (Get) super.setQuotaUser(quotaUser);
}
@Override
public Get setUploadType(java.lang.String uploadType) {
return (Get) super.setUploadType(uploadType);
}
@Override
public Get setUploadProtocol(java.lang.String uploadProtocol) {
return (Get) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Name of the TunnelDestGroup to be fetched. In the following format: `projec
* ts/{project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. Name of the TunnelDestGroup to be fetched. In the following format:
`projects/{project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. Name of the TunnelDestGroup to be fetched. In the following format: `projec
* ts/{project_number/id}/iap_tunnel/locations/{location}/destGroups/{dest_group}`.
*/
public Get setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+/destGroups/[^/]+$");
}
this.name = name;
return this;
}
@Override
public Get set(String parameterName, Object value) {
return (Get) super.set(parameterName, value);
}
}
/**
* Lists the existing TunnelDestGroups. To group across all locations, use a `-` as the location ID.
* For example: `/v1/projects/123/iap_tunnel/locations/-/destGroups`
*
* Create a request for the method "destGroups.list".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation.
*
* @param parent Required. Google Cloud Project ID and location. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}`. A `-` can be used for the
* location to group across all locations.
* @return the request
*/
public List list(java.lang.String parent) throws java.io.IOException {
List result = new List(parent);
initialize(result);
return result;
}
public class List extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+parent}/destGroups";
private final java.util.regex.Pattern PARENT_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/iap_tunnel/locations/[^/]+$");
/**
* Lists the existing TunnelDestGroups. To group across all locations, use a `-` as the location
* ID. For example: `/v1/projects/123/iap_tunnel/locations/-/destGroups`
*
* Create a request for the method "destGroups.list".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link List#execute()} method to invoke the remote operation. {@link
* List#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must be
* called to initialize this instance immediately after invoking the constructor.
*
* @param parent Required. Google Cloud Project ID and location. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}`. A `-` can be used for the
* location to group across all locations.
* @since 1.13
*/
protected List(java.lang.String parent) {
super(CloudIAP.this, "GET", REST_PATH, null, com.google.api.services.iap.v1.model.ListTunnelDestGroupsResponse.class);
this.parent = com.google.api.client.util.Preconditions.checkNotNull(parent, "Required parameter parent must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public List set$Xgafv(java.lang.String $Xgafv) {
return (List) super.set$Xgafv($Xgafv);
}
@Override
public List setAccessToken(java.lang.String accessToken) {
return (List) super.setAccessToken(accessToken);
}
@Override
public List setAlt(java.lang.String alt) {
return (List) super.setAlt(alt);
}
@Override
public List setCallback(java.lang.String callback) {
return (List) super.setCallback(callback);
}
@Override
public List setFields(java.lang.String fields) {
return (List) super.setFields(fields);
}
@Override
public List setKey(java.lang.String key) {
return (List) super.setKey(key);
}
@Override
public List setOauthToken(java.lang.String oauthToken) {
return (List) super.setOauthToken(oauthToken);
}
@Override
public List setPrettyPrint(java.lang.Boolean prettyPrint) {
return (List) super.setPrettyPrint(prettyPrint);
}
@Override
public List setQuotaUser(java.lang.String quotaUser) {
return (List) super.setQuotaUser(quotaUser);
}
@Override
public List setUploadType(java.lang.String uploadType) {
return (List) super.setUploadType(uploadType);
}
@Override
public List setUploadProtocol(java.lang.String uploadProtocol) {
return (List) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. Google Cloud Project ID and location. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}`. A `-` can be used for
* the location to group across all locations.
*/
@com.google.api.client.util.Key
private java.lang.String parent;
/** Required. Google Cloud Project ID and location. In the following format:
`projects/{project_number/id}/iap_tunnel/locations/{location}`. A `-` can be used for the location
to group across all locations.
*/
public java.lang.String getParent() {
return parent;
}
/**
* Required. Google Cloud Project ID and location. In the following format:
* `projects/{project_number/id}/iap_tunnel/locations/{location}`. A `-` can be used for
* the location to group across all locations.
*/
public List setParent(java.lang.String parent) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(PARENT_PATTERN.matcher(parent).matches(),
"Parameter parent must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+$");
}
this.parent = parent;
return this;
}
/**
* The maximum number of groups to return. The service might return fewer than this
* value. If unspecified, at most 100 groups are returned. The maximum value is 1000;
* values above 1000 are coerced to 1000.
*/
@com.google.api.client.util.Key
private java.lang.Integer pageSize;
/** The maximum number of groups to return. The service might return fewer than this value. If
unspecified, at most 100 groups are returned. The maximum value is 1000; values above 1000 are
coerced to 1000.
