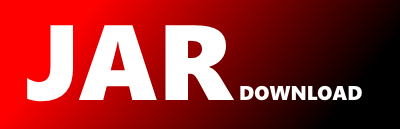
com.google.api.services.iap.v1.model.Resource Maven / Gradle / Ivy
/*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
/*
* This code was generated by https://github.com/googleapis/google-api-java-client-services/
* Modify at your own risk.
*/
package com.google.api.services.iap.v1.model;
/**
* Model definition for Resource.
*
* This is the Java data model class that specifies how to parse/serialize into the JSON that is
* transmitted over HTTP when working with the Cloud Identity-Aware Proxy API. For a detailed
* explanation see:
* https://developers.google.com/api-client-library/java/google-http-java-client/json
*
*
* @author Google, Inc.
*/
@SuppressWarnings("javadoc")
public final class Resource extends com.google.api.client.json.GenericJson {
/**
* The proto or JSON formatted expected next state of the resource, wrapped in a
* google.protobuf.Any proto, against which the policy rules are evaluated. Services not
* integrated with custom org policy can omit this field. Services integrated with custom org
* policy must populate this field for all requests where the API call changes the state of the
* resource. Custom org policy backend uses these attributes to enforce custom org policies. For
* create operations, GCP service is expected to pass resource from customer request as is. For
* update/patch operations, GCP service is expected to compute the next state with the patch
* provided by the user. See go/custom-constraints-org-policy-integration-guide for additional
* details.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map expectedNextState;
/**
* The service defined labels of the resource on which the conditions will be evaluated. The
* semantics - including the key names - are vague to IAM. If the effective condition has a
* reference to a `resource.labels[foo]` construct, IAM consults with this map to retrieve the
* values associated with `foo` key for Conditions evaluation. If the provided key is not found in
* the labels map, the condition would evaluate to false. This field is in limited use. If your
* intended use case is not expected to express resource.labels attribute in IAM Conditions, leave
* this field empty. Before planning on using this attribute please: * Read go/iam-conditions-
* labels-comm and ensure your service can meet the data availability and management requirements.
* * Talk to iam-conditions-eng@ about your use case.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.util.Map labels;
/**
* The **relative** name of the resource, which is the URI path of the resource without the
* leading "/". See https://cloud.google.com/iam/docs/conditions-resource-attributes#resource-name
* for examples used by other GCP Services. This field is **required** for services integrated
* with resource-attribute-based IAM conditions and/or CustomOrgPolicy. This field requires
* special handling for parents-only permissions such as `create` and `list`. See the document
* linked below for further details. See go/iam-conditions-sig-g3#populate-resource-attributes for
* specific details on populating this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String name;
/**
* Used for calculating the next state of tags on the resource being passed for Custom Org Policy
* enforcement. NOTE: Only one of the tags representations (i.e. numeric or namespaced) should be
* populated. The input tags will be converted to the same representation before the calculation.
* This behavior intentionally may differ from other tags related fields in CheckPolicy request,
* which may require both formats to be passed in. IMPORTANT: If tags are unchanged, this field
* should not be set.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private NextStateOfTags nextStateOfTags;
/**
* The name of the service this resource belongs to. It is configured using the
* official_service_name of the Service as defined in service configurations under
* //configs/cloud/resourcetypes. For example, the official_service_name of cloud resource manager
* service is set as 'cloudresourcemanager.googleapis.com' according to
* //configs/cloud/resourcetypes/google/cloud/resourcemanager/prod.yaml This field is **required**
* for services integrated with resource-attribute-based IAM conditions and/or CustomOrgPolicy.
* This field requires special handling for parents-only permissions such as `create` and `list`.
* See the document linked below for further details. See go/iam-conditions-sig-g3#populate-
* resource-attributes for specific details on populating this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String service;
/**
* The public resource type name of the resource. It is configured using the official_name of the
* ResourceType as defined in service configurations under //configs/cloud/resourcetypes. For
* example, the official_name for GCP projects is set as
* 'cloudresourcemanager.googleapis.com/Project' according to
* //configs/cloud/resourcetypes/google/cloud/resourcemanager/prod.yaml This field is **required**
* for services integrated with resource-attribute-based IAM conditions and/or CustomOrgPolicy.
* This field requires special handling for parents-only permissions such as `create` and `list`.