*/
public java.lang.Integer getPageSize() {
return pageSize;
}
/**
* The maximum number of groups to return. The service might return fewer than this
* value. If unspecified, at most 100 groups are returned. The maximum value is 1000;
* values above 1000 are coerced to 1000.
*/
public List setPageSize(java.lang.Integer pageSize) {
this.pageSize = pageSize;
return this;
}
/**
* A page token, received from a previous `ListTunnelDestGroups` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListTunnelDestGroups` must match the call that provided the page token.
*/
@com.google.api.client.util.Key
private java.lang.String pageToken;
/** A page token, received from a previous `ListTunnelDestGroups` call. Provide this to retrieve the
subsequent page. When paginating, all other parameters provided to `ListTunnelDestGroups` must
match the call that provided the page token.
*/
public java.lang.String getPageToken() {
return pageToken;
}
/**
* A page token, received from a previous `ListTunnelDestGroups` call. Provide this to
* retrieve the subsequent page. When paginating, all other parameters provided to
* `ListTunnelDestGroups` must match the call that provided the page token.
*/
public List setPageToken(java.lang.String pageToken) {
this.pageToken = pageToken;
return this;
}
@Override
public List set(String parameterName, Object value) {
return (List) super.set(parameterName, value);
}
}
/**
* Updates a TunnelDestGroup.
*
* Create a request for the method "destGroups.patch".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation.
*
* @param name Identifier. Identifier for the TunnelDestGroup. Must be unique within the project and contain only
* lower case letters (a-z) and dashes (-).
* @param content the {@link com.google.api.services.iap.v1.model.TunnelDestGroup}
* @return the request
*/
public Patch patch(java.lang.String name, com.google.api.services.iap.v1.model.TunnelDestGroup content) throws java.io.IOException {
Patch result = new Patch(name, content);
initialize(result);
return result;
}
public class Patch extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^projects/[^/]+/iap_tunnel/locations/[^/]+/destGroups/[^/]+$");
/**
* Updates a TunnelDestGroup.
*
* Create a request for the method "destGroups.patch".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link Patch#execute()} method to invoke the remote operation. {@link
* Patch#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)} must
* be called to initialize this instance immediately after invoking the constructor.
*
* @param name Identifier. Identifier for the TunnelDestGroup. Must be unique within the project and contain only
* lower case letters (a-z) and dashes (-).
* @param content the {@link com.google.api.services.iap.v1.model.TunnelDestGroup}
* @since 1.13
*/
protected Patch(java.lang.String name, com.google.api.services.iap.v1.model.TunnelDestGroup content) {
super(CloudIAP.this, "PATCH", REST_PATH, content, com.google.api.services.iap.v1.model.TunnelDestGroup.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+/destGroups/[^/]+$");
}
}
@Override
public Patch set$Xgafv(java.lang.String $Xgafv) {
return (Patch) super.set$Xgafv($Xgafv);
}
@Override
public Patch setAccessToken(java.lang.String accessToken) {
return (Patch) super.setAccessToken(accessToken);
}
@Override
public Patch setAlt(java.lang.String alt) {
return (Patch) super.setAlt(alt);
}
@Override
public Patch setCallback(java.lang.String callback) {
return (Patch) super.setCallback(callback);
}
@Override
public Patch setFields(java.lang.String fields) {
return (Patch) super.setFields(fields);
}
@Override
public Patch setKey(java.lang.String key) {
return (Patch) super.setKey(key);
}
@Override
public Patch setOauthToken(java.lang.String oauthToken) {
return (Patch) super.setOauthToken(oauthToken);
}
@Override
public Patch setPrettyPrint(java.lang.Boolean prettyPrint) {
return (Patch) super.setPrettyPrint(prettyPrint);
}
@Override
public Patch setQuotaUser(java.lang.String quotaUser) {
return (Patch) super.setQuotaUser(quotaUser);
}
@Override
public Patch setUploadType(java.lang.String uploadType) {
return (Patch) super.setUploadType(uploadType);
}
@Override
public Patch setUploadProtocol(java.lang.String uploadProtocol) {
return (Patch) super.setUploadProtocol(uploadProtocol);
}
/**
* Identifier. Identifier for the TunnelDestGroup. Must be unique within the project and
* contain only lower case letters (a-z) and dashes (-).
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Identifier. Identifier for the TunnelDestGroup. Must be unique within the project and contain only
lower case letters (a-z) and dashes (-).
*/
public java.lang.String getName() {
return name;
}
/**
* Identifier. Identifier for the TunnelDestGroup. Must be unique within the project and
* contain only lower case letters (a-z) and dashes (-).
*/
public Patch setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^projects/[^/]+/iap_tunnel/locations/[^/]+/destGroups/[^/]+$");
}
this.name = name;
return this;
}
/**
* A field mask that specifies which IAP settings to update. If omitted, then all of the
* settings are updated. See https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask
*/
@com.google.api.client.util.Key
private String updateMask;
/** A field mask that specifies which IAP settings to update. If omitted, then all of the settings are
updated. See https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#fieldmask
*/
public String getUpdateMask() {
return updateMask;
}
/**
* A field mask that specifies which IAP settings to update. If omitted, then all of the
* settings are updated. See https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask
*/
public Patch setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public Patch set(String parameterName, Object value) {
return (Patch) super.set(parameterName, value);
}
}
}
}
}
}
/**
* An accessor for creating requests from the V1 collection.
*
* The typical use is:
*
* {@code CloudIAP iap = new CloudIAP(...);}
* {@code CloudIAP.V1.List request = iap.v1().list(parameters ...)}
*
*
* @return the resource collection
*/
public V1 v1() {
return new V1();
}
/**
* The "v1" collection of methods.
*/
public class V1 {
/**
* Gets the access control policy for an Identity-Aware Proxy protected resource. More information
* about managing access via IAP can be found at: https://cloud.google.com/iap/docs/managing-
* access#managing_access_via_the_api
*
* Create a request for the method "v1.getIamPolicy".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.iap.v1.model.GetIamPolicyRequest}
* @return the request
*/
public GetIamPolicy getIamPolicy(java.lang.String resource, com.google.api.services.iap.v1.model.GetIamPolicyRequest content) throws java.io.IOException {
GetIamPolicy result = new GetIamPolicy(resource, content);
initialize(result);
return result;
}
public class GetIamPolicy extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+resource}:getIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^.*$");
/**
* Gets the access control policy for an Identity-Aware Proxy protected resource. More information
* about managing access via IAP can be found at: https://cloud.google.com/iap/docs/managing-
* access#managing_access_via_the_api
*
* Create a request for the method "v1.getIamPolicy".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link GetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* GetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.iap.v1.model.GetIamPolicyRequest}
* @since 1.13
*/
protected GetIamPolicy(java.lang.String resource, com.google.api.services.iap.v1.model.GetIamPolicyRequest content) {
super(CloudIAP.this, "POST", REST_PATH, content, com.google.api.services.iap.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^.*$");
}
}
@Override
public GetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (GetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public GetIamPolicy setAccessToken(java.lang.String accessToken) {
return (GetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public GetIamPolicy setAlt(java.lang.String alt) {
return (GetIamPolicy) super.setAlt(alt);
}
@Override
public GetIamPolicy setCallback(java.lang.String callback) {
return (GetIamPolicy) super.setCallback(callback);
}
@Override
public GetIamPolicy setFields(java.lang.String fields) {
return (GetIamPolicy) super.setFields(fields);
}
@Override
public GetIamPolicy setKey(java.lang.String key) {
return (GetIamPolicy) super.setKey(key);
}
@Override
public GetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (GetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public GetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (GetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public GetIamPolicy setUploadType(java.lang.String uploadType) {
return (GetIamPolicy) super.setUploadType(uploadType);
}
@Override
public GetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public GetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^.*$");
}
this.resource = resource;
return this;
}
@Override
public GetIamPolicy set(String parameterName, Object value) {
return (GetIamPolicy) super.set(parameterName, value);
}
}
/**
* Gets the IAP settings on a particular IAP protected resource.
*
* Create a request for the method "v1.getIapSettings".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link GetIapSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name for which to retrieve the settings. Authorization: Requires the
* `getSettings` permission for the associated resource.
* @return the request
*/
public GetIapSettings getIapSettings(java.lang.String name) throws java.io.IOException {
GetIapSettings result = new GetIapSettings(name);
initialize(result);
return result;
}
public class GetIapSettings extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}:iapSettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^.*$");
/**
* Gets the IAP settings on a particular IAP protected resource.
*
* Create a request for the method "v1.getIapSettings".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link GetIapSettings#execute()} method to invoke the remote operation.
* {@link GetIapSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogleCl
* ientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. The resource name for which to retrieve the settings. Authorization: Requires the
* `getSettings` permission for the associated resource.
* @since 1.13
*/
protected GetIapSettings(java.lang.String name) {
super(CloudIAP.this, "GET", REST_PATH, null, com.google.api.services.iap.v1.model.IapSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^.*$");
}
}
@Override
public com.google.api.client.http.HttpResponse executeUsingHead() throws java.io.IOException {
return super.executeUsingHead();
}
@Override
public com.google.api.client.http.HttpRequest buildHttpRequestUsingHead() throws java.io.IOException {
return super.buildHttpRequestUsingHead();
}
@Override
public GetIapSettings set$Xgafv(java.lang.String $Xgafv) {
return (GetIapSettings) super.set$Xgafv($Xgafv);
}
@Override
public GetIapSettings setAccessToken(java.lang.String accessToken) {
return (GetIapSettings) super.setAccessToken(accessToken);
}
@Override
public GetIapSettings setAlt(java.lang.String alt) {
return (GetIapSettings) super.setAlt(alt);
}
@Override
public GetIapSettings setCallback(java.lang.String callback) {
return (GetIapSettings) super.setCallback(callback);
}
@Override
public GetIapSettings setFields(java.lang.String fields) {
return (GetIapSettings) super.setFields(fields);
}
@Override
public GetIapSettings setKey(java.lang.String key) {
return (GetIapSettings) super.setKey(key);
}
@Override
public GetIapSettings setOauthToken(java.lang.String oauthToken) {
return (GetIapSettings) super.setOauthToken(oauthToken);
}
@Override
public GetIapSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (GetIapSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public GetIapSettings setQuotaUser(java.lang.String quotaUser) {
return (GetIapSettings) super.setQuotaUser(quotaUser);
}
@Override
public GetIapSettings setUploadType(java.lang.String uploadType) {
return (GetIapSettings) super.setUploadType(uploadType);
}
@Override
public GetIapSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (GetIapSettings) super.setUploadProtocol(uploadProtocol);
}
/**
* Required. The resource name for which to retrieve the settings. Authorization: Requires the
* `getSettings` permission for the associated resource.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name for which to retrieve the settings. Authorization: Requires the
`getSettings` permission for the associated resource.
*/
public java.lang.String getName() {
return name;
}
/**
* Required. The resource name for which to retrieve the settings. Authorization: Requires the
* `getSettings` permission for the associated resource.
*/
public GetIapSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^.*$");
}
this.name = name;
return this;
}
@Override
public GetIapSettings set(String parameterName, Object value) {
return (GetIapSettings) super.set(parameterName, value);
}
}
/**
* Sets the access control policy for an Identity-Aware Proxy protected resource. Replaces any
* existing policy. More information about managing access via IAP can be found at:
* https://cloud.google.com/iap/docs/managing-access#managing_access_via_the_api
*
* Create a request for the method "v1.setIamPolicy".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.iap.v1.model.SetIamPolicyRequest}
* @return the request
*/
public SetIamPolicy setIamPolicy(java.lang.String resource, com.google.api.services.iap.v1.model.SetIamPolicyRequest content) throws java.io.IOException {
SetIamPolicy result = new SetIamPolicy(resource, content);
initialize(result);
return result;
}
public class SetIamPolicy extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+resource}:setIamPolicy";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^.*$");
/**
* Sets the access control policy for an Identity-Aware Proxy protected resource. Replaces any
* existing policy. More information about managing access via IAP can be found at:
* https://cloud.google.com/iap/docs/managing-access#managing_access_via_the_api
*
* Create a request for the method "v1.setIamPolicy".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link SetIamPolicy#execute()} method to invoke the remote operation.
* {@link
* SetIamPolicy#initialize(com.google.api.client.googleapis.services.AbstractGoogleClientRequest)}
* must be called to initialize this instance immediately after invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.iap.v1.model.SetIamPolicyRequest}
* @since 1.13
*/
protected SetIamPolicy(java.lang.String resource, com.google.api.services.iap.v1.model.SetIamPolicyRequest content) {
super(CloudIAP.this, "POST", REST_PATH, content, com.google.api.services.iap.v1.model.Policy.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^.*$");
}
}
@Override
public SetIamPolicy set$Xgafv(java.lang.String $Xgafv) {
return (SetIamPolicy) super.set$Xgafv($Xgafv);
}
@Override
public SetIamPolicy setAccessToken(java.lang.String accessToken) {
return (SetIamPolicy) super.setAccessToken(accessToken);
}
@Override
public SetIamPolicy setAlt(java.lang.String alt) {
return (SetIamPolicy) super.setAlt(alt);
}
@Override
public SetIamPolicy setCallback(java.lang.String callback) {
return (SetIamPolicy) super.setCallback(callback);
}
@Override
public SetIamPolicy setFields(java.lang.String fields) {
return (SetIamPolicy) super.setFields(fields);
}
@Override
public SetIamPolicy setKey(java.lang.String key) {
return (SetIamPolicy) super.setKey(key);
}
@Override
public SetIamPolicy setOauthToken(java.lang.String oauthToken) {
return (SetIamPolicy) super.setOauthToken(oauthToken);
}
@Override
public SetIamPolicy setPrettyPrint(java.lang.Boolean prettyPrint) {
return (SetIamPolicy) super.setPrettyPrint(prettyPrint);
}
@Override
public SetIamPolicy setQuotaUser(java.lang.String quotaUser) {
return (SetIamPolicy) super.setQuotaUser(quotaUser);
}
@Override
public SetIamPolicy setUploadType(java.lang.String uploadType) {
return (SetIamPolicy) super.setUploadType(uploadType);
}
@Override
public SetIamPolicy setUploadProtocol(java.lang.String uploadProtocol) {
return (SetIamPolicy) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy is being specified. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy is being specified. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public SetIamPolicy setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^.*$");
}
this.resource = resource;
return this;
}
@Override
public SetIamPolicy set(String parameterName, Object value) {
return (SetIamPolicy) super.set(parameterName, value);
}
}
/**
* Returns permissions that a caller has on the Identity-Aware Proxy protected resource. More
* information about managing access via IAP can be found at:
* https://cloud.google.com/iap/docs/managing-access#managing_access_via_the_api
*
* Create a request for the method "v1.testIamPermissions".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote operation.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.iap.v1.model.TestIamPermissionsRequest}
* @return the request
*/
public TestIamPermissions testIamPermissions(java.lang.String resource, com.google.api.services.iap.v1.model.TestIamPermissionsRequest content) throws java.io.IOException {
TestIamPermissions result = new TestIamPermissions(resource, content);
initialize(result);
return result;
}
public class TestIamPermissions extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+resource}:testIamPermissions";
private final java.util.regex.Pattern RESOURCE_PATTERN =
java.util.regex.Pattern.compile("^.*$");
/**
* Returns permissions that a caller has on the Identity-Aware Proxy protected resource. More
* information about managing access via IAP can be found at:
* https://cloud.google.com/iap/docs/managing-access#managing_access_via_the_api
*
* Create a request for the method "v1.testIamPermissions".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link TestIamPermissions#execute()} method to invoke the remote
* operation. {@link TestIamPermissions#initialize(com.google.api.client.googleapis.services.A
* bstractGoogleClientRequest)} must be called to initialize this instance immediately after
* invoking the constructor.
*
* @param resource REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
* @param content the {@link com.google.api.services.iap.v1.model.TestIamPermissionsRequest}
* @since 1.13
*/
protected TestIamPermissions(java.lang.String resource, com.google.api.services.iap.v1.model.TestIamPermissionsRequest content) {
super(CloudIAP.this, "POST", REST_PATH, content, com.google.api.services.iap.v1.model.TestIamPermissionsResponse.class);
this.resource = com.google.api.client.util.Preconditions.checkNotNull(resource, "Required parameter resource must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^.*$");
}
}
@Override
public TestIamPermissions set$Xgafv(java.lang.String $Xgafv) {
return (TestIamPermissions) super.set$Xgafv($Xgafv);
}
@Override
public TestIamPermissions setAccessToken(java.lang.String accessToken) {
return (TestIamPermissions) super.setAccessToken(accessToken);
}
@Override
public TestIamPermissions setAlt(java.lang.String alt) {
return (TestIamPermissions) super.setAlt(alt);
}
@Override
public TestIamPermissions setCallback(java.lang.String callback) {
return (TestIamPermissions) super.setCallback(callback);
}
@Override
public TestIamPermissions setFields(java.lang.String fields) {
return (TestIamPermissions) super.setFields(fields);
}
@Override
public TestIamPermissions setKey(java.lang.String key) {
return (TestIamPermissions) super.setKey(key);
}
@Override
public TestIamPermissions setOauthToken(java.lang.String oauthToken) {
return (TestIamPermissions) super.setOauthToken(oauthToken);
}
@Override
public TestIamPermissions setPrettyPrint(java.lang.Boolean prettyPrint) {
return (TestIamPermissions) super.setPrettyPrint(prettyPrint);
}
@Override
public TestIamPermissions setQuotaUser(java.lang.String quotaUser) {
return (TestIamPermissions) super.setQuotaUser(quotaUser);
}
@Override
public TestIamPermissions setUploadType(java.lang.String uploadType) {
return (TestIamPermissions) super.setUploadType(uploadType);
}
@Override
public TestIamPermissions setUploadProtocol(java.lang.String uploadProtocol) {
return (TestIamPermissions) super.setUploadProtocol(uploadProtocol);
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
@com.google.api.client.util.Key
private java.lang.String resource;
/** REQUIRED: The resource for which the policy detail is being requested. See [Resource
names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for this
field.
*/
public java.lang.String getResource() {
return resource;
}
/**
* REQUIRED: The resource for which the policy detail is being requested. See [Resource
* names](https://cloud.google.com/apis/design/resource_names) for the appropriate value for
* this field.
*/
public TestIamPermissions setResource(java.lang.String resource) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(RESOURCE_PATTERN.matcher(resource).matches(),
"Parameter resource must conform to the pattern " +
"^.*$");
}
this.resource = resource;
return this;
}
@Override
public TestIamPermissions set(String parameterName, Object value) {
return (TestIamPermissions) super.set(parameterName, value);
}
}
/**
* Updates the IAP settings on a particular IAP protected resource. It replaces all fields unless
* the `update_mask` is set.
*
* Create a request for the method "v1.updateIapSettings".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link UpdateIapSettings#execute()} method to invoke the remote operation.
*
* @param name Required. The resource name of the IAP protected resource.
* @param content the {@link com.google.api.services.iap.v1.model.IapSettings}
* @return the request
*/
public UpdateIapSettings updateIapSettings(java.lang.String name, com.google.api.services.iap.v1.model.IapSettings content) throws java.io.IOException {
UpdateIapSettings result = new UpdateIapSettings(name, content);
initialize(result);
return result;
}
public class UpdateIapSettings extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}:iapSettings";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^.*$");
/**
* Updates the IAP settings on a particular IAP protected resource. It replaces all fields unless
* the `update_mask` is set.
*
* Create a request for the method "v1.updateIapSettings".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link UpdateIapSettings#execute()} method to invoke the remote operation.
* {@link UpdateIapSettings#initialize(com.google.api.client.googleapis.services.AbstractGoogl
* eClientRequest)} must be called to initialize this instance immediately after invoking the
* constructor.
*
* @param name Required. The resource name of the IAP protected resource.
* @param content the {@link com.google.api.services.iap.v1.model.IapSettings}
* @since 1.13
*/
protected UpdateIapSettings(java.lang.String name, com.google.api.services.iap.v1.model.IapSettings content) {
super(CloudIAP.this, "PATCH", REST_PATH, content, com.google.api.services.iap.v1.model.IapSettings.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^.*$");
}
}
@Override
public UpdateIapSettings set$Xgafv(java.lang.String $Xgafv) {
return (UpdateIapSettings) super.set$Xgafv($Xgafv);
}
@Override
public UpdateIapSettings setAccessToken(java.lang.String accessToken) {
return (UpdateIapSettings) super.setAccessToken(accessToken);
}
@Override
public UpdateIapSettings setAlt(java.lang.String alt) {
return (UpdateIapSettings) super.setAlt(alt);
}
@Override
public UpdateIapSettings setCallback(java.lang.String callback) {
return (UpdateIapSettings) super.setCallback(callback);
}
@Override
public UpdateIapSettings setFields(java.lang.String fields) {
return (UpdateIapSettings) super.setFields(fields);
}
@Override
public UpdateIapSettings setKey(java.lang.String key) {
return (UpdateIapSettings) super.setKey(key);
}
@Override
public UpdateIapSettings setOauthToken(java.lang.String oauthToken) {
return (UpdateIapSettings) super.setOauthToken(oauthToken);
}
@Override
public UpdateIapSettings setPrettyPrint(java.lang.Boolean prettyPrint) {
return (UpdateIapSettings) super.setPrettyPrint(prettyPrint);
}
@Override
public UpdateIapSettings setQuotaUser(java.lang.String quotaUser) {
return (UpdateIapSettings) super.setQuotaUser(quotaUser);
}
@Override
public UpdateIapSettings setUploadType(java.lang.String uploadType) {
return (UpdateIapSettings) super.setUploadType(uploadType);
}
@Override
public UpdateIapSettings setUploadProtocol(java.lang.String uploadProtocol) {
return (UpdateIapSettings) super.setUploadProtocol(uploadProtocol);
}
/** Required. The resource name of the IAP protected resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the IAP protected resource.
*/
public java.lang.String getName() {
return name;
}
/** Required. The resource name of the IAP protected resource. */
public UpdateIapSettings setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^.*$");
}
this.name = name;
return this;
}
/**
* The field mask specifying which IAP settings should be updated. If omitted, then all of the
* settings are updated. See https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask. Note: All IAP reauth settings must always
* be set together, using the field mask: `iapSettings.accessSettings.reauthSettings`.
*/
@com.google.api.client.util.Key
private String updateMask;
/** The field mask specifying which IAP settings should be updated. If omitted, then all of the
settings are updated. See https://developers.google.com/protocol-
buffers/docs/reference/google.protobuf#fieldmask. Note: All IAP reauth settings must always be set
together, using the field mask: `iapSettings.accessSettings.reauthSettings`.
*/
public String getUpdateMask() {
return updateMask;
}
/**
* The field mask specifying which IAP settings should be updated. If omitted, then all of the
* settings are updated. See https://developers.google.com/protocol-
* buffers/docs/reference/google.protobuf#fieldmask. Note: All IAP reauth settings must always
* be set together, using the field mask: `iapSettings.accessSettings.reauthSettings`.