* See the document linked below for further details. See go/iam-conditions-sig-g3#populate-
* resource-attributes for specific details on populating this field.
* The value may be {@code null}.
*/
@com.google.api.client.util.Key
private java.lang.String type;
/**
* The proto or JSON formatted expected next state of the resource, wrapped in a
* google.protobuf.Any proto, against which the policy rules are evaluated. Services not
* integrated with custom org policy can omit this field. Services integrated with custom org
* policy must populate this field for all requests where the API call changes the state of the
* resource. Custom org policy backend uses these attributes to enforce custom org policies. For
* create operations, GCP service is expected to pass resource from customer request as is. For
* update/patch operations, GCP service is expected to compute the next state with the patch
* provided by the user. See go/custom-constraints-org-policy-integration-guide for additional
* details.
* @return value or {@code null} for none
*/
public java.util.Map getExpectedNextState() {
return expectedNextState;
}
/**
* The proto or JSON formatted expected next state of the resource, wrapped in a
* google.protobuf.Any proto, against which the policy rules are evaluated. Services not
* integrated with custom org policy can omit this field. Services integrated with custom org
* policy must populate this field for all requests where the API call changes the state of the
* resource. Custom org policy backend uses these attributes to enforce custom org policies. For
* create operations, GCP service is expected to pass resource from customer request as is. For
* update/patch operations, GCP service is expected to compute the next state with the patch
* provided by the user. See go/custom-constraints-org-policy-integration-guide for additional
* details.
* @param expectedNextState expectedNextState or {@code null} for none
*/
public Resource setExpectedNextState(java.util.Map expectedNextState) {
this.expectedNextState = expectedNextState;
return this;
}
/**
* The service defined labels of the resource on which the conditions will be evaluated. The
* semantics - including the key names - are vague to IAM. If the effective condition has a
* reference to a `resource.labels[foo]` construct, IAM consults with this map to retrieve the
* values associated with `foo` key for Conditions evaluation. If the provided key is not found in
* the labels map, the condition would evaluate to false. This field is in limited use. If your
* intended use case is not expected to express resource.labels attribute in IAM Conditions, leave
* this field empty. Before planning on using this attribute please: * Read go/iam-conditions-
* labels-comm and ensure your service can meet the data availability and management requirements.
* * Talk to iam-conditions-eng@ about your use case.
* @return value or {@code null} for none
*/
public java.util.Map getLabels() {
return labels;
}
/**
* The service defined labels of the resource on which the conditions will be evaluated. The
* semantics - including the key names - are vague to IAM. If the effective condition has a
* reference to a `resource.labels[foo]` construct, IAM consults with this map to retrieve the
* values associated with `foo` key for Conditions evaluation. If the provided key is not found in
* the labels map, the condition would evaluate to false. This field is in limited use. If your
* intended use case is not expected to express resource.labels attribute in IAM Conditions, leave
* this field empty. Before planning on using this attribute please: * Read go/iam-conditions-
* labels-comm and ensure your service can meet the data availability and management requirements.
* * Talk to iam-conditions-eng@ about your use case.
* @param labels labels or {@code null} for none
*/
public Resource setLabels(java.util.Map labels) {
this.labels = labels;
return this;
}
/**
* The **relative** name of the resource, which is the URI path of the resource without the
* leading "/". See https://cloud.google.com/iam/docs/conditions-resource-attributes#resource-name
* for examples used by other GCP Services. This field is **required** for services integrated
* with resource-attribute-based IAM conditions and/or CustomOrgPolicy. This field requires
* special handling for parents-only permissions such as `create` and `list`. See the document
* linked below for further details. See go/iam-conditions-sig-g3#populate-resource-attributes for
* specific details on populating this field.
* @return value or {@code null} for none
*/
public java.lang.String getName() {
return name;
}
/**
* The **relative** name of the resource, which is the URI path of the resource without the
* leading "/". See https://cloud.google.com/iam/docs/conditions-resource-attributes#resource-name
* for examples used by other GCP Services. This field is **required** for services integrated
* with resource-attribute-based IAM conditions and/or CustomOrgPolicy. This field requires
* special handling for parents-only permissions such as `create` and `list`. See the document
* linked below for further details. See go/iam-conditions-sig-g3#populate-resource-attributes for
* specific details on populating this field.