*/
public UpdateIapSettings setUpdateMask(String updateMask) {
this.updateMask = updateMask;
return this;
}
@Override
public UpdateIapSettings set(String parameterName, Object value) {
return (UpdateIapSettings) super.set(parameterName, value);
}
}
/**
* Validates that a given CEL expression conforms to IAP restrictions.
*
* Create a request for the method "v1.validateAttributeExpression".
*
* This request holds the parameters needed by the iap server. After setting any optional
* parameters, call the {@link ValidateAttributeExpression#execute()} method to invoke the remote
* operation.
*
* @param name Required. The resource name of the IAP protected resource.
* @return the request
*/
public ValidateAttributeExpression validateAttributeExpression(java.lang.String name) throws java.io.IOException {
ValidateAttributeExpression result = new ValidateAttributeExpression(name);
initialize(result);
return result;
}
public class ValidateAttributeExpression extends CloudIAPRequest {
private static final String REST_PATH = "v1/{+name}:validateAttributeExpression";
private final java.util.regex.Pattern NAME_PATTERN =
java.util.regex.Pattern.compile("^.*$");
/**
* Validates that a given CEL expression conforms to IAP restrictions.
*
* Create a request for the method "v1.validateAttributeExpression".
*
* This request holds the parameters needed by the the iap server. After setting any optional
* parameters, call the {@link ValidateAttributeExpression#execute()} method to invoke the remote
* operation. {@link ValidateAttributeExpression#initialize(com.google.api.client.googleapis.s
* ervices.AbstractGoogleClientRequest)} must be called to initialize this instance immediately
* after invoking the constructor.
*
* @param name Required. The resource name of the IAP protected resource.
* @since 1.13
*/
protected ValidateAttributeExpression(java.lang.String name) {
super(CloudIAP.this, "POST", REST_PATH, null, com.google.api.services.iap.v1.model.ValidateIapAttributeExpressionResponse.class);
this.name = com.google.api.client.util.Preconditions.checkNotNull(name, "Required parameter name must be specified.");
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^.*$");
}
}
@Override
public ValidateAttributeExpression set$Xgafv(java.lang.String $Xgafv) {
return (ValidateAttributeExpression) super.set$Xgafv($Xgafv);
}
@Override
public ValidateAttributeExpression setAccessToken(java.lang.String accessToken) {
return (ValidateAttributeExpression) super.setAccessToken(accessToken);
}
@Override
public ValidateAttributeExpression setAlt(java.lang.String alt) {
return (ValidateAttributeExpression) super.setAlt(alt);
}
@Override
public ValidateAttributeExpression setCallback(java.lang.String callback) {
return (ValidateAttributeExpression) super.setCallback(callback);
}
@Override
public ValidateAttributeExpression setFields(java.lang.String fields) {
return (ValidateAttributeExpression) super.setFields(fields);
}
@Override
public ValidateAttributeExpression setKey(java.lang.String key) {
return (ValidateAttributeExpression) super.setKey(key);
}
@Override
public ValidateAttributeExpression setOauthToken(java.lang.String oauthToken) {
return (ValidateAttributeExpression) super.setOauthToken(oauthToken);
}
@Override
public ValidateAttributeExpression setPrettyPrint(java.lang.Boolean prettyPrint) {
return (ValidateAttributeExpression) super.setPrettyPrint(prettyPrint);
}
@Override
public ValidateAttributeExpression setQuotaUser(java.lang.String quotaUser) {
return (ValidateAttributeExpression) super.setQuotaUser(quotaUser);
}
@Override
public ValidateAttributeExpression setUploadType(java.lang.String uploadType) {
return (ValidateAttributeExpression) super.setUploadType(uploadType);
}
@Override
public ValidateAttributeExpression setUploadProtocol(java.lang.String uploadProtocol) {
return (ValidateAttributeExpression) super.setUploadProtocol(uploadProtocol);
}
/** Required. The resource name of the IAP protected resource. */
@com.google.api.client.util.Key
private java.lang.String name;
/** Required. The resource name of the IAP protected resource.
*/
public java.lang.String getName() {
return name;
}
/** Required. The resource name of the IAP protected resource. */
public ValidateAttributeExpression setName(java.lang.String name) {
if (!getSuppressPatternChecks()) {
com.google.api.client.util.Preconditions.checkArgument(NAME_PATTERN.matcher(name).matches(),
"Parameter name must conform to the pattern " +
"^.*$");
}
this.name = name;
return this;
}
/**
* Required. User input string expression. Should be of the form
* `attributes.saml_attributes.filter(attribute, attribute.name in ['{attribute_name}',
* '{attribute_name}'])`
*/
@com.google.api.client.util.Key
private java.lang.String expression;
/** Required. User input string expression. Should be of the form
`attributes.saml_attributes.filter(attribute, attribute.name in ['{attribute_name}',
'{attribute_name}'])`
*/
public java.lang.String getExpression() {
return expression;
}
/**
* Required. User input string expression. Should be of the form
* `attributes.saml_attributes.filter(attribute, attribute.name in ['{attribute_name}',
* '{attribute_name}'])`
*/
public ValidateAttributeExpression setExpression(java.lang.String expression) {
this.expression = expression;
return this;
}
@Override
public ValidateAttributeExpression set(String parameterName, Object value) {
return (ValidateAttributeExpression) super.set(parameterName, value);
}
}
}
/**
* Builder for {@link CloudIAP}.
*
*
* Implementation is not thread-safe.
*
*
* @since 1.3.0
*/
public static final class Builder extends com.google.api.client.googleapis.services.json.AbstractGoogleJsonClient.Builder {
private static String chooseEndpoint(com.google.api.client.http.HttpTransport transport) {
// If the GOOGLE_API_USE_MTLS_ENDPOINT environment variable value is "always", use mTLS endpoint.
// If the env variable is "auto", use mTLS endpoint if and only if the transport is mTLS.
// Use the regular endpoint for all other cases.
String useMtlsEndpoint = System.getenv("GOOGLE_API_USE_MTLS_ENDPOINT");
useMtlsEndpoint = useMtlsEndpoint == null ? "auto" : useMtlsEndpoint;
if ("always".equals(useMtlsEndpoint) || ("auto".equals(useMtlsEndpoint) && transport != null && transport.isMtls())) {
return DEFAULT_MTLS_ROOT_URL;
}
return DEFAULT_ROOT_URL;
}
/**
* Returns an instance of a new builder.
*
* @param transport HTTP transport, which should normally be:
*
* - Google App Engine:
* {@code com.google.api.client.extensions.appengine.http.UrlFetchTransport}
* - Android: {@code newCompatibleTransport} from
* {@code com.google.api.client.extensions.android.http.AndroidHttp}
* - Java: {@link com.google.api.client.googleapis.javanet.GoogleNetHttpTransport#newTrustedTransport()}
*
*
* @param jsonFactory JSON factory, which may be:
*
* - Jackson: {@code com.google.api.client.json.jackson2.JacksonFactory}
* - Google GSON: {@code com.google.api.client.json.gson.GsonFactory}
* - Android Honeycomb or higher:
* {@code com.google.api.client.extensions.android.json.AndroidJsonFactory}
*
* @param httpRequestInitializer HTTP request initializer or {@code null} for none
* @since 1.7
*/
public Builder(com.google.api.client.http.HttpTransport transport, com.google.api.client.json.JsonFactory jsonFactory,
com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
super(
transport,
jsonFactory,
Builder.chooseEndpoint(transport),
DEFAULT_SERVICE_PATH,
httpRequestInitializer,
false);
setBatchPath(DEFAULT_BATCH_PATH);
}
/** Builds a new instance of {@link CloudIAP}. */
@Override
public CloudIAP build() {
return new CloudIAP(this);
}
@Override
public Builder setRootUrl(String rootUrl) {
return (Builder) super.setRootUrl(rootUrl);
}
@Override
public Builder setServicePath(String servicePath) {
return (Builder) super.setServicePath(servicePath);
}
@Override
public Builder setBatchPath(String batchPath) {
return (Builder) super.setBatchPath(batchPath);
}
@Override
public Builder setHttpRequestInitializer(com.google.api.client.http.HttpRequestInitializer httpRequestInitializer) {
return (Builder) super.setHttpRequestInitializer(httpRequestInitializer);
}
@Override
public Builder setApplicationName(String applicationName) {
return (Builder) super.setApplicationName(applicationName);
}
@Override
public Builder setSuppressPatternChecks(boolean suppressPatternChecks) {
return (Builder) super.setSuppressPatternChecks(suppressPatternChecks);
}
@Override
public Builder setSuppressRequiredParameterChecks(boolean suppressRequiredParameterChecks) {
return (Builder) super.setSuppressRequiredParameterChecks(suppressRequiredParameterChecks);
}
@Override
public Builder setSuppressAllChecks(boolean suppressAllChecks) {
return (Builder) super.setSuppressAllChecks(suppressAllChecks);
}
/**
* Set the {@link CloudIAPRequestInitializer}.
*
* @since 1.12
*/
public Builder setCloudIAPRequestInitializer(
CloudIAPRequestInitializer cloudiapRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(cloudiapRequestInitializer);
}
@Override
public Builder setGoogleClientRequestInitializer(
com.google.api.client.googleapis.services.GoogleClientRequestInitializer googleClientRequestInitializer) {
return (Builder) super.setGoogleClientRequestInitializer(googleClientRequestInitializer);
}
@Override
public Builder setUniverseDomain(String universeDomain) {
return (Builder) super.setUniverseDomain(universeDomain);
}
}
}