* @param name name or {@code null} for none
*/
public Resource setName(java.lang.String name) {
this.name = name;
return this;
}
/**
* Used for calculating the next state of tags on the resource being passed for Custom Org Policy
* enforcement. NOTE: Only one of the tags representations (i.e. numeric or namespaced) should be
* populated. The input tags will be converted to the same representation before the calculation.
* This behavior intentionally may differ from other tags related fields in CheckPolicy request,
* which may require both formats to be passed in. IMPORTANT: If tags are unchanged, this field
* should not be set.
* @return value or {@code null} for none
*/
public NextStateOfTags getNextStateOfTags() {
return nextStateOfTags;
}
/**
* Used for calculating the next state of tags on the resource being passed for Custom Org Policy
* enforcement. NOTE: Only one of the tags representations (i.e. numeric or namespaced) should be
* populated. The input tags will be converted to the same representation before the calculation.
* This behavior intentionally may differ from other tags related fields in CheckPolicy request,
* which may require both formats to be passed in. IMPORTANT: If tags are unchanged, this field
* should not be set.
* @param nextStateOfTags nextStateOfTags or {@code null} for none
*/
public Resource setNextStateOfTags(NextStateOfTags nextStateOfTags) {
this.nextStateOfTags = nextStateOfTags;
return this;
}
/**
* The name of the service this resource belongs to. It is configured using the
* official_service_name of the Service as defined in service configurations under
* //configs/cloud/resourcetypes. For example, the official_service_name of cloud resource manager
* service is set as 'cloudresourcemanager.googleapis.com' according to
* //configs/cloud/resourcetypes/google/cloud/resourcemanager/prod.yaml This field is **required**
* for services integrated with resource-attribute-based IAM conditions and/or CustomOrgPolicy.
* This field requires special handling for parents-only permissions such as `create` and `list`.
* See the document linked below for further details. See go/iam-conditions-sig-g3#populate-
* resource-attributes for specific details on populating this field.
* @return value or {@code null} for none
*/
public java.lang.String getService() {
return service;
}
/**
* The name of the service this resource belongs to. It is configured using the
* official_service_name of the Service as defined in service configurations under
* //configs/cloud/resourcetypes. For example, the official_service_name of cloud resource manager
* service is set as 'cloudresourcemanager.googleapis.com' according to
* //configs/cloud/resourcetypes/google/cloud/resourcemanager/prod.yaml This field is **required**
* for services integrated with resource-attribute-based IAM conditions and/or CustomOrgPolicy.
* This field requires special handling for parents-only permissions such as `create` and `list`.
* See the document linked below for further details. See go/iam-conditions-sig-g3#populate-
* resource-attributes for specific details on populating this field.
* @param service service or {@code null} for none
*/
public Resource setService(java.lang.String service) {
this.service = service;
return this;
}
/**
* The public resource type name of the resource. It is configured using the official_name of the
* ResourceType as defined in service configurations under //configs/cloud/resourcetypes. For
* example, the official_name for GCP projects is set as
* 'cloudresourcemanager.googleapis.com/Project' according to
* //configs/cloud/resourcetypes/google/cloud/resourcemanager/prod.yaml This field is **required**
* for services integrated with resource-attribute-based IAM conditions and/or CustomOrgPolicy.
* This field requires special handling for parents-only permissions such as `create` and `list`.
* See the document linked below for further details. See go/iam-conditions-sig-g3#populate-
* resource-attributes for specific details on populating this field.
* @return value or {@code null} for none
*/
public java.lang.String getType() {
return type;
}
/**
* The public resource type name of the resource. It is configured using the official_name of the
* ResourceType as defined in service configurations under //configs/cloud/resourcetypes. For
* example, the official_name for GCP projects is set as
* 'cloudresourcemanager.googleapis.com/Project' according to
* //configs/cloud/resourcetypes/google/cloud/resourcemanager/prod.yaml This field is **required**
* for services integrated with resource-attribute-based IAM conditions and/or CustomOrgPolicy.
* This field requires special handling for parents-only permissions such as `create` and `list`.
* See the document linked below for further details. See go/iam-conditions-sig-g3#populate-
* resource-attributes for specific details on populating this field.
* @param type type or {@code null} for none
*/
public Resource setType(java.lang.String type) {
this.type = type;
return this;
}
@Override
public Resource set(String fieldName, Object value) {
return (Resource) super.set(fieldName, value);
}
@Override
public Resource clone() {
return (Resource) super.clone();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy